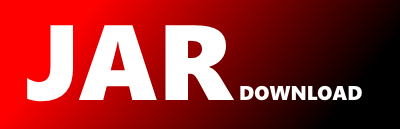
com.oracle.graal.python.runtime.PosixSupportLibraryGen Maven / Gradle / Ivy
// CheckStyle: start generated
package com.oracle.graal.python.runtime;
import com.oracle.graal.python.runtime.PosixSupportLibrary.AcceptResult;
import com.oracle.graal.python.runtime.PosixSupportLibrary.AddrInfoCursor;
import com.oracle.graal.python.runtime.PosixSupportLibrary.Buffer;
import com.oracle.graal.python.runtime.PosixSupportLibrary.FamilySpecificSockAddr;
import com.oracle.graal.python.runtime.PosixSupportLibrary.OpenPtyResult;
import com.oracle.graal.python.runtime.PosixSupportLibrary.PwdResult;
import com.oracle.graal.python.runtime.PosixSupportLibrary.RecvfromResult;
import com.oracle.graal.python.runtime.PosixSupportLibrary.SelectResult;
import com.oracle.graal.python.runtime.PosixSupportLibrary.Timeval;
import com.oracle.graal.python.runtime.PosixSupportLibrary.UniversalSockAddr;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.TruffleBoundary;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.library.DynamicDispatchLibrary;
import com.oracle.truffle.api.library.Library;
import com.oracle.truffle.api.library.LibraryExport;
import com.oracle.truffle.api.library.LibraryFactory;
import com.oracle.truffle.api.library.Message;
import com.oracle.truffle.api.library.ReflectionLibrary;
import com.oracle.truffle.api.nodes.DenyReplace;
import com.oracle.truffle.api.nodes.EncapsulatingNodeReference;
import com.oracle.truffle.api.nodes.ExplodeLoop;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.nodes.NodeCost;
import com.oracle.truffle.api.strings.TruffleString;
import com.oracle.truffle.api.utilities.FinalBitSet;
import java.util.Arrays;
import java.util.BitSet;
import java.util.Collections;
import java.util.concurrent.locks.Lock;
@GeneratedBy(PosixSupportLibrary.class)
final class PosixSupportLibraryGen extends LibraryFactory {
private static final Class LIBRARY_CLASS = PosixSupportLibraryGen.lazyLibraryClass();
private static final Message GET_BACKEND = new MessageImpl("getBackend", 0, false, TruffleString.class, Object.class);
private static final Message STRERROR = new MessageImpl("strerror", 1, false, TruffleString.class, Object.class, int.class);
private static final Message GETPID = new MessageImpl("getpid", 2, false, long.class, Object.class);
private static final Message UMASK = new MessageImpl("umask", 3, false, int.class, Object.class, int.class);
private static final Message OPENAT = new MessageImpl("openat", 4, false, int.class, Object.class, int.class, Object.class, int.class, int.class);
private static final Message CLOSE = new MessageImpl("close", 5, false, int.class, Object.class, int.class);
private static final Message READ = new MessageImpl("read", 6, false, Buffer.class, Object.class, int.class, long.class);
private static final Message WRITE = new MessageImpl("write", 7, false, long.class, Object.class, int.class, Buffer.class);
private static final Message DUP = new MessageImpl("dup", 8, false, int.class, Object.class, int.class);
private static final Message DUP2 = new MessageImpl("dup2", 9, false, int.class, Object.class, int.class, int.class, boolean.class);
private static final Message GET_INHERITABLE = new MessageImpl("getInheritable", 10, false, boolean.class, Object.class, int.class);
private static final Message SET_INHERITABLE = new MessageImpl("setInheritable", 11, false, void.class, Object.class, int.class, boolean.class);
private static final Message PIPE = new MessageImpl("pipe", 12, false, int[].class, Object.class);
private static final Message SELECT = new MessageImpl("select", 13, false, SelectResult.class, Object.class, int[].class, int[].class, int[].class, Timeval.class);
private static final Message LSEEK = new MessageImpl("lseek", 14, false, long.class, Object.class, int.class, long.class, int.class);
private static final Message FTRUNCATE = new MessageImpl("ftruncate", 15, false, void.class, Object.class, int.class, long.class);
private static final Message FSYNC = new MessageImpl("fsync", 16, false, void.class, Object.class, int.class);
private static final Message FLOCK = new MessageImpl("flock", 17, false, void.class, Object.class, int.class, int.class);
private static final Message GET_BLOCKING = new MessageImpl("getBlocking", 18, false, boolean.class, Object.class, int.class);
private static final Message SET_BLOCKING = new MessageImpl("setBlocking", 19, false, void.class, Object.class, int.class, boolean.class);
private static final Message GET_TERMINAL_SIZE = new MessageImpl("getTerminalSize", 20, false, int[].class, Object.class, int.class);
private static final Message FSTATAT = new MessageImpl("fstatat", 21, false, long[].class, Object.class, int.class, Object.class, boolean.class);
private static final Message FSTAT = new MessageImpl("fstat", 22, false, long[].class, Object.class, int.class);
private static final Message STATVFS = new MessageImpl("statvfs", 23, false, long[].class, Object.class, Object.class);
private static final Message FSTATVFS = new MessageImpl("fstatvfs", 24, false, long[].class, Object.class, int.class);
private static final Message UNAME = new MessageImpl("uname", 25, false, Object[].class, Object.class);
private static final Message UNLINKAT = new MessageImpl("unlinkat", 26, false, void.class, Object.class, int.class, Object.class, boolean.class);
private static final Message LINKAT = new MessageImpl("linkat", 27, false, void.class, Object.class, int.class, Object.class, int.class, Object.class, int.class);
private static final Message SYMLINKAT = new MessageImpl("symlinkat", 28, false, void.class, Object.class, Object.class, int.class, Object.class);
private static final Message MKDIRAT = new MessageImpl("mkdirat", 29, false, void.class, Object.class, int.class, Object.class, int.class);
private static final Message GETCWD = new MessageImpl("getcwd", 30, false, Object.class, Object.class);
private static final Message CHDIR = new MessageImpl("chdir", 31, false, void.class, Object.class, Object.class);
private static final Message FCHDIR = new MessageImpl("fchdir", 32, false, void.class, Object.class, int.class);
private static final Message ISATTY = new MessageImpl("isatty", 33, false, boolean.class, Object.class, int.class);
private static final Message OPENDIR = new MessageImpl("opendir", 34, false, Object.class, Object.class, Object.class);
private static final Message FDOPENDIR = new MessageImpl("fdopendir", 35, false, Object.class, Object.class, int.class);
private static final Message CLOSEDIR = new MessageImpl("closedir", 36, false, void.class, Object.class, Object.class);
private static final Message READDIR = new MessageImpl("readdir", 37, false, Object.class, Object.class, Object.class);
private static final Message REWINDDIR = new MessageImpl("rewinddir", 38, false, void.class, Object.class, Object.class);
private static final Message DIR_ENTRY_GET_NAME = new MessageImpl("dirEntryGetName", 39, false, Object.class, Object.class, Object.class);
private static final Message DIR_ENTRY_GET_PATH = new MessageImpl("dirEntryGetPath", 40, false, Object.class, Object.class, Object.class, Object.class);
private static final Message DIR_ENTRY_GET_INODE = new MessageImpl("dirEntryGetInode", 41, false, long.class, Object.class, Object.class);
private static final Message DIR_ENTRY_GET_TYPE = new MessageImpl("dirEntryGetType", 42, false, int.class, Object.class, Object.class);
private static final Message UTIMENSAT = new MessageImpl("utimensat", 43, false, void.class, Object.class, int.class, Object.class, long[].class, boolean.class);
private static final Message FUTIMENS = new MessageImpl("futimens", 44, false, void.class, Object.class, int.class, long[].class);
private static final Message FUTIMES = new MessageImpl("futimes", 45, false, void.class, Object.class, int.class, Timeval[].class);
private static final Message LUTIMES = new MessageImpl("lutimes", 46, false, void.class, Object.class, Object.class, Timeval[].class);
private static final Message UTIMES = new MessageImpl("utimes", 47, false, void.class, Object.class, Object.class, Timeval[].class);
private static final Message RENAMEAT = new MessageImpl("renameat", 48, false, void.class, Object.class, int.class, Object.class, int.class, Object.class);
private static final Message FACCESSAT = new MessageImpl("faccessat", 49, false, boolean.class, Object.class, int.class, Object.class, int.class, boolean.class, boolean.class);
private static final Message FCHMODAT = new MessageImpl("fchmodat", 50, false, void.class, Object.class, int.class, Object.class, int.class, boolean.class);
private static final Message FCHMOD = new MessageImpl("fchmod", 51, false, void.class, Object.class, int.class, int.class);
private static final Message READLINKAT = new MessageImpl("readlinkat", 52, false, Object.class, Object.class, int.class, Object.class);
private static final Message KILL = new MessageImpl("kill", 53, false, void.class, Object.class, long.class, int.class);
private static final Message KILLPG = new MessageImpl("killpg", 54, false, void.class, Object.class, long.class, int.class);
private static final Message WAITPID = new MessageImpl("waitpid", 55, false, long[].class, Object.class, long.class, int.class);
private static final Message ABORT = new MessageImpl("abort", 56, false, void.class, Object.class);
private static final Message WCOREDUMP = new MessageImpl("wcoredump", 57, false, boolean.class, Object.class, int.class);
private static final Message WIFCONTINUED = new MessageImpl("wifcontinued", 58, false, boolean.class, Object.class, int.class);
private static final Message WIFSTOPPED = new MessageImpl("wifstopped", 59, false, boolean.class, Object.class, int.class);
private static final Message WIFSIGNALED = new MessageImpl("wifsignaled", 60, false, boolean.class, Object.class, int.class);
private static final Message WIFEXITED = new MessageImpl("wifexited", 61, false, boolean.class, Object.class, int.class);
private static final Message WEXITSTATUS = new MessageImpl("wexitstatus", 62, false, int.class, Object.class, int.class);
private static final Message WTERMSIG = new MessageImpl("wtermsig", 63, false, int.class, Object.class, int.class);
private static final Message WSTOPSIG = new MessageImpl("wstopsig", 64, false, int.class, Object.class, int.class);
private static final Message GETUID = new MessageImpl("getuid", 65, false, long.class, Object.class);
private static final Message GETEUID = new MessageImpl("geteuid", 66, false, long.class, Object.class);
private static final Message GETGID = new MessageImpl("getgid", 67, false, long.class, Object.class);
private static final Message GETPPID = new MessageImpl("getppid", 68, false, long.class, Object.class);
private static final Message GETPGID = new MessageImpl("getpgid", 69, false, long.class, Object.class, long.class);
private static final Message SETPGID = new MessageImpl("setpgid", 70, false, void.class, Object.class, long.class, long.class);
private static final Message GETPGRP = new MessageImpl("getpgrp", 71, false, long.class, Object.class);
private static final Message GETSID = new MessageImpl("getsid", 72, false, long.class, Object.class, long.class);
private static final Message SETSID = new MessageImpl("setsid", 73, false, long.class, Object.class);
private static final Message OPENPTY = new MessageImpl("openpty", 74, false, OpenPtyResult.class, Object.class);
private static final Message CTERMID = new MessageImpl("ctermid", 75, false, TruffleString.class, Object.class);
private static final Message SETENV = new MessageImpl("setenv", 76, false, void.class, Object.class, Object.class, Object.class, boolean.class);
private static final Message UNSETENV = new MessageImpl("unsetenv", 77, false, void.class, Object.class, Object.class);
private static final Message FORK_EXEC = new MessageImpl("forkExec", 78, false, int.class, Object.class, Object[].class, Object[].class, Object.class, Object[].class, int.class, int.class, int.class, int.class, int.class, int.class, int.class, int.class, boolean.class, boolean.class, boolean.class, int[].class);
private static final Message EXECV = new MessageImpl("execv", 79, false, void.class, Object.class, Object.class, Object[].class);
private static final Message SYSTEM = new MessageImpl("system", 80, false, int.class, Object.class, Object.class);
private static final Message MMAP = new MessageImpl("mmap", 81, false, Object.class, Object.class, long.class, int.class, int.class, int.class, long.class);
private static final Message MMAP_READ_BYTE = new MessageImpl("mmapReadByte", 82, false, byte.class, Object.class, Object.class, long.class);
private static final Message MMAP_WRITE_BYTE = new MessageImpl("mmapWriteByte", 83, false, void.class, Object.class, Object.class, long.class, byte.class);
private static final Message MMAP_READ_BYTES = new MessageImpl("mmapReadBytes", 84, false, int.class, Object.class, Object.class, long.class, byte[].class, int.class);
private static final Message MMAP_WRITE_BYTES = new MessageImpl("mmapWriteBytes", 85, false, void.class, Object.class, Object.class, long.class, byte[].class, int.class);
private static final Message MMAP_FLUSH = new MessageImpl("mmapFlush", 86, false, void.class, Object.class, Object.class, long.class, long.class);
private static final Message MMAP_UNMAP = new MessageImpl("mmapUnmap", 87, false, void.class, Object.class, Object.class, long.class);
private static final Message MMAP_GET_POINTER = new MessageImpl("mmapGetPointer", 88, false, long.class, Object.class, Object.class);
private static final Message GETPWUID = new MessageImpl("getpwuid", 89, false, PwdResult.class, Object.class, long.class);
private static final Message GETPWNAM = new MessageImpl("getpwnam", 90, false, PwdResult.class, Object.class, Object.class);
private static final Message HAS_GETPWENTRIES = new MessageImpl("hasGetpwentries", 91, false, boolean.class, Object.class);
private static final Message GETPWENTRIES = new MessageImpl("getpwentries", 92, false, PwdResult[].class, Object.class);
private static final Message CREATE_PATH_FROM_STRING = new MessageImpl("createPathFromString", 93, false, Object.class, Object.class, TruffleString.class);
private static final Message CREATE_PATH_FROM_BYTES = new MessageImpl("createPathFromBytes", 94, false, Object.class, Object.class, byte[].class);
private static final Message GET_PATH_AS_STRING = new MessageImpl("getPathAsString", 95, false, TruffleString.class, Object.class, Object.class);
private static final Message GET_PATH_AS_BYTES = new MessageImpl("getPathAsBytes", 96, false, Buffer.class, Object.class, Object.class);
private static final Message SOCKET = new MessageImpl("socket", 97, false, int.class, Object.class, int.class, int.class, int.class);
private static final Message ACCEPT = new MessageImpl("accept", 98, false, AcceptResult.class, Object.class, int.class);
private static final Message BIND = new MessageImpl("bind", 99, false, void.class, Object.class, int.class, UniversalSockAddr.class);
private static final Message CONNECT = new MessageImpl("connect", 100, false, void.class, Object.class, int.class, UniversalSockAddr.class);
private static final Message LISTEN = new MessageImpl("listen", 101, false, void.class, Object.class, int.class, int.class);
private static final Message GETPEERNAME = new MessageImpl("getpeername", 102, false, UniversalSockAddr.class, Object.class, int.class);
private static final Message GETSOCKNAME = new MessageImpl("getsockname", 103, false, UniversalSockAddr.class, Object.class, int.class);
private static final Message SEND = new MessageImpl("send", 104, false, int.class, Object.class, int.class, byte[].class, int.class, int.class, int.class);
private static final Message SENDTO = new MessageImpl("sendto", 105, false, int.class, Object.class, int.class, byte[].class, int.class, int.class, int.class, UniversalSockAddr.class);
private static final Message RECV = new MessageImpl("recv", 106, false, int.class, Object.class, int.class, byte[].class, int.class, int.class, int.class);
private static final Message RECVFROM = new MessageImpl("recvfrom", 107, false, RecvfromResult.class, Object.class, int.class, byte[].class, int.class, int.class, int.class);
private static final Message SHUTDOWN = new MessageImpl("shutdown", 108, false, void.class, Object.class, int.class, int.class);
private static final Message GETSOCKOPT = new MessageImpl("getsockopt", 109, false, int.class, Object.class, int.class, int.class, int.class, byte[].class, int.class);
private static final Message SETSOCKOPT = new MessageImpl("setsockopt", 110, false, void.class, Object.class, int.class, int.class, int.class, byte[].class, int.class);
private static final Message INET_ADDR = new MessageImpl("inet_addr", 111, false, int.class, Object.class, Object.class);
private static final Message INET_ATON = new MessageImpl("inet_aton", 112, false, int.class, Object.class, Object.class);
private static final Message INET_NTOA = new MessageImpl("inet_ntoa", 113, false, Object.class, Object.class, int.class);
private static final Message INET_PTON = new MessageImpl("inet_pton", 114, false, byte[].class, Object.class, int.class, Object.class);
private static final Message INET_NTOP = new MessageImpl("inet_ntop", 115, false, Object.class, Object.class, int.class, byte[].class);
private static final Message GETHOSTNAME = new MessageImpl("gethostname", 116, false, Object.class, Object.class);
private static final Message GETNAMEINFO = new MessageImpl("getnameinfo", 117, false, Object[].class, Object.class, UniversalSockAddr.class, int.class);
private static final Message GETADDRINFO = new MessageImpl("getaddrinfo", 118, false, AddrInfoCursor.class, Object.class, Object.class, Object.class, int.class, int.class, int.class, int.class);
private static final Message CRYPT = new MessageImpl("crypt", 119, false, TruffleString.class, Object.class, TruffleString.class, TruffleString.class);
private static final Message CREATE_UNIVERSAL_SOCK_ADDR = new MessageImpl("createUniversalSockAddr", 120, false, UniversalSockAddr.class, Object.class, FamilySpecificSockAddr.class);
private static final PosixSupportLibraryGen INSTANCE = new PosixSupportLibraryGen();
private static final LibraryFactory DYNAMIC_DISPATCH_LIBRARY_ = LibraryFactory.resolve(DynamicDispatchLibrary.class);
static {
LibraryExport.register(PosixSupportLibraryGen.LIBRARY_CLASS, new Default());
LibraryFactory.register(PosixSupportLibraryGen.LIBRARY_CLASS, INSTANCE);
}
private PosixSupportLibraryGen() {
super(PosixSupportLibraryGen.LIBRARY_CLASS, Collections.unmodifiableList(Arrays.asList(PosixSupportLibraryGen.GET_BACKEND, PosixSupportLibraryGen.STRERROR, PosixSupportLibraryGen.GETPID, PosixSupportLibraryGen.UMASK, PosixSupportLibraryGen.OPENAT, PosixSupportLibraryGen.CLOSE, PosixSupportLibraryGen.READ, PosixSupportLibraryGen.WRITE, PosixSupportLibraryGen.DUP, PosixSupportLibraryGen.DUP2, PosixSupportLibraryGen.GET_INHERITABLE, PosixSupportLibraryGen.SET_INHERITABLE, PosixSupportLibraryGen.PIPE, PosixSupportLibraryGen.SELECT, PosixSupportLibraryGen.LSEEK, PosixSupportLibraryGen.FTRUNCATE, PosixSupportLibraryGen.FSYNC, PosixSupportLibraryGen.FLOCK, PosixSupportLibraryGen.GET_BLOCKING, PosixSupportLibraryGen.SET_BLOCKING, PosixSupportLibraryGen.GET_TERMINAL_SIZE, PosixSupportLibraryGen.FSTATAT, PosixSupportLibraryGen.FSTAT, PosixSupportLibraryGen.STATVFS, PosixSupportLibraryGen.FSTATVFS, PosixSupportLibraryGen.UNAME, PosixSupportLibraryGen.UNLINKAT, PosixSupportLibraryGen.LINKAT, PosixSupportLibraryGen.SYMLINKAT, PosixSupportLibraryGen.MKDIRAT, PosixSupportLibraryGen.GETCWD, PosixSupportLibraryGen.CHDIR, PosixSupportLibraryGen.FCHDIR, PosixSupportLibraryGen.ISATTY, PosixSupportLibraryGen.OPENDIR, PosixSupportLibraryGen.FDOPENDIR, PosixSupportLibraryGen.CLOSEDIR, PosixSupportLibraryGen.READDIR, PosixSupportLibraryGen.REWINDDIR, PosixSupportLibraryGen.DIR_ENTRY_GET_NAME, PosixSupportLibraryGen.DIR_ENTRY_GET_PATH, PosixSupportLibraryGen.DIR_ENTRY_GET_INODE, PosixSupportLibraryGen.DIR_ENTRY_GET_TYPE, PosixSupportLibraryGen.UTIMENSAT, PosixSupportLibraryGen.FUTIMENS, PosixSupportLibraryGen.FUTIMES, PosixSupportLibraryGen.LUTIMES, PosixSupportLibraryGen.UTIMES, PosixSupportLibraryGen.RENAMEAT, PosixSupportLibraryGen.FACCESSAT, PosixSupportLibraryGen.FCHMODAT, PosixSupportLibraryGen.FCHMOD, PosixSupportLibraryGen.READLINKAT, PosixSupportLibraryGen.KILL, PosixSupportLibraryGen.KILLPG, PosixSupportLibraryGen.WAITPID, PosixSupportLibraryGen.ABORT, PosixSupportLibraryGen.WCOREDUMP, PosixSupportLibraryGen.WIFCONTINUED, PosixSupportLibraryGen.WIFSTOPPED, PosixSupportLibraryGen.WIFSIGNALED, PosixSupportLibraryGen.WIFEXITED, PosixSupportLibraryGen.WEXITSTATUS, PosixSupportLibraryGen.WTERMSIG, PosixSupportLibraryGen.WSTOPSIG, PosixSupportLibraryGen.GETUID, PosixSupportLibraryGen.GETEUID, PosixSupportLibraryGen.GETGID, PosixSupportLibraryGen.GETPPID, PosixSupportLibraryGen.GETPGID, PosixSupportLibraryGen.SETPGID, PosixSupportLibraryGen.GETPGRP, PosixSupportLibraryGen.GETSID, PosixSupportLibraryGen.SETSID, PosixSupportLibraryGen.OPENPTY, PosixSupportLibraryGen.CTERMID, PosixSupportLibraryGen.SETENV, PosixSupportLibraryGen.UNSETENV, PosixSupportLibraryGen.FORK_EXEC, PosixSupportLibraryGen.EXECV, PosixSupportLibraryGen.SYSTEM, PosixSupportLibraryGen.MMAP, PosixSupportLibraryGen.MMAP_READ_BYTE, PosixSupportLibraryGen.MMAP_WRITE_BYTE, PosixSupportLibraryGen.MMAP_READ_BYTES, PosixSupportLibraryGen.MMAP_WRITE_BYTES, PosixSupportLibraryGen.MMAP_FLUSH, PosixSupportLibraryGen.MMAP_UNMAP, PosixSupportLibraryGen.MMAP_GET_POINTER, PosixSupportLibraryGen.GETPWUID, PosixSupportLibraryGen.GETPWNAM, PosixSupportLibraryGen.HAS_GETPWENTRIES, PosixSupportLibraryGen.GETPWENTRIES, PosixSupportLibraryGen.CREATE_PATH_FROM_STRING, PosixSupportLibraryGen.CREATE_PATH_FROM_BYTES, PosixSupportLibraryGen.GET_PATH_AS_STRING, PosixSupportLibraryGen.GET_PATH_AS_BYTES, PosixSupportLibraryGen.SOCKET, PosixSupportLibraryGen.ACCEPT, PosixSupportLibraryGen.BIND, PosixSupportLibraryGen.CONNECT, PosixSupportLibraryGen.LISTEN, PosixSupportLibraryGen.GETPEERNAME, PosixSupportLibraryGen.GETSOCKNAME, PosixSupportLibraryGen.SEND, PosixSupportLibraryGen.SENDTO, PosixSupportLibraryGen.RECV, PosixSupportLibraryGen.RECVFROM, PosixSupportLibraryGen.SHUTDOWN, PosixSupportLibraryGen.GETSOCKOPT, PosixSupportLibraryGen.SETSOCKOPT, PosixSupportLibraryGen.INET_ADDR, PosixSupportLibraryGen.INET_ATON, PosixSupportLibraryGen.INET_NTOA, PosixSupportLibraryGen.INET_PTON, PosixSupportLibraryGen.INET_NTOP, PosixSupportLibraryGen.GETHOSTNAME, PosixSupportLibraryGen.GETNAMEINFO, PosixSupportLibraryGen.GETADDRINFO, PosixSupportLibraryGen.CRYPT, PosixSupportLibraryGen.CREATE_UNIVERSAL_SOCK_ADDR)));
}
@Override
protected Class> getDefaultClass(Object receiver) {
return PosixSupportLibrary.class;
}
@Override
protected PosixSupportLibrary createProxy(ReflectionLibrary library) {
return new Proxy(library);
}
@Override
protected FinalBitSet createMessageBitSet(Message... messages) {
BitSet bitSet = new BitSet(2);
for (Message message : messages) {
bitSet.set(message.getId());
}
return FinalBitSet.valueOf(bitSet);
}
@Override
protected PosixSupportLibrary createDelegate(PosixSupportLibrary delegateLibrary) {
return new Delegate(delegateLibrary);
}
@Override
protected Object genericDispatch(Library originalLib, Object receiver, Message message, Object[] args, int offset) throws Exception {
PosixSupportLibrary lib = (PosixSupportLibrary) originalLib;
if (message.getParameterCount() - 1 != args.length - offset) {
CompilerDirectives.transferToInterpreterAndInvalidate();
throw new IllegalArgumentException("Invalid number of arguments.");
}
switch (message.getId()) {
case 0 :
return lib.getBackend(receiver);
case 1 :
return lib.strerror(receiver, (int) args[offset]);
case 2 :
return lib.getpid(receiver);
case 3 :
return lib.umask(receiver, (int) args[offset]);
case 4 :
return lib.openat(receiver, (int) args[offset], args[offset + 1], (int) args[offset + 2], (int) args[offset + 3]);
case 5 :
return lib.close(receiver, (int) args[offset]);
case 6 :
return lib.read(receiver, (int) args[offset], (long) args[offset + 1]);
case 7 :
return lib.write(receiver, (int) args[offset], (Buffer) args[offset + 1]);
case 8 :
return lib.dup(receiver, (int) args[offset]);
case 9 :
return lib.dup2(receiver, (int) args[offset], (int) args[offset + 1], (boolean) args[offset + 2]);
case 10 :
return lib.getInheritable(receiver, (int) args[offset]);
case 11 :
lib.setInheritable(receiver, (int) args[offset], (boolean) args[offset + 1]);
return null;
case 12 :
return lib.pipe(receiver);
case 13 :
return lib.select(receiver, (int[]) args[offset], (int[]) args[offset + 1], (int[]) args[offset + 2], (Timeval) args[offset + 3]);
case 14 :
return lib.lseek(receiver, (int) args[offset], (long) args[offset + 1], (int) args[offset + 2]);
case 15 :
lib.ftruncate(receiver, (int) args[offset], (long) args[offset + 1]);
return null;
case 16 :
lib.fsync(receiver, (int) args[offset]);
return null;
case 17 :
lib.flock(receiver, (int) args[offset], (int) args[offset + 1]);
return null;
case 18 :
return lib.getBlocking(receiver, (int) args[offset]);
case 19 :
lib.setBlocking(receiver, (int) args[offset], (boolean) args[offset + 1]);
return null;
case 20 :
return lib.getTerminalSize(receiver, (int) args[offset]);
case 21 :
return lib.fstatat(receiver, (int) args[offset], args[offset + 1], (boolean) args[offset + 2]);
case 22 :
return lib.fstat(receiver, (int) args[offset]);
case 23 :
return lib.statvfs(receiver, args[offset]);
case 24 :
return lib.fstatvfs(receiver, (int) args[offset]);
case 25 :
return lib.uname(receiver);
case 26 :
lib.unlinkat(receiver, (int) args[offset], args[offset + 1], (boolean) args[offset + 2]);
return null;
case 27 :
lib.linkat(receiver, (int) args[offset], args[offset + 1], (int) args[offset + 2], args[offset + 3], (int) args[offset + 4]);
return null;
case 28 :
lib.symlinkat(receiver, args[offset], (int) args[offset + 1], args[offset + 2]);
return null;
case 29 :
lib.mkdirat(receiver, (int) args[offset], args[offset + 1], (int) args[offset + 2]);
return null;
case 30 :
return lib.getcwd(receiver);
case 31 :
lib.chdir(receiver, args[offset]);
return null;
case 32 :
lib.fchdir(receiver, (int) args[offset]);
return null;
case 33 :
return lib.isatty(receiver, (int) args[offset]);
case 34 :
return lib.opendir(receiver, args[offset]);
case 35 :
return lib.fdopendir(receiver, (int) args[offset]);
case 36 :
lib.closedir(receiver, args[offset]);
return null;
case 37 :
return lib.readdir(receiver, args[offset]);
case 38 :
lib.rewinddir(receiver, args[offset]);
return null;
case 39 :
return lib.dirEntryGetName(receiver, args[offset]);
case 40 :
return lib.dirEntryGetPath(receiver, args[offset], args[offset + 1]);
case 41 :
return lib.dirEntryGetInode(receiver, args[offset]);
case 42 :
return lib.dirEntryGetType(receiver, args[offset]);
case 43 :
lib.utimensat(receiver, (int) args[offset], args[offset + 1], (long[]) args[offset + 2], (boolean) args[offset + 3]);
return null;
case 44 :
lib.futimens(receiver, (int) args[offset], (long[]) args[offset + 1]);
return null;
case 45 :
lib.futimes(receiver, (int) args[offset], (Timeval[]) args[offset + 1]);
return null;
case 46 :
lib.lutimes(receiver, args[offset], (Timeval[]) args[offset + 1]);
return null;
case 47 :
lib.utimes(receiver, args[offset], (Timeval[]) args[offset + 1]);
return null;
case 48 :
lib.renameat(receiver, (int) args[offset], args[offset + 1], (int) args[offset + 2], args[offset + 3]);
return null;
case 49 :
return lib.faccessat(receiver, (int) args[offset], args[offset + 1], (int) args[offset + 2], (boolean) args[offset + 3], (boolean) args[offset + 4]);
case 50 :
lib.fchmodat(receiver, (int) args[offset], args[offset + 1], (int) args[offset + 2], (boolean) args[offset + 3]);
return null;
case 51 :
lib.fchmod(receiver, (int) args[offset], (int) args[offset + 1]);
return null;
case 52 :
return lib.readlinkat(receiver, (int) args[offset], args[offset + 1]);
case 53 :
lib.kill(receiver, (long) args[offset], (int) args[offset + 1]);
return null;
case 54 :
lib.killpg(receiver, (long) args[offset], (int) args[offset + 1]);
return null;
case 55 :
return lib.waitpid(receiver, (long) args[offset], (int) args[offset + 1]);
case 56 :
lib.abort(receiver);
return null;
case 57 :
return lib.wcoredump(receiver, (int) args[offset]);
case 58 :
return lib.wifcontinued(receiver, (int) args[offset]);
case 59 :
return lib.wifstopped(receiver, (int) args[offset]);
case 60 :
return lib.wifsignaled(receiver, (int) args[offset]);
case 61 :
return lib.wifexited(receiver, (int) args[offset]);
case 62 :
return lib.wexitstatus(receiver, (int) args[offset]);
case 63 :
return lib.wtermsig(receiver, (int) args[offset]);
case 64 :
return lib.wstopsig(receiver, (int) args[offset]);
case 65 :
return lib.getuid(receiver);
case 66 :
return lib.geteuid(receiver);
case 67 :
return lib.getgid(receiver);
case 68 :
return lib.getppid(receiver);
case 69 :
return lib.getpgid(receiver, (long) args[offset]);
case 70 :
lib.setpgid(receiver, (long) args[offset], (long) args[offset + 1]);
return null;
case 71 :
return lib.getpgrp(receiver);
case 72 :
return lib.getsid(receiver, (long) args[offset]);
case 73 :
return lib.setsid(receiver);
case 74 :
return lib.openpty(receiver);
case 75 :
return lib.ctermid(receiver);
case 76 :
lib.setenv(receiver, args[offset], args[offset + 1], (boolean) args[offset + 2]);
return null;
case 77 :
lib.unsetenv(receiver, args[offset]);
return null;
case 78 :
return lib.forkExec(receiver, (Object[]) args[offset], (Object[]) args[offset + 1], args[offset + 2], (Object[]) args[offset + 3], (int) args[offset + 4], (int) args[offset + 5], (int) args[offset + 6], (int) args[offset + 7], (int) args[offset + 8], (int) args[offset + 9], (int) args[offset + 10], (int) args[offset + 11], (boolean) args[offset + 12], (boolean) args[offset + 13], (boolean) args[offset + 14], (int[]) args[offset + 15]);
case 79 :
lib.execv(receiver, args[offset], (Object[]) args[offset + 1]);
return null;
case 80 :
return lib.system(receiver, args[offset]);
case 81 :
return lib.mmap(receiver, (long) args[offset], (int) args[offset + 1], (int) args[offset + 2], (int) args[offset + 3], (long) args[offset + 4]);
case 82 :
return lib.mmapReadByte(receiver, args[offset], (long) args[offset + 1]);
case 83 :
lib.mmapWriteByte(receiver, args[offset], (long) args[offset + 1], (byte) args[offset + 2]);
return null;
case 84 :
return lib.mmapReadBytes(receiver, args[offset], (long) args[offset + 1], (byte[]) args[offset + 2], (int) args[offset + 3]);
case 85 :
lib.mmapWriteBytes(receiver, args[offset], (long) args[offset + 1], (byte[]) args[offset + 2], (int) args[offset + 3]);
return null;
case 86 :
lib.mmapFlush(receiver, args[offset], (long) args[offset + 1], (long) args[offset + 2]);
return null;
case 87 :
lib.mmapUnmap(receiver, args[offset], (long) args[offset + 1]);
return null;
case 88 :
return lib.mmapGetPointer(receiver, args[offset]);
case 89 :
return lib.getpwuid(receiver, (long) args[offset]);
case 90 :
return lib.getpwnam(receiver, args[offset]);
case 91 :
return lib.hasGetpwentries(receiver);
case 92 :
return lib.getpwentries(receiver);
case 93 :
return lib.createPathFromString(receiver, (TruffleString) args[offset]);
case 94 :
return lib.createPathFromBytes(receiver, (byte[]) args[offset]);
case 95 :
return lib.getPathAsString(receiver, args[offset]);
case 96 :
return lib.getPathAsBytes(receiver, args[offset]);
case 97 :
return lib.socket(receiver, (int) args[offset], (int) args[offset + 1], (int) args[offset + 2]);
case 98 :
return lib.accept(receiver, (int) args[offset]);
case 99 :
lib.bind(receiver, (int) args[offset], (UniversalSockAddr) args[offset + 1]);
return null;
case 100 :
lib.connect(receiver, (int) args[offset], (UniversalSockAddr) args[offset + 1]);
return null;
case 101 :
lib.listen(receiver, (int) args[offset], (int) args[offset + 1]);
return null;
case 102 :
return lib.getpeername(receiver, (int) args[offset]);
case 103 :
return lib.getsockname(receiver, (int) args[offset]);
case 104 :
return lib.send(receiver, (int) args[offset], (byte[]) args[offset + 1], (int) args[offset + 2], (int) args[offset + 3], (int) args[offset + 4]);
case 105 :
return lib.sendto(receiver, (int) args[offset], (byte[]) args[offset + 1], (int) args[offset + 2], (int) args[offset + 3], (int) args[offset + 4], (UniversalSockAddr) args[offset + 5]);
case 106 :
return lib.recv(receiver, (int) args[offset], (byte[]) args[offset + 1], (int) args[offset + 2], (int) args[offset + 3], (int) args[offset + 4]);
case 107 :
return lib.recvfrom(receiver, (int) args[offset], (byte[]) args[offset + 1], (int) args[offset + 2], (int) args[offset + 3], (int) args[offset + 4]);
case 108 :
lib.shutdown(receiver, (int) args[offset], (int) args[offset + 1]);
return null;
case 109 :
return lib.getsockopt(receiver, (int) args[offset], (int) args[offset + 1], (int) args[offset + 2], (byte[]) args[offset + 3], (int) args[offset + 4]);
case 110 :
lib.setsockopt(receiver, (int) args[offset], (int) args[offset + 1], (int) args[offset + 2], (byte[]) args[offset + 3], (int) args[offset + 4]);
return null;
case 111 :
return lib.inet_addr(receiver, args[offset]);
case 112 :
return lib.inet_aton(receiver, args[offset]);
case 113 :
return lib.inet_ntoa(receiver, (int) args[offset]);
case 114 :
return lib.inet_pton(receiver, (int) args[offset], args[offset + 1]);
case 115 :
return lib.inet_ntop(receiver, (int) args[offset], (byte[]) args[offset + 1]);
case 116 :
return lib.gethostname(receiver);
case 117 :
return lib.getnameinfo(receiver, (UniversalSockAddr) args[offset], (int) args[offset + 1]);
case 118 :
return lib.getaddrinfo(receiver, args[offset], args[offset + 1], (int) args[offset + 2], (int) args[offset + 3], (int) args[offset + 4], (int) args[offset + 5]);
case 119 :
return lib.crypt(receiver, (TruffleString) args[offset], (TruffleString) args[offset + 1]);
case 120 :
return lib.createUniversalSockAddr(receiver, (FamilySpecificSockAddr) args[offset]);
}
CompilerDirectives.transferToInterpreterAndInvalidate();
throw new AbstractMethodError(message.toString());
}
@Override
protected PosixSupportLibrary createDispatchImpl(int limit) {
return new CachedDispatchFirst(null, null, limit);
}
@Override
protected PosixSupportLibrary createUncachedDispatch() {
return new UncachedDispatch();
}
@SuppressWarnings("unchecked")
private static Class lazyLibraryClass() {
try {
return (Class) Class.forName("com.oracle.graal.python.runtime.PosixSupportLibrary", false, PosixSupportLibraryGen.class.getClassLoader());
} catch (ClassNotFoundException e) {
throw CompilerDirectives.shouldNotReachHere(e);
}
}
@GeneratedBy(PosixSupportLibrary.class)
private static final class Default extends LibraryExport {
private Default() {
super(PosixSupportLibrary.class, Object.class, false, false, 0);
}
@Override
protected PosixSupportLibrary createUncached(Object receiver) {
PosixSupportLibrary uncached = new Uncached(receiver);
return uncached;
}
@Override
protected PosixSupportLibrary createCached(Object receiver) {
return new Cached(receiver);
}
@GeneratedBy(PosixSupportLibrary.class)
private static final class Cached extends PosixSupportLibrary {
@Child private DynamicDispatchLibrary dynamicDispatch_;
private final Class> dynamicDispatchTarget_;
protected Cached(Object receiver) {
this.dynamicDispatch_ = insert(DYNAMIC_DISPATCH_LIBRARY_.create(receiver));
this.dynamicDispatchTarget_ = DYNAMIC_DISPATCH_LIBRARY_.getUncached(receiver).dispatch(receiver);
}
@Override
public boolean accepts(Object receiver) {
return dynamicDispatch_.accepts(receiver) && dynamicDispatch_.dispatch(receiver) == dynamicDispatchTarget_;
}
@TruffleBoundary
@Override
public TruffleString getBackend(Object receiver) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public TruffleString strerror(Object receiver, int errorCode) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public long getpid(Object receiver) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public int umask(Object receiver, int mask) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public int openat(Object receiver, int dirFd, Object pathname, int flags, int mode) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public int close(Object receiver, int fd) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public Buffer read(Object receiver, int fd, long length) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public long write(Object receiver, int fd, Buffer data) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public int dup(Object receiver, int fd) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public int dup2(Object receiver, int fd, int fd2, boolean inheritable) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public boolean getInheritable(Object receiver, int fd) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public void setInheritable(Object receiver, int fd, boolean inheritable) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public int[] pipe(Object receiver) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public SelectResult select(Object receiver, int[] readfds, int[] writefds, int[] errorfds, Timeval timeout) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public long lseek(Object receiver, int fd, long offset, int how) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public void ftruncate(Object receiver, int fd, long length) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public void fsync(Object receiver, int fd) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public void flock(Object receiver, int fd, int operation) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public boolean getBlocking(Object receiver, int fd) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public void setBlocking(Object receiver, int fd, boolean blocking) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public int[] getTerminalSize(Object receiver, int fd) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public long[] fstatat(Object receiver, int dirFd, Object pathname, boolean followSymlinks) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public long[] fstat(Object receiver, int fd) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public long[] statvfs(Object receiver, Object path) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public long[] fstatvfs(Object receiver, int fd) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public Object[] uname(Object receiver) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public void unlinkat(Object receiver, int dirFd, Object pathname, boolean rmdir) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public void linkat(Object receiver, int oldFdDir, Object oldPath, int newFdDir, Object newPath, int flags) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public void symlinkat(Object receiver, Object target, int linkpathDirFd, Object linkpath) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public void mkdirat(Object receiver, int dirFd, Object pathname, int mode) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public Object getcwd(Object receiver) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public void chdir(Object receiver, Object path) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public void fchdir(Object receiver, int fd) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public boolean isatty(Object receiver, int fd) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public Object opendir(Object receiver, Object path) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public Object fdopendir(Object receiver, int fd) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public void closedir(Object receiver, Object dirStream) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public Object readdir(Object receiver, Object dirStream) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public void rewinddir(Object receiver, Object dirStream) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public Object dirEntryGetName(Object receiver, Object dirEntry) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public Object dirEntryGetPath(Object receiver, Object dirEntry, Object scandirPath) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public long dirEntryGetInode(Object receiver, Object dirEntry) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public int dirEntryGetType(Object receiver, Object dirEntry) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public void utimensat(Object receiver, int dirFd, Object pathname, long[] timespec, boolean followSymlinks) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public void futimens(Object receiver, int fd, long[] timespec) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public void futimes(Object receiver, int fd, Timeval[] timeval) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public void lutimes(Object receiver, Object filename, Timeval[] timeval) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public void utimes(Object receiver, Object filename, Timeval[] timeval) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public void renameat(Object receiver, int oldDirFd, Object oldPath, int newDirFd, Object newPath) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public boolean faccessat(Object receiver, int dirFd, Object path, int mode, boolean effectiveIds, boolean followSymlinks) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public void fchmodat(Object receiver, int dirFd, Object path, int mode, boolean followSymlinks) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public void fchmod(Object receiver, int fd, int mode) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public Object readlinkat(Object receiver, int dirFd, Object path) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public void kill(Object receiver, long pid, int signal) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public void killpg(Object receiver, long pid, int signal) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public long[] waitpid(Object receiver, long pid, int options) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public void abort(Object receiver) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public boolean wcoredump(Object receiver, int status) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public boolean wifcontinued(Object receiver, int status) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public boolean wifstopped(Object receiver, int status) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public boolean wifsignaled(Object receiver, int status) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public boolean wifexited(Object receiver, int status) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public int wexitstatus(Object receiver, int status) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public int wtermsig(Object receiver, int status) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public int wstopsig(Object receiver, int status) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public long getuid(Object receiver) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public long geteuid(Object receiver) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public long getgid(Object receiver) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public long getppid(Object receiver) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public long getpgid(Object receiver, long pid) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public void setpgid(Object receiver, long pid, long pgid) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public long getpgrp(Object receiver) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public long getsid(Object receiver, long pid) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public long setsid(Object receiver) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public OpenPtyResult openpty(Object receiver) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public TruffleString ctermid(Object receiver) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public void setenv(Object receiver, Object name, Object value, boolean overwrite) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public void unsetenv(Object receiver, Object name) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public int forkExec(Object receiver, Object[] executables, Object[] args, Object cwd, Object[] env, int stdinReadFd, int stdinWriteFd, int stdoutReadFd, int stdoutWriteFd, int stderrReadFd, int stderrWriteFd, int errPipeReadFd, int errPipeWriteFd, boolean closeFds, boolean restoreSignals, boolean callSetsid, int[] fdsToKeep) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public void execv(Object receiver, Object pathname, Object[] args) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public int system(Object receiver, Object command) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public Object mmap(Object receiver, long length, int prot, int flags, int fd, long offset) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public byte mmapReadByte(Object receiver, Object mmap, long index) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public void mmapWriteByte(Object receiver, Object mmap, long index, byte value) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public int mmapReadBytes(Object receiver, Object mmap, long index, byte[] bytes, int length) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public void mmapWriteBytes(Object receiver, Object mmap, long index, byte[] bytes, int length) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public void mmapFlush(Object receiver, Object mmap, long offset, long length) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public void mmapUnmap(Object receiver, Object mmap, long length) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public long mmapGetPointer(Object receiver, Object mmap) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public PwdResult getpwuid(Object receiver, long uid) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public PwdResult getpwnam(Object receiver, Object name) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public boolean hasGetpwentries(Object receiver) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public PwdResult[] getpwentries(Object receiver) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public Object createPathFromString(Object receiver, TruffleString path) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public Object createPathFromBytes(Object receiver, byte[] path) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public TruffleString getPathAsString(Object receiver, Object path) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public Buffer getPathAsBytes(Object receiver, Object path) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public int socket(Object receiver, int domain, int type, int protocol) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public AcceptResult accept(Object receiver, int sockfd) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public void bind(Object receiver, int sockfd, UniversalSockAddr addr) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public void connect(Object receiver, int sockfd, UniversalSockAddr addr) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public void listen(Object receiver, int sockfd, int backlog) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public UniversalSockAddr getpeername(Object receiver, int sockfd) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public UniversalSockAddr getsockname(Object receiver, int sockfd) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public int send(Object receiver, int sockfd, byte[] buf, int offset, int len, int flags) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public int sendto(Object receiver, int sockfd, byte[] buf, int offset, int len, int flags, UniversalSockAddr destAddr) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public int recv(Object receiver, int sockfd, byte[] buf, int offset, int len, int flags) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public RecvfromResult recvfrom(Object receiver, int sockfd, byte[] buf, int offset, int len, int flags) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public void shutdown(Object receiver, int sockfd, int how) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public int getsockopt(Object receiver, int sockfd, int level, int optname, byte[] optval, int optlen) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public void setsockopt(Object receiver, int sockfd, int level, int optname, byte[] optval, int optlen) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public int inet_addr(Object receiver, Object src) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public int inet_aton(Object receiver, Object src) throws InvalidAddressException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public Object inet_ntoa(Object receiver, int address) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public byte[] inet_pton(Object receiver, int family, Object src) throws PosixException, InvalidAddressException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public Object inet_ntop(Object receiver, int family, byte[] src) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public Object gethostname(Object receiver) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public Object[] getnameinfo(Object receiver, UniversalSockAddr addr, int flags) throws GetAddrInfoException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public AddrInfoCursor getaddrinfo(Object receiver, Object node, Object service, int family, int sockType, int protocol, int flags) throws GetAddrInfoException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public TruffleString crypt(Object receiver, TruffleString word, TruffleString salt) throws PosixException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public UniversalSockAddr createUniversalSockAddr(Object receiver, FamilySpecificSockAddr src) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
}
@GeneratedBy(PosixSupportLibrary.class)
@DenyReplace
private static final class Uncached extends PosixSupportLibrary {
@Child private DynamicDispatchLibrary dynamicDispatch_;
private final Class> dynamicDispatchTarget_;
protected Uncached(Object receiver) {
this.dynamicDispatch_ = DYNAMIC_DISPATCH_LIBRARY_.getUncached(receiver);
this.dynamicDispatchTarget_ = dynamicDispatch_.dispatch(receiver);
}
@Override
@TruffleBoundary
public boolean accepts(Object receiver) {
return dynamicDispatch_.accepts(receiver) && dynamicDispatch_.dispatch(receiver) == dynamicDispatchTarget_;
}
@Override
public boolean isAdoptable() {
return false;
}
@Override
public NodeCost getCost() {
return NodeCost.MEGAMORPHIC;
}
@TruffleBoundary
@Override
public TruffleString getBackend(Object receiver) {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public TruffleString strerror(Object receiver, int errorCode) {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public long getpid(Object receiver) {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public int umask(Object receiver, int mask) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public int openat(Object receiver, int dirFd, Object pathname, int flags, int mode) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public int close(Object receiver, int fd) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public Buffer read(Object receiver, int fd, long length) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public long write(Object receiver, int fd, Buffer data) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public int dup(Object receiver, int fd) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public int dup2(Object receiver, int fd, int fd2, boolean inheritable) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public boolean getInheritable(Object receiver, int fd) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public void setInheritable(Object receiver, int fd, boolean inheritable) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public int[] pipe(Object receiver) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public SelectResult select(Object receiver, int[] readfds, int[] writefds, int[] errorfds, Timeval timeout) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public long lseek(Object receiver, int fd, long offset, int how) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public void ftruncate(Object receiver, int fd, long length) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public void fsync(Object receiver, int fd) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public void flock(Object receiver, int fd, int operation) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public boolean getBlocking(Object receiver, int fd) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public void setBlocking(Object receiver, int fd, boolean blocking) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public int[] getTerminalSize(Object receiver, int fd) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public long[] fstatat(Object receiver, int dirFd, Object pathname, boolean followSymlinks) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public long[] fstat(Object receiver, int fd) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public long[] statvfs(Object receiver, Object path) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public long[] fstatvfs(Object receiver, int fd) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public Object[] uname(Object receiver) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public void unlinkat(Object receiver, int dirFd, Object pathname, boolean rmdir) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public void linkat(Object receiver, int oldFdDir, Object oldPath, int newFdDir, Object newPath, int flags) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public void symlinkat(Object receiver, Object target, int linkpathDirFd, Object linkpath) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public void mkdirat(Object receiver, int dirFd, Object pathname, int mode) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public Object getcwd(Object receiver) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public void chdir(Object receiver, Object path) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public void fchdir(Object receiver, int fd) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public boolean isatty(Object receiver, int fd) {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public Object opendir(Object receiver, Object path) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public Object fdopendir(Object receiver, int fd) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public void closedir(Object receiver, Object dirStream) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public Object readdir(Object receiver, Object dirStream) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public void rewinddir(Object receiver, Object dirStream) {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public Object dirEntryGetName(Object receiver, Object dirEntry) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public Object dirEntryGetPath(Object receiver, Object dirEntry, Object scandirPath) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public long dirEntryGetInode(Object receiver, Object dirEntry) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public int dirEntryGetType(Object receiver, Object dirEntry) {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public void utimensat(Object receiver, int dirFd, Object pathname, long[] timespec, boolean followSymlinks) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public void futimens(Object receiver, int fd, long[] timespec) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public void futimes(Object receiver, int fd, Timeval[] timeval) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public void lutimes(Object receiver, Object filename, Timeval[] timeval) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public void utimes(Object receiver, Object filename, Timeval[] timeval) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public void renameat(Object receiver, int oldDirFd, Object oldPath, int newDirFd, Object newPath) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public boolean faccessat(Object receiver, int dirFd, Object path, int mode, boolean effectiveIds, boolean followSymlinks) {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public void fchmodat(Object receiver, int dirFd, Object path, int mode, boolean followSymlinks) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public void fchmod(Object receiver, int fd, int mode) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public Object readlinkat(Object receiver, int dirFd, Object path) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public void kill(Object receiver, long pid, int signal) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public void killpg(Object receiver, long pid, int signal) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public long[] waitpid(Object receiver, long pid, int options) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public void abort(Object receiver) {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public boolean wcoredump(Object receiver, int status) {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public boolean wifcontinued(Object receiver, int status) {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public boolean wifstopped(Object receiver, int status) {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public boolean wifsignaled(Object receiver, int status) {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public boolean wifexited(Object receiver, int status) {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public int wexitstatus(Object receiver, int status) {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public int wtermsig(Object receiver, int status) {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public int wstopsig(Object receiver, int status) {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public long getuid(Object receiver) {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public long geteuid(Object receiver) {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public long getgid(Object receiver) {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public long getppid(Object receiver) {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public long getpgid(Object receiver, long pid) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public void setpgid(Object receiver, long pid, long pgid) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public long getpgrp(Object receiver) {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public long getsid(Object receiver, long pid) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public long setsid(Object receiver) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public OpenPtyResult openpty(Object receiver) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public TruffleString ctermid(Object receiver) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public void setenv(Object receiver, Object name, Object value, boolean overwrite) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public void unsetenv(Object receiver, Object name) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public int forkExec(Object receiver, Object[] executables, Object[] args, Object cwd, Object[] env, int stdinReadFd, int stdinWriteFd, int stdoutReadFd, int stdoutWriteFd, int stderrReadFd, int stderrWriteFd, int errPipeReadFd, int errPipeWriteFd, boolean closeFds, boolean restoreSignals, boolean callSetsid, int[] fdsToKeep) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public void execv(Object receiver, Object pathname, Object[] args) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public int system(Object receiver, Object command) {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public Object mmap(Object receiver, long length, int prot, int flags, int fd, long offset) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public byte mmapReadByte(Object receiver, Object mmap, long index) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public void mmapWriteByte(Object receiver, Object mmap, long index, byte value) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public int mmapReadBytes(Object receiver, Object mmap, long index, byte[] bytes, int length) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public void mmapWriteBytes(Object receiver, Object mmap, long index, byte[] bytes, int length) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public void mmapFlush(Object receiver, Object mmap, long offset, long length) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public void mmapUnmap(Object receiver, Object mmap, long length) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public long mmapGetPointer(Object receiver, Object mmap) {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public PwdResult getpwuid(Object receiver, long uid) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public PwdResult getpwnam(Object receiver, Object name) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public boolean hasGetpwentries(Object receiver) {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public PwdResult[] getpwentries(Object receiver) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public Object createPathFromString(Object receiver, TruffleString path) {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public Object createPathFromBytes(Object receiver, byte[] path) {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public TruffleString getPathAsString(Object receiver, Object path) {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public Buffer getPathAsBytes(Object receiver, Object path) {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public int socket(Object receiver, int domain, int type, int protocol) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public AcceptResult accept(Object receiver, int sockfd) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public void bind(Object receiver, int sockfd, UniversalSockAddr addr) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public void connect(Object receiver, int sockfd, UniversalSockAddr addr) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public void listen(Object receiver, int sockfd, int backlog) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public UniversalSockAddr getpeername(Object receiver, int sockfd) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public UniversalSockAddr getsockname(Object receiver, int sockfd) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public int send(Object receiver, int sockfd, byte[] buf, int offset, int len, int flags) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public int sendto(Object receiver, int sockfd, byte[] buf, int offset, int len, int flags, UniversalSockAddr destAddr) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public int recv(Object receiver, int sockfd, byte[] buf, int offset, int len, int flags) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public RecvfromResult recvfrom(Object receiver, int sockfd, byte[] buf, int offset, int len, int flags) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public void shutdown(Object receiver, int sockfd, int how) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public int getsockopt(Object receiver, int sockfd, int level, int optname, byte[] optval, int optlen) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public void setsockopt(Object receiver, int sockfd, int level, int optname, byte[] optval, int optlen) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public int inet_addr(Object receiver, Object src) {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public int inet_aton(Object receiver, Object src) throws InvalidAddressException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public Object inet_ntoa(Object receiver, int address) {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public byte[] inet_pton(Object receiver, int family, Object src) throws PosixException, InvalidAddressException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public Object inet_ntop(Object receiver, int family, byte[] src) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public Object gethostname(Object receiver) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public Object[] getnameinfo(Object receiver, UniversalSockAddr addr, int flags) throws GetAddrInfoException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public AddrInfoCursor getaddrinfo(Object receiver, Object node, Object service, int family, int sockType, int protocol, int flags) throws GetAddrInfoException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public TruffleString crypt(Object receiver, TruffleString word, TruffleString salt) throws PosixException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
@TruffleBoundary
@Override
public UniversalSockAddr createUniversalSockAddr(Object receiver, FamilySpecificSockAddr src) {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
throw new AbstractMethodError();
}
}
}
@GeneratedBy(PosixSupportLibrary.class)
private static class MessageImpl extends Message {
MessageImpl(String name, int index, boolean deprecated, Class> returnType, Class>... parameters) {
super(PosixSupportLibraryGen.LIBRARY_CLASS, name, index, deprecated, returnType, parameters);
}
}
@GeneratedBy(PosixSupportLibrary.class)
private static final class Proxy extends PosixSupportLibrary {
@Child private ReflectionLibrary lib;
Proxy(ReflectionLibrary lib) {
this.lib = lib;
}
@Override
public TruffleString getBackend(Object receiver_) {
try {
return (TruffleString) lib.send(receiver_, PosixSupportLibraryGen.GET_BACKEND);
} catch (RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public TruffleString strerror(Object receiver_, int errorCode) {
try {
return (TruffleString) lib.send(receiver_, PosixSupportLibraryGen.STRERROR, errorCode);
} catch (RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public long getpid(Object receiver_) {
try {
return (long) lib.send(receiver_, PosixSupportLibraryGen.GETPID);
} catch (RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public int umask(Object receiver_, int mask) throws PosixException {
try {
return (int) lib.send(receiver_, PosixSupportLibraryGen.UMASK, mask);
} catch (PosixException | RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public int openat(Object receiver_, int dirFd, Object pathname, int flags, int mode) throws PosixException {
try {
return (int) lib.send(receiver_, PosixSupportLibraryGen.OPENAT, dirFd, pathname, flags, mode);
} catch (PosixException | RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public int close(Object receiver_, int fd) throws PosixException {
try {
return (int) lib.send(receiver_, PosixSupportLibraryGen.CLOSE, fd);
} catch (PosixException | RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public Buffer read(Object receiver_, int fd, long length) throws PosixException {
try {
return (Buffer) lib.send(receiver_, PosixSupportLibraryGen.READ, fd, length);
} catch (PosixException | RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public long write(Object receiver_, int fd, Buffer data) throws PosixException {
try {
return (long) lib.send(receiver_, PosixSupportLibraryGen.WRITE, fd, data);
} catch (PosixException | RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public int dup(Object receiver_, int fd) throws PosixException {
try {
return (int) lib.send(receiver_, PosixSupportLibraryGen.DUP, fd);
} catch (PosixException | RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public int dup2(Object receiver_, int fd, int fd2, boolean inheritable) throws PosixException {
try {
return (int) lib.send(receiver_, PosixSupportLibraryGen.DUP2, fd, fd2, inheritable);
} catch (PosixException | RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public boolean getInheritable(Object receiver_, int fd) throws PosixException {
try {
return (boolean) lib.send(receiver_, PosixSupportLibraryGen.GET_INHERITABLE, fd);
} catch (PosixException | RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public void setInheritable(Object receiver_, int fd, boolean inheritable) throws PosixException {
try {
lib.send(receiver_, PosixSupportLibraryGen.SET_INHERITABLE, fd, inheritable);
return;
} catch (PosixException | RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public int[] pipe(Object receiver_) throws PosixException {
try {
return (int[]) lib.send(receiver_, PosixSupportLibraryGen.PIPE);
} catch (PosixException | RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public SelectResult select(Object receiver_, int[] readfds, int[] writefds, int[] errorfds, Timeval timeout) throws PosixException {
try {
return (SelectResult) lib.send(receiver_, PosixSupportLibraryGen.SELECT, readfds, writefds, errorfds, timeout);
} catch (PosixException | RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public long lseek(Object receiver_, int fd, long offset, int how) throws PosixException {
try {
return (long) lib.send(receiver_, PosixSupportLibraryGen.LSEEK, fd, offset, how);
} catch (PosixException | RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public void ftruncate(Object receiver_, int fd, long length) throws PosixException {
try {
lib.send(receiver_, PosixSupportLibraryGen.FTRUNCATE, fd, length);
return;
} catch (PosixException | RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public void fsync(Object receiver_, int fd) throws PosixException {
try {
lib.send(receiver_, PosixSupportLibraryGen.FSYNC, fd);
return;
} catch (PosixException | RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public void flock(Object receiver_, int fd, int operation) throws PosixException {
try {
lib.send(receiver_, PosixSupportLibraryGen.FLOCK, fd, operation);
return;
} catch (PosixException | RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public boolean getBlocking(Object receiver_, int fd) throws PosixException {
try {
return (boolean) lib.send(receiver_, PosixSupportLibraryGen.GET_BLOCKING, fd);
} catch (PosixException | RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public void setBlocking(Object receiver_, int fd, boolean blocking) throws PosixException {
try {
lib.send(receiver_, PosixSupportLibraryGen.SET_BLOCKING, fd, blocking);
return;
} catch (PosixException | RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public int[] getTerminalSize(Object receiver_, int fd) throws PosixException {
try {
return (int[]) lib.send(receiver_, PosixSupportLibraryGen.GET_TERMINAL_SIZE, fd);
} catch (PosixException | RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public long[] fstatat(Object receiver_, int dirFd, Object pathname, boolean followSymlinks) throws PosixException {
try {
return (long[]) lib.send(receiver_, PosixSupportLibraryGen.FSTATAT, dirFd, pathname, followSymlinks);
} catch (PosixException | RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public long[] fstat(Object receiver_, int fd) throws PosixException {
try {
return (long[]) lib.send(receiver_, PosixSupportLibraryGen.FSTAT, fd);
} catch (PosixException | RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public long[] statvfs(Object receiver_, Object path) throws PosixException {
try {
return (long[]) lib.send(receiver_, PosixSupportLibraryGen.STATVFS, path);
} catch (PosixException | RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public long[] fstatvfs(Object receiver_, int fd) throws PosixException {
try {
return (long[]) lib.send(receiver_, PosixSupportLibraryGen.FSTATVFS, fd);
} catch (PosixException | RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public Object[] uname(Object receiver_) throws PosixException {
try {
return (Object[]) lib.send(receiver_, PosixSupportLibraryGen.UNAME);
} catch (PosixException | RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public void unlinkat(Object receiver_, int dirFd, Object pathname, boolean rmdir) throws PosixException {
try {
lib.send(receiver_, PosixSupportLibraryGen.UNLINKAT, dirFd, pathname, rmdir);
return;
} catch (PosixException | RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public void linkat(Object receiver_, int oldFdDir, Object oldPath, int newFdDir, Object newPath, int flags) throws PosixException {
try {
lib.send(receiver_, PosixSupportLibraryGen.LINKAT, oldFdDir, oldPath, newFdDir, newPath, flags);
return;
} catch (PosixException | RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public void symlinkat(Object receiver_, Object target, int linkpathDirFd, Object linkpath) throws PosixException {
try {
lib.send(receiver_, PosixSupportLibraryGen.SYMLINKAT, target, linkpathDirFd, linkpath);
return;
} catch (PosixException | RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public void mkdirat(Object receiver_, int dirFd, Object pathname, int mode) throws PosixException {
try {
lib.send(receiver_, PosixSupportLibraryGen.MKDIRAT, dirFd, pathname, mode);
return;
} catch (PosixException | RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public Object getcwd(Object receiver_) throws PosixException {
try {
return lib.send(receiver_, PosixSupportLibraryGen.GETCWD);
} catch (PosixException | RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public void chdir(Object receiver_, Object path) throws PosixException {
try {
lib.send(receiver_, PosixSupportLibraryGen.CHDIR, path);
return;
} catch (PosixException | RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public void fchdir(Object receiver_, int fd) throws PosixException {
try {
lib.send(receiver_, PosixSupportLibraryGen.FCHDIR, fd);
return;
} catch (PosixException | RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public boolean isatty(Object receiver_, int fd) {
try {
return (boolean) lib.send(receiver_, PosixSupportLibraryGen.ISATTY, fd);
} catch (RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public Object opendir(Object receiver_, Object path) throws PosixException {
try {
return lib.send(receiver_, PosixSupportLibraryGen.OPENDIR, path);
} catch (PosixException | RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public Object fdopendir(Object receiver_, int fd) throws PosixException {
try {
return lib.send(receiver_, PosixSupportLibraryGen.FDOPENDIR, fd);
} catch (PosixException | RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public void closedir(Object receiver_, Object dirStream) throws PosixException {
try {
lib.send(receiver_, PosixSupportLibraryGen.CLOSEDIR, dirStream);
return;
} catch (PosixException | RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public Object readdir(Object receiver_, Object dirStream) throws PosixException {
try {
return lib.send(receiver_, PosixSupportLibraryGen.READDIR, dirStream);
} catch (PosixException | RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public void rewinddir(Object receiver_, Object dirStream) {
try {
lib.send(receiver_, PosixSupportLibraryGen.REWINDDIR, dirStream);
return;
} catch (RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public Object dirEntryGetName(Object receiver_, Object dirEntry) throws PosixException {
try {
return lib.send(receiver_, PosixSupportLibraryGen.DIR_ENTRY_GET_NAME, dirEntry);
} catch (PosixException | RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public Object dirEntryGetPath(Object receiver_, Object dirEntry, Object scandirPath) throws PosixException {
try {
return lib.send(receiver_, PosixSupportLibraryGen.DIR_ENTRY_GET_PATH, dirEntry, scandirPath);
} catch (PosixException | RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public long dirEntryGetInode(Object receiver_, Object dirEntry) throws PosixException {
try {
return (long) lib.send(receiver_, PosixSupportLibraryGen.DIR_ENTRY_GET_INODE, dirEntry);
} catch (PosixException | RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public int dirEntryGetType(Object receiver_, Object dirEntry) {
try {
return (int) lib.send(receiver_, PosixSupportLibraryGen.DIR_ENTRY_GET_TYPE, dirEntry);
} catch (RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public void utimensat(Object receiver_, int dirFd, Object pathname, long[] timespec, boolean followSymlinks) throws PosixException {
try {
lib.send(receiver_, PosixSupportLibraryGen.UTIMENSAT, dirFd, pathname, timespec, followSymlinks);
return;
} catch (PosixException | RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public void futimens(Object receiver_, int fd, long[] timespec) throws PosixException {
try {
lib.send(receiver_, PosixSupportLibraryGen.FUTIMENS, fd, timespec);
return;
} catch (PosixException | RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public void futimes(Object receiver_, int fd, Timeval[] timeval) throws PosixException {
try {
lib.send(receiver_, PosixSupportLibraryGen.FUTIMES, fd, timeval);
return;
} catch (PosixException | RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public void lutimes(Object receiver_, Object filename, Timeval[] timeval) throws PosixException {
try {
lib.send(receiver_, PosixSupportLibraryGen.LUTIMES, filename, timeval);
return;
} catch (PosixException | RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public void utimes(Object receiver_, Object filename, Timeval[] timeval) throws PosixException {
try {
lib.send(receiver_, PosixSupportLibraryGen.UTIMES, filename, timeval);
return;
} catch (PosixException | RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public void renameat(Object receiver_, int oldDirFd, Object oldPath, int newDirFd, Object newPath) throws PosixException {
try {
lib.send(receiver_, PosixSupportLibraryGen.RENAMEAT, oldDirFd, oldPath, newDirFd, newPath);
return;
} catch (PosixException | RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public boolean faccessat(Object receiver_, int dirFd, Object path, int mode, boolean effectiveIds, boolean followSymlinks) {
try {
return (boolean) lib.send(receiver_, PosixSupportLibraryGen.FACCESSAT, dirFd, path, mode, effectiveIds, followSymlinks);
} catch (RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public void fchmodat(Object receiver_, int dirFd, Object path, int mode, boolean followSymlinks) throws PosixException {
try {
lib.send(receiver_, PosixSupportLibraryGen.FCHMODAT, dirFd, path, mode, followSymlinks);
return;
} catch (PosixException | RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public void fchmod(Object receiver_, int fd, int mode) throws PosixException {
try {
lib.send(receiver_, PosixSupportLibraryGen.FCHMOD, fd, mode);
return;
} catch (PosixException | RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public Object readlinkat(Object receiver_, int dirFd, Object path) throws PosixException {
try {
return lib.send(receiver_, PosixSupportLibraryGen.READLINKAT, dirFd, path);
} catch (PosixException | RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public void kill(Object receiver_, long pid, int signal) throws PosixException {
try {
lib.send(receiver_, PosixSupportLibraryGen.KILL, pid, signal);
return;
} catch (PosixException | RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public void killpg(Object receiver_, long pid, int signal) throws PosixException {
try {
lib.send(receiver_, PosixSupportLibraryGen.KILLPG, pid, signal);
return;
} catch (PosixException | RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public long[] waitpid(Object receiver_, long pid, int options) throws PosixException {
try {
return (long[]) lib.send(receiver_, PosixSupportLibraryGen.WAITPID, pid, options);
} catch (PosixException | RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public void abort(Object receiver_) {
try {
lib.send(receiver_, PosixSupportLibraryGen.ABORT);
return;
} catch (RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public boolean wcoredump(Object receiver_, int status) {
try {
return (boolean) lib.send(receiver_, PosixSupportLibraryGen.WCOREDUMP, status);
} catch (RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public boolean wifcontinued(Object receiver_, int status) {
try {
return (boolean) lib.send(receiver_, PosixSupportLibraryGen.WIFCONTINUED, status);
} catch (RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public boolean wifstopped(Object receiver_, int status) {
try {
return (boolean) lib.send(receiver_, PosixSupportLibraryGen.WIFSTOPPED, status);
} catch (RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public boolean wifsignaled(Object receiver_, int status) {
try {
return (boolean) lib.send(receiver_, PosixSupportLibraryGen.WIFSIGNALED, status);
} catch (RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public boolean wifexited(Object receiver_, int status) {
try {
return (boolean) lib.send(receiver_, PosixSupportLibraryGen.WIFEXITED, status);
} catch (RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public int wexitstatus(Object receiver_, int status) {
try {
return (int) lib.send(receiver_, PosixSupportLibraryGen.WEXITSTATUS, status);
} catch (RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public int wtermsig(Object receiver_, int status) {
try {
return (int) lib.send(receiver_, PosixSupportLibraryGen.WTERMSIG, status);
} catch (RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public int wstopsig(Object receiver_, int status) {
try {
return (int) lib.send(receiver_, PosixSupportLibraryGen.WSTOPSIG, status);
} catch (RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public long getuid(Object receiver_) {
try {
return (long) lib.send(receiver_, PosixSupportLibraryGen.GETUID);
} catch (RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public long geteuid(Object receiver_) {
try {
return (long) lib.send(receiver_, PosixSupportLibraryGen.GETEUID);
} catch (RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public long getgid(Object receiver_) {
try {
return (long) lib.send(receiver_, PosixSupportLibraryGen.GETGID);
} catch (RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public long getppid(Object receiver_) {
try {
return (long) lib.send(receiver_, PosixSupportLibraryGen.GETPPID);
} catch (RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public long getpgid(Object receiver_, long pid) throws PosixException {
try {
return (long) lib.send(receiver_, PosixSupportLibraryGen.GETPGID, pid);
} catch (PosixException | RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public void setpgid(Object receiver_, long pid, long pgid) throws PosixException {
try {
lib.send(receiver_, PosixSupportLibraryGen.SETPGID, pid, pgid);
return;
} catch (PosixException | RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public long getpgrp(Object receiver_) {
try {
return (long) lib.send(receiver_, PosixSupportLibraryGen.GETPGRP);
} catch (RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public long getsid(Object receiver_, long pid) throws PosixException {
try {
return (long) lib.send(receiver_, PosixSupportLibraryGen.GETSID, pid);
} catch (PosixException | RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public long setsid(Object receiver_) throws PosixException {
try {
return (long) lib.send(receiver_, PosixSupportLibraryGen.SETSID);
} catch (PosixException | RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public OpenPtyResult openpty(Object receiver_) throws PosixException {
try {
return (OpenPtyResult) lib.send(receiver_, PosixSupportLibraryGen.OPENPTY);
} catch (PosixException | RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public TruffleString ctermid(Object receiver_) throws PosixException {
try {
return (TruffleString) lib.send(receiver_, PosixSupportLibraryGen.CTERMID);
} catch (PosixException | RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public void setenv(Object receiver_, Object name, Object value, boolean overwrite) throws PosixException {
try {
lib.send(receiver_, PosixSupportLibraryGen.SETENV, name, value, overwrite);
return;
} catch (PosixException | RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public void unsetenv(Object receiver_, Object name) throws PosixException {
try {
lib.send(receiver_, PosixSupportLibraryGen.UNSETENV, name);
return;
} catch (PosixException | RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public int forkExec(Object receiver_, Object[] executables, Object[] args, Object cwd, Object[] env, int stdinReadFd, int stdinWriteFd, int stdoutReadFd, int stdoutWriteFd, int stderrReadFd, int stderrWriteFd, int errPipeReadFd, int errPipeWriteFd, boolean closeFds, boolean restoreSignals, boolean callSetsid, int[] fdsToKeep) throws PosixException {
try {
return (int) lib.send(receiver_, PosixSupportLibraryGen.FORK_EXEC, executables, args, cwd, env, stdinReadFd, stdinWriteFd, stdoutReadFd, stdoutWriteFd, stderrReadFd, stderrWriteFd, errPipeReadFd, errPipeWriteFd, closeFds, restoreSignals, callSetsid, fdsToKeep);
} catch (PosixException | RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public void execv(Object receiver_, Object pathname, Object[] args) throws PosixException {
try {
lib.send(receiver_, PosixSupportLibraryGen.EXECV, pathname, args);
return;
} catch (PosixException | RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public int system(Object receiver_, Object command) {
try {
return (int) lib.send(receiver_, PosixSupportLibraryGen.SYSTEM, command);
} catch (RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public Object mmap(Object receiver_, long length, int prot, int flags, int fd, long offset) throws PosixException {
try {
return lib.send(receiver_, PosixSupportLibraryGen.MMAP, length, prot, flags, fd, offset);
} catch (PosixException | RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public byte mmapReadByte(Object receiver_, Object mmap, long index) throws PosixException {
try {
return (byte) lib.send(receiver_, PosixSupportLibraryGen.MMAP_READ_BYTE, mmap, index);
} catch (PosixException | RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public void mmapWriteByte(Object receiver_, Object mmap, long index, byte value) throws PosixException {
try {
lib.send(receiver_, PosixSupportLibraryGen.MMAP_WRITE_BYTE, mmap, index, value);
return;
} catch (PosixException | RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public int mmapReadBytes(Object receiver_, Object mmap, long index, byte[] bytes, int length) throws PosixException {
try {
return (int) lib.send(receiver_, PosixSupportLibraryGen.MMAP_READ_BYTES, mmap, index, bytes, length);
} catch (PosixException | RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public void mmapWriteBytes(Object receiver_, Object mmap, long index, byte[] bytes, int length) throws PosixException {
try {
lib.send(receiver_, PosixSupportLibraryGen.MMAP_WRITE_BYTES, mmap, index, bytes, length);
return;
} catch (PosixException | RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public void mmapFlush(Object receiver_, Object mmap, long offset, long length) throws PosixException {
try {
lib.send(receiver_, PosixSupportLibraryGen.MMAP_FLUSH, mmap, offset, length);
return;
} catch (PosixException | RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public void mmapUnmap(Object receiver_, Object mmap, long length) throws PosixException {
try {
lib.send(receiver_, PosixSupportLibraryGen.MMAP_UNMAP, mmap, length);
return;
} catch (PosixException | RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public long mmapGetPointer(Object receiver_, Object mmap) {
try {
return (long) lib.send(receiver_, PosixSupportLibraryGen.MMAP_GET_POINTER, mmap);
} catch (RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public PwdResult getpwuid(Object receiver_, long uid) throws PosixException {
try {
return (PwdResult) lib.send(receiver_, PosixSupportLibraryGen.GETPWUID, uid);
} catch (PosixException | RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public PwdResult getpwnam(Object receiver_, Object name) throws PosixException {
try {
return (PwdResult) lib.send(receiver_, PosixSupportLibraryGen.GETPWNAM, name);
} catch (PosixException | RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public boolean hasGetpwentries(Object receiver_) {
try {
return (boolean) lib.send(receiver_, PosixSupportLibraryGen.HAS_GETPWENTRIES);
} catch (RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public PwdResult[] getpwentries(Object receiver_) throws PosixException {
try {
return (PwdResult[]) lib.send(receiver_, PosixSupportLibraryGen.GETPWENTRIES);
} catch (PosixException | RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public Object createPathFromString(Object receiver_, TruffleString path) {
try {
return lib.send(receiver_, PosixSupportLibraryGen.CREATE_PATH_FROM_STRING, path);
} catch (RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public Object createPathFromBytes(Object receiver_, byte[] path) {
try {
return lib.send(receiver_, PosixSupportLibraryGen.CREATE_PATH_FROM_BYTES, path);
} catch (RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public TruffleString getPathAsString(Object receiver_, Object path) {
try {
return (TruffleString) lib.send(receiver_, PosixSupportLibraryGen.GET_PATH_AS_STRING, path);
} catch (RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public Buffer getPathAsBytes(Object receiver_, Object path) {
try {
return (Buffer) lib.send(receiver_, PosixSupportLibraryGen.GET_PATH_AS_BYTES, path);
} catch (RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public int socket(Object receiver_, int domain, int type, int protocol) throws PosixException {
try {
return (int) lib.send(receiver_, PosixSupportLibraryGen.SOCKET, domain, type, protocol);
} catch (PosixException | RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public AcceptResult accept(Object receiver_, int sockfd) throws PosixException {
try {
return (AcceptResult) lib.send(receiver_, PosixSupportLibraryGen.ACCEPT, sockfd);
} catch (PosixException | RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public void bind(Object receiver_, int sockfd, UniversalSockAddr addr) throws PosixException {
try {
lib.send(receiver_, PosixSupportLibraryGen.BIND, sockfd, addr);
return;
} catch (PosixException | RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public void connect(Object receiver_, int sockfd, UniversalSockAddr addr) throws PosixException {
try {
lib.send(receiver_, PosixSupportLibraryGen.CONNECT, sockfd, addr);
return;
} catch (PosixException | RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public void listen(Object receiver_, int sockfd, int backlog) throws PosixException {
try {
lib.send(receiver_, PosixSupportLibraryGen.LISTEN, sockfd, backlog);
return;
} catch (PosixException | RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public UniversalSockAddr getpeername(Object receiver_, int sockfd) throws PosixException {
try {
return (UniversalSockAddr) lib.send(receiver_, PosixSupportLibraryGen.GETPEERNAME, sockfd);
} catch (PosixException | RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public UniversalSockAddr getsockname(Object receiver_, int sockfd) throws PosixException {
try {
return (UniversalSockAddr) lib.send(receiver_, PosixSupportLibraryGen.GETSOCKNAME, sockfd);
} catch (PosixException | RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public int send(Object receiver_, int sockfd, byte[] buf, int offset, int len, int flags) throws PosixException {
try {
return (int) lib.send(receiver_, PosixSupportLibraryGen.SEND, sockfd, buf, offset, len, flags);
} catch (PosixException | RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public int sendto(Object receiver_, int sockfd, byte[] buf, int offset, int len, int flags, UniversalSockAddr destAddr) throws PosixException {
try {
return (int) lib.send(receiver_, PosixSupportLibraryGen.SENDTO, sockfd, buf, offset, len, flags, destAddr);
} catch (PosixException | RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public int recv(Object receiver_, int sockfd, byte[] buf, int offset, int len, int flags) throws PosixException {
try {
return (int) lib.send(receiver_, PosixSupportLibraryGen.RECV, sockfd, buf, offset, len, flags);
} catch (PosixException | RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public RecvfromResult recvfrom(Object receiver_, int sockfd, byte[] buf, int offset, int len, int flags) throws PosixException {
try {
return (RecvfromResult) lib.send(receiver_, PosixSupportLibraryGen.RECVFROM, sockfd, buf, offset, len, flags);
} catch (PosixException | RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public void shutdown(Object receiver_, int sockfd, int how) throws PosixException {
try {
lib.send(receiver_, PosixSupportLibraryGen.SHUTDOWN, sockfd, how);
return;
} catch (PosixException | RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public int getsockopt(Object receiver_, int sockfd, int level, int optname, byte[] optval, int optlen) throws PosixException {
try {
return (int) lib.send(receiver_, PosixSupportLibraryGen.GETSOCKOPT, sockfd, level, optname, optval, optlen);
} catch (PosixException | RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public void setsockopt(Object receiver_, int sockfd, int level, int optname, byte[] optval, int optlen) throws PosixException {
try {
lib.send(receiver_, PosixSupportLibraryGen.SETSOCKOPT, sockfd, level, optname, optval, optlen);
return;
} catch (PosixException | RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public int inet_addr(Object receiver_, Object src) {
try {
return (int) lib.send(receiver_, PosixSupportLibraryGen.INET_ADDR, src);
} catch (RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public int inet_aton(Object receiver_, Object src) throws InvalidAddressException {
try {
return (int) lib.send(receiver_, PosixSupportLibraryGen.INET_ATON, src);
} catch (InvalidAddressException | RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public Object inet_ntoa(Object receiver_, int address) {
try {
return lib.send(receiver_, PosixSupportLibraryGen.INET_NTOA, address);
} catch (RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public byte[] inet_pton(Object receiver_, int family, Object src) throws PosixException, InvalidAddressException {
try {
return (byte[]) lib.send(receiver_, PosixSupportLibraryGen.INET_PTON, family, src);
} catch (PosixException | InvalidAddressException | RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public Object inet_ntop(Object receiver_, int family, byte[] src) throws PosixException {
try {
return lib.send(receiver_, PosixSupportLibraryGen.INET_NTOP, family, src);
} catch (PosixException | RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public Object gethostname(Object receiver_) throws PosixException {
try {
return lib.send(receiver_, PosixSupportLibraryGen.GETHOSTNAME);
} catch (PosixException | RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public Object[] getnameinfo(Object receiver_, UniversalSockAddr addr, int flags) throws GetAddrInfoException {
try {
return (Object[]) lib.send(receiver_, PosixSupportLibraryGen.GETNAMEINFO, addr, flags);
} catch (GetAddrInfoException | RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public AddrInfoCursor getaddrinfo(Object receiver_, Object node, Object service, int family, int sockType, int protocol, int flags) throws GetAddrInfoException {
try {
return (AddrInfoCursor) lib.send(receiver_, PosixSupportLibraryGen.GETADDRINFO, node, service, family, sockType, protocol, flags);
} catch (GetAddrInfoException | RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public TruffleString crypt(Object receiver_, TruffleString word, TruffleString salt) throws PosixException {
try {
return (TruffleString) lib.send(receiver_, PosixSupportLibraryGen.CRYPT, word, salt);
} catch (PosixException | RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public UniversalSockAddr createUniversalSockAddr(Object receiver_, FamilySpecificSockAddr src) {
try {
return (UniversalSockAddr) lib.send(receiver_, PosixSupportLibraryGen.CREATE_UNIVERSAL_SOCK_ADDR, src);
} catch (RuntimeException e_) {
throw e_;
} catch (Exception e_) {
throw CompilerDirectives.shouldNotReachHere(e_);
}
}
@Override
public boolean accepts(Object receiver_) {
return lib.accepts(receiver_);
}
}
@GeneratedBy(PosixSupportLibrary.class)
private static final class Delegate extends PosixSupportLibrary {
@Child private PosixSupportLibrary delegateLibrary;
Delegate(PosixSupportLibrary delegateLibrary) {
this.delegateLibrary = delegateLibrary;
}
@Override
public TruffleString getBackend(Object receiver_) {
if (LibraryFactory.isDelegated(delegateLibrary, 0)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
return LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).getBackend(delegate);
} else {
return this.delegateLibrary.getBackend(receiver_);
}
}
@Override
public TruffleString strerror(Object receiver_, int errorCode) {
if (LibraryFactory.isDelegated(delegateLibrary, 1)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
return LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).strerror(delegate, errorCode);
} else {
return this.delegateLibrary.strerror(receiver_, errorCode);
}
}
@Override
public long getpid(Object receiver_) {
if (LibraryFactory.isDelegated(delegateLibrary, 2)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
return LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).getpid(delegate);
} else {
return this.delegateLibrary.getpid(receiver_);
}
}
@Override
public int umask(Object receiver_, int mask) throws PosixException {
if (LibraryFactory.isDelegated(delegateLibrary, 3)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
return LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).umask(delegate, mask);
} else {
return this.delegateLibrary.umask(receiver_, mask);
}
}
@Override
public int openat(Object receiver_, int dirFd, Object pathname, int flags, int mode) throws PosixException {
if (LibraryFactory.isDelegated(delegateLibrary, 4)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
return LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).openat(delegate, dirFd, pathname, flags, mode);
} else {
return this.delegateLibrary.openat(receiver_, dirFd, pathname, flags, mode);
}
}
@Override
public int close(Object receiver_, int fd) throws PosixException {
if (LibraryFactory.isDelegated(delegateLibrary, 5)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
return LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).close(delegate, fd);
} else {
return this.delegateLibrary.close(receiver_, fd);
}
}
@Override
public Buffer read(Object receiver_, int fd, long length) throws PosixException {
if (LibraryFactory.isDelegated(delegateLibrary, 6)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
return LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).read(delegate, fd, length);
} else {
return this.delegateLibrary.read(receiver_, fd, length);
}
}
@Override
public long write(Object receiver_, int fd, Buffer data) throws PosixException {
if (LibraryFactory.isDelegated(delegateLibrary, 7)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
return LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).write(delegate, fd, data);
} else {
return this.delegateLibrary.write(receiver_, fd, data);
}
}
@Override
public int dup(Object receiver_, int fd) throws PosixException {
if (LibraryFactory.isDelegated(delegateLibrary, 8)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
return LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).dup(delegate, fd);
} else {
return this.delegateLibrary.dup(receiver_, fd);
}
}
@Override
public int dup2(Object receiver_, int fd, int fd2, boolean inheritable) throws PosixException {
if (LibraryFactory.isDelegated(delegateLibrary, 9)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
return LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).dup2(delegate, fd, fd2, inheritable);
} else {
return this.delegateLibrary.dup2(receiver_, fd, fd2, inheritable);
}
}
@Override
public boolean getInheritable(Object receiver_, int fd) throws PosixException {
if (LibraryFactory.isDelegated(delegateLibrary, 10)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
return LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).getInheritable(delegate, fd);
} else {
return this.delegateLibrary.getInheritable(receiver_, fd);
}
}
@Override
public void setInheritable(Object receiver_, int fd, boolean inheritable) throws PosixException {
if (LibraryFactory.isDelegated(delegateLibrary, 11)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).setInheritable(delegate, fd, inheritable);
return;
} else {
this.delegateLibrary.setInheritable(receiver_, fd, inheritable);
return;
}
}
@Override
public int[] pipe(Object receiver_) throws PosixException {
if (LibraryFactory.isDelegated(delegateLibrary, 12)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
return LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).pipe(delegate);
} else {
return this.delegateLibrary.pipe(receiver_);
}
}
@Override
public SelectResult select(Object receiver_, int[] readfds, int[] writefds, int[] errorfds, Timeval timeout) throws PosixException {
if (LibraryFactory.isDelegated(delegateLibrary, 13)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
return LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).select(delegate, readfds, writefds, errorfds, timeout);
} else {
return this.delegateLibrary.select(receiver_, readfds, writefds, errorfds, timeout);
}
}
@Override
public long lseek(Object receiver_, int fd, long offset, int how) throws PosixException {
if (LibraryFactory.isDelegated(delegateLibrary, 14)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
return LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).lseek(delegate, fd, offset, how);
} else {
return this.delegateLibrary.lseek(receiver_, fd, offset, how);
}
}
@Override
public void ftruncate(Object receiver_, int fd, long length) throws PosixException {
if (LibraryFactory.isDelegated(delegateLibrary, 15)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).ftruncate(delegate, fd, length);
return;
} else {
this.delegateLibrary.ftruncate(receiver_, fd, length);
return;
}
}
@Override
public void fsync(Object receiver_, int fd) throws PosixException {
if (LibraryFactory.isDelegated(delegateLibrary, 16)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).fsync(delegate, fd);
return;
} else {
this.delegateLibrary.fsync(receiver_, fd);
return;
}
}
@Override
public void flock(Object receiver_, int fd, int operation) throws PosixException {
if (LibraryFactory.isDelegated(delegateLibrary, 17)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).flock(delegate, fd, operation);
return;
} else {
this.delegateLibrary.flock(receiver_, fd, operation);
return;
}
}
@Override
public boolean getBlocking(Object receiver_, int fd) throws PosixException {
if (LibraryFactory.isDelegated(delegateLibrary, 18)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
return LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).getBlocking(delegate, fd);
} else {
return this.delegateLibrary.getBlocking(receiver_, fd);
}
}
@Override
public void setBlocking(Object receiver_, int fd, boolean blocking) throws PosixException {
if (LibraryFactory.isDelegated(delegateLibrary, 19)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).setBlocking(delegate, fd, blocking);
return;
} else {
this.delegateLibrary.setBlocking(receiver_, fd, blocking);
return;
}
}
@Override
public int[] getTerminalSize(Object receiver_, int fd) throws PosixException {
if (LibraryFactory.isDelegated(delegateLibrary, 20)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
return LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).getTerminalSize(delegate, fd);
} else {
return this.delegateLibrary.getTerminalSize(receiver_, fd);
}
}
@Override
public long[] fstatat(Object receiver_, int dirFd, Object pathname, boolean followSymlinks) throws PosixException {
if (LibraryFactory.isDelegated(delegateLibrary, 21)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
return LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).fstatat(delegate, dirFd, pathname, followSymlinks);
} else {
return this.delegateLibrary.fstatat(receiver_, dirFd, pathname, followSymlinks);
}
}
@Override
public long[] fstat(Object receiver_, int fd) throws PosixException {
if (LibraryFactory.isDelegated(delegateLibrary, 22)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
return LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).fstat(delegate, fd);
} else {
return this.delegateLibrary.fstat(receiver_, fd);
}
}
@Override
public long[] statvfs(Object receiver_, Object path) throws PosixException {
if (LibraryFactory.isDelegated(delegateLibrary, 23)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
return LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).statvfs(delegate, path);
} else {
return this.delegateLibrary.statvfs(receiver_, path);
}
}
@Override
public long[] fstatvfs(Object receiver_, int fd) throws PosixException {
if (LibraryFactory.isDelegated(delegateLibrary, 24)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
return LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).fstatvfs(delegate, fd);
} else {
return this.delegateLibrary.fstatvfs(receiver_, fd);
}
}
@Override
public Object[] uname(Object receiver_) throws PosixException {
if (LibraryFactory.isDelegated(delegateLibrary, 25)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
return LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).uname(delegate);
} else {
return this.delegateLibrary.uname(receiver_);
}
}
@Override
public void unlinkat(Object receiver_, int dirFd, Object pathname, boolean rmdir) throws PosixException {
if (LibraryFactory.isDelegated(delegateLibrary, 26)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).unlinkat(delegate, dirFd, pathname, rmdir);
return;
} else {
this.delegateLibrary.unlinkat(receiver_, dirFd, pathname, rmdir);
return;
}
}
@Override
public void linkat(Object receiver_, int oldFdDir, Object oldPath, int newFdDir, Object newPath, int flags) throws PosixException {
if (LibraryFactory.isDelegated(delegateLibrary, 27)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).linkat(delegate, oldFdDir, oldPath, newFdDir, newPath, flags);
return;
} else {
this.delegateLibrary.linkat(receiver_, oldFdDir, oldPath, newFdDir, newPath, flags);
return;
}
}
@Override
public void symlinkat(Object receiver_, Object target, int linkpathDirFd, Object linkpath) throws PosixException {
if (LibraryFactory.isDelegated(delegateLibrary, 28)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).symlinkat(delegate, target, linkpathDirFd, linkpath);
return;
} else {
this.delegateLibrary.symlinkat(receiver_, target, linkpathDirFd, linkpath);
return;
}
}
@Override
public void mkdirat(Object receiver_, int dirFd, Object pathname, int mode) throws PosixException {
if (LibraryFactory.isDelegated(delegateLibrary, 29)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).mkdirat(delegate, dirFd, pathname, mode);
return;
} else {
this.delegateLibrary.mkdirat(receiver_, dirFd, pathname, mode);
return;
}
}
@Override
public Object getcwd(Object receiver_) throws PosixException {
if (LibraryFactory.isDelegated(delegateLibrary, 30)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
return LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).getcwd(delegate);
} else {
return this.delegateLibrary.getcwd(receiver_);
}
}
@Override
public void chdir(Object receiver_, Object path) throws PosixException {
if (LibraryFactory.isDelegated(delegateLibrary, 31)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).chdir(delegate, path);
return;
} else {
this.delegateLibrary.chdir(receiver_, path);
return;
}
}
@Override
public void fchdir(Object receiver_, int fd) throws PosixException {
if (LibraryFactory.isDelegated(delegateLibrary, 32)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).fchdir(delegate, fd);
return;
} else {
this.delegateLibrary.fchdir(receiver_, fd);
return;
}
}
@Override
public boolean isatty(Object receiver_, int fd) {
if (LibraryFactory.isDelegated(delegateLibrary, 33)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
return LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).isatty(delegate, fd);
} else {
return this.delegateLibrary.isatty(receiver_, fd);
}
}
@Override
public Object opendir(Object receiver_, Object path) throws PosixException {
if (LibraryFactory.isDelegated(delegateLibrary, 34)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
return LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).opendir(delegate, path);
} else {
return this.delegateLibrary.opendir(receiver_, path);
}
}
@Override
public Object fdopendir(Object receiver_, int fd) throws PosixException {
if (LibraryFactory.isDelegated(delegateLibrary, 35)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
return LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).fdopendir(delegate, fd);
} else {
return this.delegateLibrary.fdopendir(receiver_, fd);
}
}
@Override
public void closedir(Object receiver_, Object dirStream) throws PosixException {
if (LibraryFactory.isDelegated(delegateLibrary, 36)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).closedir(delegate, dirStream);
return;
} else {
this.delegateLibrary.closedir(receiver_, dirStream);
return;
}
}
@Override
public Object readdir(Object receiver_, Object dirStream) throws PosixException {
if (LibraryFactory.isDelegated(delegateLibrary, 37)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
return LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).readdir(delegate, dirStream);
} else {
return this.delegateLibrary.readdir(receiver_, dirStream);
}
}
@Override
public void rewinddir(Object receiver_, Object dirStream) {
if (LibraryFactory.isDelegated(delegateLibrary, 38)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).rewinddir(delegate, dirStream);
return;
} else {
this.delegateLibrary.rewinddir(receiver_, dirStream);
return;
}
}
@Override
public Object dirEntryGetName(Object receiver_, Object dirEntry) throws PosixException {
if (LibraryFactory.isDelegated(delegateLibrary, 39)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
return LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).dirEntryGetName(delegate, dirEntry);
} else {
return this.delegateLibrary.dirEntryGetName(receiver_, dirEntry);
}
}
@Override
public Object dirEntryGetPath(Object receiver_, Object dirEntry, Object scandirPath) throws PosixException {
if (LibraryFactory.isDelegated(delegateLibrary, 40)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
return LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).dirEntryGetPath(delegate, dirEntry, scandirPath);
} else {
return this.delegateLibrary.dirEntryGetPath(receiver_, dirEntry, scandirPath);
}
}
@Override
public long dirEntryGetInode(Object receiver_, Object dirEntry) throws PosixException {
if (LibraryFactory.isDelegated(delegateLibrary, 41)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
return LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).dirEntryGetInode(delegate, dirEntry);
} else {
return this.delegateLibrary.dirEntryGetInode(receiver_, dirEntry);
}
}
@Override
public int dirEntryGetType(Object receiver_, Object dirEntry) {
if (LibraryFactory.isDelegated(delegateLibrary, 42)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
return LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).dirEntryGetType(delegate, dirEntry);
} else {
return this.delegateLibrary.dirEntryGetType(receiver_, dirEntry);
}
}
@Override
public void utimensat(Object receiver_, int dirFd, Object pathname, long[] timespec, boolean followSymlinks) throws PosixException {
if (LibraryFactory.isDelegated(delegateLibrary, 43)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).utimensat(delegate, dirFd, pathname, timespec, followSymlinks);
return;
} else {
this.delegateLibrary.utimensat(receiver_, dirFd, pathname, timespec, followSymlinks);
return;
}
}
@Override
public void futimens(Object receiver_, int fd, long[] timespec) throws PosixException {
if (LibraryFactory.isDelegated(delegateLibrary, 44)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).futimens(delegate, fd, timespec);
return;
} else {
this.delegateLibrary.futimens(receiver_, fd, timespec);
return;
}
}
@Override
public void futimes(Object receiver_, int fd, Timeval[] timeval) throws PosixException {
if (LibraryFactory.isDelegated(delegateLibrary, 45)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).futimes(delegate, fd, timeval);
return;
} else {
this.delegateLibrary.futimes(receiver_, fd, timeval);
return;
}
}
@Override
public void lutimes(Object receiver_, Object filename, Timeval[] timeval) throws PosixException {
if (LibraryFactory.isDelegated(delegateLibrary, 46)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).lutimes(delegate, filename, timeval);
return;
} else {
this.delegateLibrary.lutimes(receiver_, filename, timeval);
return;
}
}
@Override
public void utimes(Object receiver_, Object filename, Timeval[] timeval) throws PosixException {
if (LibraryFactory.isDelegated(delegateLibrary, 47)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).utimes(delegate, filename, timeval);
return;
} else {
this.delegateLibrary.utimes(receiver_, filename, timeval);
return;
}
}
@Override
public void renameat(Object receiver_, int oldDirFd, Object oldPath, int newDirFd, Object newPath) throws PosixException {
if (LibraryFactory.isDelegated(delegateLibrary, 48)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).renameat(delegate, oldDirFd, oldPath, newDirFd, newPath);
return;
} else {
this.delegateLibrary.renameat(receiver_, oldDirFd, oldPath, newDirFd, newPath);
return;
}
}
@Override
public boolean faccessat(Object receiver_, int dirFd, Object path, int mode, boolean effectiveIds, boolean followSymlinks) {
if (LibraryFactory.isDelegated(delegateLibrary, 49)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
return LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).faccessat(delegate, dirFd, path, mode, effectiveIds, followSymlinks);
} else {
return this.delegateLibrary.faccessat(receiver_, dirFd, path, mode, effectiveIds, followSymlinks);
}
}
@Override
public void fchmodat(Object receiver_, int dirFd, Object path, int mode, boolean followSymlinks) throws PosixException {
if (LibraryFactory.isDelegated(delegateLibrary, 50)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).fchmodat(delegate, dirFd, path, mode, followSymlinks);
return;
} else {
this.delegateLibrary.fchmodat(receiver_, dirFd, path, mode, followSymlinks);
return;
}
}
@Override
public void fchmod(Object receiver_, int fd, int mode) throws PosixException {
if (LibraryFactory.isDelegated(delegateLibrary, 51)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).fchmod(delegate, fd, mode);
return;
} else {
this.delegateLibrary.fchmod(receiver_, fd, mode);
return;
}
}
@Override
public Object readlinkat(Object receiver_, int dirFd, Object path) throws PosixException {
if (LibraryFactory.isDelegated(delegateLibrary, 52)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
return LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).readlinkat(delegate, dirFd, path);
} else {
return this.delegateLibrary.readlinkat(receiver_, dirFd, path);
}
}
@Override
public void kill(Object receiver_, long pid, int signal) throws PosixException {
if (LibraryFactory.isDelegated(delegateLibrary, 53)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).kill(delegate, pid, signal);
return;
} else {
this.delegateLibrary.kill(receiver_, pid, signal);
return;
}
}
@Override
public void killpg(Object receiver_, long pid, int signal) throws PosixException {
if (LibraryFactory.isDelegated(delegateLibrary, 54)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).killpg(delegate, pid, signal);
return;
} else {
this.delegateLibrary.killpg(receiver_, pid, signal);
return;
}
}
@Override
public long[] waitpid(Object receiver_, long pid, int options) throws PosixException {
if (LibraryFactory.isDelegated(delegateLibrary, 55)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
return LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).waitpid(delegate, pid, options);
} else {
return this.delegateLibrary.waitpid(receiver_, pid, options);
}
}
@Override
public void abort(Object receiver_) {
if (LibraryFactory.isDelegated(delegateLibrary, 56)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).abort(delegate);
return;
} else {
this.delegateLibrary.abort(receiver_);
return;
}
}
@Override
public boolean wcoredump(Object receiver_, int status) {
if (LibraryFactory.isDelegated(delegateLibrary, 57)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
return LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).wcoredump(delegate, status);
} else {
return this.delegateLibrary.wcoredump(receiver_, status);
}
}
@Override
public boolean wifcontinued(Object receiver_, int status) {
if (LibraryFactory.isDelegated(delegateLibrary, 58)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
return LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).wifcontinued(delegate, status);
} else {
return this.delegateLibrary.wifcontinued(receiver_, status);
}
}
@Override
public boolean wifstopped(Object receiver_, int status) {
if (LibraryFactory.isDelegated(delegateLibrary, 59)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
return LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).wifstopped(delegate, status);
} else {
return this.delegateLibrary.wifstopped(receiver_, status);
}
}
@Override
public boolean wifsignaled(Object receiver_, int status) {
if (LibraryFactory.isDelegated(delegateLibrary, 60)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
return LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).wifsignaled(delegate, status);
} else {
return this.delegateLibrary.wifsignaled(receiver_, status);
}
}
@Override
public boolean wifexited(Object receiver_, int status) {
if (LibraryFactory.isDelegated(delegateLibrary, 61)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
return LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).wifexited(delegate, status);
} else {
return this.delegateLibrary.wifexited(receiver_, status);
}
}
@Override
public int wexitstatus(Object receiver_, int status) {
if (LibraryFactory.isDelegated(delegateLibrary, 62)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
return LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).wexitstatus(delegate, status);
} else {
return this.delegateLibrary.wexitstatus(receiver_, status);
}
}
@Override
public int wtermsig(Object receiver_, int status) {
if (LibraryFactory.isDelegated(delegateLibrary, 63)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
return LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).wtermsig(delegate, status);
} else {
return this.delegateLibrary.wtermsig(receiver_, status);
}
}
@Override
public int wstopsig(Object receiver_, int status) {
if (LibraryFactory.isDelegated(delegateLibrary, 64)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
return LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).wstopsig(delegate, status);
} else {
return this.delegateLibrary.wstopsig(receiver_, status);
}
}
@Override
public long getuid(Object receiver_) {
if (LibraryFactory.isDelegated(delegateLibrary, 65)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
return LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).getuid(delegate);
} else {
return this.delegateLibrary.getuid(receiver_);
}
}
@Override
public long geteuid(Object receiver_) {
if (LibraryFactory.isDelegated(delegateLibrary, 66)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
return LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).geteuid(delegate);
} else {
return this.delegateLibrary.geteuid(receiver_);
}
}
@Override
public long getgid(Object receiver_) {
if (LibraryFactory.isDelegated(delegateLibrary, 67)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
return LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).getgid(delegate);
} else {
return this.delegateLibrary.getgid(receiver_);
}
}
@Override
public long getppid(Object receiver_) {
if (LibraryFactory.isDelegated(delegateLibrary, 68)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
return LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).getppid(delegate);
} else {
return this.delegateLibrary.getppid(receiver_);
}
}
@Override
public long getpgid(Object receiver_, long pid) throws PosixException {
if (LibraryFactory.isDelegated(delegateLibrary, 69)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
return LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).getpgid(delegate, pid);
} else {
return this.delegateLibrary.getpgid(receiver_, pid);
}
}
@Override
public void setpgid(Object receiver_, long pid, long pgid) throws PosixException {
if (LibraryFactory.isDelegated(delegateLibrary, 70)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).setpgid(delegate, pid, pgid);
return;
} else {
this.delegateLibrary.setpgid(receiver_, pid, pgid);
return;
}
}
@Override
public long getpgrp(Object receiver_) {
if (LibraryFactory.isDelegated(delegateLibrary, 71)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
return LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).getpgrp(delegate);
} else {
return this.delegateLibrary.getpgrp(receiver_);
}
}
@Override
public long getsid(Object receiver_, long pid) throws PosixException {
if (LibraryFactory.isDelegated(delegateLibrary, 72)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
return LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).getsid(delegate, pid);
} else {
return this.delegateLibrary.getsid(receiver_, pid);
}
}
@Override
public long setsid(Object receiver_) throws PosixException {
if (LibraryFactory.isDelegated(delegateLibrary, 73)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
return LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).setsid(delegate);
} else {
return this.delegateLibrary.setsid(receiver_);
}
}
@Override
public OpenPtyResult openpty(Object receiver_) throws PosixException {
if (LibraryFactory.isDelegated(delegateLibrary, 74)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
return LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).openpty(delegate);
} else {
return this.delegateLibrary.openpty(receiver_);
}
}
@Override
public TruffleString ctermid(Object receiver_) throws PosixException {
if (LibraryFactory.isDelegated(delegateLibrary, 75)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
return LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).ctermid(delegate);
} else {
return this.delegateLibrary.ctermid(receiver_);
}
}
@Override
public void setenv(Object receiver_, Object name, Object value, boolean overwrite) throws PosixException {
if (LibraryFactory.isDelegated(delegateLibrary, 76)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).setenv(delegate, name, value, overwrite);
return;
} else {
this.delegateLibrary.setenv(receiver_, name, value, overwrite);
return;
}
}
@Override
public void unsetenv(Object receiver_, Object name) throws PosixException {
if (LibraryFactory.isDelegated(delegateLibrary, 77)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).unsetenv(delegate, name);
return;
} else {
this.delegateLibrary.unsetenv(receiver_, name);
return;
}
}
@Override
public int forkExec(Object receiver_, Object[] executables, Object[] args, Object cwd, Object[] env, int stdinReadFd, int stdinWriteFd, int stdoutReadFd, int stdoutWriteFd, int stderrReadFd, int stderrWriteFd, int errPipeReadFd, int errPipeWriteFd, boolean closeFds, boolean restoreSignals, boolean callSetsid, int[] fdsToKeep) throws PosixException {
if (LibraryFactory.isDelegated(delegateLibrary, 78)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
return LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).forkExec(delegate, executables, args, cwd, env, stdinReadFd, stdinWriteFd, stdoutReadFd, stdoutWriteFd, stderrReadFd, stderrWriteFd, errPipeReadFd, errPipeWriteFd, closeFds, restoreSignals, callSetsid, fdsToKeep);
} else {
return this.delegateLibrary.forkExec(receiver_, executables, args, cwd, env, stdinReadFd, stdinWriteFd, stdoutReadFd, stdoutWriteFd, stderrReadFd, stderrWriteFd, errPipeReadFd, errPipeWriteFd, closeFds, restoreSignals, callSetsid, fdsToKeep);
}
}
@Override
public void execv(Object receiver_, Object pathname, Object[] args) throws PosixException {
if (LibraryFactory.isDelegated(delegateLibrary, 79)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).execv(delegate, pathname, args);
return;
} else {
this.delegateLibrary.execv(receiver_, pathname, args);
return;
}
}
@Override
public int system(Object receiver_, Object command) {
if (LibraryFactory.isDelegated(delegateLibrary, 80)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
return LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).system(delegate, command);
} else {
return this.delegateLibrary.system(receiver_, command);
}
}
@Override
public Object mmap(Object receiver_, long length, int prot, int flags, int fd, long offset) throws PosixException {
if (LibraryFactory.isDelegated(delegateLibrary, 81)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
return LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).mmap(delegate, length, prot, flags, fd, offset);
} else {
return this.delegateLibrary.mmap(receiver_, length, prot, flags, fd, offset);
}
}
@Override
public byte mmapReadByte(Object receiver_, Object mmap, long index) throws PosixException {
if (LibraryFactory.isDelegated(delegateLibrary, 82)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
return LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).mmapReadByte(delegate, mmap, index);
} else {
return this.delegateLibrary.mmapReadByte(receiver_, mmap, index);
}
}
@Override
public void mmapWriteByte(Object receiver_, Object mmap, long index, byte value) throws PosixException {
if (LibraryFactory.isDelegated(delegateLibrary, 83)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).mmapWriteByte(delegate, mmap, index, value);
return;
} else {
this.delegateLibrary.mmapWriteByte(receiver_, mmap, index, value);
return;
}
}
@Override
public int mmapReadBytes(Object receiver_, Object mmap, long index, byte[] bytes, int length) throws PosixException {
if (LibraryFactory.isDelegated(delegateLibrary, 84)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
return LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).mmapReadBytes(delegate, mmap, index, bytes, length);
} else {
return this.delegateLibrary.mmapReadBytes(receiver_, mmap, index, bytes, length);
}
}
@Override
public void mmapWriteBytes(Object receiver_, Object mmap, long index, byte[] bytes, int length) throws PosixException {
if (LibraryFactory.isDelegated(delegateLibrary, 85)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).mmapWriteBytes(delegate, mmap, index, bytes, length);
return;
} else {
this.delegateLibrary.mmapWriteBytes(receiver_, mmap, index, bytes, length);
return;
}
}
@Override
public void mmapFlush(Object receiver_, Object mmap, long offset, long length) throws PosixException {
if (LibraryFactory.isDelegated(delegateLibrary, 86)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).mmapFlush(delegate, mmap, offset, length);
return;
} else {
this.delegateLibrary.mmapFlush(receiver_, mmap, offset, length);
return;
}
}
@Override
public void mmapUnmap(Object receiver_, Object mmap, long length) throws PosixException {
if (LibraryFactory.isDelegated(delegateLibrary, 87)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).mmapUnmap(delegate, mmap, length);
return;
} else {
this.delegateLibrary.mmapUnmap(receiver_, mmap, length);
return;
}
}
@Override
public long mmapGetPointer(Object receiver_, Object mmap) {
if (LibraryFactory.isDelegated(delegateLibrary, 88)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
return LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).mmapGetPointer(delegate, mmap);
} else {
return this.delegateLibrary.mmapGetPointer(receiver_, mmap);
}
}
@Override
public PwdResult getpwuid(Object receiver_, long uid) throws PosixException {
if (LibraryFactory.isDelegated(delegateLibrary, 89)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
return LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).getpwuid(delegate, uid);
} else {
return this.delegateLibrary.getpwuid(receiver_, uid);
}
}
@Override
public PwdResult getpwnam(Object receiver_, Object name) throws PosixException {
if (LibraryFactory.isDelegated(delegateLibrary, 90)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
return LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).getpwnam(delegate, name);
} else {
return this.delegateLibrary.getpwnam(receiver_, name);
}
}
@Override
public boolean hasGetpwentries(Object receiver_) {
if (LibraryFactory.isDelegated(delegateLibrary, 91)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
return LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).hasGetpwentries(delegate);
} else {
return this.delegateLibrary.hasGetpwentries(receiver_);
}
}
@Override
public PwdResult[] getpwentries(Object receiver_) throws PosixException {
if (LibraryFactory.isDelegated(delegateLibrary, 92)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
return LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).getpwentries(delegate);
} else {
return this.delegateLibrary.getpwentries(receiver_);
}
}
@Override
public Object createPathFromString(Object receiver_, TruffleString path) {
if (LibraryFactory.isDelegated(delegateLibrary, 93)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
return LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).createPathFromString(delegate, path);
} else {
return this.delegateLibrary.createPathFromString(receiver_, path);
}
}
@Override
public Object createPathFromBytes(Object receiver_, byte[] path) {
if (LibraryFactory.isDelegated(delegateLibrary, 94)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
return LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).createPathFromBytes(delegate, path);
} else {
return this.delegateLibrary.createPathFromBytes(receiver_, path);
}
}
@Override
public TruffleString getPathAsString(Object receiver_, Object path) {
if (LibraryFactory.isDelegated(delegateLibrary, 95)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
return LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).getPathAsString(delegate, path);
} else {
return this.delegateLibrary.getPathAsString(receiver_, path);
}
}
@Override
public Buffer getPathAsBytes(Object receiver_, Object path) {
if (LibraryFactory.isDelegated(delegateLibrary, 96)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
return LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).getPathAsBytes(delegate, path);
} else {
return this.delegateLibrary.getPathAsBytes(receiver_, path);
}
}
@Override
public int socket(Object receiver_, int domain, int type, int protocol) throws PosixException {
if (LibraryFactory.isDelegated(delegateLibrary, 97)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
return LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).socket(delegate, domain, type, protocol);
} else {
return this.delegateLibrary.socket(receiver_, domain, type, protocol);
}
}
@Override
public AcceptResult accept(Object receiver_, int sockfd) throws PosixException {
if (LibraryFactory.isDelegated(delegateLibrary, 98)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
return LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).accept(delegate, sockfd);
} else {
return this.delegateLibrary.accept(receiver_, sockfd);
}
}
@Override
public void bind(Object receiver_, int sockfd, UniversalSockAddr addr) throws PosixException {
if (LibraryFactory.isDelegated(delegateLibrary, 99)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).bind(delegate, sockfd, addr);
return;
} else {
this.delegateLibrary.bind(receiver_, sockfd, addr);
return;
}
}
@Override
public void connect(Object receiver_, int sockfd, UniversalSockAddr addr) throws PosixException {
if (LibraryFactory.isDelegated(delegateLibrary, 100)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).connect(delegate, sockfd, addr);
return;
} else {
this.delegateLibrary.connect(receiver_, sockfd, addr);
return;
}
}
@Override
public void listen(Object receiver_, int sockfd, int backlog) throws PosixException {
if (LibraryFactory.isDelegated(delegateLibrary, 101)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).listen(delegate, sockfd, backlog);
return;
} else {
this.delegateLibrary.listen(receiver_, sockfd, backlog);
return;
}
}
@Override
public UniversalSockAddr getpeername(Object receiver_, int sockfd) throws PosixException {
if (LibraryFactory.isDelegated(delegateLibrary, 102)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
return LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).getpeername(delegate, sockfd);
} else {
return this.delegateLibrary.getpeername(receiver_, sockfd);
}
}
@Override
public UniversalSockAddr getsockname(Object receiver_, int sockfd) throws PosixException {
if (LibraryFactory.isDelegated(delegateLibrary, 103)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
return LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).getsockname(delegate, sockfd);
} else {
return this.delegateLibrary.getsockname(receiver_, sockfd);
}
}
@Override
public int send(Object receiver_, int sockfd, byte[] buf, int offset, int len, int flags) throws PosixException {
if (LibraryFactory.isDelegated(delegateLibrary, 104)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
return LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).send(delegate, sockfd, buf, offset, len, flags);
} else {
return this.delegateLibrary.send(receiver_, sockfd, buf, offset, len, flags);
}
}
@Override
public int sendto(Object receiver_, int sockfd, byte[] buf, int offset, int len, int flags, UniversalSockAddr destAddr) throws PosixException {
if (LibraryFactory.isDelegated(delegateLibrary, 105)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
return LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).sendto(delegate, sockfd, buf, offset, len, flags, destAddr);
} else {
return this.delegateLibrary.sendto(receiver_, sockfd, buf, offset, len, flags, destAddr);
}
}
@Override
public int recv(Object receiver_, int sockfd, byte[] buf, int offset, int len, int flags) throws PosixException {
if (LibraryFactory.isDelegated(delegateLibrary, 106)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
return LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).recv(delegate, sockfd, buf, offset, len, flags);
} else {
return this.delegateLibrary.recv(receiver_, sockfd, buf, offset, len, flags);
}
}
@Override
public RecvfromResult recvfrom(Object receiver_, int sockfd, byte[] buf, int offset, int len, int flags) throws PosixException {
if (LibraryFactory.isDelegated(delegateLibrary, 107)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
return LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).recvfrom(delegate, sockfd, buf, offset, len, flags);
} else {
return this.delegateLibrary.recvfrom(receiver_, sockfd, buf, offset, len, flags);
}
}
@Override
public void shutdown(Object receiver_, int sockfd, int how) throws PosixException {
if (LibraryFactory.isDelegated(delegateLibrary, 108)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).shutdown(delegate, sockfd, how);
return;
} else {
this.delegateLibrary.shutdown(receiver_, sockfd, how);
return;
}
}
@Override
public int getsockopt(Object receiver_, int sockfd, int level, int optname, byte[] optval, int optlen) throws PosixException {
if (LibraryFactory.isDelegated(delegateLibrary, 109)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
return LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).getsockopt(delegate, sockfd, level, optname, optval, optlen);
} else {
return this.delegateLibrary.getsockopt(receiver_, sockfd, level, optname, optval, optlen);
}
}
@Override
public void setsockopt(Object receiver_, int sockfd, int level, int optname, byte[] optval, int optlen) throws PosixException {
if (LibraryFactory.isDelegated(delegateLibrary, 110)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).setsockopt(delegate, sockfd, level, optname, optval, optlen);
return;
} else {
this.delegateLibrary.setsockopt(receiver_, sockfd, level, optname, optval, optlen);
return;
}
}
@Override
public int inet_addr(Object receiver_, Object src) {
if (LibraryFactory.isDelegated(delegateLibrary, 111)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
return LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).inet_addr(delegate, src);
} else {
return this.delegateLibrary.inet_addr(receiver_, src);
}
}
@Override
public int inet_aton(Object receiver_, Object src) throws InvalidAddressException {
if (LibraryFactory.isDelegated(delegateLibrary, 112)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
return LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).inet_aton(delegate, src);
} else {
return this.delegateLibrary.inet_aton(receiver_, src);
}
}
@Override
public Object inet_ntoa(Object receiver_, int address) {
if (LibraryFactory.isDelegated(delegateLibrary, 113)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
return LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).inet_ntoa(delegate, address);
} else {
return this.delegateLibrary.inet_ntoa(receiver_, address);
}
}
@Override
public byte[] inet_pton(Object receiver_, int family, Object src) throws PosixException, InvalidAddressException {
if (LibraryFactory.isDelegated(delegateLibrary, 114)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
return LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).inet_pton(delegate, family, src);
} else {
return this.delegateLibrary.inet_pton(receiver_, family, src);
}
}
@Override
public Object inet_ntop(Object receiver_, int family, byte[] src) throws PosixException {
if (LibraryFactory.isDelegated(delegateLibrary, 115)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
return LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).inet_ntop(delegate, family, src);
} else {
return this.delegateLibrary.inet_ntop(receiver_, family, src);
}
}
@Override
public Object gethostname(Object receiver_) throws PosixException {
if (LibraryFactory.isDelegated(delegateLibrary, 116)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
return LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).gethostname(delegate);
} else {
return this.delegateLibrary.gethostname(receiver_);
}
}
@Override
public Object[] getnameinfo(Object receiver_, UniversalSockAddr addr, int flags) throws GetAddrInfoException {
if (LibraryFactory.isDelegated(delegateLibrary, 117)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
return LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).getnameinfo(delegate, addr, flags);
} else {
return this.delegateLibrary.getnameinfo(receiver_, addr, flags);
}
}
@Override
public AddrInfoCursor getaddrinfo(Object receiver_, Object node, Object service, int family, int sockType, int protocol, int flags) throws GetAddrInfoException {
if (LibraryFactory.isDelegated(delegateLibrary, 118)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
return LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).getaddrinfo(delegate, node, service, family, sockType, protocol, flags);
} else {
return this.delegateLibrary.getaddrinfo(receiver_, node, service, family, sockType, protocol, flags);
}
}
@Override
public TruffleString crypt(Object receiver_, TruffleString word, TruffleString salt) throws PosixException {
if (LibraryFactory.isDelegated(delegateLibrary, 119)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
return LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).crypt(delegate, word, salt);
} else {
return this.delegateLibrary.crypt(receiver_, word, salt);
}
}
@Override
public UniversalSockAddr createUniversalSockAddr(Object receiver_, FamilySpecificSockAddr src) {
if (LibraryFactory.isDelegated(delegateLibrary, 120)) {
Object delegate = LibraryFactory.readDelegate(this.delegateLibrary, receiver_);
return LibraryFactory.getDelegateLibrary(this.delegateLibrary, delegate).createUniversalSockAddr(delegate, src);
} else {
return this.delegateLibrary.createUniversalSockAddr(receiver_, src);
}
}
@Override
public boolean accepts(Object receiver_) {
return delegateLibrary.accepts(receiver_);
}
@Override
public NodeCost getCost() {
return NodeCost.NONE;
}
@Override
public boolean isAdoptable() {
return this.delegateLibrary.isAdoptable();
}
}
@GeneratedBy(PosixSupportLibrary.class)
private static final class CachedToUncachedDispatch extends PosixSupportLibrary {
@Override
public NodeCost getCost() {
return NodeCost.MEGAMORPHIC;
}
@TruffleBoundary
@Override
public TruffleString getBackend(Object receiver_) {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
return INSTANCE.getUncached(receiver_).getBackend(receiver_);
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public TruffleString strerror(Object receiver_, int errorCode) {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
return INSTANCE.getUncached(receiver_).strerror(receiver_, errorCode);
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public long getpid(Object receiver_) {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
return INSTANCE.getUncached(receiver_).getpid(receiver_);
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public int umask(Object receiver_, int mask) throws PosixException {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
return INSTANCE.getUncached(receiver_).umask(receiver_, mask);
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public int openat(Object receiver_, int dirFd, Object pathname, int flags, int mode) throws PosixException {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
return INSTANCE.getUncached(receiver_).openat(receiver_, dirFd, pathname, flags, mode);
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public int close(Object receiver_, int fd) throws PosixException {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
return INSTANCE.getUncached(receiver_).close(receiver_, fd);
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public Buffer read(Object receiver_, int fd, long length) throws PosixException {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
return INSTANCE.getUncached(receiver_).read(receiver_, fd, length);
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public long write(Object receiver_, int fd, Buffer data) throws PosixException {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
return INSTANCE.getUncached(receiver_).write(receiver_, fd, data);
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public int dup(Object receiver_, int fd) throws PosixException {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
return INSTANCE.getUncached(receiver_).dup(receiver_, fd);
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public int dup2(Object receiver_, int fd, int fd2, boolean inheritable) throws PosixException {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
return INSTANCE.getUncached(receiver_).dup2(receiver_, fd, fd2, inheritable);
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public boolean getInheritable(Object receiver_, int fd) throws PosixException {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
return INSTANCE.getUncached(receiver_).getInheritable(receiver_, fd);
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public void setInheritable(Object receiver_, int fd, boolean inheritable) throws PosixException {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
INSTANCE.getUncached(receiver_).setInheritable(receiver_, fd, inheritable);
return;
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public int[] pipe(Object receiver_) throws PosixException {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
return INSTANCE.getUncached(receiver_).pipe(receiver_);
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public SelectResult select(Object receiver_, int[] readfds, int[] writefds, int[] errorfds, Timeval timeout) throws PosixException {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
return INSTANCE.getUncached(receiver_).select(receiver_, readfds, writefds, errorfds, timeout);
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public long lseek(Object receiver_, int fd, long offset, int how) throws PosixException {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
return INSTANCE.getUncached(receiver_).lseek(receiver_, fd, offset, how);
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public void ftruncate(Object receiver_, int fd, long length) throws PosixException {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
INSTANCE.getUncached(receiver_).ftruncate(receiver_, fd, length);
return;
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public void fsync(Object receiver_, int fd) throws PosixException {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
INSTANCE.getUncached(receiver_).fsync(receiver_, fd);
return;
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public void flock(Object receiver_, int fd, int operation) throws PosixException {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
INSTANCE.getUncached(receiver_).flock(receiver_, fd, operation);
return;
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public boolean getBlocking(Object receiver_, int fd) throws PosixException {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
return INSTANCE.getUncached(receiver_).getBlocking(receiver_, fd);
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public void setBlocking(Object receiver_, int fd, boolean blocking) throws PosixException {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
INSTANCE.getUncached(receiver_).setBlocking(receiver_, fd, blocking);
return;
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public int[] getTerminalSize(Object receiver_, int fd) throws PosixException {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
return INSTANCE.getUncached(receiver_).getTerminalSize(receiver_, fd);
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public long[] fstatat(Object receiver_, int dirFd, Object pathname, boolean followSymlinks) throws PosixException {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
return INSTANCE.getUncached(receiver_).fstatat(receiver_, dirFd, pathname, followSymlinks);
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public long[] fstat(Object receiver_, int fd) throws PosixException {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
return INSTANCE.getUncached(receiver_).fstat(receiver_, fd);
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public long[] statvfs(Object receiver_, Object path) throws PosixException {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
return INSTANCE.getUncached(receiver_).statvfs(receiver_, path);
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public long[] fstatvfs(Object receiver_, int fd) throws PosixException {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
return INSTANCE.getUncached(receiver_).fstatvfs(receiver_, fd);
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public Object[] uname(Object receiver_) throws PosixException {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
return INSTANCE.getUncached(receiver_).uname(receiver_);
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public void unlinkat(Object receiver_, int dirFd, Object pathname, boolean rmdir) throws PosixException {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
INSTANCE.getUncached(receiver_).unlinkat(receiver_, dirFd, pathname, rmdir);
return;
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public void linkat(Object receiver_, int oldFdDir, Object oldPath, int newFdDir, Object newPath, int flags) throws PosixException {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
INSTANCE.getUncached(receiver_).linkat(receiver_, oldFdDir, oldPath, newFdDir, newPath, flags);
return;
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public void symlinkat(Object receiver_, Object target, int linkpathDirFd, Object linkpath) throws PosixException {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
INSTANCE.getUncached(receiver_).symlinkat(receiver_, target, linkpathDirFd, linkpath);
return;
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public void mkdirat(Object receiver_, int dirFd, Object pathname, int mode) throws PosixException {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
INSTANCE.getUncached(receiver_).mkdirat(receiver_, dirFd, pathname, mode);
return;
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public Object getcwd(Object receiver_) throws PosixException {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
return INSTANCE.getUncached(receiver_).getcwd(receiver_);
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public void chdir(Object receiver_, Object path) throws PosixException {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
INSTANCE.getUncached(receiver_).chdir(receiver_, path);
return;
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public void fchdir(Object receiver_, int fd) throws PosixException {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
INSTANCE.getUncached(receiver_).fchdir(receiver_, fd);
return;
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public boolean isatty(Object receiver_, int fd) {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
return INSTANCE.getUncached(receiver_).isatty(receiver_, fd);
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public Object opendir(Object receiver_, Object path) throws PosixException {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
return INSTANCE.getUncached(receiver_).opendir(receiver_, path);
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public Object fdopendir(Object receiver_, int fd) throws PosixException {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
return INSTANCE.getUncached(receiver_).fdopendir(receiver_, fd);
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public void closedir(Object receiver_, Object dirStream) throws PosixException {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
INSTANCE.getUncached(receiver_).closedir(receiver_, dirStream);
return;
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public Object readdir(Object receiver_, Object dirStream) throws PosixException {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
return INSTANCE.getUncached(receiver_).readdir(receiver_, dirStream);
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public void rewinddir(Object receiver_, Object dirStream) {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
INSTANCE.getUncached(receiver_).rewinddir(receiver_, dirStream);
return;
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public Object dirEntryGetName(Object receiver_, Object dirEntry) throws PosixException {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
return INSTANCE.getUncached(receiver_).dirEntryGetName(receiver_, dirEntry);
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public Object dirEntryGetPath(Object receiver_, Object dirEntry, Object scandirPath) throws PosixException {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
return INSTANCE.getUncached(receiver_).dirEntryGetPath(receiver_, dirEntry, scandirPath);
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public long dirEntryGetInode(Object receiver_, Object dirEntry) throws PosixException {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
return INSTANCE.getUncached(receiver_).dirEntryGetInode(receiver_, dirEntry);
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public int dirEntryGetType(Object receiver_, Object dirEntry) {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
return INSTANCE.getUncached(receiver_).dirEntryGetType(receiver_, dirEntry);
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public void utimensat(Object receiver_, int dirFd, Object pathname, long[] timespec, boolean followSymlinks) throws PosixException {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
INSTANCE.getUncached(receiver_).utimensat(receiver_, dirFd, pathname, timespec, followSymlinks);
return;
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public void futimens(Object receiver_, int fd, long[] timespec) throws PosixException {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
INSTANCE.getUncached(receiver_).futimens(receiver_, fd, timespec);
return;
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public void futimes(Object receiver_, int fd, Timeval[] timeval) throws PosixException {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
INSTANCE.getUncached(receiver_).futimes(receiver_, fd, timeval);
return;
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public void lutimes(Object receiver_, Object filename, Timeval[] timeval) throws PosixException {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
INSTANCE.getUncached(receiver_).lutimes(receiver_, filename, timeval);
return;
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public void utimes(Object receiver_, Object filename, Timeval[] timeval) throws PosixException {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
INSTANCE.getUncached(receiver_).utimes(receiver_, filename, timeval);
return;
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public void renameat(Object receiver_, int oldDirFd, Object oldPath, int newDirFd, Object newPath) throws PosixException {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
INSTANCE.getUncached(receiver_).renameat(receiver_, oldDirFd, oldPath, newDirFd, newPath);
return;
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public boolean faccessat(Object receiver_, int dirFd, Object path, int mode, boolean effectiveIds, boolean followSymlinks) {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
return INSTANCE.getUncached(receiver_).faccessat(receiver_, dirFd, path, mode, effectiveIds, followSymlinks);
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public void fchmodat(Object receiver_, int dirFd, Object path, int mode, boolean followSymlinks) throws PosixException {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
INSTANCE.getUncached(receiver_).fchmodat(receiver_, dirFd, path, mode, followSymlinks);
return;
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public void fchmod(Object receiver_, int fd, int mode) throws PosixException {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
INSTANCE.getUncached(receiver_).fchmod(receiver_, fd, mode);
return;
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public Object readlinkat(Object receiver_, int dirFd, Object path) throws PosixException {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
return INSTANCE.getUncached(receiver_).readlinkat(receiver_, dirFd, path);
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public void kill(Object receiver_, long pid, int signal) throws PosixException {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
INSTANCE.getUncached(receiver_).kill(receiver_, pid, signal);
return;
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public void killpg(Object receiver_, long pid, int signal) throws PosixException {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
INSTANCE.getUncached(receiver_).killpg(receiver_, pid, signal);
return;
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public long[] waitpid(Object receiver_, long pid, int options) throws PosixException {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
return INSTANCE.getUncached(receiver_).waitpid(receiver_, pid, options);
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public void abort(Object receiver_) {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
INSTANCE.getUncached(receiver_).abort(receiver_);
return;
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public boolean wcoredump(Object receiver_, int status) {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
return INSTANCE.getUncached(receiver_).wcoredump(receiver_, status);
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public boolean wifcontinued(Object receiver_, int status) {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
return INSTANCE.getUncached(receiver_).wifcontinued(receiver_, status);
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public boolean wifstopped(Object receiver_, int status) {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
return INSTANCE.getUncached(receiver_).wifstopped(receiver_, status);
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public boolean wifsignaled(Object receiver_, int status) {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
return INSTANCE.getUncached(receiver_).wifsignaled(receiver_, status);
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public boolean wifexited(Object receiver_, int status) {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
return INSTANCE.getUncached(receiver_).wifexited(receiver_, status);
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public int wexitstatus(Object receiver_, int status) {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
return INSTANCE.getUncached(receiver_).wexitstatus(receiver_, status);
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public int wtermsig(Object receiver_, int status) {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
return INSTANCE.getUncached(receiver_).wtermsig(receiver_, status);
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public int wstopsig(Object receiver_, int status) {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
return INSTANCE.getUncached(receiver_).wstopsig(receiver_, status);
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public long getuid(Object receiver_) {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
return INSTANCE.getUncached(receiver_).getuid(receiver_);
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public long geteuid(Object receiver_) {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
return INSTANCE.getUncached(receiver_).geteuid(receiver_);
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public long getgid(Object receiver_) {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
return INSTANCE.getUncached(receiver_).getgid(receiver_);
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public long getppid(Object receiver_) {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
return INSTANCE.getUncached(receiver_).getppid(receiver_);
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public long getpgid(Object receiver_, long pid) throws PosixException {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
return INSTANCE.getUncached(receiver_).getpgid(receiver_, pid);
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public void setpgid(Object receiver_, long pid, long pgid) throws PosixException {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
INSTANCE.getUncached(receiver_).setpgid(receiver_, pid, pgid);
return;
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public long getpgrp(Object receiver_) {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
return INSTANCE.getUncached(receiver_).getpgrp(receiver_);
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public long getsid(Object receiver_, long pid) throws PosixException {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
return INSTANCE.getUncached(receiver_).getsid(receiver_, pid);
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public long setsid(Object receiver_) throws PosixException {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
return INSTANCE.getUncached(receiver_).setsid(receiver_);
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public OpenPtyResult openpty(Object receiver_) throws PosixException {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
return INSTANCE.getUncached(receiver_).openpty(receiver_);
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public TruffleString ctermid(Object receiver_) throws PosixException {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
return INSTANCE.getUncached(receiver_).ctermid(receiver_);
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public void setenv(Object receiver_, Object name, Object value, boolean overwrite) throws PosixException {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
INSTANCE.getUncached(receiver_).setenv(receiver_, name, value, overwrite);
return;
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public void unsetenv(Object receiver_, Object name) throws PosixException {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
INSTANCE.getUncached(receiver_).unsetenv(receiver_, name);
return;
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public int forkExec(Object receiver_, Object[] executables, Object[] args, Object cwd, Object[] env, int stdinReadFd, int stdinWriteFd, int stdoutReadFd, int stdoutWriteFd, int stderrReadFd, int stderrWriteFd, int errPipeReadFd, int errPipeWriteFd, boolean closeFds, boolean restoreSignals, boolean callSetsid, int[] fdsToKeep) throws PosixException {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
return INSTANCE.getUncached(receiver_).forkExec(receiver_, executables, args, cwd, env, stdinReadFd, stdinWriteFd, stdoutReadFd, stdoutWriteFd, stderrReadFd, stderrWriteFd, errPipeReadFd, errPipeWriteFd, closeFds, restoreSignals, callSetsid, fdsToKeep);
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public void execv(Object receiver_, Object pathname, Object[] args) throws PosixException {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
INSTANCE.getUncached(receiver_).execv(receiver_, pathname, args);
return;
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public int system(Object receiver_, Object command) {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
return INSTANCE.getUncached(receiver_).system(receiver_, command);
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public Object mmap(Object receiver_, long length, int prot, int flags, int fd, long offset) throws PosixException {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
return INSTANCE.getUncached(receiver_).mmap(receiver_, length, prot, flags, fd, offset);
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public byte mmapReadByte(Object receiver_, Object mmap, long index) throws PosixException {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
return INSTANCE.getUncached(receiver_).mmapReadByte(receiver_, mmap, index);
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public void mmapWriteByte(Object receiver_, Object mmap, long index, byte value) throws PosixException {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
INSTANCE.getUncached(receiver_).mmapWriteByte(receiver_, mmap, index, value);
return;
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public int mmapReadBytes(Object receiver_, Object mmap, long index, byte[] bytes, int length) throws PosixException {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
return INSTANCE.getUncached(receiver_).mmapReadBytes(receiver_, mmap, index, bytes, length);
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public void mmapWriteBytes(Object receiver_, Object mmap, long index, byte[] bytes, int length) throws PosixException {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
INSTANCE.getUncached(receiver_).mmapWriteBytes(receiver_, mmap, index, bytes, length);
return;
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public void mmapFlush(Object receiver_, Object mmap, long offset, long length) throws PosixException {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
INSTANCE.getUncached(receiver_).mmapFlush(receiver_, mmap, offset, length);
return;
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public void mmapUnmap(Object receiver_, Object mmap, long length) throws PosixException {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
INSTANCE.getUncached(receiver_).mmapUnmap(receiver_, mmap, length);
return;
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public long mmapGetPointer(Object receiver_, Object mmap) {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
return INSTANCE.getUncached(receiver_).mmapGetPointer(receiver_, mmap);
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public PwdResult getpwuid(Object receiver_, long uid) throws PosixException {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
return INSTANCE.getUncached(receiver_).getpwuid(receiver_, uid);
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public PwdResult getpwnam(Object receiver_, Object name) throws PosixException {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
return INSTANCE.getUncached(receiver_).getpwnam(receiver_, name);
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public boolean hasGetpwentries(Object receiver_) {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
return INSTANCE.getUncached(receiver_).hasGetpwentries(receiver_);
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public PwdResult[] getpwentries(Object receiver_) throws PosixException {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
return INSTANCE.getUncached(receiver_).getpwentries(receiver_);
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public Object createPathFromString(Object receiver_, TruffleString path) {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
return INSTANCE.getUncached(receiver_).createPathFromString(receiver_, path);
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public Object createPathFromBytes(Object receiver_, byte[] path) {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
return INSTANCE.getUncached(receiver_).createPathFromBytes(receiver_, path);
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public TruffleString getPathAsString(Object receiver_, Object path) {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
return INSTANCE.getUncached(receiver_).getPathAsString(receiver_, path);
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public Buffer getPathAsBytes(Object receiver_, Object path) {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
return INSTANCE.getUncached(receiver_).getPathAsBytes(receiver_, path);
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public int socket(Object receiver_, int domain, int type, int protocol) throws PosixException {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
return INSTANCE.getUncached(receiver_).socket(receiver_, domain, type, protocol);
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public AcceptResult accept(Object receiver_, int sockfd) throws PosixException {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
return INSTANCE.getUncached(receiver_).accept(receiver_, sockfd);
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public void bind(Object receiver_, int sockfd, UniversalSockAddr addr) throws PosixException {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
INSTANCE.getUncached(receiver_).bind(receiver_, sockfd, addr);
return;
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public void connect(Object receiver_, int sockfd, UniversalSockAddr addr) throws PosixException {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
INSTANCE.getUncached(receiver_).connect(receiver_, sockfd, addr);
return;
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public void listen(Object receiver_, int sockfd, int backlog) throws PosixException {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
INSTANCE.getUncached(receiver_).listen(receiver_, sockfd, backlog);
return;
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public UniversalSockAddr getpeername(Object receiver_, int sockfd) throws PosixException {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
return INSTANCE.getUncached(receiver_).getpeername(receiver_, sockfd);
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public UniversalSockAddr getsockname(Object receiver_, int sockfd) throws PosixException {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
return INSTANCE.getUncached(receiver_).getsockname(receiver_, sockfd);
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public int send(Object receiver_, int sockfd, byte[] buf, int offset, int len, int flags) throws PosixException {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
return INSTANCE.getUncached(receiver_).send(receiver_, sockfd, buf, offset, len, flags);
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public int sendto(Object receiver_, int sockfd, byte[] buf, int offset, int len, int flags, UniversalSockAddr destAddr) throws PosixException {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
return INSTANCE.getUncached(receiver_).sendto(receiver_, sockfd, buf, offset, len, flags, destAddr);
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public int recv(Object receiver_, int sockfd, byte[] buf, int offset, int len, int flags) throws PosixException {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
return INSTANCE.getUncached(receiver_).recv(receiver_, sockfd, buf, offset, len, flags);
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public RecvfromResult recvfrom(Object receiver_, int sockfd, byte[] buf, int offset, int len, int flags) throws PosixException {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
return INSTANCE.getUncached(receiver_).recvfrom(receiver_, sockfd, buf, offset, len, flags);
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public void shutdown(Object receiver_, int sockfd, int how) throws PosixException {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
INSTANCE.getUncached(receiver_).shutdown(receiver_, sockfd, how);
return;
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public int getsockopt(Object receiver_, int sockfd, int level, int optname, byte[] optval, int optlen) throws PosixException {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
return INSTANCE.getUncached(receiver_).getsockopt(receiver_, sockfd, level, optname, optval, optlen);
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public void setsockopt(Object receiver_, int sockfd, int level, int optname, byte[] optval, int optlen) throws PosixException {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
INSTANCE.getUncached(receiver_).setsockopt(receiver_, sockfd, level, optname, optval, optlen);
return;
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public int inet_addr(Object receiver_, Object src) {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
return INSTANCE.getUncached(receiver_).inet_addr(receiver_, src);
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public int inet_aton(Object receiver_, Object src) throws InvalidAddressException {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
return INSTANCE.getUncached(receiver_).inet_aton(receiver_, src);
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public Object inet_ntoa(Object receiver_, int address) {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
return INSTANCE.getUncached(receiver_).inet_ntoa(receiver_, address);
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public byte[] inet_pton(Object receiver_, int family, Object src) throws PosixException, InvalidAddressException {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
return INSTANCE.getUncached(receiver_).inet_pton(receiver_, family, src);
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public Object inet_ntop(Object receiver_, int family, byte[] src) throws PosixException {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
return INSTANCE.getUncached(receiver_).inet_ntop(receiver_, family, src);
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public Object gethostname(Object receiver_) throws PosixException {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
return INSTANCE.getUncached(receiver_).gethostname(receiver_);
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public Object[] getnameinfo(Object receiver_, UniversalSockAddr addr, int flags) throws GetAddrInfoException {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
return INSTANCE.getUncached(receiver_).getnameinfo(receiver_, addr, flags);
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public AddrInfoCursor getaddrinfo(Object receiver_, Object node, Object service, int family, int sockType, int protocol, int flags) throws GetAddrInfoException {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
return INSTANCE.getUncached(receiver_).getaddrinfo(receiver_, node, service, family, sockType, protocol, flags);
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public TruffleString crypt(Object receiver_, TruffleString word, TruffleString salt) throws PosixException {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
return INSTANCE.getUncached(receiver_).crypt(receiver_, word, salt);
} finally {
encapsulating_.set(prev_);
}
}
@TruffleBoundary
@Override
public UniversalSockAddr createUniversalSockAddr(Object receiver_, FamilySpecificSockAddr src) {
assert assertAdopted(this);
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(getParent());
try {
return INSTANCE.getUncached(receiver_).createUniversalSockAddr(receiver_, src);
} finally {
encapsulating_.set(prev_);
}
}
@Override
public boolean accepts(Object receiver_) {
return true;
}
}
@GeneratedBy(PosixSupportLibrary.class)
@DenyReplace
private static final class UncachedDispatch extends PosixSupportLibrary {
@Override
public NodeCost getCost() {
return NodeCost.MEGAMORPHIC;
}
@TruffleBoundary
@Override
public TruffleString getBackend(Object receiver_) {
return INSTANCE.getUncached(receiver_).getBackend(receiver_);
}
@TruffleBoundary
@Override
public TruffleString strerror(Object receiver_, int errorCode) {
return INSTANCE.getUncached(receiver_).strerror(receiver_, errorCode);
}
@TruffleBoundary
@Override
public long getpid(Object receiver_) {
return INSTANCE.getUncached(receiver_).getpid(receiver_);
}
@TruffleBoundary
@Override
public int umask(Object receiver_, int mask) throws PosixException {
return INSTANCE.getUncached(receiver_).umask(receiver_, mask);
}
@TruffleBoundary
@Override
public int openat(Object receiver_, int dirFd, Object pathname, int flags, int mode) throws PosixException {
return INSTANCE.getUncached(receiver_).openat(receiver_, dirFd, pathname, flags, mode);
}
@TruffleBoundary
@Override
public int close(Object receiver_, int fd) throws PosixException {
return INSTANCE.getUncached(receiver_).close(receiver_, fd);
}
@TruffleBoundary
@Override
public Buffer read(Object receiver_, int fd, long length) throws PosixException {
return INSTANCE.getUncached(receiver_).read(receiver_, fd, length);
}
@TruffleBoundary
@Override
public long write(Object receiver_, int fd, Buffer data) throws PosixException {
return INSTANCE.getUncached(receiver_).write(receiver_, fd, data);
}
@TruffleBoundary
@Override
public int dup(Object receiver_, int fd) throws PosixException {
return INSTANCE.getUncached(receiver_).dup(receiver_, fd);
}
@TruffleBoundary
@Override
public int dup2(Object receiver_, int fd, int fd2, boolean inheritable) throws PosixException {
return INSTANCE.getUncached(receiver_).dup2(receiver_, fd, fd2, inheritable);
}
@TruffleBoundary
@Override
public boolean getInheritable(Object receiver_, int fd) throws PosixException {
return INSTANCE.getUncached(receiver_).getInheritable(receiver_, fd);
}
@TruffleBoundary
@Override
public void setInheritable(Object receiver_, int fd, boolean inheritable) throws PosixException {
INSTANCE.getUncached(receiver_).setInheritable(receiver_, fd, inheritable);
return;
}
@TruffleBoundary
@Override
public int[] pipe(Object receiver_) throws PosixException {
return INSTANCE.getUncached(receiver_).pipe(receiver_);
}
@TruffleBoundary
@Override
public SelectResult select(Object receiver_, int[] readfds, int[] writefds, int[] errorfds, Timeval timeout) throws PosixException {
return INSTANCE.getUncached(receiver_).select(receiver_, readfds, writefds, errorfds, timeout);
}
@TruffleBoundary
@Override
public long lseek(Object receiver_, int fd, long offset, int how) throws PosixException {
return INSTANCE.getUncached(receiver_).lseek(receiver_, fd, offset, how);
}
@TruffleBoundary
@Override
public void ftruncate(Object receiver_, int fd, long length) throws PosixException {
INSTANCE.getUncached(receiver_).ftruncate(receiver_, fd, length);
return;
}
@TruffleBoundary
@Override
public void fsync(Object receiver_, int fd) throws PosixException {
INSTANCE.getUncached(receiver_).fsync(receiver_, fd);
return;
}
@TruffleBoundary
@Override
public void flock(Object receiver_, int fd, int operation) throws PosixException {
INSTANCE.getUncached(receiver_).flock(receiver_, fd, operation);
return;
}
@TruffleBoundary
@Override
public boolean getBlocking(Object receiver_, int fd) throws PosixException {
return INSTANCE.getUncached(receiver_).getBlocking(receiver_, fd);
}
@TruffleBoundary
@Override
public void setBlocking(Object receiver_, int fd, boolean blocking) throws PosixException {
INSTANCE.getUncached(receiver_).setBlocking(receiver_, fd, blocking);
return;
}
@TruffleBoundary
@Override
public int[] getTerminalSize(Object receiver_, int fd) throws PosixException {
return INSTANCE.getUncached(receiver_).getTerminalSize(receiver_, fd);
}
@TruffleBoundary
@Override
public long[] fstatat(Object receiver_, int dirFd, Object pathname, boolean followSymlinks) throws PosixException {
return INSTANCE.getUncached(receiver_).fstatat(receiver_, dirFd, pathname, followSymlinks);
}
@TruffleBoundary
@Override
public long[] fstat(Object receiver_, int fd) throws PosixException {
return INSTANCE.getUncached(receiver_).fstat(receiver_, fd);
}
@TruffleBoundary
@Override
public long[] statvfs(Object receiver_, Object path) throws PosixException {
return INSTANCE.getUncached(receiver_).statvfs(receiver_, path);
}
@TruffleBoundary
@Override
public long[] fstatvfs(Object receiver_, int fd) throws PosixException {
return INSTANCE.getUncached(receiver_).fstatvfs(receiver_, fd);
}
@TruffleBoundary
@Override
public Object[] uname(Object receiver_) throws PosixException {
return INSTANCE.getUncached(receiver_).uname(receiver_);
}
@TruffleBoundary
@Override
public void unlinkat(Object receiver_, int dirFd, Object pathname, boolean rmdir) throws PosixException {
INSTANCE.getUncached(receiver_).unlinkat(receiver_, dirFd, pathname, rmdir);
return;
}
@TruffleBoundary
@Override
public void linkat(Object receiver_, int oldFdDir, Object oldPath, int newFdDir, Object newPath, int flags) throws PosixException {
INSTANCE.getUncached(receiver_).linkat(receiver_, oldFdDir, oldPath, newFdDir, newPath, flags);
return;
}
@TruffleBoundary
@Override
public void symlinkat(Object receiver_, Object target, int linkpathDirFd, Object linkpath) throws PosixException {
INSTANCE.getUncached(receiver_).symlinkat(receiver_, target, linkpathDirFd, linkpath);
return;
}
@TruffleBoundary
@Override
public void mkdirat(Object receiver_, int dirFd, Object pathname, int mode) throws PosixException {
INSTANCE.getUncached(receiver_).mkdirat(receiver_, dirFd, pathname, mode);
return;
}
@TruffleBoundary
@Override
public Object getcwd(Object receiver_) throws PosixException {
return INSTANCE.getUncached(receiver_).getcwd(receiver_);
}
@TruffleBoundary
@Override
public void chdir(Object receiver_, Object path) throws PosixException {
INSTANCE.getUncached(receiver_).chdir(receiver_, path);
return;
}
@TruffleBoundary
@Override
public void fchdir(Object receiver_, int fd) throws PosixException {
INSTANCE.getUncached(receiver_).fchdir(receiver_, fd);
return;
}
@TruffleBoundary
@Override
public boolean isatty(Object receiver_, int fd) {
return INSTANCE.getUncached(receiver_).isatty(receiver_, fd);
}
@TruffleBoundary
@Override
public Object opendir(Object receiver_, Object path) throws PosixException {
return INSTANCE.getUncached(receiver_).opendir(receiver_, path);
}
@TruffleBoundary
@Override
public Object fdopendir(Object receiver_, int fd) throws PosixException {
return INSTANCE.getUncached(receiver_).fdopendir(receiver_, fd);
}
@TruffleBoundary
@Override
public void closedir(Object receiver_, Object dirStream) throws PosixException {
INSTANCE.getUncached(receiver_).closedir(receiver_, dirStream);
return;
}
@TruffleBoundary
@Override
public Object readdir(Object receiver_, Object dirStream) throws PosixException {
return INSTANCE.getUncached(receiver_).readdir(receiver_, dirStream);
}
@TruffleBoundary
@Override
public void rewinddir(Object receiver_, Object dirStream) {
INSTANCE.getUncached(receiver_).rewinddir(receiver_, dirStream);
return;
}
@TruffleBoundary
@Override
public Object dirEntryGetName(Object receiver_, Object dirEntry) throws PosixException {
return INSTANCE.getUncached(receiver_).dirEntryGetName(receiver_, dirEntry);
}
@TruffleBoundary
@Override
public Object dirEntryGetPath(Object receiver_, Object dirEntry, Object scandirPath) throws PosixException {
return INSTANCE.getUncached(receiver_).dirEntryGetPath(receiver_, dirEntry, scandirPath);
}
@TruffleBoundary
@Override
public long dirEntryGetInode(Object receiver_, Object dirEntry) throws PosixException {
return INSTANCE.getUncached(receiver_).dirEntryGetInode(receiver_, dirEntry);
}
@TruffleBoundary
@Override
public int dirEntryGetType(Object receiver_, Object dirEntry) {
return INSTANCE.getUncached(receiver_).dirEntryGetType(receiver_, dirEntry);
}
@TruffleBoundary
@Override
public void utimensat(Object receiver_, int dirFd, Object pathname, long[] timespec, boolean followSymlinks) throws PosixException {
INSTANCE.getUncached(receiver_).utimensat(receiver_, dirFd, pathname, timespec, followSymlinks);
return;
}
@TruffleBoundary
@Override
public void futimens(Object receiver_, int fd, long[] timespec) throws PosixException {
INSTANCE.getUncached(receiver_).futimens(receiver_, fd, timespec);
return;
}
@TruffleBoundary
@Override
public void futimes(Object receiver_, int fd, Timeval[] timeval) throws PosixException {
INSTANCE.getUncached(receiver_).futimes(receiver_, fd, timeval);
return;
}
@TruffleBoundary
@Override
public void lutimes(Object receiver_, Object filename, Timeval[] timeval) throws PosixException {
INSTANCE.getUncached(receiver_).lutimes(receiver_, filename, timeval);
return;
}
@TruffleBoundary
@Override
public void utimes(Object receiver_, Object filename, Timeval[] timeval) throws PosixException {
INSTANCE.getUncached(receiver_).utimes(receiver_, filename, timeval);
return;
}
@TruffleBoundary
@Override
public void renameat(Object receiver_, int oldDirFd, Object oldPath, int newDirFd, Object newPath) throws PosixException {
INSTANCE.getUncached(receiver_).renameat(receiver_, oldDirFd, oldPath, newDirFd, newPath);
return;
}
@TruffleBoundary
@Override
public boolean faccessat(Object receiver_, int dirFd, Object path, int mode, boolean effectiveIds, boolean followSymlinks) {
return INSTANCE.getUncached(receiver_).faccessat(receiver_, dirFd, path, mode, effectiveIds, followSymlinks);
}
@TruffleBoundary
@Override
public void fchmodat(Object receiver_, int dirFd, Object path, int mode, boolean followSymlinks) throws PosixException {
INSTANCE.getUncached(receiver_).fchmodat(receiver_, dirFd, path, mode, followSymlinks);
return;
}
@TruffleBoundary
@Override
public void fchmod(Object receiver_, int fd, int mode) throws PosixException {
INSTANCE.getUncached(receiver_).fchmod(receiver_, fd, mode);
return;
}
@TruffleBoundary
@Override
public Object readlinkat(Object receiver_, int dirFd, Object path) throws PosixException {
return INSTANCE.getUncached(receiver_).readlinkat(receiver_, dirFd, path);
}
@TruffleBoundary
@Override
public void kill(Object receiver_, long pid, int signal) throws PosixException {
INSTANCE.getUncached(receiver_).kill(receiver_, pid, signal);
return;
}
@TruffleBoundary
@Override
public void killpg(Object receiver_, long pid, int signal) throws PosixException {
INSTANCE.getUncached(receiver_).killpg(receiver_, pid, signal);
return;
}
@TruffleBoundary
@Override
public long[] waitpid(Object receiver_, long pid, int options) throws PosixException {
return INSTANCE.getUncached(receiver_).waitpid(receiver_, pid, options);
}
@TruffleBoundary
@Override
public void abort(Object receiver_) {
INSTANCE.getUncached(receiver_).abort(receiver_);
return;
}
@TruffleBoundary
@Override
public boolean wcoredump(Object receiver_, int status) {
return INSTANCE.getUncached(receiver_).wcoredump(receiver_, status);
}
@TruffleBoundary
@Override
public boolean wifcontinued(Object receiver_, int status) {
return INSTANCE.getUncached(receiver_).wifcontinued(receiver_, status);
}
@TruffleBoundary
@Override
public boolean wifstopped(Object receiver_, int status) {
return INSTANCE.getUncached(receiver_).wifstopped(receiver_, status);
}
@TruffleBoundary
@Override
public boolean wifsignaled(Object receiver_, int status) {
return INSTANCE.getUncached(receiver_).wifsignaled(receiver_, status);
}
@TruffleBoundary
@Override
public boolean wifexited(Object receiver_, int status) {
return INSTANCE.getUncached(receiver_).wifexited(receiver_, status);
}
@TruffleBoundary
@Override
public int wexitstatus(Object receiver_, int status) {
return INSTANCE.getUncached(receiver_).wexitstatus(receiver_, status);
}
@TruffleBoundary
@Override
public int wtermsig(Object receiver_, int status) {
return INSTANCE.getUncached(receiver_).wtermsig(receiver_, status);
}
@TruffleBoundary
@Override
public int wstopsig(Object receiver_, int status) {
return INSTANCE.getUncached(receiver_).wstopsig(receiver_, status);
}
@TruffleBoundary
@Override
public long getuid(Object receiver_) {
return INSTANCE.getUncached(receiver_).getuid(receiver_);
}
@TruffleBoundary
@Override
public long geteuid(Object receiver_) {
return INSTANCE.getUncached(receiver_).geteuid(receiver_);
}
@TruffleBoundary
@Override
public long getgid(Object receiver_) {
return INSTANCE.getUncached(receiver_).getgid(receiver_);
}
@TruffleBoundary
@Override
public long getppid(Object receiver_) {
return INSTANCE.getUncached(receiver_).getppid(receiver_);
}
@TruffleBoundary
@Override
public long getpgid(Object receiver_, long pid) throws PosixException {
return INSTANCE.getUncached(receiver_).getpgid(receiver_, pid);
}
@TruffleBoundary
@Override
public void setpgid(Object receiver_, long pid, long pgid) throws PosixException {
INSTANCE.getUncached(receiver_).setpgid(receiver_, pid, pgid);
return;
}
@TruffleBoundary
@Override
public long getpgrp(Object receiver_) {
return INSTANCE.getUncached(receiver_).getpgrp(receiver_);
}
@TruffleBoundary
@Override
public long getsid(Object receiver_, long pid) throws PosixException {
return INSTANCE.getUncached(receiver_).getsid(receiver_, pid);
}
@TruffleBoundary
@Override
public long setsid(Object receiver_) throws PosixException {
return INSTANCE.getUncached(receiver_).setsid(receiver_);
}
@TruffleBoundary
@Override
public OpenPtyResult openpty(Object receiver_) throws PosixException {
return INSTANCE.getUncached(receiver_).openpty(receiver_);
}
@TruffleBoundary
@Override
public TruffleString ctermid(Object receiver_) throws PosixException {
return INSTANCE.getUncached(receiver_).ctermid(receiver_);
}
@TruffleBoundary
@Override
public void setenv(Object receiver_, Object name, Object value, boolean overwrite) throws PosixException {
INSTANCE.getUncached(receiver_).setenv(receiver_, name, value, overwrite);
return;
}
@TruffleBoundary
@Override
public void unsetenv(Object receiver_, Object name) throws PosixException {
INSTANCE.getUncached(receiver_).unsetenv(receiver_, name);
return;
}
@TruffleBoundary
@Override
public int forkExec(Object receiver_, Object[] executables, Object[] args, Object cwd, Object[] env, int stdinReadFd, int stdinWriteFd, int stdoutReadFd, int stdoutWriteFd, int stderrReadFd, int stderrWriteFd, int errPipeReadFd, int errPipeWriteFd, boolean closeFds, boolean restoreSignals, boolean callSetsid, int[] fdsToKeep) throws PosixException {
return INSTANCE.getUncached(receiver_).forkExec(receiver_, executables, args, cwd, env, stdinReadFd, stdinWriteFd, stdoutReadFd, stdoutWriteFd, stderrReadFd, stderrWriteFd, errPipeReadFd, errPipeWriteFd, closeFds, restoreSignals, callSetsid, fdsToKeep);
}
@TruffleBoundary
@Override
public void execv(Object receiver_, Object pathname, Object[] args) throws PosixException {
INSTANCE.getUncached(receiver_).execv(receiver_, pathname, args);
return;
}
@TruffleBoundary
@Override
public int system(Object receiver_, Object command) {
return INSTANCE.getUncached(receiver_).system(receiver_, command);
}
@TruffleBoundary
@Override
public Object mmap(Object receiver_, long length, int prot, int flags, int fd, long offset) throws PosixException {
return INSTANCE.getUncached(receiver_).mmap(receiver_, length, prot, flags, fd, offset);
}
@TruffleBoundary
@Override
public byte mmapReadByte(Object receiver_, Object mmap, long index) throws PosixException {
return INSTANCE.getUncached(receiver_).mmapReadByte(receiver_, mmap, index);
}
@TruffleBoundary
@Override
public void mmapWriteByte(Object receiver_, Object mmap, long index, byte value) throws PosixException {
INSTANCE.getUncached(receiver_).mmapWriteByte(receiver_, mmap, index, value);
return;
}
@TruffleBoundary
@Override
public int mmapReadBytes(Object receiver_, Object mmap, long index, byte[] bytes, int length) throws PosixException {
return INSTANCE.getUncached(receiver_).mmapReadBytes(receiver_, mmap, index, bytes, length);
}
@TruffleBoundary
@Override
public void mmapWriteBytes(Object receiver_, Object mmap, long index, byte[] bytes, int length) throws PosixException {
INSTANCE.getUncached(receiver_).mmapWriteBytes(receiver_, mmap, index, bytes, length);
return;
}
@TruffleBoundary
@Override
public void mmapFlush(Object receiver_, Object mmap, long offset, long length) throws PosixException {
INSTANCE.getUncached(receiver_).mmapFlush(receiver_, mmap, offset, length);
return;
}
@TruffleBoundary
@Override
public void mmapUnmap(Object receiver_, Object mmap, long length) throws PosixException {
INSTANCE.getUncached(receiver_).mmapUnmap(receiver_, mmap, length);
return;
}
@TruffleBoundary
@Override
public long mmapGetPointer(Object receiver_, Object mmap) {
return INSTANCE.getUncached(receiver_).mmapGetPointer(receiver_, mmap);
}
@TruffleBoundary
@Override
public PwdResult getpwuid(Object receiver_, long uid) throws PosixException {
return INSTANCE.getUncached(receiver_).getpwuid(receiver_, uid);
}
@TruffleBoundary
@Override
public PwdResult getpwnam(Object receiver_, Object name) throws PosixException {
return INSTANCE.getUncached(receiver_).getpwnam(receiver_, name);
}
@TruffleBoundary
@Override
public boolean hasGetpwentries(Object receiver_) {
return INSTANCE.getUncached(receiver_).hasGetpwentries(receiver_);
}
@TruffleBoundary
@Override
public PwdResult[] getpwentries(Object receiver_) throws PosixException {
return INSTANCE.getUncached(receiver_).getpwentries(receiver_);
}
@TruffleBoundary
@Override
public Object createPathFromString(Object receiver_, TruffleString path) {
return INSTANCE.getUncached(receiver_).createPathFromString(receiver_, path);
}
@TruffleBoundary
@Override
public Object createPathFromBytes(Object receiver_, byte[] path) {
return INSTANCE.getUncached(receiver_).createPathFromBytes(receiver_, path);
}
@TruffleBoundary
@Override
public TruffleString getPathAsString(Object receiver_, Object path) {
return INSTANCE.getUncached(receiver_).getPathAsString(receiver_, path);
}
@TruffleBoundary
@Override
public Buffer getPathAsBytes(Object receiver_, Object path) {
return INSTANCE.getUncached(receiver_).getPathAsBytes(receiver_, path);
}
@TruffleBoundary
@Override
public int socket(Object receiver_, int domain, int type, int protocol) throws PosixException {
return INSTANCE.getUncached(receiver_).socket(receiver_, domain, type, protocol);
}
@TruffleBoundary
@Override
public AcceptResult accept(Object receiver_, int sockfd) throws PosixException {
return INSTANCE.getUncached(receiver_).accept(receiver_, sockfd);
}
@TruffleBoundary
@Override
public void bind(Object receiver_, int sockfd, UniversalSockAddr addr) throws PosixException {
INSTANCE.getUncached(receiver_).bind(receiver_, sockfd, addr);
return;
}
@TruffleBoundary
@Override
public void connect(Object receiver_, int sockfd, UniversalSockAddr addr) throws PosixException {
INSTANCE.getUncached(receiver_).connect(receiver_, sockfd, addr);
return;
}
@TruffleBoundary
@Override
public void listen(Object receiver_, int sockfd, int backlog) throws PosixException {
INSTANCE.getUncached(receiver_).listen(receiver_, sockfd, backlog);
return;
}
@TruffleBoundary
@Override
public UniversalSockAddr getpeername(Object receiver_, int sockfd) throws PosixException {
return INSTANCE.getUncached(receiver_).getpeername(receiver_, sockfd);
}
@TruffleBoundary
@Override
public UniversalSockAddr getsockname(Object receiver_, int sockfd) throws PosixException {
return INSTANCE.getUncached(receiver_).getsockname(receiver_, sockfd);
}
@TruffleBoundary
@Override
public int send(Object receiver_, int sockfd, byte[] buf, int offset, int len, int flags) throws PosixException {
return INSTANCE.getUncached(receiver_).send(receiver_, sockfd, buf, offset, len, flags);
}
@TruffleBoundary
@Override
public int sendto(Object receiver_, int sockfd, byte[] buf, int offset, int len, int flags, UniversalSockAddr destAddr) throws PosixException {
return INSTANCE.getUncached(receiver_).sendto(receiver_, sockfd, buf, offset, len, flags, destAddr);
}
@TruffleBoundary
@Override
public int recv(Object receiver_, int sockfd, byte[] buf, int offset, int len, int flags) throws PosixException {
return INSTANCE.getUncached(receiver_).recv(receiver_, sockfd, buf, offset, len, flags);
}
@TruffleBoundary
@Override
public RecvfromResult recvfrom(Object receiver_, int sockfd, byte[] buf, int offset, int len, int flags) throws PosixException {
return INSTANCE.getUncached(receiver_).recvfrom(receiver_, sockfd, buf, offset, len, flags);
}
@TruffleBoundary
@Override
public void shutdown(Object receiver_, int sockfd, int how) throws PosixException {
INSTANCE.getUncached(receiver_).shutdown(receiver_, sockfd, how);
return;
}
@TruffleBoundary
@Override
public int getsockopt(Object receiver_, int sockfd, int level, int optname, byte[] optval, int optlen) throws PosixException {
return INSTANCE.getUncached(receiver_).getsockopt(receiver_, sockfd, level, optname, optval, optlen);
}
@TruffleBoundary
@Override
public void setsockopt(Object receiver_, int sockfd, int level, int optname, byte[] optval, int optlen) throws PosixException {
INSTANCE.getUncached(receiver_).setsockopt(receiver_, sockfd, level, optname, optval, optlen);
return;
}
@TruffleBoundary
@Override
public int inet_addr(Object receiver_, Object src) {
return INSTANCE.getUncached(receiver_).inet_addr(receiver_, src);
}
@TruffleBoundary
@Override
public int inet_aton(Object receiver_, Object src) throws InvalidAddressException {
return INSTANCE.getUncached(receiver_).inet_aton(receiver_, src);
}
@TruffleBoundary
@Override
public Object inet_ntoa(Object receiver_, int address) {
return INSTANCE.getUncached(receiver_).inet_ntoa(receiver_, address);
}
@TruffleBoundary
@Override
public byte[] inet_pton(Object receiver_, int family, Object src) throws PosixException, InvalidAddressException {
return INSTANCE.getUncached(receiver_).inet_pton(receiver_, family, src);
}
@TruffleBoundary
@Override
public Object inet_ntop(Object receiver_, int family, byte[] src) throws PosixException {
return INSTANCE.getUncached(receiver_).inet_ntop(receiver_, family, src);
}
@TruffleBoundary
@Override
public Object gethostname(Object receiver_) throws PosixException {
return INSTANCE.getUncached(receiver_).gethostname(receiver_);
}
@TruffleBoundary
@Override
public Object[] getnameinfo(Object receiver_, UniversalSockAddr addr, int flags) throws GetAddrInfoException {
return INSTANCE.getUncached(receiver_).getnameinfo(receiver_, addr, flags);
}
@TruffleBoundary
@Override
public AddrInfoCursor getaddrinfo(Object receiver_, Object node, Object service, int family, int sockType, int protocol, int flags) throws GetAddrInfoException {
return INSTANCE.getUncached(receiver_).getaddrinfo(receiver_, node, service, family, sockType, protocol, flags);
}
@TruffleBoundary
@Override
public TruffleString crypt(Object receiver_, TruffleString word, TruffleString salt) throws PosixException {
return INSTANCE.getUncached(receiver_).crypt(receiver_, word, salt);
}
@TruffleBoundary
@Override
public UniversalSockAddr createUniversalSockAddr(Object receiver_, FamilySpecificSockAddr src) {
return INSTANCE.getUncached(receiver_).createUniversalSockAddr(receiver_, src);
}
@TruffleBoundary
@Override
public boolean accepts(Object receiver_) {
return true;
}
@Override
public boolean isAdoptable() {
return false;
}
}
@GeneratedBy(PosixSupportLibrary.class)
private static final class CachedDispatchNext extends CachedDispatch {
CachedDispatchNext(PosixSupportLibrary library, CachedDispatch next) {
super(library, next);
}
@Override
int getLimit() {
throw CompilerDirectives.shouldNotReachHere();
}
@Override
public NodeCost getCost() {
return NodeCost.NONE;
}
}
@GeneratedBy(PosixSupportLibrary.class)
private static final class CachedDispatchFirst extends CachedDispatch {
private final int limit_;
CachedDispatchFirst(PosixSupportLibrary library, CachedDispatch next, int limit_) {
super(library, next);
this.limit_ = limit_;
}
@Override
int getLimit() {
return this.limit_;
}
@Override
public NodeCost getCost() {
if (this.library instanceof CachedToUncachedDispatch) {
return NodeCost.MEGAMORPHIC;
}
CachedDispatch current = this;
int count = 0;
do {
if (current.library != null) {
count++;
}
current = current.next;
} while (current != null);
return NodeCost.fromCount(count);
}
}
@GeneratedBy(PosixSupportLibrary.class)
private abstract static class CachedDispatch extends PosixSupportLibrary {
@Child PosixSupportLibrary library;
@Child CachedDispatch next;
CachedDispatch(PosixSupportLibrary library, CachedDispatch next) {
this.library = library;
this.next = next;
}
abstract int getLimit();
@ExplodeLoop
@Override
public TruffleString getBackend(Object receiver_) {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
return thisLibrary.getBackend(receiver_);
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public TruffleString strerror(Object receiver_, int errorCode) {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
return thisLibrary.strerror(receiver_, errorCode);
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public long getpid(Object receiver_) {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
return thisLibrary.getpid(receiver_);
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public int umask(Object receiver_, int mask) throws PosixException {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
return thisLibrary.umask(receiver_, mask);
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public int openat(Object receiver_, int dirFd, Object pathname, int flags, int mode) throws PosixException {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
return thisLibrary.openat(receiver_, dirFd, pathname, flags, mode);
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public int close(Object receiver_, int fd) throws PosixException {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
return thisLibrary.close(receiver_, fd);
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public Buffer read(Object receiver_, int fd, long length) throws PosixException {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
return thisLibrary.read(receiver_, fd, length);
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public long write(Object receiver_, int fd, Buffer data) throws PosixException {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
return thisLibrary.write(receiver_, fd, data);
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public int dup(Object receiver_, int fd) throws PosixException {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
return thisLibrary.dup(receiver_, fd);
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public int dup2(Object receiver_, int fd, int fd2, boolean inheritable) throws PosixException {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
return thisLibrary.dup2(receiver_, fd, fd2, inheritable);
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public boolean getInheritable(Object receiver_, int fd) throws PosixException {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
return thisLibrary.getInheritable(receiver_, fd);
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public void setInheritable(Object receiver_, int fd, boolean inheritable) throws PosixException {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
thisLibrary.setInheritable(receiver_, fd, inheritable);
return;
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public int[] pipe(Object receiver_) throws PosixException {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
return thisLibrary.pipe(receiver_);
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public SelectResult select(Object receiver_, int[] readfds, int[] writefds, int[] errorfds, Timeval timeout) throws PosixException {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
return thisLibrary.select(receiver_, readfds, writefds, errorfds, timeout);
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public long lseek(Object receiver_, int fd, long offset, int how) throws PosixException {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
return thisLibrary.lseek(receiver_, fd, offset, how);
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public void ftruncate(Object receiver_, int fd, long length) throws PosixException {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
thisLibrary.ftruncate(receiver_, fd, length);
return;
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public void fsync(Object receiver_, int fd) throws PosixException {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
thisLibrary.fsync(receiver_, fd);
return;
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public void flock(Object receiver_, int fd, int operation) throws PosixException {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
thisLibrary.flock(receiver_, fd, operation);
return;
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public boolean getBlocking(Object receiver_, int fd) throws PosixException {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
return thisLibrary.getBlocking(receiver_, fd);
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public void setBlocking(Object receiver_, int fd, boolean blocking) throws PosixException {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
thisLibrary.setBlocking(receiver_, fd, blocking);
return;
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public int[] getTerminalSize(Object receiver_, int fd) throws PosixException {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
return thisLibrary.getTerminalSize(receiver_, fd);
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public long[] fstatat(Object receiver_, int dirFd, Object pathname, boolean followSymlinks) throws PosixException {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
return thisLibrary.fstatat(receiver_, dirFd, pathname, followSymlinks);
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public long[] fstat(Object receiver_, int fd) throws PosixException {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
return thisLibrary.fstat(receiver_, fd);
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public long[] statvfs(Object receiver_, Object path) throws PosixException {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
return thisLibrary.statvfs(receiver_, path);
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public long[] fstatvfs(Object receiver_, int fd) throws PosixException {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
return thisLibrary.fstatvfs(receiver_, fd);
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public Object[] uname(Object receiver_) throws PosixException {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
return thisLibrary.uname(receiver_);
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public void unlinkat(Object receiver_, int dirFd, Object pathname, boolean rmdir) throws PosixException {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
thisLibrary.unlinkat(receiver_, dirFd, pathname, rmdir);
return;
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public void linkat(Object receiver_, int oldFdDir, Object oldPath, int newFdDir, Object newPath, int flags) throws PosixException {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
thisLibrary.linkat(receiver_, oldFdDir, oldPath, newFdDir, newPath, flags);
return;
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public void symlinkat(Object receiver_, Object target, int linkpathDirFd, Object linkpath) throws PosixException {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
thisLibrary.symlinkat(receiver_, target, linkpathDirFd, linkpath);
return;
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public void mkdirat(Object receiver_, int dirFd, Object pathname, int mode) throws PosixException {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
thisLibrary.mkdirat(receiver_, dirFd, pathname, mode);
return;
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public Object getcwd(Object receiver_) throws PosixException {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
return thisLibrary.getcwd(receiver_);
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public void chdir(Object receiver_, Object path) throws PosixException {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
thisLibrary.chdir(receiver_, path);
return;
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public void fchdir(Object receiver_, int fd) throws PosixException {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
thisLibrary.fchdir(receiver_, fd);
return;
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public boolean isatty(Object receiver_, int fd) {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
return thisLibrary.isatty(receiver_, fd);
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public Object opendir(Object receiver_, Object path) throws PosixException {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
return thisLibrary.opendir(receiver_, path);
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public Object fdopendir(Object receiver_, int fd) throws PosixException {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
return thisLibrary.fdopendir(receiver_, fd);
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public void closedir(Object receiver_, Object dirStream) throws PosixException {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
thisLibrary.closedir(receiver_, dirStream);
return;
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public Object readdir(Object receiver_, Object dirStream) throws PosixException {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
return thisLibrary.readdir(receiver_, dirStream);
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public void rewinddir(Object receiver_, Object dirStream) {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
thisLibrary.rewinddir(receiver_, dirStream);
return;
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public Object dirEntryGetName(Object receiver_, Object dirEntry) throws PosixException {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
return thisLibrary.dirEntryGetName(receiver_, dirEntry);
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public Object dirEntryGetPath(Object receiver_, Object dirEntry, Object scandirPath) throws PosixException {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
return thisLibrary.dirEntryGetPath(receiver_, dirEntry, scandirPath);
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public long dirEntryGetInode(Object receiver_, Object dirEntry) throws PosixException {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
return thisLibrary.dirEntryGetInode(receiver_, dirEntry);
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public int dirEntryGetType(Object receiver_, Object dirEntry) {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
return thisLibrary.dirEntryGetType(receiver_, dirEntry);
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public void utimensat(Object receiver_, int dirFd, Object pathname, long[] timespec, boolean followSymlinks) throws PosixException {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
thisLibrary.utimensat(receiver_, dirFd, pathname, timespec, followSymlinks);
return;
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public void futimens(Object receiver_, int fd, long[] timespec) throws PosixException {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
thisLibrary.futimens(receiver_, fd, timespec);
return;
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public void futimes(Object receiver_, int fd, Timeval[] timeval) throws PosixException {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
thisLibrary.futimes(receiver_, fd, timeval);
return;
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public void lutimes(Object receiver_, Object filename, Timeval[] timeval) throws PosixException {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
thisLibrary.lutimes(receiver_, filename, timeval);
return;
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public void utimes(Object receiver_, Object filename, Timeval[] timeval) throws PosixException {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
thisLibrary.utimes(receiver_, filename, timeval);
return;
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public void renameat(Object receiver_, int oldDirFd, Object oldPath, int newDirFd, Object newPath) throws PosixException {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
thisLibrary.renameat(receiver_, oldDirFd, oldPath, newDirFd, newPath);
return;
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public boolean faccessat(Object receiver_, int dirFd, Object path, int mode, boolean effectiveIds, boolean followSymlinks) {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
return thisLibrary.faccessat(receiver_, dirFd, path, mode, effectiveIds, followSymlinks);
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public void fchmodat(Object receiver_, int dirFd, Object path, int mode, boolean followSymlinks) throws PosixException {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
thisLibrary.fchmodat(receiver_, dirFd, path, mode, followSymlinks);
return;
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public void fchmod(Object receiver_, int fd, int mode) throws PosixException {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
thisLibrary.fchmod(receiver_, fd, mode);
return;
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public Object readlinkat(Object receiver_, int dirFd, Object path) throws PosixException {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
return thisLibrary.readlinkat(receiver_, dirFd, path);
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public void kill(Object receiver_, long pid, int signal) throws PosixException {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
thisLibrary.kill(receiver_, pid, signal);
return;
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public void killpg(Object receiver_, long pid, int signal) throws PosixException {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
thisLibrary.killpg(receiver_, pid, signal);
return;
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public long[] waitpid(Object receiver_, long pid, int options) throws PosixException {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
return thisLibrary.waitpid(receiver_, pid, options);
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public void abort(Object receiver_) {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
thisLibrary.abort(receiver_);
return;
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public boolean wcoredump(Object receiver_, int status) {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
return thisLibrary.wcoredump(receiver_, status);
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public boolean wifcontinued(Object receiver_, int status) {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
return thisLibrary.wifcontinued(receiver_, status);
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public boolean wifstopped(Object receiver_, int status) {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
return thisLibrary.wifstopped(receiver_, status);
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public boolean wifsignaled(Object receiver_, int status) {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
return thisLibrary.wifsignaled(receiver_, status);
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public boolean wifexited(Object receiver_, int status) {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
return thisLibrary.wifexited(receiver_, status);
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public int wexitstatus(Object receiver_, int status) {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
return thisLibrary.wexitstatus(receiver_, status);
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public int wtermsig(Object receiver_, int status) {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
return thisLibrary.wtermsig(receiver_, status);
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public int wstopsig(Object receiver_, int status) {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
return thisLibrary.wstopsig(receiver_, status);
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public long getuid(Object receiver_) {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
return thisLibrary.getuid(receiver_);
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public long geteuid(Object receiver_) {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
return thisLibrary.geteuid(receiver_);
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public long getgid(Object receiver_) {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
return thisLibrary.getgid(receiver_);
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public long getppid(Object receiver_) {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
return thisLibrary.getppid(receiver_);
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public long getpgid(Object receiver_, long pid) throws PosixException {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
return thisLibrary.getpgid(receiver_, pid);
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public void setpgid(Object receiver_, long pid, long pgid) throws PosixException {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
thisLibrary.setpgid(receiver_, pid, pgid);
return;
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public long getpgrp(Object receiver_) {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
return thisLibrary.getpgrp(receiver_);
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public long getsid(Object receiver_, long pid) throws PosixException {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
return thisLibrary.getsid(receiver_, pid);
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public long setsid(Object receiver_) throws PosixException {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
return thisLibrary.setsid(receiver_);
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public OpenPtyResult openpty(Object receiver_) throws PosixException {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
return thisLibrary.openpty(receiver_);
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public TruffleString ctermid(Object receiver_) throws PosixException {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
return thisLibrary.ctermid(receiver_);
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public void setenv(Object receiver_, Object name, Object value, boolean overwrite) throws PosixException {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
thisLibrary.setenv(receiver_, name, value, overwrite);
return;
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public void unsetenv(Object receiver_, Object name) throws PosixException {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
thisLibrary.unsetenv(receiver_, name);
return;
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public int forkExec(Object receiver_, Object[] executables, Object[] args, Object cwd, Object[] env, int stdinReadFd, int stdinWriteFd, int stdoutReadFd, int stdoutWriteFd, int stderrReadFd, int stderrWriteFd, int errPipeReadFd, int errPipeWriteFd, boolean closeFds, boolean restoreSignals, boolean callSetsid, int[] fdsToKeep) throws PosixException {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
return thisLibrary.forkExec(receiver_, executables, args, cwd, env, stdinReadFd, stdinWriteFd, stdoutReadFd, stdoutWriteFd, stderrReadFd, stderrWriteFd, errPipeReadFd, errPipeWriteFd, closeFds, restoreSignals, callSetsid, fdsToKeep);
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public void execv(Object receiver_, Object pathname, Object[] args) throws PosixException {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
thisLibrary.execv(receiver_, pathname, args);
return;
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public int system(Object receiver_, Object command) {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
return thisLibrary.system(receiver_, command);
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public Object mmap(Object receiver_, long length, int prot, int flags, int fd, long offset) throws PosixException {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
return thisLibrary.mmap(receiver_, length, prot, flags, fd, offset);
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public byte mmapReadByte(Object receiver_, Object mmap, long index) throws PosixException {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
return thisLibrary.mmapReadByte(receiver_, mmap, index);
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public void mmapWriteByte(Object receiver_, Object mmap, long index, byte value) throws PosixException {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
thisLibrary.mmapWriteByte(receiver_, mmap, index, value);
return;
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public int mmapReadBytes(Object receiver_, Object mmap, long index, byte[] bytes, int length) throws PosixException {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
return thisLibrary.mmapReadBytes(receiver_, mmap, index, bytes, length);
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public void mmapWriteBytes(Object receiver_, Object mmap, long index, byte[] bytes, int length) throws PosixException {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
thisLibrary.mmapWriteBytes(receiver_, mmap, index, bytes, length);
return;
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public void mmapFlush(Object receiver_, Object mmap, long offset, long length) throws PosixException {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
thisLibrary.mmapFlush(receiver_, mmap, offset, length);
return;
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public void mmapUnmap(Object receiver_, Object mmap, long length) throws PosixException {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
thisLibrary.mmapUnmap(receiver_, mmap, length);
return;
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public long mmapGetPointer(Object receiver_, Object mmap) {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
return thisLibrary.mmapGetPointer(receiver_, mmap);
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public PwdResult getpwuid(Object receiver_, long uid) throws PosixException {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
return thisLibrary.getpwuid(receiver_, uid);
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public PwdResult getpwnam(Object receiver_, Object name) throws PosixException {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
return thisLibrary.getpwnam(receiver_, name);
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public boolean hasGetpwentries(Object receiver_) {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
return thisLibrary.hasGetpwentries(receiver_);
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public PwdResult[] getpwentries(Object receiver_) throws PosixException {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
return thisLibrary.getpwentries(receiver_);
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public Object createPathFromString(Object receiver_, TruffleString path) {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
return thisLibrary.createPathFromString(receiver_, path);
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public Object createPathFromBytes(Object receiver_, byte[] path) {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
return thisLibrary.createPathFromBytes(receiver_, path);
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public TruffleString getPathAsString(Object receiver_, Object path) {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
return thisLibrary.getPathAsString(receiver_, path);
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public Buffer getPathAsBytes(Object receiver_, Object path) {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
return thisLibrary.getPathAsBytes(receiver_, path);
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public int socket(Object receiver_, int domain, int type, int protocol) throws PosixException {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
return thisLibrary.socket(receiver_, domain, type, protocol);
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public AcceptResult accept(Object receiver_, int sockfd) throws PosixException {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
return thisLibrary.accept(receiver_, sockfd);
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public void bind(Object receiver_, int sockfd, UniversalSockAddr addr) throws PosixException {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
thisLibrary.bind(receiver_, sockfd, addr);
return;
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public void connect(Object receiver_, int sockfd, UniversalSockAddr addr) throws PosixException {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
thisLibrary.connect(receiver_, sockfd, addr);
return;
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public void listen(Object receiver_, int sockfd, int backlog) throws PosixException {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
thisLibrary.listen(receiver_, sockfd, backlog);
return;
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public UniversalSockAddr getpeername(Object receiver_, int sockfd) throws PosixException {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
return thisLibrary.getpeername(receiver_, sockfd);
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public UniversalSockAddr getsockname(Object receiver_, int sockfd) throws PosixException {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
return thisLibrary.getsockname(receiver_, sockfd);
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public int send(Object receiver_, int sockfd, byte[] buf, int offset, int len, int flags) throws PosixException {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
return thisLibrary.send(receiver_, sockfd, buf, offset, len, flags);
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public int sendto(Object receiver_, int sockfd, byte[] buf, int offset, int len, int flags, UniversalSockAddr destAddr) throws PosixException {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
return thisLibrary.sendto(receiver_, sockfd, buf, offset, len, flags, destAddr);
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public int recv(Object receiver_, int sockfd, byte[] buf, int offset, int len, int flags) throws PosixException {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
return thisLibrary.recv(receiver_, sockfd, buf, offset, len, flags);
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public RecvfromResult recvfrom(Object receiver_, int sockfd, byte[] buf, int offset, int len, int flags) throws PosixException {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
return thisLibrary.recvfrom(receiver_, sockfd, buf, offset, len, flags);
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public void shutdown(Object receiver_, int sockfd, int how) throws PosixException {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
thisLibrary.shutdown(receiver_, sockfd, how);
return;
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public int getsockopt(Object receiver_, int sockfd, int level, int optname, byte[] optval, int optlen) throws PosixException {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
return thisLibrary.getsockopt(receiver_, sockfd, level, optname, optval, optlen);
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public void setsockopt(Object receiver_, int sockfd, int level, int optname, byte[] optval, int optlen) throws PosixException {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
thisLibrary.setsockopt(receiver_, sockfd, level, optname, optval, optlen);
return;
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public int inet_addr(Object receiver_, Object src) {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
return thisLibrary.inet_addr(receiver_, src);
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public int inet_aton(Object receiver_, Object src) throws InvalidAddressException {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
return thisLibrary.inet_aton(receiver_, src);
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public Object inet_ntoa(Object receiver_, int address) {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
return thisLibrary.inet_ntoa(receiver_, address);
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public byte[] inet_pton(Object receiver_, int family, Object src) throws PosixException, InvalidAddressException {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
return thisLibrary.inet_pton(receiver_, family, src);
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public Object inet_ntop(Object receiver_, int family, byte[] src) throws PosixException {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
return thisLibrary.inet_ntop(receiver_, family, src);
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public Object gethostname(Object receiver_) throws PosixException {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
return thisLibrary.gethostname(receiver_);
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public Object[] getnameinfo(Object receiver_, UniversalSockAddr addr, int flags) throws GetAddrInfoException {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
return thisLibrary.getnameinfo(receiver_, addr, flags);
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public AddrInfoCursor getaddrinfo(Object receiver_, Object node, Object service, int family, int sockType, int protocol, int flags) throws GetAddrInfoException {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
return thisLibrary.getaddrinfo(receiver_, node, service, family, sockType, protocol, flags);
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public TruffleString crypt(Object receiver_, TruffleString word, TruffleString salt) throws PosixException {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
return thisLibrary.crypt(receiver_, word, salt);
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@ExplodeLoop
@Override
public UniversalSockAddr createUniversalSockAddr(Object receiver_, FamilySpecificSockAddr src) {
do {
CachedDispatch current = this;
do {
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary != null && thisLibrary.accepts(receiver_)) {
return thisLibrary.createUniversalSockAddr(receiver_, src);
}
current = current.next;
} while (current != null);
CompilerDirectives.transferToInterpreterAndInvalidate();
specialize(receiver_);
} while (true);
}
@Override
public boolean accepts(Object receiver_) {
return true;
}
private void specialize(Object receiver_) {
Lock lock = getLock();
lock.lock();
try {
CachedDispatch current = this;
PosixSupportLibrary thisLibrary = current.library;
if (thisLibrary == null) {
this.library = insert(INSTANCE.create(receiver_));
} else {
int count = 0;
do {
PosixSupportLibrary currentLibrary = current.library;
if (currentLibrary != null && currentLibrary.accepts(receiver_)) {
return;
}
count++;
current = current.next;
} while (current != null);
if (count >= getLimit()) {
this.library = insert(new CachedToUncachedDispatch());
this.next = null;
} else {
this.next = insert(new CachedDispatchNext(INSTANCE.create(receiver_), next));
}
}
} finally {
lock.unlock();
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy