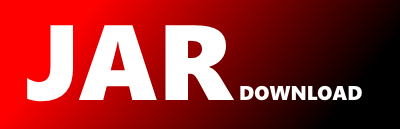
com.oracle.graal.python.runtime.EmulatedPosixSupportGen Maven / Gradle / Ivy
// CheckStyle: start generated
package com.oracle.graal.python.runtime;
import com.oracle.graal.python.nodes.attributes.ReadAttributeFromObjectNode;
import com.oracle.graal.python.nodes.object.IsNode;
import com.oracle.graal.python.runtime.EmulatedPosixSupport.CreateUniversalSockAddr;
import com.oracle.graal.python.runtime.EmulatedPosixSupport.Getpid;
import com.oracle.graal.python.runtime.EmulatedPosixSupport.MMapHandle;
import com.oracle.graal.python.runtime.EmulatedPosixSupport.SetUTimeNode;
import com.oracle.graal.python.runtime.EmulatedPosixSupportFactory.SetUTimeNodeGen;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.CompilerDirectives.TruffleBoundary;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.UnsupportedSpecializationException;
import com.oracle.truffle.api.dsl.DSLSupport.SpecializationDataNode;
import com.oracle.truffle.api.dsl.InlineSupport.InlineTarget;
import com.oracle.truffle.api.dsl.InlineSupport.ReferenceField;
import com.oracle.truffle.api.dsl.InlineSupport.StateField;
import com.oracle.truffle.api.dsl.InlineSupport.UnsafeAccessedField;
import com.oracle.truffle.api.library.DynamicDispatchLibrary;
import com.oracle.truffle.api.library.LibraryExport;
import com.oracle.truffle.api.library.LibraryFactory;
import com.oracle.truffle.api.nodes.DenyReplace;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.nodes.NodeCost;
import com.oracle.truffle.api.profiles.InlinedBranchProfile;
import com.oracle.truffle.api.profiles.InlinedConditionProfile;
import com.oracle.truffle.api.strings.TruffleString;
import com.oracle.truffle.api.strings.TruffleString.EqualNode;
import com.oracle.truffle.api.strings.TruffleString.FromJavaStringNode;
import com.oracle.truffle.api.strings.TruffleString.ToJavaStringNode;
import java.lang.invoke.MethodHandles;
import java.lang.invoke.VarHandle;
import java.util.Objects;
@GeneratedBy(EmulatedPosixSupport.class)
@SuppressWarnings("javadoc")
final class EmulatedPosixSupportGen {
private static final LibraryFactory DYNAMIC_DISPATCH_LIBRARY_ = LibraryFactory.resolve(DynamicDispatchLibrary.class);
static {
LibraryExport.register(EmulatedPosixSupport.class, new PosixSupportLibraryExports());
}
private EmulatedPosixSupportGen() {
}
@GeneratedBy(EmulatedPosixSupport.class)
private static final class PosixSupportLibraryExports extends LibraryExport {
private PosixSupportLibraryExports() {
super(PosixSupportLibrary.class, EmulatedPosixSupport.class, false, false, 0);
}
@Override
protected PosixSupportLibrary createUncached(Object receiver) {
assert receiver instanceof EmulatedPosixSupport;
PosixSupportLibrary uncached = new Uncached();
return uncached;
}
@Override
protected PosixSupportLibrary createCached(Object receiver) {
assert receiver instanceof EmulatedPosixSupport;
return new Cached();
}
@GeneratedBy(EmulatedPosixSupport.class)
private static final class Cached extends PosixSupportLibrary {
private static final StateField STATE_1_UPDATER = StateField.create(MethodHandles.lookup(), "state_1_");
private static final StateField STATE_2_UPDATER = StateField.create(MethodHandles.lookup(), "state_2_");
private static final StateField STATE_1_LseekNode__UPDATER = StateField.create(MethodHandles.lookup(), "state_1_");
private static final StateField STATE_1_FstatatNode__UPDATER = StateField.create(MethodHandles.lookup(), "state_1_");
private static final StateField STATE_2_FstatatNode__UPDATER = StateField.create(MethodHandles.lookup(), "state_2_");
private static final StateField STATE_1_FstatNode__UPDATER = StateField.create(MethodHandles.lookup(), "state_1_");
private static final StateField STATE_2_FstatNode__UPDATER = StateField.create(MethodHandles.lookup(), "state_2_");
/**
* Source Info:
* Specialization: {@link EmulatedPosixSupport#strerror}
* Parameter: {@link InlinedBranchProfile} errorBranch
* Inline method: {@link InlinedBranchProfile#inline}
*/
private static final InlinedBranchProfile INLINED_ERROR_BRANCH = InlinedBranchProfile.inline(InlineTarget.create(InlinedBranchProfile.class, STATE_1_UPDATER.subUpdater(20, 1)));
/**
* Source Info:
* Specialization: {@link EmulatedPosixSupport#openat}
* Parameter: {@link InlinedConditionProfile} defaultDirFdPofile
* Inline method: {@link InlinedConditionProfile#inline}
*/
private static final InlinedConditionProfile INLINED_DEFAULT_DIR_PROFILE = InlinedConditionProfile.inline(InlineTarget.create(InlinedConditionProfile.class, STATE_1_UPDATER.subUpdater(21, 2)));
/**
* Source Info:
* Specialization: {@link EmulatedPosixSupport#utimensat}
* Parameter: {@link SetUTimeNode} setUTimeNode
* Inline method: {@link SetUTimeNodeGen#inline}
*/
private static final SetUTimeNode INLINED_SET_U_TIME = SetUTimeNodeGen.inline(InlineTarget.create(SetUTimeNode.class, STATE_2_UPDATER.subUpdater(5, 3), ReferenceField.create(MethodHandles.lookup(), "setUTime_field1_", Node.class)));
/**
* Source Info:
* Specialization: {@link EmulatedPosixSupport#lseek(EmulatedPosixSupport, int, long, int, Node, InlinedBranchProfile, InlinedConditionProfile, InlinedConditionProfile, InlinedConditionProfile, EqualNode)}
* Parameter: {@link InlinedBranchProfile} errorBranch
* Inline method: {@link InlinedBranchProfile#inline}
*/
private static final InlinedBranchProfile INLINED_LSEEK_NODE__LSEEK_ERROR_BRANCH_ = InlinedBranchProfile.inline(InlineTarget.create(InlinedBranchProfile.class, STATE_1_LseekNode__UPDATER.subUpdater(23, 1)));
/**
* Source Info:
* Specialization: {@link EmulatedPosixSupport#lseek(EmulatedPosixSupport, int, long, int, Node, InlinedBranchProfile, InlinedConditionProfile, InlinedConditionProfile, InlinedConditionProfile, EqualNode)}
* Parameter: {@link InlinedConditionProfile} notSupported
* Inline method: {@link InlinedConditionProfile#inline}
*/
private static final InlinedConditionProfile INLINED_LSEEK_NODE__LSEEK_NOT_SUPPORTED_ = InlinedConditionProfile.inline(InlineTarget.create(InlinedConditionProfile.class, STATE_1_LseekNode__UPDATER.subUpdater(24, 2)));
/**
* Source Info:
* Specialization: {@link EmulatedPosixSupport#lseek(EmulatedPosixSupport, int, long, int, Node, InlinedBranchProfile, InlinedConditionProfile, InlinedConditionProfile, InlinedConditionProfile, EqualNode)}
* Parameter: {@link InlinedConditionProfile} noFile
* Inline method: {@link InlinedConditionProfile#inline}
*/
private static final InlinedConditionProfile INLINED_LSEEK_NODE__LSEEK_NO_FILE_ = InlinedConditionProfile.inline(InlineTarget.create(InlinedConditionProfile.class, STATE_1_LseekNode__UPDATER.subUpdater(26, 2)));
/**
* Source Info:
* Specialization: {@link EmulatedPosixSupport#lseek(EmulatedPosixSupport, int, long, int, Node, InlinedBranchProfile, InlinedConditionProfile, InlinedConditionProfile, InlinedConditionProfile, EqualNode)}
* Parameter: {@link InlinedConditionProfile} notSeekable
* Inline method: {@link InlinedConditionProfile#inline}
*/
private static final InlinedConditionProfile INLINED_LSEEK_NODE__LSEEK_NOT_SEEKABLE_ = InlinedConditionProfile.inline(InlineTarget.create(InlinedConditionProfile.class, STATE_1_LseekNode__UPDATER.subUpdater(28, 2)));
/**
* Source Info:
* Specialization: {@link EmulatedPosixSupport#fstatat(EmulatedPosixSupport, int, Object, boolean, Node, InlinedBranchProfile, InlinedConditionProfile, EqualNode, FromJavaStringNode, ToJavaStringNode)}
* Parameter: {@link InlinedBranchProfile} errorBranch
* Inline method: {@link InlinedBranchProfile#inline}
*/
private static final InlinedBranchProfile INLINED_FSTATAT_NODE__FSTATAT_ERROR_BRANCH_ = InlinedBranchProfile.inline(InlineTarget.create(InlinedBranchProfile.class, STATE_1_FstatatNode__UPDATER.subUpdater(30, 1)));
/**
* Source Info:
* Specialization: {@link EmulatedPosixSupport#fstatat(EmulatedPosixSupport, int, Object, boolean, Node, InlinedBranchProfile, InlinedConditionProfile, EqualNode, FromJavaStringNode, ToJavaStringNode)}
* Parameter: {@link InlinedConditionProfile} defaultDirFdPofile
* Inline method: {@link InlinedConditionProfile#inline}
*/
private static final InlinedConditionProfile INLINED_FSTATAT_NODE__FSTATAT_DEFAULT_DIR_FD_POFILE_ = InlinedConditionProfile.inline(InlineTarget.create(InlinedConditionProfile.class, STATE_2_FstatatNode__UPDATER.subUpdater(0, 2)));
/**
* Source Info:
* Specialization: {@link EmulatedPosixSupport#fstat(EmulatedPosixSupport, int, Node, InlinedBranchProfile, InlinedBranchProfile, InlinedConditionProfile, EqualNode, FromJavaStringNode)}
* Parameter: {@link InlinedBranchProfile} nullPathProfile
* Inline method: {@link InlinedBranchProfile#inline}
*/
private static final InlinedBranchProfile INLINED_FSTAT_NODE__FSTAT_NULL_PATH_PROFILE_ = InlinedBranchProfile.inline(InlineTarget.create(InlinedBranchProfile.class, STATE_1_FstatNode__UPDATER.subUpdater(31, 1)));
/**
* Source Info:
* Specialization: {@link EmulatedPosixSupport#fstat(EmulatedPosixSupport, int, Node, InlinedBranchProfile, InlinedBranchProfile, InlinedConditionProfile, EqualNode, FromJavaStringNode)}
* Parameter: {@link InlinedBranchProfile} errorBranch
* Inline method: {@link InlinedBranchProfile#inline}
*/
private static final InlinedBranchProfile INLINED_FSTAT_NODE__FSTAT_ERROR_BRANCH_ = InlinedBranchProfile.inline(InlineTarget.create(InlinedBranchProfile.class, STATE_2_FstatNode__UPDATER.subUpdater(2, 1)));
/**
* Source Info:
* Specialization: {@link EmulatedPosixSupport#fstat(EmulatedPosixSupport, int, Node, InlinedBranchProfile, InlinedBranchProfile, InlinedConditionProfile, EqualNode, FromJavaStringNode)}
* Parameter: {@link InlinedConditionProfile} defaultDirFdPofile
* Inline method: {@link InlinedConditionProfile#inline}
*/
private static final InlinedConditionProfile INLINED_FSTAT_NODE__FSTAT_DEFAULT_DIR_FD_POFILE_ = InlinedConditionProfile.inline(InlineTarget.create(InlinedConditionProfile.class, STATE_2_FstatNode__UPDATER.subUpdater(3, 2)));
/**
* State Info:
* 0: SpecializationActive {@link Getpid#getPid}
* 1: SpecializationExcluded {@link Getpid#getPid}
* 2: SpecializationActive {@link Getpid#getPidFallback}
* 3: SpecializationActive {@link CreateUniversalSockAddr#inet4}
* 4: SpecializationActive {@link CreateUniversalSockAddr#inet6}
* 5: SpecializationActive {@link CreateUniversalSockAddr#unix}
* 6: SpecializationActive {@link EmulatedPosixSupport#openat(EmulatedPosixSupport, int, Object, int, int, Node, InlinedBranchProfile, InlinedConditionProfile, EqualNode, FromJavaStringNode, ToJavaStringNode)}
* 7: SpecializationActive {@link EmulatedPosixSupport#write(EmulatedPosixSupport, int, Buffer, Node, InlinedBranchProfile, EqualNode)}
* 8: SpecializationActive {@link EmulatedPosixSupport#read(EmulatedPosixSupport, int, long, Node, InlinedBranchProfile, EqualNode)}
* 9: SpecializationActive {@link EmulatedPosixSupport#dup2(EmulatedPosixSupport, int, int, boolean, EqualNode)}
* 10: SpecializationActive {@link EmulatedPosixSupport#pipeMessage(EmulatedPosixSupport, EqualNode)}
* 11: SpecializationActive {@link EmulatedPosixSupport#lseek(EmulatedPosixSupport, int, long, int, Node, InlinedBranchProfile, InlinedConditionProfile, InlinedConditionProfile, InlinedConditionProfile, EqualNode)}
* 12: SpecializationActive {@link EmulatedPosixSupport#ftruncateMessage(EmulatedPosixSupport, int, long, Node, InlinedBranchProfile, EqualNode)}
* 13: SpecializationActive {@link EmulatedPosixSupport#flock(EmulatedPosixSupport, int, int, Node, InlinedBranchProfile, EqualNode)}
* 14: SpecializationActive {@link EmulatedPosixSupport#setBlocking(EmulatedPosixSupport, int, boolean, EqualNode)}
* 15: SpecializationActive {@link EmulatedPosixSupport#fstatat(EmulatedPosixSupport, int, Object, boolean, Node, InlinedBranchProfile, InlinedConditionProfile, EqualNode, FromJavaStringNode, ToJavaStringNode)}
* 16: SpecializationActive {@link EmulatedPosixSupport#fstat(EmulatedPosixSupport, int, Node, InlinedBranchProfile, InlinedBranchProfile, InlinedConditionProfile, EqualNode, FromJavaStringNode)}
* 17: SpecializationActive {@link EmulatedPosixSupport#uname(EmulatedPosixSupport, FromJavaStringNode)}
* 18: SpecializationActive {@link EmulatedPosixSupport#unlinkat(EmulatedPosixSupport, int, Object, boolean, Node, InlinedBranchProfile, InlinedConditionProfile, EqualNode, FromJavaStringNode, ToJavaStringNode)}
* 19: SpecializationActive {@link EmulatedPosixSupport#linkat(EmulatedPosixSupport, int, Object, int, Object, int, Node, InlinedBranchProfile, InlinedConditionProfile, EqualNode, FromJavaStringNode, ToJavaStringNode)}
* 20: SpecializationActive {@link EmulatedPosixSupport#symlinkat(EmulatedPosixSupport, Object, int, Object, Node, InlinedBranchProfile, InlinedConditionProfile, EqualNode, FromJavaStringNode, ToJavaStringNode)}
* 21: SpecializationActive {@link EmulatedPosixSupport#mkdirat(EmulatedPosixSupport, int, Object, int, Node, InlinedBranchProfile, InlinedConditionProfile, EqualNode, FromJavaStringNode, ToJavaStringNode)}
* 22: SpecializationActive {@link EmulatedPosixSupport#chdir(EmulatedPosixSupport, Object, Node, InlinedBranchProfile, EqualNode, FromJavaStringNode)}
* 23: SpecializationActive {@link EmulatedPosixSupport#fchdir(EmulatedPosixSupport, int, Node, InlinedBranchProfile, EqualNode, FromJavaStringNode)}
* 24: SpecializationActive {@link EmulatedPosixSupport#opendir(EmulatedPosixSupport, Object, EqualNode, FromJavaStringNode)}
* 25: SpecializationActive {@link EmulatedPosixSupport#fdopendir(EmulatedPosixSupport, int, Node, InlinedBranchProfile, EqualNode, FromJavaStringNode)}
* 26: SpecializationActive {@link EmulatedPosixSupport#closedir(EmulatedPosixSupport, Object, EqualNode)}
* 27: SpecializationActive {@link EmulatedPosixSupport#readdir(EmulatedPosixSupport, Object, EqualNode)}
* 28: SpecializationActive {@link EmulatedPosixSupport#dirEntryGetPath(EmulatedPosixSupport, Object, Object, FromJavaStringNode)}
* 29: SpecializationActive {@link EmulatedPosixSupport#dirEntryGetInode(EmulatedPosixSupport, Object, EqualNode)}
* 30: SpecializationActive {@link EmulatedPosixSupport#utimensat(EmulatedPosixSupport, int, Object, long[], boolean, Node, SetUTimeNode, InlinedConditionProfile, EqualNode, FromJavaStringNode, ToJavaStringNode)}
* 31: SpecializationActive {@link EmulatedPosixSupport#futimens(EmulatedPosixSupport, int, long[], Node, SetUTimeNode, EqualNode, FromJavaStringNode)}
*
*/
@CompilationFinal private int state_0_;
/**
* State Info:
* 0: SpecializationActive {@link EmulatedPosixSupport#futimes(EmulatedPosixSupport, int, Timeval[], Node, SetUTimeNode, EqualNode, FromJavaStringNode)}
* 1: SpecializationActive {@link EmulatedPosixSupport#lutimes(EmulatedPosixSupport, Object, Timeval[], Node, SetUTimeNode, EqualNode, FromJavaStringNode)}
* 2: SpecializationActive {@link EmulatedPosixSupport#utimes(EmulatedPosixSupport, Object, Timeval[], Node, SetUTimeNode, EqualNode, FromJavaStringNode)}
* 3: SpecializationActive {@link EmulatedPosixSupport#renameat(EmulatedPosixSupport, int, Object, int, Object, Node, InlinedConditionProfile, EqualNode, FromJavaStringNode, ToJavaStringNode)}
* 4: SpecializationActive {@link EmulatedPosixSupport#faccessat(EmulatedPosixSupport, int, Object, int, boolean, boolean, Node, InlinedBranchProfile, InlinedConditionProfile, EqualNode, FromJavaStringNode, ToJavaStringNode)}
* 5: SpecializationActive {@link EmulatedPosixSupport#fchmodat(EmulatedPosixSupport, int, Object, int, boolean, Node, InlinedConditionProfile, EqualNode, FromJavaStringNode, ToJavaStringNode)}
* 6: SpecializationActive {@link EmulatedPosixSupport#fchmod(EmulatedPosixSupport, int, int, EqualNode, FromJavaStringNode)}
* 7: SpecializationActive {@link EmulatedPosixSupport#readlinkat(EmulatedPosixSupport, int, Object, Node, InlinedConditionProfile, EqualNode, FromJavaStringNode, ToJavaStringNode)}
* 8: SpecializationActive {@link EmulatedPosixSupport#kill(EmulatedPosixSupport, long, int, ReadAttributeFromObjectNode, IsNode)}
* 9: SpecializationActive {@link EmulatedPosixSupport#forkExec(EmulatedPosixSupport, Object[], Object[], Object, Object[], int, int, int, int, int, int, int, int, boolean, boolean, boolean, int[], FromJavaStringNode)}
* 10: SpecializationActive {@link EmulatedPosixSupport#execv(EmulatedPosixSupport, Object, Object[], EqualNode)}
* 11: SpecializationActive {@link EmulatedPosixSupport#mmap(EmulatedPosixSupport, long, int, int, int, long, Node, InlinedConditionProfile, EqualNode, FromJavaStringNode)}
* 12: SpecializationActive {@link EmulatedPosixSupport#mmapReadByte(EmulatedPosixSupport, Object, long, Node, InlinedBranchProfile, EqualNode)}
* 13: SpecializationActive {@link EmulatedPosixSupport#mmapWriteByte(EmulatedPosixSupport, Object, long, byte, Node, InlinedBranchProfile, EqualNode)}
* 14: SpecializationActive {@link EmulatedPosixSupport#mmapReadBytes(EmulatedPosixSupport, Object, long, byte[], int, Node, InlinedBranchProfile, EqualNode)}
* 15: SpecializationActive {@link EmulatedPosixSupport#mmapWriteBytes(EmulatedPosixSupport, Object, long, byte[], int, Node, InlinedBranchProfile, EqualNode)}
* 16: SpecializationActive {@link EmulatedPosixSupport#mmapUnmap(EmulatedPosixSupport, Object, long, EqualNode)}
* 17: SpecializationActive {@link EmulatedPosixSupport#socket(EmulatedPosixSupport, int, int, int, EqualNode)}
* 18: SpecializationActive {@link EmulatedPosixSupport#createPathFromString(EmulatedPosixSupport, TruffleString, ToJavaStringNode)}
* 19: SpecializationActive {@link EmulatedPosixSupport#getPathAsString(EmulatedPosixSupport, Object, FromJavaStringNode)}
* 20: InlinedCache
* Specialization: {@link EmulatedPosixSupport#strerror}
* Parameter: {@link InlinedBranchProfile} errorBranch
* Inline method: {@link InlinedBranchProfile#inline}
* 21-22: InlinedCache
* Specialization: {@link EmulatedPosixSupport#openat}
* Parameter: {@link InlinedConditionProfile} defaultDirFdPofile
* Inline method: {@link InlinedConditionProfile#inline}
* 23: InlinedCache
* Specialization: {@link EmulatedPosixSupport#lseek}
* Parameter: {@link InlinedBranchProfile} errorBranch
* Inline method: {@link InlinedBranchProfile#inline}
* 24-25: InlinedCache
* Specialization: {@link EmulatedPosixSupport#lseek}
* Parameter: {@link InlinedConditionProfile} notSupported
* Inline method: {@link InlinedConditionProfile#inline}
* 26-27: InlinedCache
* Specialization: {@link EmulatedPosixSupport#lseek}
* Parameter: {@link InlinedConditionProfile} noFile
* Inline method: {@link InlinedConditionProfile#inline}
* 28-29: InlinedCache
* Specialization: {@link EmulatedPosixSupport#lseek}
* Parameter: {@link InlinedConditionProfile} notSeekable
* Inline method: {@link InlinedConditionProfile#inline}
* 30: InlinedCache
* Specialization: {@link EmulatedPosixSupport#fstatat}
* Parameter: {@link InlinedBranchProfile} errorBranch
* Inline method: {@link InlinedBranchProfile#inline}
* 31: InlinedCache
* Specialization: {@link EmulatedPosixSupport#fstat(int, Node, InlinedBranchProfile, InlinedBranchProfile, InlinedConditionProfile, EqualNode, FromJavaStringNode)}
* Parameter: {@link InlinedBranchProfile} nullPathProfile
* Inline method: {@link InlinedBranchProfile#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int state_1_;
/**
* State Info:
* 0-1: InlinedCache
* Specialization: {@link EmulatedPosixSupport#fstatat}
* Parameter: {@link InlinedConditionProfile} defaultDirFdPofile
* Inline method: {@link InlinedConditionProfile#inline}
* 2: InlinedCache
* Specialization: {@link EmulatedPosixSupport#fstat(int, Node, InlinedBranchProfile, InlinedBranchProfile, InlinedConditionProfile, EqualNode, FromJavaStringNode)}
* Parameter: {@link InlinedBranchProfile} errorBranch
* Inline method: {@link InlinedBranchProfile#inline}
* 3-4: InlinedCache
* Specialization: {@link EmulatedPosixSupport#fstat(int, Node, InlinedBranchProfile, InlinedBranchProfile, InlinedConditionProfile, EqualNode, FromJavaStringNode)}
* Parameter: {@link InlinedConditionProfile} defaultDirFdPofile
* Inline method: {@link InlinedConditionProfile#inline}
* 5-7: InlinedCache
* Specialization: {@link EmulatedPosixSupport#utimensat}
* Parameter: {@link SetUTimeNode} setUTimeNode
* Inline method: {@link SetUTimeNodeGen#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int state_2_;
/**
* Source Info:
* Specialization: {@link EmulatedPosixSupport#openat}
* Parameter: {@link EqualNode} eqNode
*/
@Child private EqualNode eq;
/**
* Source Info:
* Specialization: {@link EmulatedPosixSupport#openat}
* Parameter: {@link FromJavaStringNode} fromJavaStringNode
*/
@Child private FromJavaStringNode js2ts;
/**
* Source Info:
* Specialization: {@link EmulatedPosixSupport#openat}
* Parameter: {@link ToJavaStringNode} toJavaStringNode
*/
@Child private ToJavaStringNode ts2js;
/**
* Source Info:
* Specialization: {@link EmulatedPosixSupport#utimensat}
* Parameter: {@link SetUTimeNode} setUTimeNode
* Inline method: {@link SetUTimeNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node setUTime_field1_;
@Child private KillNode_KillData killNode__kill_cache;
protected Cached() {
}
@Override
public boolean accepts(Object receiver) {
assert !(receiver instanceof EmulatedPosixSupport) || DYNAMIC_DISPATCH_LIBRARY_.getUncached().dispatch(receiver) == null : "Invalid library export. Exported receiver with dynamic dispatch found but not expected.";
return receiver instanceof EmulatedPosixSupport;
}
/**
* Debug Info:
* Specialization {@link Getpid#getPid}
* Activation probability: 0.01275
* With/without class size: 4/0 bytes
* Specialization {@link Getpid#getPidFallback}
* Activation probability: 0.00686
* With/without class size: 4/0 bytes
*
*/
@Override
public long getpid(Object arg0Value_) {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
int state_0 = this.state_0_;
if ((state_0 & 0b101) != 0 /* is SpecializationActive[EmulatedPosixSupport.Getpid.getPid(EmulatedPosixSupport)] || SpecializationActive[EmulatedPosixSupport.Getpid.getPidFallback(EmulatedPosixSupport)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[EmulatedPosixSupport.Getpid.getPid(EmulatedPosixSupport)] */) {
try {
return Getpid.getPid(arg0Value);
} catch (Exception ex) {
CompilerDirectives.transferToInterpreterAndInvalidate();
state_0 = this.state_0_;
state_0 = state_0 & 0xfffffffe /* remove SpecializationActive[EmulatedPosixSupport.Getpid.getPid(EmulatedPosixSupport)] */;
state_0 = state_0 | 0b10 /* add SpecializationExcluded */;
this.state_0_ = state_0;
return getpidAndSpecialize(arg0Value);
}
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[EmulatedPosixSupport.Getpid.getPidFallback(EmulatedPosixSupport)] */) {
return Getpid.getPidFallback(arg0Value);
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return getpidAndSpecialize(arg0Value);
}
private long getpidAndSpecialize(EmulatedPosixSupport arg0Value) {
int state_0 = this.state_0_;
if (((state_0 & 0b100)) == 0 /* is-not SpecializationActive[EmulatedPosixSupport.Getpid.getPidFallback(EmulatedPosixSupport)] */ && ((state_0 & 0b10)) == 0 /* is-not SpecializationExcluded */) {
state_0 = state_0 | 0b1 /* add SpecializationActive[EmulatedPosixSupport.Getpid.getPid(EmulatedPosixSupport)] */;
this.state_0_ = state_0;
try {
return Getpid.getPid(arg0Value);
} catch (Exception ex) {
CompilerDirectives.transferToInterpreterAndInvalidate();
state_0 = this.state_0_;
state_0 = state_0 & 0xfffffffe /* remove SpecializationActive[EmulatedPosixSupport.Getpid.getPid(EmulatedPosixSupport)] */;
state_0 = state_0 | 0b10 /* add SpecializationExcluded */;
this.state_0_ = state_0;
return getpidAndSpecialize(arg0Value);
}
}
state_0 = state_0 & 0xfffffffe /* remove SpecializationActive[EmulatedPosixSupport.Getpid.getPid(EmulatedPosixSupport)] */;
state_0 = state_0 | 0b100 /* add SpecializationActive[EmulatedPosixSupport.Getpid.getPidFallback(EmulatedPosixSupport)] */;
this.state_0_ = state_0;
return Getpid.getPidFallback(arg0Value);
}
@Override
public NodeCost getCost() {
int state_0 = this.state_0_;
if ((state_0 & 0b101) == 0) {
return NodeCost.UNINITIALIZED;
} else {
if (((state_0 & 0b101) & ((state_0 & 0b101) - 1)) == 0 /* is-single */) {
return NodeCost.MONOMORPHIC;
}
}
return NodeCost.POLYMORPHIC;
}
/**
* Debug Info:
* Specialization {@link CreateUniversalSockAddr#inet4}
* Activation probability: 0.00948
* With/without class size: 4/0 bytes
* Specialization {@link CreateUniversalSockAddr#inet6}
* Activation probability: 0.00654
* With/without class size: 4/0 bytes
* Specialization {@link CreateUniversalSockAddr#unix}
* Activation probability: 0.00359
* With/without class size: 4/0 bytes
*
*/
@Override
public UniversalSockAddr createUniversalSockAddr(Object arg0Value_, FamilySpecificSockAddr arg1Value) {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
int state_0 = this.state_0_;
if ((state_0 & 0b111000) != 0 /* is SpecializationActive[EmulatedPosixSupport.CreateUniversalSockAddr.inet4(EmulatedPosixSupport, Inet4SockAddr)] || SpecializationActive[EmulatedPosixSupport.CreateUniversalSockAddr.inet6(EmulatedPosixSupport, Inet6SockAddr)] || SpecializationActive[EmulatedPosixSupport.CreateUniversalSockAddr.unix(EmulatedPosixSupport, UnixSockAddr)] */) {
if ((state_0 & 0b1000) != 0 /* is SpecializationActive[EmulatedPosixSupport.CreateUniversalSockAddr.inet4(EmulatedPosixSupport, Inet4SockAddr)] */ && arg1Value instanceof Inet4SockAddr) {
Inet4SockAddr arg1Value_ = (Inet4SockAddr) arg1Value;
return CreateUniversalSockAddr.inet4(arg0Value, arg1Value_);
}
if ((state_0 & 0b10000) != 0 /* is SpecializationActive[EmulatedPosixSupport.CreateUniversalSockAddr.inet6(EmulatedPosixSupport, Inet6SockAddr)] */ && arg1Value instanceof Inet6SockAddr) {
Inet6SockAddr arg1Value_ = (Inet6SockAddr) arg1Value;
return CreateUniversalSockAddr.inet6(arg0Value, arg1Value_);
}
if ((state_0 & 0b100000) != 0 /* is SpecializationActive[EmulatedPosixSupport.CreateUniversalSockAddr.unix(EmulatedPosixSupport, UnixSockAddr)] */ && arg1Value instanceof UnixSockAddr) {
UnixSockAddr arg1Value_ = (UnixSockAddr) arg1Value;
return CreateUniversalSockAddr.unix(arg0Value, arg1Value_);
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return createUniversalSockAddrAndSpecialize(arg0Value, arg1Value);
}
private UniversalSockAddr createUniversalSockAddrAndSpecialize(EmulatedPosixSupport arg0Value, FamilySpecificSockAddr arg1Value) {
int state_0 = this.state_0_;
if (arg1Value instanceof Inet4SockAddr) {
Inet4SockAddr arg1Value_ = (Inet4SockAddr) arg1Value;
state_0 = state_0 | 0b1000 /* add SpecializationActive[EmulatedPosixSupport.CreateUniversalSockAddr.inet4(EmulatedPosixSupport, Inet4SockAddr)] */;
this.state_0_ = state_0;
return CreateUniversalSockAddr.inet4(arg0Value, arg1Value_);
}
if (arg1Value instanceof Inet6SockAddr) {
Inet6SockAddr arg1Value_ = (Inet6SockAddr) arg1Value;
state_0 = state_0 | 0b10000 /* add SpecializationActive[EmulatedPosixSupport.CreateUniversalSockAddr.inet6(EmulatedPosixSupport, Inet6SockAddr)] */;
this.state_0_ = state_0;
return CreateUniversalSockAddr.inet6(arg0Value, arg1Value_);
}
if (arg1Value instanceof UnixSockAddr) {
UnixSockAddr arg1Value_ = (UnixSockAddr) arg1Value;
state_0 = state_0 | 0b100000 /* add SpecializationActive[EmulatedPosixSupport.CreateUniversalSockAddr.unix(EmulatedPosixSupport, UnixSockAddr)] */;
this.state_0_ = state_0;
return CreateUniversalSockAddr.unix(arg0Value, arg1Value_);
}
throw new UnsupportedSpecializationException(this, new Node[] {null, null}, arg0Value, arg1Value);
}
@Override
public TruffleString getBackend(Object receiver) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
return (((EmulatedPosixSupport) receiver)).getBackend();
}
@Override
public int umask(Object receiver, int mask) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
return (((EmulatedPosixSupport) receiver)).umask(mask);
}
/**
* Debug Info:
* Specialization {@link EmulatedPosixSupport#strerror(EmulatedPosixSupport, int, Node, InlinedBranchProfile)}
* Activation probability: 0.01961
* With/without class size: 4/0 bytes
*
*/
@Override
public TruffleString strerror(Object arg0Value_, int arg1Value) {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
{
Node inliningTarget__ = (this);
return arg0Value.strerror(arg1Value, inliningTarget__, INLINED_ERROR_BRANCH);
}
}
@Override
public int close(Object receiver, int fd) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
return (((EmulatedPosixSupport) receiver)).closeMessage(fd);
}
/**
* Debug Info:
* Specialization {@link EmulatedPosixSupport#openat(EmulatedPosixSupport, int, Object, int, int, Node, InlinedBranchProfile, InlinedConditionProfile, EqualNode, FromJavaStringNode, ToJavaStringNode)}
* Activation probability: 0.01961
* With/without class size: 4/0 bytes
*
*/
@Override
public int openat(Object arg0Value_, int arg1Value, Object arg2Value, int arg3Value, int arg4Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
int state_0 = this.state_0_;
if ((state_0 & 0b1000000) != 0 /* is SpecializationActive[EmulatedPosixSupport.openat(EmulatedPosixSupport, int, Object, int, int, Node, InlinedBranchProfile, InlinedConditionProfile, EqualNode, FromJavaStringNode, ToJavaStringNode)] */) {
{
EqualNode eq_ = this.eq;
if (eq_ != null) {
FromJavaStringNode js2ts_ = this.js2ts;
if (js2ts_ != null) {
ToJavaStringNode ts2js_ = this.ts2js;
if (ts2js_ != null) {
Node inliningTarget__ = (this);
return arg0Value.openat(arg1Value, arg2Value, arg3Value, arg4Value, inliningTarget__, INLINED_ERROR_BRANCH, INLINED_DEFAULT_DIR_PROFILE, eq_, js2ts_, ts2js_);
}
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return openatNode_AndSpecialize(arg0Value, arg1Value, arg2Value, arg3Value, arg4Value);
}
private int openatNode_AndSpecialize(EmulatedPosixSupport arg0Value, int arg1Value, Object arg2Value, int arg3Value, int arg4Value) throws PosixException {
int state_0 = this.state_0_;
{
Node inliningTarget__ = null;
inliningTarget__ = (this);
EqualNode eq_;
EqualNode eq__shared = this.eq;
if (eq__shared != null) {
eq_ = eq__shared;
} else {
eq_ = this.insert((EqualNode.create()));
if (eq_ == null) {
throw new IllegalStateException("Specialization 'openat(EmulatedPosixSupport, int, Object, int, int, Node, InlinedBranchProfile, InlinedConditionProfile, EqualNode, FromJavaStringNode, ToJavaStringNode)' contains a shared cache with name 'eqNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.eq == null) {
VarHandle.storeStoreFence();
this.eq = eq_;
}
FromJavaStringNode js2ts_;
FromJavaStringNode js2ts__shared = this.js2ts;
if (js2ts__shared != null) {
js2ts_ = js2ts__shared;
} else {
js2ts_ = this.insert((FromJavaStringNode.create()));
if (js2ts_ == null) {
throw new IllegalStateException("Specialization 'openat(EmulatedPosixSupport, int, Object, int, int, Node, InlinedBranchProfile, InlinedConditionProfile, EqualNode, FromJavaStringNode, ToJavaStringNode)' contains a shared cache with name 'fromJavaStringNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.js2ts == null) {
VarHandle.storeStoreFence();
this.js2ts = js2ts_;
}
ToJavaStringNode ts2js_;
ToJavaStringNode ts2js__shared = this.ts2js;
if (ts2js__shared != null) {
ts2js_ = ts2js__shared;
} else {
ts2js_ = this.insert((ToJavaStringNode.create()));
if (ts2js_ == null) {
throw new IllegalStateException("Specialization 'openat(EmulatedPosixSupport, int, Object, int, int, Node, InlinedBranchProfile, InlinedConditionProfile, EqualNode, FromJavaStringNode, ToJavaStringNode)' contains a shared cache with name 'toJavaStringNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.ts2js == null) {
VarHandle.storeStoreFence();
this.ts2js = ts2js_;
}
state_0 = state_0 | 0b1000000 /* add SpecializationActive[EmulatedPosixSupport.openat(EmulatedPosixSupport, int, Object, int, int, Node, InlinedBranchProfile, InlinedConditionProfile, EqualNode, FromJavaStringNode, ToJavaStringNode)] */;
this.state_0_ = state_0;
return arg0Value.openat(arg1Value, arg2Value, arg3Value, arg4Value, inliningTarget__, INLINED_ERROR_BRANCH, INLINED_DEFAULT_DIR_PROFILE, eq_, js2ts_, ts2js_);
}
}
/**
* Debug Info:
* Specialization {@link EmulatedPosixSupport#write(EmulatedPosixSupport, int, Buffer, Node, InlinedBranchProfile, EqualNode)}
* Activation probability: 0.01961
* With/without class size: 4/0 bytes
*
*/
@Override
public long write(Object arg0Value_, int arg1Value, Buffer arg2Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
int state_0 = this.state_0_;
if ((state_0 & 0b10000000) != 0 /* is SpecializationActive[EmulatedPosixSupport.write(EmulatedPosixSupport, int, Buffer, Node, InlinedBranchProfile, EqualNode)] */) {
{
EqualNode eq_ = this.eq;
if (eq_ != null) {
Node inliningTarget__ = (this);
return arg0Value.write(arg1Value, arg2Value, inliningTarget__, INLINED_ERROR_BRANCH, eq_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return writeNode_AndSpecialize(arg0Value, arg1Value, arg2Value);
}
private long writeNode_AndSpecialize(EmulatedPosixSupport arg0Value, int arg1Value, Buffer arg2Value) throws PosixException {
int state_0 = this.state_0_;
{
Node inliningTarget__ = null;
inliningTarget__ = (this);
EqualNode eq_;
EqualNode eq__shared = this.eq;
if (eq__shared != null) {
eq_ = eq__shared;
} else {
eq_ = this.insert((EqualNode.create()));
if (eq_ == null) {
throw new IllegalStateException("Specialization 'write(EmulatedPosixSupport, int, Buffer, Node, InlinedBranchProfile, EqualNode)' contains a shared cache with name 'eqNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.eq == null) {
VarHandle.storeStoreFence();
this.eq = eq_;
}
state_0 = state_0 | 0b10000000 /* add SpecializationActive[EmulatedPosixSupport.write(EmulatedPosixSupport, int, Buffer, Node, InlinedBranchProfile, EqualNode)] */;
this.state_0_ = state_0;
return arg0Value.write(arg1Value, arg2Value, inliningTarget__, INLINED_ERROR_BRANCH, eq_);
}
}
/**
* Debug Info:
* Specialization {@link EmulatedPosixSupport#read(EmulatedPosixSupport, int, long, Node, InlinedBranchProfile, EqualNode)}
* Activation probability: 0.01961
* With/without class size: 4/0 bytes
*
*/
@Override
public Buffer read(Object arg0Value_, int arg1Value, long arg2Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
int state_0 = this.state_0_;
if ((state_0 & 0b100000000) != 0 /* is SpecializationActive[EmulatedPosixSupport.read(EmulatedPosixSupport, int, long, Node, InlinedBranchProfile, EqualNode)] */) {
{
EqualNode eq_ = this.eq;
if (eq_ != null) {
Node inliningTarget__ = (this);
return arg0Value.read(arg1Value, arg2Value, inliningTarget__, INLINED_ERROR_BRANCH, eq_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return readNode_AndSpecialize(arg0Value, arg1Value, arg2Value);
}
private Buffer readNode_AndSpecialize(EmulatedPosixSupport arg0Value, int arg1Value, long arg2Value) throws PosixException {
int state_0 = this.state_0_;
{
Node inliningTarget__ = null;
inliningTarget__ = (this);
EqualNode eq_;
EqualNode eq__shared = this.eq;
if (eq__shared != null) {
eq_ = eq__shared;
} else {
eq_ = this.insert((EqualNode.create()));
if (eq_ == null) {
throw new IllegalStateException("Specialization 'read(EmulatedPosixSupport, int, long, Node, InlinedBranchProfile, EqualNode)' contains a shared cache with name 'eqNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.eq == null) {
VarHandle.storeStoreFence();
this.eq = eq_;
}
state_0 = state_0 | 0b100000000 /* add SpecializationActive[EmulatedPosixSupport.read(EmulatedPosixSupport, int, long, Node, InlinedBranchProfile, EqualNode)] */;
this.state_0_ = state_0;
return arg0Value.read(arg1Value, arg2Value, inliningTarget__, INLINED_ERROR_BRANCH, eq_);
}
}
@Override
public int dup(Object receiver, int fd) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
return (((EmulatedPosixSupport) receiver)).dup(fd);
}
/**
* Debug Info:
* Specialization {@link EmulatedPosixSupport#dup2(EmulatedPosixSupport, int, int, boolean, EqualNode)}
* Activation probability: 0.01961
* With/without class size: 4/0 bytes
*
*/
@Override
public int dup2(Object arg0Value_, int arg1Value, int arg2Value, boolean arg3Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
int state_0 = this.state_0_;
if ((state_0 & 0b1000000000) != 0 /* is SpecializationActive[EmulatedPosixSupport.dup2(EmulatedPosixSupport, int, int, boolean, EqualNode)] */) {
{
EqualNode eq_ = this.eq;
if (eq_ != null) {
return arg0Value.dup2(arg1Value, arg2Value, arg3Value, eq_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return dup2Node_AndSpecialize(arg0Value, arg1Value, arg2Value, arg3Value);
}
private int dup2Node_AndSpecialize(EmulatedPosixSupport arg0Value, int arg1Value, int arg2Value, boolean arg3Value) throws PosixException {
int state_0 = this.state_0_;
EqualNode eq_;
EqualNode eq__shared = this.eq;
if (eq__shared != null) {
eq_ = eq__shared;
} else {
eq_ = this.insert((EqualNode.create()));
if (eq_ == null) {
throw new IllegalStateException("Specialization 'dup2(EmulatedPosixSupport, int, int, boolean, EqualNode)' contains a shared cache with name 'eqNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.eq == null) {
VarHandle.storeStoreFence();
this.eq = eq_;
}
state_0 = state_0 | 0b1000000000 /* add SpecializationActive[EmulatedPosixSupport.dup2(EmulatedPosixSupport, int, int, boolean, EqualNode)] */;
this.state_0_ = state_0;
return arg0Value.dup2(arg1Value, arg2Value, arg3Value, eq_);
}
@Override
public boolean getInheritable(Object receiver, int fd) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
return (((EmulatedPosixSupport) receiver)).getInheritable(fd);
}
@Override
public void setInheritable(Object receiver, int fd, boolean inheritable) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
(((EmulatedPosixSupport) receiver)).setInheritable(fd, inheritable);
return;
}
/**
* Debug Info:
* Specialization {@link EmulatedPosixSupport#pipeMessage(EmulatedPosixSupport, EqualNode)}
* Activation probability: 0.01961
* With/without class size: 4/0 bytes
*
*/
@Override
public int[] pipe(Object arg0Value_) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
int state_0 = this.state_0_;
if ((state_0 & 0b10000000000) != 0 /* is SpecializationActive[EmulatedPosixSupport.pipeMessage(EmulatedPosixSupport, EqualNode)] */) {
{
EqualNode eq_ = this.eq;
if (eq_ != null) {
return arg0Value.pipeMessage(eq_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return pipeMessageNode_AndSpecialize(arg0Value);
}
private int[] pipeMessageNode_AndSpecialize(EmulatedPosixSupport arg0Value) throws PosixException {
int state_0 = this.state_0_;
EqualNode eq_;
EqualNode eq__shared = this.eq;
if (eq__shared != null) {
eq_ = eq__shared;
} else {
eq_ = this.insert((EqualNode.create()));
if (eq_ == null) {
throw new IllegalStateException("Specialization 'pipeMessage(EmulatedPosixSupport, EqualNode)' contains a shared cache with name 'eqNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.eq == null) {
VarHandle.storeStoreFence();
this.eq = eq_;
}
state_0 = state_0 | 0b10000000000 /* add SpecializationActive[EmulatedPosixSupport.pipeMessage(EmulatedPosixSupport, EqualNode)] */;
this.state_0_ = state_0;
return arg0Value.pipeMessage(eq_);
}
@Override
public SelectResult select(Object receiver, int[] readfds, int[] writefds, int[] errorfds, Timeval timeout) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
return (((EmulatedPosixSupport) receiver)).select(readfds, writefds, errorfds, timeout);
}
/**
* Debug Info:
* Specialization {@link EmulatedPosixSupport#lseek(EmulatedPosixSupport, int, long, int, Node, InlinedBranchProfile, InlinedConditionProfile, InlinedConditionProfile, InlinedConditionProfile, EqualNode)}
* Activation probability: 0.01961
* With/without class size: 4/1 bytes
*
*/
@Override
public long lseek(Object arg0Value_, int arg1Value, long arg2Value, int arg3Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
int state_0 = this.state_0_;
if ((state_0 & 0b100000000000) != 0 /* is SpecializationActive[EmulatedPosixSupport.lseek(EmulatedPosixSupport, int, long, int, Node, InlinedBranchProfile, InlinedConditionProfile, InlinedConditionProfile, InlinedConditionProfile, EqualNode)] */) {
{
EqualNode eq_ = this.eq;
if (eq_ != null) {
Node inliningTarget__ = (this);
return arg0Value.lseek(arg1Value, arg2Value, arg3Value, inliningTarget__, INLINED_LSEEK_NODE__LSEEK_ERROR_BRANCH_, INLINED_LSEEK_NODE__LSEEK_NOT_SUPPORTED_, INLINED_LSEEK_NODE__LSEEK_NO_FILE_, INLINED_LSEEK_NODE__LSEEK_NOT_SEEKABLE_, eq_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return lseekNode_AndSpecialize(arg0Value, arg1Value, arg2Value, arg3Value);
}
private long lseekNode_AndSpecialize(EmulatedPosixSupport arg0Value, int arg1Value, long arg2Value, int arg3Value) throws PosixException {
int state_0 = this.state_0_;
{
Node inliningTarget__ = null;
inliningTarget__ = (this);
EqualNode eq_;
EqualNode eq__shared = this.eq;
if (eq__shared != null) {
eq_ = eq__shared;
} else {
eq_ = this.insert((EqualNode.create()));
if (eq_ == null) {
throw new IllegalStateException("Specialization 'lseek(EmulatedPosixSupport, int, long, int, Node, InlinedBranchProfile, InlinedConditionProfile, InlinedConditionProfile, InlinedConditionProfile, EqualNode)' contains a shared cache with name 'eqNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.eq == null) {
VarHandle.storeStoreFence();
this.eq = eq_;
}
state_0 = state_0 | 0b100000000000 /* add SpecializationActive[EmulatedPosixSupport.lseek(EmulatedPosixSupport, int, long, int, Node, InlinedBranchProfile, InlinedConditionProfile, InlinedConditionProfile, InlinedConditionProfile, EqualNode)] */;
this.state_0_ = state_0;
return arg0Value.lseek(arg1Value, arg2Value, arg3Value, inliningTarget__, INLINED_LSEEK_NODE__LSEEK_ERROR_BRANCH_, INLINED_LSEEK_NODE__LSEEK_NOT_SUPPORTED_, INLINED_LSEEK_NODE__LSEEK_NO_FILE_, INLINED_LSEEK_NODE__LSEEK_NOT_SEEKABLE_, eq_);
}
}
/**
* Debug Info:
* Specialization {@link EmulatedPosixSupport#ftruncateMessage(EmulatedPosixSupport, int, long, Node, InlinedBranchProfile, EqualNode)}
* Activation probability: 0.01961
* With/without class size: 4/0 bytes
*
*/
@Override
public void ftruncate(Object arg0Value_, int arg1Value, long arg2Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
int state_0 = this.state_0_;
if ((state_0 & 0b1000000000000) != 0 /* is SpecializationActive[EmulatedPosixSupport.ftruncateMessage(EmulatedPosixSupport, int, long, Node, InlinedBranchProfile, EqualNode)] */) {
{
EqualNode eq_ = this.eq;
if (eq_ != null) {
Node inliningTarget__ = (this);
arg0Value.ftruncateMessage(arg1Value, arg2Value, inliningTarget__, INLINED_ERROR_BRANCH, eq_);
return;
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
ftruncateMessageNode_AndSpecialize(arg0Value, arg1Value, arg2Value);
return;
}
private void ftruncateMessageNode_AndSpecialize(EmulatedPosixSupport arg0Value, int arg1Value, long arg2Value) throws PosixException {
int state_0 = this.state_0_;
{
Node inliningTarget__ = null;
inliningTarget__ = (this);
EqualNode eq_;
EqualNode eq__shared = this.eq;
if (eq__shared != null) {
eq_ = eq__shared;
} else {
eq_ = this.insert((EqualNode.create()));
if (eq_ == null) {
throw new IllegalStateException("Specialization 'ftruncateMessage(EmulatedPosixSupport, int, long, Node, InlinedBranchProfile, EqualNode)' contains a shared cache with name 'eqNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.eq == null) {
VarHandle.storeStoreFence();
this.eq = eq_;
}
state_0 = state_0 | 0b1000000000000 /* add SpecializationActive[EmulatedPosixSupport.ftruncateMessage(EmulatedPosixSupport, int, long, Node, InlinedBranchProfile, EqualNode)] */;
this.state_0_ = state_0;
arg0Value.ftruncateMessage(arg1Value, arg2Value, inliningTarget__, INLINED_ERROR_BRANCH, eq_);
return;
}
}
@Override
public void fsync(Object receiver, int fd) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
(((EmulatedPosixSupport) receiver)).fsyncMessage(fd);
return;
}
/**
* Debug Info:
* Specialization {@link EmulatedPosixSupport#flock(EmulatedPosixSupport, int, int, Node, InlinedBranchProfile, EqualNode)}
* Activation probability: 0.01961
* With/without class size: 4/0 bytes
*
*/
@Override
public void flock(Object arg0Value_, int arg1Value, int arg2Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
int state_0 = this.state_0_;
if ((state_0 & 0b10000000000000) != 0 /* is SpecializationActive[EmulatedPosixSupport.flock(EmulatedPosixSupport, int, int, Node, InlinedBranchProfile, EqualNode)] */) {
{
EqualNode eq_ = this.eq;
if (eq_ != null) {
Node inliningTarget__ = (this);
arg0Value.flock(arg1Value, arg2Value, inliningTarget__, INLINED_ERROR_BRANCH, eq_);
return;
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
flockNode_AndSpecialize(arg0Value, arg1Value, arg2Value);
return;
}
private void flockNode_AndSpecialize(EmulatedPosixSupport arg0Value, int arg1Value, int arg2Value) throws PosixException {
int state_0 = this.state_0_;
{
Node inliningTarget__ = null;
inliningTarget__ = (this);
EqualNode eq_;
EqualNode eq__shared = this.eq;
if (eq__shared != null) {
eq_ = eq__shared;
} else {
eq_ = this.insert((EqualNode.create()));
if (eq_ == null) {
throw new IllegalStateException("Specialization 'flock(EmulatedPosixSupport, int, int, Node, InlinedBranchProfile, EqualNode)' contains a shared cache with name 'eqNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.eq == null) {
VarHandle.storeStoreFence();
this.eq = eq_;
}
state_0 = state_0 | 0b10000000000000 /* add SpecializationActive[EmulatedPosixSupport.flock(EmulatedPosixSupport, int, int, Node, InlinedBranchProfile, EqualNode)] */;
this.state_0_ = state_0;
arg0Value.flock(arg1Value, arg2Value, inliningTarget__, INLINED_ERROR_BRANCH, eq_);
return;
}
}
@Override
public boolean getBlocking(Object receiver, int fd) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
return (((EmulatedPosixSupport) receiver)).getBlocking(fd);
}
/**
* Debug Info:
* Specialization {@link EmulatedPosixSupport#setBlocking(EmulatedPosixSupport, int, boolean, EqualNode)}
* Activation probability: 0.01961
* With/without class size: 4/0 bytes
*
*/
@Override
public void setBlocking(Object arg0Value_, int arg1Value, boolean arg2Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
int state_0 = this.state_0_;
if ((state_0 & 0b100000000000000) != 0 /* is SpecializationActive[EmulatedPosixSupport.setBlocking(EmulatedPosixSupport, int, boolean, EqualNode)] */) {
{
EqualNode eq_ = this.eq;
if (eq_ != null) {
arg0Value.setBlocking(arg1Value, arg2Value, eq_);
return;
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
setBlockingNode_AndSpecialize(arg0Value, arg1Value, arg2Value);
return;
}
private void setBlockingNode_AndSpecialize(EmulatedPosixSupport arg0Value, int arg1Value, boolean arg2Value) throws PosixException {
int state_0 = this.state_0_;
EqualNode eq_;
EqualNode eq__shared = this.eq;
if (eq__shared != null) {
eq_ = eq__shared;
} else {
eq_ = this.insert((EqualNode.create()));
if (eq_ == null) {
throw new IllegalStateException("Specialization 'setBlocking(EmulatedPosixSupport, int, boolean, EqualNode)' contains a shared cache with name 'eqNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.eq == null) {
VarHandle.storeStoreFence();
this.eq = eq_;
}
state_0 = state_0 | 0b100000000000000 /* add SpecializationActive[EmulatedPosixSupport.setBlocking(EmulatedPosixSupport, int, boolean, EqualNode)] */;
this.state_0_ = state_0;
arg0Value.setBlocking(arg1Value, arg2Value, eq_);
return;
}
@Override
public int[] getTerminalSize(Object receiver, int fd) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
return (((EmulatedPosixSupport) receiver)).getTerminalSize(fd);
}
/**
* Debug Info:
* Specialization {@link EmulatedPosixSupport#fstatat(EmulatedPosixSupport, int, Object, boolean, Node, InlinedBranchProfile, InlinedConditionProfile, EqualNode, FromJavaStringNode, ToJavaStringNode)}
* Activation probability: 0.01961
* With/without class size: 4/1 bytes
*
*/
@Override
public long[] fstatat(Object arg0Value_, int arg1Value, Object arg2Value, boolean arg3Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
int state_0 = this.state_0_;
if ((state_0 & 0b1000000000000000) != 0 /* is SpecializationActive[EmulatedPosixSupport.fstatat(EmulatedPosixSupport, int, Object, boolean, Node, InlinedBranchProfile, InlinedConditionProfile, EqualNode, FromJavaStringNode, ToJavaStringNode)] */) {
{
EqualNode eq_ = this.eq;
if (eq_ != null) {
FromJavaStringNode js2ts_ = this.js2ts;
if (js2ts_ != null) {
ToJavaStringNode ts2js_ = this.ts2js;
if (ts2js_ != null) {
Node inliningTarget__ = (this);
return arg0Value.fstatat(arg1Value, arg2Value, arg3Value, inliningTarget__, INLINED_FSTATAT_NODE__FSTATAT_ERROR_BRANCH_, INLINED_FSTATAT_NODE__FSTATAT_DEFAULT_DIR_FD_POFILE_, eq_, js2ts_, ts2js_);
}
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return fstatatNode_AndSpecialize(arg0Value, arg1Value, arg2Value, arg3Value);
}
private long[] fstatatNode_AndSpecialize(EmulatedPosixSupport arg0Value, int arg1Value, Object arg2Value, boolean arg3Value) throws PosixException {
int state_0 = this.state_0_;
{
Node inliningTarget__ = null;
inliningTarget__ = (this);
EqualNode eq_;
EqualNode eq__shared = this.eq;
if (eq__shared != null) {
eq_ = eq__shared;
} else {
eq_ = this.insert((EqualNode.create()));
if (eq_ == null) {
throw new IllegalStateException("Specialization 'fstatat(EmulatedPosixSupport, int, Object, boolean, Node, InlinedBranchProfile, InlinedConditionProfile, EqualNode, FromJavaStringNode, ToJavaStringNode)' contains a shared cache with name 'eqNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.eq == null) {
VarHandle.storeStoreFence();
this.eq = eq_;
}
FromJavaStringNode js2ts_;
FromJavaStringNode js2ts__shared = this.js2ts;
if (js2ts__shared != null) {
js2ts_ = js2ts__shared;
} else {
js2ts_ = this.insert((FromJavaStringNode.create()));
if (js2ts_ == null) {
throw new IllegalStateException("Specialization 'fstatat(EmulatedPosixSupport, int, Object, boolean, Node, InlinedBranchProfile, InlinedConditionProfile, EqualNode, FromJavaStringNode, ToJavaStringNode)' contains a shared cache with name 'fromJavaStringNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.js2ts == null) {
VarHandle.storeStoreFence();
this.js2ts = js2ts_;
}
ToJavaStringNode ts2js_;
ToJavaStringNode ts2js__shared = this.ts2js;
if (ts2js__shared != null) {
ts2js_ = ts2js__shared;
} else {
ts2js_ = this.insert((ToJavaStringNode.create()));
if (ts2js_ == null) {
throw new IllegalStateException("Specialization 'fstatat(EmulatedPosixSupport, int, Object, boolean, Node, InlinedBranchProfile, InlinedConditionProfile, EqualNode, FromJavaStringNode, ToJavaStringNode)' contains a shared cache with name 'toJavaStringNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.ts2js == null) {
VarHandle.storeStoreFence();
this.ts2js = ts2js_;
}
state_0 = state_0 | 0b1000000000000000 /* add SpecializationActive[EmulatedPosixSupport.fstatat(EmulatedPosixSupport, int, Object, boolean, Node, InlinedBranchProfile, InlinedConditionProfile, EqualNode, FromJavaStringNode, ToJavaStringNode)] */;
this.state_0_ = state_0;
return arg0Value.fstatat(arg1Value, arg2Value, arg3Value, inliningTarget__, INLINED_FSTATAT_NODE__FSTATAT_ERROR_BRANCH_, INLINED_FSTATAT_NODE__FSTATAT_DEFAULT_DIR_FD_POFILE_, eq_, js2ts_, ts2js_);
}
}
/**
* Debug Info:
* Specialization {@link EmulatedPosixSupport#fstat(EmulatedPosixSupport, int, Node, InlinedBranchProfile, InlinedBranchProfile, InlinedConditionProfile, EqualNode, FromJavaStringNode)}
* Activation probability: 0.01961
* With/without class size: 4/1 bytes
*
*/
@Override
public long[] fstat(Object arg0Value_, int arg1Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
int state_0 = this.state_0_;
if ((state_0 & 0x10000) != 0 /* is SpecializationActive[EmulatedPosixSupport.fstat(EmulatedPosixSupport, int, Node, InlinedBranchProfile, InlinedBranchProfile, InlinedConditionProfile, EqualNode, FromJavaStringNode)] */) {
{
EqualNode eq_ = this.eq;
if (eq_ != null) {
FromJavaStringNode js2ts_ = this.js2ts;
if (js2ts_ != null) {
Node inliningTarget__ = (this);
return arg0Value.fstat(arg1Value, inliningTarget__, INLINED_FSTAT_NODE__FSTAT_NULL_PATH_PROFILE_, INLINED_FSTAT_NODE__FSTAT_ERROR_BRANCH_, INLINED_FSTAT_NODE__FSTAT_DEFAULT_DIR_FD_POFILE_, eq_, js2ts_);
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return fstatNode_AndSpecialize(arg0Value, arg1Value);
}
private long[] fstatNode_AndSpecialize(EmulatedPosixSupport arg0Value, int arg1Value) throws PosixException {
int state_0 = this.state_0_;
{
Node inliningTarget__ = null;
inliningTarget__ = (this);
EqualNode eq_;
EqualNode eq__shared = this.eq;
if (eq__shared != null) {
eq_ = eq__shared;
} else {
eq_ = this.insert((EqualNode.create()));
if (eq_ == null) {
throw new IllegalStateException("Specialization 'fstat(EmulatedPosixSupport, int, Node, InlinedBranchProfile, InlinedBranchProfile, InlinedConditionProfile, EqualNode, FromJavaStringNode)' contains a shared cache with name 'eqNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.eq == null) {
VarHandle.storeStoreFence();
this.eq = eq_;
}
FromJavaStringNode js2ts_;
FromJavaStringNode js2ts__shared = this.js2ts;
if (js2ts__shared != null) {
js2ts_ = js2ts__shared;
} else {
js2ts_ = this.insert((FromJavaStringNode.create()));
if (js2ts_ == null) {
throw new IllegalStateException("Specialization 'fstat(EmulatedPosixSupport, int, Node, InlinedBranchProfile, InlinedBranchProfile, InlinedConditionProfile, EqualNode, FromJavaStringNode)' contains a shared cache with name 'fromJavaStringNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.js2ts == null) {
VarHandle.storeStoreFence();
this.js2ts = js2ts_;
}
state_0 = state_0 | 0x10000 /* add SpecializationActive[EmulatedPosixSupport.fstat(EmulatedPosixSupport, int, Node, InlinedBranchProfile, InlinedBranchProfile, InlinedConditionProfile, EqualNode, FromJavaStringNode)] */;
this.state_0_ = state_0;
return arg0Value.fstat(arg1Value, inliningTarget__, INLINED_FSTAT_NODE__FSTAT_NULL_PATH_PROFILE_, INLINED_FSTAT_NODE__FSTAT_ERROR_BRANCH_, INLINED_FSTAT_NODE__FSTAT_DEFAULT_DIR_FD_POFILE_, eq_, js2ts_);
}
}
@Override
public long[] statvfs(Object receiver, Object path) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
return (((EmulatedPosixSupport) receiver)).statvfs(path);
}
@Override
public long[] fstatvfs(Object receiver, int fd) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
return (((EmulatedPosixSupport) receiver)).fstatvfs(fd);
}
/**
* Debug Info:
* Specialization {@link EmulatedPosixSupport#uname(EmulatedPosixSupport, FromJavaStringNode)}
* Activation probability: 0.01961
* With/without class size: 4/0 bytes
*
*/
@Override
public Object[] uname(Object arg0Value_) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
int state_0 = this.state_0_;
if ((state_0 & 0x20000) != 0 /* is SpecializationActive[EmulatedPosixSupport.uname(EmulatedPosixSupport, FromJavaStringNode)] */) {
{
FromJavaStringNode js2ts_ = this.js2ts;
if (js2ts_ != null) {
return arg0Value.uname(js2ts_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return unameNode_AndSpecialize(arg0Value);
}
private Object[] unameNode_AndSpecialize(EmulatedPosixSupport arg0Value) {
int state_0 = this.state_0_;
FromJavaStringNode js2ts_;
FromJavaStringNode js2ts__shared = this.js2ts;
if (js2ts__shared != null) {
js2ts_ = js2ts__shared;
} else {
js2ts_ = this.insert((FromJavaStringNode.create()));
if (js2ts_ == null) {
throw new IllegalStateException("Specialization 'uname(EmulatedPosixSupport, FromJavaStringNode)' contains a shared cache with name 'fromJavaStringNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.js2ts == null) {
VarHandle.storeStoreFence();
this.js2ts = js2ts_;
}
state_0 = state_0 | 0x20000 /* add SpecializationActive[EmulatedPosixSupport.uname(EmulatedPosixSupport, FromJavaStringNode)] */;
this.state_0_ = state_0;
return arg0Value.uname(js2ts_);
}
/**
* Debug Info:
* Specialization {@link EmulatedPosixSupport#unlinkat(EmulatedPosixSupport, int, Object, boolean, Node, InlinedBranchProfile, InlinedConditionProfile, EqualNode, FromJavaStringNode, ToJavaStringNode)}
* Activation probability: 0.01961
* With/without class size: 4/0 bytes
*
*/
@Override
public void unlinkat(Object arg0Value_, int arg1Value, Object arg2Value, boolean arg3Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
int state_0 = this.state_0_;
if ((state_0 & 0x40000) != 0 /* is SpecializationActive[EmulatedPosixSupport.unlinkat(EmulatedPosixSupport, int, Object, boolean, Node, InlinedBranchProfile, InlinedConditionProfile, EqualNode, FromJavaStringNode, ToJavaStringNode)] */) {
{
EqualNode eq_ = this.eq;
if (eq_ != null) {
FromJavaStringNode js2ts_ = this.js2ts;
if (js2ts_ != null) {
ToJavaStringNode ts2js_ = this.ts2js;
if (ts2js_ != null) {
Node inliningTarget__ = (this);
arg0Value.unlinkat(arg1Value, arg2Value, arg3Value, inliningTarget__, INLINED_ERROR_BRANCH, INLINED_DEFAULT_DIR_PROFILE, eq_, js2ts_, ts2js_);
return;
}
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
unlinkatNode_AndSpecialize(arg0Value, arg1Value, arg2Value, arg3Value);
return;
}
private void unlinkatNode_AndSpecialize(EmulatedPosixSupport arg0Value, int arg1Value, Object arg2Value, boolean arg3Value) throws PosixException {
int state_0 = this.state_0_;
{
Node inliningTarget__ = null;
inliningTarget__ = (this);
EqualNode eq_;
EqualNode eq__shared = this.eq;
if (eq__shared != null) {
eq_ = eq__shared;
} else {
eq_ = this.insert((EqualNode.create()));
if (eq_ == null) {
throw new IllegalStateException("Specialization 'unlinkat(EmulatedPosixSupport, int, Object, boolean, Node, InlinedBranchProfile, InlinedConditionProfile, EqualNode, FromJavaStringNode, ToJavaStringNode)' contains a shared cache with name 'eqNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.eq == null) {
VarHandle.storeStoreFence();
this.eq = eq_;
}
FromJavaStringNode js2ts_;
FromJavaStringNode js2ts__shared = this.js2ts;
if (js2ts__shared != null) {
js2ts_ = js2ts__shared;
} else {
js2ts_ = this.insert((FromJavaStringNode.create()));
if (js2ts_ == null) {
throw new IllegalStateException("Specialization 'unlinkat(EmulatedPosixSupport, int, Object, boolean, Node, InlinedBranchProfile, InlinedConditionProfile, EqualNode, FromJavaStringNode, ToJavaStringNode)' contains a shared cache with name 'fromJavaStringNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.js2ts == null) {
VarHandle.storeStoreFence();
this.js2ts = js2ts_;
}
ToJavaStringNode ts2js_;
ToJavaStringNode ts2js__shared = this.ts2js;
if (ts2js__shared != null) {
ts2js_ = ts2js__shared;
} else {
ts2js_ = this.insert((ToJavaStringNode.create()));
if (ts2js_ == null) {
throw new IllegalStateException("Specialization 'unlinkat(EmulatedPosixSupport, int, Object, boolean, Node, InlinedBranchProfile, InlinedConditionProfile, EqualNode, FromJavaStringNode, ToJavaStringNode)' contains a shared cache with name 'toJavaStringNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.ts2js == null) {
VarHandle.storeStoreFence();
this.ts2js = ts2js_;
}
state_0 = state_0 | 0x40000 /* add SpecializationActive[EmulatedPosixSupport.unlinkat(EmulatedPosixSupport, int, Object, boolean, Node, InlinedBranchProfile, InlinedConditionProfile, EqualNode, FromJavaStringNode, ToJavaStringNode)] */;
this.state_0_ = state_0;
arg0Value.unlinkat(arg1Value, arg2Value, arg3Value, inliningTarget__, INLINED_ERROR_BRANCH, INLINED_DEFAULT_DIR_PROFILE, eq_, js2ts_, ts2js_);
return;
}
}
/**
* Debug Info:
* Specialization {@link EmulatedPosixSupport#linkat(EmulatedPosixSupport, int, Object, int, Object, int, Node, InlinedBranchProfile, InlinedConditionProfile, EqualNode, FromJavaStringNode, ToJavaStringNode)}
* Activation probability: 0.01961
* With/without class size: 4/0 bytes
*
*/
@Override
public void linkat(Object arg0Value_, int arg1Value, Object arg2Value, int arg3Value, Object arg4Value, int arg5Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
int state_0 = this.state_0_;
if ((state_0 & 0x80000) != 0 /* is SpecializationActive[EmulatedPosixSupport.linkat(EmulatedPosixSupport, int, Object, int, Object, int, Node, InlinedBranchProfile, InlinedConditionProfile, EqualNode, FromJavaStringNode, ToJavaStringNode)] */) {
{
EqualNode eq_ = this.eq;
if (eq_ != null) {
FromJavaStringNode js2ts_ = this.js2ts;
if (js2ts_ != null) {
ToJavaStringNode ts2js_ = this.ts2js;
if (ts2js_ != null) {
Node inliningTarget__ = (this);
arg0Value.linkat(arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, inliningTarget__, INLINED_ERROR_BRANCH, INLINED_DEFAULT_DIR_PROFILE, eq_, js2ts_, ts2js_);
return;
}
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
linkatNode_AndSpecialize(arg0Value, arg1Value, arg2Value, arg3Value, arg4Value, arg5Value);
return;
}
private void linkatNode_AndSpecialize(EmulatedPosixSupport arg0Value, int arg1Value, Object arg2Value, int arg3Value, Object arg4Value, int arg5Value) throws PosixException {
int state_0 = this.state_0_;
{
Node inliningTarget__ = null;
inliningTarget__ = (this);
EqualNode eq_;
EqualNode eq__shared = this.eq;
if (eq__shared != null) {
eq_ = eq__shared;
} else {
eq_ = this.insert((EqualNode.create()));
if (eq_ == null) {
throw new IllegalStateException("Specialization 'linkat(EmulatedPosixSupport, int, Object, int, Object, int, Node, InlinedBranchProfile, InlinedConditionProfile, EqualNode, FromJavaStringNode, ToJavaStringNode)' contains a shared cache with name 'eqNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.eq == null) {
VarHandle.storeStoreFence();
this.eq = eq_;
}
FromJavaStringNode js2ts_;
FromJavaStringNode js2ts__shared = this.js2ts;
if (js2ts__shared != null) {
js2ts_ = js2ts__shared;
} else {
js2ts_ = this.insert((FromJavaStringNode.create()));
if (js2ts_ == null) {
throw new IllegalStateException("Specialization 'linkat(EmulatedPosixSupport, int, Object, int, Object, int, Node, InlinedBranchProfile, InlinedConditionProfile, EqualNode, FromJavaStringNode, ToJavaStringNode)' contains a shared cache with name 'fromJavaStringNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.js2ts == null) {
VarHandle.storeStoreFence();
this.js2ts = js2ts_;
}
ToJavaStringNode ts2js_;
ToJavaStringNode ts2js__shared = this.ts2js;
if (ts2js__shared != null) {
ts2js_ = ts2js__shared;
} else {
ts2js_ = this.insert((ToJavaStringNode.create()));
if (ts2js_ == null) {
throw new IllegalStateException("Specialization 'linkat(EmulatedPosixSupport, int, Object, int, Object, int, Node, InlinedBranchProfile, InlinedConditionProfile, EqualNode, FromJavaStringNode, ToJavaStringNode)' contains a shared cache with name 'toJavaStringNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.ts2js == null) {
VarHandle.storeStoreFence();
this.ts2js = ts2js_;
}
state_0 = state_0 | 0x80000 /* add SpecializationActive[EmulatedPosixSupport.linkat(EmulatedPosixSupport, int, Object, int, Object, int, Node, InlinedBranchProfile, InlinedConditionProfile, EqualNode, FromJavaStringNode, ToJavaStringNode)] */;
this.state_0_ = state_0;
arg0Value.linkat(arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, inliningTarget__, INLINED_ERROR_BRANCH, INLINED_DEFAULT_DIR_PROFILE, eq_, js2ts_, ts2js_);
return;
}
}
/**
* Debug Info:
* Specialization {@link EmulatedPosixSupport#symlinkat(EmulatedPosixSupport, Object, int, Object, Node, InlinedBranchProfile, InlinedConditionProfile, EqualNode, FromJavaStringNode, ToJavaStringNode)}
* Activation probability: 0.01961
* With/without class size: 4/0 bytes
*
*/
@Override
public void symlinkat(Object arg0Value_, Object arg1Value, int arg2Value, Object arg3Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
int state_0 = this.state_0_;
if ((state_0 & 0x100000) != 0 /* is SpecializationActive[EmulatedPosixSupport.symlinkat(EmulatedPosixSupport, Object, int, Object, Node, InlinedBranchProfile, InlinedConditionProfile, EqualNode, FromJavaStringNode, ToJavaStringNode)] */) {
{
EqualNode eq_ = this.eq;
if (eq_ != null) {
FromJavaStringNode js2ts_ = this.js2ts;
if (js2ts_ != null) {
ToJavaStringNode ts2js_ = this.ts2js;
if (ts2js_ != null) {
Node inliningTarget__ = (this);
arg0Value.symlinkat(arg1Value, arg2Value, arg3Value, inliningTarget__, INLINED_ERROR_BRANCH, INLINED_DEFAULT_DIR_PROFILE, eq_, js2ts_, ts2js_);
return;
}
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
symlinkatNode_AndSpecialize(arg0Value, arg1Value, arg2Value, arg3Value);
return;
}
private void symlinkatNode_AndSpecialize(EmulatedPosixSupport arg0Value, Object arg1Value, int arg2Value, Object arg3Value) throws PosixException {
int state_0 = this.state_0_;
{
Node inliningTarget__ = null;
inliningTarget__ = (this);
EqualNode eq_;
EqualNode eq__shared = this.eq;
if (eq__shared != null) {
eq_ = eq__shared;
} else {
eq_ = this.insert((EqualNode.create()));
if (eq_ == null) {
throw new IllegalStateException("Specialization 'symlinkat(EmulatedPosixSupport, Object, int, Object, Node, InlinedBranchProfile, InlinedConditionProfile, EqualNode, FromJavaStringNode, ToJavaStringNode)' contains a shared cache with name 'eqNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.eq == null) {
VarHandle.storeStoreFence();
this.eq = eq_;
}
FromJavaStringNode js2ts_;
FromJavaStringNode js2ts__shared = this.js2ts;
if (js2ts__shared != null) {
js2ts_ = js2ts__shared;
} else {
js2ts_ = this.insert((FromJavaStringNode.create()));
if (js2ts_ == null) {
throw new IllegalStateException("Specialization 'symlinkat(EmulatedPosixSupport, Object, int, Object, Node, InlinedBranchProfile, InlinedConditionProfile, EqualNode, FromJavaStringNode, ToJavaStringNode)' contains a shared cache with name 'fromJavaStringNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.js2ts == null) {
VarHandle.storeStoreFence();
this.js2ts = js2ts_;
}
ToJavaStringNode ts2js_;
ToJavaStringNode ts2js__shared = this.ts2js;
if (ts2js__shared != null) {
ts2js_ = ts2js__shared;
} else {
ts2js_ = this.insert((ToJavaStringNode.create()));
if (ts2js_ == null) {
throw new IllegalStateException("Specialization 'symlinkat(EmulatedPosixSupport, Object, int, Object, Node, InlinedBranchProfile, InlinedConditionProfile, EqualNode, FromJavaStringNode, ToJavaStringNode)' contains a shared cache with name 'toJavaStringNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.ts2js == null) {
VarHandle.storeStoreFence();
this.ts2js = ts2js_;
}
state_0 = state_0 | 0x100000 /* add SpecializationActive[EmulatedPosixSupport.symlinkat(EmulatedPosixSupport, Object, int, Object, Node, InlinedBranchProfile, InlinedConditionProfile, EqualNode, FromJavaStringNode, ToJavaStringNode)] */;
this.state_0_ = state_0;
arg0Value.symlinkat(arg1Value, arg2Value, arg3Value, inliningTarget__, INLINED_ERROR_BRANCH, INLINED_DEFAULT_DIR_PROFILE, eq_, js2ts_, ts2js_);
return;
}
}
/**
* Debug Info:
* Specialization {@link EmulatedPosixSupport#mkdirat(EmulatedPosixSupport, int, Object, int, Node, InlinedBranchProfile, InlinedConditionProfile, EqualNode, FromJavaStringNode, ToJavaStringNode)}
* Activation probability: 0.01961
* With/without class size: 4/0 bytes
*
*/
@Override
public void mkdirat(Object arg0Value_, int arg1Value, Object arg2Value, int arg3Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
int state_0 = this.state_0_;
if ((state_0 & 0x200000) != 0 /* is SpecializationActive[EmulatedPosixSupport.mkdirat(EmulatedPosixSupport, int, Object, int, Node, InlinedBranchProfile, InlinedConditionProfile, EqualNode, FromJavaStringNode, ToJavaStringNode)] */) {
{
EqualNode eq_ = this.eq;
if (eq_ != null) {
FromJavaStringNode js2ts_ = this.js2ts;
if (js2ts_ != null) {
ToJavaStringNode ts2js_ = this.ts2js;
if (ts2js_ != null) {
Node inliningTarget__ = (this);
arg0Value.mkdirat(arg1Value, arg2Value, arg3Value, inliningTarget__, INLINED_ERROR_BRANCH, INLINED_DEFAULT_DIR_PROFILE, eq_, js2ts_, ts2js_);
return;
}
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
mkdiratNode_AndSpecialize(arg0Value, arg1Value, arg2Value, arg3Value);
return;
}
private void mkdiratNode_AndSpecialize(EmulatedPosixSupport arg0Value, int arg1Value, Object arg2Value, int arg3Value) throws PosixException {
int state_0 = this.state_0_;
{
Node inliningTarget__ = null;
inliningTarget__ = (this);
EqualNode eq_;
EqualNode eq__shared = this.eq;
if (eq__shared != null) {
eq_ = eq__shared;
} else {
eq_ = this.insert((EqualNode.create()));
if (eq_ == null) {
throw new IllegalStateException("Specialization 'mkdirat(EmulatedPosixSupport, int, Object, int, Node, InlinedBranchProfile, InlinedConditionProfile, EqualNode, FromJavaStringNode, ToJavaStringNode)' contains a shared cache with name 'eqNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.eq == null) {
VarHandle.storeStoreFence();
this.eq = eq_;
}
FromJavaStringNode js2ts_;
FromJavaStringNode js2ts__shared = this.js2ts;
if (js2ts__shared != null) {
js2ts_ = js2ts__shared;
} else {
js2ts_ = this.insert((FromJavaStringNode.create()));
if (js2ts_ == null) {
throw new IllegalStateException("Specialization 'mkdirat(EmulatedPosixSupport, int, Object, int, Node, InlinedBranchProfile, InlinedConditionProfile, EqualNode, FromJavaStringNode, ToJavaStringNode)' contains a shared cache with name 'fromJavaStringNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.js2ts == null) {
VarHandle.storeStoreFence();
this.js2ts = js2ts_;
}
ToJavaStringNode ts2js_;
ToJavaStringNode ts2js__shared = this.ts2js;
if (ts2js__shared != null) {
ts2js_ = ts2js__shared;
} else {
ts2js_ = this.insert((ToJavaStringNode.create()));
if (ts2js_ == null) {
throw new IllegalStateException("Specialization 'mkdirat(EmulatedPosixSupport, int, Object, int, Node, InlinedBranchProfile, InlinedConditionProfile, EqualNode, FromJavaStringNode, ToJavaStringNode)' contains a shared cache with name 'toJavaStringNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.ts2js == null) {
VarHandle.storeStoreFence();
this.ts2js = ts2js_;
}
state_0 = state_0 | 0x200000 /* add SpecializationActive[EmulatedPosixSupport.mkdirat(EmulatedPosixSupport, int, Object, int, Node, InlinedBranchProfile, InlinedConditionProfile, EqualNode, FromJavaStringNode, ToJavaStringNode)] */;
this.state_0_ = state_0;
arg0Value.mkdirat(arg1Value, arg2Value, arg3Value, inliningTarget__, INLINED_ERROR_BRANCH, INLINED_DEFAULT_DIR_PROFILE, eq_, js2ts_, ts2js_);
return;
}
}
@Override
public Object getcwd(Object receiver) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
return (((EmulatedPosixSupport) receiver)).getcwd();
}
/**
* Debug Info:
* Specialization {@link EmulatedPosixSupport#chdir(EmulatedPosixSupport, Object, Node, InlinedBranchProfile, EqualNode, FromJavaStringNode)}
* Activation probability: 0.01961
* With/without class size: 4/0 bytes
*
*/
@Override
public void chdir(Object arg0Value_, Object arg1Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
int state_0 = this.state_0_;
if ((state_0 & 0x400000) != 0 /* is SpecializationActive[EmulatedPosixSupport.chdir(EmulatedPosixSupport, Object, Node, InlinedBranchProfile, EqualNode, FromJavaStringNode)] */) {
{
EqualNode eq_ = this.eq;
if (eq_ != null) {
FromJavaStringNode js2ts_ = this.js2ts;
if (js2ts_ != null) {
Node inliningTarget__ = (this);
arg0Value.chdir(arg1Value, inliningTarget__, INLINED_ERROR_BRANCH, eq_, js2ts_);
return;
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
chdirNode_AndSpecialize(arg0Value, arg1Value);
return;
}
private void chdirNode_AndSpecialize(EmulatedPosixSupport arg0Value, Object arg1Value) throws PosixException {
int state_0 = this.state_0_;
{
Node inliningTarget__ = null;
inliningTarget__ = (this);
EqualNode eq_;
EqualNode eq__shared = this.eq;
if (eq__shared != null) {
eq_ = eq__shared;
} else {
eq_ = this.insert((EqualNode.create()));
if (eq_ == null) {
throw new IllegalStateException("Specialization 'chdir(EmulatedPosixSupport, Object, Node, InlinedBranchProfile, EqualNode, FromJavaStringNode)' contains a shared cache with name 'eqNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.eq == null) {
VarHandle.storeStoreFence();
this.eq = eq_;
}
FromJavaStringNode js2ts_;
FromJavaStringNode js2ts__shared = this.js2ts;
if (js2ts__shared != null) {
js2ts_ = js2ts__shared;
} else {
js2ts_ = this.insert((FromJavaStringNode.create()));
if (js2ts_ == null) {
throw new IllegalStateException("Specialization 'chdir(EmulatedPosixSupport, Object, Node, InlinedBranchProfile, EqualNode, FromJavaStringNode)' contains a shared cache with name 'fromJavaStringNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.js2ts == null) {
VarHandle.storeStoreFence();
this.js2ts = js2ts_;
}
state_0 = state_0 | 0x400000 /* add SpecializationActive[EmulatedPosixSupport.chdir(EmulatedPosixSupport, Object, Node, InlinedBranchProfile, EqualNode, FromJavaStringNode)] */;
this.state_0_ = state_0;
arg0Value.chdir(arg1Value, inliningTarget__, INLINED_ERROR_BRANCH, eq_, js2ts_);
return;
}
}
/**
* Debug Info:
* Specialization {@link EmulatedPosixSupport#fchdir(EmulatedPosixSupport, int, Node, InlinedBranchProfile, EqualNode, FromJavaStringNode)}
* Activation probability: 0.01961
* With/without class size: 4/0 bytes
*
*/
@Override
public void fchdir(Object arg0Value_, int arg1Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
int state_0 = this.state_0_;
if ((state_0 & 0x800000) != 0 /* is SpecializationActive[EmulatedPosixSupport.fchdir(EmulatedPosixSupport, int, Node, InlinedBranchProfile, EqualNode, FromJavaStringNode)] */) {
{
EqualNode eq_ = this.eq;
if (eq_ != null) {
FromJavaStringNode js2ts_ = this.js2ts;
if (js2ts_ != null) {
Node inliningTarget__ = (this);
arg0Value.fchdir(arg1Value, inliningTarget__, INLINED_ERROR_BRANCH, eq_, js2ts_);
return;
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
fchdirNode_AndSpecialize(arg0Value, arg1Value);
return;
}
private void fchdirNode_AndSpecialize(EmulatedPosixSupport arg0Value, int arg1Value) throws PosixException {
int state_0 = this.state_0_;
{
Node inliningTarget__ = null;
inliningTarget__ = (this);
EqualNode eq_;
EqualNode eq__shared = this.eq;
if (eq__shared != null) {
eq_ = eq__shared;
} else {
eq_ = this.insert((EqualNode.create()));
if (eq_ == null) {
throw new IllegalStateException("Specialization 'fchdir(EmulatedPosixSupport, int, Node, InlinedBranchProfile, EqualNode, FromJavaStringNode)' contains a shared cache with name 'eqNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.eq == null) {
VarHandle.storeStoreFence();
this.eq = eq_;
}
FromJavaStringNode js2ts_;
FromJavaStringNode js2ts__shared = this.js2ts;
if (js2ts__shared != null) {
js2ts_ = js2ts__shared;
} else {
js2ts_ = this.insert((FromJavaStringNode.create()));
if (js2ts_ == null) {
throw new IllegalStateException("Specialization 'fchdir(EmulatedPosixSupport, int, Node, InlinedBranchProfile, EqualNode, FromJavaStringNode)' contains a shared cache with name 'fromJavaStringNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.js2ts == null) {
VarHandle.storeStoreFence();
this.js2ts = js2ts_;
}
state_0 = state_0 | 0x800000 /* add SpecializationActive[EmulatedPosixSupport.fchdir(EmulatedPosixSupport, int, Node, InlinedBranchProfile, EqualNode, FromJavaStringNode)] */;
this.state_0_ = state_0;
arg0Value.fchdir(arg1Value, inliningTarget__, INLINED_ERROR_BRANCH, eq_, js2ts_);
return;
}
}
@Override
public boolean isatty(Object receiver, int fd) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
return (((EmulatedPosixSupport) receiver)).isatty(fd);
}
/**
* Debug Info:
* Specialization {@link EmulatedPosixSupport#opendir(EmulatedPosixSupport, Object, EqualNode, FromJavaStringNode)}
* Activation probability: 0.01961
* With/without class size: 4/0 bytes
*
*/
@Override
public Object opendir(Object arg0Value_, Object arg1Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
int state_0 = this.state_0_;
if ((state_0 & 0x1000000) != 0 /* is SpecializationActive[EmulatedPosixSupport.opendir(EmulatedPosixSupport, Object, EqualNode, FromJavaStringNode)] */) {
{
EqualNode eq_ = this.eq;
if (eq_ != null) {
FromJavaStringNode js2ts_ = this.js2ts;
if (js2ts_ != null) {
return arg0Value.opendir(arg1Value, eq_, js2ts_);
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return opendirNode_AndSpecialize(arg0Value, arg1Value);
}
private Object opendirNode_AndSpecialize(EmulatedPosixSupport arg0Value, Object arg1Value) throws PosixException {
int state_0 = this.state_0_;
EqualNode eq_;
EqualNode eq__shared = this.eq;
if (eq__shared != null) {
eq_ = eq__shared;
} else {
eq_ = this.insert((EqualNode.create()));
if (eq_ == null) {
throw new IllegalStateException("Specialization 'opendir(EmulatedPosixSupport, Object, EqualNode, FromJavaStringNode)' contains a shared cache with name 'eqNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.eq == null) {
VarHandle.storeStoreFence();
this.eq = eq_;
}
FromJavaStringNode js2ts_;
FromJavaStringNode js2ts__shared = this.js2ts;
if (js2ts__shared != null) {
js2ts_ = js2ts__shared;
} else {
js2ts_ = this.insert((FromJavaStringNode.create()));
if (js2ts_ == null) {
throw new IllegalStateException("Specialization 'opendir(EmulatedPosixSupport, Object, EqualNode, FromJavaStringNode)' contains a shared cache with name 'fromJavaStringNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.js2ts == null) {
VarHandle.storeStoreFence();
this.js2ts = js2ts_;
}
state_0 = state_0 | 0x1000000 /* add SpecializationActive[EmulatedPosixSupport.opendir(EmulatedPosixSupport, Object, EqualNode, FromJavaStringNode)] */;
this.state_0_ = state_0;
return arg0Value.opendir(arg1Value, eq_, js2ts_);
}
/**
* Debug Info:
* Specialization {@link EmulatedPosixSupport#fdopendir(EmulatedPosixSupport, int, Node, InlinedBranchProfile, EqualNode, FromJavaStringNode)}
* Activation probability: 0.01961
* With/without class size: 4/0 bytes
*
*/
@Override
public Object fdopendir(Object arg0Value_, int arg1Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
int state_0 = this.state_0_;
if ((state_0 & 0x2000000) != 0 /* is SpecializationActive[EmulatedPosixSupport.fdopendir(EmulatedPosixSupport, int, Node, InlinedBranchProfile, EqualNode, FromJavaStringNode)] */) {
{
EqualNode eq_ = this.eq;
if (eq_ != null) {
FromJavaStringNode js2ts_ = this.js2ts;
if (js2ts_ != null) {
Node inliningTarget__ = (this);
return arg0Value.fdopendir(arg1Value, inliningTarget__, INLINED_ERROR_BRANCH, eq_, js2ts_);
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return fdopendirNode_AndSpecialize(arg0Value, arg1Value);
}
private Object fdopendirNode_AndSpecialize(EmulatedPosixSupport arg0Value, int arg1Value) throws PosixException {
int state_0 = this.state_0_;
{
Node inliningTarget__ = null;
inliningTarget__ = (this);
EqualNode eq_;
EqualNode eq__shared = this.eq;
if (eq__shared != null) {
eq_ = eq__shared;
} else {
eq_ = this.insert((EqualNode.create()));
if (eq_ == null) {
throw new IllegalStateException("Specialization 'fdopendir(EmulatedPosixSupport, int, Node, InlinedBranchProfile, EqualNode, FromJavaStringNode)' contains a shared cache with name 'eqNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.eq == null) {
VarHandle.storeStoreFence();
this.eq = eq_;
}
FromJavaStringNode js2ts_;
FromJavaStringNode js2ts__shared = this.js2ts;
if (js2ts__shared != null) {
js2ts_ = js2ts__shared;
} else {
js2ts_ = this.insert((FromJavaStringNode.create()));
if (js2ts_ == null) {
throw new IllegalStateException("Specialization 'fdopendir(EmulatedPosixSupport, int, Node, InlinedBranchProfile, EqualNode, FromJavaStringNode)' contains a shared cache with name 'fromJavaStringNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.js2ts == null) {
VarHandle.storeStoreFence();
this.js2ts = js2ts_;
}
state_0 = state_0 | 0x2000000 /* add SpecializationActive[EmulatedPosixSupport.fdopendir(EmulatedPosixSupport, int, Node, InlinedBranchProfile, EqualNode, FromJavaStringNode)] */;
this.state_0_ = state_0;
return arg0Value.fdopendir(arg1Value, inliningTarget__, INLINED_ERROR_BRANCH, eq_, js2ts_);
}
}
/**
* Debug Info:
* Specialization {@link EmulatedPosixSupport#closedir(EmulatedPosixSupport, Object, EqualNode)}
* Activation probability: 0.01961
* With/without class size: 4/0 bytes
*
*/
@Override
public void closedir(Object arg0Value_, Object arg1Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
int state_0 = this.state_0_;
if ((state_0 & 0x4000000) != 0 /* is SpecializationActive[EmulatedPosixSupport.closedir(EmulatedPosixSupport, Object, EqualNode)] */) {
{
EqualNode eq_ = this.eq;
if (eq_ != null) {
arg0Value.closedir(arg1Value, eq_);
return;
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
closedirNode_AndSpecialize(arg0Value, arg1Value);
return;
}
private void closedirNode_AndSpecialize(EmulatedPosixSupport arg0Value, Object arg1Value) throws PosixException {
int state_0 = this.state_0_;
EqualNode eq_;
EqualNode eq__shared = this.eq;
if (eq__shared != null) {
eq_ = eq__shared;
} else {
eq_ = this.insert((EqualNode.create()));
if (eq_ == null) {
throw new IllegalStateException("Specialization 'closedir(EmulatedPosixSupport, Object, EqualNode)' contains a shared cache with name 'eqNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.eq == null) {
VarHandle.storeStoreFence();
this.eq = eq_;
}
state_0 = state_0 | 0x4000000 /* add SpecializationActive[EmulatedPosixSupport.closedir(EmulatedPosixSupport, Object, EqualNode)] */;
this.state_0_ = state_0;
arg0Value.closedir(arg1Value, eq_);
return;
}
/**
* Debug Info:
* Specialization {@link EmulatedPosixSupport#readdir(EmulatedPosixSupport, Object, EqualNode)}
* Activation probability: 0.01961
* With/without class size: 4/0 bytes
*
*/
@Override
public Object readdir(Object arg0Value_, Object arg1Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
int state_0 = this.state_0_;
if ((state_0 & 0x8000000) != 0 /* is SpecializationActive[EmulatedPosixSupport.readdir(EmulatedPosixSupport, Object, EqualNode)] */) {
{
EqualNode eq_ = this.eq;
if (eq_ != null) {
return arg0Value.readdir(arg1Value, eq_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return readdirNode_AndSpecialize(arg0Value, arg1Value);
}
private Object readdirNode_AndSpecialize(EmulatedPosixSupport arg0Value, Object arg1Value) throws PosixException {
int state_0 = this.state_0_;
EqualNode eq_;
EqualNode eq__shared = this.eq;
if (eq__shared != null) {
eq_ = eq__shared;
} else {
eq_ = this.insert((EqualNode.create()));
if (eq_ == null) {
throw new IllegalStateException("Specialization 'readdir(EmulatedPosixSupport, Object, EqualNode)' contains a shared cache with name 'eqNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.eq == null) {
VarHandle.storeStoreFence();
this.eq = eq_;
}
state_0 = state_0 | 0x8000000 /* add SpecializationActive[EmulatedPosixSupport.readdir(EmulatedPosixSupport, Object, EqualNode)] */;
this.state_0_ = state_0;
return arg0Value.readdir(arg1Value, eq_);
}
@Override
public void rewinddir(Object receiver, Object dirStream) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
(((EmulatedPosixSupport) receiver)).rewinddir(dirStream);
return;
}
@Override
public Object dirEntryGetName(Object receiver, Object dirEntry) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
return (((EmulatedPosixSupport) receiver)).dirEntryGetName(dirEntry);
}
/**
* Debug Info:
* Specialization {@link EmulatedPosixSupport#dirEntryGetPath(EmulatedPosixSupport, Object, Object, FromJavaStringNode)}
* Activation probability: 0.01961
* With/without class size: 4/0 bytes
*
*/
@Override
public Object dirEntryGetPath(Object arg0Value_, Object arg1Value, Object arg2Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
int state_0 = this.state_0_;
if ((state_0 & 0x10000000) != 0 /* is SpecializationActive[EmulatedPosixSupport.dirEntryGetPath(EmulatedPosixSupport, Object, Object, FromJavaStringNode)] */) {
{
FromJavaStringNode js2ts_ = this.js2ts;
if (js2ts_ != null) {
return arg0Value.dirEntryGetPath(arg1Value, arg2Value, js2ts_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return dirEntryGetPathNode_AndSpecialize(arg0Value, arg1Value, arg2Value);
}
private Object dirEntryGetPathNode_AndSpecialize(EmulatedPosixSupport arg0Value, Object arg1Value, Object arg2Value) {
int state_0 = this.state_0_;
FromJavaStringNode js2ts_;
FromJavaStringNode js2ts__shared = this.js2ts;
if (js2ts__shared != null) {
js2ts_ = js2ts__shared;
} else {
js2ts_ = this.insert((FromJavaStringNode.create()));
if (js2ts_ == null) {
throw new IllegalStateException("Specialization 'dirEntryGetPath(EmulatedPosixSupport, Object, Object, FromJavaStringNode)' contains a shared cache with name 'fromJavaStringNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.js2ts == null) {
VarHandle.storeStoreFence();
this.js2ts = js2ts_;
}
state_0 = state_0 | 0x10000000 /* add SpecializationActive[EmulatedPosixSupport.dirEntryGetPath(EmulatedPosixSupport, Object, Object, FromJavaStringNode)] */;
this.state_0_ = state_0;
return arg0Value.dirEntryGetPath(arg1Value, arg2Value, js2ts_);
}
/**
* Debug Info:
* Specialization {@link EmulatedPosixSupport#dirEntryGetInode(EmulatedPosixSupport, Object, EqualNode)}
* Activation probability: 0.01961
* With/without class size: 4/0 bytes
*
*/
@Override
public long dirEntryGetInode(Object arg0Value_, Object arg1Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
int state_0 = this.state_0_;
if ((state_0 & 0x20000000) != 0 /* is SpecializationActive[EmulatedPosixSupport.dirEntryGetInode(EmulatedPosixSupport, Object, EqualNode)] */) {
{
EqualNode eq_ = this.eq;
if (eq_ != null) {
return arg0Value.dirEntryGetInode(arg1Value, eq_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return dirEntryGetInodeNode_AndSpecialize(arg0Value, arg1Value);
}
private long dirEntryGetInodeNode_AndSpecialize(EmulatedPosixSupport arg0Value, Object arg1Value) throws PosixException {
int state_0 = this.state_0_;
EqualNode eq_;
EqualNode eq__shared = this.eq;
if (eq__shared != null) {
eq_ = eq__shared;
} else {
eq_ = this.insert((EqualNode.create()));
if (eq_ == null) {
throw new IllegalStateException("Specialization 'dirEntryGetInode(EmulatedPosixSupport, Object, EqualNode)' contains a shared cache with name 'eqNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.eq == null) {
VarHandle.storeStoreFence();
this.eq = eq_;
}
state_0 = state_0 | 0x20000000 /* add SpecializationActive[EmulatedPosixSupport.dirEntryGetInode(EmulatedPosixSupport, Object, EqualNode)] */;
this.state_0_ = state_0;
return arg0Value.dirEntryGetInode(arg1Value, eq_);
}
@Override
public int dirEntryGetType(Object receiver, Object dirEntry) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
return (((EmulatedPosixSupport) receiver)).dirEntryGetType(dirEntry);
}
/**
* Debug Info:
* Specialization {@link EmulatedPosixSupport#utimensat(EmulatedPosixSupport, int, Object, long[], boolean, Node, SetUTimeNode, InlinedConditionProfile, EqualNode, FromJavaStringNode, ToJavaStringNode)}
* Activation probability: 0.01961
* With/without class size: 4/0 bytes
*
*/
@Override
public void utimensat(Object arg0Value_, int arg1Value, Object arg2Value, long[] arg3Value, boolean arg4Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
int state_0 = this.state_0_;
if ((state_0 & 0x40000000) != 0 /* is SpecializationActive[EmulatedPosixSupport.utimensat(EmulatedPosixSupport, int, Object, long[], boolean, Node, SetUTimeNode, InlinedConditionProfile, EqualNode, FromJavaStringNode, ToJavaStringNode)] */) {
{
EqualNode eq_ = this.eq;
if (eq_ != null) {
FromJavaStringNode js2ts_ = this.js2ts;
if (js2ts_ != null) {
ToJavaStringNode ts2js_ = this.ts2js;
if (ts2js_ != null) {
Node inliningTarget__ = (this);
arg0Value.utimensat(arg1Value, arg2Value, arg3Value, arg4Value, inliningTarget__, INLINED_SET_U_TIME, INLINED_DEFAULT_DIR_PROFILE, eq_, js2ts_, ts2js_);
return;
}
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
utimensatNode_AndSpecialize(arg0Value, arg1Value, arg2Value, arg3Value, arg4Value);
return;
}
private void utimensatNode_AndSpecialize(EmulatedPosixSupport arg0Value, int arg1Value, Object arg2Value, long[] arg3Value, boolean arg4Value) throws PosixException {
int state_0 = this.state_0_;
{
Node inliningTarget__ = null;
inliningTarget__ = (this);
EqualNode eq_;
EqualNode eq__shared = this.eq;
if (eq__shared != null) {
eq_ = eq__shared;
} else {
eq_ = this.insert((EqualNode.create()));
if (eq_ == null) {
throw new IllegalStateException("Specialization 'utimensat(EmulatedPosixSupport, int, Object, long[], boolean, Node, SetUTimeNode, InlinedConditionProfile, EqualNode, FromJavaStringNode, ToJavaStringNode)' contains a shared cache with name 'eqNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.eq == null) {
VarHandle.storeStoreFence();
this.eq = eq_;
}
FromJavaStringNode js2ts_;
FromJavaStringNode js2ts__shared = this.js2ts;
if (js2ts__shared != null) {
js2ts_ = js2ts__shared;
} else {
js2ts_ = this.insert((FromJavaStringNode.create()));
if (js2ts_ == null) {
throw new IllegalStateException("Specialization 'utimensat(EmulatedPosixSupport, int, Object, long[], boolean, Node, SetUTimeNode, InlinedConditionProfile, EqualNode, FromJavaStringNode, ToJavaStringNode)' contains a shared cache with name 'fromJavaStringNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.js2ts == null) {
VarHandle.storeStoreFence();
this.js2ts = js2ts_;
}
ToJavaStringNode ts2js_;
ToJavaStringNode ts2js__shared = this.ts2js;
if (ts2js__shared != null) {
ts2js_ = ts2js__shared;
} else {
ts2js_ = this.insert((ToJavaStringNode.create()));
if (ts2js_ == null) {
throw new IllegalStateException("Specialization 'utimensat(EmulatedPosixSupport, int, Object, long[], boolean, Node, SetUTimeNode, InlinedConditionProfile, EqualNode, FromJavaStringNode, ToJavaStringNode)' contains a shared cache with name 'toJavaStringNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.ts2js == null) {
VarHandle.storeStoreFence();
this.ts2js = ts2js_;
}
state_0 = state_0 | 0x40000000 /* add SpecializationActive[EmulatedPosixSupport.utimensat(EmulatedPosixSupport, int, Object, long[], boolean, Node, SetUTimeNode, InlinedConditionProfile, EqualNode, FromJavaStringNode, ToJavaStringNode)] */;
this.state_0_ = state_0;
arg0Value.utimensat(arg1Value, arg2Value, arg3Value, arg4Value, inliningTarget__, INLINED_SET_U_TIME, INLINED_DEFAULT_DIR_PROFILE, eq_, js2ts_, ts2js_);
return;
}
}
/**
* Debug Info:
* Specialization {@link EmulatedPosixSupport#futimens(EmulatedPosixSupport, int, long[], Node, SetUTimeNode, EqualNode, FromJavaStringNode)}
* Activation probability: 0.01961
* With/without class size: 4/0 bytes
*
*/
@Override
public void futimens(Object arg0Value_, int arg1Value, long[] arg2Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
int state_0 = this.state_0_;
if ((state_0 & 0x80000000) != 0 /* is SpecializationActive[EmulatedPosixSupport.futimens(EmulatedPosixSupport, int, long[], Node, SetUTimeNode, EqualNode, FromJavaStringNode)] */) {
{
EqualNode eq_ = this.eq;
if (eq_ != null) {
FromJavaStringNode js2ts_ = this.js2ts;
if (js2ts_ != null) {
Node inliningTarget__ = (this);
arg0Value.futimens(arg1Value, arg2Value, inliningTarget__, INLINED_SET_U_TIME, eq_, js2ts_);
return;
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
futimensNode_AndSpecialize(arg0Value, arg1Value, arg2Value);
return;
}
private void futimensNode_AndSpecialize(EmulatedPosixSupport arg0Value, int arg1Value, long[] arg2Value) throws PosixException {
int state_0 = this.state_0_;
{
Node inliningTarget__ = null;
inliningTarget__ = (this);
EqualNode eq_;
EqualNode eq__shared = this.eq;
if (eq__shared != null) {
eq_ = eq__shared;
} else {
eq_ = this.insert((EqualNode.create()));
if (eq_ == null) {
throw new IllegalStateException("Specialization 'futimens(EmulatedPosixSupport, int, long[], Node, SetUTimeNode, EqualNode, FromJavaStringNode)' contains a shared cache with name 'eqNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.eq == null) {
VarHandle.storeStoreFence();
this.eq = eq_;
}
FromJavaStringNode js2ts_;
FromJavaStringNode js2ts__shared = this.js2ts;
if (js2ts__shared != null) {
js2ts_ = js2ts__shared;
} else {
js2ts_ = this.insert((FromJavaStringNode.create()));
if (js2ts_ == null) {
throw new IllegalStateException("Specialization 'futimens(EmulatedPosixSupport, int, long[], Node, SetUTimeNode, EqualNode, FromJavaStringNode)' contains a shared cache with name 'fromJavaStringNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.js2ts == null) {
VarHandle.storeStoreFence();
this.js2ts = js2ts_;
}
state_0 = state_0 | 0x80000000 /* add SpecializationActive[EmulatedPosixSupport.futimens(EmulatedPosixSupport, int, long[], Node, SetUTimeNode, EqualNode, FromJavaStringNode)] */;
this.state_0_ = state_0;
arg0Value.futimens(arg1Value, arg2Value, inliningTarget__, INLINED_SET_U_TIME, eq_, js2ts_);
return;
}
}
/**
* Debug Info:
* Specialization {@link EmulatedPosixSupport#futimes(EmulatedPosixSupport, int, Timeval[], Node, SetUTimeNode, EqualNode, FromJavaStringNode)}
* Activation probability: 0.01961
* With/without class size: 4/0 bytes
*
*/
@Override
public void futimes(Object arg0Value_, int arg1Value, Timeval[] arg2Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
int state_1 = this.state_1_;
if ((state_1 & 0b1) != 0 /* is SpecializationActive[EmulatedPosixSupport.futimes(EmulatedPosixSupport, int, Timeval[], Node, SetUTimeNode, EqualNode, FromJavaStringNode)] */) {
{
EqualNode eq_ = this.eq;
if (eq_ != null) {
FromJavaStringNode js2ts_ = this.js2ts;
if (js2ts_ != null) {
Node inliningTarget__ = (this);
arg0Value.futimes(arg1Value, arg2Value, inliningTarget__, INLINED_SET_U_TIME, eq_, js2ts_);
return;
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
futimesNode_AndSpecialize(arg0Value, arg1Value, arg2Value);
return;
}
private void futimesNode_AndSpecialize(EmulatedPosixSupport arg0Value, int arg1Value, Timeval[] arg2Value) throws PosixException {
int state_1 = this.state_1_;
{
Node inliningTarget__ = null;
inliningTarget__ = (this);
EqualNode eq_;
EqualNode eq__shared = this.eq;
if (eq__shared != null) {
eq_ = eq__shared;
} else {
eq_ = this.insert((EqualNode.create()));
if (eq_ == null) {
throw new IllegalStateException("Specialization 'futimes(EmulatedPosixSupport, int, Timeval[], Node, SetUTimeNode, EqualNode, FromJavaStringNode)' contains a shared cache with name 'eqNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.eq == null) {
VarHandle.storeStoreFence();
this.eq = eq_;
}
FromJavaStringNode js2ts_;
FromJavaStringNode js2ts__shared = this.js2ts;
if (js2ts__shared != null) {
js2ts_ = js2ts__shared;
} else {
js2ts_ = this.insert((FromJavaStringNode.create()));
if (js2ts_ == null) {
throw new IllegalStateException("Specialization 'futimes(EmulatedPosixSupport, int, Timeval[], Node, SetUTimeNode, EqualNode, FromJavaStringNode)' contains a shared cache with name 'fromJavaStringNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.js2ts == null) {
VarHandle.storeStoreFence();
this.js2ts = js2ts_;
}
state_1 = state_1 | 0b1 /* add SpecializationActive[EmulatedPosixSupport.futimes(EmulatedPosixSupport, int, Timeval[], Node, SetUTimeNode, EqualNode, FromJavaStringNode)] */;
this.state_1_ = state_1;
arg0Value.futimes(arg1Value, arg2Value, inliningTarget__, INLINED_SET_U_TIME, eq_, js2ts_);
return;
}
}
/**
* Debug Info:
* Specialization {@link EmulatedPosixSupport#lutimes(EmulatedPosixSupport, Object, Timeval[], Node, SetUTimeNode, EqualNode, FromJavaStringNode)}
* Activation probability: 0.01961
* With/without class size: 4/0 bytes
*
*/
@Override
public void lutimes(Object arg0Value_, Object arg1Value, Timeval[] arg2Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
int state_1 = this.state_1_;
if ((state_1 & 0b10) != 0 /* is SpecializationActive[EmulatedPosixSupport.lutimes(EmulatedPosixSupport, Object, Timeval[], Node, SetUTimeNode, EqualNode, FromJavaStringNode)] */) {
{
EqualNode eq_ = this.eq;
if (eq_ != null) {
FromJavaStringNode js2ts_ = this.js2ts;
if (js2ts_ != null) {
Node inliningTarget__ = (this);
arg0Value.lutimes(arg1Value, arg2Value, inliningTarget__, INLINED_SET_U_TIME, eq_, js2ts_);
return;
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
lutimesNode_AndSpecialize(arg0Value, arg1Value, arg2Value);
return;
}
private void lutimesNode_AndSpecialize(EmulatedPosixSupport arg0Value, Object arg1Value, Timeval[] arg2Value) throws PosixException {
int state_1 = this.state_1_;
{
Node inliningTarget__ = null;
inliningTarget__ = (this);
EqualNode eq_;
EqualNode eq__shared = this.eq;
if (eq__shared != null) {
eq_ = eq__shared;
} else {
eq_ = this.insert((EqualNode.create()));
if (eq_ == null) {
throw new IllegalStateException("Specialization 'lutimes(EmulatedPosixSupport, Object, Timeval[], Node, SetUTimeNode, EqualNode, FromJavaStringNode)' contains a shared cache with name 'eqNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.eq == null) {
VarHandle.storeStoreFence();
this.eq = eq_;
}
FromJavaStringNode js2ts_;
FromJavaStringNode js2ts__shared = this.js2ts;
if (js2ts__shared != null) {
js2ts_ = js2ts__shared;
} else {
js2ts_ = this.insert((FromJavaStringNode.create()));
if (js2ts_ == null) {
throw new IllegalStateException("Specialization 'lutimes(EmulatedPosixSupport, Object, Timeval[], Node, SetUTimeNode, EqualNode, FromJavaStringNode)' contains a shared cache with name 'fromJavaStringNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.js2ts == null) {
VarHandle.storeStoreFence();
this.js2ts = js2ts_;
}
state_1 = state_1 | 0b10 /* add SpecializationActive[EmulatedPosixSupport.lutimes(EmulatedPosixSupport, Object, Timeval[], Node, SetUTimeNode, EqualNode, FromJavaStringNode)] */;
this.state_1_ = state_1;
arg0Value.lutimes(arg1Value, arg2Value, inliningTarget__, INLINED_SET_U_TIME, eq_, js2ts_);
return;
}
}
/**
* Debug Info:
* Specialization {@link EmulatedPosixSupport#utimes(EmulatedPosixSupport, Object, Timeval[], Node, SetUTimeNode, EqualNode, FromJavaStringNode)}
* Activation probability: 0.01961
* With/without class size: 4/0 bytes
*
*/
@Override
public void utimes(Object arg0Value_, Object arg1Value, Timeval[] arg2Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
int state_1 = this.state_1_;
if ((state_1 & 0b100) != 0 /* is SpecializationActive[EmulatedPosixSupport.utimes(EmulatedPosixSupport, Object, Timeval[], Node, SetUTimeNode, EqualNode, FromJavaStringNode)] */) {
{
EqualNode eq_ = this.eq;
if (eq_ != null) {
FromJavaStringNode js2ts_ = this.js2ts;
if (js2ts_ != null) {
Node inliningTarget__ = (this);
arg0Value.utimes(arg1Value, arg2Value, inliningTarget__, INLINED_SET_U_TIME, eq_, js2ts_);
return;
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
utimesNode_AndSpecialize(arg0Value, arg1Value, arg2Value);
return;
}
private void utimesNode_AndSpecialize(EmulatedPosixSupport arg0Value, Object arg1Value, Timeval[] arg2Value) throws PosixException {
int state_1 = this.state_1_;
{
Node inliningTarget__ = null;
inliningTarget__ = (this);
EqualNode eq_;
EqualNode eq__shared = this.eq;
if (eq__shared != null) {
eq_ = eq__shared;
} else {
eq_ = this.insert((EqualNode.create()));
if (eq_ == null) {
throw new IllegalStateException("Specialization 'utimes(EmulatedPosixSupport, Object, Timeval[], Node, SetUTimeNode, EqualNode, FromJavaStringNode)' contains a shared cache with name 'eqNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.eq == null) {
VarHandle.storeStoreFence();
this.eq = eq_;
}
FromJavaStringNode js2ts_;
FromJavaStringNode js2ts__shared = this.js2ts;
if (js2ts__shared != null) {
js2ts_ = js2ts__shared;
} else {
js2ts_ = this.insert((FromJavaStringNode.create()));
if (js2ts_ == null) {
throw new IllegalStateException("Specialization 'utimes(EmulatedPosixSupport, Object, Timeval[], Node, SetUTimeNode, EqualNode, FromJavaStringNode)' contains a shared cache with name 'fromJavaStringNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.js2ts == null) {
VarHandle.storeStoreFence();
this.js2ts = js2ts_;
}
state_1 = state_1 | 0b100 /* add SpecializationActive[EmulatedPosixSupport.utimes(EmulatedPosixSupport, Object, Timeval[], Node, SetUTimeNode, EqualNode, FromJavaStringNode)] */;
this.state_1_ = state_1;
arg0Value.utimes(arg1Value, arg2Value, inliningTarget__, INLINED_SET_U_TIME, eq_, js2ts_);
return;
}
}
/**
* Debug Info:
* Specialization {@link EmulatedPosixSupport#renameat(EmulatedPosixSupport, int, Object, int, Object, Node, InlinedConditionProfile, EqualNode, FromJavaStringNode, ToJavaStringNode)}
* Activation probability: 0.01961
* With/without class size: 4/0 bytes
*
*/
@Override
public void renameat(Object arg0Value_, int arg1Value, Object arg2Value, int arg3Value, Object arg4Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
int state_1 = this.state_1_;
if ((state_1 & 0b1000) != 0 /* is SpecializationActive[EmulatedPosixSupport.renameat(EmulatedPosixSupport, int, Object, int, Object, Node, InlinedConditionProfile, EqualNode, FromJavaStringNode, ToJavaStringNode)] */) {
{
EqualNode eq_ = this.eq;
if (eq_ != null) {
FromJavaStringNode js2ts_ = this.js2ts;
if (js2ts_ != null) {
ToJavaStringNode ts2js_ = this.ts2js;
if (ts2js_ != null) {
Node inliningTarget__ = (this);
arg0Value.renameat(arg1Value, arg2Value, arg3Value, arg4Value, inliningTarget__, INLINED_DEFAULT_DIR_PROFILE, eq_, js2ts_, ts2js_);
return;
}
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
renameatNode_AndSpecialize(arg0Value, arg1Value, arg2Value, arg3Value, arg4Value);
return;
}
private void renameatNode_AndSpecialize(EmulatedPosixSupport arg0Value, int arg1Value, Object arg2Value, int arg3Value, Object arg4Value) throws PosixException {
int state_1 = this.state_1_;
{
Node inliningTarget__ = null;
inliningTarget__ = (this);
EqualNode eq_;
EqualNode eq__shared = this.eq;
if (eq__shared != null) {
eq_ = eq__shared;
} else {
eq_ = this.insert((EqualNode.create()));
if (eq_ == null) {
throw new IllegalStateException("Specialization 'renameat(EmulatedPosixSupport, int, Object, int, Object, Node, InlinedConditionProfile, EqualNode, FromJavaStringNode, ToJavaStringNode)' contains a shared cache with name 'eqNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.eq == null) {
VarHandle.storeStoreFence();
this.eq = eq_;
}
FromJavaStringNode js2ts_;
FromJavaStringNode js2ts__shared = this.js2ts;
if (js2ts__shared != null) {
js2ts_ = js2ts__shared;
} else {
js2ts_ = this.insert((FromJavaStringNode.create()));
if (js2ts_ == null) {
throw new IllegalStateException("Specialization 'renameat(EmulatedPosixSupport, int, Object, int, Object, Node, InlinedConditionProfile, EqualNode, FromJavaStringNode, ToJavaStringNode)' contains a shared cache with name 'fromJavaStringNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.js2ts == null) {
VarHandle.storeStoreFence();
this.js2ts = js2ts_;
}
ToJavaStringNode ts2js_;
ToJavaStringNode ts2js__shared = this.ts2js;
if (ts2js__shared != null) {
ts2js_ = ts2js__shared;
} else {
ts2js_ = this.insert((ToJavaStringNode.create()));
if (ts2js_ == null) {
throw new IllegalStateException("Specialization 'renameat(EmulatedPosixSupport, int, Object, int, Object, Node, InlinedConditionProfile, EqualNode, FromJavaStringNode, ToJavaStringNode)' contains a shared cache with name 'toJavaStringNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.ts2js == null) {
VarHandle.storeStoreFence();
this.ts2js = ts2js_;
}
state_1 = state_1 | 0b1000 /* add SpecializationActive[EmulatedPosixSupport.renameat(EmulatedPosixSupport, int, Object, int, Object, Node, InlinedConditionProfile, EqualNode, FromJavaStringNode, ToJavaStringNode)] */;
this.state_1_ = state_1;
arg0Value.renameat(arg1Value, arg2Value, arg3Value, arg4Value, inliningTarget__, INLINED_DEFAULT_DIR_PROFILE, eq_, js2ts_, ts2js_);
return;
}
}
/**
* Debug Info:
* Specialization {@link EmulatedPosixSupport#faccessat(EmulatedPosixSupport, int, Object, int, boolean, boolean, Node, InlinedBranchProfile, InlinedConditionProfile, EqualNode, FromJavaStringNode, ToJavaStringNode)}
* Activation probability: 0.01961
* With/without class size: 4/0 bytes
*
*/
@Override
public boolean faccessat(Object arg0Value_, int arg1Value, Object arg2Value, int arg3Value, boolean arg4Value, boolean arg5Value) {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
int state_1 = this.state_1_;
if ((state_1 & 0b10000) != 0 /* is SpecializationActive[EmulatedPosixSupport.faccessat(EmulatedPosixSupport, int, Object, int, boolean, boolean, Node, InlinedBranchProfile, InlinedConditionProfile, EqualNode, FromJavaStringNode, ToJavaStringNode)] */) {
{
EqualNode eq_ = this.eq;
if (eq_ != null) {
FromJavaStringNode js2ts_ = this.js2ts;
if (js2ts_ != null) {
ToJavaStringNode ts2js_ = this.ts2js;
if (ts2js_ != null) {
Node inliningTarget__ = (this);
return arg0Value.faccessat(arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, inliningTarget__, INLINED_ERROR_BRANCH, INLINED_DEFAULT_DIR_PROFILE, eq_, js2ts_, ts2js_);
}
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return faccessatNode_AndSpecialize(arg0Value, arg1Value, arg2Value, arg3Value, arg4Value, arg5Value);
}
private boolean faccessatNode_AndSpecialize(EmulatedPosixSupport arg0Value, int arg1Value, Object arg2Value, int arg3Value, boolean arg4Value, boolean arg5Value) {
int state_1 = this.state_1_;
{
Node inliningTarget__ = null;
inliningTarget__ = (this);
EqualNode eq_;
EqualNode eq__shared = this.eq;
if (eq__shared != null) {
eq_ = eq__shared;
} else {
eq_ = this.insert((EqualNode.create()));
if (eq_ == null) {
throw new IllegalStateException("Specialization 'faccessat(EmulatedPosixSupport, int, Object, int, boolean, boolean, Node, InlinedBranchProfile, InlinedConditionProfile, EqualNode, FromJavaStringNode, ToJavaStringNode)' contains a shared cache with name 'eqNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.eq == null) {
VarHandle.storeStoreFence();
this.eq = eq_;
}
FromJavaStringNode js2ts_;
FromJavaStringNode js2ts__shared = this.js2ts;
if (js2ts__shared != null) {
js2ts_ = js2ts__shared;
} else {
js2ts_ = this.insert((FromJavaStringNode.create()));
if (js2ts_ == null) {
throw new IllegalStateException("Specialization 'faccessat(EmulatedPosixSupport, int, Object, int, boolean, boolean, Node, InlinedBranchProfile, InlinedConditionProfile, EqualNode, FromJavaStringNode, ToJavaStringNode)' contains a shared cache with name 'fromJavaStringNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.js2ts == null) {
VarHandle.storeStoreFence();
this.js2ts = js2ts_;
}
ToJavaStringNode ts2js_;
ToJavaStringNode ts2js__shared = this.ts2js;
if (ts2js__shared != null) {
ts2js_ = ts2js__shared;
} else {
ts2js_ = this.insert((ToJavaStringNode.create()));
if (ts2js_ == null) {
throw new IllegalStateException("Specialization 'faccessat(EmulatedPosixSupport, int, Object, int, boolean, boolean, Node, InlinedBranchProfile, InlinedConditionProfile, EqualNode, FromJavaStringNode, ToJavaStringNode)' contains a shared cache with name 'toJavaStringNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.ts2js == null) {
VarHandle.storeStoreFence();
this.ts2js = ts2js_;
}
state_1 = state_1 | 0b10000 /* add SpecializationActive[EmulatedPosixSupport.faccessat(EmulatedPosixSupport, int, Object, int, boolean, boolean, Node, InlinedBranchProfile, InlinedConditionProfile, EqualNode, FromJavaStringNode, ToJavaStringNode)] */;
this.state_1_ = state_1;
return arg0Value.faccessat(arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, inliningTarget__, INLINED_ERROR_BRANCH, INLINED_DEFAULT_DIR_PROFILE, eq_, js2ts_, ts2js_);
}
}
/**
* Debug Info:
* Specialization {@link EmulatedPosixSupport#fchmodat(EmulatedPosixSupport, int, Object, int, boolean, Node, InlinedConditionProfile, EqualNode, FromJavaStringNode, ToJavaStringNode)}
* Activation probability: 0.01961
* With/without class size: 4/0 bytes
*
*/
@Override
public void fchmodat(Object arg0Value_, int arg1Value, Object arg2Value, int arg3Value, boolean arg4Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
int state_1 = this.state_1_;
if ((state_1 & 0b100000) != 0 /* is SpecializationActive[EmulatedPosixSupport.fchmodat(EmulatedPosixSupport, int, Object, int, boolean, Node, InlinedConditionProfile, EqualNode, FromJavaStringNode, ToJavaStringNode)] */) {
{
EqualNode eq_ = this.eq;
if (eq_ != null) {
FromJavaStringNode js2ts_ = this.js2ts;
if (js2ts_ != null) {
ToJavaStringNode ts2js_ = this.ts2js;
if (ts2js_ != null) {
Node inliningTarget__ = (this);
arg0Value.fchmodat(arg1Value, arg2Value, arg3Value, arg4Value, inliningTarget__, INLINED_DEFAULT_DIR_PROFILE, eq_, js2ts_, ts2js_);
return;
}
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
fchmodatNode_AndSpecialize(arg0Value, arg1Value, arg2Value, arg3Value, arg4Value);
return;
}
private void fchmodatNode_AndSpecialize(EmulatedPosixSupport arg0Value, int arg1Value, Object arg2Value, int arg3Value, boolean arg4Value) throws PosixException {
int state_1 = this.state_1_;
{
Node inliningTarget__ = null;
inliningTarget__ = (this);
EqualNode eq_;
EqualNode eq__shared = this.eq;
if (eq__shared != null) {
eq_ = eq__shared;
} else {
eq_ = this.insert((EqualNode.create()));
if (eq_ == null) {
throw new IllegalStateException("Specialization 'fchmodat(EmulatedPosixSupport, int, Object, int, boolean, Node, InlinedConditionProfile, EqualNode, FromJavaStringNode, ToJavaStringNode)' contains a shared cache with name 'eqNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.eq == null) {
VarHandle.storeStoreFence();
this.eq = eq_;
}
FromJavaStringNode js2ts_;
FromJavaStringNode js2ts__shared = this.js2ts;
if (js2ts__shared != null) {
js2ts_ = js2ts__shared;
} else {
js2ts_ = this.insert((FromJavaStringNode.create()));
if (js2ts_ == null) {
throw new IllegalStateException("Specialization 'fchmodat(EmulatedPosixSupport, int, Object, int, boolean, Node, InlinedConditionProfile, EqualNode, FromJavaStringNode, ToJavaStringNode)' contains a shared cache with name 'fromJavaStringNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.js2ts == null) {
VarHandle.storeStoreFence();
this.js2ts = js2ts_;
}
ToJavaStringNode ts2js_;
ToJavaStringNode ts2js__shared = this.ts2js;
if (ts2js__shared != null) {
ts2js_ = ts2js__shared;
} else {
ts2js_ = this.insert((ToJavaStringNode.create()));
if (ts2js_ == null) {
throw new IllegalStateException("Specialization 'fchmodat(EmulatedPosixSupport, int, Object, int, boolean, Node, InlinedConditionProfile, EqualNode, FromJavaStringNode, ToJavaStringNode)' contains a shared cache with name 'toJavaStringNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.ts2js == null) {
VarHandle.storeStoreFence();
this.ts2js = ts2js_;
}
state_1 = state_1 | 0b100000 /* add SpecializationActive[EmulatedPosixSupport.fchmodat(EmulatedPosixSupport, int, Object, int, boolean, Node, InlinedConditionProfile, EqualNode, FromJavaStringNode, ToJavaStringNode)] */;
this.state_1_ = state_1;
arg0Value.fchmodat(arg1Value, arg2Value, arg3Value, arg4Value, inliningTarget__, INLINED_DEFAULT_DIR_PROFILE, eq_, js2ts_, ts2js_);
return;
}
}
/**
* Debug Info:
* Specialization {@link EmulatedPosixSupport#fchmod(EmulatedPosixSupport, int, int, EqualNode, FromJavaStringNode)}
* Activation probability: 0.01961
* With/without class size: 4/0 bytes
*
*/
@Override
public void fchmod(Object arg0Value_, int arg1Value, int arg2Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
int state_1 = this.state_1_;
if ((state_1 & 0b1000000) != 0 /* is SpecializationActive[EmulatedPosixSupport.fchmod(EmulatedPosixSupport, int, int, EqualNode, FromJavaStringNode)] */) {
{
EqualNode eq_ = this.eq;
if (eq_ != null) {
FromJavaStringNode js2ts_ = this.js2ts;
if (js2ts_ != null) {
arg0Value.fchmod(arg1Value, arg2Value, eq_, js2ts_);
return;
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
fchmodNode_AndSpecialize(arg0Value, arg1Value, arg2Value);
return;
}
private void fchmodNode_AndSpecialize(EmulatedPosixSupport arg0Value, int arg1Value, int arg2Value) throws PosixException {
int state_1 = this.state_1_;
EqualNode eq_;
EqualNode eq__shared = this.eq;
if (eq__shared != null) {
eq_ = eq__shared;
} else {
eq_ = this.insert((EqualNode.create()));
if (eq_ == null) {
throw new IllegalStateException("Specialization 'fchmod(EmulatedPosixSupport, int, int, EqualNode, FromJavaStringNode)' contains a shared cache with name 'eqNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.eq == null) {
VarHandle.storeStoreFence();
this.eq = eq_;
}
FromJavaStringNode js2ts_;
FromJavaStringNode js2ts__shared = this.js2ts;
if (js2ts__shared != null) {
js2ts_ = js2ts__shared;
} else {
js2ts_ = this.insert((FromJavaStringNode.create()));
if (js2ts_ == null) {
throw new IllegalStateException("Specialization 'fchmod(EmulatedPosixSupport, int, int, EqualNode, FromJavaStringNode)' contains a shared cache with name 'fromJavaStringNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.js2ts == null) {
VarHandle.storeStoreFence();
this.js2ts = js2ts_;
}
state_1 = state_1 | 0b1000000 /* add SpecializationActive[EmulatedPosixSupport.fchmod(EmulatedPosixSupport, int, int, EqualNode, FromJavaStringNode)] */;
this.state_1_ = state_1;
arg0Value.fchmod(arg1Value, arg2Value, eq_, js2ts_);
return;
}
/**
* Debug Info:
* Specialization {@link EmulatedPosixSupport#readlinkat(EmulatedPosixSupport, int, Object, Node, InlinedConditionProfile, EqualNode, FromJavaStringNode, ToJavaStringNode)}
* Activation probability: 0.01961
* With/without class size: 4/0 bytes
*
*/
@Override
public Object readlinkat(Object arg0Value_, int arg1Value, Object arg2Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
int state_1 = this.state_1_;
if ((state_1 & 0b10000000) != 0 /* is SpecializationActive[EmulatedPosixSupport.readlinkat(EmulatedPosixSupport, int, Object, Node, InlinedConditionProfile, EqualNode, FromJavaStringNode, ToJavaStringNode)] */) {
{
EqualNode eq_ = this.eq;
if (eq_ != null) {
FromJavaStringNode js2ts_ = this.js2ts;
if (js2ts_ != null) {
ToJavaStringNode ts2js_ = this.ts2js;
if (ts2js_ != null) {
Node inliningTarget__ = (this);
return arg0Value.readlinkat(arg1Value, arg2Value, inliningTarget__, INLINED_DEFAULT_DIR_PROFILE, eq_, js2ts_, ts2js_);
}
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return readlinkatNode_AndSpecialize(arg0Value, arg1Value, arg2Value);
}
private Object readlinkatNode_AndSpecialize(EmulatedPosixSupport arg0Value, int arg1Value, Object arg2Value) throws PosixException {
int state_1 = this.state_1_;
{
Node inliningTarget__ = null;
inliningTarget__ = (this);
EqualNode eq_;
EqualNode eq__shared = this.eq;
if (eq__shared != null) {
eq_ = eq__shared;
} else {
eq_ = this.insert((EqualNode.create()));
if (eq_ == null) {
throw new IllegalStateException("Specialization 'readlinkat(EmulatedPosixSupport, int, Object, Node, InlinedConditionProfile, EqualNode, FromJavaStringNode, ToJavaStringNode)' contains a shared cache with name 'eqNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.eq == null) {
VarHandle.storeStoreFence();
this.eq = eq_;
}
FromJavaStringNode js2ts_;
FromJavaStringNode js2ts__shared = this.js2ts;
if (js2ts__shared != null) {
js2ts_ = js2ts__shared;
} else {
js2ts_ = this.insert((FromJavaStringNode.create()));
if (js2ts_ == null) {
throw new IllegalStateException("Specialization 'readlinkat(EmulatedPosixSupport, int, Object, Node, InlinedConditionProfile, EqualNode, FromJavaStringNode, ToJavaStringNode)' contains a shared cache with name 'fromJavaStringNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.js2ts == null) {
VarHandle.storeStoreFence();
this.js2ts = js2ts_;
}
ToJavaStringNode ts2js_;
ToJavaStringNode ts2js__shared = this.ts2js;
if (ts2js__shared != null) {
ts2js_ = ts2js__shared;
} else {
ts2js_ = this.insert((ToJavaStringNode.create()));
if (ts2js_ == null) {
throw new IllegalStateException("Specialization 'readlinkat(EmulatedPosixSupport, int, Object, Node, InlinedConditionProfile, EqualNode, FromJavaStringNode, ToJavaStringNode)' contains a shared cache with name 'toJavaStringNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.ts2js == null) {
VarHandle.storeStoreFence();
this.ts2js = ts2js_;
}
state_1 = state_1 | 0b10000000 /* add SpecializationActive[EmulatedPosixSupport.readlinkat(EmulatedPosixSupport, int, Object, Node, InlinedConditionProfile, EqualNode, FromJavaStringNode, ToJavaStringNode)] */;
this.state_1_ = state_1;
return arg0Value.readlinkat(arg1Value, arg2Value, inliningTarget__, INLINED_DEFAULT_DIR_PROFILE, eq_, js2ts_, ts2js_);
}
}
/**
* Debug Info:
* Specialization {@link EmulatedPosixSupport#kill(EmulatedPosixSupport, long, int, ReadAttributeFromObjectNode, IsNode)}
* Activation probability: 0.01961
* With/without class size: 4/8 bytes
*
*/
@Override
public void kill(Object arg0Value_, long arg1Value, int arg2Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
int state_1 = this.state_1_;
if ((state_1 & 0b100000000) != 0 /* is SpecializationActive[EmulatedPosixSupport.kill(EmulatedPosixSupport, long, int, ReadAttributeFromObjectNode, IsNode)] */) {
KillNode_KillData s0_ = this.killNode__kill_cache;
if (s0_ != null) {
arg0Value.kill(arg1Value, arg2Value, s0_.readSignalNode_, s0_.isNode_);
return;
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
killNode_AndSpecialize(arg0Value, arg1Value, arg2Value);
return;
}
private void killNode_AndSpecialize(EmulatedPosixSupport arg0Value, long arg1Value, int arg2Value) throws PosixException {
int state_1 = this.state_1_;
KillNode_KillData s0_ = this.insert(new KillNode_KillData());
ReadAttributeFromObjectNode readSignalNode__ = s0_.insert((ReadAttributeFromObjectNode.create()));
Objects.requireNonNull(readSignalNode__, "Specialization 'kill(EmulatedPosixSupport, long, int, ReadAttributeFromObjectNode, IsNode)' cache 'readSignalNode' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s0_.readSignalNode_ = readSignalNode__;
IsNode isNode__ = s0_.insert((IsNode.create()));
Objects.requireNonNull(isNode__, "Specialization 'kill(EmulatedPosixSupport, long, int, ReadAttributeFromObjectNode, IsNode)' cache 'isNode' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s0_.isNode_ = isNode__;
VarHandle.storeStoreFence();
this.killNode__kill_cache = s0_;
state_1 = state_1 | 0b100000000 /* add SpecializationActive[EmulatedPosixSupport.kill(EmulatedPosixSupport, long, int, ReadAttributeFromObjectNode, IsNode)] */;
this.state_1_ = state_1;
arg0Value.kill(arg1Value, arg2Value, readSignalNode__, isNode__);
return;
}
@Override
public void killpg(Object receiver, long pid, int signal) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
(((EmulatedPosixSupport) receiver)).killpg(pid, signal);
return;
}
/**
* Debug Info:
* Specialization {@link EmulatedPosixSupport#waitpid(EmulatedPosixSupport, long, int, PosixSupportLibrary)}
* Activation probability: 0.01961
* With/without class size: 4/0 bytes
*
*/
@Override
public long[] waitpid(Object arg0Value_, long arg1Value, int arg2Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
{
PosixSupportLibrary posixLib__ = (this);
return arg0Value.waitpid(arg1Value, arg2Value, posixLib__);
}
}
/**
* Debug Info:
* Specialization {@link EmulatedPosixSupport#abort(EmulatedPosixSupport, PosixSupportLibrary)}
* Activation probability: 0.01961
* With/without class size: 4/0 bytes
*
*/
@Override
public void abort(Object arg0Value_) {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
{
PosixSupportLibrary thisLib__ = (this);
arg0Value.abort(thisLib__);
return;
}
}
@Override
public boolean wcoredump(Object receiver, int status) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
return (((EmulatedPosixSupport) receiver)).wcoredump(status);
}
@Override
public boolean wifcontinued(Object receiver, int status) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
return (((EmulatedPosixSupport) receiver)).wifcontinued(status);
}
@Override
public boolean wifstopped(Object receiver, int status) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
return (((EmulatedPosixSupport) receiver)).wifstopped(status);
}
@Override
public boolean wifsignaled(Object receiver, int status) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
return (((EmulatedPosixSupport) receiver)).wifsignaled(status);
}
@Override
public boolean wifexited(Object receiver, int status) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
return (((EmulatedPosixSupport) receiver)).wifexited(status);
}
@Override
public int wexitstatus(Object receiver, int status) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
return (((EmulatedPosixSupport) receiver)).wexitstatus(status);
}
@Override
public int wtermsig(Object receiver, int status) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
return (((EmulatedPosixSupport) receiver)).wtermsig(status);
}
@Override
public int wstopsig(Object receiver, int status) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
return (((EmulatedPosixSupport) receiver)).wstopsig(status);
}
@Override
public long getuid(Object receiver) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
return (((EmulatedPosixSupport) receiver)).getuid();
}
@Override
public long geteuid(Object receiver) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
return (((EmulatedPosixSupport) receiver)).geteuid();
}
@Override
public long getgid(Object receiver) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
return (((EmulatedPosixSupport) receiver)).getgid();
}
@Override
public long getppid(Object receiver) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
return (((EmulatedPosixSupport) receiver)).getppid();
}
@Override
public long getpgid(Object receiver, long pid) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
return (((EmulatedPosixSupport) receiver)).getpgid(pid);
}
@Override
public void setpgid(Object receiver, long pid, long pgid) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
(((EmulatedPosixSupport) receiver)).setpgid(pid, pgid);
return;
}
@Override
public long getpgrp(Object receiver) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
return (((EmulatedPosixSupport) receiver)).getpgrp();
}
@Override
public long getsid(Object receiver, long pid) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
return (((EmulatedPosixSupport) receiver)).getsid(pid);
}
@Override
public long setsid(Object receiver) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
return (((EmulatedPosixSupport) receiver)).setsid();
}
@Override
public OpenPtyResult openpty(Object receiver) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
return (((EmulatedPosixSupport) receiver)).openpty();
}
@Override
public TruffleString ctermid(Object receiver) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
return (((EmulatedPosixSupport) receiver)).ctermid();
}
@Override
public void setenv(Object receiver, Object name, Object value, boolean overwrite) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
(((EmulatedPosixSupport) receiver)).setenv(name, value, overwrite);
return;
}
@Override
public void unsetenv(Object receiver, Object name) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
(((EmulatedPosixSupport) receiver)).unsetenv(name);
return;
}
/**
* Debug Info:
* Specialization {@link EmulatedPosixSupport#forkExec(EmulatedPosixSupport, Object[], Object[], Object, Object[], int, int, int, int, int, int, int, int, boolean, boolean, boolean, int[], FromJavaStringNode)}
* Activation probability: 0.01961
* With/without class size: 4/0 bytes
*
*/
@Override
public int forkExec(Object arg0Value_, Object[] arg1Value, Object[] arg2Value, Object arg3Value, Object[] arg4Value, int arg5Value, int arg6Value, int arg7Value, int arg8Value, int arg9Value, int arg10Value, int arg11Value, int arg12Value, boolean arg13Value, boolean arg14Value, boolean arg15Value, int[] arg16Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
int state_1 = this.state_1_;
if ((state_1 & 0b1000000000) != 0 /* is SpecializationActive[EmulatedPosixSupport.forkExec(EmulatedPosixSupport, Object[], Object[], Object, Object[], int, int, int, int, int, int, int, int, boolean, boolean, boolean, int[], FromJavaStringNode)] */) {
{
FromJavaStringNode js2ts_ = this.js2ts;
if (js2ts_ != null) {
return arg0Value.forkExec(arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, arg6Value, arg7Value, arg8Value, arg9Value, arg10Value, arg11Value, arg12Value, arg13Value, arg14Value, arg15Value, arg16Value, js2ts_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return forkExecNode_AndSpecialize(arg0Value, arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, arg6Value, arg7Value, arg8Value, arg9Value, arg10Value, arg11Value, arg12Value, arg13Value, arg14Value, arg15Value, arg16Value);
}
private int forkExecNode_AndSpecialize(EmulatedPosixSupport arg0Value, Object[] arg1Value, Object[] arg2Value, Object arg3Value, Object[] arg4Value, int arg5Value, int arg6Value, int arg7Value, int arg8Value, int arg9Value, int arg10Value, int arg11Value, int arg12Value, boolean arg13Value, boolean arg14Value, boolean arg15Value, int[] arg16Value) throws PosixException {
int state_1 = this.state_1_;
FromJavaStringNode js2ts_;
FromJavaStringNode js2ts__shared = this.js2ts;
if (js2ts__shared != null) {
js2ts_ = js2ts__shared;
} else {
js2ts_ = this.insert((FromJavaStringNode.create()));
if (js2ts_ == null) {
throw new IllegalStateException("Specialization 'forkExec(EmulatedPosixSupport, Object[], Object[], Object, Object[], int, int, int, int, int, int, int, int, boolean, boolean, boolean, int[], FromJavaStringNode)' contains a shared cache with name 'fromJavaStringNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.js2ts == null) {
VarHandle.storeStoreFence();
this.js2ts = js2ts_;
}
state_1 = state_1 | 0b1000000000 /* add SpecializationActive[EmulatedPosixSupport.forkExec(EmulatedPosixSupport, Object[], Object[], Object, Object[], int, int, int, int, int, int, int, int, boolean, boolean, boolean, int[], FromJavaStringNode)] */;
this.state_1_ = state_1;
return arg0Value.forkExec(arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, arg6Value, arg7Value, arg8Value, arg9Value, arg10Value, arg11Value, arg12Value, arg13Value, arg14Value, arg15Value, arg16Value, js2ts_);
}
/**
* Debug Info:
* Specialization {@link EmulatedPosixSupport#execv(EmulatedPosixSupport, Object, Object[], EqualNode)}
* Activation probability: 0.01961
* With/without class size: 4/0 bytes
*
*/
@Override
public void execv(Object arg0Value_, Object arg1Value, Object[] arg2Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
int state_1 = this.state_1_;
if ((state_1 & 0b10000000000) != 0 /* is SpecializationActive[EmulatedPosixSupport.execv(EmulatedPosixSupport, Object, Object[], EqualNode)] */) {
{
EqualNode eq_ = this.eq;
if (eq_ != null) {
arg0Value.execv(arg1Value, arg2Value, eq_);
return;
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
execvNode_AndSpecialize(arg0Value, arg1Value, arg2Value);
return;
}
private void execvNode_AndSpecialize(EmulatedPosixSupport arg0Value, Object arg1Value, Object[] arg2Value) throws PosixException {
int state_1 = this.state_1_;
EqualNode eq_;
EqualNode eq__shared = this.eq;
if (eq__shared != null) {
eq_ = eq__shared;
} else {
eq_ = this.insert((EqualNode.create()));
if (eq_ == null) {
throw new IllegalStateException("Specialization 'execv(EmulatedPosixSupport, Object, Object[], EqualNode)' contains a shared cache with name 'eqNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.eq == null) {
VarHandle.storeStoreFence();
this.eq = eq_;
}
state_1 = state_1 | 0b10000000000 /* add SpecializationActive[EmulatedPosixSupport.execv(EmulatedPosixSupport, Object, Object[], EqualNode)] */;
this.state_1_ = state_1;
arg0Value.execv(arg1Value, arg2Value, eq_);
return;
}
@Override
public int system(Object receiver, Object command) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
return (((EmulatedPosixSupport) receiver)).system(command);
}
/**
* Debug Info:
* Specialization {@link EmulatedPosixSupport#mmap(EmulatedPosixSupport, long, int, int, int, long, Node, InlinedConditionProfile, EqualNode, FromJavaStringNode)}
* Activation probability: 0.01961
* With/without class size: 4/0 bytes
*
*/
@Override
public Object mmap(Object arg0Value_, long arg1Value, int arg2Value, int arg3Value, int arg4Value, long arg5Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
int state_1 = this.state_1_;
if ((state_1 & 0b100000000000) != 0 /* is SpecializationActive[EmulatedPosixSupport.mmap(EmulatedPosixSupport, long, int, int, int, long, Node, InlinedConditionProfile, EqualNode, FromJavaStringNode)] */) {
{
EqualNode eq_ = this.eq;
if (eq_ != null) {
FromJavaStringNode js2ts_ = this.js2ts;
if (js2ts_ != null) {
Node inliningTarget__ = (this);
return arg0Value.mmap(arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, inliningTarget__, INLINED_DEFAULT_DIR_PROFILE, eq_, js2ts_);
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return mmapNode_AndSpecialize(arg0Value, arg1Value, arg2Value, arg3Value, arg4Value, arg5Value);
}
private MMapHandle mmapNode_AndSpecialize(EmulatedPosixSupport arg0Value, long arg1Value, int arg2Value, int arg3Value, int arg4Value, long arg5Value) throws PosixException {
int state_1 = this.state_1_;
{
Node inliningTarget__ = null;
inliningTarget__ = (this);
EqualNode eq_;
EqualNode eq__shared = this.eq;
if (eq__shared != null) {
eq_ = eq__shared;
} else {
eq_ = this.insert((EqualNode.create()));
if (eq_ == null) {
throw new IllegalStateException("Specialization 'mmap(EmulatedPosixSupport, long, int, int, int, long, Node, InlinedConditionProfile, EqualNode, FromJavaStringNode)' contains a shared cache with name 'eqNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.eq == null) {
VarHandle.storeStoreFence();
this.eq = eq_;
}
FromJavaStringNode js2ts_;
FromJavaStringNode js2ts__shared = this.js2ts;
if (js2ts__shared != null) {
js2ts_ = js2ts__shared;
} else {
js2ts_ = this.insert((FromJavaStringNode.create()));
if (js2ts_ == null) {
throw new IllegalStateException("Specialization 'mmap(EmulatedPosixSupport, long, int, int, int, long, Node, InlinedConditionProfile, EqualNode, FromJavaStringNode)' contains a shared cache with name 'fromJavaStringNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.js2ts == null) {
VarHandle.storeStoreFence();
this.js2ts = js2ts_;
}
state_1 = state_1 | 0b100000000000 /* add SpecializationActive[EmulatedPosixSupport.mmap(EmulatedPosixSupport, long, int, int, int, long, Node, InlinedConditionProfile, EqualNode, FromJavaStringNode)] */;
this.state_1_ = state_1;
return arg0Value.mmap(arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, inliningTarget__, INLINED_DEFAULT_DIR_PROFILE, eq_, js2ts_);
}
}
/**
* Debug Info:
* Specialization {@link EmulatedPosixSupport#mmapReadByte(EmulatedPosixSupport, Object, long, Node, InlinedBranchProfile, EqualNode)}
* Activation probability: 0.01961
* With/without class size: 4/0 bytes
*
*/
@Override
public byte mmapReadByte(Object arg0Value_, Object arg1Value, long arg2Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
int state_1 = this.state_1_;
if ((state_1 & 0b1000000000000) != 0 /* is SpecializationActive[EmulatedPosixSupport.mmapReadByte(EmulatedPosixSupport, Object, long, Node, InlinedBranchProfile, EqualNode)] */) {
{
EqualNode eq_ = this.eq;
if (eq_ != null) {
Node inliningTarget__ = (this);
return arg0Value.mmapReadByte(arg1Value, arg2Value, inliningTarget__, INLINED_ERROR_BRANCH, eq_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return mmapReadByteNode_AndSpecialize(arg0Value, arg1Value, arg2Value);
}
private byte mmapReadByteNode_AndSpecialize(EmulatedPosixSupport arg0Value, Object arg1Value, long arg2Value) throws PosixException {
int state_1 = this.state_1_;
{
Node inliningTarget__ = null;
inliningTarget__ = (this);
EqualNode eq_;
EqualNode eq__shared = this.eq;
if (eq__shared != null) {
eq_ = eq__shared;
} else {
eq_ = this.insert((EqualNode.create()));
if (eq_ == null) {
throw new IllegalStateException("Specialization 'mmapReadByte(EmulatedPosixSupport, Object, long, Node, InlinedBranchProfile, EqualNode)' contains a shared cache with name 'eqNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.eq == null) {
VarHandle.storeStoreFence();
this.eq = eq_;
}
state_1 = state_1 | 0b1000000000000 /* add SpecializationActive[EmulatedPosixSupport.mmapReadByte(EmulatedPosixSupport, Object, long, Node, InlinedBranchProfile, EqualNode)] */;
this.state_1_ = state_1;
return arg0Value.mmapReadByte(arg1Value, arg2Value, inliningTarget__, INLINED_ERROR_BRANCH, eq_);
}
}
/**
* Debug Info:
* Specialization {@link EmulatedPosixSupport#mmapWriteByte(EmulatedPosixSupport, Object, long, byte, Node, InlinedBranchProfile, EqualNode)}
* Activation probability: 0.01961
* With/without class size: 4/0 bytes
*
*/
@Override
public void mmapWriteByte(Object arg0Value_, Object arg1Value, long arg2Value, byte arg3Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
int state_1 = this.state_1_;
if ((state_1 & 0b10000000000000) != 0 /* is SpecializationActive[EmulatedPosixSupport.mmapWriteByte(EmulatedPosixSupport, Object, long, byte, Node, InlinedBranchProfile, EqualNode)] */) {
{
EqualNode eq_ = this.eq;
if (eq_ != null) {
Node inliningTarget__ = (this);
arg0Value.mmapWriteByte(arg1Value, arg2Value, arg3Value, inliningTarget__, INLINED_ERROR_BRANCH, eq_);
return;
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
mmapWriteByteNode_AndSpecialize(arg0Value, arg1Value, arg2Value, arg3Value);
return;
}
private void mmapWriteByteNode_AndSpecialize(EmulatedPosixSupport arg0Value, Object arg1Value, long arg2Value, byte arg3Value) throws PosixException {
int state_1 = this.state_1_;
{
Node inliningTarget__ = null;
inliningTarget__ = (this);
EqualNode eq_;
EqualNode eq__shared = this.eq;
if (eq__shared != null) {
eq_ = eq__shared;
} else {
eq_ = this.insert((EqualNode.create()));
if (eq_ == null) {
throw new IllegalStateException("Specialization 'mmapWriteByte(EmulatedPosixSupport, Object, long, byte, Node, InlinedBranchProfile, EqualNode)' contains a shared cache with name 'eqNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.eq == null) {
VarHandle.storeStoreFence();
this.eq = eq_;
}
state_1 = state_1 | 0b10000000000000 /* add SpecializationActive[EmulatedPosixSupport.mmapWriteByte(EmulatedPosixSupport, Object, long, byte, Node, InlinedBranchProfile, EqualNode)] */;
this.state_1_ = state_1;
arg0Value.mmapWriteByte(arg1Value, arg2Value, arg3Value, inliningTarget__, INLINED_ERROR_BRANCH, eq_);
return;
}
}
/**
* Debug Info:
* Specialization {@link EmulatedPosixSupport#mmapReadBytes(EmulatedPosixSupport, Object, long, byte[], int, Node, InlinedBranchProfile, EqualNode)}
* Activation probability: 0.01961
* With/without class size: 4/0 bytes
*
*/
@Override
public int mmapReadBytes(Object arg0Value_, Object arg1Value, long arg2Value, byte[] arg3Value, int arg4Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
int state_1 = this.state_1_;
if ((state_1 & 0b100000000000000) != 0 /* is SpecializationActive[EmulatedPosixSupport.mmapReadBytes(EmulatedPosixSupport, Object, long, byte[], int, Node, InlinedBranchProfile, EqualNode)] */) {
{
EqualNode eq_ = this.eq;
if (eq_ != null) {
Node inliningTarget__ = (this);
return arg0Value.mmapReadBytes(arg1Value, arg2Value, arg3Value, arg4Value, inliningTarget__, INLINED_ERROR_BRANCH, eq_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return mmapReadBytesNode_AndSpecialize(arg0Value, arg1Value, arg2Value, arg3Value, arg4Value);
}
private int mmapReadBytesNode_AndSpecialize(EmulatedPosixSupport arg0Value, Object arg1Value, long arg2Value, byte[] arg3Value, int arg4Value) throws PosixException {
int state_1 = this.state_1_;
{
Node inliningTarget__ = null;
inliningTarget__ = (this);
EqualNode eq_;
EqualNode eq__shared = this.eq;
if (eq__shared != null) {
eq_ = eq__shared;
} else {
eq_ = this.insert((EqualNode.create()));
if (eq_ == null) {
throw new IllegalStateException("Specialization 'mmapReadBytes(EmulatedPosixSupport, Object, long, byte[], int, Node, InlinedBranchProfile, EqualNode)' contains a shared cache with name 'eqNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.eq == null) {
VarHandle.storeStoreFence();
this.eq = eq_;
}
state_1 = state_1 | 0b100000000000000 /* add SpecializationActive[EmulatedPosixSupport.mmapReadBytes(EmulatedPosixSupport, Object, long, byte[], int, Node, InlinedBranchProfile, EqualNode)] */;
this.state_1_ = state_1;
return arg0Value.mmapReadBytes(arg1Value, arg2Value, arg3Value, arg4Value, inliningTarget__, INLINED_ERROR_BRANCH, eq_);
}
}
/**
* Debug Info:
* Specialization {@link EmulatedPosixSupport#mmapWriteBytes(EmulatedPosixSupport, Object, long, byte[], int, Node, InlinedBranchProfile, EqualNode)}
* Activation probability: 0.01961
* With/without class size: 4/0 bytes
*
*/
@Override
public void mmapWriteBytes(Object arg0Value_, Object arg1Value, long arg2Value, byte[] arg3Value, int arg4Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
int state_1 = this.state_1_;
if ((state_1 & 0b1000000000000000) != 0 /* is SpecializationActive[EmulatedPosixSupport.mmapWriteBytes(EmulatedPosixSupport, Object, long, byte[], int, Node, InlinedBranchProfile, EqualNode)] */) {
{
EqualNode eq_ = this.eq;
if (eq_ != null) {
Node inliningTarget__ = (this);
arg0Value.mmapWriteBytes(arg1Value, arg2Value, arg3Value, arg4Value, inliningTarget__, INLINED_ERROR_BRANCH, eq_);
return;
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
mmapWriteBytesNode_AndSpecialize(arg0Value, arg1Value, arg2Value, arg3Value, arg4Value);
return;
}
private void mmapWriteBytesNode_AndSpecialize(EmulatedPosixSupport arg0Value, Object arg1Value, long arg2Value, byte[] arg3Value, int arg4Value) throws PosixException {
int state_1 = this.state_1_;
{
Node inliningTarget__ = null;
inliningTarget__ = (this);
EqualNode eq_;
EqualNode eq__shared = this.eq;
if (eq__shared != null) {
eq_ = eq__shared;
} else {
eq_ = this.insert((EqualNode.create()));
if (eq_ == null) {
throw new IllegalStateException("Specialization 'mmapWriteBytes(EmulatedPosixSupport, Object, long, byte[], int, Node, InlinedBranchProfile, EqualNode)' contains a shared cache with name 'eqNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.eq == null) {
VarHandle.storeStoreFence();
this.eq = eq_;
}
state_1 = state_1 | 0b1000000000000000 /* add SpecializationActive[EmulatedPosixSupport.mmapWriteBytes(EmulatedPosixSupport, Object, long, byte[], int, Node, InlinedBranchProfile, EqualNode)] */;
this.state_1_ = state_1;
arg0Value.mmapWriteBytes(arg1Value, arg2Value, arg3Value, arg4Value, inliningTarget__, INLINED_ERROR_BRANCH, eq_);
return;
}
}
@Override
public void mmapFlush(Object receiver, Object mmap, long offset, long length) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
(((EmulatedPosixSupport) receiver)).mmapFlush(mmap, offset, length);
return;
}
/**
* Debug Info:
* Specialization {@link EmulatedPosixSupport#mmapUnmap(EmulatedPosixSupport, Object, long, EqualNode)}
* Activation probability: 0.01961
* With/without class size: 4/0 bytes
*
*/
@Override
public void mmapUnmap(Object arg0Value_, Object arg1Value, long arg2Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
int state_1 = this.state_1_;
if ((state_1 & 0x10000) != 0 /* is SpecializationActive[EmulatedPosixSupport.mmapUnmap(EmulatedPosixSupport, Object, long, EqualNode)] */) {
{
EqualNode eq_ = this.eq;
if (eq_ != null) {
arg0Value.mmapUnmap(arg1Value, arg2Value, eq_);
return;
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
mmapUnmapNode_AndSpecialize(arg0Value, arg1Value, arg2Value);
return;
}
private void mmapUnmapNode_AndSpecialize(EmulatedPosixSupport arg0Value, Object arg1Value, long arg2Value) throws PosixException {
int state_1 = this.state_1_;
EqualNode eq_;
EqualNode eq__shared = this.eq;
if (eq__shared != null) {
eq_ = eq__shared;
} else {
eq_ = this.insert((EqualNode.create()));
if (eq_ == null) {
throw new IllegalStateException("Specialization 'mmapUnmap(EmulatedPosixSupport, Object, long, EqualNode)' contains a shared cache with name 'eqNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.eq == null) {
VarHandle.storeStoreFence();
this.eq = eq_;
}
state_1 = state_1 | 0x10000 /* add SpecializationActive[EmulatedPosixSupport.mmapUnmap(EmulatedPosixSupport, Object, long, EqualNode)] */;
this.state_1_ = state_1;
arg0Value.mmapUnmap(arg1Value, arg2Value, eq_);
return;
}
@Override
public long mmapGetPointer(Object receiver, Object mmap) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
return (((EmulatedPosixSupport) receiver)).mmapGetPointer(mmap);
}
@Override
public TruffleString crypt(Object receiver, TruffleString word, TruffleString salt) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
return (((EmulatedPosixSupport) receiver)).crypt(word, salt);
}
@Override
public PwdResult getpwuid(Object receiver, long uid) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
return (((EmulatedPosixSupport) receiver)).getpwuid(uid);
}
@Override
public PwdResult getpwnam(Object receiver, Object name) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
return (((EmulatedPosixSupport) receiver)).getpwnam(name);
}
@Override
public boolean hasGetpwentries(Object receiver) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
return (((EmulatedPosixSupport) receiver)).hasGetpwentries();
}
@Override
public PwdResult[] getpwentries(Object receiver) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
return (((EmulatedPosixSupport) receiver)).getpwentries();
}
/**
* Debug Info:
* Specialization {@link EmulatedPosixSupport#socket(EmulatedPosixSupport, int, int, int, EqualNode)}
* Activation probability: 0.01961
* With/without class size: 4/0 bytes
*
*/
@Override
public int socket(Object arg0Value_, int arg1Value, int arg2Value, int arg3Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
int state_1 = this.state_1_;
if ((state_1 & 0x20000) != 0 /* is SpecializationActive[EmulatedPosixSupport.socket(EmulatedPosixSupport, int, int, int, EqualNode)] */) {
{
EqualNode eq_ = this.eq;
if (eq_ != null) {
return arg0Value.socket(arg1Value, arg2Value, arg3Value, eq_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return socketNode_AndSpecialize(arg0Value, arg1Value, arg2Value, arg3Value);
}
private int socketNode_AndSpecialize(EmulatedPosixSupport arg0Value, int arg1Value, int arg2Value, int arg3Value) throws PosixException {
int state_1 = this.state_1_;
EqualNode eq_;
EqualNode eq__shared = this.eq;
if (eq__shared != null) {
eq_ = eq__shared;
} else {
eq_ = this.insert((EqualNode.create()));
if (eq_ == null) {
throw new IllegalStateException("Specialization 'socket(EmulatedPosixSupport, int, int, int, EqualNode)' contains a shared cache with name 'eqNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.eq == null) {
VarHandle.storeStoreFence();
this.eq = eq_;
}
state_1 = state_1 | 0x20000 /* add SpecializationActive[EmulatedPosixSupport.socket(EmulatedPosixSupport, int, int, int, EqualNode)] */;
this.state_1_ = state_1;
return arg0Value.socket(arg1Value, arg2Value, arg3Value, eq_);
}
@Override
public AcceptResult accept(Object receiver, int sockfd) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
return (((EmulatedPosixSupport) receiver)).accept(sockfd);
}
@Override
public void bind(Object receiver, int sockfd, UniversalSockAddr addr) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
(((EmulatedPosixSupport) receiver)).bind(sockfd, addr);
return;
}
@Override
public void connect(Object receiver, int sockfd, UniversalSockAddr addr) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
(((EmulatedPosixSupport) receiver)).connect(sockfd, addr);
return;
}
@Override
public void listen(Object receiver, int sockfd, int backlog) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
(((EmulatedPosixSupport) receiver)).listen(sockfd, backlog);
return;
}
@Override
public UniversalSockAddr getpeername(Object receiver, int sockfd) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
return (((EmulatedPosixSupport) receiver)).getpeername(sockfd);
}
@Override
public UniversalSockAddr getsockname(Object receiver, int sockfd) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
return (((EmulatedPosixSupport) receiver)).getsockname(sockfd);
}
@Override
public int send(Object receiver, int sockfd, byte[] buf, int offset, int len, int flags) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
return (((EmulatedPosixSupport) receiver)).send(sockfd, buf, offset, len, flags);
}
@Override
public int sendto(Object receiver, int sockfd, byte[] buf, int offset, int len, int flags, UniversalSockAddr destAddr) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
return (((EmulatedPosixSupport) receiver)).sendto(sockfd, buf, offset, len, flags, destAddr);
}
@Override
public int recv(Object receiver, int sockfd, byte[] buf, int offset, int len, int flags) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
return (((EmulatedPosixSupport) receiver)).recv(sockfd, buf, offset, len, flags);
}
@Override
public RecvfromResult recvfrom(Object receiver, int sockfd, byte[] buf, int offset, int len, int flags) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
return (((EmulatedPosixSupport) receiver)).recvfrom(sockfd, buf, offset, len, flags);
}
@Override
public void shutdown(Object receiver, int sockfd, int how) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
(((EmulatedPosixSupport) receiver)).shutdown(sockfd, how);
return;
}
@Override
public int getsockopt(Object receiver, int sockfd, int level, int optname, byte[] optval, int optlen) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
return (((EmulatedPosixSupport) receiver)).getsockopt(sockfd, level, optname, optval, optlen);
}
@Override
public void setsockopt(Object receiver, int sockfd, int level, int optname, byte[] optval, int optlen) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
(((EmulatedPosixSupport) receiver)).setsockopt(sockfd, level, optname, optval, optlen);
return;
}
@Override
public int inet_addr(Object receiver, Object src) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
return (((EmulatedPosixSupport) receiver)).inet_addr(src);
}
@Override
public int inet_aton(Object receiver, Object src) throws InvalidAddressException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
return (((EmulatedPosixSupport) receiver)).inet_aton(src);
}
@Override
public Object inet_ntoa(Object receiver, int address) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
return (((EmulatedPosixSupport) receiver)).inet_ntoa(address);
}
@Override
public byte[] inet_pton(Object receiver, int family, Object src) throws PosixException, InvalidAddressException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
return (((EmulatedPosixSupport) receiver)).inet_pton(family, src);
}
@Override
public Object inet_ntop(Object receiver, int family, byte[] src) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
return (((EmulatedPosixSupport) receiver)).inet_ntop(family, src);
}
@Override
public Object gethostname(Object receiver) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
return (((EmulatedPosixSupport) receiver)).gethostname();
}
@Override
public Object[] getnameinfo(Object receiver, UniversalSockAddr addr, int flags) throws GetAddrInfoException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
return (((EmulatedPosixSupport) receiver)).getnameinfo(addr, flags);
}
@Override
public AddrInfoCursor getaddrinfo(Object receiver, Object node, Object service, int family, int sockType, int protocol, int flags) throws GetAddrInfoException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
return (((EmulatedPosixSupport) receiver)).getaddrinfo(node, service, family, sockType, protocol, flags);
}
/**
* Debug Info:
* Specialization {@link EmulatedPosixSupport#createPathFromString(EmulatedPosixSupport, TruffleString, ToJavaStringNode)}
* Activation probability: 0.01961
* With/without class size: 4/0 bytes
*
*/
@Override
public Object createPathFromString(Object arg0Value_, TruffleString arg1Value) {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
int state_1 = this.state_1_;
if ((state_1 & 0x40000) != 0 /* is SpecializationActive[EmulatedPosixSupport.createPathFromString(EmulatedPosixSupport, TruffleString, ToJavaStringNode)] */) {
{
ToJavaStringNode ts2js_ = this.ts2js;
if (ts2js_ != null) {
return arg0Value.createPathFromString(arg1Value, ts2js_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return createPathFromStringNode_AndSpecialize(arg0Value, arg1Value);
}
private Object createPathFromStringNode_AndSpecialize(EmulatedPosixSupport arg0Value, TruffleString arg1Value) {
int state_1 = this.state_1_;
ToJavaStringNode ts2js_;
ToJavaStringNode ts2js__shared = this.ts2js;
if (ts2js__shared != null) {
ts2js_ = ts2js__shared;
} else {
ts2js_ = this.insert((ToJavaStringNode.create()));
if (ts2js_ == null) {
throw new IllegalStateException("Specialization 'createPathFromString(EmulatedPosixSupport, TruffleString, ToJavaStringNode)' contains a shared cache with name 'toJavaStringNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.ts2js == null) {
VarHandle.storeStoreFence();
this.ts2js = ts2js_;
}
state_1 = state_1 | 0x40000 /* add SpecializationActive[EmulatedPosixSupport.createPathFromString(EmulatedPosixSupport, TruffleString, ToJavaStringNode)] */;
this.state_1_ = state_1;
return arg0Value.createPathFromString(arg1Value, ts2js_);
}
@Override
public Object createPathFromBytes(Object receiver, byte[] path) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
return (((EmulatedPosixSupport) receiver)).createPathFromBytes(path);
}
/**
* Debug Info:
* Specialization {@link EmulatedPosixSupport#getPathAsString(EmulatedPosixSupport, Object, FromJavaStringNode)}
* Activation probability: 0.01961
* With/without class size: 4/0 bytes
*
*/
@Override
public TruffleString getPathAsString(Object arg0Value_, Object arg1Value) {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
int state_1 = this.state_1_;
if ((state_1 & 0x80000) != 0 /* is SpecializationActive[EmulatedPosixSupport.getPathAsString(EmulatedPosixSupport, Object, FromJavaStringNode)] */) {
{
FromJavaStringNode js2ts_ = this.js2ts;
if (js2ts_ != null) {
return arg0Value.getPathAsString(arg1Value, js2ts_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return getPathAsStringNode_AndSpecialize(arg0Value, arg1Value);
}
private TruffleString getPathAsStringNode_AndSpecialize(EmulatedPosixSupport arg0Value, Object arg1Value) {
int state_1 = this.state_1_;
FromJavaStringNode js2ts_;
FromJavaStringNode js2ts__shared = this.js2ts;
if (js2ts__shared != null) {
js2ts_ = js2ts__shared;
} else {
js2ts_ = this.insert((FromJavaStringNode.create()));
if (js2ts_ == null) {
throw new IllegalStateException("Specialization 'getPathAsString(EmulatedPosixSupport, Object, FromJavaStringNode)' contains a shared cache with name 'fromJavaStringNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.js2ts == null) {
VarHandle.storeStoreFence();
this.js2ts = js2ts_;
}
state_1 = state_1 | 0x80000 /* add SpecializationActive[EmulatedPosixSupport.getPathAsString(EmulatedPosixSupport, Object, FromJavaStringNode)] */;
this.state_1_ = state_1;
return arg0Value.getPathAsString(arg1Value, js2ts_);
}
@Override
public Buffer getPathAsBytes(Object receiver, Object path) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
return (((EmulatedPosixSupport) receiver)).getPathAsBytes(path);
}
@GeneratedBy(EmulatedPosixSupport.class)
@DenyReplace
private static final class KillNode_KillData extends Node implements SpecializationDataNode {
/**
* Source Info:
* Specialization: {@link EmulatedPosixSupport#kill(EmulatedPosixSupport, long, int, ReadAttributeFromObjectNode, IsNode)}
* Parameter: {@link ReadAttributeFromObjectNode} readSignalNode
*/
@Child ReadAttributeFromObjectNode readSignalNode_;
/**
* Source Info:
* Specialization: {@link EmulatedPosixSupport#kill(EmulatedPosixSupport, long, int, ReadAttributeFromObjectNode, IsNode)}
* Parameter: {@link IsNode} isNode
*/
@Child IsNode isNode_;
KillNode_KillData() {
}
@Override
public NodeCost getCost() {
return NodeCost.NONE;
}
}
}
@GeneratedBy(EmulatedPosixSupport.class)
@DenyReplace
private static final class Uncached extends PosixSupportLibrary {
protected Uncached() {
}
@Override
@TruffleBoundary
public boolean accepts(Object receiver) {
assert !(receiver instanceof EmulatedPosixSupport) || DYNAMIC_DISPATCH_LIBRARY_.getUncached().dispatch(receiver) == null : "Invalid library export. Exported receiver with dynamic dispatch found but not expected.";
return receiver instanceof EmulatedPosixSupport;
}
@Override
public boolean isAdoptable() {
return false;
}
@Override
public NodeCost getCost() {
return NodeCost.MEGAMORPHIC;
}
@TruffleBoundary
@Override
public long getpid(Object arg0Value_) {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
return Getpid.getPidFallback(arg0Value);
}
@TruffleBoundary
@Override
public UniversalSockAddr createUniversalSockAddr(Object arg0Value_, FamilySpecificSockAddr arg1Value) {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
if (arg1Value instanceof Inet4SockAddr) {
Inet4SockAddr arg1Value_ = (Inet4SockAddr) arg1Value;
return CreateUniversalSockAddr.inet4(arg0Value, arg1Value_);
}
if (arg1Value instanceof Inet6SockAddr) {
Inet6SockAddr arg1Value_ = (Inet6SockAddr) arg1Value;
return CreateUniversalSockAddr.inet6(arg0Value, arg1Value_);
}
if (arg1Value instanceof UnixSockAddr) {
UnixSockAddr arg1Value_ = (UnixSockAddr) arg1Value;
return CreateUniversalSockAddr.unix(arg0Value, arg1Value_);
}
throw new UnsupportedSpecializationException(this, new Node[] {null, null}, arg0Value, arg1Value);
}
@TruffleBoundary
@Override
public TruffleString getBackend(Object receiver) {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((EmulatedPosixSupport) receiver) .getBackend();
}
@TruffleBoundary
@Override
public int umask(Object receiver, int mask) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((EmulatedPosixSupport) receiver) .umask(mask);
}
@TruffleBoundary
@Override
public TruffleString strerror(Object arg0Value_, int arg1Value) {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
return arg0Value.strerror(arg1Value, (this), (InlinedBranchProfile.getUncached()));
}
@TruffleBoundary
@Override
public int close(Object receiver, int fd) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((EmulatedPosixSupport) receiver) .closeMessage(fd);
}
@TruffleBoundary
@Override
public int openat(Object arg0Value_, int arg1Value, Object arg2Value, int arg3Value, int arg4Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
return arg0Value.openat(arg1Value, arg2Value, arg3Value, arg4Value, (this), (InlinedBranchProfile.getUncached()), (InlinedConditionProfile.getUncached()), (EqualNode.getUncached()), (FromJavaStringNode.getUncached()), (ToJavaStringNode.getUncached()));
}
@TruffleBoundary
@Override
public long write(Object arg0Value_, int arg1Value, Buffer arg2Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
return arg0Value.write(arg1Value, arg2Value, (this), (InlinedBranchProfile.getUncached()), (EqualNode.getUncached()));
}
@TruffleBoundary
@Override
public Buffer read(Object arg0Value_, int arg1Value, long arg2Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
return arg0Value.read(arg1Value, arg2Value, (this), (InlinedBranchProfile.getUncached()), (EqualNode.getUncached()));
}
@TruffleBoundary
@Override
public int dup(Object receiver, int fd) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((EmulatedPosixSupport) receiver) .dup(fd);
}
@TruffleBoundary
@Override
public int dup2(Object arg0Value_, int arg1Value, int arg2Value, boolean arg3Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
return arg0Value.dup2(arg1Value, arg2Value, arg3Value, (EqualNode.getUncached()));
}
@TruffleBoundary
@Override
public boolean getInheritable(Object receiver, int fd) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((EmulatedPosixSupport) receiver) .getInheritable(fd);
}
@TruffleBoundary
@Override
public void setInheritable(Object receiver, int fd, boolean inheritable) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
((EmulatedPosixSupport) receiver) .setInheritable(fd, inheritable);
return;
}
@TruffleBoundary
@Override
public int[] pipe(Object arg0Value_) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
return arg0Value.pipeMessage((EqualNode.getUncached()));
}
@TruffleBoundary
@Override
public SelectResult select(Object receiver, int[] readfds, int[] writefds, int[] errorfds, Timeval timeout) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((EmulatedPosixSupport) receiver) .select(readfds, writefds, errorfds, timeout);
}
@TruffleBoundary
@Override
public long lseek(Object arg0Value_, int arg1Value, long arg2Value, int arg3Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
return arg0Value.lseek(arg1Value, arg2Value, arg3Value, (this), (InlinedBranchProfile.getUncached()), (InlinedConditionProfile.getUncached()), (InlinedConditionProfile.getUncached()), (InlinedConditionProfile.getUncached()), (EqualNode.getUncached()));
}
@TruffleBoundary
@Override
public void ftruncate(Object arg0Value_, int arg1Value, long arg2Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
arg0Value.ftruncateMessage(arg1Value, arg2Value, (this), (InlinedBranchProfile.getUncached()), (EqualNode.getUncached()));
return;
}
@TruffleBoundary
@Override
public void fsync(Object receiver, int fd) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
((EmulatedPosixSupport) receiver) .fsyncMessage(fd);
return;
}
@TruffleBoundary
@Override
public void flock(Object arg0Value_, int arg1Value, int arg2Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
arg0Value.flock(arg1Value, arg2Value, (this), (InlinedBranchProfile.getUncached()), (EqualNode.getUncached()));
return;
}
@TruffleBoundary
@Override
public boolean getBlocking(Object receiver, int fd) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((EmulatedPosixSupport) receiver) .getBlocking(fd);
}
@TruffleBoundary
@Override
public void setBlocking(Object arg0Value_, int arg1Value, boolean arg2Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
arg0Value.setBlocking(arg1Value, arg2Value, (EqualNode.getUncached()));
return;
}
@TruffleBoundary
@Override
public int[] getTerminalSize(Object receiver, int fd) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((EmulatedPosixSupport) receiver) .getTerminalSize(fd);
}
@TruffleBoundary
@Override
public long[] fstatat(Object arg0Value_, int arg1Value, Object arg2Value, boolean arg3Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
return arg0Value.fstatat(arg1Value, arg2Value, arg3Value, (this), (InlinedBranchProfile.getUncached()), (InlinedConditionProfile.getUncached()), (EqualNode.getUncached()), (FromJavaStringNode.getUncached()), (ToJavaStringNode.getUncached()));
}
@TruffleBoundary
@Override
public long[] fstat(Object arg0Value_, int arg1Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
return arg0Value.fstat(arg1Value, (this), (InlinedBranchProfile.getUncached()), (InlinedBranchProfile.getUncached()), (InlinedConditionProfile.getUncached()), (EqualNode.getUncached()), (FromJavaStringNode.getUncached()));
}
@TruffleBoundary
@Override
public long[] statvfs(Object receiver, Object path) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((EmulatedPosixSupport) receiver) .statvfs(path);
}
@TruffleBoundary
@Override
public long[] fstatvfs(Object receiver, int fd) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((EmulatedPosixSupport) receiver) .fstatvfs(fd);
}
@TruffleBoundary
@Override
public Object[] uname(Object arg0Value_) {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
return arg0Value.uname((FromJavaStringNode.getUncached()));
}
@TruffleBoundary
@Override
public void unlinkat(Object arg0Value_, int arg1Value, Object arg2Value, boolean arg3Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
arg0Value.unlinkat(arg1Value, arg2Value, arg3Value, (this), (InlinedBranchProfile.getUncached()), (InlinedConditionProfile.getUncached()), (EqualNode.getUncached()), (FromJavaStringNode.getUncached()), (ToJavaStringNode.getUncached()));
return;
}
@TruffleBoundary
@Override
public void linkat(Object arg0Value_, int arg1Value, Object arg2Value, int arg3Value, Object arg4Value, int arg5Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
arg0Value.linkat(arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, (this), (InlinedBranchProfile.getUncached()), (InlinedConditionProfile.getUncached()), (EqualNode.getUncached()), (FromJavaStringNode.getUncached()), (ToJavaStringNode.getUncached()));
return;
}
@TruffleBoundary
@Override
public void symlinkat(Object arg0Value_, Object arg1Value, int arg2Value, Object arg3Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
arg0Value.symlinkat(arg1Value, arg2Value, arg3Value, (this), (InlinedBranchProfile.getUncached()), (InlinedConditionProfile.getUncached()), (EqualNode.getUncached()), (FromJavaStringNode.getUncached()), (ToJavaStringNode.getUncached()));
return;
}
@TruffleBoundary
@Override
public void mkdirat(Object arg0Value_, int arg1Value, Object arg2Value, int arg3Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
arg0Value.mkdirat(arg1Value, arg2Value, arg3Value, (this), (InlinedBranchProfile.getUncached()), (InlinedConditionProfile.getUncached()), (EqualNode.getUncached()), (FromJavaStringNode.getUncached()), (ToJavaStringNode.getUncached()));
return;
}
@TruffleBoundary
@Override
public Object getcwd(Object receiver) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((EmulatedPosixSupport) receiver) .getcwd();
}
@TruffleBoundary
@Override
public void chdir(Object arg0Value_, Object arg1Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
arg0Value.chdir(arg1Value, (this), (InlinedBranchProfile.getUncached()), (EqualNode.getUncached()), (FromJavaStringNode.getUncached()));
return;
}
@TruffleBoundary
@Override
public void fchdir(Object arg0Value_, int arg1Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
arg0Value.fchdir(arg1Value, (this), (InlinedBranchProfile.getUncached()), (EqualNode.getUncached()), (FromJavaStringNode.getUncached()));
return;
}
@TruffleBoundary
@Override
public boolean isatty(Object receiver, int fd) {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((EmulatedPosixSupport) receiver) .isatty(fd);
}
@TruffleBoundary
@Override
public Object opendir(Object arg0Value_, Object arg1Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
return arg0Value.opendir(arg1Value, (EqualNode.getUncached()), (FromJavaStringNode.getUncached()));
}
@TruffleBoundary
@Override
public Object fdopendir(Object arg0Value_, int arg1Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
return arg0Value.fdopendir(arg1Value, (this), (InlinedBranchProfile.getUncached()), (EqualNode.getUncached()), (FromJavaStringNode.getUncached()));
}
@TruffleBoundary
@Override
public void closedir(Object arg0Value_, Object arg1Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
arg0Value.closedir(arg1Value, (EqualNode.getUncached()));
return;
}
@TruffleBoundary
@Override
public Object readdir(Object arg0Value_, Object arg1Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
return arg0Value.readdir(arg1Value, (EqualNode.getUncached()));
}
@TruffleBoundary
@Override
public void rewinddir(Object receiver, Object dirStream) {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
((EmulatedPosixSupport) receiver) .rewinddir(dirStream);
return;
}
@TruffleBoundary
@Override
public Object dirEntryGetName(Object receiver, Object dirEntry) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((EmulatedPosixSupport) receiver) .dirEntryGetName(dirEntry);
}
@TruffleBoundary
@Override
public Object dirEntryGetPath(Object arg0Value_, Object arg1Value, Object arg2Value) {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
return arg0Value.dirEntryGetPath(arg1Value, arg2Value, (FromJavaStringNode.getUncached()));
}
@TruffleBoundary
@Override
public long dirEntryGetInode(Object arg0Value_, Object arg1Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
return arg0Value.dirEntryGetInode(arg1Value, (EqualNode.getUncached()));
}
@TruffleBoundary
@Override
public int dirEntryGetType(Object receiver, Object dirEntry) {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((EmulatedPosixSupport) receiver) .dirEntryGetType(dirEntry);
}
@TruffleBoundary
@Override
public void utimensat(Object arg0Value_, int arg1Value, Object arg2Value, long[] arg3Value, boolean arg4Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
arg0Value.utimensat(arg1Value, arg2Value, arg3Value, arg4Value, (this), (SetUTimeNodeGen.getUncached()), (InlinedConditionProfile.getUncached()), (EqualNode.getUncached()), (FromJavaStringNode.getUncached()), (ToJavaStringNode.getUncached()));
return;
}
@TruffleBoundary
@Override
public void futimens(Object arg0Value_, int arg1Value, long[] arg2Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
arg0Value.futimens(arg1Value, arg2Value, (this), (SetUTimeNodeGen.getUncached()), (EqualNode.getUncached()), (FromJavaStringNode.getUncached()));
return;
}
@TruffleBoundary
@Override
public void futimes(Object arg0Value_, int arg1Value, Timeval[] arg2Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
arg0Value.futimes(arg1Value, arg2Value, (this), (SetUTimeNodeGen.getUncached()), (EqualNode.getUncached()), (FromJavaStringNode.getUncached()));
return;
}
@TruffleBoundary
@Override
public void lutimes(Object arg0Value_, Object arg1Value, Timeval[] arg2Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
arg0Value.lutimes(arg1Value, arg2Value, (this), (SetUTimeNodeGen.getUncached()), (EqualNode.getUncached()), (FromJavaStringNode.getUncached()));
return;
}
@TruffleBoundary
@Override
public void utimes(Object arg0Value_, Object arg1Value, Timeval[] arg2Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
arg0Value.utimes(arg1Value, arg2Value, (this), (SetUTimeNodeGen.getUncached()), (EqualNode.getUncached()), (FromJavaStringNode.getUncached()));
return;
}
@TruffleBoundary
@Override
public void renameat(Object arg0Value_, int arg1Value, Object arg2Value, int arg3Value, Object arg4Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
arg0Value.renameat(arg1Value, arg2Value, arg3Value, arg4Value, (this), (InlinedConditionProfile.getUncached()), (EqualNode.getUncached()), (FromJavaStringNode.getUncached()), (ToJavaStringNode.getUncached()));
return;
}
@TruffleBoundary
@Override
public boolean faccessat(Object arg0Value_, int arg1Value, Object arg2Value, int arg3Value, boolean arg4Value, boolean arg5Value) {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
return arg0Value.faccessat(arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, (this), (InlinedBranchProfile.getUncached()), (InlinedConditionProfile.getUncached()), (EqualNode.getUncached()), (FromJavaStringNode.getUncached()), (ToJavaStringNode.getUncached()));
}
@TruffleBoundary
@Override
public void fchmodat(Object arg0Value_, int arg1Value, Object arg2Value, int arg3Value, boolean arg4Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
arg0Value.fchmodat(arg1Value, arg2Value, arg3Value, arg4Value, (this), (InlinedConditionProfile.getUncached()), (EqualNode.getUncached()), (FromJavaStringNode.getUncached()), (ToJavaStringNode.getUncached()));
return;
}
@TruffleBoundary
@Override
public void fchmod(Object arg0Value_, int arg1Value, int arg2Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
arg0Value.fchmod(arg1Value, arg2Value, (EqualNode.getUncached()), (FromJavaStringNode.getUncached()));
return;
}
@TruffleBoundary
@Override
public Object readlinkat(Object arg0Value_, int arg1Value, Object arg2Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
return arg0Value.readlinkat(arg1Value, arg2Value, (this), (InlinedConditionProfile.getUncached()), (EqualNode.getUncached()), (FromJavaStringNode.getUncached()), (ToJavaStringNode.getUncached()));
}
@TruffleBoundary
@Override
public void kill(Object arg0Value_, long arg1Value, int arg2Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
arg0Value.kill(arg1Value, arg2Value, (ReadAttributeFromObjectNode.getUncached()), (IsNode.getUncached()));
return;
}
@TruffleBoundary
@Override
public void killpg(Object receiver, long pid, int signal) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
((EmulatedPosixSupport) receiver) .killpg(pid, signal);
return;
}
@TruffleBoundary
@Override
public long[] waitpid(Object arg0Value_, long arg1Value, int arg2Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
return arg0Value.waitpid(arg1Value, arg2Value, (this));
}
@TruffleBoundary
@Override
public void abort(Object arg0Value_) {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
arg0Value.abort((this));
return;
}
@TruffleBoundary
@Override
public boolean wcoredump(Object receiver, int status) {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((EmulatedPosixSupport) receiver) .wcoredump(status);
}
@TruffleBoundary
@Override
public boolean wifcontinued(Object receiver, int status) {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((EmulatedPosixSupport) receiver) .wifcontinued(status);
}
@TruffleBoundary
@Override
public boolean wifstopped(Object receiver, int status) {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((EmulatedPosixSupport) receiver) .wifstopped(status);
}
@TruffleBoundary
@Override
public boolean wifsignaled(Object receiver, int status) {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((EmulatedPosixSupport) receiver) .wifsignaled(status);
}
@TruffleBoundary
@Override
public boolean wifexited(Object receiver, int status) {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((EmulatedPosixSupport) receiver) .wifexited(status);
}
@TruffleBoundary
@Override
public int wexitstatus(Object receiver, int status) {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((EmulatedPosixSupport) receiver) .wexitstatus(status);
}
@TruffleBoundary
@Override
public int wtermsig(Object receiver, int status) {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((EmulatedPosixSupport) receiver) .wtermsig(status);
}
@TruffleBoundary
@Override
public int wstopsig(Object receiver, int status) {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((EmulatedPosixSupport) receiver) .wstopsig(status);
}
@TruffleBoundary
@Override
public long getuid(Object receiver) {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((EmulatedPosixSupport) receiver) .getuid();
}
@TruffleBoundary
@Override
public long geteuid(Object receiver) {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((EmulatedPosixSupport) receiver) .geteuid();
}
@TruffleBoundary
@Override
public long getgid(Object receiver) {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((EmulatedPosixSupport) receiver) .getgid();
}
@TruffleBoundary
@Override
public long getppid(Object receiver) {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((EmulatedPosixSupport) receiver) .getppid();
}
@TruffleBoundary
@Override
public long getpgid(Object receiver, long pid) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((EmulatedPosixSupport) receiver) .getpgid(pid);
}
@TruffleBoundary
@Override
public void setpgid(Object receiver, long pid, long pgid) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
((EmulatedPosixSupport) receiver) .setpgid(pid, pgid);
return;
}
@TruffleBoundary
@Override
public long getpgrp(Object receiver) {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((EmulatedPosixSupport) receiver) .getpgrp();
}
@TruffleBoundary
@Override
public long getsid(Object receiver, long pid) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((EmulatedPosixSupport) receiver) .getsid(pid);
}
@TruffleBoundary
@Override
public long setsid(Object receiver) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((EmulatedPosixSupport) receiver) .setsid();
}
@TruffleBoundary
@Override
public OpenPtyResult openpty(Object receiver) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((EmulatedPosixSupport) receiver) .openpty();
}
@TruffleBoundary
@Override
public TruffleString ctermid(Object receiver) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((EmulatedPosixSupport) receiver) .ctermid();
}
@TruffleBoundary
@Override
public void setenv(Object receiver, Object name, Object value, boolean overwrite) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
((EmulatedPosixSupport) receiver) .setenv(name, value, overwrite);
return;
}
@TruffleBoundary
@Override
public void unsetenv(Object receiver, Object name) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
((EmulatedPosixSupport) receiver) .unsetenv(name);
return;
}
@TruffleBoundary
@Override
public int forkExec(Object arg0Value_, Object[] arg1Value, Object[] arg2Value, Object arg3Value, Object[] arg4Value, int arg5Value, int arg6Value, int arg7Value, int arg8Value, int arg9Value, int arg10Value, int arg11Value, int arg12Value, boolean arg13Value, boolean arg14Value, boolean arg15Value, int[] arg16Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
return arg0Value.forkExec(arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, arg6Value, arg7Value, arg8Value, arg9Value, arg10Value, arg11Value, arg12Value, arg13Value, arg14Value, arg15Value, arg16Value, (FromJavaStringNode.getUncached()));
}
@TruffleBoundary
@Override
public void execv(Object arg0Value_, Object arg1Value, Object[] arg2Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
arg0Value.execv(arg1Value, arg2Value, (EqualNode.getUncached()));
return;
}
@TruffleBoundary
@Override
public int system(Object receiver, Object command) {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((EmulatedPosixSupport) receiver) .system(command);
}
@TruffleBoundary
@Override
public Object mmap(Object arg0Value_, long arg1Value, int arg2Value, int arg3Value, int arg4Value, long arg5Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
return arg0Value.mmap(arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, (this), (InlinedConditionProfile.getUncached()), (EqualNode.getUncached()), (FromJavaStringNode.getUncached()));
}
@TruffleBoundary
@Override
public byte mmapReadByte(Object arg0Value_, Object arg1Value, long arg2Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
return arg0Value.mmapReadByte(arg1Value, arg2Value, (this), (InlinedBranchProfile.getUncached()), (EqualNode.getUncached()));
}
@TruffleBoundary
@Override
public void mmapWriteByte(Object arg0Value_, Object arg1Value, long arg2Value, byte arg3Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
arg0Value.mmapWriteByte(arg1Value, arg2Value, arg3Value, (this), (InlinedBranchProfile.getUncached()), (EqualNode.getUncached()));
return;
}
@TruffleBoundary
@Override
public int mmapReadBytes(Object arg0Value_, Object arg1Value, long arg2Value, byte[] arg3Value, int arg4Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
return arg0Value.mmapReadBytes(arg1Value, arg2Value, arg3Value, arg4Value, (this), (InlinedBranchProfile.getUncached()), (EqualNode.getUncached()));
}
@TruffleBoundary
@Override
public void mmapWriteBytes(Object arg0Value_, Object arg1Value, long arg2Value, byte[] arg3Value, int arg4Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
arg0Value.mmapWriteBytes(arg1Value, arg2Value, arg3Value, arg4Value, (this), (InlinedBranchProfile.getUncached()), (EqualNode.getUncached()));
return;
}
@TruffleBoundary
@Override
public void mmapFlush(Object receiver, Object mmap, long offset, long length) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
((EmulatedPosixSupport) receiver) .mmapFlush(mmap, offset, length);
return;
}
@TruffleBoundary
@Override
public void mmapUnmap(Object arg0Value_, Object arg1Value, long arg2Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
arg0Value.mmapUnmap(arg1Value, arg2Value, (EqualNode.getUncached()));
return;
}
@TruffleBoundary
@Override
public long mmapGetPointer(Object receiver, Object mmap) {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((EmulatedPosixSupport) receiver) .mmapGetPointer(mmap);
}
@TruffleBoundary
@Override
public TruffleString crypt(Object receiver, TruffleString word, TruffleString salt) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((EmulatedPosixSupport) receiver) .crypt(word, salt);
}
@TruffleBoundary
@Override
public PwdResult getpwuid(Object receiver, long uid) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((EmulatedPosixSupport) receiver) .getpwuid(uid);
}
@TruffleBoundary
@Override
public PwdResult getpwnam(Object receiver, Object name) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((EmulatedPosixSupport) receiver) .getpwnam(name);
}
@TruffleBoundary
@Override
public boolean hasGetpwentries(Object receiver) {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((EmulatedPosixSupport) receiver) .hasGetpwentries();
}
@TruffleBoundary
@Override
public PwdResult[] getpwentries(Object receiver) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((EmulatedPosixSupport) receiver) .getpwentries();
}
@TruffleBoundary
@Override
public int socket(Object arg0Value_, int arg1Value, int arg2Value, int arg3Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
return arg0Value.socket(arg1Value, arg2Value, arg3Value, (EqualNode.getUncached()));
}
@TruffleBoundary
@Override
public AcceptResult accept(Object receiver, int sockfd) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((EmulatedPosixSupport) receiver) .accept(sockfd);
}
@TruffleBoundary
@Override
public void bind(Object receiver, int sockfd, UniversalSockAddr addr) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
((EmulatedPosixSupport) receiver) .bind(sockfd, addr);
return;
}
@TruffleBoundary
@Override
public void connect(Object receiver, int sockfd, UniversalSockAddr addr) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
((EmulatedPosixSupport) receiver) .connect(sockfd, addr);
return;
}
@TruffleBoundary
@Override
public void listen(Object receiver, int sockfd, int backlog) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
((EmulatedPosixSupport) receiver) .listen(sockfd, backlog);
return;
}
@TruffleBoundary
@Override
public UniversalSockAddr getpeername(Object receiver, int sockfd) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((EmulatedPosixSupport) receiver) .getpeername(sockfd);
}
@TruffleBoundary
@Override
public UniversalSockAddr getsockname(Object receiver, int sockfd) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((EmulatedPosixSupport) receiver) .getsockname(sockfd);
}
@TruffleBoundary
@Override
public int send(Object receiver, int sockfd, byte[] buf, int offset, int len, int flags) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((EmulatedPosixSupport) receiver) .send(sockfd, buf, offset, len, flags);
}
@TruffleBoundary
@Override
public int sendto(Object receiver, int sockfd, byte[] buf, int offset, int len, int flags, UniversalSockAddr destAddr) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((EmulatedPosixSupport) receiver) .sendto(sockfd, buf, offset, len, flags, destAddr);
}
@TruffleBoundary
@Override
public int recv(Object receiver, int sockfd, byte[] buf, int offset, int len, int flags) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((EmulatedPosixSupport) receiver) .recv(sockfd, buf, offset, len, flags);
}
@TruffleBoundary
@Override
public RecvfromResult recvfrom(Object receiver, int sockfd, byte[] buf, int offset, int len, int flags) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((EmulatedPosixSupport) receiver) .recvfrom(sockfd, buf, offset, len, flags);
}
@TruffleBoundary
@Override
public void shutdown(Object receiver, int sockfd, int how) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
((EmulatedPosixSupport) receiver) .shutdown(sockfd, how);
return;
}
@TruffleBoundary
@Override
public int getsockopt(Object receiver, int sockfd, int level, int optname, byte[] optval, int optlen) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((EmulatedPosixSupport) receiver) .getsockopt(sockfd, level, optname, optval, optlen);
}
@TruffleBoundary
@Override
public void setsockopt(Object receiver, int sockfd, int level, int optname, byte[] optval, int optlen) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
((EmulatedPosixSupport) receiver) .setsockopt(sockfd, level, optname, optval, optlen);
return;
}
@TruffleBoundary
@Override
public int inet_addr(Object receiver, Object src) {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((EmulatedPosixSupport) receiver) .inet_addr(src);
}
@TruffleBoundary
@Override
public int inet_aton(Object receiver, Object src) throws InvalidAddressException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((EmulatedPosixSupport) receiver) .inet_aton(src);
}
@TruffleBoundary
@Override
public Object inet_ntoa(Object receiver, int address) {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((EmulatedPosixSupport) receiver) .inet_ntoa(address);
}
@TruffleBoundary
@Override
public byte[] inet_pton(Object receiver, int family, Object src) throws PosixException, InvalidAddressException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((EmulatedPosixSupport) receiver) .inet_pton(family, src);
}
@TruffleBoundary
@Override
public Object inet_ntop(Object receiver, int family, byte[] src) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((EmulatedPosixSupport) receiver) .inet_ntop(family, src);
}
@TruffleBoundary
@Override
public Object gethostname(Object receiver) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((EmulatedPosixSupport) receiver) .gethostname();
}
@TruffleBoundary
@Override
public Object[] getnameinfo(Object receiver, UniversalSockAddr addr, int flags) throws GetAddrInfoException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((EmulatedPosixSupport) receiver) .getnameinfo(addr, flags);
}
@TruffleBoundary
@Override
public AddrInfoCursor getaddrinfo(Object receiver, Object node, Object service, int family, int sockType, int protocol, int flags) throws GetAddrInfoException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((EmulatedPosixSupport) receiver) .getaddrinfo(node, service, family, sockType, protocol, flags);
}
@TruffleBoundary
@Override
public Object createPathFromString(Object arg0Value_, TruffleString arg1Value) {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
return arg0Value.createPathFromString(arg1Value, (ToJavaStringNode.getUncached()));
}
@TruffleBoundary
@Override
public Object createPathFromBytes(Object receiver, byte[] path) {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((EmulatedPosixSupport) receiver) .createPathFromBytes(path);
}
@TruffleBoundary
@Override
public TruffleString getPathAsString(Object arg0Value_, Object arg1Value) {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
EmulatedPosixSupport arg0Value = ((EmulatedPosixSupport) arg0Value_);
return arg0Value.getPathAsString(arg1Value, (FromJavaStringNode.getUncached()));
}
@TruffleBoundary
@Override
public Buffer getPathAsBytes(Object receiver, Object path) {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((EmulatedPosixSupport) receiver) .getPathAsBytes(path);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy