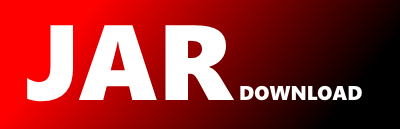
com.oracle.graal.python.runtime.NFIPosixSupportGen Maven / Gradle / Ivy
// CheckStyle: start generated
package com.oracle.graal.python.runtime;
import com.oracle.graal.python.runtime.NFIPosixSupport.CreateUniversalSockAddr;
import com.oracle.graal.python.runtime.NFIPosixSupport.DirEntry;
import com.oracle.graal.python.runtime.NFIPosixSupport.DirEntryGetPath;
import com.oracle.graal.python.runtime.NFIPosixSupport.InvokeNativeFunction;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.CompilerDirectives.TruffleBoundary;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.UnsupportedSpecializationException;
import com.oracle.truffle.api.library.DynamicDispatchLibrary;
import com.oracle.truffle.api.library.LibraryExport;
import com.oracle.truffle.api.library.LibraryFactory;
import com.oracle.truffle.api.nodes.DenyReplace;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.nodes.NodeCost;
import com.oracle.truffle.api.strings.TruffleString;
import com.oracle.truffle.api.strings.TruffleString.CopyToByteArrayNode;
import com.oracle.truffle.api.strings.TruffleString.FromByteArrayNode;
import com.oracle.truffle.api.strings.TruffleString.SwitchEncodingNode;
import java.lang.invoke.VarHandle;
@GeneratedBy(NFIPosixSupport.class)
@SuppressWarnings("javadoc")
final class NFIPosixSupportGen {
private static final LibraryFactory DYNAMIC_DISPATCH_LIBRARY_ = LibraryFactory.resolve(DynamicDispatchLibrary.class);
static {
LibraryExport.register(NFIPosixSupport.class, new PosixSupportLibraryExports());
}
private NFIPosixSupportGen() {
}
@GeneratedBy(NFIPosixSupport.class)
private static final class PosixSupportLibraryExports extends LibraryExport {
private PosixSupportLibraryExports() {
super(PosixSupportLibrary.class, NFIPosixSupport.class, false, false, 0);
}
@Override
protected PosixSupportLibrary createUncached(Object receiver) {
assert receiver instanceof NFIPosixSupport;
PosixSupportLibrary uncached = new Uncached();
return uncached;
}
@Override
protected PosixSupportLibrary createCached(Object receiver) {
assert receiver instanceof NFIPosixSupport;
return new Cached();
}
@GeneratedBy(NFIPosixSupport.class)
private static final class Cached extends PosixSupportLibrary {
/**
* State Info:
* 0: SpecializationActive {@link DirEntryGetPath#withSlash}
* 1: SpecializationActive {@link DirEntryGetPath#withoutSlash}
* 2: SpecializationActive {@link CreateUniversalSockAddr#inet4}
* 3: SpecializationActive {@link CreateUniversalSockAddr#inet6}
* 4: SpecializationActive {@link CreateUniversalSockAddr#unix}
* 5: SpecializationActive {@link NFIPosixSupport#strerror(NFIPosixSupport, int, InvokeNativeFunction, FromByteArrayNode, SwitchEncodingNode)}
* 6: SpecializationActive {@link NFIPosixSupport#getpid(NFIPosixSupport, InvokeNativeFunction)}
* 7: SpecializationActive {@link NFIPosixSupport#umask(NFIPosixSupport, int, InvokeNativeFunction)}
* 8: SpecializationActive {@link NFIPosixSupport#openat(NFIPosixSupport, int, Object, int, int, InvokeNativeFunction)}
* 9: SpecializationActive {@link NFIPosixSupport#close(NFIPosixSupport, int, InvokeNativeFunction)}
* 10: SpecializationActive {@link NFIPosixSupport#read(NFIPosixSupport, int, long, InvokeNativeFunction)}
* 11: SpecializationActive {@link NFIPosixSupport#write(NFIPosixSupport, int, Buffer, InvokeNativeFunction)}
* 12: SpecializationActive {@link NFIPosixSupport#dup(NFIPosixSupport, int, InvokeNativeFunction)}
* 13: SpecializationActive {@link NFIPosixSupport#dup2(NFIPosixSupport, int, int, boolean, InvokeNativeFunction)}
* 14: SpecializationActive {@link NFIPosixSupport#getInheritable(NFIPosixSupport, int, InvokeNativeFunction)}
* 15: SpecializationActive {@link NFIPosixSupport#setInheritable(NFIPosixSupport, int, boolean, InvokeNativeFunction)}
* 16: SpecializationActive {@link NFIPosixSupport#pipe(NFIPosixSupport, InvokeNativeFunction)}
* 17: SpecializationActive {@link NFIPosixSupport#select(NFIPosixSupport, int[], int[], int[], Timeval, InvokeNativeFunction)}
* 18: SpecializationActive {@link NFIPosixSupport#lseek(NFIPosixSupport, int, long, int, InvokeNativeFunction)}
* 19: SpecializationActive {@link NFIPosixSupport#ftruncate(NFIPosixSupport, int, long, InvokeNativeFunction)}
* 20: SpecializationActive {@link NFIPosixSupport#fsync(NFIPosixSupport, int, InvokeNativeFunction)}
* 21: SpecializationActive {@link NFIPosixSupport#flock(NFIPosixSupport, int, int, InvokeNativeFunction)}
* 22: SpecializationActive {@link NFIPosixSupport#getBlocking(NFIPosixSupport, int, InvokeNativeFunction)}
* 23: SpecializationActive {@link NFIPosixSupport#setBlocking(NFIPosixSupport, int, boolean, InvokeNativeFunction)}
* 24: SpecializationActive {@link NFIPosixSupport#getTerminalSize(NFIPosixSupport, int, InvokeNativeFunction)}
* 25: SpecializationActive {@link NFIPosixSupport#fstatat(NFIPosixSupport, int, Object, boolean, InvokeNativeFunction)}
* 26: SpecializationActive {@link NFIPosixSupport#fstat(NFIPosixSupport, int, InvokeNativeFunction)}
* 27: SpecializationActive {@link NFIPosixSupport#statvfs(NFIPosixSupport, Object, InvokeNativeFunction)}
* 28: SpecializationActive {@link NFIPosixSupport#fstatvfs(NFIPosixSupport, int, InvokeNativeFunction)}
* 29: SpecializationActive {@link NFIPosixSupport#uname(NFIPosixSupport, InvokeNativeFunction, FromByteArrayNode, SwitchEncodingNode)}
* 30: SpecializationActive {@link NFIPosixSupport#unlinkat(NFIPosixSupport, int, Object, boolean, InvokeNativeFunction)}
* 31: SpecializationActive {@link NFIPosixSupport#linkat(NFIPosixSupport, int, Object, int, Object, int, InvokeNativeFunction)}
*
*/
@CompilationFinal private int state_0_;
/**
* State Info:
* 0: SpecializationActive {@link NFIPosixSupport#symlinkat(NFIPosixSupport, Object, int, Object, InvokeNativeFunction)}
* 1: SpecializationActive {@link NFIPosixSupport#mkdirat(NFIPosixSupport, int, Object, int, InvokeNativeFunction)}
* 2: SpecializationActive {@link NFIPosixSupport#getcwd(NFIPosixSupport, InvokeNativeFunction)}
* 3: SpecializationActive {@link NFIPosixSupport#chdir(NFIPosixSupport, Object, InvokeNativeFunction)}
* 4: SpecializationActive {@link NFIPosixSupport#fchdir(NFIPosixSupport, int, InvokeNativeFunction)}
* 5: SpecializationActive {@link NFIPosixSupport#isatty(NFIPosixSupport, int, InvokeNativeFunction)}
* 6: SpecializationActive {@link NFIPosixSupport#opendir(NFIPosixSupport, Object, InvokeNativeFunction)}
* 7: SpecializationActive {@link NFIPosixSupport#fdopendir(NFIPosixSupport, int, InvokeNativeFunction)}
* 8: SpecializationActive {@link NFIPosixSupport#closedir(NFIPosixSupport, Object, InvokeNativeFunction)}
* 9: SpecializationActive {@link NFIPosixSupport#readdir(NFIPosixSupport, Object, InvokeNativeFunction)}
* 10: SpecializationActive {@link NFIPosixSupport#rewinddir(NFIPosixSupport, Object, InvokeNativeFunction)}
* 11: SpecializationActive {@link NFIPosixSupport#utimensat(NFIPosixSupport, int, Object, long[], boolean, InvokeNativeFunction)}
* 12: SpecializationActive {@link NFIPosixSupport#futimens(NFIPosixSupport, int, long[], InvokeNativeFunction)}
* 13: SpecializationActive {@link NFIPosixSupport#futimes(NFIPosixSupport, int, Timeval[], InvokeNativeFunction)}
* 14: SpecializationActive {@link NFIPosixSupport#lutimes(NFIPosixSupport, Object, Timeval[], InvokeNativeFunction)}
* 15: SpecializationActive {@link NFIPosixSupport#utimes(NFIPosixSupport, Object, Timeval[], InvokeNativeFunction)}
* 16: SpecializationActive {@link NFIPosixSupport#renameat(NFIPosixSupport, int, Object, int, Object, InvokeNativeFunction)}
* 17: SpecializationActive {@link NFIPosixSupport#faccessat(NFIPosixSupport, int, Object, int, boolean, boolean, InvokeNativeFunction)}
* 18: SpecializationActive {@link NFIPosixSupport#fchmodat(NFIPosixSupport, int, Object, int, boolean, InvokeNativeFunction)}
* 19: SpecializationActive {@link NFIPosixSupport#fchmod(NFIPosixSupport, int, int, InvokeNativeFunction)}
* 20: SpecializationActive {@link NFIPosixSupport#readlinkat(NFIPosixSupport, int, Object, InvokeNativeFunction)}
* 21: SpecializationActive {@link NFIPosixSupport#kill(NFIPosixSupport, long, int, InvokeNativeFunction)}
* 22: SpecializationActive {@link NFIPosixSupport#killpg(NFIPosixSupport, long, int, InvokeNativeFunction)}
* 23: SpecializationActive {@link NFIPosixSupport#waitpid(NFIPosixSupport, long, int, InvokeNativeFunction)}
* 24: SpecializationActive {@link NFIPosixSupport#wcoredump(NFIPosixSupport, int, InvokeNativeFunction)}
* 25: SpecializationActive {@link NFIPosixSupport#abort(NFIPosixSupport, InvokeNativeFunction)}
* 26: SpecializationActive {@link NFIPosixSupport#wifcontinued(NFIPosixSupport, int, InvokeNativeFunction)}
* 27: SpecializationActive {@link NFIPosixSupport#wifstopped(NFIPosixSupport, int, InvokeNativeFunction)}
* 28: SpecializationActive {@link NFIPosixSupport#wifsignaled(NFIPosixSupport, int, InvokeNativeFunction)}
* 29: SpecializationActive {@link NFIPosixSupport#wifexited(NFIPosixSupport, int, InvokeNativeFunction)}
* 30: SpecializationActive {@link NFIPosixSupport#wexitstatus(NFIPosixSupport, int, InvokeNativeFunction)}
* 31: SpecializationActive {@link NFIPosixSupport#wtermsig(NFIPosixSupport, int, InvokeNativeFunction)}
*
*/
@CompilationFinal private int state_1_;
/**
* State Info:
* 0: SpecializationActive {@link NFIPosixSupport#wstopsig(NFIPosixSupport, int, InvokeNativeFunction)}
* 1: SpecializationActive {@link NFIPosixSupport#getuid(NFIPosixSupport, InvokeNativeFunction)}
* 2: SpecializationActive {@link NFIPosixSupport#geteuid(NFIPosixSupport, InvokeNativeFunction)}
* 3: SpecializationActive {@link NFIPosixSupport#getgid(NFIPosixSupport, InvokeNativeFunction)}
* 4: SpecializationActive {@link NFIPosixSupport#getppid(NFIPosixSupport, InvokeNativeFunction)}
* 5: SpecializationActive {@link NFIPosixSupport#setpgid(NFIPosixSupport, long, long, InvokeNativeFunction)}
* 6: SpecializationActive {@link NFIPosixSupport#getpgid(NFIPosixSupport, long, InvokeNativeFunction)}
* 7: SpecializationActive {@link NFIPosixSupport#getpgrp(NFIPosixSupport, InvokeNativeFunction)}
* 8: SpecializationActive {@link NFIPosixSupport#getsid(NFIPosixSupport, long, InvokeNativeFunction)}
* 9: SpecializationActive {@link NFIPosixSupport#setsid(NFIPosixSupport, InvokeNativeFunction)}
* 10: SpecializationActive {@link NFIPosixSupport#openpty(NFIPosixSupport, InvokeNativeFunction)}
* 11: SpecializationActive {@link NFIPosixSupport#ctermid(NFIPosixSupport, InvokeNativeFunction, FromByteArrayNode, SwitchEncodingNode)}
* 12: SpecializationActive {@link NFIPosixSupport#setenv(NFIPosixSupport, Object, Object, boolean, InvokeNativeFunction)}
* 13: SpecializationActive {@link NFIPosixSupport#unsetenv(NFIPosixSupport, Object, InvokeNativeFunction)}
* 14: SpecializationActive {@link NFIPosixSupport#forkExec(NFIPosixSupport, Object[], Object[], Object, Object[], int, int, int, int, int, int, int, int, boolean, boolean, boolean, int[], InvokeNativeFunction)}
* 15: SpecializationActive {@link NFIPosixSupport#execv(NFIPosixSupport, Object, Object[], InvokeNativeFunction)}
* 16: SpecializationActive {@link NFIPosixSupport#system(NFIPosixSupport, Object, InvokeNativeFunction)}
* 17: SpecializationActive {@link NFIPosixSupport#mmap(NFIPosixSupport, long, int, int, int, long, InvokeNativeFunction)}
* 18: SpecializationActive {@link NFIPosixSupport#mmapFlush(NFIPosixSupport, Object, long, long, InvokeNativeFunction)}
* 19: SpecializationActive {@link NFIPosixSupport#mmapUnmap(NFIPosixSupport, Object, long, InvokeNativeFunction)}
* 20: SpecializationActive {@link NFIPosixSupport#socket(NFIPosixSupport, int, int, int, InvokeNativeFunction)}
* 21: SpecializationActive {@link NFIPosixSupport#accept(NFIPosixSupport, int, InvokeNativeFunction)}
* 22: SpecializationActive {@link NFIPosixSupport#bind(NFIPosixSupport, int, UniversalSockAddr, InvokeNativeFunction)}
* 23: SpecializationActive {@link NFIPosixSupport#connect(NFIPosixSupport, int, UniversalSockAddr, InvokeNativeFunction)}
* 24: SpecializationActive {@link NFIPosixSupport#listen(NFIPosixSupport, int, int, InvokeNativeFunction)}
* 25: SpecializationActive {@link NFIPosixSupport#getpeername(NFIPosixSupport, int, InvokeNativeFunction)}
* 26: SpecializationActive {@link NFIPosixSupport#getsockname(NFIPosixSupport, int, InvokeNativeFunction)}
* 27: SpecializationActive {@link NFIPosixSupport#send(NFIPosixSupport, int, byte[], int, int, int, InvokeNativeFunction)}
* 28: SpecializationActive {@link NFIPosixSupport#sendto(NFIPosixSupport, int, byte[], int, int, int, UniversalSockAddr, InvokeNativeFunction)}
* 29: SpecializationActive {@link NFIPosixSupport#recv(NFIPosixSupport, int, byte[], int, int, int, InvokeNativeFunction)}
* 30: SpecializationActive {@link NFIPosixSupport#recvfrom(NFIPosixSupport, int, byte[], int, int, int, InvokeNativeFunction)}
* 31: SpecializationActive {@link NFIPosixSupport#shutdown(NFIPosixSupport, int, int, InvokeNativeFunction)}
*
*/
@CompilationFinal private int state_2_;
/**
* State Info:
* 0: SpecializationActive {@link NFIPosixSupport#getsockopt(NFIPosixSupport, int, int, int, byte[], int, InvokeNativeFunction)}
* 1: SpecializationActive {@link NFIPosixSupport#setsockopt(NFIPosixSupport, int, int, int, byte[], int, InvokeNativeFunction)}
* 2: SpecializationActive {@link NFIPosixSupport#inet_addr(NFIPosixSupport, Object, InvokeNativeFunction)}
* 3: SpecializationActive {@link NFIPosixSupport#inet_aton(NFIPosixSupport, Object, InvokeNativeFunction)}
* 4: SpecializationActive {@link NFIPosixSupport#inet_ntoa(NFIPosixSupport, int, InvokeNativeFunction)}
* 5: SpecializationActive {@link NFIPosixSupport#inet_pton(NFIPosixSupport, int, Object, InvokeNativeFunction)}
* 6: SpecializationActive {@link NFIPosixSupport#inet_ntop(NFIPosixSupport, int, byte[], InvokeNativeFunction)}
* 7: SpecializationActive {@link NFIPosixSupport#gethostname(NFIPosixSupport, InvokeNativeFunction)}
* 8: SpecializationActive {@link NFIPosixSupport#getnameinfo(NFIPosixSupport, UniversalSockAddr, int, InvokeNativeFunction, FromByteArrayNode, SwitchEncodingNode)}
* 9: SpecializationActive {@link NFIPosixSupport#getaddrinfo(NFIPosixSupport, Object, Object, int, int, int, int, InvokeNativeFunction, FromByteArrayNode, SwitchEncodingNode)}
* 10: SpecializationActive {@link NFIPosixSupport#crypt(NFIPosixSupport, TruffleString, TruffleString, InvokeNativeFunction, SwitchEncodingNode, CopyToByteArrayNode, FromByteArrayNode, SwitchEncodingNode)}
* 11: SpecializationActive {@link NFIPosixSupport#getpwuid(NFIPosixSupport, long, InvokeNativeFunction, FromByteArrayNode, SwitchEncodingNode)}
* 12: SpecializationActive {@link NFIPosixSupport#getpwnam(NFIPosixSupport, Object, InvokeNativeFunction, FromByteArrayNode, SwitchEncodingNode)}
* 13: SpecializationActive {@link NFIPosixSupport#getpwentries(NFIPosixSupport, InvokeNativeFunction, FromByteArrayNode, SwitchEncodingNode)}
* 14: SpecializationActive {@link NFIPosixSupport#createPathFromString(NFIPosixSupport, TruffleString, SwitchEncodingNode, CopyToByteArrayNode)}
* 15: SpecializationActive {@link NFIPosixSupport#getPathAsString(NFIPosixSupport, Object, FromByteArrayNode, SwitchEncodingNode)}
*
*/
@CompilationFinal private int state_3_;
/**
* Source Info:
* Specialization: {@link CreateUniversalSockAddr#inet4}
* Parameter: {@link InvokeNativeFunction} invokeNode
*/
@Child private InvokeNativeFunction invoke;
/**
* Source Info:
* Specialization: {@link NFIPosixSupport#strerror}
* Parameter: {@link FromByteArrayNode} fromByteArrayNode
*/
@Child private FromByteArrayNode tsFromBytes;
/**
* Source Info:
* Specialization: {@link NFIPosixSupport#strerror}
* Parameter: {@link SwitchEncodingNode} switchEncodingFromUtf8Node
*/
@Child private SwitchEncodingNode fromUtf8;
/**
* Source Info:
* Specialization: {@link NFIPosixSupport#crypt}
* Parameter: {@link SwitchEncodingNode} switchEncodingToUtf8Node
*/
@Child private SwitchEncodingNode toUtf8;
/**
* Source Info:
* Specialization: {@link NFIPosixSupport#crypt}
* Parameter: {@link CopyToByteArrayNode} copyToByteArrayNode
*/
@Child private CopyToByteArrayNode tsCopyBytes;
protected Cached() {
}
@Override
public boolean accepts(Object receiver) {
assert !(receiver instanceof NFIPosixSupport) || DYNAMIC_DISPATCH_LIBRARY_.getUncached().dispatch(receiver) == null : "Invalid library export. Exported receiver with dynamic dispatch found but not expected.";
return receiver instanceof NFIPosixSupport;
}
/**
* Debug Info:
* Specialization {@link DirEntryGetPath#withSlash}
* Activation probability: 0.00596
* With/without class size: 4/0 bytes
* Specialization {@link DirEntryGetPath#withoutSlash}
* Activation probability: 0.00321
* With/without class size: 4/0 bytes
*
*/
@Override
public Object dirEntryGetPath(Object arg0Value_, Object arg1Value, Object arg2Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_0 = this.state_0_;
if ((state_0 & 0b11) != 0 /* is SpecializationActive[NFIPosixSupport.DirEntryGetPath.withSlash(NFIPosixSupport, DirEntry, Object)] || SpecializationActive[NFIPosixSupport.DirEntryGetPath.withoutSlash(NFIPosixSupport, DirEntry, Object)] */ && arg1Value instanceof DirEntry) {
DirEntry arg1Value_ = (DirEntry) arg1Value;
if ((state_0 & 0b1) != 0 /* is SpecializationActive[NFIPosixSupport.DirEntryGetPath.withSlash(NFIPosixSupport, DirEntry, Object)] */) {
if ((DirEntryGetPath.endsWithSlash(arg2Value))) {
return DirEntryGetPath.withSlash(arg0Value, arg1Value_, arg2Value);
}
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[NFIPosixSupport.DirEntryGetPath.withoutSlash(NFIPosixSupport, DirEntry, Object)] */) {
if ((!(DirEntryGetPath.endsWithSlash(arg2Value)))) {
return DirEntryGetPath.withoutSlash(arg0Value, arg1Value_, arg2Value);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return dirEntryGetPathAndSpecialize(arg0Value, arg1Value, arg2Value);
}
private Buffer dirEntryGetPathAndSpecialize(NFIPosixSupport arg0Value, Object arg1Value, Object arg2Value) {
int state_0 = this.state_0_;
if (arg1Value instanceof DirEntry) {
DirEntry arg1Value_ = (DirEntry) arg1Value;
if ((DirEntryGetPath.endsWithSlash(arg2Value))) {
state_0 = state_0 | 0b1 /* add SpecializationActive[NFIPosixSupport.DirEntryGetPath.withSlash(NFIPosixSupport, DirEntry, Object)] */;
this.state_0_ = state_0;
return DirEntryGetPath.withSlash(arg0Value, arg1Value_, arg2Value);
}
if ((!(DirEntryGetPath.endsWithSlash(arg2Value)))) {
state_0 = state_0 | 0b10 /* add SpecializationActive[NFIPosixSupport.DirEntryGetPath.withoutSlash(NFIPosixSupport, DirEntry, Object)] */;
this.state_0_ = state_0;
return DirEntryGetPath.withoutSlash(arg0Value, arg1Value_, arg2Value);
}
}
throw new UnsupportedSpecializationException(this, new Node[] {null, null, null}, arg0Value, arg1Value, arg2Value);
}
@Override
public NodeCost getCost() {
int state_0 = this.state_0_;
if ((state_0 & 0b11) == 0) {
return NodeCost.UNINITIALIZED;
} else {
if (((state_0 & 0b11) & ((state_0 & 0b11) - 1)) == 0 /* is-single */) {
return NodeCost.MONOMORPHIC;
}
}
return NodeCost.POLYMORPHIC;
}
/**
* Debug Info:
* Specialization {@link CreateUniversalSockAddr#inet4}
* Activation probability: 0.00443
* With/without class size: 4/0 bytes
* Specialization {@link CreateUniversalSockAddr#inet6}
* Activation probability: 0.00306
* With/without class size: 4/0 bytes
* Specialization {@link CreateUniversalSockAddr#unix}
* Activation probability: 0.00168
* With/without class size: 4/0 bytes
*
*/
@Override
public UniversalSockAddr createUniversalSockAddr(Object arg0Value_, FamilySpecificSockAddr arg1Value) {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_0 = this.state_0_;
if ((state_0 & 0b11100) != 0 /* is SpecializationActive[NFIPosixSupport.CreateUniversalSockAddr.inet4(NFIPosixSupport, Inet4SockAddr, InvokeNativeFunction)] || SpecializationActive[NFIPosixSupport.CreateUniversalSockAddr.inet6(NFIPosixSupport, Inet6SockAddr, InvokeNativeFunction)] || SpecializationActive[NFIPosixSupport.CreateUniversalSockAddr.unix(NFIPosixSupport, UnixSockAddr, InvokeNativeFunction)] */) {
if ((state_0 & 0b100) != 0 /* is SpecializationActive[NFIPosixSupport.CreateUniversalSockAddr.inet4(NFIPosixSupport, Inet4SockAddr, InvokeNativeFunction)] */ && arg1Value instanceof Inet4SockAddr) {
Inet4SockAddr arg1Value_ = (Inet4SockAddr) arg1Value;
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
return CreateUniversalSockAddr.inet4(arg0Value, arg1Value_, invoke_);
}
}
}
if ((state_0 & 0b1000) != 0 /* is SpecializationActive[NFIPosixSupport.CreateUniversalSockAddr.inet6(NFIPosixSupport, Inet6SockAddr, InvokeNativeFunction)] */ && arg1Value instanceof Inet6SockAddr) {
Inet6SockAddr arg1Value_ = (Inet6SockAddr) arg1Value;
{
InvokeNativeFunction invoke_1 = this.invoke;
if (invoke_1 != null) {
return CreateUniversalSockAddr.inet6(arg0Value, arg1Value_, invoke_1);
}
}
}
if ((state_0 & 0b10000) != 0 /* is SpecializationActive[NFIPosixSupport.CreateUniversalSockAddr.unix(NFIPosixSupport, UnixSockAddr, InvokeNativeFunction)] */ && arg1Value instanceof UnixSockAddr) {
UnixSockAddr arg1Value_ = (UnixSockAddr) arg1Value;
{
InvokeNativeFunction invoke_2 = this.invoke;
if (invoke_2 != null) {
return CreateUniversalSockAddr.unix(arg0Value, arg1Value_, invoke_2);
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return createUniversalSockAddrAndSpecialize(arg0Value, arg1Value);
}
private UniversalSockAddr createUniversalSockAddrAndSpecialize(NFIPosixSupport arg0Value, FamilySpecificSockAddr arg1Value) {
int state_0 = this.state_0_;
if (arg1Value instanceof Inet4SockAddr) {
Inet4SockAddr arg1Value_ = (Inet4SockAddr) arg1Value;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'inet4(NFIPosixSupport, Inet4SockAddr, InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_0 = state_0 | 0b100 /* add SpecializationActive[NFIPosixSupport.CreateUniversalSockAddr.inet4(NFIPosixSupport, Inet4SockAddr, InvokeNativeFunction)] */;
this.state_0_ = state_0;
return CreateUniversalSockAddr.inet4(arg0Value, arg1Value_, invoke_);
}
if (arg1Value instanceof Inet6SockAddr) {
Inet6SockAddr arg1Value_ = (Inet6SockAddr) arg1Value;
InvokeNativeFunction invoke_1;
InvokeNativeFunction invoke_1_shared = this.invoke;
if (invoke_1_shared != null) {
invoke_1 = invoke_1_shared;
} else {
invoke_1 = this.insert((InvokeNativeFunction.create()));
if (invoke_1 == null) {
throw new IllegalStateException("Specialization 'inet6(NFIPosixSupport, Inet6SockAddr, InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_1;
}
state_0 = state_0 | 0b1000 /* add SpecializationActive[NFIPosixSupport.CreateUniversalSockAddr.inet6(NFIPosixSupport, Inet6SockAddr, InvokeNativeFunction)] */;
this.state_0_ = state_0;
return CreateUniversalSockAddr.inet6(arg0Value, arg1Value_, invoke_1);
}
if (arg1Value instanceof UnixSockAddr) {
UnixSockAddr arg1Value_ = (UnixSockAddr) arg1Value;
InvokeNativeFunction invoke_2;
InvokeNativeFunction invoke_2_shared = this.invoke;
if (invoke_2_shared != null) {
invoke_2 = invoke_2_shared;
} else {
invoke_2 = this.insert((InvokeNativeFunction.create()));
if (invoke_2 == null) {
throw new IllegalStateException("Specialization 'unix(NFIPosixSupport, UnixSockAddr, InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_2;
}
state_0 = state_0 | 0b10000 /* add SpecializationActive[NFIPosixSupport.CreateUniversalSockAddr.unix(NFIPosixSupport, UnixSockAddr, InvokeNativeFunction)] */;
this.state_0_ = state_0;
return CreateUniversalSockAddr.unix(arg0Value, arg1Value_, invoke_2);
}
throw new UnsupportedSpecializationException(this, new Node[] {null, null}, arg0Value, arg1Value);
}
@Override
public TruffleString getBackend(Object receiver) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
return (((NFIPosixSupport) receiver)).getBackend();
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#strerror(NFIPosixSupport, int, InvokeNativeFunction, FromByteArrayNode, SwitchEncodingNode)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public TruffleString strerror(Object arg0Value_, int arg1Value) {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_0 = this.state_0_;
if ((state_0 & 0b100000) != 0 /* is SpecializationActive[NFIPosixSupport.strerror(NFIPosixSupport, int, InvokeNativeFunction, FromByteArrayNode, SwitchEncodingNode)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
FromByteArrayNode tsFromBytes_ = this.tsFromBytes;
if (tsFromBytes_ != null) {
SwitchEncodingNode fromUtf8_ = this.fromUtf8;
if (fromUtf8_ != null) {
return arg0Value.strerror(arg1Value, invoke_, tsFromBytes_, fromUtf8_);
}
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return strerrorNode_AndSpecialize(arg0Value, arg1Value);
}
private TruffleString strerrorNode_AndSpecialize(NFIPosixSupport arg0Value, int arg1Value) {
int state_0 = this.state_0_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'strerror(NFIPosixSupport, int, InvokeNativeFunction, FromByteArrayNode, SwitchEncodingNode)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
FromByteArrayNode tsFromBytes_;
FromByteArrayNode tsFromBytes__shared = this.tsFromBytes;
if (tsFromBytes__shared != null) {
tsFromBytes_ = tsFromBytes__shared;
} else {
tsFromBytes_ = this.insert((FromByteArrayNode.create()));
if (tsFromBytes_ == null) {
throw new IllegalStateException("Specialization 'strerror(NFIPosixSupport, int, InvokeNativeFunction, FromByteArrayNode, SwitchEncodingNode)' contains a shared cache with name 'fromByteArrayNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.tsFromBytes == null) {
VarHandle.storeStoreFence();
this.tsFromBytes = tsFromBytes_;
}
SwitchEncodingNode fromUtf8_;
SwitchEncodingNode fromUtf8__shared = this.fromUtf8;
if (fromUtf8__shared != null) {
fromUtf8_ = fromUtf8__shared;
} else {
fromUtf8_ = this.insert((SwitchEncodingNode.create()));
if (fromUtf8_ == null) {
throw new IllegalStateException("Specialization 'strerror(NFIPosixSupport, int, InvokeNativeFunction, FromByteArrayNode, SwitchEncodingNode)' contains a shared cache with name 'switchEncodingFromUtf8Node' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.fromUtf8 == null) {
VarHandle.storeStoreFence();
this.fromUtf8 = fromUtf8_;
}
state_0 = state_0 | 0b100000 /* add SpecializationActive[NFIPosixSupport.strerror(NFIPosixSupport, int, InvokeNativeFunction, FromByteArrayNode, SwitchEncodingNode)] */;
this.state_0_ = state_0;
return arg0Value.strerror(arg1Value, invoke_, tsFromBytes_, fromUtf8_);
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#getpid(NFIPosixSupport, InvokeNativeFunction)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public long getpid(Object arg0Value_) {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_0 = this.state_0_;
if ((state_0 & 0b1000000) != 0 /* is SpecializationActive[NFIPosixSupport.getpid(NFIPosixSupport, InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
return arg0Value.getpid(invoke_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return getpidNode_AndSpecialize(arg0Value);
}
private long getpidNode_AndSpecialize(NFIPosixSupport arg0Value) {
int state_0 = this.state_0_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'getpid(NFIPosixSupport, InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_0 = state_0 | 0b1000000 /* add SpecializationActive[NFIPosixSupport.getpid(NFIPosixSupport, InvokeNativeFunction)] */;
this.state_0_ = state_0;
return arg0Value.getpid(invoke_);
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#umask(NFIPosixSupport, int, InvokeNativeFunction)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public int umask(Object arg0Value_, int arg1Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_0 = this.state_0_;
if ((state_0 & 0b10000000) != 0 /* is SpecializationActive[NFIPosixSupport.umask(NFIPosixSupport, int, InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
return arg0Value.umask(arg1Value, invoke_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return umaskNode_AndSpecialize(arg0Value, arg1Value);
}
private int umaskNode_AndSpecialize(NFIPosixSupport arg0Value, int arg1Value) throws PosixException {
int state_0 = this.state_0_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'umask(NFIPosixSupport, int, InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_0 = state_0 | 0b10000000 /* add SpecializationActive[NFIPosixSupport.umask(NFIPosixSupport, int, InvokeNativeFunction)] */;
this.state_0_ = state_0;
return arg0Value.umask(arg1Value, invoke_);
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#openat(NFIPosixSupport, int, Object, int, int, InvokeNativeFunction)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public int openat(Object arg0Value_, int arg1Value, Object arg2Value, int arg3Value, int arg4Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_0 = this.state_0_;
if ((state_0 & 0b100000000) != 0 /* is SpecializationActive[NFIPosixSupport.openat(NFIPosixSupport, int, Object, int, int, InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
return arg0Value.openat(arg1Value, arg2Value, arg3Value, arg4Value, invoke_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return openatNode_AndSpecialize(arg0Value, arg1Value, arg2Value, arg3Value, arg4Value);
}
private int openatNode_AndSpecialize(NFIPosixSupport arg0Value, int arg1Value, Object arg2Value, int arg3Value, int arg4Value) throws PosixException {
int state_0 = this.state_0_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'openat(NFIPosixSupport, int, Object, int, int, InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_0 = state_0 | 0b100000000 /* add SpecializationActive[NFIPosixSupport.openat(NFIPosixSupport, int, Object, int, int, InvokeNativeFunction)] */;
this.state_0_ = state_0;
return arg0Value.openat(arg1Value, arg2Value, arg3Value, arg4Value, invoke_);
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#close(NFIPosixSupport, int, InvokeNativeFunction)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public int close(Object arg0Value_, int arg1Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_0 = this.state_0_;
if ((state_0 & 0b1000000000) != 0 /* is SpecializationActive[NFIPosixSupport.close(NFIPosixSupport, int, InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
return arg0Value.close(arg1Value, invoke_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return closeNode_AndSpecialize(arg0Value, arg1Value);
}
private int closeNode_AndSpecialize(NFIPosixSupport arg0Value, int arg1Value) throws PosixException {
int state_0 = this.state_0_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'close(NFIPosixSupport, int, InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_0 = state_0 | 0b1000000000 /* add SpecializationActive[NFIPosixSupport.close(NFIPosixSupport, int, InvokeNativeFunction)] */;
this.state_0_ = state_0;
return arg0Value.close(arg1Value, invoke_);
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#read(NFIPosixSupport, int, long, InvokeNativeFunction)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public Buffer read(Object arg0Value_, int arg1Value, long arg2Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_0 = this.state_0_;
if ((state_0 & 0b10000000000) != 0 /* is SpecializationActive[NFIPosixSupport.read(NFIPosixSupport, int, long, InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
return arg0Value.read(arg1Value, arg2Value, invoke_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return readNode_AndSpecialize(arg0Value, arg1Value, arg2Value);
}
private Buffer readNode_AndSpecialize(NFIPosixSupport arg0Value, int arg1Value, long arg2Value) throws PosixException {
int state_0 = this.state_0_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'read(NFIPosixSupport, int, long, InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_0 = state_0 | 0b10000000000 /* add SpecializationActive[NFIPosixSupport.read(NFIPosixSupport, int, long, InvokeNativeFunction)] */;
this.state_0_ = state_0;
return arg0Value.read(arg1Value, arg2Value, invoke_);
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#write(NFIPosixSupport, int, Buffer, InvokeNativeFunction)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public long write(Object arg0Value_, int arg1Value, Buffer arg2Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_0 = this.state_0_;
if ((state_0 & 0b100000000000) != 0 /* is SpecializationActive[NFIPosixSupport.write(NFIPosixSupport, int, Buffer, InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
return arg0Value.write(arg1Value, arg2Value, invoke_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return writeNode_AndSpecialize(arg0Value, arg1Value, arg2Value);
}
private long writeNode_AndSpecialize(NFIPosixSupport arg0Value, int arg1Value, Buffer arg2Value) throws PosixException {
int state_0 = this.state_0_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'write(NFIPosixSupport, int, Buffer, InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_0 = state_0 | 0b100000000000 /* add SpecializationActive[NFIPosixSupport.write(NFIPosixSupport, int, Buffer, InvokeNativeFunction)] */;
this.state_0_ = state_0;
return arg0Value.write(arg1Value, arg2Value, invoke_);
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#dup(NFIPosixSupport, int, InvokeNativeFunction)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public int dup(Object arg0Value_, int arg1Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_0 = this.state_0_;
if ((state_0 & 0b1000000000000) != 0 /* is SpecializationActive[NFIPosixSupport.dup(NFIPosixSupport, int, InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
return arg0Value.dup(arg1Value, invoke_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return dupNode_AndSpecialize(arg0Value, arg1Value);
}
private int dupNode_AndSpecialize(NFIPosixSupport arg0Value, int arg1Value) throws PosixException {
int state_0 = this.state_0_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'dup(NFIPosixSupport, int, InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_0 = state_0 | 0b1000000000000 /* add SpecializationActive[NFIPosixSupport.dup(NFIPosixSupport, int, InvokeNativeFunction)] */;
this.state_0_ = state_0;
return arg0Value.dup(arg1Value, invoke_);
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#dup2(NFIPosixSupport, int, int, boolean, InvokeNativeFunction)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public int dup2(Object arg0Value_, int arg1Value, int arg2Value, boolean arg3Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_0 = this.state_0_;
if ((state_0 & 0b10000000000000) != 0 /* is SpecializationActive[NFIPosixSupport.dup2(NFIPosixSupport, int, int, boolean, InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
return arg0Value.dup2(arg1Value, arg2Value, arg3Value, invoke_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return dup2Node_AndSpecialize(arg0Value, arg1Value, arg2Value, arg3Value);
}
private int dup2Node_AndSpecialize(NFIPosixSupport arg0Value, int arg1Value, int arg2Value, boolean arg3Value) throws PosixException {
int state_0 = this.state_0_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'dup2(NFIPosixSupport, int, int, boolean, InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_0 = state_0 | 0b10000000000000 /* add SpecializationActive[NFIPosixSupport.dup2(NFIPosixSupport, int, int, boolean, InvokeNativeFunction)] */;
this.state_0_ = state_0;
return arg0Value.dup2(arg1Value, arg2Value, arg3Value, invoke_);
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#getInheritable(NFIPosixSupport, int, InvokeNativeFunction)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public boolean getInheritable(Object arg0Value_, int arg1Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_0 = this.state_0_;
if ((state_0 & 0b100000000000000) != 0 /* is SpecializationActive[NFIPosixSupport.getInheritable(NFIPosixSupport, int, InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
return arg0Value.getInheritable(arg1Value, invoke_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return getInheritableNode_AndSpecialize(arg0Value, arg1Value);
}
private boolean getInheritableNode_AndSpecialize(NFIPosixSupport arg0Value, int arg1Value) throws PosixException {
int state_0 = this.state_0_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'getInheritable(NFIPosixSupport, int, InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_0 = state_0 | 0b100000000000000 /* add SpecializationActive[NFIPosixSupport.getInheritable(NFIPosixSupport, int, InvokeNativeFunction)] */;
this.state_0_ = state_0;
return arg0Value.getInheritable(arg1Value, invoke_);
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#setInheritable(NFIPosixSupport, int, boolean, InvokeNativeFunction)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public void setInheritable(Object arg0Value_, int arg1Value, boolean arg2Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_0 = this.state_0_;
if ((state_0 & 0b1000000000000000) != 0 /* is SpecializationActive[NFIPosixSupport.setInheritable(NFIPosixSupport, int, boolean, InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
arg0Value.setInheritable(arg1Value, arg2Value, invoke_);
return;
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
setInheritableNode_AndSpecialize(arg0Value, arg1Value, arg2Value);
return;
}
private void setInheritableNode_AndSpecialize(NFIPosixSupport arg0Value, int arg1Value, boolean arg2Value) throws PosixException {
int state_0 = this.state_0_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'setInheritable(NFIPosixSupport, int, boolean, InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_0 = state_0 | 0b1000000000000000 /* add SpecializationActive[NFIPosixSupport.setInheritable(NFIPosixSupport, int, boolean, InvokeNativeFunction)] */;
this.state_0_ = state_0;
arg0Value.setInheritable(arg1Value, arg2Value, invoke_);
return;
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#pipe(NFIPosixSupport, InvokeNativeFunction)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public int[] pipe(Object arg0Value_) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_0 = this.state_0_;
if ((state_0 & 0x10000) != 0 /* is SpecializationActive[NFIPosixSupport.pipe(NFIPosixSupport, InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
return arg0Value.pipe(invoke_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return pipeNode_AndSpecialize(arg0Value);
}
private int[] pipeNode_AndSpecialize(NFIPosixSupport arg0Value) throws PosixException {
int state_0 = this.state_0_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'pipe(NFIPosixSupport, InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_0 = state_0 | 0x10000 /* add SpecializationActive[NFIPosixSupport.pipe(NFIPosixSupport, InvokeNativeFunction)] */;
this.state_0_ = state_0;
return arg0Value.pipe(invoke_);
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#select(NFIPosixSupport, int[], int[], int[], Timeval, InvokeNativeFunction)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public SelectResult select(Object arg0Value_, int[] arg1Value, int[] arg2Value, int[] arg3Value, Timeval arg4Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_0 = this.state_0_;
if ((state_0 & 0x20000) != 0 /* is SpecializationActive[NFIPosixSupport.select(NFIPosixSupport, int[], int[], int[], Timeval, InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
return arg0Value.select(arg1Value, arg2Value, arg3Value, arg4Value, invoke_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return selectNode_AndSpecialize(arg0Value, arg1Value, arg2Value, arg3Value, arg4Value);
}
private SelectResult selectNode_AndSpecialize(NFIPosixSupport arg0Value, int[] arg1Value, int[] arg2Value, int[] arg3Value, Timeval arg4Value) throws PosixException {
int state_0 = this.state_0_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'select(NFIPosixSupport, int[], int[], int[], Timeval, InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_0 = state_0 | 0x20000 /* add SpecializationActive[NFIPosixSupport.select(NFIPosixSupport, int[], int[], int[], Timeval, InvokeNativeFunction)] */;
this.state_0_ = state_0;
return arg0Value.select(arg1Value, arg2Value, arg3Value, arg4Value, invoke_);
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#lseek(NFIPosixSupport, int, long, int, InvokeNativeFunction)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public long lseek(Object arg0Value_, int arg1Value, long arg2Value, int arg3Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_0 = this.state_0_;
if ((state_0 & 0x40000) != 0 /* is SpecializationActive[NFIPosixSupport.lseek(NFIPosixSupport, int, long, int, InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
return arg0Value.lseek(arg1Value, arg2Value, arg3Value, invoke_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return lseekNode_AndSpecialize(arg0Value, arg1Value, arg2Value, arg3Value);
}
private long lseekNode_AndSpecialize(NFIPosixSupport arg0Value, int arg1Value, long arg2Value, int arg3Value) throws PosixException {
int state_0 = this.state_0_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'lseek(NFIPosixSupport, int, long, int, InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_0 = state_0 | 0x40000 /* add SpecializationActive[NFIPosixSupport.lseek(NFIPosixSupport, int, long, int, InvokeNativeFunction)] */;
this.state_0_ = state_0;
return arg0Value.lseek(arg1Value, arg2Value, arg3Value, invoke_);
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#ftruncate(NFIPosixSupport, int, long, InvokeNativeFunction)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public void ftruncate(Object arg0Value_, int arg1Value, long arg2Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_0 = this.state_0_;
if ((state_0 & 0x80000) != 0 /* is SpecializationActive[NFIPosixSupport.ftruncate(NFIPosixSupport, int, long, InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
arg0Value.ftruncate(arg1Value, arg2Value, invoke_);
return;
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
ftruncateNode_AndSpecialize(arg0Value, arg1Value, arg2Value);
return;
}
private void ftruncateNode_AndSpecialize(NFIPosixSupport arg0Value, int arg1Value, long arg2Value) throws PosixException {
int state_0 = this.state_0_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'ftruncate(NFIPosixSupport, int, long, InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_0 = state_0 | 0x80000 /* add SpecializationActive[NFIPosixSupport.ftruncate(NFIPosixSupport, int, long, InvokeNativeFunction)] */;
this.state_0_ = state_0;
arg0Value.ftruncate(arg1Value, arg2Value, invoke_);
return;
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#fsync(NFIPosixSupport, int, InvokeNativeFunction)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public void fsync(Object arg0Value_, int arg1Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_0 = this.state_0_;
if ((state_0 & 0x100000) != 0 /* is SpecializationActive[NFIPosixSupport.fsync(NFIPosixSupport, int, InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
arg0Value.fsync(arg1Value, invoke_);
return;
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
fsyncNode_AndSpecialize(arg0Value, arg1Value);
return;
}
private void fsyncNode_AndSpecialize(NFIPosixSupport arg0Value, int arg1Value) throws PosixException {
int state_0 = this.state_0_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'fsync(NFIPosixSupport, int, InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_0 = state_0 | 0x100000 /* add SpecializationActive[NFIPosixSupport.fsync(NFIPosixSupport, int, InvokeNativeFunction)] */;
this.state_0_ = state_0;
arg0Value.fsync(arg1Value, invoke_);
return;
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#flock(NFIPosixSupport, int, int, InvokeNativeFunction)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public void flock(Object arg0Value_, int arg1Value, int arg2Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_0 = this.state_0_;
if ((state_0 & 0x200000) != 0 /* is SpecializationActive[NFIPosixSupport.flock(NFIPosixSupport, int, int, InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
arg0Value.flock(arg1Value, arg2Value, invoke_);
return;
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
flockNode_AndSpecialize(arg0Value, arg1Value, arg2Value);
return;
}
private void flockNode_AndSpecialize(NFIPosixSupport arg0Value, int arg1Value, int arg2Value) throws PosixException {
int state_0 = this.state_0_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'flock(NFIPosixSupport, int, int, InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_0 = state_0 | 0x200000 /* add SpecializationActive[NFIPosixSupport.flock(NFIPosixSupport, int, int, InvokeNativeFunction)] */;
this.state_0_ = state_0;
arg0Value.flock(arg1Value, arg2Value, invoke_);
return;
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#getBlocking(NFIPosixSupport, int, InvokeNativeFunction)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public boolean getBlocking(Object arg0Value_, int arg1Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_0 = this.state_0_;
if ((state_0 & 0x400000) != 0 /* is SpecializationActive[NFIPosixSupport.getBlocking(NFIPosixSupport, int, InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
return arg0Value.getBlocking(arg1Value, invoke_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return getBlockingNode_AndSpecialize(arg0Value, arg1Value);
}
private boolean getBlockingNode_AndSpecialize(NFIPosixSupport arg0Value, int arg1Value) throws PosixException {
int state_0 = this.state_0_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'getBlocking(NFIPosixSupport, int, InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_0 = state_0 | 0x400000 /* add SpecializationActive[NFIPosixSupport.getBlocking(NFIPosixSupport, int, InvokeNativeFunction)] */;
this.state_0_ = state_0;
return arg0Value.getBlocking(arg1Value, invoke_);
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#setBlocking(NFIPosixSupport, int, boolean, InvokeNativeFunction)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public void setBlocking(Object arg0Value_, int arg1Value, boolean arg2Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_0 = this.state_0_;
if ((state_0 & 0x800000) != 0 /* is SpecializationActive[NFIPosixSupport.setBlocking(NFIPosixSupport, int, boolean, InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
arg0Value.setBlocking(arg1Value, arg2Value, invoke_);
return;
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
setBlockingNode_AndSpecialize(arg0Value, arg1Value, arg2Value);
return;
}
private void setBlockingNode_AndSpecialize(NFIPosixSupport arg0Value, int arg1Value, boolean arg2Value) throws PosixException {
int state_0 = this.state_0_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'setBlocking(NFIPosixSupport, int, boolean, InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_0 = state_0 | 0x800000 /* add SpecializationActive[NFIPosixSupport.setBlocking(NFIPosixSupport, int, boolean, InvokeNativeFunction)] */;
this.state_0_ = state_0;
arg0Value.setBlocking(arg1Value, arg2Value, invoke_);
return;
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#getTerminalSize(NFIPosixSupport, int, InvokeNativeFunction)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public int[] getTerminalSize(Object arg0Value_, int arg1Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_0 = this.state_0_;
if ((state_0 & 0x1000000) != 0 /* is SpecializationActive[NFIPosixSupport.getTerminalSize(NFIPosixSupport, int, InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
return arg0Value.getTerminalSize(arg1Value, invoke_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return getTerminalSizeNode_AndSpecialize(arg0Value, arg1Value);
}
private int[] getTerminalSizeNode_AndSpecialize(NFIPosixSupport arg0Value, int arg1Value) throws PosixException {
int state_0 = this.state_0_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'getTerminalSize(NFIPosixSupport, int, InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_0 = state_0 | 0x1000000 /* add SpecializationActive[NFIPosixSupport.getTerminalSize(NFIPosixSupport, int, InvokeNativeFunction)] */;
this.state_0_ = state_0;
return arg0Value.getTerminalSize(arg1Value, invoke_);
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#fstatat(NFIPosixSupport, int, Object, boolean, InvokeNativeFunction)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public long[] fstatat(Object arg0Value_, int arg1Value, Object arg2Value, boolean arg3Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_0 = this.state_0_;
if ((state_0 & 0x2000000) != 0 /* is SpecializationActive[NFIPosixSupport.fstatat(NFIPosixSupport, int, Object, boolean, InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
return arg0Value.fstatat(arg1Value, arg2Value, arg3Value, invoke_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return fstatatNode_AndSpecialize(arg0Value, arg1Value, arg2Value, arg3Value);
}
private long[] fstatatNode_AndSpecialize(NFIPosixSupport arg0Value, int arg1Value, Object arg2Value, boolean arg3Value) throws PosixException {
int state_0 = this.state_0_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'fstatat(NFIPosixSupport, int, Object, boolean, InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_0 = state_0 | 0x2000000 /* add SpecializationActive[NFIPosixSupport.fstatat(NFIPosixSupport, int, Object, boolean, InvokeNativeFunction)] */;
this.state_0_ = state_0;
return arg0Value.fstatat(arg1Value, arg2Value, arg3Value, invoke_);
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#fstat(NFIPosixSupport, int, InvokeNativeFunction)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public long[] fstat(Object arg0Value_, int arg1Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_0 = this.state_0_;
if ((state_0 & 0x4000000) != 0 /* is SpecializationActive[NFIPosixSupport.fstat(NFIPosixSupport, int, InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
return arg0Value.fstat(arg1Value, invoke_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return fstatNode_AndSpecialize(arg0Value, arg1Value);
}
private long[] fstatNode_AndSpecialize(NFIPosixSupport arg0Value, int arg1Value) throws PosixException {
int state_0 = this.state_0_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'fstat(NFIPosixSupport, int, InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_0 = state_0 | 0x4000000 /* add SpecializationActive[NFIPosixSupport.fstat(NFIPosixSupport, int, InvokeNativeFunction)] */;
this.state_0_ = state_0;
return arg0Value.fstat(arg1Value, invoke_);
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#statvfs(NFIPosixSupport, Object, InvokeNativeFunction)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public long[] statvfs(Object arg0Value_, Object arg1Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_0 = this.state_0_;
if ((state_0 & 0x8000000) != 0 /* is SpecializationActive[NFIPosixSupport.statvfs(NFIPosixSupport, Object, InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
return arg0Value.statvfs(arg1Value, invoke_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return statvfsNode_AndSpecialize(arg0Value, arg1Value);
}
private long[] statvfsNode_AndSpecialize(NFIPosixSupport arg0Value, Object arg1Value) throws PosixException {
int state_0 = this.state_0_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'statvfs(NFIPosixSupport, Object, InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_0 = state_0 | 0x8000000 /* add SpecializationActive[NFIPosixSupport.statvfs(NFIPosixSupport, Object, InvokeNativeFunction)] */;
this.state_0_ = state_0;
return arg0Value.statvfs(arg1Value, invoke_);
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#fstatvfs(NFIPosixSupport, int, InvokeNativeFunction)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public long[] fstatvfs(Object arg0Value_, int arg1Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_0 = this.state_0_;
if ((state_0 & 0x10000000) != 0 /* is SpecializationActive[NFIPosixSupport.fstatvfs(NFIPosixSupport, int, InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
return arg0Value.fstatvfs(arg1Value, invoke_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return fstatvfsNode_AndSpecialize(arg0Value, arg1Value);
}
private long[] fstatvfsNode_AndSpecialize(NFIPosixSupport arg0Value, int arg1Value) throws PosixException {
int state_0 = this.state_0_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'fstatvfs(NFIPosixSupport, int, InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_0 = state_0 | 0x10000000 /* add SpecializationActive[NFIPosixSupport.fstatvfs(NFIPosixSupport, int, InvokeNativeFunction)] */;
this.state_0_ = state_0;
return arg0Value.fstatvfs(arg1Value, invoke_);
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#uname(NFIPosixSupport, InvokeNativeFunction, FromByteArrayNode, SwitchEncodingNode)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public Object[] uname(Object arg0Value_) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_0 = this.state_0_;
if ((state_0 & 0x20000000) != 0 /* is SpecializationActive[NFIPosixSupport.uname(NFIPosixSupport, InvokeNativeFunction, FromByteArrayNode, SwitchEncodingNode)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
FromByteArrayNode tsFromBytes_ = this.tsFromBytes;
if (tsFromBytes_ != null) {
SwitchEncodingNode fromUtf8_ = this.fromUtf8;
if (fromUtf8_ != null) {
return arg0Value.uname(invoke_, tsFromBytes_, fromUtf8_);
}
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return unameNode_AndSpecialize(arg0Value);
}
private Object[] unameNode_AndSpecialize(NFIPosixSupport arg0Value) throws PosixException {
int state_0 = this.state_0_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'uname(NFIPosixSupport, InvokeNativeFunction, FromByteArrayNode, SwitchEncodingNode)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
FromByteArrayNode tsFromBytes_;
FromByteArrayNode tsFromBytes__shared = this.tsFromBytes;
if (tsFromBytes__shared != null) {
tsFromBytes_ = tsFromBytes__shared;
} else {
tsFromBytes_ = this.insert((FromByteArrayNode.create()));
if (tsFromBytes_ == null) {
throw new IllegalStateException("Specialization 'uname(NFIPosixSupport, InvokeNativeFunction, FromByteArrayNode, SwitchEncodingNode)' contains a shared cache with name 'fromByteArrayNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.tsFromBytes == null) {
VarHandle.storeStoreFence();
this.tsFromBytes = tsFromBytes_;
}
SwitchEncodingNode fromUtf8_;
SwitchEncodingNode fromUtf8__shared = this.fromUtf8;
if (fromUtf8__shared != null) {
fromUtf8_ = fromUtf8__shared;
} else {
fromUtf8_ = this.insert((SwitchEncodingNode.create()));
if (fromUtf8_ == null) {
throw new IllegalStateException("Specialization 'uname(NFIPosixSupport, InvokeNativeFunction, FromByteArrayNode, SwitchEncodingNode)' contains a shared cache with name 'switchEncodingFromUtf8Node' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.fromUtf8 == null) {
VarHandle.storeStoreFence();
this.fromUtf8 = fromUtf8_;
}
state_0 = state_0 | 0x20000000 /* add SpecializationActive[NFIPosixSupport.uname(NFIPosixSupport, InvokeNativeFunction, FromByteArrayNode, SwitchEncodingNode)] */;
this.state_0_ = state_0;
return arg0Value.uname(invoke_, tsFromBytes_, fromUtf8_);
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#unlinkat(NFIPosixSupport, int, Object, boolean, InvokeNativeFunction)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public void unlinkat(Object arg0Value_, int arg1Value, Object arg2Value, boolean arg3Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_0 = this.state_0_;
if ((state_0 & 0x40000000) != 0 /* is SpecializationActive[NFIPosixSupport.unlinkat(NFIPosixSupport, int, Object, boolean, InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
arg0Value.unlinkat(arg1Value, arg2Value, arg3Value, invoke_);
return;
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
unlinkatNode_AndSpecialize(arg0Value, arg1Value, arg2Value, arg3Value);
return;
}
private void unlinkatNode_AndSpecialize(NFIPosixSupport arg0Value, int arg1Value, Object arg2Value, boolean arg3Value) throws PosixException {
int state_0 = this.state_0_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'unlinkat(NFIPosixSupport, int, Object, boolean, InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_0 = state_0 | 0x40000000 /* add SpecializationActive[NFIPosixSupport.unlinkat(NFIPosixSupport, int, Object, boolean, InvokeNativeFunction)] */;
this.state_0_ = state_0;
arg0Value.unlinkat(arg1Value, arg2Value, arg3Value, invoke_);
return;
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#linkat(NFIPosixSupport, int, Object, int, Object, int, InvokeNativeFunction)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public void linkat(Object arg0Value_, int arg1Value, Object arg2Value, int arg3Value, Object arg4Value, int arg5Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_0 = this.state_0_;
if ((state_0 & 0x80000000) != 0 /* is SpecializationActive[NFIPosixSupport.linkat(NFIPosixSupport, int, Object, int, Object, int, InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
arg0Value.linkat(arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, invoke_);
return;
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
linkatNode_AndSpecialize(arg0Value, arg1Value, arg2Value, arg3Value, arg4Value, arg5Value);
return;
}
private void linkatNode_AndSpecialize(NFIPosixSupport arg0Value, int arg1Value, Object arg2Value, int arg3Value, Object arg4Value, int arg5Value) throws PosixException {
int state_0 = this.state_0_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'linkat(NFIPosixSupport, int, Object, int, Object, int, InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_0 = state_0 | 0x80000000 /* add SpecializationActive[NFIPosixSupport.linkat(NFIPosixSupport, int, Object, int, Object, int, InvokeNativeFunction)] */;
this.state_0_ = state_0;
arg0Value.linkat(arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, invoke_);
return;
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#symlinkat(NFIPosixSupport, Object, int, Object, InvokeNativeFunction)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public void symlinkat(Object arg0Value_, Object arg1Value, int arg2Value, Object arg3Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_1 = this.state_1_;
if ((state_1 & 0b1) != 0 /* is SpecializationActive[NFIPosixSupport.symlinkat(NFIPosixSupport, Object, int, Object, InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
arg0Value.symlinkat(arg1Value, arg2Value, arg3Value, invoke_);
return;
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
symlinkatNode_AndSpecialize(arg0Value, arg1Value, arg2Value, arg3Value);
return;
}
private void symlinkatNode_AndSpecialize(NFIPosixSupport arg0Value, Object arg1Value, int arg2Value, Object arg3Value) throws PosixException {
int state_1 = this.state_1_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'symlinkat(NFIPosixSupport, Object, int, Object, InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_1 = state_1 | 0b1 /* add SpecializationActive[NFIPosixSupport.symlinkat(NFIPosixSupport, Object, int, Object, InvokeNativeFunction)] */;
this.state_1_ = state_1;
arg0Value.symlinkat(arg1Value, arg2Value, arg3Value, invoke_);
return;
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#mkdirat(NFIPosixSupport, int, Object, int, InvokeNativeFunction)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public void mkdirat(Object arg0Value_, int arg1Value, Object arg2Value, int arg3Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_1 = this.state_1_;
if ((state_1 & 0b10) != 0 /* is SpecializationActive[NFIPosixSupport.mkdirat(NFIPosixSupport, int, Object, int, InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
arg0Value.mkdirat(arg1Value, arg2Value, arg3Value, invoke_);
return;
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
mkdiratNode_AndSpecialize(arg0Value, arg1Value, arg2Value, arg3Value);
return;
}
private void mkdiratNode_AndSpecialize(NFIPosixSupport arg0Value, int arg1Value, Object arg2Value, int arg3Value) throws PosixException {
int state_1 = this.state_1_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'mkdirat(NFIPosixSupport, int, Object, int, InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_1 = state_1 | 0b10 /* add SpecializationActive[NFIPosixSupport.mkdirat(NFIPosixSupport, int, Object, int, InvokeNativeFunction)] */;
this.state_1_ = state_1;
arg0Value.mkdirat(arg1Value, arg2Value, arg3Value, invoke_);
return;
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#getcwd(NFIPosixSupport, InvokeNativeFunction)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public Object getcwd(Object arg0Value_) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_1 = this.state_1_;
if ((state_1 & 0b100) != 0 /* is SpecializationActive[NFIPosixSupport.getcwd(NFIPosixSupport, InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
return arg0Value.getcwd(invoke_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return getcwdNode_AndSpecialize(arg0Value);
}
private Object getcwdNode_AndSpecialize(NFIPosixSupport arg0Value) throws PosixException {
int state_1 = this.state_1_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'getcwd(NFIPosixSupport, InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_1 = state_1 | 0b100 /* add SpecializationActive[NFIPosixSupport.getcwd(NFIPosixSupport, InvokeNativeFunction)] */;
this.state_1_ = state_1;
return arg0Value.getcwd(invoke_);
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#chdir(NFIPosixSupport, Object, InvokeNativeFunction)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public void chdir(Object arg0Value_, Object arg1Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_1 = this.state_1_;
if ((state_1 & 0b1000) != 0 /* is SpecializationActive[NFIPosixSupport.chdir(NFIPosixSupport, Object, InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
arg0Value.chdir(arg1Value, invoke_);
return;
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
chdirNode_AndSpecialize(arg0Value, arg1Value);
return;
}
private void chdirNode_AndSpecialize(NFIPosixSupport arg0Value, Object arg1Value) throws PosixException {
int state_1 = this.state_1_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'chdir(NFIPosixSupport, Object, InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_1 = state_1 | 0b1000 /* add SpecializationActive[NFIPosixSupport.chdir(NFIPosixSupport, Object, InvokeNativeFunction)] */;
this.state_1_ = state_1;
arg0Value.chdir(arg1Value, invoke_);
return;
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#fchdir(NFIPosixSupport, int, InvokeNativeFunction)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public void fchdir(Object arg0Value_, int arg1Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_1 = this.state_1_;
if ((state_1 & 0b10000) != 0 /* is SpecializationActive[NFIPosixSupport.fchdir(NFIPosixSupport, int, InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
arg0Value.fchdir(arg1Value, invoke_);
return;
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
fchdirNode_AndSpecialize(arg0Value, arg1Value);
return;
}
private void fchdirNode_AndSpecialize(NFIPosixSupport arg0Value, int arg1Value) throws PosixException {
int state_1 = this.state_1_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'fchdir(NFIPosixSupport, int, InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_1 = state_1 | 0b10000 /* add SpecializationActive[NFIPosixSupport.fchdir(NFIPosixSupport, int, InvokeNativeFunction)] */;
this.state_1_ = state_1;
arg0Value.fchdir(arg1Value, invoke_);
return;
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#isatty(NFIPosixSupport, int, InvokeNativeFunction)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public boolean isatty(Object arg0Value_, int arg1Value) {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_1 = this.state_1_;
if ((state_1 & 0b100000) != 0 /* is SpecializationActive[NFIPosixSupport.isatty(NFIPosixSupport, int, InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
return arg0Value.isatty(arg1Value, invoke_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return isattyNode_AndSpecialize(arg0Value, arg1Value);
}
private boolean isattyNode_AndSpecialize(NFIPosixSupport arg0Value, int arg1Value) {
int state_1 = this.state_1_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'isatty(NFIPosixSupport, int, InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_1 = state_1 | 0b100000 /* add SpecializationActive[NFIPosixSupport.isatty(NFIPosixSupport, int, InvokeNativeFunction)] */;
this.state_1_ = state_1;
return arg0Value.isatty(arg1Value, invoke_);
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#opendir(NFIPosixSupport, Object, InvokeNativeFunction)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public Object opendir(Object arg0Value_, Object arg1Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_1 = this.state_1_;
if ((state_1 & 0b1000000) != 0 /* is SpecializationActive[NFIPosixSupport.opendir(NFIPosixSupport, Object, InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
return arg0Value.opendir(arg1Value, invoke_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return opendirNode_AndSpecialize(arg0Value, arg1Value);
}
private Object opendirNode_AndSpecialize(NFIPosixSupport arg0Value, Object arg1Value) throws PosixException {
int state_1 = this.state_1_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'opendir(NFIPosixSupport, Object, InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_1 = state_1 | 0b1000000 /* add SpecializationActive[NFIPosixSupport.opendir(NFIPosixSupport, Object, InvokeNativeFunction)] */;
this.state_1_ = state_1;
return arg0Value.opendir(arg1Value, invoke_);
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#fdopendir(NFIPosixSupport, int, InvokeNativeFunction)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public Object fdopendir(Object arg0Value_, int arg1Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_1 = this.state_1_;
if ((state_1 & 0b10000000) != 0 /* is SpecializationActive[NFIPosixSupport.fdopendir(NFIPosixSupport, int, InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
return arg0Value.fdopendir(arg1Value, invoke_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return fdopendirNode_AndSpecialize(arg0Value, arg1Value);
}
private Object fdopendirNode_AndSpecialize(NFIPosixSupport arg0Value, int arg1Value) throws PosixException {
int state_1 = this.state_1_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'fdopendir(NFIPosixSupport, int, InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_1 = state_1 | 0b10000000 /* add SpecializationActive[NFIPosixSupport.fdopendir(NFIPosixSupport, int, InvokeNativeFunction)] */;
this.state_1_ = state_1;
return arg0Value.fdopendir(arg1Value, invoke_);
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#closedir(NFIPosixSupport, Object, InvokeNativeFunction)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public void closedir(Object arg0Value_, Object arg1Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_1 = this.state_1_;
if ((state_1 & 0b100000000) != 0 /* is SpecializationActive[NFIPosixSupport.closedir(NFIPosixSupport, Object, InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
arg0Value.closedir(arg1Value, invoke_);
return;
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
closedirNode_AndSpecialize(arg0Value, arg1Value);
return;
}
private void closedirNode_AndSpecialize(NFIPosixSupport arg0Value, Object arg1Value) throws PosixException {
int state_1 = this.state_1_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'closedir(NFIPosixSupport, Object, InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_1 = state_1 | 0b100000000 /* add SpecializationActive[NFIPosixSupport.closedir(NFIPosixSupport, Object, InvokeNativeFunction)] */;
this.state_1_ = state_1;
arg0Value.closedir(arg1Value, invoke_);
return;
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#readdir(NFIPosixSupport, Object, InvokeNativeFunction)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public Object readdir(Object arg0Value_, Object arg1Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_1 = this.state_1_;
if ((state_1 & 0b1000000000) != 0 /* is SpecializationActive[NFIPosixSupport.readdir(NFIPosixSupport, Object, InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
return arg0Value.readdir(arg1Value, invoke_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return readdirNode_AndSpecialize(arg0Value, arg1Value);
}
private Object readdirNode_AndSpecialize(NFIPosixSupport arg0Value, Object arg1Value) throws PosixException {
int state_1 = this.state_1_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'readdir(NFIPosixSupport, Object, InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_1 = state_1 | 0b1000000000 /* add SpecializationActive[NFIPosixSupport.readdir(NFIPosixSupport, Object, InvokeNativeFunction)] */;
this.state_1_ = state_1;
return arg0Value.readdir(arg1Value, invoke_);
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#rewinddir(NFIPosixSupport, Object, InvokeNativeFunction)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public void rewinddir(Object arg0Value_, Object arg1Value) {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_1 = this.state_1_;
if ((state_1 & 0b10000000000) != 0 /* is SpecializationActive[NFIPosixSupport.rewinddir(NFIPosixSupport, Object, InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
arg0Value.rewinddir(arg1Value, invoke_);
return;
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
rewinddirNode_AndSpecialize(arg0Value, arg1Value);
return;
}
private void rewinddirNode_AndSpecialize(NFIPosixSupport arg0Value, Object arg1Value) {
int state_1 = this.state_1_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'rewinddir(NFIPosixSupport, Object, InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_1 = state_1 | 0b10000000000 /* add SpecializationActive[NFIPosixSupport.rewinddir(NFIPosixSupport, Object, InvokeNativeFunction)] */;
this.state_1_ = state_1;
arg0Value.rewinddir(arg1Value, invoke_);
return;
}
@Override
public Object dirEntryGetName(Object receiver, Object dirEntry) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
return (((NFIPosixSupport) receiver)).dirEntryGetName(dirEntry);
}
@Override
public long dirEntryGetInode(Object receiver, Object dirEntry) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
return (((NFIPosixSupport) receiver)).dirEntryGetInode(dirEntry);
}
@Override
public int dirEntryGetType(Object receiver, Object dirEntry) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
return (((NFIPosixSupport) receiver)).dirEntryGetType(dirEntry);
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#utimensat(NFIPosixSupport, int, Object, long[], boolean, InvokeNativeFunction)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public void utimensat(Object arg0Value_, int arg1Value, Object arg2Value, long[] arg3Value, boolean arg4Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_1 = this.state_1_;
if ((state_1 & 0b100000000000) != 0 /* is SpecializationActive[NFIPosixSupport.utimensat(NFIPosixSupport, int, Object, long[], boolean, InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
arg0Value.utimensat(arg1Value, arg2Value, arg3Value, arg4Value, invoke_);
return;
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
utimensatNode_AndSpecialize(arg0Value, arg1Value, arg2Value, arg3Value, arg4Value);
return;
}
private void utimensatNode_AndSpecialize(NFIPosixSupport arg0Value, int arg1Value, Object arg2Value, long[] arg3Value, boolean arg4Value) throws PosixException {
int state_1 = this.state_1_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'utimensat(NFIPosixSupport, int, Object, long[], boolean, InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_1 = state_1 | 0b100000000000 /* add SpecializationActive[NFIPosixSupport.utimensat(NFIPosixSupport, int, Object, long[], boolean, InvokeNativeFunction)] */;
this.state_1_ = state_1;
arg0Value.utimensat(arg1Value, arg2Value, arg3Value, arg4Value, invoke_);
return;
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#futimens(NFIPosixSupport, int, long[], InvokeNativeFunction)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public void futimens(Object arg0Value_, int arg1Value, long[] arg2Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_1 = this.state_1_;
if ((state_1 & 0b1000000000000) != 0 /* is SpecializationActive[NFIPosixSupport.futimens(NFIPosixSupport, int, long[], InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
arg0Value.futimens(arg1Value, arg2Value, invoke_);
return;
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
futimensNode_AndSpecialize(arg0Value, arg1Value, arg2Value);
return;
}
private void futimensNode_AndSpecialize(NFIPosixSupport arg0Value, int arg1Value, long[] arg2Value) throws PosixException {
int state_1 = this.state_1_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'futimens(NFIPosixSupport, int, long[], InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_1 = state_1 | 0b1000000000000 /* add SpecializationActive[NFIPosixSupport.futimens(NFIPosixSupport, int, long[], InvokeNativeFunction)] */;
this.state_1_ = state_1;
arg0Value.futimens(arg1Value, arg2Value, invoke_);
return;
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#futimes(NFIPosixSupport, int, Timeval[], InvokeNativeFunction)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public void futimes(Object arg0Value_, int arg1Value, Timeval[] arg2Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_1 = this.state_1_;
if ((state_1 & 0b10000000000000) != 0 /* is SpecializationActive[NFIPosixSupport.futimes(NFIPosixSupport, int, Timeval[], InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
arg0Value.futimes(arg1Value, arg2Value, invoke_);
return;
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
futimesNode_AndSpecialize(arg0Value, arg1Value, arg2Value);
return;
}
private void futimesNode_AndSpecialize(NFIPosixSupport arg0Value, int arg1Value, Timeval[] arg2Value) throws PosixException {
int state_1 = this.state_1_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'futimes(NFIPosixSupport, int, Timeval[], InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_1 = state_1 | 0b10000000000000 /* add SpecializationActive[NFIPosixSupport.futimes(NFIPosixSupport, int, Timeval[], InvokeNativeFunction)] */;
this.state_1_ = state_1;
arg0Value.futimes(arg1Value, arg2Value, invoke_);
return;
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#lutimes(NFIPosixSupport, Object, Timeval[], InvokeNativeFunction)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public void lutimes(Object arg0Value_, Object arg1Value, Timeval[] arg2Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_1 = this.state_1_;
if ((state_1 & 0b100000000000000) != 0 /* is SpecializationActive[NFIPosixSupport.lutimes(NFIPosixSupport, Object, Timeval[], InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
arg0Value.lutimes(arg1Value, arg2Value, invoke_);
return;
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
lutimesNode_AndSpecialize(arg0Value, arg1Value, arg2Value);
return;
}
private void lutimesNode_AndSpecialize(NFIPosixSupport arg0Value, Object arg1Value, Timeval[] arg2Value) throws PosixException {
int state_1 = this.state_1_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'lutimes(NFIPosixSupport, Object, Timeval[], InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_1 = state_1 | 0b100000000000000 /* add SpecializationActive[NFIPosixSupport.lutimes(NFIPosixSupport, Object, Timeval[], InvokeNativeFunction)] */;
this.state_1_ = state_1;
arg0Value.lutimes(arg1Value, arg2Value, invoke_);
return;
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#utimes(NFIPosixSupport, Object, Timeval[], InvokeNativeFunction)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public void utimes(Object arg0Value_, Object arg1Value, Timeval[] arg2Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_1 = this.state_1_;
if ((state_1 & 0b1000000000000000) != 0 /* is SpecializationActive[NFIPosixSupport.utimes(NFIPosixSupport, Object, Timeval[], InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
arg0Value.utimes(arg1Value, arg2Value, invoke_);
return;
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
utimesNode_AndSpecialize(arg0Value, arg1Value, arg2Value);
return;
}
private void utimesNode_AndSpecialize(NFIPosixSupport arg0Value, Object arg1Value, Timeval[] arg2Value) throws PosixException {
int state_1 = this.state_1_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'utimes(NFIPosixSupport, Object, Timeval[], InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_1 = state_1 | 0b1000000000000000 /* add SpecializationActive[NFIPosixSupport.utimes(NFIPosixSupport, Object, Timeval[], InvokeNativeFunction)] */;
this.state_1_ = state_1;
arg0Value.utimes(arg1Value, arg2Value, invoke_);
return;
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#renameat(NFIPosixSupport, int, Object, int, Object, InvokeNativeFunction)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public void renameat(Object arg0Value_, int arg1Value, Object arg2Value, int arg3Value, Object arg4Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_1 = this.state_1_;
if ((state_1 & 0x10000) != 0 /* is SpecializationActive[NFIPosixSupport.renameat(NFIPosixSupport, int, Object, int, Object, InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
arg0Value.renameat(arg1Value, arg2Value, arg3Value, arg4Value, invoke_);
return;
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
renameatNode_AndSpecialize(arg0Value, arg1Value, arg2Value, arg3Value, arg4Value);
return;
}
private void renameatNode_AndSpecialize(NFIPosixSupport arg0Value, int arg1Value, Object arg2Value, int arg3Value, Object arg4Value) throws PosixException {
int state_1 = this.state_1_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'renameat(NFIPosixSupport, int, Object, int, Object, InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_1 = state_1 | 0x10000 /* add SpecializationActive[NFIPosixSupport.renameat(NFIPosixSupport, int, Object, int, Object, InvokeNativeFunction)] */;
this.state_1_ = state_1;
arg0Value.renameat(arg1Value, arg2Value, arg3Value, arg4Value, invoke_);
return;
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#faccessat(NFIPosixSupport, int, Object, int, boolean, boolean, InvokeNativeFunction)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public boolean faccessat(Object arg0Value_, int arg1Value, Object arg2Value, int arg3Value, boolean arg4Value, boolean arg5Value) {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_1 = this.state_1_;
if ((state_1 & 0x20000) != 0 /* is SpecializationActive[NFIPosixSupport.faccessat(NFIPosixSupport, int, Object, int, boolean, boolean, InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
return arg0Value.faccessat(arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, invoke_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return faccessatNode_AndSpecialize(arg0Value, arg1Value, arg2Value, arg3Value, arg4Value, arg5Value);
}
private boolean faccessatNode_AndSpecialize(NFIPosixSupport arg0Value, int arg1Value, Object arg2Value, int arg3Value, boolean arg4Value, boolean arg5Value) {
int state_1 = this.state_1_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'faccessat(NFIPosixSupport, int, Object, int, boolean, boolean, InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_1 = state_1 | 0x20000 /* add SpecializationActive[NFIPosixSupport.faccessat(NFIPosixSupport, int, Object, int, boolean, boolean, InvokeNativeFunction)] */;
this.state_1_ = state_1;
return arg0Value.faccessat(arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, invoke_);
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#fchmodat(NFIPosixSupport, int, Object, int, boolean, InvokeNativeFunction)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public void fchmodat(Object arg0Value_, int arg1Value, Object arg2Value, int arg3Value, boolean arg4Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_1 = this.state_1_;
if ((state_1 & 0x40000) != 0 /* is SpecializationActive[NFIPosixSupport.fchmodat(NFIPosixSupport, int, Object, int, boolean, InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
arg0Value.fchmodat(arg1Value, arg2Value, arg3Value, arg4Value, invoke_);
return;
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
fchmodatNode_AndSpecialize(arg0Value, arg1Value, arg2Value, arg3Value, arg4Value);
return;
}
private void fchmodatNode_AndSpecialize(NFIPosixSupport arg0Value, int arg1Value, Object arg2Value, int arg3Value, boolean arg4Value) throws PosixException {
int state_1 = this.state_1_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'fchmodat(NFIPosixSupport, int, Object, int, boolean, InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_1 = state_1 | 0x40000 /* add SpecializationActive[NFIPosixSupport.fchmodat(NFIPosixSupport, int, Object, int, boolean, InvokeNativeFunction)] */;
this.state_1_ = state_1;
arg0Value.fchmodat(arg1Value, arg2Value, arg3Value, arg4Value, invoke_);
return;
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#fchmod(NFIPosixSupport, int, int, InvokeNativeFunction)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public void fchmod(Object arg0Value_, int arg1Value, int arg2Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_1 = this.state_1_;
if ((state_1 & 0x80000) != 0 /* is SpecializationActive[NFIPosixSupport.fchmod(NFIPosixSupport, int, int, InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
arg0Value.fchmod(arg1Value, arg2Value, invoke_);
return;
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
fchmodNode_AndSpecialize(arg0Value, arg1Value, arg2Value);
return;
}
private void fchmodNode_AndSpecialize(NFIPosixSupport arg0Value, int arg1Value, int arg2Value) throws PosixException {
int state_1 = this.state_1_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'fchmod(NFIPosixSupport, int, int, InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_1 = state_1 | 0x80000 /* add SpecializationActive[NFIPosixSupport.fchmod(NFIPosixSupport, int, int, InvokeNativeFunction)] */;
this.state_1_ = state_1;
arg0Value.fchmod(arg1Value, arg2Value, invoke_);
return;
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#readlinkat(NFIPosixSupport, int, Object, InvokeNativeFunction)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public Object readlinkat(Object arg0Value_, int arg1Value, Object arg2Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_1 = this.state_1_;
if ((state_1 & 0x100000) != 0 /* is SpecializationActive[NFIPosixSupport.readlinkat(NFIPosixSupport, int, Object, InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
return arg0Value.readlinkat(arg1Value, arg2Value, invoke_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return readlinkatNode_AndSpecialize(arg0Value, arg1Value, arg2Value);
}
private Object readlinkatNode_AndSpecialize(NFIPosixSupport arg0Value, int arg1Value, Object arg2Value) throws PosixException {
int state_1 = this.state_1_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'readlinkat(NFIPosixSupport, int, Object, InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_1 = state_1 | 0x100000 /* add SpecializationActive[NFIPosixSupport.readlinkat(NFIPosixSupport, int, Object, InvokeNativeFunction)] */;
this.state_1_ = state_1;
return arg0Value.readlinkat(arg1Value, arg2Value, invoke_);
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#kill(NFIPosixSupport, long, int, InvokeNativeFunction)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public void kill(Object arg0Value_, long arg1Value, int arg2Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_1 = this.state_1_;
if ((state_1 & 0x200000) != 0 /* is SpecializationActive[NFIPosixSupport.kill(NFIPosixSupport, long, int, InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
arg0Value.kill(arg1Value, arg2Value, invoke_);
return;
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
killNode_AndSpecialize(arg0Value, arg1Value, arg2Value);
return;
}
private void killNode_AndSpecialize(NFIPosixSupport arg0Value, long arg1Value, int arg2Value) throws PosixException {
int state_1 = this.state_1_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'kill(NFIPosixSupport, long, int, InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_1 = state_1 | 0x200000 /* add SpecializationActive[NFIPosixSupport.kill(NFIPosixSupport, long, int, InvokeNativeFunction)] */;
this.state_1_ = state_1;
arg0Value.kill(arg1Value, arg2Value, invoke_);
return;
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#killpg(NFIPosixSupport, long, int, InvokeNativeFunction)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public void killpg(Object arg0Value_, long arg1Value, int arg2Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_1 = this.state_1_;
if ((state_1 & 0x400000) != 0 /* is SpecializationActive[NFIPosixSupport.killpg(NFIPosixSupport, long, int, InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
arg0Value.killpg(arg1Value, arg2Value, invoke_);
return;
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
killpgNode_AndSpecialize(arg0Value, arg1Value, arg2Value);
return;
}
private void killpgNode_AndSpecialize(NFIPosixSupport arg0Value, long arg1Value, int arg2Value) throws PosixException {
int state_1 = this.state_1_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'killpg(NFIPosixSupport, long, int, InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_1 = state_1 | 0x400000 /* add SpecializationActive[NFIPosixSupport.killpg(NFIPosixSupport, long, int, InvokeNativeFunction)] */;
this.state_1_ = state_1;
arg0Value.killpg(arg1Value, arg2Value, invoke_);
return;
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#waitpid(NFIPosixSupport, long, int, InvokeNativeFunction)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public long[] waitpid(Object arg0Value_, long arg1Value, int arg2Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_1 = this.state_1_;
if ((state_1 & 0x800000) != 0 /* is SpecializationActive[NFIPosixSupport.waitpid(NFIPosixSupport, long, int, InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
return arg0Value.waitpid(arg1Value, arg2Value, invoke_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return waitpidNode_AndSpecialize(arg0Value, arg1Value, arg2Value);
}
private long[] waitpidNode_AndSpecialize(NFIPosixSupport arg0Value, long arg1Value, int arg2Value) throws PosixException {
int state_1 = this.state_1_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'waitpid(NFIPosixSupport, long, int, InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_1 = state_1 | 0x800000 /* add SpecializationActive[NFIPosixSupport.waitpid(NFIPosixSupport, long, int, InvokeNativeFunction)] */;
this.state_1_ = state_1;
return arg0Value.waitpid(arg1Value, arg2Value, invoke_);
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#wcoredump(NFIPosixSupport, int, InvokeNativeFunction)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public boolean wcoredump(Object arg0Value_, int arg1Value) {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_1 = this.state_1_;
if ((state_1 & 0x1000000) != 0 /* is SpecializationActive[NFIPosixSupport.wcoredump(NFIPosixSupport, int, InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
return arg0Value.wcoredump(arg1Value, invoke_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return wcoredumpNode_AndSpecialize(arg0Value, arg1Value);
}
private boolean wcoredumpNode_AndSpecialize(NFIPosixSupport arg0Value, int arg1Value) {
int state_1 = this.state_1_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'wcoredump(NFIPosixSupport, int, InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_1 = state_1 | 0x1000000 /* add SpecializationActive[NFIPosixSupport.wcoredump(NFIPosixSupport, int, InvokeNativeFunction)] */;
this.state_1_ = state_1;
return arg0Value.wcoredump(arg1Value, invoke_);
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#abort(NFIPosixSupport, InvokeNativeFunction)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public void abort(Object arg0Value_) {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_1 = this.state_1_;
if ((state_1 & 0x2000000) != 0 /* is SpecializationActive[NFIPosixSupport.abort(NFIPosixSupport, InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
arg0Value.abort(invoke_);
return;
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
abortNode_AndSpecialize(arg0Value);
return;
}
private void abortNode_AndSpecialize(NFIPosixSupport arg0Value) {
int state_1 = this.state_1_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'abort(NFIPosixSupport, InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_1 = state_1 | 0x2000000 /* add SpecializationActive[NFIPosixSupport.abort(NFIPosixSupport, InvokeNativeFunction)] */;
this.state_1_ = state_1;
arg0Value.abort(invoke_);
return;
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#wifcontinued(NFIPosixSupport, int, InvokeNativeFunction)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public boolean wifcontinued(Object arg0Value_, int arg1Value) {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_1 = this.state_1_;
if ((state_1 & 0x4000000) != 0 /* is SpecializationActive[NFIPosixSupport.wifcontinued(NFIPosixSupport, int, InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
return arg0Value.wifcontinued(arg1Value, invoke_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return wifcontinuedNode_AndSpecialize(arg0Value, arg1Value);
}
private boolean wifcontinuedNode_AndSpecialize(NFIPosixSupport arg0Value, int arg1Value) {
int state_1 = this.state_1_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'wifcontinued(NFIPosixSupport, int, InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_1 = state_1 | 0x4000000 /* add SpecializationActive[NFIPosixSupport.wifcontinued(NFIPosixSupport, int, InvokeNativeFunction)] */;
this.state_1_ = state_1;
return arg0Value.wifcontinued(arg1Value, invoke_);
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#wifstopped(NFIPosixSupport, int, InvokeNativeFunction)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public boolean wifstopped(Object arg0Value_, int arg1Value) {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_1 = this.state_1_;
if ((state_1 & 0x8000000) != 0 /* is SpecializationActive[NFIPosixSupport.wifstopped(NFIPosixSupport, int, InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
return arg0Value.wifstopped(arg1Value, invoke_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return wifstoppedNode_AndSpecialize(arg0Value, arg1Value);
}
private boolean wifstoppedNode_AndSpecialize(NFIPosixSupport arg0Value, int arg1Value) {
int state_1 = this.state_1_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'wifstopped(NFIPosixSupport, int, InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_1 = state_1 | 0x8000000 /* add SpecializationActive[NFIPosixSupport.wifstopped(NFIPosixSupport, int, InvokeNativeFunction)] */;
this.state_1_ = state_1;
return arg0Value.wifstopped(arg1Value, invoke_);
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#wifsignaled(NFIPosixSupport, int, InvokeNativeFunction)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public boolean wifsignaled(Object arg0Value_, int arg1Value) {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_1 = this.state_1_;
if ((state_1 & 0x10000000) != 0 /* is SpecializationActive[NFIPosixSupport.wifsignaled(NFIPosixSupport, int, InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
return arg0Value.wifsignaled(arg1Value, invoke_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return wifsignaledNode_AndSpecialize(arg0Value, arg1Value);
}
private boolean wifsignaledNode_AndSpecialize(NFIPosixSupport arg0Value, int arg1Value) {
int state_1 = this.state_1_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'wifsignaled(NFIPosixSupport, int, InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_1 = state_1 | 0x10000000 /* add SpecializationActive[NFIPosixSupport.wifsignaled(NFIPosixSupport, int, InvokeNativeFunction)] */;
this.state_1_ = state_1;
return arg0Value.wifsignaled(arg1Value, invoke_);
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#wifexited(NFIPosixSupport, int, InvokeNativeFunction)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public boolean wifexited(Object arg0Value_, int arg1Value) {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_1 = this.state_1_;
if ((state_1 & 0x20000000) != 0 /* is SpecializationActive[NFIPosixSupport.wifexited(NFIPosixSupport, int, InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
return arg0Value.wifexited(arg1Value, invoke_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return wifexitedNode_AndSpecialize(arg0Value, arg1Value);
}
private boolean wifexitedNode_AndSpecialize(NFIPosixSupport arg0Value, int arg1Value) {
int state_1 = this.state_1_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'wifexited(NFIPosixSupport, int, InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_1 = state_1 | 0x20000000 /* add SpecializationActive[NFIPosixSupport.wifexited(NFIPosixSupport, int, InvokeNativeFunction)] */;
this.state_1_ = state_1;
return arg0Value.wifexited(arg1Value, invoke_);
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#wexitstatus(NFIPosixSupport, int, InvokeNativeFunction)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public int wexitstatus(Object arg0Value_, int arg1Value) {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_1 = this.state_1_;
if ((state_1 & 0x40000000) != 0 /* is SpecializationActive[NFIPosixSupport.wexitstatus(NFIPosixSupport, int, InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
return arg0Value.wexitstatus(arg1Value, invoke_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return wexitstatusNode_AndSpecialize(arg0Value, arg1Value);
}
private int wexitstatusNode_AndSpecialize(NFIPosixSupport arg0Value, int arg1Value) {
int state_1 = this.state_1_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'wexitstatus(NFIPosixSupport, int, InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_1 = state_1 | 0x40000000 /* add SpecializationActive[NFIPosixSupport.wexitstatus(NFIPosixSupport, int, InvokeNativeFunction)] */;
this.state_1_ = state_1;
return arg0Value.wexitstatus(arg1Value, invoke_);
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#wtermsig(NFIPosixSupport, int, InvokeNativeFunction)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public int wtermsig(Object arg0Value_, int arg1Value) {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_1 = this.state_1_;
if ((state_1 & 0x80000000) != 0 /* is SpecializationActive[NFIPosixSupport.wtermsig(NFIPosixSupport, int, InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
return arg0Value.wtermsig(arg1Value, invoke_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return wtermsigNode_AndSpecialize(arg0Value, arg1Value);
}
private int wtermsigNode_AndSpecialize(NFIPosixSupport arg0Value, int arg1Value) {
int state_1 = this.state_1_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'wtermsig(NFIPosixSupport, int, InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_1 = state_1 | 0x80000000 /* add SpecializationActive[NFIPosixSupport.wtermsig(NFIPosixSupport, int, InvokeNativeFunction)] */;
this.state_1_ = state_1;
return arg0Value.wtermsig(arg1Value, invoke_);
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#wstopsig(NFIPosixSupport, int, InvokeNativeFunction)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public int wstopsig(Object arg0Value_, int arg1Value) {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_2 = this.state_2_;
if ((state_2 & 0b1) != 0 /* is SpecializationActive[NFIPosixSupport.wstopsig(NFIPosixSupport, int, InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
return arg0Value.wstopsig(arg1Value, invoke_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return wstopsigNode_AndSpecialize(arg0Value, arg1Value);
}
private int wstopsigNode_AndSpecialize(NFIPosixSupport arg0Value, int arg1Value) {
int state_2 = this.state_2_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'wstopsig(NFIPosixSupport, int, InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_2 = state_2 | 0b1 /* add SpecializationActive[NFIPosixSupport.wstopsig(NFIPosixSupport, int, InvokeNativeFunction)] */;
this.state_2_ = state_2;
return arg0Value.wstopsig(arg1Value, invoke_);
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#getuid(NFIPosixSupport, InvokeNativeFunction)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public long getuid(Object arg0Value_) {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_2 = this.state_2_;
if ((state_2 & 0b10) != 0 /* is SpecializationActive[NFIPosixSupport.getuid(NFIPosixSupport, InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
return arg0Value.getuid(invoke_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return getuidNode_AndSpecialize(arg0Value);
}
private long getuidNode_AndSpecialize(NFIPosixSupport arg0Value) {
int state_2 = this.state_2_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'getuid(NFIPosixSupport, InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_2 = state_2 | 0b10 /* add SpecializationActive[NFIPosixSupport.getuid(NFIPosixSupport, InvokeNativeFunction)] */;
this.state_2_ = state_2;
return arg0Value.getuid(invoke_);
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#geteuid(NFIPosixSupport, InvokeNativeFunction)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public long geteuid(Object arg0Value_) {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_2 = this.state_2_;
if ((state_2 & 0b100) != 0 /* is SpecializationActive[NFIPosixSupport.geteuid(NFIPosixSupport, InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
return arg0Value.geteuid(invoke_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return geteuidNode_AndSpecialize(arg0Value);
}
private long geteuidNode_AndSpecialize(NFIPosixSupport arg0Value) {
int state_2 = this.state_2_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'geteuid(NFIPosixSupport, InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_2 = state_2 | 0b100 /* add SpecializationActive[NFIPosixSupport.geteuid(NFIPosixSupport, InvokeNativeFunction)] */;
this.state_2_ = state_2;
return arg0Value.geteuid(invoke_);
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#getgid(NFIPosixSupport, InvokeNativeFunction)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public long getgid(Object arg0Value_) {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_2 = this.state_2_;
if ((state_2 & 0b1000) != 0 /* is SpecializationActive[NFIPosixSupport.getgid(NFIPosixSupport, InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
return arg0Value.getgid(invoke_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return getgidNode_AndSpecialize(arg0Value);
}
private long getgidNode_AndSpecialize(NFIPosixSupport arg0Value) {
int state_2 = this.state_2_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'getgid(NFIPosixSupport, InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_2 = state_2 | 0b1000 /* add SpecializationActive[NFIPosixSupport.getgid(NFIPosixSupport, InvokeNativeFunction)] */;
this.state_2_ = state_2;
return arg0Value.getgid(invoke_);
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#getppid(NFIPosixSupport, InvokeNativeFunction)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public long getppid(Object arg0Value_) {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_2 = this.state_2_;
if ((state_2 & 0b10000) != 0 /* is SpecializationActive[NFIPosixSupport.getppid(NFIPosixSupport, InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
return arg0Value.getppid(invoke_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return getppidNode_AndSpecialize(arg0Value);
}
private long getppidNode_AndSpecialize(NFIPosixSupport arg0Value) {
int state_2 = this.state_2_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'getppid(NFIPosixSupport, InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_2 = state_2 | 0b10000 /* add SpecializationActive[NFIPosixSupport.getppid(NFIPosixSupport, InvokeNativeFunction)] */;
this.state_2_ = state_2;
return arg0Value.getppid(invoke_);
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#setpgid(NFIPosixSupport, long, long, InvokeNativeFunction)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public void setpgid(Object arg0Value_, long arg1Value, long arg2Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_2 = this.state_2_;
if ((state_2 & 0b100000) != 0 /* is SpecializationActive[NFIPosixSupport.setpgid(NFIPosixSupport, long, long, InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
arg0Value.setpgid(arg1Value, arg2Value, invoke_);
return;
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
setpgidNode_AndSpecialize(arg0Value, arg1Value, arg2Value);
return;
}
private void setpgidNode_AndSpecialize(NFIPosixSupport arg0Value, long arg1Value, long arg2Value) throws PosixException {
int state_2 = this.state_2_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'setpgid(NFIPosixSupport, long, long, InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_2 = state_2 | 0b100000 /* add SpecializationActive[NFIPosixSupport.setpgid(NFIPosixSupport, long, long, InvokeNativeFunction)] */;
this.state_2_ = state_2;
arg0Value.setpgid(arg1Value, arg2Value, invoke_);
return;
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#getpgid(NFIPosixSupport, long, InvokeNativeFunction)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public long getpgid(Object arg0Value_, long arg1Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_2 = this.state_2_;
if ((state_2 & 0b1000000) != 0 /* is SpecializationActive[NFIPosixSupport.getpgid(NFIPosixSupport, long, InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
return arg0Value.getpgid(arg1Value, invoke_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return getpgidNode_AndSpecialize(arg0Value, arg1Value);
}
private long getpgidNode_AndSpecialize(NFIPosixSupport arg0Value, long arg1Value) throws PosixException {
int state_2 = this.state_2_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'getpgid(NFIPosixSupport, long, InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_2 = state_2 | 0b1000000 /* add SpecializationActive[NFIPosixSupport.getpgid(NFIPosixSupport, long, InvokeNativeFunction)] */;
this.state_2_ = state_2;
return arg0Value.getpgid(arg1Value, invoke_);
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#getpgrp(NFIPosixSupport, InvokeNativeFunction)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public long getpgrp(Object arg0Value_) {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_2 = this.state_2_;
if ((state_2 & 0b10000000) != 0 /* is SpecializationActive[NFIPosixSupport.getpgrp(NFIPosixSupport, InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
return arg0Value.getpgrp(invoke_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return getpgrpNode_AndSpecialize(arg0Value);
}
private long getpgrpNode_AndSpecialize(NFIPosixSupport arg0Value) {
int state_2 = this.state_2_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'getpgrp(NFIPosixSupport, InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_2 = state_2 | 0b10000000 /* add SpecializationActive[NFIPosixSupport.getpgrp(NFIPosixSupport, InvokeNativeFunction)] */;
this.state_2_ = state_2;
return arg0Value.getpgrp(invoke_);
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#getsid(NFIPosixSupport, long, InvokeNativeFunction)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public long getsid(Object arg0Value_, long arg1Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_2 = this.state_2_;
if ((state_2 & 0b100000000) != 0 /* is SpecializationActive[NFIPosixSupport.getsid(NFIPosixSupport, long, InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
return arg0Value.getsid(arg1Value, invoke_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return getsidNode_AndSpecialize(arg0Value, arg1Value);
}
private long getsidNode_AndSpecialize(NFIPosixSupport arg0Value, long arg1Value) throws PosixException {
int state_2 = this.state_2_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'getsid(NFIPosixSupport, long, InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_2 = state_2 | 0b100000000 /* add SpecializationActive[NFIPosixSupport.getsid(NFIPosixSupport, long, InvokeNativeFunction)] */;
this.state_2_ = state_2;
return arg0Value.getsid(arg1Value, invoke_);
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#setsid(NFIPosixSupport, InvokeNativeFunction)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public long setsid(Object arg0Value_) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_2 = this.state_2_;
if ((state_2 & 0b1000000000) != 0 /* is SpecializationActive[NFIPosixSupport.setsid(NFIPosixSupport, InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
return arg0Value.setsid(invoke_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return setsidNode_AndSpecialize(arg0Value);
}
private long setsidNode_AndSpecialize(NFIPosixSupport arg0Value) throws PosixException {
int state_2 = this.state_2_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'setsid(NFIPosixSupport, InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_2 = state_2 | 0b1000000000 /* add SpecializationActive[NFIPosixSupport.setsid(NFIPosixSupport, InvokeNativeFunction)] */;
this.state_2_ = state_2;
return arg0Value.setsid(invoke_);
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#openpty(NFIPosixSupport, InvokeNativeFunction)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public OpenPtyResult openpty(Object arg0Value_) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_2 = this.state_2_;
if ((state_2 & 0b10000000000) != 0 /* is SpecializationActive[NFIPosixSupport.openpty(NFIPosixSupport, InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
return arg0Value.openpty(invoke_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return openptyNode_AndSpecialize(arg0Value);
}
private OpenPtyResult openptyNode_AndSpecialize(NFIPosixSupport arg0Value) throws PosixException {
int state_2 = this.state_2_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'openpty(NFIPosixSupport, InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_2 = state_2 | 0b10000000000 /* add SpecializationActive[NFIPosixSupport.openpty(NFIPosixSupport, InvokeNativeFunction)] */;
this.state_2_ = state_2;
return arg0Value.openpty(invoke_);
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#ctermid(NFIPosixSupport, InvokeNativeFunction, FromByteArrayNode, SwitchEncodingNode)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public TruffleString ctermid(Object arg0Value_) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_2 = this.state_2_;
if ((state_2 & 0b100000000000) != 0 /* is SpecializationActive[NFIPosixSupport.ctermid(NFIPosixSupport, InvokeNativeFunction, FromByteArrayNode, SwitchEncodingNode)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
FromByteArrayNode tsFromBytes_ = this.tsFromBytes;
if (tsFromBytes_ != null) {
SwitchEncodingNode fromUtf8_ = this.fromUtf8;
if (fromUtf8_ != null) {
return arg0Value.ctermid(invoke_, tsFromBytes_, fromUtf8_);
}
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return ctermidNode_AndSpecialize(arg0Value);
}
private TruffleString ctermidNode_AndSpecialize(NFIPosixSupport arg0Value) throws PosixException {
int state_2 = this.state_2_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'ctermid(NFIPosixSupport, InvokeNativeFunction, FromByteArrayNode, SwitchEncodingNode)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
FromByteArrayNode tsFromBytes_;
FromByteArrayNode tsFromBytes__shared = this.tsFromBytes;
if (tsFromBytes__shared != null) {
tsFromBytes_ = tsFromBytes__shared;
} else {
tsFromBytes_ = this.insert((FromByteArrayNode.create()));
if (tsFromBytes_ == null) {
throw new IllegalStateException("Specialization 'ctermid(NFIPosixSupport, InvokeNativeFunction, FromByteArrayNode, SwitchEncodingNode)' contains a shared cache with name 'fromByteArrayNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.tsFromBytes == null) {
VarHandle.storeStoreFence();
this.tsFromBytes = tsFromBytes_;
}
SwitchEncodingNode fromUtf8_;
SwitchEncodingNode fromUtf8__shared = this.fromUtf8;
if (fromUtf8__shared != null) {
fromUtf8_ = fromUtf8__shared;
} else {
fromUtf8_ = this.insert((SwitchEncodingNode.create()));
if (fromUtf8_ == null) {
throw new IllegalStateException("Specialization 'ctermid(NFIPosixSupport, InvokeNativeFunction, FromByteArrayNode, SwitchEncodingNode)' contains a shared cache with name 'switchEncodingFromUtf8Node' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.fromUtf8 == null) {
VarHandle.storeStoreFence();
this.fromUtf8 = fromUtf8_;
}
state_2 = state_2 | 0b100000000000 /* add SpecializationActive[NFIPosixSupport.ctermid(NFIPosixSupport, InvokeNativeFunction, FromByteArrayNode, SwitchEncodingNode)] */;
this.state_2_ = state_2;
return arg0Value.ctermid(invoke_, tsFromBytes_, fromUtf8_);
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#setenv(NFIPosixSupport, Object, Object, boolean, InvokeNativeFunction)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public void setenv(Object arg0Value_, Object arg1Value, Object arg2Value, boolean arg3Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_2 = this.state_2_;
if ((state_2 & 0b1000000000000) != 0 /* is SpecializationActive[NFIPosixSupport.setenv(NFIPosixSupport, Object, Object, boolean, InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
arg0Value.setenv(arg1Value, arg2Value, arg3Value, invoke_);
return;
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
setenvNode_AndSpecialize(arg0Value, arg1Value, arg2Value, arg3Value);
return;
}
private void setenvNode_AndSpecialize(NFIPosixSupport arg0Value, Object arg1Value, Object arg2Value, boolean arg3Value) throws PosixException {
int state_2 = this.state_2_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'setenv(NFIPosixSupport, Object, Object, boolean, InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_2 = state_2 | 0b1000000000000 /* add SpecializationActive[NFIPosixSupport.setenv(NFIPosixSupport, Object, Object, boolean, InvokeNativeFunction)] */;
this.state_2_ = state_2;
arg0Value.setenv(arg1Value, arg2Value, arg3Value, invoke_);
return;
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#unsetenv(NFIPosixSupport, Object, InvokeNativeFunction)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public void unsetenv(Object arg0Value_, Object arg1Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_2 = this.state_2_;
if ((state_2 & 0b10000000000000) != 0 /* is SpecializationActive[NFIPosixSupport.unsetenv(NFIPosixSupport, Object, InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
arg0Value.unsetenv(arg1Value, invoke_);
return;
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
unsetenvNode_AndSpecialize(arg0Value, arg1Value);
return;
}
private void unsetenvNode_AndSpecialize(NFIPosixSupport arg0Value, Object arg1Value) throws PosixException {
int state_2 = this.state_2_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'unsetenv(NFIPosixSupport, Object, InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_2 = state_2 | 0b10000000000000 /* add SpecializationActive[NFIPosixSupport.unsetenv(NFIPosixSupport, Object, InvokeNativeFunction)] */;
this.state_2_ = state_2;
arg0Value.unsetenv(arg1Value, invoke_);
return;
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#forkExec(NFIPosixSupport, Object[], Object[], Object, Object[], int, int, int, int, int, int, int, int, boolean, boolean, boolean, int[], InvokeNativeFunction)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public int forkExec(Object arg0Value_, Object[] arg1Value, Object[] arg2Value, Object arg3Value, Object[] arg4Value, int arg5Value, int arg6Value, int arg7Value, int arg8Value, int arg9Value, int arg10Value, int arg11Value, int arg12Value, boolean arg13Value, boolean arg14Value, boolean arg15Value, int[] arg16Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_2 = this.state_2_;
if ((state_2 & 0b100000000000000) != 0 /* is SpecializationActive[NFIPosixSupport.forkExec(NFIPosixSupport, Object[], Object[], Object, Object[], int, int, int, int, int, int, int, int, boolean, boolean, boolean, int[], InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
return arg0Value.forkExec(arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, arg6Value, arg7Value, arg8Value, arg9Value, arg10Value, arg11Value, arg12Value, arg13Value, arg14Value, arg15Value, arg16Value, invoke_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return forkExecNode_AndSpecialize(arg0Value, arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, arg6Value, arg7Value, arg8Value, arg9Value, arg10Value, arg11Value, arg12Value, arg13Value, arg14Value, arg15Value, arg16Value);
}
private int forkExecNode_AndSpecialize(NFIPosixSupport arg0Value, Object[] arg1Value, Object[] arg2Value, Object arg3Value, Object[] arg4Value, int arg5Value, int arg6Value, int arg7Value, int arg8Value, int arg9Value, int arg10Value, int arg11Value, int arg12Value, boolean arg13Value, boolean arg14Value, boolean arg15Value, int[] arg16Value) throws PosixException {
int state_2 = this.state_2_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'forkExec(NFIPosixSupport, Object[], Object[], Object, Object[], int, int, int, int, int, int, int, int, boolean, boolean, boolean, int[], InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_2 = state_2 | 0b100000000000000 /* add SpecializationActive[NFIPosixSupport.forkExec(NFIPosixSupport, Object[], Object[], Object, Object[], int, int, int, int, int, int, int, int, boolean, boolean, boolean, int[], InvokeNativeFunction)] */;
this.state_2_ = state_2;
return arg0Value.forkExec(arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, arg6Value, arg7Value, arg8Value, arg9Value, arg10Value, arg11Value, arg12Value, arg13Value, arg14Value, arg15Value, arg16Value, invoke_);
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#execv(NFIPosixSupport, Object, Object[], InvokeNativeFunction)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public void execv(Object arg0Value_, Object arg1Value, Object[] arg2Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_2 = this.state_2_;
if ((state_2 & 0b1000000000000000) != 0 /* is SpecializationActive[NFIPosixSupport.execv(NFIPosixSupport, Object, Object[], InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
arg0Value.execv(arg1Value, arg2Value, invoke_);
return;
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
execvNode_AndSpecialize(arg0Value, arg1Value, arg2Value);
return;
}
private void execvNode_AndSpecialize(NFIPosixSupport arg0Value, Object arg1Value, Object[] arg2Value) throws PosixException {
int state_2 = this.state_2_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'execv(NFIPosixSupport, Object, Object[], InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_2 = state_2 | 0b1000000000000000 /* add SpecializationActive[NFIPosixSupport.execv(NFIPosixSupport, Object, Object[], InvokeNativeFunction)] */;
this.state_2_ = state_2;
arg0Value.execv(arg1Value, arg2Value, invoke_);
return;
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#system(NFIPosixSupport, Object, InvokeNativeFunction)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public int system(Object arg0Value_, Object arg1Value) {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_2 = this.state_2_;
if ((state_2 & 0x10000) != 0 /* is SpecializationActive[NFIPosixSupport.system(NFIPosixSupport, Object, InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
return arg0Value.system(arg1Value, invoke_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return systemNode_AndSpecialize(arg0Value, arg1Value);
}
private int systemNode_AndSpecialize(NFIPosixSupport arg0Value, Object arg1Value) {
int state_2 = this.state_2_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'system(NFIPosixSupport, Object, InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_2 = state_2 | 0x10000 /* add SpecializationActive[NFIPosixSupport.system(NFIPosixSupport, Object, InvokeNativeFunction)] */;
this.state_2_ = state_2;
return arg0Value.system(arg1Value, invoke_);
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#mmap(NFIPosixSupport, long, int, int, int, long, InvokeNativeFunction)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public Object mmap(Object arg0Value_, long arg1Value, int arg2Value, int arg3Value, int arg4Value, long arg5Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_2 = this.state_2_;
if ((state_2 & 0x20000) != 0 /* is SpecializationActive[NFIPosixSupport.mmap(NFIPosixSupport, long, int, int, int, long, InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
return arg0Value.mmap(arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, invoke_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return mmapNode_AndSpecialize(arg0Value, arg1Value, arg2Value, arg3Value, arg4Value, arg5Value);
}
private Object mmapNode_AndSpecialize(NFIPosixSupport arg0Value, long arg1Value, int arg2Value, int arg3Value, int arg4Value, long arg5Value) throws PosixException {
int state_2 = this.state_2_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'mmap(NFIPosixSupport, long, int, int, int, long, InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_2 = state_2 | 0x20000 /* add SpecializationActive[NFIPosixSupport.mmap(NFIPosixSupport, long, int, int, int, long, InvokeNativeFunction)] */;
this.state_2_ = state_2;
return arg0Value.mmap(arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, invoke_);
}
@Override
public byte mmapReadByte(Object receiver, Object mmap, long index) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
return (((NFIPosixSupport) receiver)).mmapReadByte(mmap, index);
}
@Override
public void mmapWriteByte(Object receiver, Object mmap, long index, byte value) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
(((NFIPosixSupport) receiver)).mmapWriteByte(mmap, index, value);
return;
}
@Override
public int mmapReadBytes(Object receiver, Object mmap, long index, byte[] bytes, int length) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
return (((NFIPosixSupport) receiver)).mmapReadBytes(mmap, index, bytes, length);
}
@Override
public void mmapWriteBytes(Object receiver, Object mmap, long index, byte[] bytes, int length) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
(((NFIPosixSupport) receiver)).mmapWriteBytes(mmap, index, bytes, length);
return;
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#mmapFlush(NFIPosixSupport, Object, long, long, InvokeNativeFunction)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public void mmapFlush(Object arg0Value_, Object arg1Value, long arg2Value, long arg3Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_2 = this.state_2_;
if ((state_2 & 0x40000) != 0 /* is SpecializationActive[NFIPosixSupport.mmapFlush(NFIPosixSupport, Object, long, long, InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
arg0Value.mmapFlush(arg1Value, arg2Value, arg3Value, invoke_);
return;
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
mmapFlushNode_AndSpecialize(arg0Value, arg1Value, arg2Value, arg3Value);
return;
}
private void mmapFlushNode_AndSpecialize(NFIPosixSupport arg0Value, Object arg1Value, long arg2Value, long arg3Value) {
int state_2 = this.state_2_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'mmapFlush(NFIPosixSupport, Object, long, long, InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_2 = state_2 | 0x40000 /* add SpecializationActive[NFIPosixSupport.mmapFlush(NFIPosixSupport, Object, long, long, InvokeNativeFunction)] */;
this.state_2_ = state_2;
arg0Value.mmapFlush(arg1Value, arg2Value, arg3Value, invoke_);
return;
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#mmapUnmap(NFIPosixSupport, Object, long, InvokeNativeFunction)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public void mmapUnmap(Object arg0Value_, Object arg1Value, long arg2Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_2 = this.state_2_;
if ((state_2 & 0x80000) != 0 /* is SpecializationActive[NFIPosixSupport.mmapUnmap(NFIPosixSupport, Object, long, InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
arg0Value.mmapUnmap(arg1Value, arg2Value, invoke_);
return;
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
mmapUnmapNode_AndSpecialize(arg0Value, arg1Value, arg2Value);
return;
}
private void mmapUnmapNode_AndSpecialize(NFIPosixSupport arg0Value, Object arg1Value, long arg2Value) throws PosixException {
int state_2 = this.state_2_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'mmapUnmap(NFIPosixSupport, Object, long, InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_2 = state_2 | 0x80000 /* add SpecializationActive[NFIPosixSupport.mmapUnmap(NFIPosixSupport, Object, long, InvokeNativeFunction)] */;
this.state_2_ = state_2;
arg0Value.mmapUnmap(arg1Value, arg2Value, invoke_);
return;
}
@Override
public long mmapGetPointer(Object receiver, Object mmap) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
return (((NFIPosixSupport) receiver)).mmapGetPointer(mmap);
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#socket(NFIPosixSupport, int, int, int, InvokeNativeFunction)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public int socket(Object arg0Value_, int arg1Value, int arg2Value, int arg3Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_2 = this.state_2_;
if ((state_2 & 0x100000) != 0 /* is SpecializationActive[NFIPosixSupport.socket(NFIPosixSupport, int, int, int, InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
return arg0Value.socket(arg1Value, arg2Value, arg3Value, invoke_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return socketNode_AndSpecialize(arg0Value, arg1Value, arg2Value, arg3Value);
}
private int socketNode_AndSpecialize(NFIPosixSupport arg0Value, int arg1Value, int arg2Value, int arg3Value) throws PosixException {
int state_2 = this.state_2_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'socket(NFIPosixSupport, int, int, int, InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_2 = state_2 | 0x100000 /* add SpecializationActive[NFIPosixSupport.socket(NFIPosixSupport, int, int, int, InvokeNativeFunction)] */;
this.state_2_ = state_2;
return arg0Value.socket(arg1Value, arg2Value, arg3Value, invoke_);
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#accept(NFIPosixSupport, int, InvokeNativeFunction)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public AcceptResult accept(Object arg0Value_, int arg1Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_2 = this.state_2_;
if ((state_2 & 0x200000) != 0 /* is SpecializationActive[NFIPosixSupport.accept(NFIPosixSupport, int, InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
return arg0Value.accept(arg1Value, invoke_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return acceptNode_AndSpecialize(arg0Value, arg1Value);
}
private AcceptResult acceptNode_AndSpecialize(NFIPosixSupport arg0Value, int arg1Value) throws PosixException {
int state_2 = this.state_2_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'accept(NFIPosixSupport, int, InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_2 = state_2 | 0x200000 /* add SpecializationActive[NFIPosixSupport.accept(NFIPosixSupport, int, InvokeNativeFunction)] */;
this.state_2_ = state_2;
return arg0Value.accept(arg1Value, invoke_);
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#bind(NFIPosixSupport, int, UniversalSockAddr, InvokeNativeFunction)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public void bind(Object arg0Value_, int arg1Value, UniversalSockAddr arg2Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_2 = this.state_2_;
if ((state_2 & 0x400000) != 0 /* is SpecializationActive[NFIPosixSupport.bind(NFIPosixSupport, int, UniversalSockAddr, InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
arg0Value.bind(arg1Value, arg2Value, invoke_);
return;
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
bindNode_AndSpecialize(arg0Value, arg1Value, arg2Value);
return;
}
private void bindNode_AndSpecialize(NFIPosixSupport arg0Value, int arg1Value, UniversalSockAddr arg2Value) throws PosixException {
int state_2 = this.state_2_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'bind(NFIPosixSupport, int, UniversalSockAddr, InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_2 = state_2 | 0x400000 /* add SpecializationActive[NFIPosixSupport.bind(NFIPosixSupport, int, UniversalSockAddr, InvokeNativeFunction)] */;
this.state_2_ = state_2;
arg0Value.bind(arg1Value, arg2Value, invoke_);
return;
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#connect(NFIPosixSupport, int, UniversalSockAddr, InvokeNativeFunction)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public void connect(Object arg0Value_, int arg1Value, UniversalSockAddr arg2Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_2 = this.state_2_;
if ((state_2 & 0x800000) != 0 /* is SpecializationActive[NFIPosixSupport.connect(NFIPosixSupport, int, UniversalSockAddr, InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
arg0Value.connect(arg1Value, arg2Value, invoke_);
return;
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
connectNode_AndSpecialize(arg0Value, arg1Value, arg2Value);
return;
}
private void connectNode_AndSpecialize(NFIPosixSupport arg0Value, int arg1Value, UniversalSockAddr arg2Value) throws PosixException {
int state_2 = this.state_2_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'connect(NFIPosixSupport, int, UniversalSockAddr, InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_2 = state_2 | 0x800000 /* add SpecializationActive[NFIPosixSupport.connect(NFIPosixSupport, int, UniversalSockAddr, InvokeNativeFunction)] */;
this.state_2_ = state_2;
arg0Value.connect(arg1Value, arg2Value, invoke_);
return;
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#listen(NFIPosixSupport, int, int, InvokeNativeFunction)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public void listen(Object arg0Value_, int arg1Value, int arg2Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_2 = this.state_2_;
if ((state_2 & 0x1000000) != 0 /* is SpecializationActive[NFIPosixSupport.listen(NFIPosixSupport, int, int, InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
arg0Value.listen(arg1Value, arg2Value, invoke_);
return;
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
listenNode_AndSpecialize(arg0Value, arg1Value, arg2Value);
return;
}
private void listenNode_AndSpecialize(NFIPosixSupport arg0Value, int arg1Value, int arg2Value) throws PosixException {
int state_2 = this.state_2_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'listen(NFIPosixSupport, int, int, InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_2 = state_2 | 0x1000000 /* add SpecializationActive[NFIPosixSupport.listen(NFIPosixSupport, int, int, InvokeNativeFunction)] */;
this.state_2_ = state_2;
arg0Value.listen(arg1Value, arg2Value, invoke_);
return;
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#getpeername(NFIPosixSupport, int, InvokeNativeFunction)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public UniversalSockAddr getpeername(Object arg0Value_, int arg1Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_2 = this.state_2_;
if ((state_2 & 0x2000000) != 0 /* is SpecializationActive[NFIPosixSupport.getpeername(NFIPosixSupport, int, InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
return arg0Value.getpeername(arg1Value, invoke_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return getpeernameNode_AndSpecialize(arg0Value, arg1Value);
}
private UniversalSockAddr getpeernameNode_AndSpecialize(NFIPosixSupport arg0Value, int arg1Value) throws PosixException {
int state_2 = this.state_2_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'getpeername(NFIPosixSupport, int, InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_2 = state_2 | 0x2000000 /* add SpecializationActive[NFIPosixSupport.getpeername(NFIPosixSupport, int, InvokeNativeFunction)] */;
this.state_2_ = state_2;
return arg0Value.getpeername(arg1Value, invoke_);
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#getsockname(NFIPosixSupport, int, InvokeNativeFunction)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public UniversalSockAddr getsockname(Object arg0Value_, int arg1Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_2 = this.state_2_;
if ((state_2 & 0x4000000) != 0 /* is SpecializationActive[NFIPosixSupport.getsockname(NFIPosixSupport, int, InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
return arg0Value.getsockname(arg1Value, invoke_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return getsocknameNode_AndSpecialize(arg0Value, arg1Value);
}
private UniversalSockAddr getsocknameNode_AndSpecialize(NFIPosixSupport arg0Value, int arg1Value) throws PosixException {
int state_2 = this.state_2_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'getsockname(NFIPosixSupport, int, InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_2 = state_2 | 0x4000000 /* add SpecializationActive[NFIPosixSupport.getsockname(NFIPosixSupport, int, InvokeNativeFunction)] */;
this.state_2_ = state_2;
return arg0Value.getsockname(arg1Value, invoke_);
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#send(NFIPosixSupport, int, byte[], int, int, int, InvokeNativeFunction)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public int send(Object arg0Value_, int arg1Value, byte[] arg2Value, int arg3Value, int arg4Value, int arg5Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_2 = this.state_2_;
if ((state_2 & 0x8000000) != 0 /* is SpecializationActive[NFIPosixSupport.send(NFIPosixSupport, int, byte[], int, int, int, InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
return arg0Value.send(arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, invoke_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return sendNode_AndSpecialize(arg0Value, arg1Value, arg2Value, arg3Value, arg4Value, arg5Value);
}
private int sendNode_AndSpecialize(NFIPosixSupport arg0Value, int arg1Value, byte[] arg2Value, int arg3Value, int arg4Value, int arg5Value) throws PosixException {
int state_2 = this.state_2_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'send(NFIPosixSupport, int, byte[], int, int, int, InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_2 = state_2 | 0x8000000 /* add SpecializationActive[NFIPosixSupport.send(NFIPosixSupport, int, byte[], int, int, int, InvokeNativeFunction)] */;
this.state_2_ = state_2;
return arg0Value.send(arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, invoke_);
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#sendto(NFIPosixSupport, int, byte[], int, int, int, UniversalSockAddr, InvokeNativeFunction)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public int sendto(Object arg0Value_, int arg1Value, byte[] arg2Value, int arg3Value, int arg4Value, int arg5Value, UniversalSockAddr arg6Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_2 = this.state_2_;
if ((state_2 & 0x10000000) != 0 /* is SpecializationActive[NFIPosixSupport.sendto(NFIPosixSupport, int, byte[], int, int, int, UniversalSockAddr, InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
return arg0Value.sendto(arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, arg6Value, invoke_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return sendtoNode_AndSpecialize(arg0Value, arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, arg6Value);
}
private int sendtoNode_AndSpecialize(NFIPosixSupport arg0Value, int arg1Value, byte[] arg2Value, int arg3Value, int arg4Value, int arg5Value, UniversalSockAddr arg6Value) throws PosixException {
int state_2 = this.state_2_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'sendto(NFIPosixSupport, int, byte[], int, int, int, UniversalSockAddr, InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_2 = state_2 | 0x10000000 /* add SpecializationActive[NFIPosixSupport.sendto(NFIPosixSupport, int, byte[], int, int, int, UniversalSockAddr, InvokeNativeFunction)] */;
this.state_2_ = state_2;
return arg0Value.sendto(arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, arg6Value, invoke_);
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#recv(NFIPosixSupport, int, byte[], int, int, int, InvokeNativeFunction)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public int recv(Object arg0Value_, int arg1Value, byte[] arg2Value, int arg3Value, int arg4Value, int arg5Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_2 = this.state_2_;
if ((state_2 & 0x20000000) != 0 /* is SpecializationActive[NFIPosixSupport.recv(NFIPosixSupport, int, byte[], int, int, int, InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
return arg0Value.recv(arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, invoke_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return recvNode_AndSpecialize(arg0Value, arg1Value, arg2Value, arg3Value, arg4Value, arg5Value);
}
private int recvNode_AndSpecialize(NFIPosixSupport arg0Value, int arg1Value, byte[] arg2Value, int arg3Value, int arg4Value, int arg5Value) throws PosixException {
int state_2 = this.state_2_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'recv(NFIPosixSupport, int, byte[], int, int, int, InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_2 = state_2 | 0x20000000 /* add SpecializationActive[NFIPosixSupport.recv(NFIPosixSupport, int, byte[], int, int, int, InvokeNativeFunction)] */;
this.state_2_ = state_2;
return arg0Value.recv(arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, invoke_);
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#recvfrom(NFIPosixSupport, int, byte[], int, int, int, InvokeNativeFunction)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public RecvfromResult recvfrom(Object arg0Value_, int arg1Value, byte[] arg2Value, int arg3Value, int arg4Value, int arg5Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_2 = this.state_2_;
if ((state_2 & 0x40000000) != 0 /* is SpecializationActive[NFIPosixSupport.recvfrom(NFIPosixSupport, int, byte[], int, int, int, InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
return arg0Value.recvfrom(arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, invoke_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return recvfromNode_AndSpecialize(arg0Value, arg1Value, arg2Value, arg3Value, arg4Value, arg5Value);
}
private RecvfromResult recvfromNode_AndSpecialize(NFIPosixSupport arg0Value, int arg1Value, byte[] arg2Value, int arg3Value, int arg4Value, int arg5Value) throws PosixException {
int state_2 = this.state_2_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'recvfrom(NFIPosixSupport, int, byte[], int, int, int, InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_2 = state_2 | 0x40000000 /* add SpecializationActive[NFIPosixSupport.recvfrom(NFIPosixSupport, int, byte[], int, int, int, InvokeNativeFunction)] */;
this.state_2_ = state_2;
return arg0Value.recvfrom(arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, invoke_);
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#shutdown(NFIPosixSupport, int, int, InvokeNativeFunction)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public void shutdown(Object arg0Value_, int arg1Value, int arg2Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_2 = this.state_2_;
if ((state_2 & 0x80000000) != 0 /* is SpecializationActive[NFIPosixSupport.shutdown(NFIPosixSupport, int, int, InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
arg0Value.shutdown(arg1Value, arg2Value, invoke_);
return;
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
shutdownNode_AndSpecialize(arg0Value, arg1Value, arg2Value);
return;
}
private void shutdownNode_AndSpecialize(NFIPosixSupport arg0Value, int arg1Value, int arg2Value) throws PosixException {
int state_2 = this.state_2_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'shutdown(NFIPosixSupport, int, int, InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_2 = state_2 | 0x80000000 /* add SpecializationActive[NFIPosixSupport.shutdown(NFIPosixSupport, int, int, InvokeNativeFunction)] */;
this.state_2_ = state_2;
arg0Value.shutdown(arg1Value, arg2Value, invoke_);
return;
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#getsockopt(NFIPosixSupport, int, int, int, byte[], int, InvokeNativeFunction)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public int getsockopt(Object arg0Value_, int arg1Value, int arg2Value, int arg3Value, byte[] arg4Value, int arg5Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_3 = this.state_3_;
if ((state_3 & 0b1) != 0 /* is SpecializationActive[NFIPosixSupport.getsockopt(NFIPosixSupport, int, int, int, byte[], int, InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
return arg0Value.getsockopt(arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, invoke_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return getsockoptNode_AndSpecialize(arg0Value, arg1Value, arg2Value, arg3Value, arg4Value, arg5Value);
}
private int getsockoptNode_AndSpecialize(NFIPosixSupport arg0Value, int arg1Value, int arg2Value, int arg3Value, byte[] arg4Value, int arg5Value) throws PosixException {
int state_3 = this.state_3_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'getsockopt(NFIPosixSupport, int, int, int, byte[], int, InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_3 = state_3 | 0b1 /* add SpecializationActive[NFIPosixSupport.getsockopt(NFIPosixSupport, int, int, int, byte[], int, InvokeNativeFunction)] */;
this.state_3_ = state_3;
return arg0Value.getsockopt(arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, invoke_);
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#setsockopt(NFIPosixSupport, int, int, int, byte[], int, InvokeNativeFunction)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public void setsockopt(Object arg0Value_, int arg1Value, int arg2Value, int arg3Value, byte[] arg4Value, int arg5Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_3 = this.state_3_;
if ((state_3 & 0b10) != 0 /* is SpecializationActive[NFIPosixSupport.setsockopt(NFIPosixSupport, int, int, int, byte[], int, InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
arg0Value.setsockopt(arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, invoke_);
return;
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
setsockoptNode_AndSpecialize(arg0Value, arg1Value, arg2Value, arg3Value, arg4Value, arg5Value);
return;
}
private void setsockoptNode_AndSpecialize(NFIPosixSupport arg0Value, int arg1Value, int arg2Value, int arg3Value, byte[] arg4Value, int arg5Value) throws PosixException {
int state_3 = this.state_3_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'setsockopt(NFIPosixSupport, int, int, int, byte[], int, InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_3 = state_3 | 0b10 /* add SpecializationActive[NFIPosixSupport.setsockopt(NFIPosixSupport, int, int, int, byte[], int, InvokeNativeFunction)] */;
this.state_3_ = state_3;
arg0Value.setsockopt(arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, invoke_);
return;
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#inet_addr(NFIPosixSupport, Object, InvokeNativeFunction)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public int inet_addr(Object arg0Value_, Object arg1Value) {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_3 = this.state_3_;
if ((state_3 & 0b100) != 0 /* is SpecializationActive[NFIPosixSupport.inet_addr(NFIPosixSupport, Object, InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
return arg0Value.inet_addr(arg1Value, invoke_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return inet_addrNode_AndSpecialize(arg0Value, arg1Value);
}
private int inet_addrNode_AndSpecialize(NFIPosixSupport arg0Value, Object arg1Value) {
int state_3 = this.state_3_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'inet_addr(NFIPosixSupport, Object, InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_3 = state_3 | 0b100 /* add SpecializationActive[NFIPosixSupport.inet_addr(NFIPosixSupport, Object, InvokeNativeFunction)] */;
this.state_3_ = state_3;
return arg0Value.inet_addr(arg1Value, invoke_);
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#inet_aton(NFIPosixSupport, Object, InvokeNativeFunction)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public int inet_aton(Object arg0Value_, Object arg1Value) throws InvalidAddressException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_3 = this.state_3_;
if ((state_3 & 0b1000) != 0 /* is SpecializationActive[NFIPosixSupport.inet_aton(NFIPosixSupport, Object, InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
return arg0Value.inet_aton(arg1Value, invoke_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return inet_atonNode_AndSpecialize(arg0Value, arg1Value);
}
private int inet_atonNode_AndSpecialize(NFIPosixSupport arg0Value, Object arg1Value) throws InvalidAddressException {
int state_3 = this.state_3_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'inet_aton(NFIPosixSupport, Object, InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_3 = state_3 | 0b1000 /* add SpecializationActive[NFIPosixSupport.inet_aton(NFIPosixSupport, Object, InvokeNativeFunction)] */;
this.state_3_ = state_3;
return arg0Value.inet_aton(arg1Value, invoke_);
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#inet_ntoa(NFIPosixSupport, int, InvokeNativeFunction)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public Object inet_ntoa(Object arg0Value_, int arg1Value) {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_3 = this.state_3_;
if ((state_3 & 0b10000) != 0 /* is SpecializationActive[NFIPosixSupport.inet_ntoa(NFIPosixSupport, int, InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
return arg0Value.inet_ntoa(arg1Value, invoke_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return inet_ntoaNode_AndSpecialize(arg0Value, arg1Value);
}
private Object inet_ntoaNode_AndSpecialize(NFIPosixSupport arg0Value, int arg1Value) {
int state_3 = this.state_3_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'inet_ntoa(NFIPosixSupport, int, InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_3 = state_3 | 0b10000 /* add SpecializationActive[NFIPosixSupport.inet_ntoa(NFIPosixSupport, int, InvokeNativeFunction)] */;
this.state_3_ = state_3;
return arg0Value.inet_ntoa(arg1Value, invoke_);
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#inet_pton(NFIPosixSupport, int, Object, InvokeNativeFunction)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public byte[] inet_pton(Object arg0Value_, int arg1Value, Object arg2Value) throws PosixException, InvalidAddressException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_3 = this.state_3_;
if ((state_3 & 0b100000) != 0 /* is SpecializationActive[NFIPosixSupport.inet_pton(NFIPosixSupport, int, Object, InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
return arg0Value.inet_pton(arg1Value, arg2Value, invoke_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return inet_ptonNode_AndSpecialize(arg0Value, arg1Value, arg2Value);
}
private byte[] inet_ptonNode_AndSpecialize(NFIPosixSupport arg0Value, int arg1Value, Object arg2Value) throws PosixException, InvalidAddressException {
int state_3 = this.state_3_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'inet_pton(NFIPosixSupport, int, Object, InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_3 = state_3 | 0b100000 /* add SpecializationActive[NFIPosixSupport.inet_pton(NFIPosixSupport, int, Object, InvokeNativeFunction)] */;
this.state_3_ = state_3;
return arg0Value.inet_pton(arg1Value, arg2Value, invoke_);
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#inet_ntop(NFIPosixSupport, int, byte[], InvokeNativeFunction)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public Object inet_ntop(Object arg0Value_, int arg1Value, byte[] arg2Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_3 = this.state_3_;
if ((state_3 & 0b1000000) != 0 /* is SpecializationActive[NFIPosixSupport.inet_ntop(NFIPosixSupport, int, byte[], InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
return arg0Value.inet_ntop(arg1Value, arg2Value, invoke_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return inet_ntopNode_AndSpecialize(arg0Value, arg1Value, arg2Value);
}
private Object inet_ntopNode_AndSpecialize(NFIPosixSupport arg0Value, int arg1Value, byte[] arg2Value) throws PosixException {
int state_3 = this.state_3_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'inet_ntop(NFIPosixSupport, int, byte[], InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_3 = state_3 | 0b1000000 /* add SpecializationActive[NFIPosixSupport.inet_ntop(NFIPosixSupport, int, byte[], InvokeNativeFunction)] */;
this.state_3_ = state_3;
return arg0Value.inet_ntop(arg1Value, arg2Value, invoke_);
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#gethostname(NFIPosixSupport, InvokeNativeFunction)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public Object gethostname(Object arg0Value_) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_3 = this.state_3_;
if ((state_3 & 0b10000000) != 0 /* is SpecializationActive[NFIPosixSupport.gethostname(NFIPosixSupport, InvokeNativeFunction)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
return arg0Value.gethostname(invoke_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return gethostnameNode_AndSpecialize(arg0Value);
}
private Object gethostnameNode_AndSpecialize(NFIPosixSupport arg0Value) throws PosixException {
int state_3 = this.state_3_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'gethostname(NFIPosixSupport, InvokeNativeFunction)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
state_3 = state_3 | 0b10000000 /* add SpecializationActive[NFIPosixSupport.gethostname(NFIPosixSupport, InvokeNativeFunction)] */;
this.state_3_ = state_3;
return arg0Value.gethostname(invoke_);
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#getnameinfo(NFIPosixSupport, UniversalSockAddr, int, InvokeNativeFunction, FromByteArrayNode, SwitchEncodingNode)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public Object[] getnameinfo(Object arg0Value_, UniversalSockAddr arg1Value, int arg2Value) throws GetAddrInfoException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_3 = this.state_3_;
if ((state_3 & 0b100000000) != 0 /* is SpecializationActive[NFIPosixSupport.getnameinfo(NFIPosixSupport, UniversalSockAddr, int, InvokeNativeFunction, FromByteArrayNode, SwitchEncodingNode)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
FromByteArrayNode tsFromBytes_ = this.tsFromBytes;
if (tsFromBytes_ != null) {
SwitchEncodingNode fromUtf8_ = this.fromUtf8;
if (fromUtf8_ != null) {
return arg0Value.getnameinfo(arg1Value, arg2Value, invoke_, tsFromBytes_, fromUtf8_);
}
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return getnameinfoNode_AndSpecialize(arg0Value, arg1Value, arg2Value);
}
private Object[] getnameinfoNode_AndSpecialize(NFIPosixSupport arg0Value, UniversalSockAddr arg1Value, int arg2Value) throws GetAddrInfoException {
int state_3 = this.state_3_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'getnameinfo(NFIPosixSupport, UniversalSockAddr, int, InvokeNativeFunction, FromByteArrayNode, SwitchEncodingNode)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
FromByteArrayNode tsFromBytes_;
FromByteArrayNode tsFromBytes__shared = this.tsFromBytes;
if (tsFromBytes__shared != null) {
tsFromBytes_ = tsFromBytes__shared;
} else {
tsFromBytes_ = this.insert((FromByteArrayNode.create()));
if (tsFromBytes_ == null) {
throw new IllegalStateException("Specialization 'getnameinfo(NFIPosixSupport, UniversalSockAddr, int, InvokeNativeFunction, FromByteArrayNode, SwitchEncodingNode)' contains a shared cache with name 'fromByteArrayNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.tsFromBytes == null) {
VarHandle.storeStoreFence();
this.tsFromBytes = tsFromBytes_;
}
SwitchEncodingNode fromUtf8_;
SwitchEncodingNode fromUtf8__shared = this.fromUtf8;
if (fromUtf8__shared != null) {
fromUtf8_ = fromUtf8__shared;
} else {
fromUtf8_ = this.insert((SwitchEncodingNode.create()));
if (fromUtf8_ == null) {
throw new IllegalStateException("Specialization 'getnameinfo(NFIPosixSupport, UniversalSockAddr, int, InvokeNativeFunction, FromByteArrayNode, SwitchEncodingNode)' contains a shared cache with name 'switchEncodingFromUtf8Node' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.fromUtf8 == null) {
VarHandle.storeStoreFence();
this.fromUtf8 = fromUtf8_;
}
state_3 = state_3 | 0b100000000 /* add SpecializationActive[NFIPosixSupport.getnameinfo(NFIPosixSupport, UniversalSockAddr, int, InvokeNativeFunction, FromByteArrayNode, SwitchEncodingNode)] */;
this.state_3_ = state_3;
return arg0Value.getnameinfo(arg1Value, arg2Value, invoke_, tsFromBytes_, fromUtf8_);
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#getaddrinfo(NFIPosixSupport, Object, Object, int, int, int, int, InvokeNativeFunction, FromByteArrayNode, SwitchEncodingNode)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public AddrInfoCursor getaddrinfo(Object arg0Value_, Object arg1Value, Object arg2Value, int arg3Value, int arg4Value, int arg5Value, int arg6Value) throws GetAddrInfoException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_3 = this.state_3_;
if ((state_3 & 0b1000000000) != 0 /* is SpecializationActive[NFIPosixSupport.getaddrinfo(NFIPosixSupport, Object, Object, int, int, int, int, InvokeNativeFunction, FromByteArrayNode, SwitchEncodingNode)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
FromByteArrayNode tsFromBytes_ = this.tsFromBytes;
if (tsFromBytes_ != null) {
SwitchEncodingNode fromUtf8_ = this.fromUtf8;
if (fromUtf8_ != null) {
return arg0Value.getaddrinfo(arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, arg6Value, invoke_, tsFromBytes_, fromUtf8_);
}
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return getaddrinfoNode_AndSpecialize(arg0Value, arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, arg6Value);
}
private AddrInfoCursor getaddrinfoNode_AndSpecialize(NFIPosixSupport arg0Value, Object arg1Value, Object arg2Value, int arg3Value, int arg4Value, int arg5Value, int arg6Value) throws GetAddrInfoException {
int state_3 = this.state_3_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'getaddrinfo(NFIPosixSupport, Object, Object, int, int, int, int, InvokeNativeFunction, FromByteArrayNode, SwitchEncodingNode)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
FromByteArrayNode tsFromBytes_;
FromByteArrayNode tsFromBytes__shared = this.tsFromBytes;
if (tsFromBytes__shared != null) {
tsFromBytes_ = tsFromBytes__shared;
} else {
tsFromBytes_ = this.insert((FromByteArrayNode.create()));
if (tsFromBytes_ == null) {
throw new IllegalStateException("Specialization 'getaddrinfo(NFIPosixSupport, Object, Object, int, int, int, int, InvokeNativeFunction, FromByteArrayNode, SwitchEncodingNode)' contains a shared cache with name 'fromByteArrayNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.tsFromBytes == null) {
VarHandle.storeStoreFence();
this.tsFromBytes = tsFromBytes_;
}
SwitchEncodingNode fromUtf8_;
SwitchEncodingNode fromUtf8__shared = this.fromUtf8;
if (fromUtf8__shared != null) {
fromUtf8_ = fromUtf8__shared;
} else {
fromUtf8_ = this.insert((SwitchEncodingNode.create()));
if (fromUtf8_ == null) {
throw new IllegalStateException("Specialization 'getaddrinfo(NFIPosixSupport, Object, Object, int, int, int, int, InvokeNativeFunction, FromByteArrayNode, SwitchEncodingNode)' contains a shared cache with name 'switchEncodingFromUtf8Node' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.fromUtf8 == null) {
VarHandle.storeStoreFence();
this.fromUtf8 = fromUtf8_;
}
state_3 = state_3 | 0b1000000000 /* add SpecializationActive[NFIPosixSupport.getaddrinfo(NFIPosixSupport, Object, Object, int, int, int, int, InvokeNativeFunction, FromByteArrayNode, SwitchEncodingNode)] */;
this.state_3_ = state_3;
return arg0Value.getaddrinfo(arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, arg6Value, invoke_, tsFromBytes_, fromUtf8_);
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#crypt(NFIPosixSupport, TruffleString, TruffleString, InvokeNativeFunction, SwitchEncodingNode, CopyToByteArrayNode, FromByteArrayNode, SwitchEncodingNode)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public TruffleString crypt(Object arg0Value_, TruffleString arg1Value, TruffleString arg2Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_3 = this.state_3_;
if ((state_3 & 0b10000000000) != 0 /* is SpecializationActive[NFIPosixSupport.crypt(NFIPosixSupport, TruffleString, TruffleString, InvokeNativeFunction, SwitchEncodingNode, CopyToByteArrayNode, FromByteArrayNode, SwitchEncodingNode)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
SwitchEncodingNode toUtf8_ = this.toUtf8;
if (toUtf8_ != null) {
CopyToByteArrayNode tsCopyBytes_ = this.tsCopyBytes;
if (tsCopyBytes_ != null) {
FromByteArrayNode tsFromBytes_ = this.tsFromBytes;
if (tsFromBytes_ != null) {
SwitchEncodingNode fromUtf8_ = this.fromUtf8;
if (fromUtf8_ != null) {
return arg0Value.crypt(arg1Value, arg2Value, invoke_, toUtf8_, tsCopyBytes_, tsFromBytes_, fromUtf8_);
}
}
}
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return cryptNode_AndSpecialize(arg0Value, arg1Value, arg2Value);
}
private TruffleString cryptNode_AndSpecialize(NFIPosixSupport arg0Value, TruffleString arg1Value, TruffleString arg2Value) throws PosixException {
int state_3 = this.state_3_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'crypt(NFIPosixSupport, TruffleString, TruffleString, InvokeNativeFunction, SwitchEncodingNode, CopyToByteArrayNode, FromByteArrayNode, SwitchEncodingNode)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
SwitchEncodingNode toUtf8_;
SwitchEncodingNode toUtf8__shared = this.toUtf8;
if (toUtf8__shared != null) {
toUtf8_ = toUtf8__shared;
} else {
toUtf8_ = this.insert((SwitchEncodingNode.create()));
if (toUtf8_ == null) {
throw new IllegalStateException("Specialization 'crypt(NFIPosixSupport, TruffleString, TruffleString, InvokeNativeFunction, SwitchEncodingNode, CopyToByteArrayNode, FromByteArrayNode, SwitchEncodingNode)' contains a shared cache with name 'switchEncodingToUtf8Node' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.toUtf8 == null) {
VarHandle.storeStoreFence();
this.toUtf8 = toUtf8_;
}
CopyToByteArrayNode tsCopyBytes_;
CopyToByteArrayNode tsCopyBytes__shared = this.tsCopyBytes;
if (tsCopyBytes__shared != null) {
tsCopyBytes_ = tsCopyBytes__shared;
} else {
tsCopyBytes_ = this.insert((CopyToByteArrayNode.create()));
if (tsCopyBytes_ == null) {
throw new IllegalStateException("Specialization 'crypt(NFIPosixSupport, TruffleString, TruffleString, InvokeNativeFunction, SwitchEncodingNode, CopyToByteArrayNode, FromByteArrayNode, SwitchEncodingNode)' contains a shared cache with name 'copyToByteArrayNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.tsCopyBytes == null) {
VarHandle.storeStoreFence();
this.tsCopyBytes = tsCopyBytes_;
}
FromByteArrayNode tsFromBytes_;
FromByteArrayNode tsFromBytes__shared = this.tsFromBytes;
if (tsFromBytes__shared != null) {
tsFromBytes_ = tsFromBytes__shared;
} else {
tsFromBytes_ = this.insert((FromByteArrayNode.create()));
if (tsFromBytes_ == null) {
throw new IllegalStateException("Specialization 'crypt(NFIPosixSupport, TruffleString, TruffleString, InvokeNativeFunction, SwitchEncodingNode, CopyToByteArrayNode, FromByteArrayNode, SwitchEncodingNode)' contains a shared cache with name 'fromByteArrayNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.tsFromBytes == null) {
VarHandle.storeStoreFence();
this.tsFromBytes = tsFromBytes_;
}
SwitchEncodingNode fromUtf8_;
SwitchEncodingNode fromUtf8__shared = this.fromUtf8;
if (fromUtf8__shared != null) {
fromUtf8_ = fromUtf8__shared;
} else {
fromUtf8_ = this.insert((SwitchEncodingNode.create()));
if (fromUtf8_ == null) {
throw new IllegalStateException("Specialization 'crypt(NFIPosixSupport, TruffleString, TruffleString, InvokeNativeFunction, SwitchEncodingNode, CopyToByteArrayNode, FromByteArrayNode, SwitchEncodingNode)' contains a shared cache with name 'switchEncodingFromUtf8Node' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.fromUtf8 == null) {
VarHandle.storeStoreFence();
this.fromUtf8 = fromUtf8_;
}
state_3 = state_3 | 0b10000000000 /* add SpecializationActive[NFIPosixSupport.crypt(NFIPosixSupport, TruffleString, TruffleString, InvokeNativeFunction, SwitchEncodingNode, CopyToByteArrayNode, FromByteArrayNode, SwitchEncodingNode)] */;
this.state_3_ = state_3;
return arg0Value.crypt(arg1Value, arg2Value, invoke_, toUtf8_, tsCopyBytes_, tsFromBytes_, fromUtf8_);
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#getpwuid(NFIPosixSupport, long, InvokeNativeFunction, FromByteArrayNode, SwitchEncodingNode)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public PwdResult getpwuid(Object arg0Value_, long arg1Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_3 = this.state_3_;
if ((state_3 & 0b100000000000) != 0 /* is SpecializationActive[NFIPosixSupport.getpwuid(NFIPosixSupport, long, InvokeNativeFunction, FromByteArrayNode, SwitchEncodingNode)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
FromByteArrayNode tsFromBytes_ = this.tsFromBytes;
if (tsFromBytes_ != null) {
SwitchEncodingNode fromUtf8_ = this.fromUtf8;
if (fromUtf8_ != null) {
return arg0Value.getpwuid(arg1Value, invoke_, tsFromBytes_, fromUtf8_);
}
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return getpwuidNode_AndSpecialize(arg0Value, arg1Value);
}
private PwdResult getpwuidNode_AndSpecialize(NFIPosixSupport arg0Value, long arg1Value) throws PosixException {
int state_3 = this.state_3_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'getpwuid(NFIPosixSupport, long, InvokeNativeFunction, FromByteArrayNode, SwitchEncodingNode)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
FromByteArrayNode tsFromBytes_;
FromByteArrayNode tsFromBytes__shared = this.tsFromBytes;
if (tsFromBytes__shared != null) {
tsFromBytes_ = tsFromBytes__shared;
} else {
tsFromBytes_ = this.insert((FromByteArrayNode.create()));
if (tsFromBytes_ == null) {
throw new IllegalStateException("Specialization 'getpwuid(NFIPosixSupport, long, InvokeNativeFunction, FromByteArrayNode, SwitchEncodingNode)' contains a shared cache with name 'fromByteArrayNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.tsFromBytes == null) {
VarHandle.storeStoreFence();
this.tsFromBytes = tsFromBytes_;
}
SwitchEncodingNode fromUtf8_;
SwitchEncodingNode fromUtf8__shared = this.fromUtf8;
if (fromUtf8__shared != null) {
fromUtf8_ = fromUtf8__shared;
} else {
fromUtf8_ = this.insert((SwitchEncodingNode.create()));
if (fromUtf8_ == null) {
throw new IllegalStateException("Specialization 'getpwuid(NFIPosixSupport, long, InvokeNativeFunction, FromByteArrayNode, SwitchEncodingNode)' contains a shared cache with name 'switchEncodingFromUtf8Node' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.fromUtf8 == null) {
VarHandle.storeStoreFence();
this.fromUtf8 = fromUtf8_;
}
state_3 = state_3 | 0b100000000000 /* add SpecializationActive[NFIPosixSupport.getpwuid(NFIPosixSupport, long, InvokeNativeFunction, FromByteArrayNode, SwitchEncodingNode)] */;
this.state_3_ = state_3;
return arg0Value.getpwuid(arg1Value, invoke_, tsFromBytes_, fromUtf8_);
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#getpwnam(NFIPosixSupport, Object, InvokeNativeFunction, FromByteArrayNode, SwitchEncodingNode)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public PwdResult getpwnam(Object arg0Value_, Object arg1Value) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_3 = this.state_3_;
if ((state_3 & 0b1000000000000) != 0 /* is SpecializationActive[NFIPosixSupport.getpwnam(NFIPosixSupport, Object, InvokeNativeFunction, FromByteArrayNode, SwitchEncodingNode)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
FromByteArrayNode tsFromBytes_ = this.tsFromBytes;
if (tsFromBytes_ != null) {
SwitchEncodingNode fromUtf8_ = this.fromUtf8;
if (fromUtf8_ != null) {
return arg0Value.getpwnam(arg1Value, invoke_, tsFromBytes_, fromUtf8_);
}
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return getpwnamNode_AndSpecialize(arg0Value, arg1Value);
}
private PwdResult getpwnamNode_AndSpecialize(NFIPosixSupport arg0Value, Object arg1Value) throws PosixException {
int state_3 = this.state_3_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'getpwnam(NFIPosixSupport, Object, InvokeNativeFunction, FromByteArrayNode, SwitchEncodingNode)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
FromByteArrayNode tsFromBytes_;
FromByteArrayNode tsFromBytes__shared = this.tsFromBytes;
if (tsFromBytes__shared != null) {
tsFromBytes_ = tsFromBytes__shared;
} else {
tsFromBytes_ = this.insert((FromByteArrayNode.create()));
if (tsFromBytes_ == null) {
throw new IllegalStateException("Specialization 'getpwnam(NFIPosixSupport, Object, InvokeNativeFunction, FromByteArrayNode, SwitchEncodingNode)' contains a shared cache with name 'fromByteArrayNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.tsFromBytes == null) {
VarHandle.storeStoreFence();
this.tsFromBytes = tsFromBytes_;
}
SwitchEncodingNode fromUtf8_;
SwitchEncodingNode fromUtf8__shared = this.fromUtf8;
if (fromUtf8__shared != null) {
fromUtf8_ = fromUtf8__shared;
} else {
fromUtf8_ = this.insert((SwitchEncodingNode.create()));
if (fromUtf8_ == null) {
throw new IllegalStateException("Specialization 'getpwnam(NFIPosixSupport, Object, InvokeNativeFunction, FromByteArrayNode, SwitchEncodingNode)' contains a shared cache with name 'switchEncodingFromUtf8Node' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.fromUtf8 == null) {
VarHandle.storeStoreFence();
this.fromUtf8 = fromUtf8_;
}
state_3 = state_3 | 0b1000000000000 /* add SpecializationActive[NFIPosixSupport.getpwnam(NFIPosixSupport, Object, InvokeNativeFunction, FromByteArrayNode, SwitchEncodingNode)] */;
this.state_3_ = state_3;
return arg0Value.getpwnam(arg1Value, invoke_, tsFromBytes_, fromUtf8_);
}
@Override
public boolean hasGetpwentries(Object receiver) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
return (((NFIPosixSupport) receiver)).hasGetpwentries();
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#getpwentries(NFIPosixSupport, InvokeNativeFunction, FromByteArrayNode, SwitchEncodingNode)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public PwdResult[] getpwentries(Object arg0Value_) throws PosixException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_3 = this.state_3_;
if ((state_3 & 0b10000000000000) != 0 /* is SpecializationActive[NFIPosixSupport.getpwentries(NFIPosixSupport, InvokeNativeFunction, FromByteArrayNode, SwitchEncodingNode)] */) {
{
InvokeNativeFunction invoke_ = this.invoke;
if (invoke_ != null) {
FromByteArrayNode tsFromBytes_ = this.tsFromBytes;
if (tsFromBytes_ != null) {
SwitchEncodingNode fromUtf8_ = this.fromUtf8;
if (fromUtf8_ != null) {
return arg0Value.getpwentries(invoke_, tsFromBytes_, fromUtf8_);
}
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return getpwentriesNode_AndSpecialize(arg0Value);
}
private PwdResult[] getpwentriesNode_AndSpecialize(NFIPosixSupport arg0Value) throws PosixException {
int state_3 = this.state_3_;
InvokeNativeFunction invoke_;
InvokeNativeFunction invoke__shared = this.invoke;
if (invoke__shared != null) {
invoke_ = invoke__shared;
} else {
invoke_ = this.insert((InvokeNativeFunction.create()));
if (invoke_ == null) {
throw new IllegalStateException("Specialization 'getpwentries(NFIPosixSupport, InvokeNativeFunction, FromByteArrayNode, SwitchEncodingNode)' contains a shared cache with name 'invokeNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.invoke == null) {
VarHandle.storeStoreFence();
this.invoke = invoke_;
}
FromByteArrayNode tsFromBytes_;
FromByteArrayNode tsFromBytes__shared = this.tsFromBytes;
if (tsFromBytes__shared != null) {
tsFromBytes_ = tsFromBytes__shared;
} else {
tsFromBytes_ = this.insert((FromByteArrayNode.create()));
if (tsFromBytes_ == null) {
throw new IllegalStateException("Specialization 'getpwentries(NFIPosixSupport, InvokeNativeFunction, FromByteArrayNode, SwitchEncodingNode)' contains a shared cache with name 'fromByteArrayNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.tsFromBytes == null) {
VarHandle.storeStoreFence();
this.tsFromBytes = tsFromBytes_;
}
SwitchEncodingNode fromUtf8_;
SwitchEncodingNode fromUtf8__shared = this.fromUtf8;
if (fromUtf8__shared != null) {
fromUtf8_ = fromUtf8__shared;
} else {
fromUtf8_ = this.insert((SwitchEncodingNode.create()));
if (fromUtf8_ == null) {
throw new IllegalStateException("Specialization 'getpwentries(NFIPosixSupport, InvokeNativeFunction, FromByteArrayNode, SwitchEncodingNode)' contains a shared cache with name 'switchEncodingFromUtf8Node' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.fromUtf8 == null) {
VarHandle.storeStoreFence();
this.fromUtf8 = fromUtf8_;
}
state_3 = state_3 | 0b10000000000000 /* add SpecializationActive[NFIPosixSupport.getpwentries(NFIPosixSupport, InvokeNativeFunction, FromByteArrayNode, SwitchEncodingNode)] */;
this.state_3_ = state_3;
return arg0Value.getpwentries(invoke_, tsFromBytes_, fromUtf8_);
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#createPathFromString(NFIPosixSupport, TruffleString, SwitchEncodingNode, CopyToByteArrayNode)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public Object createPathFromString(Object arg0Value_, TruffleString arg1Value) {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_3 = this.state_3_;
if ((state_3 & 0b100000000000000) != 0 /* is SpecializationActive[NFIPosixSupport.createPathFromString(NFIPosixSupport, TruffleString, SwitchEncodingNode, CopyToByteArrayNode)] */) {
{
SwitchEncodingNode toUtf8_ = this.toUtf8;
if (toUtf8_ != null) {
CopyToByteArrayNode tsCopyBytes_ = this.tsCopyBytes;
if (tsCopyBytes_ != null) {
return arg0Value.createPathFromString(arg1Value, toUtf8_, tsCopyBytes_);
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return createPathFromStringNode_AndSpecialize(arg0Value, arg1Value);
}
private Object createPathFromStringNode_AndSpecialize(NFIPosixSupport arg0Value, TruffleString arg1Value) {
int state_3 = this.state_3_;
SwitchEncodingNode toUtf8_;
SwitchEncodingNode toUtf8__shared = this.toUtf8;
if (toUtf8__shared != null) {
toUtf8_ = toUtf8__shared;
} else {
toUtf8_ = this.insert((SwitchEncodingNode.create()));
if (toUtf8_ == null) {
throw new IllegalStateException("Specialization 'createPathFromString(NFIPosixSupport, TruffleString, SwitchEncodingNode, CopyToByteArrayNode)' contains a shared cache with name 'switchEncodingNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.toUtf8 == null) {
VarHandle.storeStoreFence();
this.toUtf8 = toUtf8_;
}
CopyToByteArrayNode tsCopyBytes_;
CopyToByteArrayNode tsCopyBytes__shared = this.tsCopyBytes;
if (tsCopyBytes__shared != null) {
tsCopyBytes_ = tsCopyBytes__shared;
} else {
tsCopyBytes_ = this.insert((CopyToByteArrayNode.create()));
if (tsCopyBytes_ == null) {
throw new IllegalStateException("Specialization 'createPathFromString(NFIPosixSupport, TruffleString, SwitchEncodingNode, CopyToByteArrayNode)' contains a shared cache with name 'copyToByteArrayNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.tsCopyBytes == null) {
VarHandle.storeStoreFence();
this.tsCopyBytes = tsCopyBytes_;
}
state_3 = state_3 | 0b100000000000000 /* add SpecializationActive[NFIPosixSupport.createPathFromString(NFIPosixSupport, TruffleString, SwitchEncodingNode, CopyToByteArrayNode)] */;
this.state_3_ = state_3;
return arg0Value.createPathFromString(arg1Value, toUtf8_, tsCopyBytes_);
}
@Override
public Object createPathFromBytes(Object receiver, byte[] path) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
return (((NFIPosixSupport) receiver)).createPathFromBytes(path);
}
/**
* Debug Info:
* Specialization {@link NFIPosixSupport#getPathAsString(NFIPosixSupport, Object, FromByteArrayNode, SwitchEncodingNode)}
* Activation probability: 0.00917
* With/without class size: 4/0 bytes
*
*/
@Override
public TruffleString getPathAsString(Object arg0Value_, Object arg1Value) {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
int state_3 = this.state_3_;
if ((state_3 & 0b1000000000000000) != 0 /* is SpecializationActive[NFIPosixSupport.getPathAsString(NFIPosixSupport, Object, FromByteArrayNode, SwitchEncodingNode)] */) {
{
FromByteArrayNode tsFromBytes_ = this.tsFromBytes;
if (tsFromBytes_ != null) {
SwitchEncodingNode fromUtf8_ = this.fromUtf8;
if (fromUtf8_ != null) {
return arg0Value.getPathAsString(arg1Value, tsFromBytes_, fromUtf8_);
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return getPathAsStringNode_AndSpecialize(arg0Value, arg1Value);
}
private TruffleString getPathAsStringNode_AndSpecialize(NFIPosixSupport arg0Value, Object arg1Value) {
int state_3 = this.state_3_;
FromByteArrayNode tsFromBytes_;
FromByteArrayNode tsFromBytes__shared = this.tsFromBytes;
if (tsFromBytes__shared != null) {
tsFromBytes_ = tsFromBytes__shared;
} else {
tsFromBytes_ = this.insert((FromByteArrayNode.create()));
if (tsFromBytes_ == null) {
throw new IllegalStateException("Specialization 'getPathAsString(NFIPosixSupport, Object, FromByteArrayNode, SwitchEncodingNode)' contains a shared cache with name 'fromByteArrayNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.tsFromBytes == null) {
VarHandle.storeStoreFence();
this.tsFromBytes = tsFromBytes_;
}
SwitchEncodingNode fromUtf8_;
SwitchEncodingNode fromUtf8__shared = this.fromUtf8;
if (fromUtf8__shared != null) {
fromUtf8_ = fromUtf8__shared;
} else {
fromUtf8_ = this.insert((SwitchEncodingNode.create()));
if (fromUtf8_ == null) {
throw new IllegalStateException("Specialization 'getPathAsString(NFIPosixSupport, Object, FromByteArrayNode, SwitchEncodingNode)' contains a shared cache with name 'switchEncodingNode' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.fromUtf8 == null) {
VarHandle.storeStoreFence();
this.fromUtf8 = fromUtf8_;
}
state_3 = state_3 | 0b1000000000000000 /* add SpecializationActive[NFIPosixSupport.getPathAsString(NFIPosixSupport, Object, FromByteArrayNode, SwitchEncodingNode)] */;
this.state_3_ = state_3;
return arg0Value.getPathAsString(arg1Value, tsFromBytes_, fromUtf8_);
}
@Override
public Buffer getPathAsBytes(Object receiver, Object path) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
return (((NFIPosixSupport) receiver)).getPathAsBytes(path);
}
}
@GeneratedBy(NFIPosixSupport.class)
@DenyReplace
private static final class Uncached extends PosixSupportLibrary {
protected Uncached() {
}
@Override
@TruffleBoundary
public boolean accepts(Object receiver) {
assert !(receiver instanceof NFIPosixSupport) || DYNAMIC_DISPATCH_LIBRARY_.getUncached().dispatch(receiver) == null : "Invalid library export. Exported receiver with dynamic dispatch found but not expected.";
return receiver instanceof NFIPosixSupport;
}
@Override
public boolean isAdoptable() {
return false;
}
@Override
public NodeCost getCost() {
return NodeCost.MEGAMORPHIC;
}
@TruffleBoundary
@Override
public Object dirEntryGetPath(Object arg0Value_, Object arg1Value, Object arg2Value) {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
if (arg1Value instanceof DirEntry) {
DirEntry arg1Value_ = (DirEntry) arg1Value;
if ((DirEntryGetPath.endsWithSlash(arg2Value))) {
return DirEntryGetPath.withSlash(arg0Value, arg1Value_, arg2Value);
}
if ((!(DirEntryGetPath.endsWithSlash(arg2Value)))) {
return DirEntryGetPath.withoutSlash(arg0Value, arg1Value_, arg2Value);
}
}
throw new UnsupportedSpecializationException(this, new Node[] {null, null, null}, arg0Value, arg1Value, arg2Value);
}
@TruffleBoundary
@Override
public UniversalSockAddr createUniversalSockAddr(Object arg0Value_, FamilySpecificSockAddr arg1Value) {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
if (arg1Value instanceof Inet4SockAddr) {
Inet4SockAddr arg1Value_ = (Inet4SockAddr) arg1Value;
return CreateUniversalSockAddr.inet4(arg0Value, arg1Value_, (InvokeNativeFunction.getUncached()));
}
if (arg1Value instanceof Inet6SockAddr) {
Inet6SockAddr arg1Value_ = (Inet6SockAddr) arg1Value;
return CreateUniversalSockAddr.inet6(arg0Value, arg1Value_, (InvokeNativeFunction.getUncached()));
}
if (arg1Value instanceof UnixSockAddr) {
UnixSockAddr arg1Value_ = (UnixSockAddr) arg1Value;
return CreateUniversalSockAddr.unix(arg0Value, arg1Value_, (InvokeNativeFunction.getUncached()));
}
throw new UnsupportedSpecializationException(this, new Node[] {null, null}, arg0Value, arg1Value);
}
@TruffleBoundary
@Override
public TruffleString getBackend(Object receiver) {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((NFIPosixSupport) receiver) .getBackend();
}
@TruffleBoundary
@Override
public TruffleString strerror(Object arg0Value_, int arg1Value) {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
return arg0Value.strerror(arg1Value, (InvokeNativeFunction.getUncached()), (FromByteArrayNode.getUncached()), (SwitchEncodingNode.getUncached()));
}
@TruffleBoundary
@Override
public long getpid(Object arg0Value_) {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
return arg0Value.getpid((InvokeNativeFunction.getUncached()));
}
@TruffleBoundary
@Override
public int umask(Object arg0Value_, int arg1Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
return arg0Value.umask(arg1Value, (InvokeNativeFunction.getUncached()));
}
@TruffleBoundary
@Override
public int openat(Object arg0Value_, int arg1Value, Object arg2Value, int arg3Value, int arg4Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
return arg0Value.openat(arg1Value, arg2Value, arg3Value, arg4Value, (InvokeNativeFunction.getUncached()));
}
@TruffleBoundary
@Override
public int close(Object arg0Value_, int arg1Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
return arg0Value.close(arg1Value, (InvokeNativeFunction.getUncached()));
}
@TruffleBoundary
@Override
public Buffer read(Object arg0Value_, int arg1Value, long arg2Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
return arg0Value.read(arg1Value, arg2Value, (InvokeNativeFunction.getUncached()));
}
@TruffleBoundary
@Override
public long write(Object arg0Value_, int arg1Value, Buffer arg2Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
return arg0Value.write(arg1Value, arg2Value, (InvokeNativeFunction.getUncached()));
}
@TruffleBoundary
@Override
public int dup(Object arg0Value_, int arg1Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
return arg0Value.dup(arg1Value, (InvokeNativeFunction.getUncached()));
}
@TruffleBoundary
@Override
public int dup2(Object arg0Value_, int arg1Value, int arg2Value, boolean arg3Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
return arg0Value.dup2(arg1Value, arg2Value, arg3Value, (InvokeNativeFunction.getUncached()));
}
@TruffleBoundary
@Override
public boolean getInheritable(Object arg0Value_, int arg1Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
return arg0Value.getInheritable(arg1Value, (InvokeNativeFunction.getUncached()));
}
@TruffleBoundary
@Override
public void setInheritable(Object arg0Value_, int arg1Value, boolean arg2Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
arg0Value.setInheritable(arg1Value, arg2Value, (InvokeNativeFunction.getUncached()));
return;
}
@TruffleBoundary
@Override
public int[] pipe(Object arg0Value_) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
return arg0Value.pipe((InvokeNativeFunction.getUncached()));
}
@TruffleBoundary
@Override
public SelectResult select(Object arg0Value_, int[] arg1Value, int[] arg2Value, int[] arg3Value, Timeval arg4Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
return arg0Value.select(arg1Value, arg2Value, arg3Value, arg4Value, (InvokeNativeFunction.getUncached()));
}
@TruffleBoundary
@Override
public long lseek(Object arg0Value_, int arg1Value, long arg2Value, int arg3Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
return arg0Value.lseek(arg1Value, arg2Value, arg3Value, (InvokeNativeFunction.getUncached()));
}
@TruffleBoundary
@Override
public void ftruncate(Object arg0Value_, int arg1Value, long arg2Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
arg0Value.ftruncate(arg1Value, arg2Value, (InvokeNativeFunction.getUncached()));
return;
}
@TruffleBoundary
@Override
public void fsync(Object arg0Value_, int arg1Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
arg0Value.fsync(arg1Value, (InvokeNativeFunction.getUncached()));
return;
}
@TruffleBoundary
@Override
public void flock(Object arg0Value_, int arg1Value, int arg2Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
arg0Value.flock(arg1Value, arg2Value, (InvokeNativeFunction.getUncached()));
return;
}
@TruffleBoundary
@Override
public boolean getBlocking(Object arg0Value_, int arg1Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
return arg0Value.getBlocking(arg1Value, (InvokeNativeFunction.getUncached()));
}
@TruffleBoundary
@Override
public void setBlocking(Object arg0Value_, int arg1Value, boolean arg2Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
arg0Value.setBlocking(arg1Value, arg2Value, (InvokeNativeFunction.getUncached()));
return;
}
@TruffleBoundary
@Override
public int[] getTerminalSize(Object arg0Value_, int arg1Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
return arg0Value.getTerminalSize(arg1Value, (InvokeNativeFunction.getUncached()));
}
@TruffleBoundary
@Override
public long[] fstatat(Object arg0Value_, int arg1Value, Object arg2Value, boolean arg3Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
return arg0Value.fstatat(arg1Value, arg2Value, arg3Value, (InvokeNativeFunction.getUncached()));
}
@TruffleBoundary
@Override
public long[] fstat(Object arg0Value_, int arg1Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
return arg0Value.fstat(arg1Value, (InvokeNativeFunction.getUncached()));
}
@TruffleBoundary
@Override
public long[] statvfs(Object arg0Value_, Object arg1Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
return arg0Value.statvfs(arg1Value, (InvokeNativeFunction.getUncached()));
}
@TruffleBoundary
@Override
public long[] fstatvfs(Object arg0Value_, int arg1Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
return arg0Value.fstatvfs(arg1Value, (InvokeNativeFunction.getUncached()));
}
@TruffleBoundary
@Override
public Object[] uname(Object arg0Value_) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
return arg0Value.uname((InvokeNativeFunction.getUncached()), (FromByteArrayNode.getUncached()), (SwitchEncodingNode.getUncached()));
}
@TruffleBoundary
@Override
public void unlinkat(Object arg0Value_, int arg1Value, Object arg2Value, boolean arg3Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
arg0Value.unlinkat(arg1Value, arg2Value, arg3Value, (InvokeNativeFunction.getUncached()));
return;
}
@TruffleBoundary
@Override
public void linkat(Object arg0Value_, int arg1Value, Object arg2Value, int arg3Value, Object arg4Value, int arg5Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
arg0Value.linkat(arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, (InvokeNativeFunction.getUncached()));
return;
}
@TruffleBoundary
@Override
public void symlinkat(Object arg0Value_, Object arg1Value, int arg2Value, Object arg3Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
arg0Value.symlinkat(arg1Value, arg2Value, arg3Value, (InvokeNativeFunction.getUncached()));
return;
}
@TruffleBoundary
@Override
public void mkdirat(Object arg0Value_, int arg1Value, Object arg2Value, int arg3Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
arg0Value.mkdirat(arg1Value, arg2Value, arg3Value, (InvokeNativeFunction.getUncached()));
return;
}
@TruffleBoundary
@Override
public Object getcwd(Object arg0Value_) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
return arg0Value.getcwd((InvokeNativeFunction.getUncached()));
}
@TruffleBoundary
@Override
public void chdir(Object arg0Value_, Object arg1Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
arg0Value.chdir(arg1Value, (InvokeNativeFunction.getUncached()));
return;
}
@TruffleBoundary
@Override
public void fchdir(Object arg0Value_, int arg1Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
arg0Value.fchdir(arg1Value, (InvokeNativeFunction.getUncached()));
return;
}
@TruffleBoundary
@Override
public boolean isatty(Object arg0Value_, int arg1Value) {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
return arg0Value.isatty(arg1Value, (InvokeNativeFunction.getUncached()));
}
@TruffleBoundary
@Override
public Object opendir(Object arg0Value_, Object arg1Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
return arg0Value.opendir(arg1Value, (InvokeNativeFunction.getUncached()));
}
@TruffleBoundary
@Override
public Object fdopendir(Object arg0Value_, int arg1Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
return arg0Value.fdopendir(arg1Value, (InvokeNativeFunction.getUncached()));
}
@TruffleBoundary
@Override
public void closedir(Object arg0Value_, Object arg1Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
arg0Value.closedir(arg1Value, (InvokeNativeFunction.getUncached()));
return;
}
@TruffleBoundary
@Override
public Object readdir(Object arg0Value_, Object arg1Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
return arg0Value.readdir(arg1Value, (InvokeNativeFunction.getUncached()));
}
@TruffleBoundary
@Override
public void rewinddir(Object arg0Value_, Object arg1Value) {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
arg0Value.rewinddir(arg1Value, (InvokeNativeFunction.getUncached()));
return;
}
@TruffleBoundary
@Override
public Object dirEntryGetName(Object receiver, Object dirEntry) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((NFIPosixSupport) receiver) .dirEntryGetName(dirEntry);
}
@TruffleBoundary
@Override
public long dirEntryGetInode(Object receiver, Object dirEntry) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((NFIPosixSupport) receiver) .dirEntryGetInode(dirEntry);
}
@TruffleBoundary
@Override
public int dirEntryGetType(Object receiver, Object dirEntry) {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((NFIPosixSupport) receiver) .dirEntryGetType(dirEntry);
}
@TruffleBoundary
@Override
public void utimensat(Object arg0Value_, int arg1Value, Object arg2Value, long[] arg3Value, boolean arg4Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
arg0Value.utimensat(arg1Value, arg2Value, arg3Value, arg4Value, (InvokeNativeFunction.getUncached()));
return;
}
@TruffleBoundary
@Override
public void futimens(Object arg0Value_, int arg1Value, long[] arg2Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
arg0Value.futimens(arg1Value, arg2Value, (InvokeNativeFunction.getUncached()));
return;
}
@TruffleBoundary
@Override
public void futimes(Object arg0Value_, int arg1Value, Timeval[] arg2Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
arg0Value.futimes(arg1Value, arg2Value, (InvokeNativeFunction.getUncached()));
return;
}
@TruffleBoundary
@Override
public void lutimes(Object arg0Value_, Object arg1Value, Timeval[] arg2Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
arg0Value.lutimes(arg1Value, arg2Value, (InvokeNativeFunction.getUncached()));
return;
}
@TruffleBoundary
@Override
public void utimes(Object arg0Value_, Object arg1Value, Timeval[] arg2Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
arg0Value.utimes(arg1Value, arg2Value, (InvokeNativeFunction.getUncached()));
return;
}
@TruffleBoundary
@Override
public void renameat(Object arg0Value_, int arg1Value, Object arg2Value, int arg3Value, Object arg4Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
arg0Value.renameat(arg1Value, arg2Value, arg3Value, arg4Value, (InvokeNativeFunction.getUncached()));
return;
}
@TruffleBoundary
@Override
public boolean faccessat(Object arg0Value_, int arg1Value, Object arg2Value, int arg3Value, boolean arg4Value, boolean arg5Value) {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
return arg0Value.faccessat(arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, (InvokeNativeFunction.getUncached()));
}
@TruffleBoundary
@Override
public void fchmodat(Object arg0Value_, int arg1Value, Object arg2Value, int arg3Value, boolean arg4Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
arg0Value.fchmodat(arg1Value, arg2Value, arg3Value, arg4Value, (InvokeNativeFunction.getUncached()));
return;
}
@TruffleBoundary
@Override
public void fchmod(Object arg0Value_, int arg1Value, int arg2Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
arg0Value.fchmod(arg1Value, arg2Value, (InvokeNativeFunction.getUncached()));
return;
}
@TruffleBoundary
@Override
public Object readlinkat(Object arg0Value_, int arg1Value, Object arg2Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
return arg0Value.readlinkat(arg1Value, arg2Value, (InvokeNativeFunction.getUncached()));
}
@TruffleBoundary
@Override
public void kill(Object arg0Value_, long arg1Value, int arg2Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
arg0Value.kill(arg1Value, arg2Value, (InvokeNativeFunction.getUncached()));
return;
}
@TruffleBoundary
@Override
public void killpg(Object arg0Value_, long arg1Value, int arg2Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
arg0Value.killpg(arg1Value, arg2Value, (InvokeNativeFunction.getUncached()));
return;
}
@TruffleBoundary
@Override
public long[] waitpid(Object arg0Value_, long arg1Value, int arg2Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
return arg0Value.waitpid(arg1Value, arg2Value, (InvokeNativeFunction.getUncached()));
}
@TruffleBoundary
@Override
public boolean wcoredump(Object arg0Value_, int arg1Value) {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
return arg0Value.wcoredump(arg1Value, (InvokeNativeFunction.getUncached()));
}
@TruffleBoundary
@Override
public void abort(Object arg0Value_) {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
arg0Value.abort((InvokeNativeFunction.getUncached()));
return;
}
@TruffleBoundary
@Override
public boolean wifcontinued(Object arg0Value_, int arg1Value) {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
return arg0Value.wifcontinued(arg1Value, (InvokeNativeFunction.getUncached()));
}
@TruffleBoundary
@Override
public boolean wifstopped(Object arg0Value_, int arg1Value) {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
return arg0Value.wifstopped(arg1Value, (InvokeNativeFunction.getUncached()));
}
@TruffleBoundary
@Override
public boolean wifsignaled(Object arg0Value_, int arg1Value) {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
return arg0Value.wifsignaled(arg1Value, (InvokeNativeFunction.getUncached()));
}
@TruffleBoundary
@Override
public boolean wifexited(Object arg0Value_, int arg1Value) {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
return arg0Value.wifexited(arg1Value, (InvokeNativeFunction.getUncached()));
}
@TruffleBoundary
@Override
public int wexitstatus(Object arg0Value_, int arg1Value) {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
return arg0Value.wexitstatus(arg1Value, (InvokeNativeFunction.getUncached()));
}
@TruffleBoundary
@Override
public int wtermsig(Object arg0Value_, int arg1Value) {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
return arg0Value.wtermsig(arg1Value, (InvokeNativeFunction.getUncached()));
}
@TruffleBoundary
@Override
public int wstopsig(Object arg0Value_, int arg1Value) {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
return arg0Value.wstopsig(arg1Value, (InvokeNativeFunction.getUncached()));
}
@TruffleBoundary
@Override
public long getuid(Object arg0Value_) {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
return arg0Value.getuid((InvokeNativeFunction.getUncached()));
}
@TruffleBoundary
@Override
public long geteuid(Object arg0Value_) {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
return arg0Value.geteuid((InvokeNativeFunction.getUncached()));
}
@TruffleBoundary
@Override
public long getgid(Object arg0Value_) {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
return arg0Value.getgid((InvokeNativeFunction.getUncached()));
}
@TruffleBoundary
@Override
public long getppid(Object arg0Value_) {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
return arg0Value.getppid((InvokeNativeFunction.getUncached()));
}
@TruffleBoundary
@Override
public void setpgid(Object arg0Value_, long arg1Value, long arg2Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
arg0Value.setpgid(arg1Value, arg2Value, (InvokeNativeFunction.getUncached()));
return;
}
@TruffleBoundary
@Override
public long getpgid(Object arg0Value_, long arg1Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
return arg0Value.getpgid(arg1Value, (InvokeNativeFunction.getUncached()));
}
@TruffleBoundary
@Override
public long getpgrp(Object arg0Value_) {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
return arg0Value.getpgrp((InvokeNativeFunction.getUncached()));
}
@TruffleBoundary
@Override
public long getsid(Object arg0Value_, long arg1Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
return arg0Value.getsid(arg1Value, (InvokeNativeFunction.getUncached()));
}
@TruffleBoundary
@Override
public long setsid(Object arg0Value_) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
return arg0Value.setsid((InvokeNativeFunction.getUncached()));
}
@TruffleBoundary
@Override
public OpenPtyResult openpty(Object arg0Value_) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
return arg0Value.openpty((InvokeNativeFunction.getUncached()));
}
@TruffleBoundary
@Override
public TruffleString ctermid(Object arg0Value_) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
return arg0Value.ctermid((InvokeNativeFunction.getUncached()), (FromByteArrayNode.getUncached()), (SwitchEncodingNode.getUncached()));
}
@TruffleBoundary
@Override
public void setenv(Object arg0Value_, Object arg1Value, Object arg2Value, boolean arg3Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
arg0Value.setenv(arg1Value, arg2Value, arg3Value, (InvokeNativeFunction.getUncached()));
return;
}
@TruffleBoundary
@Override
public void unsetenv(Object arg0Value_, Object arg1Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
arg0Value.unsetenv(arg1Value, (InvokeNativeFunction.getUncached()));
return;
}
@TruffleBoundary
@Override
public int forkExec(Object arg0Value_, Object[] arg1Value, Object[] arg2Value, Object arg3Value, Object[] arg4Value, int arg5Value, int arg6Value, int arg7Value, int arg8Value, int arg9Value, int arg10Value, int arg11Value, int arg12Value, boolean arg13Value, boolean arg14Value, boolean arg15Value, int[] arg16Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
return arg0Value.forkExec(arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, arg6Value, arg7Value, arg8Value, arg9Value, arg10Value, arg11Value, arg12Value, arg13Value, arg14Value, arg15Value, arg16Value, (InvokeNativeFunction.getUncached()));
}
@TruffleBoundary
@Override
public void execv(Object arg0Value_, Object arg1Value, Object[] arg2Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
arg0Value.execv(arg1Value, arg2Value, (InvokeNativeFunction.getUncached()));
return;
}
@TruffleBoundary
@Override
public int system(Object arg0Value_, Object arg1Value) {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
return arg0Value.system(arg1Value, (InvokeNativeFunction.getUncached()));
}
@TruffleBoundary
@Override
public Object mmap(Object arg0Value_, long arg1Value, int arg2Value, int arg3Value, int arg4Value, long arg5Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
return arg0Value.mmap(arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, (InvokeNativeFunction.getUncached()));
}
@TruffleBoundary
@Override
public byte mmapReadByte(Object receiver, Object mmap, long index) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((NFIPosixSupport) receiver) .mmapReadByte(mmap, index);
}
@TruffleBoundary
@Override
public void mmapWriteByte(Object receiver, Object mmap, long index, byte value) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
((NFIPosixSupport) receiver) .mmapWriteByte(mmap, index, value);
return;
}
@TruffleBoundary
@Override
public int mmapReadBytes(Object receiver, Object mmap, long index, byte[] bytes, int length) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((NFIPosixSupport) receiver) .mmapReadBytes(mmap, index, bytes, length);
}
@TruffleBoundary
@Override
public void mmapWriteBytes(Object receiver, Object mmap, long index, byte[] bytes, int length) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
((NFIPosixSupport) receiver) .mmapWriteBytes(mmap, index, bytes, length);
return;
}
@TruffleBoundary
@Override
public void mmapFlush(Object arg0Value_, Object arg1Value, long arg2Value, long arg3Value) {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
arg0Value.mmapFlush(arg1Value, arg2Value, arg3Value, (InvokeNativeFunction.getUncached()));
return;
}
@TruffleBoundary
@Override
public void mmapUnmap(Object arg0Value_, Object arg1Value, long arg2Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
arg0Value.mmapUnmap(arg1Value, arg2Value, (InvokeNativeFunction.getUncached()));
return;
}
@TruffleBoundary
@Override
public long mmapGetPointer(Object receiver, Object mmap) {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((NFIPosixSupport) receiver) .mmapGetPointer(mmap);
}
@TruffleBoundary
@Override
public int socket(Object arg0Value_, int arg1Value, int arg2Value, int arg3Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
return arg0Value.socket(arg1Value, arg2Value, arg3Value, (InvokeNativeFunction.getUncached()));
}
@TruffleBoundary
@Override
public AcceptResult accept(Object arg0Value_, int arg1Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
return arg0Value.accept(arg1Value, (InvokeNativeFunction.getUncached()));
}
@TruffleBoundary
@Override
public void bind(Object arg0Value_, int arg1Value, UniversalSockAddr arg2Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
arg0Value.bind(arg1Value, arg2Value, (InvokeNativeFunction.getUncached()));
return;
}
@TruffleBoundary
@Override
public void connect(Object arg0Value_, int arg1Value, UniversalSockAddr arg2Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
arg0Value.connect(arg1Value, arg2Value, (InvokeNativeFunction.getUncached()));
return;
}
@TruffleBoundary
@Override
public void listen(Object arg0Value_, int arg1Value, int arg2Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
arg0Value.listen(arg1Value, arg2Value, (InvokeNativeFunction.getUncached()));
return;
}
@TruffleBoundary
@Override
public UniversalSockAddr getpeername(Object arg0Value_, int arg1Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
return arg0Value.getpeername(arg1Value, (InvokeNativeFunction.getUncached()));
}
@TruffleBoundary
@Override
public UniversalSockAddr getsockname(Object arg0Value_, int arg1Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
return arg0Value.getsockname(arg1Value, (InvokeNativeFunction.getUncached()));
}
@TruffleBoundary
@Override
public int send(Object arg0Value_, int arg1Value, byte[] arg2Value, int arg3Value, int arg4Value, int arg5Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
return arg0Value.send(arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, (InvokeNativeFunction.getUncached()));
}
@TruffleBoundary
@Override
public int sendto(Object arg0Value_, int arg1Value, byte[] arg2Value, int arg3Value, int arg4Value, int arg5Value, UniversalSockAddr arg6Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
return arg0Value.sendto(arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, arg6Value, (InvokeNativeFunction.getUncached()));
}
@TruffleBoundary
@Override
public int recv(Object arg0Value_, int arg1Value, byte[] arg2Value, int arg3Value, int arg4Value, int arg5Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
return arg0Value.recv(arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, (InvokeNativeFunction.getUncached()));
}
@TruffleBoundary
@Override
public RecvfromResult recvfrom(Object arg0Value_, int arg1Value, byte[] arg2Value, int arg3Value, int arg4Value, int arg5Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
return arg0Value.recvfrom(arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, (InvokeNativeFunction.getUncached()));
}
@TruffleBoundary
@Override
public void shutdown(Object arg0Value_, int arg1Value, int arg2Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
arg0Value.shutdown(arg1Value, arg2Value, (InvokeNativeFunction.getUncached()));
return;
}
@TruffleBoundary
@Override
public int getsockopt(Object arg0Value_, int arg1Value, int arg2Value, int arg3Value, byte[] arg4Value, int arg5Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
return arg0Value.getsockopt(arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, (InvokeNativeFunction.getUncached()));
}
@TruffleBoundary
@Override
public void setsockopt(Object arg0Value_, int arg1Value, int arg2Value, int arg3Value, byte[] arg4Value, int arg5Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
arg0Value.setsockopt(arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, (InvokeNativeFunction.getUncached()));
return;
}
@TruffleBoundary
@Override
public int inet_addr(Object arg0Value_, Object arg1Value) {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
return arg0Value.inet_addr(arg1Value, (InvokeNativeFunction.getUncached()));
}
@TruffleBoundary
@Override
public int inet_aton(Object arg0Value_, Object arg1Value) throws InvalidAddressException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
return arg0Value.inet_aton(arg1Value, (InvokeNativeFunction.getUncached()));
}
@TruffleBoundary
@Override
public Object inet_ntoa(Object arg0Value_, int arg1Value) {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
return arg0Value.inet_ntoa(arg1Value, (InvokeNativeFunction.getUncached()));
}
@TruffleBoundary
@Override
public byte[] inet_pton(Object arg0Value_, int arg1Value, Object arg2Value) throws PosixException, InvalidAddressException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
return arg0Value.inet_pton(arg1Value, arg2Value, (InvokeNativeFunction.getUncached()));
}
@TruffleBoundary
@Override
public Object inet_ntop(Object arg0Value_, int arg1Value, byte[] arg2Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
return arg0Value.inet_ntop(arg1Value, arg2Value, (InvokeNativeFunction.getUncached()));
}
@TruffleBoundary
@Override
public Object gethostname(Object arg0Value_) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
return arg0Value.gethostname((InvokeNativeFunction.getUncached()));
}
@TruffleBoundary
@Override
public Object[] getnameinfo(Object arg0Value_, UniversalSockAddr arg1Value, int arg2Value) throws GetAddrInfoException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
return arg0Value.getnameinfo(arg1Value, arg2Value, (InvokeNativeFunction.getUncached()), (FromByteArrayNode.getUncached()), (SwitchEncodingNode.getUncached()));
}
@TruffleBoundary
@Override
public AddrInfoCursor getaddrinfo(Object arg0Value_, Object arg1Value, Object arg2Value, int arg3Value, int arg4Value, int arg5Value, int arg6Value) throws GetAddrInfoException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
return arg0Value.getaddrinfo(arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, arg6Value, (InvokeNativeFunction.getUncached()), (FromByteArrayNode.getUncached()), (SwitchEncodingNode.getUncached()));
}
@TruffleBoundary
@Override
public TruffleString crypt(Object arg0Value_, TruffleString arg1Value, TruffleString arg2Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
return arg0Value.crypt(arg1Value, arg2Value, (InvokeNativeFunction.getUncached()), (SwitchEncodingNode.getUncached()), (CopyToByteArrayNode.getUncached()), (FromByteArrayNode.getUncached()), (SwitchEncodingNode.getUncached()));
}
@TruffleBoundary
@Override
public PwdResult getpwuid(Object arg0Value_, long arg1Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
return arg0Value.getpwuid(arg1Value, (InvokeNativeFunction.getUncached()), (FromByteArrayNode.getUncached()), (SwitchEncodingNode.getUncached()));
}
@TruffleBoundary
@Override
public PwdResult getpwnam(Object arg0Value_, Object arg1Value) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
return arg0Value.getpwnam(arg1Value, (InvokeNativeFunction.getUncached()), (FromByteArrayNode.getUncached()), (SwitchEncodingNode.getUncached()));
}
@TruffleBoundary
@Override
public boolean hasGetpwentries(Object receiver) {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((NFIPosixSupport) receiver) .hasGetpwentries();
}
@TruffleBoundary
@Override
public PwdResult[] getpwentries(Object arg0Value_) throws PosixException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
return arg0Value.getpwentries((InvokeNativeFunction.getUncached()), (FromByteArrayNode.getUncached()), (SwitchEncodingNode.getUncached()));
}
@TruffleBoundary
@Override
public Object createPathFromString(Object arg0Value_, TruffleString arg1Value) {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
return arg0Value.createPathFromString(arg1Value, (SwitchEncodingNode.getUncached()), (CopyToByteArrayNode.getUncached()));
}
@TruffleBoundary
@Override
public Object createPathFromBytes(Object receiver, byte[] path) {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((NFIPosixSupport) receiver) .createPathFromBytes(path);
}
@TruffleBoundary
@Override
public TruffleString getPathAsString(Object arg0Value_, Object arg1Value) {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
NFIPosixSupport arg0Value = ((NFIPosixSupport) arg0Value_);
return arg0Value.getPathAsString(arg1Value, (FromByteArrayNode.getUncached()), (SwitchEncodingNode.getUncached()));
}
@TruffleBoundary
@Override
public Buffer getPathAsBytes(Object receiver, Object path) {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((NFIPosixSupport) receiver) .getPathAsBytes(path);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy