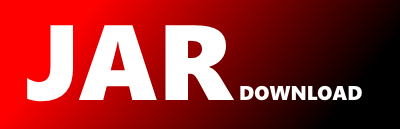
com.oracle.graal.python.runtime.sequence.PSequenceGen Maven / Gradle / Ivy
// CheckStyle: start generated
package com.oracle.graal.python.runtime.sequence;
import com.oracle.graal.python.builtins.objects.PythonAbstractObject;
import com.oracle.graal.python.builtins.objects.PythonAbstractObjectGen;
import com.oracle.graal.python.builtins.objects.PythonAbstractObject.PInteropSubscriptNode;
import com.oracle.graal.python.builtins.objects.PythonAbstractObject.PKeyInfoNode;
import com.oracle.graal.python.builtins.objects.common.SequenceNodes.GetSequenceStorageNode;
import com.oracle.graal.python.builtins.objects.common.SequenceNodesFactory.GetSequenceStorageNodeGen;
import com.oracle.graal.python.builtins.objects.common.SequenceStorageNodes.GetItemScalarNode;
import com.oracle.graal.python.builtins.objects.common.SequenceStorageNodesFactory.GetItemScalarNodeGen;
import com.oracle.graal.python.builtins.objects.type.TypeNodes.IsTypeNode;
import com.oracle.graal.python.nodes.attributes.ReadAttributeFromObjectNode;
import com.oracle.graal.python.nodes.classes.IsSubtypeNode;
import com.oracle.graal.python.nodes.object.GetClassNode;
import com.oracle.graal.python.runtime.GilNode;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.CompilerDirectives.TruffleBoundary;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.DSLSupport.SpecializationDataNode;
import com.oracle.truffle.api.dsl.InlineSupport.InlineTarget;
import com.oracle.truffle.api.dsl.InlineSupport.ReferenceField;
import com.oracle.truffle.api.dsl.InlineSupport.StateField;
import com.oracle.truffle.api.dsl.InlineSupport.UnsafeAccessedField;
import com.oracle.truffle.api.interop.InteropLibrary;
import com.oracle.truffle.api.interop.InvalidArrayIndexException;
import com.oracle.truffle.api.interop.UnsupportedMessageException;
import com.oracle.truffle.api.library.LibraryExport;
import com.oracle.truffle.api.nodes.DenyReplace;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.nodes.NodeCost;
import com.oracle.truffle.api.object.DynamicObjectLibrary;
import com.oracle.truffle.api.strings.TruffleString.FromJavaStringNode;
import java.lang.invoke.MethodHandles;
import java.lang.invoke.VarHandle;
import java.lang.invoke.MethodHandles.Lookup;
import java.util.Objects;
@GeneratedBy(PSequence.class)
@SuppressWarnings("javadoc")
public final class PSequenceGen {
static {
LibraryExport.register(PSequence.class, new InteropLibraryExports());
}
private PSequenceGen() {
}
@GeneratedBy(PSequence.class)
public static class InteropLibraryExports extends LibraryExport {
private InteropLibraryExports() {
super(InteropLibrary.class, PSequence.class, false, false, 0);
}
@Override
protected InteropLibrary createUncached(Object receiver) {
assert receiver instanceof PSequence;
InteropLibrary uncached = new Uncached(receiver);
return uncached;
}
@Override
protected InteropLibrary createCached(Object receiver) {
assert receiver instanceof PSequence;
return new Cached(receiver);
}
@GeneratedBy(PSequence.class)
public static class Cached extends PythonAbstractObjectGen.InteropLibraryExports.Cached {
private static final StateField READ_ARRAY_ELEMENT__READ_ARRAY_ELEMENT_NODE__READ_ARRAY_ELEMENT_STATE_0_UPDATER = StateField.create(ReadArrayElementNode_ReadArrayElementData.lookup_(), "readArrayElement_state_0_");
private static final StateField GET_ARRAY_SIZE__GET_ARRAY_SIZE_NODE__GET_ARRAY_SIZE_STATE_0_UPDATER = StateField.create(GetArraySizeNode_GetArraySizeData.lookup_(), "getArraySize_state_0_");
/**
* Source Info:
* Specialization: {@link PSequence#readArrayElement(PSequence, long, Node, GetSequenceStorageNode, GetItemScalarNode, GilNode)}
* Parameter: {@link GetSequenceStorageNode} getSequenceStorageNode
* Inline method: {@link GetSequenceStorageNodeGen#inline}
*/
private static final GetSequenceStorageNode INLINED_READ_ARRAY_ELEMENT_NODE__READ_ARRAY_ELEMENT_GET_SEQUENCE_STORAGE_NODE_ = GetSequenceStorageNodeGen.inline(InlineTarget.create(GetSequenceStorageNode.class, READ_ARRAY_ELEMENT__READ_ARRAY_ELEMENT_NODE__READ_ARRAY_ELEMENT_STATE_0_UPDATER.subUpdater(0, 3), ReferenceField.create(ReadArrayElementNode_ReadArrayElementData.lookup_(), "readArrayElementNode__readArrayElement_getSequenceStorageNode__field1_", Object.class)));
/**
* Source Info:
* Specialization: {@link PSequence#readArrayElement(PSequence, long, Node, GetSequenceStorageNode, GetItemScalarNode, GilNode)}
* Parameter: {@link GetItemScalarNode} getItem
* Inline method: {@link GetItemScalarNodeGen#inline}
*/
private static final GetItemScalarNode INLINED_READ_ARRAY_ELEMENT_NODE__READ_ARRAY_ELEMENT_GET_ITEM_ = GetItemScalarNodeGen.inline(InlineTarget.create(GetItemScalarNode.class, READ_ARRAY_ELEMENT__READ_ARRAY_ELEMENT_NODE__READ_ARRAY_ELEMENT_STATE_0_UPDATER.subUpdater(3, 8), ReferenceField.create(ReadArrayElementNode_ReadArrayElementData.lookup_(), "readArrayElementNode__readArrayElement_getItem__field1_", Node.class)));
/**
* Source Info:
* Specialization: {@link PSequence#getArraySize(PSequence, Node, GetSequenceStorageNode, GilNode)}
* Parameter: {@link GetSequenceStorageNode} getSequenceStorageNode
* Inline method: {@link GetSequenceStorageNodeGen#inline}
*/
private static final GetSequenceStorageNode INLINED_GET_ARRAY_SIZE_NODE__GET_ARRAY_SIZE_GET_SEQUENCE_STORAGE_NODE_ = GetSequenceStorageNodeGen.inline(InlineTarget.create(GetSequenceStorageNode.class, GET_ARRAY_SIZE__GET_ARRAY_SIZE_NODE__GET_ARRAY_SIZE_STATE_0_UPDATER.subUpdater(0, 3), ReferenceField.create(GetArraySizeNode_GetArraySizeData.lookup_(), "getArraySizeNode__getArraySize_getSequenceStorageNode__field1_", Object.class)));
/**
* State Info:
* 0: SpecializationActive {@link PSequence#readArrayElement(PSequence, long, Node, GetSequenceStorageNode, GetItemScalarNode, GilNode)}
* 1: SpecializationActive {@link PSequence#getArraySize(PSequence, Node, GetSequenceStorageNode, GilNode)}
*
*/
@CompilationFinal private int state_0_;
/**
* Source Info:
* Specialization: {@link PythonAbstractObject#writeMember}
* Parameter: {@link FromJavaStringNode} fromJavaStringNode
*/
@Child private FromJavaStringNode js2ts;
/**
* Source Info:
* Specialization: {@link PythonAbstractObject#isArrayElementReadable}
* Parameter: {@link PInteropSubscriptNode} getItemNode
*/
@Child private PInteropSubscriptNode getItemNode;
/**
* Source Info:
* Specialization: {@link PythonAbstractObject#isMemberReadable}
* Parameter: {@link PKeyInfoNode} keyInfoNode
*/
@Child private PKeyInfoNode keyInfoNode;
/**
* Source Info:
* Specialization: {@link PythonAbstractObject#getMembers}
* Parameter: {@link GetClassNode} getClass
*/
@Child private GetClassNode getClass;
/**
* Source Info:
* Specialization: {@link PythonAbstractObject#isInstantiable}
* Parameter: {@link IsTypeNode} isTypeNode
*/
@Child private IsTypeNode isTypeNode;
/**
* Source Info:
* Specialization: {@link PythonAbstractObject#isDate}
* Parameter: {@link ReadAttributeFromObjectNode} readTypeNode
*/
@Child private ReadAttributeFromObjectNode readTypeNode;
/**
* Source Info:
* Specialization: {@link PythonAbstractObject#isDate}
* Parameter: {@link IsSubtypeNode} isSubtypeNode
*/
@Child private IsSubtypeNode isSubtypeNode;
/**
* Source Info:
* Specialization: {@link PythonAbstractObject#hasIteratorNextElement}
* Parameter: {@link DynamicObjectLibrary} dylib
*/
@Child private DynamicObjectLibrary dylib;
@Child private ReadArrayElementNode_ReadArrayElementData readArrayElementNode__readArrayElement_cache;
@Child private GetArraySizeNode_GetArraySizeData getArraySizeNode__getArraySize_cache;
protected Cached(Object receiver) {
super(receiver);
}
@Override
public boolean hasArrayElements(Object receiver) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
return (((PSequence) receiver)).hasArrayElements();
}
/**
* Debug Info:
* Specialization {@link PSequence#readArrayElement(PSequence, long, Node, GetSequenceStorageNode, GetItemScalarNode, GilNode)}
* Activation probability: 0.02564
* With/without class size: 4/14 bytes
*
*/
@Override
public Object readArrayElement(Object arg0Value_, long arg1Value) throws UnsupportedMessageException, InvalidArrayIndexException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
PSequence arg0Value = ((PSequence) arg0Value_);
int state_0 = this.state_0_;
if ((state_0 & 0b1) != 0 /* is SpecializationActive[PSequence.readArrayElement(PSequence, long, Node, GetSequenceStorageNode, GetItemScalarNode, GilNode)] */) {
ReadArrayElementNode_ReadArrayElementData s0_ = this.readArrayElementNode__readArrayElement_cache;
if (s0_ != null) {
{
Node inliningTarget__ = (s0_);
return arg0Value.readArrayElement(arg1Value, inliningTarget__, INLINED_READ_ARRAY_ELEMENT_NODE__READ_ARRAY_ELEMENT_GET_SEQUENCE_STORAGE_NODE_, INLINED_READ_ARRAY_ELEMENT_NODE__READ_ARRAY_ELEMENT_GET_ITEM_, s0_.gil_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return readArrayElementNode_AndSpecialize(arg0Value, arg1Value);
}
private Object readArrayElementNode_AndSpecialize(PSequence arg0Value, long arg1Value) throws InvalidArrayIndexException {
int state_0 = this.state_0_;
{
Node inliningTarget__ = null;
ReadArrayElementNode_ReadArrayElementData s0_ = this.insert(new ReadArrayElementNode_ReadArrayElementData());
inliningTarget__ = (s0_);
GilNode gil__ = s0_.insert((GilNode.create()));
Objects.requireNonNull(gil__, "Specialization 'readArrayElement(PSequence, long, Node, GetSequenceStorageNode, GetItemScalarNode, GilNode)' cache 'gil' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s0_.gil_ = gil__;
VarHandle.storeStoreFence();
this.readArrayElementNode__readArrayElement_cache = s0_;
state_0 = state_0 | 0b1 /* add SpecializationActive[PSequence.readArrayElement(PSequence, long, Node, GetSequenceStorageNode, GetItemScalarNode, GilNode)] */;
this.state_0_ = state_0;
return arg0Value.readArrayElement(arg1Value, inliningTarget__, INLINED_READ_ARRAY_ELEMENT_NODE__READ_ARRAY_ELEMENT_GET_SEQUENCE_STORAGE_NODE_, INLINED_READ_ARRAY_ELEMENT_NODE__READ_ARRAY_ELEMENT_GET_ITEM_, gil__);
}
}
@Override
public NodeCost getCost() {
int state_0 = this.state_0_;
if ((state_0 & 0b1) == 0) {
return NodeCost.UNINITIALIZED;
} else {
return NodeCost.MONOMORPHIC;
}
}
/**
* Debug Info:
* Specialization {@link PSequence#getArraySize(PSequence, Node, GetSequenceStorageNode, GilNode)}
* Activation probability: 0.02564
* With/without class size: 4/9 bytes
*
*/
@Override
public long getArraySize(Object arg0Value_) throws UnsupportedMessageException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
PSequence arg0Value = ((PSequence) arg0Value_);
int state_0 = this.state_0_;
if ((state_0 & 0b10) != 0 /* is SpecializationActive[PSequence.getArraySize(PSequence, Node, GetSequenceStorageNode, GilNode)] */) {
GetArraySizeNode_GetArraySizeData s0_ = this.getArraySizeNode__getArraySize_cache;
if (s0_ != null) {
{
Node inliningTarget__ = (s0_);
return arg0Value.getArraySize(inliningTarget__, INLINED_GET_ARRAY_SIZE_NODE__GET_ARRAY_SIZE_GET_SEQUENCE_STORAGE_NODE_, s0_.gil_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return getArraySizeNode_AndSpecialize(arg0Value);
}
private long getArraySizeNode_AndSpecialize(PSequence arg0Value) {
int state_0 = this.state_0_;
{
Node inliningTarget__ = null;
GetArraySizeNode_GetArraySizeData s0_ = this.insert(new GetArraySizeNode_GetArraySizeData());
inliningTarget__ = (s0_);
GilNode gil__ = s0_.insert((GilNode.create()));
Objects.requireNonNull(gil__, "Specialization 'getArraySize(PSequence, Node, GetSequenceStorageNode, GilNode)' cache 'gil' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
s0_.gil_ = gil__;
VarHandle.storeStoreFence();
this.getArraySizeNode__getArraySize_cache = s0_;
state_0 = state_0 | 0b10 /* add SpecializationActive[PSequence.getArraySize(PSequence, Node, GetSequenceStorageNode, GilNode)] */;
this.state_0_ = state_0;
return arg0Value.getArraySize(inliningTarget__, INLINED_GET_ARRAY_SIZE_NODE__GET_ARRAY_SIZE_GET_SEQUENCE_STORAGE_NODE_, gil__);
}
}
@Override
public boolean isNumber(Object receiver) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
return (((PSequence) receiver)).isNumber();
}
@Override
public byte asByte(Object receiver) throws UnsupportedMessageException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
return (((PSequence) receiver)).asByte();
}
@Override
public boolean fitsInByte(Object receiver) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
return (((PSequence) receiver)).fitsInByte();
}
@Override
public short asShort(Object receiver) throws UnsupportedMessageException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
return (((PSequence) receiver)).asShort();
}
@Override
public boolean fitsInShort(Object receiver) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
return (((PSequence) receiver)).fitsInShort();
}
@Override
public int asInt(Object receiver) throws UnsupportedMessageException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
return (((PSequence) receiver)).asInt();
}
@Override
public boolean fitsInInt(Object receiver) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
return (((PSequence) receiver)).fitsInInt();
}
@Override
public long asLong(Object receiver) throws UnsupportedMessageException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
return (((PSequence) receiver)).asLong();
}
@Override
public boolean fitsInLong(Object receiver) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
return (((PSequence) receiver)).fitsInLong();
}
@Override
public float asFloat(Object receiver) throws UnsupportedMessageException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
return (((PSequence) receiver)).asFloat();
}
@Override
public boolean fitsInFloat(Object receiver) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
return (((PSequence) receiver)).fitsInFloat();
}
@Override
public double asDouble(Object receiver) throws UnsupportedMessageException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
return (((PSequence) receiver)).asDouble();
}
@Override
public boolean fitsInDouble(Object receiver) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
return (((PSequence) receiver)).fitsInDouble();
}
@Override
public boolean isString(Object receiver) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
return (((PSequence) receiver)).isString();
}
@Override
public String asString(Object receiver) throws UnsupportedMessageException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
return (((PSequence) receiver)).asString();
}
@GeneratedBy(PSequence.class)
@DenyReplace
private static final class ReadArrayElementNode_ReadArrayElementData extends Node implements SpecializationDataNode {
/**
* State Info:
* 0-2: InlinedCache
* Specialization: {@link PSequence#readArrayElement}
* Parameter: {@link GetSequenceStorageNode} getSequenceStorageNode
* Inline method: {@link GetSequenceStorageNodeGen#inline}
* 3-10: InlinedCache
* Specialization: {@link PSequence#readArrayElement}
* Parameter: {@link GetItemScalarNode} getItem
* Inline method: {@link GetItemScalarNodeGen#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int readArrayElement_state_0_;
/**
* Source Info:
* Specialization: {@link PSequence#readArrayElement(PSequence, long, Node, GetSequenceStorageNode, GetItemScalarNode, GilNode)}
* Parameter: {@link GetSequenceStorageNode} getSequenceStorageNode
* Inline method: {@link GetSequenceStorageNodeGen#inline}
* Inline field: {@link Object} field1
*/
@CompilationFinal @UnsafeAccessedField @SuppressWarnings("unused") private Object readArrayElementNode__readArrayElement_getSequenceStorageNode__field1_;
/**
* Source Info:
* Specialization: {@link PSequence#readArrayElement(PSequence, long, Node, GetSequenceStorageNode, GetItemScalarNode, GilNode)}
* Parameter: {@link GetItemScalarNode} getItem
* Inline method: {@link GetItemScalarNodeGen#inline}
* Inline field: {@link Node} field1
*/
@Child @UnsafeAccessedField @SuppressWarnings("unused") private Node readArrayElementNode__readArrayElement_getItem__field1_;
/**
* Source Info:
* Specialization: {@link PSequence#readArrayElement(PSequence, long, Node, GetSequenceStorageNode, GetItemScalarNode, GilNode)}
* Parameter: {@link GilNode} gil
*/
@Child GilNode gil_;
ReadArrayElementNode_ReadArrayElementData() {
}
@Override
public NodeCost getCost() {
return NodeCost.NONE;
}
private static Lookup lookup_() {
return MethodHandles.lookup();
}
}
@GeneratedBy(PSequence.class)
@DenyReplace
private static final class GetArraySizeNode_GetArraySizeData extends Node implements SpecializationDataNode {
/**
* State Info:
* 0-2: InlinedCache
* Specialization: {@link PSequence#getArraySize}
* Parameter: {@link GetSequenceStorageNode} getSequenceStorageNode
* Inline method: {@link GetSequenceStorageNodeGen#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int getArraySize_state_0_;
/**
* Source Info:
* Specialization: {@link PSequence#getArraySize(PSequence, Node, GetSequenceStorageNode, GilNode)}
* Parameter: {@link GetSequenceStorageNode} getSequenceStorageNode
* Inline method: {@link GetSequenceStorageNodeGen#inline}
* Inline field: {@link Object} field1
*/
@CompilationFinal @UnsafeAccessedField @SuppressWarnings("unused") private Object getArraySizeNode__getArraySize_getSequenceStorageNode__field1_;
/**
* Source Info:
* Specialization: {@link PSequence#getArraySize(PSequence, Node, GetSequenceStorageNode, GilNode)}
* Parameter: {@link GilNode} gil
*/
@Child GilNode gil_;
GetArraySizeNode_GetArraySizeData() {
}
@Override
public NodeCost getCost() {
return NodeCost.NONE;
}
private static Lookup lookup_() {
return MethodHandles.lookup();
}
}
}
@GeneratedBy(PSequence.class)
public static class Uncached extends PythonAbstractObjectGen.InteropLibraryExports.Uncached {
protected Uncached(Object receiver) {
super(receiver);
}
@Override
@TruffleBoundary
public boolean accepts(Object receiver) {
return super.accepts(receiver);
}
@TruffleBoundary
@Override
public boolean hasArrayElements(Object receiver) {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((PSequence) receiver) .hasArrayElements();
}
@TruffleBoundary
@Override
public Object readArrayElement(Object arg0Value_, long arg1Value) throws InvalidArrayIndexException, UnsupportedMessageException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
PSequence arg0Value = ((PSequence) arg0Value_);
return arg0Value.readArrayElement(arg1Value, (this), (GetSequenceStorageNode.getUncached()), (GetItemScalarNodeGen.getUncached()), (GilNode.getUncached()));
}
@TruffleBoundary
@Override
public long getArraySize(Object arg0Value_) throws UnsupportedMessageException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
PSequence arg0Value = ((PSequence) arg0Value_);
return arg0Value.getArraySize((this), (GetSequenceStorageNode.getUncached()), (GilNode.getUncached()));
}
@TruffleBoundary
@Override
public boolean isNumber(Object receiver) {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((PSequence) receiver) .isNumber();
}
@TruffleBoundary
@Override
public byte asByte(Object receiver) throws UnsupportedMessageException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((PSequence) receiver) .asByte();
}
@TruffleBoundary
@Override
public boolean fitsInByte(Object receiver) {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((PSequence) receiver) .fitsInByte();
}
@TruffleBoundary
@Override
public short asShort(Object receiver) throws UnsupportedMessageException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((PSequence) receiver) .asShort();
}
@TruffleBoundary
@Override
public boolean fitsInShort(Object receiver) {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((PSequence) receiver) .fitsInShort();
}
@TruffleBoundary
@Override
public int asInt(Object receiver) throws UnsupportedMessageException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((PSequence) receiver) .asInt();
}
@TruffleBoundary
@Override
public boolean fitsInInt(Object receiver) {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((PSequence) receiver) .fitsInInt();
}
@TruffleBoundary
@Override
public long asLong(Object receiver) throws UnsupportedMessageException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((PSequence) receiver) .asLong();
}
@TruffleBoundary
@Override
public boolean fitsInLong(Object receiver) {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((PSequence) receiver) .fitsInLong();
}
@TruffleBoundary
@Override
public float asFloat(Object receiver) throws UnsupportedMessageException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((PSequence) receiver) .asFloat();
}
@TruffleBoundary
@Override
public boolean fitsInFloat(Object receiver) {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((PSequence) receiver) .fitsInFloat();
}
@TruffleBoundary
@Override
public double asDouble(Object receiver) throws UnsupportedMessageException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((PSequence) receiver) .asDouble();
}
@TruffleBoundary
@Override
public boolean fitsInDouble(Object receiver) {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((PSequence) receiver) .fitsInDouble();
}
@TruffleBoundary
@Override
public boolean isString(Object receiver) {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((PSequence) receiver) .isString();
}
@TruffleBoundary
@Override
public String asString(Object receiver) throws UnsupportedMessageException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((PSequence) receiver) .asString();
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy