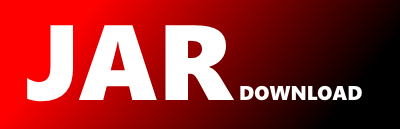
com.oracle.graal.python.runtime.ImageBuildtimePosixSupportGen Maven / Gradle / Ivy
// CheckStyle: start generated
package com.oracle.graal.python.runtime;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.TruffleBoundary;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.library.DynamicDispatchLibrary;
import com.oracle.truffle.api.library.LibraryExport;
import com.oracle.truffle.api.library.LibraryFactory;
import com.oracle.truffle.api.nodes.NodeCost;
import com.oracle.truffle.api.strings.TruffleString;
@GeneratedBy(ImageBuildtimePosixSupport.class)
@SuppressWarnings("javadoc")
public final class ImageBuildtimePosixSupportGen {
private static final LibraryFactory DYNAMIC_DISPATCH_LIBRARY_ = LibraryFactory.resolve(DynamicDispatchLibrary.class);
private static final LibraryFactory POSIX_SUPPORT_LIBRARY_ = LibraryFactory.resolve(PosixSupportLibrary.class);
static {
LibraryExport.register(ImageBuildtimePosixSupport.class, new PosixSupportLibraryExports());
}
private ImageBuildtimePosixSupportGen() {
}
@GeneratedBy(ImageBuildtimePosixSupport.class)
public static class PosixSupportLibraryExports extends LibraryExport {
private PosixSupportLibraryExports() {
super(PosixSupportLibrary.class, ImageBuildtimePosixSupport.class, false, false, 0);
}
@Override
protected PosixSupportLibrary createUncached(Object receiver) {
assert receiver instanceof ImageBuildtimePosixSupport;
PosixSupportLibrary uncached = new Uncached(receiver);
return uncached;
}
@Override
protected PosixSupportLibrary createCached(Object receiver) {
assert receiver instanceof ImageBuildtimePosixSupport;
return new Cached(receiver);
}
@GeneratedBy(ImageBuildtimePosixSupport.class)
public static class Cached extends PosixSupportLibrary {
@Child private PosixSupportLibrary receiverNativePosixSupportPosixSupportLibrary_;
private final Class extends ImageBuildtimePosixSupport> receiverClass_;
protected Cached(Object receiver) {
ImageBuildtimePosixSupport castReceiver = ((ImageBuildtimePosixSupport) receiver) ;
this.receiverNativePosixSupportPosixSupportLibrary_ = POSIX_SUPPORT_LIBRARY_.create((castReceiver.nativePosixSupport));
this.receiverClass_ = castReceiver.getClass();
}
@Override
public boolean accepts(Object receiver) {
assert receiver.getClass() != this.receiverClass_ || DYNAMIC_DISPATCH_LIBRARY_.getUncached().dispatch(receiver) == null : "Invalid library export. Exported receiver with dynamic dispatch found but not expected.";
if (!(CompilerDirectives.isExact(receiver, this.receiverClass_))) {
return false;
} else if (!this.receiverNativePosixSupportPosixSupportLibrary_.accepts((((ImageBuildtimePosixSupport) receiver).nativePosixSupport))) {
return false;
} else {
return true;
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#getBackend(ImageBuildtimePosixSupport, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public TruffleString getBackend(Object arg0Value_) {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
return arg0Value.getBackend(nativeLib__);
}
}
@Override
public NodeCost getCost() {
return NodeCost.MONOMORPHIC;
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#strerror(ImageBuildtimePosixSupport, int, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public TruffleString strerror(Object arg0Value_, int arg1Value) {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
return arg0Value.strerror(arg1Value, nativeLib__);
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#getpid(ImageBuildtimePosixSupport, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public long getpid(Object arg0Value_) {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
return arg0Value.getpid(nativeLib__);
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#umask(ImageBuildtimePosixSupport, int, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public int umask(Object arg0Value_, int arg1Value) throws PosixException {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
return arg0Value.umask(arg1Value, nativeLib__);
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#openat(ImageBuildtimePosixSupport, int, Object, int, int, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public int openat(Object arg0Value_, int arg1Value, Object arg2Value, int arg3Value, int arg4Value) throws PosixException {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
return arg0Value.openat(arg1Value, arg2Value, arg3Value, arg4Value, nativeLib__);
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#close(ImageBuildtimePosixSupport, int, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public int close(Object arg0Value_, int arg1Value) throws PosixException {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
return arg0Value.close(arg1Value, nativeLib__);
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#read(ImageBuildtimePosixSupport, int, long, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public Buffer read(Object arg0Value_, int arg1Value, long arg2Value) throws PosixException {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
return arg0Value.read(arg1Value, arg2Value, nativeLib__);
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#write(ImageBuildtimePosixSupport, int, Buffer, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public long write(Object arg0Value_, int arg1Value, Buffer arg2Value) throws PosixException {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
return arg0Value.write(arg1Value, arg2Value, nativeLib__);
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#dup(ImageBuildtimePosixSupport, int, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public int dup(Object arg0Value_, int arg1Value) throws PosixException {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
return arg0Value.dup(arg1Value, nativeLib__);
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#dup2(ImageBuildtimePosixSupport, int, int, boolean, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public int dup2(Object arg0Value_, int arg1Value, int arg2Value, boolean arg3Value) throws PosixException {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
return arg0Value.dup2(arg1Value, arg2Value, arg3Value, nativeLib__);
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#getInheritable(ImageBuildtimePosixSupport, int, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public boolean getInheritable(Object arg0Value_, int arg1Value) throws PosixException {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
return arg0Value.getInheritable(arg1Value, nativeLib__);
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#setInheritable(ImageBuildtimePosixSupport, int, boolean, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public void setInheritable(Object arg0Value_, int arg1Value, boolean arg2Value) throws PosixException {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
arg0Value.setInheritable(arg1Value, arg2Value, nativeLib__);
return;
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#pipe(ImageBuildtimePosixSupport, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public int[] pipe(Object arg0Value_) throws PosixException {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
return arg0Value.pipe(nativeLib__);
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#select(ImageBuildtimePosixSupport, int[], int[], int[], Timeval, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public SelectResult select(Object arg0Value_, int[] arg1Value, int[] arg2Value, int[] arg3Value, Timeval arg4Value) throws PosixException {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
return arg0Value.select(arg1Value, arg2Value, arg3Value, arg4Value, nativeLib__);
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#lseek(ImageBuildtimePosixSupport, int, long, int, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public long lseek(Object arg0Value_, int arg1Value, long arg2Value, int arg3Value) throws PosixException {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
return arg0Value.lseek(arg1Value, arg2Value, arg3Value, nativeLib__);
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#ftruncate(ImageBuildtimePosixSupport, int, long, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public void ftruncate(Object arg0Value_, int arg1Value, long arg2Value) throws PosixException {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
arg0Value.ftruncate(arg1Value, arg2Value, nativeLib__);
return;
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#fsync(ImageBuildtimePosixSupport, int, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public void fsync(Object arg0Value_, int arg1Value) throws PosixException {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
arg0Value.fsync(arg1Value, nativeLib__);
return;
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#flock(ImageBuildtimePosixSupport, int, int, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public void flock(Object arg0Value_, int arg1Value, int arg2Value) throws PosixException {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
arg0Value.flock(arg1Value, arg2Value, nativeLib__);
return;
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#getBlocking(ImageBuildtimePosixSupport, int, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public boolean getBlocking(Object arg0Value_, int arg1Value) throws PosixException {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
return arg0Value.getBlocking(arg1Value, nativeLib__);
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#setBlocking(ImageBuildtimePosixSupport, int, boolean, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public void setBlocking(Object arg0Value_, int arg1Value, boolean arg2Value) throws PosixException {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
arg0Value.setBlocking(arg1Value, arg2Value, nativeLib__);
return;
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#getTerminalSize(ImageBuildtimePosixSupport, int, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public int[] getTerminalSize(Object arg0Value_, int arg1Value) throws PosixException {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
return arg0Value.getTerminalSize(arg1Value, nativeLib__);
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#fstatat(ImageBuildtimePosixSupport, int, Object, boolean, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public long[] fstatat(Object arg0Value_, int arg1Value, Object arg2Value, boolean arg3Value) throws PosixException {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
return arg0Value.fstatat(arg1Value, arg2Value, arg3Value, nativeLib__);
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#fstat(ImageBuildtimePosixSupport, int, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public long[] fstat(Object arg0Value_, int arg1Value) throws PosixException {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
return arg0Value.fstat(arg1Value, nativeLib__);
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#statvfs(ImageBuildtimePosixSupport, Object, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public long[] statvfs(Object arg0Value_, Object arg1Value) throws PosixException {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
return arg0Value.statvfs(arg1Value, nativeLib__);
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#fstatvfs(ImageBuildtimePosixSupport, int, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public long[] fstatvfs(Object arg0Value_, int arg1Value) throws PosixException {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
return arg0Value.fstatvfs(arg1Value, nativeLib__);
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#uname(ImageBuildtimePosixSupport, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public Object[] uname(Object arg0Value_) throws PosixException {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
return arg0Value.uname(nativeLib__);
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#unlinkat(ImageBuildtimePosixSupport, int, Object, boolean, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public void unlinkat(Object arg0Value_, int arg1Value, Object arg2Value, boolean arg3Value) throws PosixException {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
arg0Value.unlinkat(arg1Value, arg2Value, arg3Value, nativeLib__);
return;
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#linkat(ImageBuildtimePosixSupport, int, Object, int, Object, int, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public void linkat(Object arg0Value_, int arg1Value, Object arg2Value, int arg3Value, Object arg4Value, int arg5Value) throws PosixException {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
arg0Value.linkat(arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, nativeLib__);
return;
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#symlinkat(ImageBuildtimePosixSupport, Object, int, Object, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public void symlinkat(Object arg0Value_, Object arg1Value, int arg2Value, Object arg3Value) throws PosixException {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
arg0Value.symlinkat(arg1Value, arg2Value, arg3Value, nativeLib__);
return;
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#mkdirat(ImageBuildtimePosixSupport, int, Object, int, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public void mkdirat(Object arg0Value_, int arg1Value, Object arg2Value, int arg3Value) throws PosixException {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
arg0Value.mkdirat(arg1Value, arg2Value, arg3Value, nativeLib__);
return;
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#getcwd(ImageBuildtimePosixSupport, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public Object getcwd(Object arg0Value_) throws PosixException {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
return arg0Value.getcwd(nativeLib__);
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#chdir(ImageBuildtimePosixSupport, Object, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public void chdir(Object arg0Value_, Object arg1Value) throws PosixException {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
arg0Value.chdir(arg1Value, nativeLib__);
return;
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#fchdir(ImageBuildtimePosixSupport, int, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public void fchdir(Object arg0Value_, int arg1Value) throws PosixException {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
arg0Value.fchdir(arg1Value, nativeLib__);
return;
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#isatty(ImageBuildtimePosixSupport, int, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public boolean isatty(Object arg0Value_, int arg1Value) {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
return arg0Value.isatty(arg1Value, nativeLib__);
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#opendir(ImageBuildtimePosixSupport, Object, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public Object opendir(Object arg0Value_, Object arg1Value) throws PosixException {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
return arg0Value.opendir(arg1Value, nativeLib__);
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#fdopendir(ImageBuildtimePosixSupport, int, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public Object fdopendir(Object arg0Value_, int arg1Value) throws PosixException {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
return arg0Value.fdopendir(arg1Value, nativeLib__);
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#closedir(ImageBuildtimePosixSupport, Object, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public void closedir(Object arg0Value_, Object arg1Value) throws PosixException {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
arg0Value.closedir(arg1Value, nativeLib__);
return;
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#readdir(ImageBuildtimePosixSupport, Object, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public Object readdir(Object arg0Value_, Object arg1Value) throws PosixException {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
return arg0Value.readdir(arg1Value, nativeLib__);
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#rewinddir(ImageBuildtimePosixSupport, Object, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public void rewinddir(Object arg0Value_, Object arg1Value) {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
arg0Value.rewinddir(arg1Value, nativeLib__);
return;
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#dirEntryGetName(ImageBuildtimePosixSupport, Object, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public Object dirEntryGetName(Object arg0Value_, Object arg1Value) throws PosixException {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
return arg0Value.dirEntryGetName(arg1Value, nativeLib__);
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#dirEntryGetPath(ImageBuildtimePosixSupport, Object, Object, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public Object dirEntryGetPath(Object arg0Value_, Object arg1Value, Object arg2Value) throws PosixException {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
return arg0Value.dirEntryGetPath(arg1Value, arg2Value, nativeLib__);
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#dirEntryGetInode(ImageBuildtimePosixSupport, Object, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public long dirEntryGetInode(Object arg0Value_, Object arg1Value) throws PosixException {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
return arg0Value.dirEntryGetInode(arg1Value, nativeLib__);
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#dirEntryGetType(ImageBuildtimePosixSupport, Object, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public int dirEntryGetType(Object arg0Value_, Object arg1Value) {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
return arg0Value.dirEntryGetType(arg1Value, nativeLib__);
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#utimensat(ImageBuildtimePosixSupport, int, Object, long[], boolean, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public void utimensat(Object arg0Value_, int arg1Value, Object arg2Value, long[] arg3Value, boolean arg4Value) throws PosixException {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
arg0Value.utimensat(arg1Value, arg2Value, arg3Value, arg4Value, nativeLib__);
return;
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#futimens(ImageBuildtimePosixSupport, int, long[], PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public void futimens(Object arg0Value_, int arg1Value, long[] arg2Value) throws PosixException {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
arg0Value.futimens(arg1Value, arg2Value, nativeLib__);
return;
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#futimes(ImageBuildtimePosixSupport, int, Timeval[], PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public void futimes(Object arg0Value_, int arg1Value, Timeval[] arg2Value) throws PosixException {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
arg0Value.futimes(arg1Value, arg2Value, nativeLib__);
return;
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#lutimes(ImageBuildtimePosixSupport, Object, Timeval[], PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public void lutimes(Object arg0Value_, Object arg1Value, Timeval[] arg2Value) throws PosixException {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
arg0Value.lutimes(arg1Value, arg2Value, nativeLib__);
return;
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#utimes(ImageBuildtimePosixSupport, Object, Timeval[], PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public void utimes(Object arg0Value_, Object arg1Value, Timeval[] arg2Value) throws PosixException {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
arg0Value.utimes(arg1Value, arg2Value, nativeLib__);
return;
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#renameat(ImageBuildtimePosixSupport, int, Object, int, Object, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public void renameat(Object arg0Value_, int arg1Value, Object arg2Value, int arg3Value, Object arg4Value) throws PosixException {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
arg0Value.renameat(arg1Value, arg2Value, arg3Value, arg4Value, nativeLib__);
return;
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#faccessat(ImageBuildtimePosixSupport, int, Object, int, boolean, boolean, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public boolean faccessat(Object arg0Value_, int arg1Value, Object arg2Value, int arg3Value, boolean arg4Value, boolean arg5Value) {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
return arg0Value.faccessat(arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, nativeLib__);
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#fchmodat(ImageBuildtimePosixSupport, int, Object, int, boolean, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public void fchmodat(Object arg0Value_, int arg1Value, Object arg2Value, int arg3Value, boolean arg4Value) throws PosixException {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
arg0Value.fchmodat(arg1Value, arg2Value, arg3Value, arg4Value, nativeLib__);
return;
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#fchmod(ImageBuildtimePosixSupport, int, int, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public void fchmod(Object arg0Value_, int arg1Value, int arg2Value) throws PosixException {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
arg0Value.fchmod(arg1Value, arg2Value, nativeLib__);
return;
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#readlinkat(ImageBuildtimePosixSupport, int, Object, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public Object readlinkat(Object arg0Value_, int arg1Value, Object arg2Value) throws PosixException {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
return arg0Value.readlinkat(arg1Value, arg2Value, nativeLib__);
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#kill(ImageBuildtimePosixSupport, long, int, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public void kill(Object arg0Value_, long arg1Value, int arg2Value) throws PosixException {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
arg0Value.kill(arg1Value, arg2Value, nativeLib__);
return;
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#killpg(ImageBuildtimePosixSupport, long, int, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public void killpg(Object arg0Value_, long arg1Value, int arg2Value) throws PosixException {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
arg0Value.killpg(arg1Value, arg2Value, nativeLib__);
return;
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#waitpid(ImageBuildtimePosixSupport, long, int, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public long[] waitpid(Object arg0Value_, long arg1Value, int arg2Value) throws PosixException {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
return arg0Value.waitpid(arg1Value, arg2Value, nativeLib__);
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#abort(ImageBuildtimePosixSupport, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public void abort(Object arg0Value_) {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
arg0Value.abort(nativeLib__);
return;
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#wcoredump(ImageBuildtimePosixSupport, int, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public boolean wcoredump(Object arg0Value_, int arg1Value) {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
return arg0Value.wcoredump(arg1Value, nativeLib__);
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#wifcontinued(ImageBuildtimePosixSupport, int, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public boolean wifcontinued(Object arg0Value_, int arg1Value) {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
return arg0Value.wifcontinued(arg1Value, nativeLib__);
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#wifstopped(ImageBuildtimePosixSupport, int, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public boolean wifstopped(Object arg0Value_, int arg1Value) {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
return arg0Value.wifstopped(arg1Value, nativeLib__);
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#wifsignaled(ImageBuildtimePosixSupport, int, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public boolean wifsignaled(Object arg0Value_, int arg1Value) {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
return arg0Value.wifsignaled(arg1Value, nativeLib__);
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#wifexited(ImageBuildtimePosixSupport, int, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public boolean wifexited(Object arg0Value_, int arg1Value) {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
return arg0Value.wifexited(arg1Value, nativeLib__);
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#wexitstatus(ImageBuildtimePosixSupport, int, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public int wexitstatus(Object arg0Value_, int arg1Value) {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
return arg0Value.wexitstatus(arg1Value, nativeLib__);
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#wtermsig(ImageBuildtimePosixSupport, int, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public int wtermsig(Object arg0Value_, int arg1Value) {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
return arg0Value.wtermsig(arg1Value, nativeLib__);
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#wstopsig(ImageBuildtimePosixSupport, int, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public int wstopsig(Object arg0Value_, int arg1Value) {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
return arg0Value.wstopsig(arg1Value, nativeLib__);
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#getuid(ImageBuildtimePosixSupport, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public long getuid(Object arg0Value_) {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
return arg0Value.getuid(nativeLib__);
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#geteuid(ImageBuildtimePosixSupport, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public long geteuid(Object arg0Value_) {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
return arg0Value.geteuid(nativeLib__);
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#getgid(ImageBuildtimePosixSupport, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public long getgid(Object arg0Value_) {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
return arg0Value.getgid(nativeLib__);
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#getppid(ImageBuildtimePosixSupport, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public long getppid(Object arg0Value_) {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
return arg0Value.getppid(nativeLib__);
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#getpgid(ImageBuildtimePosixSupport, long, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public long getpgid(Object arg0Value_, long arg1Value) throws PosixException {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
return arg0Value.getpgid(arg1Value, nativeLib__);
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#setpgid(ImageBuildtimePosixSupport, long, long, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public void setpgid(Object arg0Value_, long arg1Value, long arg2Value) throws PosixException {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
arg0Value.setpgid(arg1Value, arg2Value, nativeLib__);
return;
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#getpgrp(ImageBuildtimePosixSupport, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public long getpgrp(Object arg0Value_) {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
return arg0Value.getpgrp(nativeLib__);
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#getsid(ImageBuildtimePosixSupport, long, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public long getsid(Object arg0Value_, long arg1Value) throws PosixException {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
return arg0Value.getsid(arg1Value, nativeLib__);
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#setsid(ImageBuildtimePosixSupport, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public long setsid(Object arg0Value_) throws PosixException {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
return arg0Value.setsid(nativeLib__);
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#openpty(ImageBuildtimePosixSupport, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public OpenPtyResult openpty(Object arg0Value_) throws PosixException {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
return arg0Value.openpty(nativeLib__);
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#ctermid(ImageBuildtimePosixSupport, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public TruffleString ctermid(Object arg0Value_) throws PosixException {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
return arg0Value.ctermid(nativeLib__);
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#setenv(ImageBuildtimePosixSupport, Object, Object, boolean, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public void setenv(Object arg0Value_, Object arg1Value, Object arg2Value, boolean arg3Value) throws PosixException {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
arg0Value.setenv(arg1Value, arg2Value, arg3Value, nativeLib__);
return;
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#unsetenv(ImageBuildtimePosixSupport, Object, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public void unsetenv(Object arg0Value_, Object arg1Value) throws PosixException {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
arg0Value.unsetenv(arg1Value, nativeLib__);
return;
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#forkExec(ImageBuildtimePosixSupport, Object[], Object[], Object, Object[], int, int, int, int, int, int, int, int, boolean, boolean, boolean, int[], PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public int forkExec(Object arg0Value_, Object[] arg1Value, Object[] arg2Value, Object arg3Value, Object[] arg4Value, int arg5Value, int arg6Value, int arg7Value, int arg8Value, int arg9Value, int arg10Value, int arg11Value, int arg12Value, boolean arg13Value, boolean arg14Value, boolean arg15Value, int[] arg16Value) throws PosixException {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
return arg0Value.forkExec(arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, arg6Value, arg7Value, arg8Value, arg9Value, arg10Value, arg11Value, arg12Value, arg13Value, arg14Value, arg15Value, arg16Value, nativeLib__);
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#execv(ImageBuildtimePosixSupport, Object, Object[], PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public void execv(Object arg0Value_, Object arg1Value, Object[] arg2Value) throws PosixException {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
arg0Value.execv(arg1Value, arg2Value, nativeLib__);
return;
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#system(ImageBuildtimePosixSupport, Object, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public int system(Object arg0Value_, Object arg1Value) {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
return arg0Value.system(arg1Value, nativeLib__);
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#mmap(ImageBuildtimePosixSupport, long, int, int, int, long, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public Object mmap(Object arg0Value_, long arg1Value, int arg2Value, int arg3Value, int arg4Value, long arg5Value) throws PosixException {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
return arg0Value.mmap(arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, nativeLib__);
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#mmapReadByte(ImageBuildtimePosixSupport, Object, long, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public byte mmapReadByte(Object arg0Value_, Object arg1Value, long arg2Value) throws PosixException {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
return arg0Value.mmapReadByte(arg1Value, arg2Value, nativeLib__);
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#mmapWriteByte(ImageBuildtimePosixSupport, Object, long, byte, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public void mmapWriteByte(Object arg0Value_, Object arg1Value, long arg2Value, byte arg3Value) throws PosixException {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
arg0Value.mmapWriteByte(arg1Value, arg2Value, arg3Value, nativeLib__);
return;
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#mmapReadBytes(ImageBuildtimePosixSupport, Object, long, byte[], int, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public int mmapReadBytes(Object arg0Value_, Object arg1Value, long arg2Value, byte[] arg3Value, int arg4Value) throws PosixException {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
return arg0Value.mmapReadBytes(arg1Value, arg2Value, arg3Value, arg4Value, nativeLib__);
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#mmapWriteBytes(ImageBuildtimePosixSupport, Object, long, byte[], int, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public void mmapWriteBytes(Object arg0Value_, Object arg1Value, long arg2Value, byte[] arg3Value, int arg4Value) throws PosixException {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
arg0Value.mmapWriteBytes(arg1Value, arg2Value, arg3Value, arg4Value, nativeLib__);
return;
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#mmapFlush(ImageBuildtimePosixSupport, Object, long, long, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public void mmapFlush(Object arg0Value_, Object arg1Value, long arg2Value, long arg3Value) throws PosixException {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
arg0Value.mmapFlush(arg1Value, arg2Value, arg3Value, nativeLib__);
return;
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#mmapUnmap(ImageBuildtimePosixSupport, Object, long, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public void mmapUnmap(Object arg0Value_, Object arg1Value, long arg2Value) throws PosixException {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
arg0Value.mmapUnmap(arg1Value, arg2Value, nativeLib__);
return;
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#mmapGetPointer(ImageBuildtimePosixSupport, Object, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public long mmapGetPointer(Object arg0Value_, Object arg1Value) {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
return arg0Value.mmapGetPointer(arg1Value, nativeLib__);
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#getpwuid(ImageBuildtimePosixSupport, long, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public PwdResult getpwuid(Object arg0Value_, long arg1Value) throws PosixException {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
return arg0Value.getpwuid(arg1Value, nativeLib__);
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#getpwnam(ImageBuildtimePosixSupport, Object, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public PwdResult getpwnam(Object arg0Value_, Object arg1Value) throws PosixException {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
return arg0Value.getpwnam(arg1Value, nativeLib__);
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#hasGetpwentries(ImageBuildtimePosixSupport, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public boolean hasGetpwentries(Object arg0Value_) {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
return arg0Value.hasGetpwentries(nativeLib__);
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#getpwentries(ImageBuildtimePosixSupport, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public PwdResult[] getpwentries(Object arg0Value_) throws PosixException {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
return arg0Value.getpwentries(nativeLib__);
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#socket(ImageBuildtimePosixSupport, int, int, int, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public int socket(Object arg0Value_, int arg1Value, int arg2Value, int arg3Value) throws PosixException {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
return arg0Value.socket(arg1Value, arg2Value, arg3Value, nativeLib__);
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#accept(ImageBuildtimePosixSupport, int, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public AcceptResult accept(Object arg0Value_, int arg1Value) throws PosixException {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
return arg0Value.accept(arg1Value, nativeLib__);
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#bind(ImageBuildtimePosixSupport, int, UniversalSockAddr, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public void bind(Object arg0Value_, int arg1Value, UniversalSockAddr arg2Value) throws PosixException {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
arg0Value.bind(arg1Value, arg2Value, nativeLib__);
return;
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#connect(ImageBuildtimePosixSupport, int, UniversalSockAddr, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public void connect(Object arg0Value_, int arg1Value, UniversalSockAddr arg2Value) throws PosixException {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
arg0Value.connect(arg1Value, arg2Value, nativeLib__);
return;
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#listen(ImageBuildtimePosixSupport, int, int, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public void listen(Object arg0Value_, int arg1Value, int arg2Value) throws PosixException {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
arg0Value.listen(arg1Value, arg2Value, nativeLib__);
return;
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#getpeername(ImageBuildtimePosixSupport, int, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public UniversalSockAddr getpeername(Object arg0Value_, int arg1Value) throws PosixException {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
return arg0Value.getpeername(arg1Value, nativeLib__);
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#getsockname(ImageBuildtimePosixSupport, int, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public UniversalSockAddr getsockname(Object arg0Value_, int arg1Value) throws PosixException {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
return arg0Value.getsockname(arg1Value, nativeLib__);
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#send(ImageBuildtimePosixSupport, int, byte[], int, int, int, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public int send(Object arg0Value_, int arg1Value, byte[] arg2Value, int arg3Value, int arg4Value, int arg5Value) throws PosixException {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
return arg0Value.send(arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, nativeLib__);
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#sendto(ImageBuildtimePosixSupport, int, byte[], int, int, int, UniversalSockAddr, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public int sendto(Object arg0Value_, int arg1Value, byte[] arg2Value, int arg3Value, int arg4Value, int arg5Value, UniversalSockAddr arg6Value) throws PosixException {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
return arg0Value.sendto(arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, arg6Value, nativeLib__);
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#recv(ImageBuildtimePosixSupport, int, byte[], int, int, int, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public int recv(Object arg0Value_, int arg1Value, byte[] arg2Value, int arg3Value, int arg4Value, int arg5Value) throws PosixException {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
return arg0Value.recv(arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, nativeLib__);
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#recvfrom(ImageBuildtimePosixSupport, int, byte[], int, int, int, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public RecvfromResult recvfrom(Object arg0Value_, int arg1Value, byte[] arg2Value, int arg3Value, int arg4Value, int arg5Value) throws PosixException {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
return arg0Value.recvfrom(arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, nativeLib__);
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#shutdown(ImageBuildtimePosixSupport, int, int, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public void shutdown(Object arg0Value_, int arg1Value, int arg2Value) throws PosixException {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
arg0Value.shutdown(arg1Value, arg2Value, nativeLib__);
return;
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#getsockopt(ImageBuildtimePosixSupport, int, int, int, byte[], int, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public int getsockopt(Object arg0Value_, int arg1Value, int arg2Value, int arg3Value, byte[] arg4Value, int arg5Value) throws PosixException {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
return arg0Value.getsockopt(arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, nativeLib__);
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#setsockopt(ImageBuildtimePosixSupport, int, int, int, byte[], int, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public void setsockopt(Object arg0Value_, int arg1Value, int arg2Value, int arg3Value, byte[] arg4Value, int arg5Value) throws PosixException {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
arg0Value.setsockopt(arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, nativeLib__);
return;
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#inet_addr(ImageBuildtimePosixSupport, Object, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public int inet_addr(Object arg0Value_, Object arg1Value) {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
return arg0Value.inet_addr(arg1Value, nativeLib__);
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#inet_aton(ImageBuildtimePosixSupport, Object, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public int inet_aton(Object arg0Value_, Object arg1Value) throws InvalidAddressException {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
return arg0Value.inet_aton(arg1Value, nativeLib__);
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#inet_ntoa(ImageBuildtimePosixSupport, int, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public Object inet_ntoa(Object arg0Value_, int arg1Value) {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
return arg0Value.inet_ntoa(arg1Value, nativeLib__);
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#inet_pton(ImageBuildtimePosixSupport, int, Object, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public byte[] inet_pton(Object arg0Value_, int arg1Value, Object arg2Value) throws PosixException, InvalidAddressException {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
return arg0Value.inet_pton(arg1Value, arg2Value, nativeLib__);
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#inet_ntop(ImageBuildtimePosixSupport, int, byte[], PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public Object inet_ntop(Object arg0Value_, int arg1Value, byte[] arg2Value) throws PosixException {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
return arg0Value.inet_ntop(arg1Value, arg2Value, nativeLib__);
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#gethostname(ImageBuildtimePosixSupport, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public Object gethostname(Object arg0Value_) throws PosixException {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
return arg0Value.gethostname(nativeLib__);
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#getnameinfo(ImageBuildtimePosixSupport, UniversalSockAddr, int, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public Object[] getnameinfo(Object arg0Value_, UniversalSockAddr arg1Value, int arg2Value) throws GetAddrInfoException {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
return arg0Value.getnameinfo(arg1Value, arg2Value, nativeLib__);
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#getaddrinfo(ImageBuildtimePosixSupport, Object, Object, int, int, int, int, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public AddrInfoCursor getaddrinfo(Object arg0Value_, Object arg1Value, Object arg2Value, int arg3Value, int arg4Value, int arg5Value, int arg6Value) throws GetAddrInfoException {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
return arg0Value.getaddrinfo(arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, arg6Value, nativeLib__);
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#crypt(ImageBuildtimePosixSupport, TruffleString, TruffleString, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public TruffleString crypt(Object arg0Value_, TruffleString arg1Value, TruffleString arg2Value) throws PosixException {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
return arg0Value.crypt(arg1Value, arg2Value, nativeLib__);
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#createUniversalSockAddr(ImageBuildtimePosixSupport, FamilySpecificSockAddr, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public UniversalSockAddr createUniversalSockAddr(Object arg0Value_, FamilySpecificSockAddr arg1Value) {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
return arg0Value.createUniversalSockAddr(arg1Value, nativeLib__);
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#createPathFromString(ImageBuildtimePosixSupport, TruffleString, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public Object createPathFromString(Object arg0Value_, TruffleString arg1Value) {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
return arg0Value.createPathFromString(arg1Value, nativeLib__);
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#createPathFromBytes(ImageBuildtimePosixSupport, byte[], PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public Object createPathFromBytes(Object arg0Value_, byte[] arg1Value) {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
return arg0Value.createPathFromBytes(arg1Value, nativeLib__);
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#getPathAsString(ImageBuildtimePosixSupport, Object, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public TruffleString getPathAsString(Object arg0Value_, Object arg1Value) {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
return arg0Value.getPathAsString(arg1Value, nativeLib__);
}
}
/**
* Debug Info:
* Specialization {@link ImageBuildtimePosixSupport#getPathAsBytes(ImageBuildtimePosixSupport, Object, PosixSupportLibrary)}
* Activation probability: 0.00826
* With/without class size: 4/0 bytes
*
*/
@Override
public Buffer getPathAsBytes(Object arg0Value_, Object arg1Value) {
assert CompilerDirectives.isExact(arg0Value_, this.receiverClass_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
{
PosixSupportLibrary nativeLib__ = this.receiverNativePosixSupportPosixSupportLibrary_;
return arg0Value.getPathAsBytes(arg1Value, nativeLib__);
}
}
}
@GeneratedBy(ImageBuildtimePosixSupport.class)
public static class Uncached extends PosixSupportLibrary {
private final Class extends ImageBuildtimePosixSupport> receiverClass_;
protected Uncached(Object receiver) {
this.receiverClass_ = ((ImageBuildtimePosixSupport) receiver).getClass();
}
@Override
@TruffleBoundary
public boolean accepts(Object receiver) {
assert receiver.getClass() != this.receiverClass_ || DYNAMIC_DISPATCH_LIBRARY_.getUncached().dispatch(receiver) == null : "Invalid library export. Exported receiver with dynamic dispatch found but not expected.";
return CompilerDirectives.isExact(receiver, this.receiverClass_);
}
@Override
public final boolean isAdoptable() {
return false;
}
@Override
public final NodeCost getCost() {
return NodeCost.MEGAMORPHIC;
}
@TruffleBoundary
@Override
public TruffleString getBackend(Object arg0Value_) {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
return arg0Value.getBackend(POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
}
@TruffleBoundary
@Override
public TruffleString strerror(Object arg0Value_, int arg1Value) {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
return arg0Value.strerror(arg1Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
}
@TruffleBoundary
@Override
public long getpid(Object arg0Value_) {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
return arg0Value.getpid(POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
}
@TruffleBoundary
@Override
public int umask(Object arg0Value_, int arg1Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
return arg0Value.umask(arg1Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
}
@TruffleBoundary
@Override
public int openat(Object arg0Value_, int arg1Value, Object arg2Value, int arg3Value, int arg4Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
return arg0Value.openat(arg1Value, arg2Value, arg3Value, arg4Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
}
@TruffleBoundary
@Override
public int close(Object arg0Value_, int arg1Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
return arg0Value.close(arg1Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
}
@TruffleBoundary
@Override
public Buffer read(Object arg0Value_, int arg1Value, long arg2Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
return arg0Value.read(arg1Value, arg2Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
}
@TruffleBoundary
@Override
public long write(Object arg0Value_, int arg1Value, Buffer arg2Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
return arg0Value.write(arg1Value, arg2Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
}
@TruffleBoundary
@Override
public int dup(Object arg0Value_, int arg1Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
return arg0Value.dup(arg1Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
}
@TruffleBoundary
@Override
public int dup2(Object arg0Value_, int arg1Value, int arg2Value, boolean arg3Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
return arg0Value.dup2(arg1Value, arg2Value, arg3Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
}
@TruffleBoundary
@Override
public boolean getInheritable(Object arg0Value_, int arg1Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
return arg0Value.getInheritable(arg1Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
}
@TruffleBoundary
@Override
public void setInheritable(Object arg0Value_, int arg1Value, boolean arg2Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
arg0Value.setInheritable(arg1Value, arg2Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
return;
}
@TruffleBoundary
@Override
public int[] pipe(Object arg0Value_) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
return arg0Value.pipe(POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
}
@TruffleBoundary
@Override
public SelectResult select(Object arg0Value_, int[] arg1Value, int[] arg2Value, int[] arg3Value, Timeval arg4Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
return arg0Value.select(arg1Value, arg2Value, arg3Value, arg4Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
}
@TruffleBoundary
@Override
public long lseek(Object arg0Value_, int arg1Value, long arg2Value, int arg3Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
return arg0Value.lseek(arg1Value, arg2Value, arg3Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
}
@TruffleBoundary
@Override
public void ftruncate(Object arg0Value_, int arg1Value, long arg2Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
arg0Value.ftruncate(arg1Value, arg2Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
return;
}
@TruffleBoundary
@Override
public void fsync(Object arg0Value_, int arg1Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
arg0Value.fsync(arg1Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
return;
}
@TruffleBoundary
@Override
public void flock(Object arg0Value_, int arg1Value, int arg2Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
arg0Value.flock(arg1Value, arg2Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
return;
}
@TruffleBoundary
@Override
public boolean getBlocking(Object arg0Value_, int arg1Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
return arg0Value.getBlocking(arg1Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
}
@TruffleBoundary
@Override
public void setBlocking(Object arg0Value_, int arg1Value, boolean arg2Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
arg0Value.setBlocking(arg1Value, arg2Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
return;
}
@TruffleBoundary
@Override
public int[] getTerminalSize(Object arg0Value_, int arg1Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
return arg0Value.getTerminalSize(arg1Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
}
@TruffleBoundary
@Override
public long[] fstatat(Object arg0Value_, int arg1Value, Object arg2Value, boolean arg3Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
return arg0Value.fstatat(arg1Value, arg2Value, arg3Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
}
@TruffleBoundary
@Override
public long[] fstat(Object arg0Value_, int arg1Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
return arg0Value.fstat(arg1Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
}
@TruffleBoundary
@Override
public long[] statvfs(Object arg0Value_, Object arg1Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
return arg0Value.statvfs(arg1Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
}
@TruffleBoundary
@Override
public long[] fstatvfs(Object arg0Value_, int arg1Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
return arg0Value.fstatvfs(arg1Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
}
@TruffleBoundary
@Override
public Object[] uname(Object arg0Value_) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
return arg0Value.uname(POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
}
@TruffleBoundary
@Override
public void unlinkat(Object arg0Value_, int arg1Value, Object arg2Value, boolean arg3Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
arg0Value.unlinkat(arg1Value, arg2Value, arg3Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
return;
}
@TruffleBoundary
@Override
public void linkat(Object arg0Value_, int arg1Value, Object arg2Value, int arg3Value, Object arg4Value, int arg5Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
arg0Value.linkat(arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
return;
}
@TruffleBoundary
@Override
public void symlinkat(Object arg0Value_, Object arg1Value, int arg2Value, Object arg3Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
arg0Value.symlinkat(arg1Value, arg2Value, arg3Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
return;
}
@TruffleBoundary
@Override
public void mkdirat(Object arg0Value_, int arg1Value, Object arg2Value, int arg3Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
arg0Value.mkdirat(arg1Value, arg2Value, arg3Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
return;
}
@TruffleBoundary
@Override
public Object getcwd(Object arg0Value_) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
return arg0Value.getcwd(POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
}
@TruffleBoundary
@Override
public void chdir(Object arg0Value_, Object arg1Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
arg0Value.chdir(arg1Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
return;
}
@TruffleBoundary
@Override
public void fchdir(Object arg0Value_, int arg1Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
arg0Value.fchdir(arg1Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
return;
}
@TruffleBoundary
@Override
public boolean isatty(Object arg0Value_, int arg1Value) {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
return arg0Value.isatty(arg1Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
}
@TruffleBoundary
@Override
public Object opendir(Object arg0Value_, Object arg1Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
return arg0Value.opendir(arg1Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
}
@TruffleBoundary
@Override
public Object fdopendir(Object arg0Value_, int arg1Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
return arg0Value.fdopendir(arg1Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
}
@TruffleBoundary
@Override
public void closedir(Object arg0Value_, Object arg1Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
arg0Value.closedir(arg1Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
return;
}
@TruffleBoundary
@Override
public Object readdir(Object arg0Value_, Object arg1Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
return arg0Value.readdir(arg1Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
}
@TruffleBoundary
@Override
public void rewinddir(Object arg0Value_, Object arg1Value) {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
arg0Value.rewinddir(arg1Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
return;
}
@TruffleBoundary
@Override
public Object dirEntryGetName(Object arg0Value_, Object arg1Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
return arg0Value.dirEntryGetName(arg1Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
}
@TruffleBoundary
@Override
public Object dirEntryGetPath(Object arg0Value_, Object arg1Value, Object arg2Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
return arg0Value.dirEntryGetPath(arg1Value, arg2Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
}
@TruffleBoundary
@Override
public long dirEntryGetInode(Object arg0Value_, Object arg1Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
return arg0Value.dirEntryGetInode(arg1Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
}
@TruffleBoundary
@Override
public int dirEntryGetType(Object arg0Value_, Object arg1Value) {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
return arg0Value.dirEntryGetType(arg1Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
}
@TruffleBoundary
@Override
public void utimensat(Object arg0Value_, int arg1Value, Object arg2Value, long[] arg3Value, boolean arg4Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
arg0Value.utimensat(arg1Value, arg2Value, arg3Value, arg4Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
return;
}
@TruffleBoundary
@Override
public void futimens(Object arg0Value_, int arg1Value, long[] arg2Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
arg0Value.futimens(arg1Value, arg2Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
return;
}
@TruffleBoundary
@Override
public void futimes(Object arg0Value_, int arg1Value, Timeval[] arg2Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
arg0Value.futimes(arg1Value, arg2Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
return;
}
@TruffleBoundary
@Override
public void lutimes(Object arg0Value_, Object arg1Value, Timeval[] arg2Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
arg0Value.lutimes(arg1Value, arg2Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
return;
}
@TruffleBoundary
@Override
public void utimes(Object arg0Value_, Object arg1Value, Timeval[] arg2Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
arg0Value.utimes(arg1Value, arg2Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
return;
}
@TruffleBoundary
@Override
public void renameat(Object arg0Value_, int arg1Value, Object arg2Value, int arg3Value, Object arg4Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
arg0Value.renameat(arg1Value, arg2Value, arg3Value, arg4Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
return;
}
@TruffleBoundary
@Override
public boolean faccessat(Object arg0Value_, int arg1Value, Object arg2Value, int arg3Value, boolean arg4Value, boolean arg5Value) {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
return arg0Value.faccessat(arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
}
@TruffleBoundary
@Override
public void fchmodat(Object arg0Value_, int arg1Value, Object arg2Value, int arg3Value, boolean arg4Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
arg0Value.fchmodat(arg1Value, arg2Value, arg3Value, arg4Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
return;
}
@TruffleBoundary
@Override
public void fchmod(Object arg0Value_, int arg1Value, int arg2Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
arg0Value.fchmod(arg1Value, arg2Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
return;
}
@TruffleBoundary
@Override
public Object readlinkat(Object arg0Value_, int arg1Value, Object arg2Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
return arg0Value.readlinkat(arg1Value, arg2Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
}
@TruffleBoundary
@Override
public void kill(Object arg0Value_, long arg1Value, int arg2Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
arg0Value.kill(arg1Value, arg2Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
return;
}
@TruffleBoundary
@Override
public void killpg(Object arg0Value_, long arg1Value, int arg2Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
arg0Value.killpg(arg1Value, arg2Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
return;
}
@TruffleBoundary
@Override
public long[] waitpid(Object arg0Value_, long arg1Value, int arg2Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
return arg0Value.waitpid(arg1Value, arg2Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
}
@TruffleBoundary
@Override
public void abort(Object arg0Value_) {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
arg0Value.abort(POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
return;
}
@TruffleBoundary
@Override
public boolean wcoredump(Object arg0Value_, int arg1Value) {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
return arg0Value.wcoredump(arg1Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
}
@TruffleBoundary
@Override
public boolean wifcontinued(Object arg0Value_, int arg1Value) {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
return arg0Value.wifcontinued(arg1Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
}
@TruffleBoundary
@Override
public boolean wifstopped(Object arg0Value_, int arg1Value) {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
return arg0Value.wifstopped(arg1Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
}
@TruffleBoundary
@Override
public boolean wifsignaled(Object arg0Value_, int arg1Value) {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
return arg0Value.wifsignaled(arg1Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
}
@TruffleBoundary
@Override
public boolean wifexited(Object arg0Value_, int arg1Value) {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
return arg0Value.wifexited(arg1Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
}
@TruffleBoundary
@Override
public int wexitstatus(Object arg0Value_, int arg1Value) {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
return arg0Value.wexitstatus(arg1Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
}
@TruffleBoundary
@Override
public int wtermsig(Object arg0Value_, int arg1Value) {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
return arg0Value.wtermsig(arg1Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
}
@TruffleBoundary
@Override
public int wstopsig(Object arg0Value_, int arg1Value) {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
return arg0Value.wstopsig(arg1Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
}
@TruffleBoundary
@Override
public long getuid(Object arg0Value_) {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
return arg0Value.getuid(POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
}
@TruffleBoundary
@Override
public long geteuid(Object arg0Value_) {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
return arg0Value.geteuid(POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
}
@TruffleBoundary
@Override
public long getgid(Object arg0Value_) {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
return arg0Value.getgid(POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
}
@TruffleBoundary
@Override
public long getppid(Object arg0Value_) {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
return arg0Value.getppid(POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
}
@TruffleBoundary
@Override
public long getpgid(Object arg0Value_, long arg1Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
return arg0Value.getpgid(arg1Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
}
@TruffleBoundary
@Override
public void setpgid(Object arg0Value_, long arg1Value, long arg2Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
arg0Value.setpgid(arg1Value, arg2Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
return;
}
@TruffleBoundary
@Override
public long getpgrp(Object arg0Value_) {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
return arg0Value.getpgrp(POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
}
@TruffleBoundary
@Override
public long getsid(Object arg0Value_, long arg1Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
return arg0Value.getsid(arg1Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
}
@TruffleBoundary
@Override
public long setsid(Object arg0Value_) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
return arg0Value.setsid(POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
}
@TruffleBoundary
@Override
public OpenPtyResult openpty(Object arg0Value_) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
return arg0Value.openpty(POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
}
@TruffleBoundary
@Override
public TruffleString ctermid(Object arg0Value_) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
return arg0Value.ctermid(POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
}
@TruffleBoundary
@Override
public void setenv(Object arg0Value_, Object arg1Value, Object arg2Value, boolean arg3Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
arg0Value.setenv(arg1Value, arg2Value, arg3Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
return;
}
@TruffleBoundary
@Override
public void unsetenv(Object arg0Value_, Object arg1Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
arg0Value.unsetenv(arg1Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
return;
}
@TruffleBoundary
@Override
public int forkExec(Object arg0Value_, Object[] arg1Value, Object[] arg2Value, Object arg3Value, Object[] arg4Value, int arg5Value, int arg6Value, int arg7Value, int arg8Value, int arg9Value, int arg10Value, int arg11Value, int arg12Value, boolean arg13Value, boolean arg14Value, boolean arg15Value, int[] arg16Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
return arg0Value.forkExec(arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, arg6Value, arg7Value, arg8Value, arg9Value, arg10Value, arg11Value, arg12Value, arg13Value, arg14Value, arg15Value, arg16Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
}
@TruffleBoundary
@Override
public void execv(Object arg0Value_, Object arg1Value, Object[] arg2Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
arg0Value.execv(arg1Value, arg2Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
return;
}
@TruffleBoundary
@Override
public int system(Object arg0Value_, Object arg1Value) {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
return arg0Value.system(arg1Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
}
@TruffleBoundary
@Override
public Object mmap(Object arg0Value_, long arg1Value, int arg2Value, int arg3Value, int arg4Value, long arg5Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
return arg0Value.mmap(arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
}
@TruffleBoundary
@Override
public byte mmapReadByte(Object arg0Value_, Object arg1Value, long arg2Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
return arg0Value.mmapReadByte(arg1Value, arg2Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
}
@TruffleBoundary
@Override
public void mmapWriteByte(Object arg0Value_, Object arg1Value, long arg2Value, byte arg3Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
arg0Value.mmapWriteByte(arg1Value, arg2Value, arg3Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
return;
}
@TruffleBoundary
@Override
public int mmapReadBytes(Object arg0Value_, Object arg1Value, long arg2Value, byte[] arg3Value, int arg4Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
return arg0Value.mmapReadBytes(arg1Value, arg2Value, arg3Value, arg4Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
}
@TruffleBoundary
@Override
public void mmapWriteBytes(Object arg0Value_, Object arg1Value, long arg2Value, byte[] arg3Value, int arg4Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
arg0Value.mmapWriteBytes(arg1Value, arg2Value, arg3Value, arg4Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
return;
}
@TruffleBoundary
@Override
public void mmapFlush(Object arg0Value_, Object arg1Value, long arg2Value, long arg3Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
arg0Value.mmapFlush(arg1Value, arg2Value, arg3Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
return;
}
@TruffleBoundary
@Override
public void mmapUnmap(Object arg0Value_, Object arg1Value, long arg2Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
arg0Value.mmapUnmap(arg1Value, arg2Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
return;
}
@TruffleBoundary
@Override
public long mmapGetPointer(Object arg0Value_, Object arg1Value) {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
return arg0Value.mmapGetPointer(arg1Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
}
@TruffleBoundary
@Override
public PwdResult getpwuid(Object arg0Value_, long arg1Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
return arg0Value.getpwuid(arg1Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
}
@TruffleBoundary
@Override
public PwdResult getpwnam(Object arg0Value_, Object arg1Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
return arg0Value.getpwnam(arg1Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
}
@TruffleBoundary
@Override
public boolean hasGetpwentries(Object arg0Value_) {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
return arg0Value.hasGetpwentries(POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
}
@TruffleBoundary
@Override
public PwdResult[] getpwentries(Object arg0Value_) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
return arg0Value.getpwentries(POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
}
@TruffleBoundary
@Override
public int socket(Object arg0Value_, int arg1Value, int arg2Value, int arg3Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
return arg0Value.socket(arg1Value, arg2Value, arg3Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
}
@TruffleBoundary
@Override
public AcceptResult accept(Object arg0Value_, int arg1Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
return arg0Value.accept(arg1Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
}
@TruffleBoundary
@Override
public void bind(Object arg0Value_, int arg1Value, UniversalSockAddr arg2Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
arg0Value.bind(arg1Value, arg2Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
return;
}
@TruffleBoundary
@Override
public void connect(Object arg0Value_, int arg1Value, UniversalSockAddr arg2Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
arg0Value.connect(arg1Value, arg2Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
return;
}
@TruffleBoundary
@Override
public void listen(Object arg0Value_, int arg1Value, int arg2Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
arg0Value.listen(arg1Value, arg2Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
return;
}
@TruffleBoundary
@Override
public UniversalSockAddr getpeername(Object arg0Value_, int arg1Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
return arg0Value.getpeername(arg1Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
}
@TruffleBoundary
@Override
public UniversalSockAddr getsockname(Object arg0Value_, int arg1Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
return arg0Value.getsockname(arg1Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
}
@TruffleBoundary
@Override
public int send(Object arg0Value_, int arg1Value, byte[] arg2Value, int arg3Value, int arg4Value, int arg5Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
return arg0Value.send(arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
}
@TruffleBoundary
@Override
public int sendto(Object arg0Value_, int arg1Value, byte[] arg2Value, int arg3Value, int arg4Value, int arg5Value, UniversalSockAddr arg6Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
return arg0Value.sendto(arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, arg6Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
}
@TruffleBoundary
@Override
public int recv(Object arg0Value_, int arg1Value, byte[] arg2Value, int arg3Value, int arg4Value, int arg5Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
return arg0Value.recv(arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
}
@TruffleBoundary
@Override
public RecvfromResult recvfrom(Object arg0Value_, int arg1Value, byte[] arg2Value, int arg3Value, int arg4Value, int arg5Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
return arg0Value.recvfrom(arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
}
@TruffleBoundary
@Override
public void shutdown(Object arg0Value_, int arg1Value, int arg2Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
arg0Value.shutdown(arg1Value, arg2Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
return;
}
@TruffleBoundary
@Override
public int getsockopt(Object arg0Value_, int arg1Value, int arg2Value, int arg3Value, byte[] arg4Value, int arg5Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
return arg0Value.getsockopt(arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
}
@TruffleBoundary
@Override
public void setsockopt(Object arg0Value_, int arg1Value, int arg2Value, int arg3Value, byte[] arg4Value, int arg5Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
arg0Value.setsockopt(arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
return;
}
@TruffleBoundary
@Override
public int inet_addr(Object arg0Value_, Object arg1Value) {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
return arg0Value.inet_addr(arg1Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
}
@TruffleBoundary
@Override
public int inet_aton(Object arg0Value_, Object arg1Value) throws InvalidAddressException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
return arg0Value.inet_aton(arg1Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
}
@TruffleBoundary
@Override
public Object inet_ntoa(Object arg0Value_, int arg1Value) {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
return arg0Value.inet_ntoa(arg1Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
}
@TruffleBoundary
@Override
public byte[] inet_pton(Object arg0Value_, int arg1Value, Object arg2Value) throws PosixException, InvalidAddressException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
return arg0Value.inet_pton(arg1Value, arg2Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
}
@TruffleBoundary
@Override
public Object inet_ntop(Object arg0Value_, int arg1Value, byte[] arg2Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
return arg0Value.inet_ntop(arg1Value, arg2Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
}
@TruffleBoundary
@Override
public Object gethostname(Object arg0Value_) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
return arg0Value.gethostname(POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
}
@TruffleBoundary
@Override
public Object[] getnameinfo(Object arg0Value_, UniversalSockAddr arg1Value, int arg2Value) throws GetAddrInfoException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
return arg0Value.getnameinfo(arg1Value, arg2Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
}
@TruffleBoundary
@Override
public AddrInfoCursor getaddrinfo(Object arg0Value_, Object arg1Value, Object arg2Value, int arg3Value, int arg4Value, int arg5Value, int arg6Value) throws GetAddrInfoException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
return arg0Value.getaddrinfo(arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, arg6Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
}
@TruffleBoundary
@Override
public TruffleString crypt(Object arg0Value_, TruffleString arg1Value, TruffleString arg2Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
return arg0Value.crypt(arg1Value, arg2Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
}
@TruffleBoundary
@Override
public UniversalSockAddr createUniversalSockAddr(Object arg0Value_, FamilySpecificSockAddr arg1Value) {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
return arg0Value.createUniversalSockAddr(arg1Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
}
@TruffleBoundary
@Override
public Object createPathFromString(Object arg0Value_, TruffleString arg1Value) {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
return arg0Value.createPathFromString(arg1Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
}
@TruffleBoundary
@Override
public Object createPathFromBytes(Object arg0Value_, byte[] arg1Value) {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
return arg0Value.createPathFromBytes(arg1Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
}
@TruffleBoundary
@Override
public TruffleString getPathAsString(Object arg0Value_, Object arg1Value) {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
return arg0Value.getPathAsString(arg1Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
}
@TruffleBoundary
@Override
public Buffer getPathAsBytes(Object arg0Value_, Object arg1Value) {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
ImageBuildtimePosixSupport arg0Value = ((ImageBuildtimePosixSupport) arg0Value_);
return arg0Value.getPathAsBytes(arg1Value, POSIX_SUPPORT_LIBRARY_.getUncached((arg0Value.nativePosixSupport)));
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy