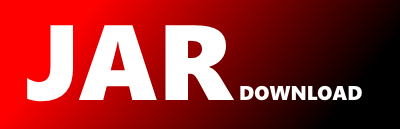
com.oracle.graal.python.nodes.frame.MaterializeFrameNodeGen Maven / Gradle / Ivy
// CheckStyle: start generated
package com.oracle.graal.python.nodes.frame;
import com.oracle.graal.python.builtins.objects.frame.PFrame;
import com.oracle.graal.python.runtime.object.PythonObjectFactory;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.dsl.DSLSupport;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.NeverDefault;
import com.oracle.truffle.api.dsl.UnsupportedSpecializationException;
import com.oracle.truffle.api.dsl.DSLSupport.SpecializationDataNode;
import com.oracle.truffle.api.dsl.InlineSupport.InlineTarget;
import com.oracle.truffle.api.dsl.InlineSupport.ReferenceField;
import com.oracle.truffle.api.dsl.InlineSupport.StateField;
import com.oracle.truffle.api.dsl.InlineSupport.UnsafeAccessedField;
import com.oracle.truffle.api.frame.Frame;
import com.oracle.truffle.api.frame.FrameDescriptor;
import com.oracle.truffle.api.nodes.DenyReplace;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.nodes.NodeCost;
import com.oracle.truffle.api.profiles.InlinedConditionProfile;
import java.lang.invoke.MethodHandles;
import java.lang.invoke.VarHandle;
/**
* Debug Info:
* Specialization {@link MaterializeFrameNode#freshPFrameCachedFD}
* Activation probability: 0.32000
* With/without class size: 9/4 bytes
* Specialization {@link MaterializeFrameNode#freshPFrame}
* Activation probability: 0.26000
* With/without class size: 7/0 bytes
* Specialization {@link MaterializeFrameNode#freshPFrameCusstomLocals}
* Activation probability: 0.20000
* With/without class size: 6/0 bytes
* Specialization {@link MaterializeFrameNode#freshPFrameForGenerator}
* Activation probability: 0.14000
* With/without class size: 5/0 bytes
* Specialization {@link MaterializeFrameNode#alreadyEscapedFrame}
* Activation probability: 0.08000
* With/without class size: 5/1 bytes
*
*/
@GeneratedBy(MaterializeFrameNode.class)
@SuppressWarnings("javadoc")
public final class MaterializeFrameNodeGen extends MaterializeFrameNode {
private static final StateField STATE_0_MaterializeFrameNode_UPDATER = StateField.create(MethodHandles.lookup(), "state_0_");
static final ReferenceField FRESH_P_FRAME_CACHED_F_D_CACHE_UPDATER = ReferenceField.create(MethodHandles.lookup(), "freshPFrameCachedFD_cache", FreshPFrameCachedFDData.class);
/**
* Source Info:
* Specialization: {@link MaterializeFrameNode#alreadyEscapedFrame}
* Parameter: {@link InlinedConditionProfile} syncProfile
* Inline method: {@link InlinedConditionProfile#inline}
*/
private static final InlinedConditionProfile INLINED_ALREADY_ESCAPED_FRAME_SYNC_PROFILE_ = InlinedConditionProfile.inline(InlineTarget.create(InlinedConditionProfile.class, STATE_0_MaterializeFrameNode_UPDATER.subUpdater(5, 2)));
private static final Uncached UNCACHED = new Uncached();
/**
* State Info:
* 0: SpecializationActive {@link MaterializeFrameNode#freshPFrameCachedFD}
* 1: SpecializationActive {@link MaterializeFrameNode#freshPFrame}
* 2: SpecializationActive {@link MaterializeFrameNode#freshPFrameCusstomLocals}
* 3: SpecializationActive {@link MaterializeFrameNode#freshPFrameForGenerator}
* 4: SpecializationActive {@link MaterializeFrameNode#alreadyEscapedFrame}
* 5-6: InlinedCache
* Specialization: {@link MaterializeFrameNode#alreadyEscapedFrame}
* Parameter: {@link InlinedConditionProfile} syncProfile
* Inline method: {@link InlinedConditionProfile#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int state_0_;
/**
* Source Info:
* Specialization: {@link MaterializeFrameNode#freshPFrameCachedFD}
* Parameter: {@link PythonObjectFactory} factory
*/
@Child private PythonObjectFactory factory;
/**
* Source Info:
* Specialization: {@link MaterializeFrameNode#freshPFrameCachedFD}
* Parameter: {@link SyncFrameValuesNode} syncValuesNode
*/
@Child private SyncFrameValuesNode syncValuesNode;
@UnsafeAccessedField @CompilationFinal private FreshPFrameCachedFDData freshPFrameCachedFD_cache;
private MaterializeFrameNodeGen() {
}
@Override
public PFrame execute(Node arg0Value, boolean arg1Value, boolean arg2Value, Frame arg3Value) {
int state_0 = this.state_0_;
if ((state_0 & 0b11111) != 0 /* is SpecializationActive[MaterializeFrameNode.freshPFrameCachedFD(Node, boolean, boolean, Frame, FrameDescriptor, PythonObjectFactory, SyncFrameValuesNode)] || SpecializationActive[MaterializeFrameNode.freshPFrame(Node, boolean, boolean, Frame, PythonObjectFactory, SyncFrameValuesNode)] || SpecializationActive[MaterializeFrameNode.freshPFrameCusstomLocals(Node, boolean, boolean, Frame, PythonObjectFactory)] || SpecializationActive[MaterializeFrameNode.freshPFrameForGenerator(Node, boolean, boolean, Frame, PythonObjectFactory)] || SpecializationActive[MaterializeFrameNode.alreadyEscapedFrame(Node, boolean, boolean, Frame, Node, SyncFrameValuesNode, InlinedConditionProfile)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[MaterializeFrameNode.freshPFrameCachedFD(Node, boolean, boolean, Frame, FrameDescriptor, PythonObjectFactory, SyncFrameValuesNode)] */) {
FreshPFrameCachedFDData s0_ = this.freshPFrameCachedFD_cache;
if (s0_ != null) {
{
PythonObjectFactory factory_ = this.factory;
if (factory_ != null) {
SyncFrameValuesNode syncValuesNode_ = this.syncValuesNode;
if (syncValuesNode_ != null) {
if ((s0_.cachedFD_ == arg3Value.getFrameDescriptor()) && (MaterializeFrameNode.getPFrame(arg3Value) == null) && (!(MaterializeFrameNode.hasGeneratorFrame(arg3Value))) && (!(MaterializeFrameNode.hasCustomLocals(arg3Value)))) {
return MaterializeFrameNode.freshPFrameCachedFD(arg0Value, arg1Value, arg2Value, arg3Value, s0_.cachedFD_, factory_, syncValuesNode_);
}
}
}
}
}
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[MaterializeFrameNode.freshPFrame(Node, boolean, boolean, Frame, PythonObjectFactory, SyncFrameValuesNode)] */) {
{
PythonObjectFactory factory_1 = this.factory;
if (factory_1 != null) {
SyncFrameValuesNode syncValuesNode_1 = this.syncValuesNode;
if (syncValuesNode_1 != null) {
if ((MaterializeFrameNode.getPFrame(arg3Value) == null) && (!(MaterializeFrameNode.hasGeneratorFrame(arg3Value))) && (!(MaterializeFrameNode.hasCustomLocals(arg3Value)))) {
return MaterializeFrameNode.freshPFrame(arg0Value, arg1Value, arg2Value, arg3Value, factory_1, syncValuesNode_1);
}
}
}
}
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[MaterializeFrameNode.freshPFrameCusstomLocals(Node, boolean, boolean, Frame, PythonObjectFactory)] */) {
{
PythonObjectFactory factory_2 = this.factory;
if (factory_2 != null) {
if ((MaterializeFrameNode.getPFrame(arg3Value) == null) && (!(MaterializeFrameNode.hasGeneratorFrame(arg3Value))) && (MaterializeFrameNode.hasCustomLocals(arg3Value))) {
return MaterializeFrameNode.freshPFrameCusstomLocals(arg0Value, arg1Value, arg2Value, arg3Value, factory_2);
}
}
}
}
if ((state_0 & 0b1000) != 0 /* is SpecializationActive[MaterializeFrameNode.freshPFrameForGenerator(Node, boolean, boolean, Frame, PythonObjectFactory)] */) {
{
PythonObjectFactory factory_3 = this.factory;
if (factory_3 != null) {
if ((MaterializeFrameNode.getPFrame(arg3Value) == null) && (MaterializeFrameNode.hasGeneratorFrame(arg3Value))) {
return MaterializeFrameNode.freshPFrameForGenerator(arg0Value, arg1Value, arg2Value, arg3Value, factory_3);
}
}
}
}
if ((state_0 & 0b10000) != 0 /* is SpecializationActive[MaterializeFrameNode.alreadyEscapedFrame(Node, boolean, boolean, Frame, Node, SyncFrameValuesNode, InlinedConditionProfile)] */) {
{
SyncFrameValuesNode syncValuesNode_2 = this.syncValuesNode;
if (syncValuesNode_2 != null) {
if ((MaterializeFrameNode.getPFrame(arg3Value) != null)) {
Node inliningTarget__ = (this);
return MaterializeFrameNode.alreadyEscapedFrame(arg0Value, arg1Value, arg2Value, arg3Value, inliningTarget__, syncValuesNode_2, INLINED_ALREADY_ESCAPED_FRAME_SYNC_PROFILE_);
}
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value, arg1Value, arg2Value, arg3Value);
}
private PFrame executeAndSpecialize(Node arg0Value, boolean arg1Value, boolean arg2Value, Frame arg3Value) {
int state_0 = this.state_0_;
int oldState_0 = (state_0 & 0b11111);
try {
if (((state_0 & 0b10)) == 0 /* is-not SpecializationActive[MaterializeFrameNode.freshPFrame(Node, boolean, boolean, Frame, PythonObjectFactory, SyncFrameValuesNode)] */) {
while (true) {
int count0_ = 0;
FreshPFrameCachedFDData s0_ = FRESH_P_FRAME_CACHED_F_D_CACHE_UPDATER.getVolatile(this);
FreshPFrameCachedFDData s0_original = s0_;
while (s0_ != null) {
{
PythonObjectFactory factory_ = this.factory;
if (factory_ != null) {
SyncFrameValuesNode syncValuesNode_ = this.syncValuesNode;
if (syncValuesNode_ != null) {
if ((s0_.cachedFD_ == arg3Value.getFrameDescriptor()) && (MaterializeFrameNode.getPFrame(arg3Value) == null) && (!(MaterializeFrameNode.hasGeneratorFrame(arg3Value))) && (!(MaterializeFrameNode.hasCustomLocals(arg3Value)))) {
break;
}
}
}
}
count0_++;
s0_ = null;
break;
}
if (s0_ == null && count0_ < 1) {
{
FrameDescriptor cachedFD__ = (arg3Value.getFrameDescriptor());
if ((cachedFD__ == arg3Value.getFrameDescriptor()) && (MaterializeFrameNode.getPFrame(arg3Value) == null) && (!(MaterializeFrameNode.hasGeneratorFrame(arg3Value))) && (!(MaterializeFrameNode.hasCustomLocals(arg3Value)))) {
s0_ = new FreshPFrameCachedFDData();
s0_.cachedFD_ = cachedFD__;
PythonObjectFactory factory_;
PythonObjectFactory factory__shared = this.factory;
if (factory__shared != null) {
factory_ = factory__shared;
} else {
factory_ = this.insert((PythonObjectFactory.create()));
if (factory_ == null) {
throw new IllegalStateException("Specialization 'freshPFrameCachedFD(Node, boolean, boolean, Frame, FrameDescriptor, PythonObjectFactory, SyncFrameValuesNode)' contains a shared cache with name 'factory' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.factory == null) {
this.factory = factory_;
}
SyncFrameValuesNode syncValuesNode_;
SyncFrameValuesNode syncValuesNode__shared = this.syncValuesNode;
if (syncValuesNode__shared != null) {
syncValuesNode_ = syncValuesNode__shared;
} else {
syncValuesNode_ = this.insert((SyncFrameValuesNodeGen.create()));
if (syncValuesNode_ == null) {
throw new IllegalStateException("Specialization 'freshPFrameCachedFD(Node, boolean, boolean, Frame, FrameDescriptor, PythonObjectFactory, SyncFrameValuesNode)' contains a shared cache with name 'syncValuesNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.syncValuesNode == null) {
this.syncValuesNode = syncValuesNode_;
}
if (!FRESH_P_FRAME_CACHED_F_D_CACHE_UPDATER.compareAndSet(this, s0_original, s0_)) {
continue;
}
state_0 = state_0 | 0b1 /* add SpecializationActive[MaterializeFrameNode.freshPFrameCachedFD(Node, boolean, boolean, Frame, FrameDescriptor, PythonObjectFactory, SyncFrameValuesNode)] */;
this.state_0_ = state_0;
}
}
}
if (s0_ != null) {
return MaterializeFrameNode.freshPFrameCachedFD(arg0Value, arg1Value, arg2Value, arg3Value, s0_.cachedFD_, this.factory, this.syncValuesNode);
}
break;
}
}
if ((MaterializeFrameNode.getPFrame(arg3Value) == null) && (!(MaterializeFrameNode.hasGeneratorFrame(arg3Value))) && (!(MaterializeFrameNode.hasCustomLocals(arg3Value)))) {
PythonObjectFactory factory_1;
PythonObjectFactory factory_1_shared = this.factory;
if (factory_1_shared != null) {
factory_1 = factory_1_shared;
} else {
factory_1 = this.insert((PythonObjectFactory.create()));
if (factory_1 == null) {
throw new IllegalStateException("Specialization 'freshPFrame(Node, boolean, boolean, Frame, PythonObjectFactory, SyncFrameValuesNode)' contains a shared cache with name 'factory' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.factory == null) {
VarHandle.storeStoreFence();
this.factory = factory_1;
}
SyncFrameValuesNode syncValuesNode_1;
SyncFrameValuesNode syncValuesNode_1_shared = this.syncValuesNode;
if (syncValuesNode_1_shared != null) {
syncValuesNode_1 = syncValuesNode_1_shared;
} else {
syncValuesNode_1 = this.insert((SyncFrameValuesNodeGen.create()));
if (syncValuesNode_1 == null) {
throw new IllegalStateException("Specialization 'freshPFrame(Node, boolean, boolean, Frame, PythonObjectFactory, SyncFrameValuesNode)' contains a shared cache with name 'syncValuesNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.syncValuesNode == null) {
VarHandle.storeStoreFence();
this.syncValuesNode = syncValuesNode_1;
}
this.freshPFrameCachedFD_cache = null;
state_0 = state_0 & 0xfffffffe /* remove SpecializationActive[MaterializeFrameNode.freshPFrameCachedFD(Node, boolean, boolean, Frame, FrameDescriptor, PythonObjectFactory, SyncFrameValuesNode)] */;
state_0 = state_0 | 0b10 /* add SpecializationActive[MaterializeFrameNode.freshPFrame(Node, boolean, boolean, Frame, PythonObjectFactory, SyncFrameValuesNode)] */;
this.state_0_ = state_0;
return MaterializeFrameNode.freshPFrame(arg0Value, arg1Value, arg2Value, arg3Value, factory_1, syncValuesNode_1);
}
if ((MaterializeFrameNode.getPFrame(arg3Value) == null) && (!(MaterializeFrameNode.hasGeneratorFrame(arg3Value))) && (MaterializeFrameNode.hasCustomLocals(arg3Value))) {
PythonObjectFactory factory_2;
PythonObjectFactory factory_2_shared = this.factory;
if (factory_2_shared != null) {
factory_2 = factory_2_shared;
} else {
factory_2 = this.insert((PythonObjectFactory.create()));
if (factory_2 == null) {
throw new IllegalStateException("Specialization 'freshPFrameCusstomLocals(Node, boolean, boolean, Frame, PythonObjectFactory)' contains a shared cache with name 'factory' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.factory == null) {
VarHandle.storeStoreFence();
this.factory = factory_2;
}
state_0 = state_0 | 0b100 /* add SpecializationActive[MaterializeFrameNode.freshPFrameCusstomLocals(Node, boolean, boolean, Frame, PythonObjectFactory)] */;
this.state_0_ = state_0;
return MaterializeFrameNode.freshPFrameCusstomLocals(arg0Value, arg1Value, arg2Value, arg3Value, factory_2);
}
if ((MaterializeFrameNode.getPFrame(arg3Value) == null) && (MaterializeFrameNode.hasGeneratorFrame(arg3Value))) {
PythonObjectFactory factory_3;
PythonObjectFactory factory_3_shared = this.factory;
if (factory_3_shared != null) {
factory_3 = factory_3_shared;
} else {
factory_3 = this.insert((PythonObjectFactory.create()));
if (factory_3 == null) {
throw new IllegalStateException("Specialization 'freshPFrameForGenerator(Node, boolean, boolean, Frame, PythonObjectFactory)' contains a shared cache with name 'factory' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.factory == null) {
VarHandle.storeStoreFence();
this.factory = factory_3;
}
state_0 = state_0 | 0b1000 /* add SpecializationActive[MaterializeFrameNode.freshPFrameForGenerator(Node, boolean, boolean, Frame, PythonObjectFactory)] */;
this.state_0_ = state_0;
return MaterializeFrameNode.freshPFrameForGenerator(arg0Value, arg1Value, arg2Value, arg3Value, factory_3);
}
{
Node inliningTarget__ = null;
if ((MaterializeFrameNode.getPFrame(arg3Value) != null)) {
inliningTarget__ = (this);
SyncFrameValuesNode syncValuesNode_2;
SyncFrameValuesNode syncValuesNode_2_shared = this.syncValuesNode;
if (syncValuesNode_2_shared != null) {
syncValuesNode_2 = syncValuesNode_2_shared;
} else {
syncValuesNode_2 = this.insert((SyncFrameValuesNodeGen.create()));
if (syncValuesNode_2 == null) {
throw new IllegalStateException("Specialization 'alreadyEscapedFrame(Node, boolean, boolean, Frame, Node, SyncFrameValuesNode, InlinedConditionProfile)' contains a shared cache with name 'syncValuesNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.syncValuesNode == null) {
VarHandle.storeStoreFence();
this.syncValuesNode = syncValuesNode_2;
}
state_0 = state_0 | 0b10000 /* add SpecializationActive[MaterializeFrameNode.alreadyEscapedFrame(Node, boolean, boolean, Frame, Node, SyncFrameValuesNode, InlinedConditionProfile)] */;
this.state_0_ = state_0;
return MaterializeFrameNode.alreadyEscapedFrame(arg0Value, arg1Value, arg2Value, arg3Value, inliningTarget__, syncValuesNode_2, INLINED_ALREADY_ESCAPED_FRAME_SYNC_PROFILE_);
}
}
throw new UnsupportedSpecializationException(this, new Node[] {null, null, null, null}, arg0Value, arg1Value, arg2Value, arg3Value);
} finally {
if (oldState_0 != 0) {
checkForPolymorphicSpecialize(oldState_0);
}
}
}
private void checkForPolymorphicSpecialize(int oldState_0) {
int state_0 = this.state_0_;
int newState_0 = (state_0 & 0b11111);
if (((oldState_0 ^ newState_0) != 0)) {
this.reportPolymorphicSpecialize();
}
}
@Override
public NodeCost getCost() {
int state_0 = this.state_0_;
if ((state_0 & 0b11111) == 0) {
return NodeCost.UNINITIALIZED;
} else {
if (((state_0 & 0b11111) & ((state_0 & 0b11111) - 1)) == 0 /* is-single */) {
return NodeCost.MONOMORPHIC;
}
}
return NodeCost.POLYMORPHIC;
}
@NeverDefault
public static MaterializeFrameNode create() {
return new MaterializeFrameNodeGen();
}
@NeverDefault
public static MaterializeFrameNode getUncached() {
return MaterializeFrameNodeGen.UNCACHED;
}
@GeneratedBy(MaterializeFrameNode.class)
@DenyReplace
private static final class FreshPFrameCachedFDData implements SpecializationDataNode {
/**
* Source Info:
* Specialization: {@link MaterializeFrameNode#freshPFrameCachedFD}
* Parameter: {@link FrameDescriptor} cachedFD
*/
@CompilationFinal FrameDescriptor cachedFD_;
FreshPFrameCachedFDData() {
}
}
@GeneratedBy(MaterializeFrameNode.class)
@DenyReplace
private static final class Uncached extends MaterializeFrameNode {
@Override
public PFrame execute(Node arg0Value, boolean arg1Value, boolean arg2Value, Frame arg3Value) {
CompilerDirectives.transferToInterpreterAndInvalidate();
if ((MaterializeFrameNode.getPFrame(arg3Value) == null) && (!(MaterializeFrameNode.hasGeneratorFrame(arg3Value))) && (!(MaterializeFrameNode.hasCustomLocals(arg3Value)))) {
return MaterializeFrameNode.freshPFrame(arg0Value, arg1Value, arg2Value, arg3Value, (PythonObjectFactory.getUncached()), (SyncFrameValuesNodeGen.getUncached()));
}
if ((MaterializeFrameNode.getPFrame(arg3Value) == null) && (!(MaterializeFrameNode.hasGeneratorFrame(arg3Value))) && (MaterializeFrameNode.hasCustomLocals(arg3Value))) {
return MaterializeFrameNode.freshPFrameCusstomLocals(arg0Value, arg1Value, arg2Value, arg3Value, (PythonObjectFactory.getUncached()));
}
if ((MaterializeFrameNode.getPFrame(arg3Value) == null) && (MaterializeFrameNode.hasGeneratorFrame(arg3Value))) {
return MaterializeFrameNode.freshPFrameForGenerator(arg0Value, arg1Value, arg2Value, arg3Value, (PythonObjectFactory.getUncached()));
}
if ((MaterializeFrameNode.getPFrame(arg3Value) != null)) {
return MaterializeFrameNode.alreadyEscapedFrame(arg0Value, arg1Value, arg2Value, arg3Value, (this), (SyncFrameValuesNodeGen.getUncached()), (InlinedConditionProfile.getUncached()));
}
throw new UnsupportedSpecializationException(this, new Node[] {null, null, null, null}, arg0Value, arg1Value, arg2Value, arg3Value);
}
@Override
public NodeCost getCost() {
return NodeCost.MEGAMORPHIC;
}
@Override
public boolean isAdoptable() {
return false;
}
}
/**
* Debug Info:
* Specialization {@link SyncFrameValuesNode#doSyncExploded}
* Activation probability: 0.48333
* With/without class size: 11/4 bytes
* Specialization {@link SyncFrameValuesNode#doSyncLoop}
* Activation probability: 0.33333
* With/without class size: 8/0 bytes
* Specialization {@link SyncFrameValuesNode#doCustomLocals}
* Activation probability: 0.18333
* With/without class size: 6/0 bytes
*
*/
@GeneratedBy(SyncFrameValuesNode.class)
@SuppressWarnings("javadoc")
public static final class SyncFrameValuesNodeGen extends SyncFrameValuesNode {
static final ReferenceField SYNC_EXPLODED_CACHE_UPDATER = ReferenceField.create(MethodHandles.lookup(), "syncExploded_cache", SyncExplodedData.class);
private static final Uncached UNCACHED = new Uncached();
/**
* State Info:
* 0: SpecializationActive {@link SyncFrameValuesNode#doSyncExploded}
* 1: SpecializationActive {@link SyncFrameValuesNode#doSyncLoop}
* 2: SpecializationActive {@link SyncFrameValuesNode#doCustomLocals}
*
*/
@CompilationFinal private int state_0_;
@UnsafeAccessedField @CompilationFinal private SyncExplodedData syncExploded_cache;
private SyncFrameValuesNodeGen() {
}
@Override
public void execute(PFrame arg0Value, Frame arg1Value) {
int state_0 = this.state_0_;
if (state_0 != 0 /* is SpecializationActive[MaterializeFrameNode.SyncFrameValuesNode.doSyncExploded(PFrame, Frame, FrameDescriptor)] || SpecializationActive[MaterializeFrameNode.SyncFrameValuesNode.doSyncLoop(PFrame, Frame)] || SpecializationActive[MaterializeFrameNode.SyncFrameValuesNode.doCustomLocals(PFrame, Frame)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[MaterializeFrameNode.SyncFrameValuesNode.doSyncExploded(PFrame, Frame, FrameDescriptor)] */) {
SyncExplodedData s0_ = this.syncExploded_cache;
if (s0_ != null) {
if ((!(arg0Value.hasCustomLocals())) && (arg1Value.getFrameDescriptor() == s0_.cachedFd_)) {
assert DSLSupport.assertIdempotence((SyncFrameValuesNode.variableSlotCount(s0_.cachedFd_) < 32));
SyncFrameValuesNode.doSyncExploded(arg0Value, arg1Value, s0_.cachedFd_);
return;
}
}
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[MaterializeFrameNode.SyncFrameValuesNode.doSyncLoop(PFrame, Frame)] */) {
if ((!(arg0Value.hasCustomLocals()))) {
SyncFrameValuesNode.doSyncLoop(arg0Value, arg1Value);
return;
}
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[MaterializeFrameNode.SyncFrameValuesNode.doCustomLocals(PFrame, Frame)] */) {
if ((arg0Value.hasCustomLocals())) {
SyncFrameValuesNode.doCustomLocals(arg0Value, arg1Value);
return;
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
executeAndSpecialize(arg0Value, arg1Value);
return;
}
private void executeAndSpecialize(PFrame arg0Value, Frame arg1Value) {
int state_0 = this.state_0_;
if (((state_0 & 0b10)) == 0 /* is-not SpecializationActive[MaterializeFrameNode.SyncFrameValuesNode.doSyncLoop(PFrame, Frame)] */) {
while (true) {
int count0_ = 0;
SyncExplodedData s0_ = SYNC_EXPLODED_CACHE_UPDATER.getVolatile(this);
SyncExplodedData s0_original = s0_;
while (s0_ != null) {
if ((!(arg0Value.hasCustomLocals())) && (arg1Value.getFrameDescriptor() == s0_.cachedFd_)) {
assert DSLSupport.assertIdempotence((SyncFrameValuesNode.variableSlotCount(s0_.cachedFd_) < 32));
break;
}
count0_++;
s0_ = null;
break;
}
if (s0_ == null && count0_ < 1) {
if ((!(arg0Value.hasCustomLocals()))) {
FrameDescriptor cachedFd__ = (arg1Value.getFrameDescriptor());
if ((arg1Value.getFrameDescriptor() == cachedFd__) && (SyncFrameValuesNode.variableSlotCount(cachedFd__) < 32)) {
s0_ = new SyncExplodedData();
s0_.cachedFd_ = cachedFd__;
if (!SYNC_EXPLODED_CACHE_UPDATER.compareAndSet(this, s0_original, s0_)) {
continue;
}
state_0 = state_0 | 0b1 /* add SpecializationActive[MaterializeFrameNode.SyncFrameValuesNode.doSyncExploded(PFrame, Frame, FrameDescriptor)] */;
this.state_0_ = state_0;
}
}
}
if (s0_ != null) {
SyncFrameValuesNode.doSyncExploded(arg0Value, arg1Value, s0_.cachedFd_);
return;
}
break;
}
}
if ((!(arg0Value.hasCustomLocals()))) {
this.syncExploded_cache = null;
state_0 = state_0 & 0xfffffffe /* remove SpecializationActive[MaterializeFrameNode.SyncFrameValuesNode.doSyncExploded(PFrame, Frame, FrameDescriptor)] */;
state_0 = state_0 | 0b10 /* add SpecializationActive[MaterializeFrameNode.SyncFrameValuesNode.doSyncLoop(PFrame, Frame)] */;
this.state_0_ = state_0;
SyncFrameValuesNode.doSyncLoop(arg0Value, arg1Value);
return;
}
if ((arg0Value.hasCustomLocals())) {
state_0 = state_0 | 0b100 /* add SpecializationActive[MaterializeFrameNode.SyncFrameValuesNode.doCustomLocals(PFrame, Frame)] */;
this.state_0_ = state_0;
SyncFrameValuesNode.doCustomLocals(arg0Value, arg1Value);
return;
}
throw new UnsupportedSpecializationException(this, new Node[] {null, null}, arg0Value, arg1Value);
}
@Override
public NodeCost getCost() {
int state_0 = this.state_0_;
if (state_0 == 0) {
return NodeCost.UNINITIALIZED;
} else {
if ((state_0 & (state_0 - 1)) == 0 /* is-single */) {
return NodeCost.MONOMORPHIC;
}
}
return NodeCost.POLYMORPHIC;
}
@NeverDefault
public static SyncFrameValuesNode create() {
return new SyncFrameValuesNodeGen();
}
@NeverDefault
public static SyncFrameValuesNode getUncached() {
return SyncFrameValuesNodeGen.UNCACHED;
}
@GeneratedBy(SyncFrameValuesNode.class)
@DenyReplace
private static final class SyncExplodedData implements SpecializationDataNode {
/**
* Source Info:
* Specialization: {@link SyncFrameValuesNode#doSyncExploded}
* Parameter: {@link FrameDescriptor} cachedFd
*/
@CompilationFinal FrameDescriptor cachedFd_;
SyncExplodedData() {
}
}
@GeneratedBy(SyncFrameValuesNode.class)
@DenyReplace
private static final class Uncached extends SyncFrameValuesNode {
@Override
public void execute(PFrame arg0Value, Frame arg1Value) {
CompilerDirectives.transferToInterpreterAndInvalidate();
if ((!(arg0Value.hasCustomLocals()))) {
SyncFrameValuesNode.doSyncLoop(arg0Value, arg1Value);
return;
}
if ((arg0Value.hasCustomLocals())) {
SyncFrameValuesNode.doCustomLocals(arg0Value, arg1Value);
return;
}
throw new UnsupportedSpecializationException(this, new Node[] {null, null}, arg0Value, arg1Value);
}
@Override
public NodeCost getCost() {
return NodeCost.MEGAMORPHIC;
}
@Override
public boolean isAdoptable() {
return false;
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy