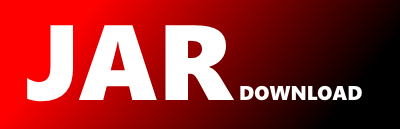
com.oracle.truffle.api.strings.TStringOpsNodesFactory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of truffle-api Show documentation
Show all versions of truffle-api Show documentation
Truffle is a multi-language framework for executing dynamic languages
that achieves high performance when combined with Graal.
// CheckStyle: start generated
package com.oracle.truffle.api.strings;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.CompilerDirectives.TruffleBoundary;
import com.oracle.truffle.api.dsl.DSLSupport;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.NeverDefault;
import com.oracle.truffle.api.dsl.UnsupportedSpecializationException;
import com.oracle.truffle.api.dsl.DSLSupport.SpecializationDataNode;
import com.oracle.truffle.api.dsl.InlineSupport.InlineTarget;
import com.oracle.truffle.api.dsl.InlineSupport.ReferenceField;
import com.oracle.truffle.api.dsl.InlineSupport.RequiredField;
import com.oracle.truffle.api.dsl.InlineSupport.StateField;
import com.oracle.truffle.api.dsl.InlineSupport.UnsafeAccessedField;
import com.oracle.truffle.api.nodes.DenyReplace;
import com.oracle.truffle.api.nodes.ExplodeLoop;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.nodes.NodeCost;
import com.oracle.truffle.api.profiles.InlinedConditionProfile;
import com.oracle.truffle.api.strings.TStringOpsNodes.CalculateHashCodeNode;
import com.oracle.truffle.api.strings.TStringOpsNodes.IndexOfAnyCharNode;
import com.oracle.truffle.api.strings.TStringOpsNodes.IndexOfAnyIntNode;
import com.oracle.truffle.api.strings.TStringOpsNodes.RawIndexOfCodePointNode;
import com.oracle.truffle.api.strings.TStringOpsNodes.RawIndexOfStringNode;
import com.oracle.truffle.api.strings.TStringOpsNodes.RawLastIndexOfCodePointNode;
import com.oracle.truffle.api.strings.TStringOpsNodes.RawLastIndexOfStringNode;
import com.oracle.truffle.api.strings.TStringOpsNodes.RawReadValueNode;
import com.oracle.truffle.api.strings.TruffleString.CompactionLevel;
import java.lang.invoke.MethodHandles;
import java.lang.invoke.MethodHandles.Lookup;
@GeneratedBy(TStringOpsNodes.class)
@SuppressWarnings("javadoc")
final class TStringOpsNodesFactory {
@CompilationFinal(dimensions = 1) private static final CompactionLevel[] COMPACTION_LEVEL_VALUES = DSLSupport.lookupEnumConstants(CompactionLevel.class);
private static CompactionLevel decodeCompactionLevel(int state) {
if (state >= 0) {
return COMPACTION_LEVEL_VALUES[state];
} else {
return null;
}
}
private static int encodeCompactionLevel(CompactionLevel e) {
if (e != null) {
return e.ordinal();
} else {
return -1;
}
}
/**
* Debug Info:
* Specialization {@link RawReadValueNode#cached}
* Activation probability: 0.48333
* With/without class size: 11/4 bytes
* Specialization {@link RawReadValueNode#cached}
* Activation probability: 0.33333
* With/without class size: 9/4 bytes
* Specialization {@link RawReadValueNode#cached}
* Activation probability: 0.18333
* With/without class size: 6/4 bytes
*
*/
@GeneratedBy(RawReadValueNode.class)
@SuppressWarnings("javadoc")
static final class RawReadValueNodeGen {
private static final Uncached UNCACHED = new Uncached();
@NeverDefault
public static RawReadValueNode getUncached() {
return RawReadValueNodeGen.UNCACHED;
}
/**
* Required Fields:
* - {@link Inlined#state_0_}
*
*/
@NeverDefault
public static RawReadValueNode inline(@RequiredField(bits = 12, value = StateField.class) InlineTarget target) {
return new RawReadValueNodeGen.Inlined(target);
}
@GeneratedBy(RawReadValueNode.class)
@DenyReplace
private static final class Inlined extends RawReadValueNode {
/**
* State Info:
* 0: SpecializationActive {@link RawReadValueNode#cached}
* 1-3: EncodedEnum[cache=TStringOpsNodes.RawReadValueNode.cached(..., CompactionLevel cachedCompaction)]
* 4: SpecializationActive {@link RawReadValueNode#cached}
* 5-7: EncodedEnum[cache=TStringOpsNodes.RawReadValueNode.cached(..., CompactionLevel cachedCompaction)]
* 8: SpecializationActive {@link RawReadValueNode#cached}
* 9-11: EncodedEnum[cache=TStringOpsNodes.RawReadValueNode.cached(..., CompactionLevel cachedCompaction)]
*
*/
private final StateField state_0_;
private Inlined(InlineTarget target) {
assert target.getTargetClass().isAssignableFrom(RawReadValueNode.class);
this.state_0_ = target.getState(0, 12);
}
@Override
int execute(Node arg0Value, AbstractTruffleString arg1Value, Object arg2Value, int arg3Value) {
int state_0 = this.state_0_.get(arg0Value);
if ((state_0 & 0b100010001) != 0 /* is SpecializationActive[TStringOpsNodes.RawReadValueNode.cached(Node, AbstractTruffleString, Object, int, CompactionLevel, CompactionLevel)] || SpecializationActive[TStringOpsNodes.RawReadValueNode.cached(Node, AbstractTruffleString, Object, int, CompactionLevel, CompactionLevel)] || SpecializationActive[TStringOpsNodes.RawReadValueNode.cached(Node, AbstractTruffleString, Object, int, CompactionLevel, CompactionLevel)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[TStringOpsNodes.RawReadValueNode.cached(Node, AbstractTruffleString, Object, int, CompactionLevel, CompactionLevel)] */) {
if ((state_0 & 0b1110) >>> 1 /* get-int EncodedEnum[cache=TStringOpsNodes.RawReadValueNode.cached(..., CompactionLevel cachedCompaction)] */ != 0) {
CompactionLevel compaction__ = (CompactionLevel.fromStride(arg1Value.stride()));
if ((compaction__ == decodeCompactionLevel(((state_0 & 0b1110) >>> 1 /* get-int EncodedEnum[cache=TStringOpsNodes.RawReadValueNode.cached(..., CompactionLevel cachedCompaction)] */) - 2))) {
return RawReadValueNode.cached(arg0Value, arg1Value, arg2Value, arg3Value, compaction__, decodeCompactionLevel(((state_0 & 0b1110) >>> 1 /* get-int EncodedEnum[cache=TStringOpsNodes.RawReadValueNode.cached(..., CompactionLevel cachedCompaction)] */) - 2));
}
}
}
if ((state_0 & 0b10000) != 0 /* is SpecializationActive[TStringOpsNodes.RawReadValueNode.cached(Node, AbstractTruffleString, Object, int, CompactionLevel, CompactionLevel)] */) {
if ((state_0 & 0b11100000) >>> 5 /* get-int EncodedEnum[cache=TStringOpsNodes.RawReadValueNode.cached(..., CompactionLevel cachedCompaction)] */ != 0) {
CompactionLevel compaction__ = (CompactionLevel.fromStride(arg1Value.stride()));
if ((compaction__ == decodeCompactionLevel(((state_0 & 0b11100000) >>> 5 /* get-int EncodedEnum[cache=TStringOpsNodes.RawReadValueNode.cached(..., CompactionLevel cachedCompaction)] */) - 2))) {
return RawReadValueNode.cached(arg0Value, arg1Value, arg2Value, arg3Value, compaction__, decodeCompactionLevel(((state_0 & 0b11100000) >>> 5 /* get-int EncodedEnum[cache=TStringOpsNodes.RawReadValueNode.cached(..., CompactionLevel cachedCompaction)] */) - 2));
}
}
}
if ((state_0 & 0b100000000) != 0 /* is SpecializationActive[TStringOpsNodes.RawReadValueNode.cached(Node, AbstractTruffleString, Object, int, CompactionLevel, CompactionLevel)] */) {
if ((state_0 & 0b111000000000) >>> 9 /* get-int EncodedEnum[cache=TStringOpsNodes.RawReadValueNode.cached(..., CompactionLevel cachedCompaction)] */ != 0) {
CompactionLevel compaction__ = (CompactionLevel.fromStride(arg1Value.stride()));
if ((compaction__ == decodeCompactionLevel(((state_0 & 0b111000000000) >>> 9 /* get-int EncodedEnum[cache=TStringOpsNodes.RawReadValueNode.cached(..., CompactionLevel cachedCompaction)] */) - 2))) {
return RawReadValueNode.cached(arg0Value, arg1Value, arg2Value, arg3Value, compaction__, decodeCompactionLevel(((state_0 & 0b111000000000) >>> 9 /* get-int EncodedEnum[cache=TStringOpsNodes.RawReadValueNode.cached(..., CompactionLevel cachedCompaction)] */) - 2));
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value, arg1Value, arg2Value, arg3Value);
}
private int executeAndSpecialize(Node arg0Value, AbstractTruffleString arg1Value, Object arg2Value, int arg3Value) {
int state_0 = this.state_0_.get(arg0Value);
{
CompactionLevel compaction__ = null;
while (true) {
boolean Cached0_duplicateFound_ = false;
if ((state_0 & 0b1) != 0 /* is SpecializationActive[TStringOpsNodes.RawReadValueNode.cached(Node, AbstractTruffleString, Object, int, CompactionLevel, CompactionLevel)] */ && (state_0 & 0b1110) >>> 1 /* get-int EncodedEnum[cache=TStringOpsNodes.RawReadValueNode.cached(..., CompactionLevel cachedCompaction)] */ != 0) {
compaction__ = (CompactionLevel.fromStride(arg1Value.stride()));
if ((compaction__ == decodeCompactionLevel(((state_0 & 0b1110) >>> 1 /* get-int EncodedEnum[cache=TStringOpsNodes.RawReadValueNode.cached(..., CompactionLevel cachedCompaction)] */) - 2))) {
Cached0_duplicateFound_ = true;
}
}
if (!Cached0_duplicateFound_) {
// assert ((CompactionLevel.fromStride(arg1Value.stride())) == decodeCompactionLevel(((state_0 & 0b1110) >>> 1 /* get-int EncodedEnum[cache=TStringOpsNodes.RawReadValueNode.cached(..., CompactionLevel cachedCompaction)] */) - 2));
if (((state_0 & 0b1)) == 0 /* is-not SpecializationActive[TStringOpsNodes.RawReadValueNode.cached(Node, AbstractTruffleString, Object, int, CompactionLevel, CompactionLevel)] */) {
compaction__ = (CompactionLevel.fromStride(arg1Value.stride()));
state_0 = (state_0 | ((encodeCompactionLevel((compaction__)) + 2) << 1) /* set-int EncodedEnum[cache=TStringOpsNodes.RawReadValueNode.cached(..., CompactionLevel cachedCompaction)] */);
state_0 = state_0 | 0b1 /* add SpecializationActive[TStringOpsNodes.RawReadValueNode.cached(Node, AbstractTruffleString, Object, int, CompactionLevel, CompactionLevel)] */;
this.state_0_.set(arg0Value, state_0);
Cached0_duplicateFound_ = true;
}
}
if (Cached0_duplicateFound_) {
return RawReadValueNode.cached(arg0Value, arg1Value, arg2Value, arg3Value, compaction__, decodeCompactionLevel(((state_0 & 0b1110) >>> 1 /* get-int EncodedEnum[cache=TStringOpsNodes.RawReadValueNode.cached(..., CompactionLevel cachedCompaction)] */) - 2));
}
break;
}
}
{
CompactionLevel compaction__ = null;
while (true) {
boolean Cached1_duplicateFound_ = false;
if ((state_0 & 0b10000) != 0 /* is SpecializationActive[TStringOpsNodes.RawReadValueNode.cached(Node, AbstractTruffleString, Object, int, CompactionLevel, CompactionLevel)] */ && (state_0 & 0b11100000) >>> 5 /* get-int EncodedEnum[cache=TStringOpsNodes.RawReadValueNode.cached(..., CompactionLevel cachedCompaction)] */ != 0) {
compaction__ = (CompactionLevel.fromStride(arg1Value.stride()));
if ((compaction__ == decodeCompactionLevel(((state_0 & 0b11100000) >>> 5 /* get-int EncodedEnum[cache=TStringOpsNodes.RawReadValueNode.cached(..., CompactionLevel cachedCompaction)] */) - 2))) {
Cached1_duplicateFound_ = true;
}
}
if (!Cached1_duplicateFound_) {
// assert ((CompactionLevel.fromStride(arg1Value.stride())) == decodeCompactionLevel(((state_0 & 0b11100000) >>> 5 /* get-int EncodedEnum[cache=TStringOpsNodes.RawReadValueNode.cached(..., CompactionLevel cachedCompaction)] */) - 2));
if (((state_0 & 0b10000)) == 0 /* is-not SpecializationActive[TStringOpsNodes.RawReadValueNode.cached(Node, AbstractTruffleString, Object, int, CompactionLevel, CompactionLevel)] */) {
compaction__ = (CompactionLevel.fromStride(arg1Value.stride()));
state_0 = (state_0 | ((encodeCompactionLevel((compaction__)) + 2) << 5) /* set-int EncodedEnum[cache=TStringOpsNodes.RawReadValueNode.cached(..., CompactionLevel cachedCompaction)] */);
state_0 = state_0 | 0b10000 /* add SpecializationActive[TStringOpsNodes.RawReadValueNode.cached(Node, AbstractTruffleString, Object, int, CompactionLevel, CompactionLevel)] */;
this.state_0_.set(arg0Value, state_0);
Cached1_duplicateFound_ = true;
}
}
if (Cached1_duplicateFound_) {
return RawReadValueNode.cached(arg0Value, arg1Value, arg2Value, arg3Value, compaction__, decodeCompactionLevel(((state_0 & 0b11100000) >>> 5 /* get-int EncodedEnum[cache=TStringOpsNodes.RawReadValueNode.cached(..., CompactionLevel cachedCompaction)] */) - 2));
}
break;
}
}
{
CompactionLevel compaction__ = null;
while (true) {
boolean Cached2_duplicateFound_ = false;
if ((state_0 & 0b100000000) != 0 /* is SpecializationActive[TStringOpsNodes.RawReadValueNode.cached(Node, AbstractTruffleString, Object, int, CompactionLevel, CompactionLevel)] */ && (state_0 & 0b111000000000) >>> 9 /* get-int EncodedEnum[cache=TStringOpsNodes.RawReadValueNode.cached(..., CompactionLevel cachedCompaction)] */ != 0) {
compaction__ = (CompactionLevel.fromStride(arg1Value.stride()));
if ((compaction__ == decodeCompactionLevel(((state_0 & 0b111000000000) >>> 9 /* get-int EncodedEnum[cache=TStringOpsNodes.RawReadValueNode.cached(..., CompactionLevel cachedCompaction)] */) - 2))) {
Cached2_duplicateFound_ = true;
}
}
if (!Cached2_duplicateFound_) {
// assert ((CompactionLevel.fromStride(arg1Value.stride())) == decodeCompactionLevel(((state_0 & 0b111000000000) >>> 9 /* get-int EncodedEnum[cache=TStringOpsNodes.RawReadValueNode.cached(..., CompactionLevel cachedCompaction)] */) - 2));
if (((state_0 & 0b100000000)) == 0 /* is-not SpecializationActive[TStringOpsNodes.RawReadValueNode.cached(Node, AbstractTruffleString, Object, int, CompactionLevel, CompactionLevel)] */) {
compaction__ = (CompactionLevel.fromStride(arg1Value.stride()));
state_0 = (state_0 | ((encodeCompactionLevel((compaction__)) + 2) << 9) /* set-int EncodedEnum[cache=TStringOpsNodes.RawReadValueNode.cached(..., CompactionLevel cachedCompaction)] */);
state_0 = state_0 | 0b100000000 /* add SpecializationActive[TStringOpsNodes.RawReadValueNode.cached(Node, AbstractTruffleString, Object, int, CompactionLevel, CompactionLevel)] */;
this.state_0_.set(arg0Value, state_0);
Cached2_duplicateFound_ = true;
}
}
if (Cached2_duplicateFound_) {
return RawReadValueNode.cached(arg0Value, arg1Value, arg2Value, arg3Value, compaction__, decodeCompactionLevel(((state_0 & 0b111000000000) >>> 9 /* get-int EncodedEnum[cache=TStringOpsNodes.RawReadValueNode.cached(..., CompactionLevel cachedCompaction)] */) - 2));
}
break;
}
}
throw new UnsupportedSpecializationException(this, new Node[] {null, null, null, null}, arg0Value, arg1Value, arg2Value, arg3Value);
}
@Override
public boolean isAdoptable() {
return false;
}
}
@GeneratedBy(RawReadValueNode.class)
@DenyReplace
private static final class Uncached extends RawReadValueNode {
@TruffleBoundary
@Override
int execute(Node arg0Value, AbstractTruffleString arg1Value, Object arg2Value, int arg3Value) {
// assert ((CompactionLevel.fromStride(arg1Value.stride())) == ((CompactionLevel.fromStride(arg1Value.stride()))));
return RawReadValueNode.cached(arg0Value, arg1Value, arg2Value, arg3Value, (CompactionLevel.fromStride(arg1Value.stride())), ((CompactionLevel.fromStride(arg1Value.stride()))));
}
@Override
public NodeCost getCost() {
return NodeCost.MEGAMORPHIC;
}
@Override
public boolean isAdoptable() {
return false;
}
}
}
/**
* Debug Info:
* Specialization {@link IndexOfAnyCharNode#stride0}
* Activation probability: 0.48333
* With/without class size: 9/0 bytes
* Specialization {@link IndexOfAnyCharNode#stride0MultiValue}
* Activation probability: 0.33333
* With/without class size: 8/0 bytes
* Specialization {@link IndexOfAnyCharNode#stride1}
* Activation probability: 0.18333
* With/without class size: 6/0 bytes
*
*/
@GeneratedBy(IndexOfAnyCharNode.class)
@SuppressWarnings("javadoc")
static final class IndexOfAnyCharNodeGen {
private static final Uncached UNCACHED = new Uncached();
@NeverDefault
public static IndexOfAnyCharNode getUncached() {
return IndexOfAnyCharNodeGen.UNCACHED;
}
/**
* Required Fields:
* - {@link Inlined#state_0_}
*
*/
@NeverDefault
public static IndexOfAnyCharNode inline(@RequiredField(bits = 3, value = StateField.class) InlineTarget target) {
return new IndexOfAnyCharNodeGen.Inlined(target);
}
@GeneratedBy(IndexOfAnyCharNode.class)
@DenyReplace
private static final class Inlined extends IndexOfAnyCharNode {
/**
* State Info:
* 0: SpecializationActive {@link IndexOfAnyCharNode#stride0}
* 1: SpecializationActive {@link IndexOfAnyCharNode#stride0MultiValue}
* 2: SpecializationActive {@link IndexOfAnyCharNode#stride1}
*
*/
private final StateField state_0_;
private Inlined(InlineTarget target) {
assert target.getTargetClass().isAssignableFrom(IndexOfAnyCharNode.class);
this.state_0_ = target.getState(0, 3);
}
@Override
int execute(Node arg0Value, AbstractTruffleString arg1Value, Object arg2Value, int arg3Value, int arg4Value, char[] arg5Value) {
int state_0 = this.state_0_.get(arg0Value);
if (state_0 != 0 /* is SpecializationActive[TStringOpsNodes.IndexOfAnyCharNode.stride0(AbstractTruffleString, Object, int, int, char[])] || SpecializationActive[TStringOpsNodes.IndexOfAnyCharNode.stride0MultiValue(AbstractTruffleString, Object, int, int, char[])] || SpecializationActive[TStringOpsNodes.IndexOfAnyCharNode.stride1(AbstractTruffleString, Object, int, int, char[])] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[TStringOpsNodes.IndexOfAnyCharNode.stride0(AbstractTruffleString, Object, int, int, char[])] */) {
if ((TStringGuards.isStride0(arg1Value)) && (arg5Value.length == 1)) {
return stride0(arg1Value, arg2Value, arg3Value, arg4Value, arg5Value);
}
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[TStringOpsNodes.IndexOfAnyCharNode.stride0MultiValue(AbstractTruffleString, Object, int, int, char[])] */) {
if ((TStringGuards.isStride0(arg1Value)) && (arg5Value.length > 1)) {
return stride0MultiValue(arg1Value, arg2Value, arg3Value, arg4Value, arg5Value);
}
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[TStringOpsNodes.IndexOfAnyCharNode.stride1(AbstractTruffleString, Object, int, int, char[])] */) {
if ((TStringGuards.isStride1(arg1Value))) {
return stride1(arg1Value, arg2Value, arg3Value, arg4Value, arg5Value);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value, arg1Value, arg2Value, arg3Value, arg4Value, arg5Value);
}
private int executeAndSpecialize(Node arg0Value, AbstractTruffleString arg1Value, Object arg2Value, int arg3Value, int arg4Value, char[] arg5Value) {
int state_0 = this.state_0_.get(arg0Value);
if ((TStringGuards.isStride0(arg1Value)) && (arg5Value.length == 1)) {
state_0 = state_0 | 0b1 /* add SpecializationActive[TStringOpsNodes.IndexOfAnyCharNode.stride0(AbstractTruffleString, Object, int, int, char[])] */;
this.state_0_.set(arg0Value, state_0);
return stride0(arg1Value, arg2Value, arg3Value, arg4Value, arg5Value);
}
if ((TStringGuards.isStride0(arg1Value)) && (arg5Value.length > 1)) {
state_0 = state_0 | 0b10 /* add SpecializationActive[TStringOpsNodes.IndexOfAnyCharNode.stride0MultiValue(AbstractTruffleString, Object, int, int, char[])] */;
this.state_0_.set(arg0Value, state_0);
return stride0MultiValue(arg1Value, arg2Value, arg3Value, arg4Value, arg5Value);
}
if ((TStringGuards.isStride1(arg1Value))) {
state_0 = state_0 | 0b100 /* add SpecializationActive[TStringOpsNodes.IndexOfAnyCharNode.stride1(AbstractTruffleString, Object, int, int, char[])] */;
this.state_0_.set(arg0Value, state_0);
return stride1(arg1Value, arg2Value, arg3Value, arg4Value, arg5Value);
}
throw new UnsupportedSpecializationException(this, new Node[] {null, null, null, null, null, null}, arg0Value, arg1Value, arg2Value, arg3Value, arg4Value, arg5Value);
}
@Override
public boolean isAdoptable() {
return false;
}
}
@GeneratedBy(IndexOfAnyCharNode.class)
@DenyReplace
private static final class Uncached extends IndexOfAnyCharNode {
@TruffleBoundary
@Override
int execute(Node arg0Value, AbstractTruffleString arg1Value, Object arg2Value, int arg3Value, int arg4Value, char[] arg5Value) {
if ((TStringGuards.isStride0(arg1Value)) && (arg5Value.length == 1)) {
return stride0(arg1Value, arg2Value, arg3Value, arg4Value, arg5Value);
}
if ((TStringGuards.isStride0(arg1Value)) && (arg5Value.length > 1)) {
return stride0MultiValue(arg1Value, arg2Value, arg3Value, arg4Value, arg5Value);
}
if ((TStringGuards.isStride1(arg1Value))) {
return stride1(arg1Value, arg2Value, arg3Value, arg4Value, arg5Value);
}
throw new UnsupportedSpecializationException(this, new Node[] {null, null, null, null, null, null}, arg0Value, arg1Value, arg2Value, arg3Value, arg4Value, arg5Value);
}
@Override
public NodeCost getCost() {
return NodeCost.MEGAMORPHIC;
}
@Override
public boolean isAdoptable() {
return false;
}
}
}
/**
* Debug Info:
* Specialization {@link IndexOfAnyIntNode#stride0}
* Activation probability: 0.32000
* With/without class size: 7/0 bytes
* Specialization {@link IndexOfAnyIntNode#stride0MultiValue}
* Activation probability: 0.26000
* With/without class size: 7/0 bytes
* Specialization {@link IndexOfAnyIntNode#stride1}
* Activation probability: 0.20000
* With/without class size: 6/0 bytes
* Specialization {@link IndexOfAnyIntNode#stride1MultiValue}
* Activation probability: 0.14000
* With/without class size: 5/0 bytes
* Specialization {@link IndexOfAnyIntNode#stride2}
* Activation probability: 0.08000
* With/without class size: 4/0 bytes
*
*/
@GeneratedBy(IndexOfAnyIntNode.class)
@SuppressWarnings("javadoc")
static final class IndexOfAnyIntNodeGen {
private static final Uncached UNCACHED = new Uncached();
@NeverDefault
public static IndexOfAnyIntNode getUncached() {
return IndexOfAnyIntNodeGen.UNCACHED;
}
/**
* Required Fields:
* - {@link Inlined#state_0_}
*
*/
@NeverDefault
public static IndexOfAnyIntNode inline(@RequiredField(bits = 5, value = StateField.class) InlineTarget target) {
return new IndexOfAnyIntNodeGen.Inlined(target);
}
@GeneratedBy(IndexOfAnyIntNode.class)
@DenyReplace
private static final class Inlined extends IndexOfAnyIntNode {
/**
* State Info:
* 0: SpecializationActive {@link IndexOfAnyIntNode#stride0}
* 1: SpecializationActive {@link IndexOfAnyIntNode#stride0MultiValue}
* 2: SpecializationActive {@link IndexOfAnyIntNode#stride1}
* 3: SpecializationActive {@link IndexOfAnyIntNode#stride1MultiValue}
* 4: SpecializationActive {@link IndexOfAnyIntNode#stride2}
*
*/
private final StateField state_0_;
private Inlined(InlineTarget target) {
assert target.getTargetClass().isAssignableFrom(IndexOfAnyIntNode.class);
this.state_0_ = target.getState(0, 5);
}
@Override
int execute(Node arg0Value, AbstractTruffleString arg1Value, Object arg2Value, int arg3Value, int arg4Value, int[] arg5Value) {
int state_0 = this.state_0_.get(arg0Value);
if (state_0 != 0 /* is SpecializationActive[TStringOpsNodes.IndexOfAnyIntNode.stride0(AbstractTruffleString, Object, int, int, int[])] || SpecializationActive[TStringOpsNodes.IndexOfAnyIntNode.stride0MultiValue(AbstractTruffleString, Object, int, int, int[])] || SpecializationActive[TStringOpsNodes.IndexOfAnyIntNode.stride1(AbstractTruffleString, Object, int, int, int[])] || SpecializationActive[TStringOpsNodes.IndexOfAnyIntNode.stride1MultiValue(AbstractTruffleString, Object, int, int, int[])] || SpecializationActive[TStringOpsNodes.IndexOfAnyIntNode.stride2(AbstractTruffleString, Object, int, int, int[])] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[TStringOpsNodes.IndexOfAnyIntNode.stride0(AbstractTruffleString, Object, int, int, int[])] */) {
if ((TStringGuards.isStride0(arg1Value)) && (arg5Value.length == 1)) {
return stride0(arg1Value, arg2Value, arg3Value, arg4Value, arg5Value);
}
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[TStringOpsNodes.IndexOfAnyIntNode.stride0MultiValue(AbstractTruffleString, Object, int, int, int[])] */) {
if ((TStringGuards.isStride0(arg1Value)) && (arg5Value.length > 1)) {
return stride0MultiValue(arg1Value, arg2Value, arg3Value, arg4Value, arg5Value);
}
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[TStringOpsNodes.IndexOfAnyIntNode.stride1(AbstractTruffleString, Object, int, int, int[])] */) {
if ((TStringGuards.isStride1(arg1Value)) && (arg5Value.length == 1)) {
return stride1(arg1Value, arg2Value, arg3Value, arg4Value, arg5Value);
}
}
if ((state_0 & 0b1000) != 0 /* is SpecializationActive[TStringOpsNodes.IndexOfAnyIntNode.stride1MultiValue(AbstractTruffleString, Object, int, int, int[])] */) {
if ((TStringGuards.isStride1(arg1Value)) && (arg5Value.length > 1)) {
return stride1MultiValue(arg1Value, arg2Value, arg3Value, arg4Value, arg5Value);
}
}
if ((state_0 & 0b10000) != 0 /* is SpecializationActive[TStringOpsNodes.IndexOfAnyIntNode.stride2(AbstractTruffleString, Object, int, int, int[])] */) {
if ((TStringGuards.isStride2(arg1Value))) {
return stride2(arg1Value, arg2Value, arg3Value, arg4Value, arg5Value);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value, arg1Value, arg2Value, arg3Value, arg4Value, arg5Value);
}
private int executeAndSpecialize(Node arg0Value, AbstractTruffleString arg1Value, Object arg2Value, int arg3Value, int arg4Value, int[] arg5Value) {
int state_0 = this.state_0_.get(arg0Value);
if ((TStringGuards.isStride0(arg1Value)) && (arg5Value.length == 1)) {
state_0 = state_0 | 0b1 /* add SpecializationActive[TStringOpsNodes.IndexOfAnyIntNode.stride0(AbstractTruffleString, Object, int, int, int[])] */;
this.state_0_.set(arg0Value, state_0);
return stride0(arg1Value, arg2Value, arg3Value, arg4Value, arg5Value);
}
if ((TStringGuards.isStride0(arg1Value)) && (arg5Value.length > 1)) {
state_0 = state_0 | 0b10 /* add SpecializationActive[TStringOpsNodes.IndexOfAnyIntNode.stride0MultiValue(AbstractTruffleString, Object, int, int, int[])] */;
this.state_0_.set(arg0Value, state_0);
return stride0MultiValue(arg1Value, arg2Value, arg3Value, arg4Value, arg5Value);
}
if ((TStringGuards.isStride1(arg1Value)) && (arg5Value.length == 1)) {
state_0 = state_0 | 0b100 /* add SpecializationActive[TStringOpsNodes.IndexOfAnyIntNode.stride1(AbstractTruffleString, Object, int, int, int[])] */;
this.state_0_.set(arg0Value, state_0);
return stride1(arg1Value, arg2Value, arg3Value, arg4Value, arg5Value);
}
if ((TStringGuards.isStride1(arg1Value)) && (arg5Value.length > 1)) {
state_0 = state_0 | 0b1000 /* add SpecializationActive[TStringOpsNodes.IndexOfAnyIntNode.stride1MultiValue(AbstractTruffleString, Object, int, int, int[])] */;
this.state_0_.set(arg0Value, state_0);
return stride1MultiValue(arg1Value, arg2Value, arg3Value, arg4Value, arg5Value);
}
if ((TStringGuards.isStride2(arg1Value))) {
state_0 = state_0 | 0b10000 /* add SpecializationActive[TStringOpsNodes.IndexOfAnyIntNode.stride2(AbstractTruffleString, Object, int, int, int[])] */;
this.state_0_.set(arg0Value, state_0);
return stride2(arg1Value, arg2Value, arg3Value, arg4Value, arg5Value);
}
throw new UnsupportedSpecializationException(this, new Node[] {null, null, null, null, null, null}, arg0Value, arg1Value, arg2Value, arg3Value, arg4Value, arg5Value);
}
@Override
public boolean isAdoptable() {
return false;
}
}
@GeneratedBy(IndexOfAnyIntNode.class)
@DenyReplace
private static final class Uncached extends IndexOfAnyIntNode {
@TruffleBoundary
@Override
int execute(Node arg0Value, AbstractTruffleString arg1Value, Object arg2Value, int arg3Value, int arg4Value, int[] arg5Value) {
if ((TStringGuards.isStride0(arg1Value)) && (arg5Value.length == 1)) {
return stride0(arg1Value, arg2Value, arg3Value, arg4Value, arg5Value);
}
if ((TStringGuards.isStride0(arg1Value)) && (arg5Value.length > 1)) {
return stride0MultiValue(arg1Value, arg2Value, arg3Value, arg4Value, arg5Value);
}
if ((TStringGuards.isStride1(arg1Value)) && (arg5Value.length == 1)) {
return stride1(arg1Value, arg2Value, arg3Value, arg4Value, arg5Value);
}
if ((TStringGuards.isStride1(arg1Value)) && (arg5Value.length > 1)) {
return stride1MultiValue(arg1Value, arg2Value, arg3Value, arg4Value, arg5Value);
}
if ((TStringGuards.isStride2(arg1Value))) {
return stride2(arg1Value, arg2Value, arg3Value, arg4Value, arg5Value);
}
throw new UnsupportedSpecializationException(this, new Node[] {null, null, null, null, null, null}, arg0Value, arg1Value, arg2Value, arg3Value, arg4Value, arg5Value);
}
@Override
public NodeCost getCost() {
return NodeCost.MEGAMORPHIC;
}
@Override
public boolean isAdoptable() {
return false;
}
}
}
/**
* Debug Info:
* Specialization {@link RawIndexOfCodePointNode#cached}
* Activation probability: 0.48333
* With/without class size: 11/4 bytes
* Specialization {@link RawIndexOfCodePointNode#cached}
* Activation probability: 0.33333
* With/without class size: 9/4 bytes
* Specialization {@link RawIndexOfCodePointNode#cached}
* Activation probability: 0.18333
* With/without class size: 6/4 bytes
*
*/
@GeneratedBy(RawIndexOfCodePointNode.class)
@SuppressWarnings("javadoc")
static final class RawIndexOfCodePointNodeGen {
private static final Uncached UNCACHED = new Uncached();
@NeverDefault
public static RawIndexOfCodePointNode getUncached() {
return RawIndexOfCodePointNodeGen.UNCACHED;
}
/**
* Required Fields:
* - {@link Inlined#state_0_}
*
*/
@NeverDefault
public static RawIndexOfCodePointNode inline(@RequiredField(bits = 12, value = StateField.class) InlineTarget target) {
return new RawIndexOfCodePointNodeGen.Inlined(target);
}
@GeneratedBy(RawIndexOfCodePointNode.class)
@DenyReplace
private static final class Inlined extends RawIndexOfCodePointNode {
/**
* State Info:
* 0: SpecializationActive {@link RawIndexOfCodePointNode#cached}
* 1-3: EncodedEnum[cache=TStringOpsNodes.RawIndexOfCodePointNode.cached(..., CompactionLevel cachedCompaction)]
* 4: SpecializationActive {@link RawIndexOfCodePointNode#cached}
* 5-7: EncodedEnum[cache=TStringOpsNodes.RawIndexOfCodePointNode.cached(..., CompactionLevel cachedCompaction)]
* 8: SpecializationActive {@link RawIndexOfCodePointNode#cached}
* 9-11: EncodedEnum[cache=TStringOpsNodes.RawIndexOfCodePointNode.cached(..., CompactionLevel cachedCompaction)]
*
*/
private final StateField state_0_;
private Inlined(InlineTarget target) {
assert target.getTargetClass().isAssignableFrom(RawIndexOfCodePointNode.class);
this.state_0_ = target.getState(0, 12);
}
@Override
int execute(Node arg0Value, AbstractTruffleString arg1Value, Object arg2Value, int arg3Value, int arg4Value, int arg5Value) {
int state_0 = this.state_0_.get(arg0Value);
if ((state_0 & 0b100010001) != 0 /* is SpecializationActive[TStringOpsNodes.RawIndexOfCodePointNode.cached(Node, AbstractTruffleString, Object, int, int, int, CompactionLevel, CompactionLevel)] || SpecializationActive[TStringOpsNodes.RawIndexOfCodePointNode.cached(Node, AbstractTruffleString, Object, int, int, int, CompactionLevel, CompactionLevel)] || SpecializationActive[TStringOpsNodes.RawIndexOfCodePointNode.cached(Node, AbstractTruffleString, Object, int, int, int, CompactionLevel, CompactionLevel)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[TStringOpsNodes.RawIndexOfCodePointNode.cached(Node, AbstractTruffleString, Object, int, int, int, CompactionLevel, CompactionLevel)] */) {
if ((state_0 & 0b1110) >>> 1 /* get-int EncodedEnum[cache=TStringOpsNodes.RawIndexOfCodePointNode.cached(..., CompactionLevel cachedCompaction)] */ != 0) {
CompactionLevel compaction__ = (CompactionLevel.fromStride(arg1Value.stride()));
if ((compaction__ == decodeCompactionLevel(((state_0 & 0b1110) >>> 1 /* get-int EncodedEnum[cache=TStringOpsNodes.RawIndexOfCodePointNode.cached(..., CompactionLevel cachedCompaction)] */) - 2))) {
return RawIndexOfCodePointNode.cached(arg0Value, arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, compaction__, decodeCompactionLevel(((state_0 & 0b1110) >>> 1 /* get-int EncodedEnum[cache=TStringOpsNodes.RawIndexOfCodePointNode.cached(..., CompactionLevel cachedCompaction)] */) - 2));
}
}
}
if ((state_0 & 0b10000) != 0 /* is SpecializationActive[TStringOpsNodes.RawIndexOfCodePointNode.cached(Node, AbstractTruffleString, Object, int, int, int, CompactionLevel, CompactionLevel)] */) {
if ((state_0 & 0b11100000) >>> 5 /* get-int EncodedEnum[cache=TStringOpsNodes.RawIndexOfCodePointNode.cached(..., CompactionLevel cachedCompaction)] */ != 0) {
CompactionLevel compaction__ = (CompactionLevel.fromStride(arg1Value.stride()));
if ((compaction__ == decodeCompactionLevel(((state_0 & 0b11100000) >>> 5 /* get-int EncodedEnum[cache=TStringOpsNodes.RawIndexOfCodePointNode.cached(..., CompactionLevel cachedCompaction)] */) - 2))) {
return RawIndexOfCodePointNode.cached(arg0Value, arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, compaction__, decodeCompactionLevel(((state_0 & 0b11100000) >>> 5 /* get-int EncodedEnum[cache=TStringOpsNodes.RawIndexOfCodePointNode.cached(..., CompactionLevel cachedCompaction)] */) - 2));
}
}
}
if ((state_0 & 0b100000000) != 0 /* is SpecializationActive[TStringOpsNodes.RawIndexOfCodePointNode.cached(Node, AbstractTruffleString, Object, int, int, int, CompactionLevel, CompactionLevel)] */) {
if ((state_0 & 0b111000000000) >>> 9 /* get-int EncodedEnum[cache=TStringOpsNodes.RawIndexOfCodePointNode.cached(..., CompactionLevel cachedCompaction)] */ != 0) {
CompactionLevel compaction__ = (CompactionLevel.fromStride(arg1Value.stride()));
if ((compaction__ == decodeCompactionLevel(((state_0 & 0b111000000000) >>> 9 /* get-int EncodedEnum[cache=TStringOpsNodes.RawIndexOfCodePointNode.cached(..., CompactionLevel cachedCompaction)] */) - 2))) {
return RawIndexOfCodePointNode.cached(arg0Value, arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, compaction__, decodeCompactionLevel(((state_0 & 0b111000000000) >>> 9 /* get-int EncodedEnum[cache=TStringOpsNodes.RawIndexOfCodePointNode.cached(..., CompactionLevel cachedCompaction)] */) - 2));
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value, arg1Value, arg2Value, arg3Value, arg4Value, arg5Value);
}
private int executeAndSpecialize(Node arg0Value, AbstractTruffleString arg1Value, Object arg2Value, int arg3Value, int arg4Value, int arg5Value) {
int state_0 = this.state_0_.get(arg0Value);
{
CompactionLevel compaction__ = null;
while (true) {
boolean Cached0_duplicateFound_ = false;
if ((state_0 & 0b1) != 0 /* is SpecializationActive[TStringOpsNodes.RawIndexOfCodePointNode.cached(Node, AbstractTruffleString, Object, int, int, int, CompactionLevel, CompactionLevel)] */ && (state_0 & 0b1110) >>> 1 /* get-int EncodedEnum[cache=TStringOpsNodes.RawIndexOfCodePointNode.cached(..., CompactionLevel cachedCompaction)] */ != 0) {
compaction__ = (CompactionLevel.fromStride(arg1Value.stride()));
if ((compaction__ == decodeCompactionLevel(((state_0 & 0b1110) >>> 1 /* get-int EncodedEnum[cache=TStringOpsNodes.RawIndexOfCodePointNode.cached(..., CompactionLevel cachedCompaction)] */) - 2))) {
Cached0_duplicateFound_ = true;
}
}
if (!Cached0_duplicateFound_) {
// assert ((CompactionLevel.fromStride(arg1Value.stride())) == decodeCompactionLevel(((state_0 & 0b1110) >>> 1 /* get-int EncodedEnum[cache=TStringOpsNodes.RawIndexOfCodePointNode.cached(..., CompactionLevel cachedCompaction)] */) - 2));
if (((state_0 & 0b1)) == 0 /* is-not SpecializationActive[TStringOpsNodes.RawIndexOfCodePointNode.cached(Node, AbstractTruffleString, Object, int, int, int, CompactionLevel, CompactionLevel)] */) {
compaction__ = (CompactionLevel.fromStride(arg1Value.stride()));
state_0 = (state_0 | ((encodeCompactionLevel((compaction__)) + 2) << 1) /* set-int EncodedEnum[cache=TStringOpsNodes.RawIndexOfCodePointNode.cached(..., CompactionLevel cachedCompaction)] */);
state_0 = state_0 | 0b1 /* add SpecializationActive[TStringOpsNodes.RawIndexOfCodePointNode.cached(Node, AbstractTruffleString, Object, int, int, int, CompactionLevel, CompactionLevel)] */;
this.state_0_.set(arg0Value, state_0);
Cached0_duplicateFound_ = true;
}
}
if (Cached0_duplicateFound_) {
return RawIndexOfCodePointNode.cached(arg0Value, arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, compaction__, decodeCompactionLevel(((state_0 & 0b1110) >>> 1 /* get-int EncodedEnum[cache=TStringOpsNodes.RawIndexOfCodePointNode.cached(..., CompactionLevel cachedCompaction)] */) - 2));
}
break;
}
}
{
CompactionLevel compaction__ = null;
while (true) {
boolean Cached1_duplicateFound_ = false;
if ((state_0 & 0b10000) != 0 /* is SpecializationActive[TStringOpsNodes.RawIndexOfCodePointNode.cached(Node, AbstractTruffleString, Object, int, int, int, CompactionLevel, CompactionLevel)] */ && (state_0 & 0b11100000) >>> 5 /* get-int EncodedEnum[cache=TStringOpsNodes.RawIndexOfCodePointNode.cached(..., CompactionLevel cachedCompaction)] */ != 0) {
compaction__ = (CompactionLevel.fromStride(arg1Value.stride()));
if ((compaction__ == decodeCompactionLevel(((state_0 & 0b11100000) >>> 5 /* get-int EncodedEnum[cache=TStringOpsNodes.RawIndexOfCodePointNode.cached(..., CompactionLevel cachedCompaction)] */) - 2))) {
Cached1_duplicateFound_ = true;
}
}
if (!Cached1_duplicateFound_) {
// assert ((CompactionLevel.fromStride(arg1Value.stride())) == decodeCompactionLevel(((state_0 & 0b11100000) >>> 5 /* get-int EncodedEnum[cache=TStringOpsNodes.RawIndexOfCodePointNode.cached(..., CompactionLevel cachedCompaction)] */) - 2));
if (((state_0 & 0b10000)) == 0 /* is-not SpecializationActive[TStringOpsNodes.RawIndexOfCodePointNode.cached(Node, AbstractTruffleString, Object, int, int, int, CompactionLevel, CompactionLevel)] */) {
compaction__ = (CompactionLevel.fromStride(arg1Value.stride()));
state_0 = (state_0 | ((encodeCompactionLevel((compaction__)) + 2) << 5) /* set-int EncodedEnum[cache=TStringOpsNodes.RawIndexOfCodePointNode.cached(..., CompactionLevel cachedCompaction)] */);
state_0 = state_0 | 0b10000 /* add SpecializationActive[TStringOpsNodes.RawIndexOfCodePointNode.cached(Node, AbstractTruffleString, Object, int, int, int, CompactionLevel, CompactionLevel)] */;
this.state_0_.set(arg0Value, state_0);
Cached1_duplicateFound_ = true;
}
}
if (Cached1_duplicateFound_) {
return RawIndexOfCodePointNode.cached(arg0Value, arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, compaction__, decodeCompactionLevel(((state_0 & 0b11100000) >>> 5 /* get-int EncodedEnum[cache=TStringOpsNodes.RawIndexOfCodePointNode.cached(..., CompactionLevel cachedCompaction)] */) - 2));
}
break;
}
}
{
CompactionLevel compaction__ = null;
while (true) {
boolean Cached2_duplicateFound_ = false;
if ((state_0 & 0b100000000) != 0 /* is SpecializationActive[TStringOpsNodes.RawIndexOfCodePointNode.cached(Node, AbstractTruffleString, Object, int, int, int, CompactionLevel, CompactionLevel)] */ && (state_0 & 0b111000000000) >>> 9 /* get-int EncodedEnum[cache=TStringOpsNodes.RawIndexOfCodePointNode.cached(..., CompactionLevel cachedCompaction)] */ != 0) {
compaction__ = (CompactionLevel.fromStride(arg1Value.stride()));
if ((compaction__ == decodeCompactionLevel(((state_0 & 0b111000000000) >>> 9 /* get-int EncodedEnum[cache=TStringOpsNodes.RawIndexOfCodePointNode.cached(..., CompactionLevel cachedCompaction)] */) - 2))) {
Cached2_duplicateFound_ = true;
}
}
if (!Cached2_duplicateFound_) {
// assert ((CompactionLevel.fromStride(arg1Value.stride())) == decodeCompactionLevel(((state_0 & 0b111000000000) >>> 9 /* get-int EncodedEnum[cache=TStringOpsNodes.RawIndexOfCodePointNode.cached(..., CompactionLevel cachedCompaction)] */) - 2));
if (((state_0 & 0b100000000)) == 0 /* is-not SpecializationActive[TStringOpsNodes.RawIndexOfCodePointNode.cached(Node, AbstractTruffleString, Object, int, int, int, CompactionLevel, CompactionLevel)] */) {
compaction__ = (CompactionLevel.fromStride(arg1Value.stride()));
state_0 = (state_0 | ((encodeCompactionLevel((compaction__)) + 2) << 9) /* set-int EncodedEnum[cache=TStringOpsNodes.RawIndexOfCodePointNode.cached(..., CompactionLevel cachedCompaction)] */);
state_0 = state_0 | 0b100000000 /* add SpecializationActive[TStringOpsNodes.RawIndexOfCodePointNode.cached(Node, AbstractTruffleString, Object, int, int, int, CompactionLevel, CompactionLevel)] */;
this.state_0_.set(arg0Value, state_0);
Cached2_duplicateFound_ = true;
}
}
if (Cached2_duplicateFound_) {
return RawIndexOfCodePointNode.cached(arg0Value, arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, compaction__, decodeCompactionLevel(((state_0 & 0b111000000000) >>> 9 /* get-int EncodedEnum[cache=TStringOpsNodes.RawIndexOfCodePointNode.cached(..., CompactionLevel cachedCompaction)] */) - 2));
}
break;
}
}
throw new UnsupportedSpecializationException(this, new Node[] {null, null, null, null, null, null}, arg0Value, arg1Value, arg2Value, arg3Value, arg4Value, arg5Value);
}
@Override
public boolean isAdoptable() {
return false;
}
}
@GeneratedBy(RawIndexOfCodePointNode.class)
@DenyReplace
private static final class Uncached extends RawIndexOfCodePointNode {
@TruffleBoundary
@Override
int execute(Node arg0Value, AbstractTruffleString arg1Value, Object arg2Value, int arg3Value, int arg4Value, int arg5Value) {
// assert ((CompactionLevel.fromStride(arg1Value.stride())) == ((CompactionLevel.fromStride(arg1Value.stride()))));
return RawIndexOfCodePointNode.cached(arg0Value, arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, (CompactionLevel.fromStride(arg1Value.stride())), ((CompactionLevel.fromStride(arg1Value.stride()))));
}
@Override
public NodeCost getCost() {
return NodeCost.MEGAMORPHIC;
}
@Override
public boolean isAdoptable() {
return false;
}
}
}
/**
* Debug Info:
* Specialization {@link RawLastIndexOfCodePointNode#cached}
* Activation probability: 0.48333
* With/without class size: 11/4 bytes
* Specialization {@link RawLastIndexOfCodePointNode#cached}
* Activation probability: 0.33333
* With/without class size: 9/4 bytes
* Specialization {@link RawLastIndexOfCodePointNode#cached}
* Activation probability: 0.18333
* With/without class size: 6/4 bytes
*
*/
@GeneratedBy(RawLastIndexOfCodePointNode.class)
@SuppressWarnings("javadoc")
static final class RawLastIndexOfCodePointNodeGen {
private static final Uncached UNCACHED = new Uncached();
@NeverDefault
public static RawLastIndexOfCodePointNode getUncached() {
return RawLastIndexOfCodePointNodeGen.UNCACHED;
}
/**
* Required Fields:
* - {@link Inlined#state_0_}
*
*/
@NeverDefault
public static RawLastIndexOfCodePointNode inline(@RequiredField(bits = 12, value = StateField.class) InlineTarget target) {
return new RawLastIndexOfCodePointNodeGen.Inlined(target);
}
@GeneratedBy(RawLastIndexOfCodePointNode.class)
@DenyReplace
private static final class Inlined extends RawLastIndexOfCodePointNode {
/**
* State Info:
* 0: SpecializationActive {@link RawLastIndexOfCodePointNode#cached}
* 1-3: EncodedEnum[cache=TStringOpsNodes.RawLastIndexOfCodePointNode.cached(..., CompactionLevel cachedCompaction)]
* 4: SpecializationActive {@link RawLastIndexOfCodePointNode#cached}
* 5-7: EncodedEnum[cache=TStringOpsNodes.RawLastIndexOfCodePointNode.cached(..., CompactionLevel cachedCompaction)]
* 8: SpecializationActive {@link RawLastIndexOfCodePointNode#cached}
* 9-11: EncodedEnum[cache=TStringOpsNodes.RawLastIndexOfCodePointNode.cached(..., CompactionLevel cachedCompaction)]
*
*/
private final StateField state_0_;
private Inlined(InlineTarget target) {
assert target.getTargetClass().isAssignableFrom(RawLastIndexOfCodePointNode.class);
this.state_0_ = target.getState(0, 12);
}
@Override
int execute(Node arg0Value, AbstractTruffleString arg1Value, Object arg2Value, int arg3Value, int arg4Value, int arg5Value) {
int state_0 = this.state_0_.get(arg0Value);
if ((state_0 & 0b100010001) != 0 /* is SpecializationActive[TStringOpsNodes.RawLastIndexOfCodePointNode.cached(Node, AbstractTruffleString, Object, int, int, int, CompactionLevel, CompactionLevel)] || SpecializationActive[TStringOpsNodes.RawLastIndexOfCodePointNode.cached(Node, AbstractTruffleString, Object, int, int, int, CompactionLevel, CompactionLevel)] || SpecializationActive[TStringOpsNodes.RawLastIndexOfCodePointNode.cached(Node, AbstractTruffleString, Object, int, int, int, CompactionLevel, CompactionLevel)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[TStringOpsNodes.RawLastIndexOfCodePointNode.cached(Node, AbstractTruffleString, Object, int, int, int, CompactionLevel, CompactionLevel)] */) {
if ((state_0 & 0b1110) >>> 1 /* get-int EncodedEnum[cache=TStringOpsNodes.RawLastIndexOfCodePointNode.cached(..., CompactionLevel cachedCompaction)] */ != 0) {
CompactionLevel compaction__ = (CompactionLevel.fromStride(arg1Value.stride()));
if ((compaction__ == decodeCompactionLevel(((state_0 & 0b1110) >>> 1 /* get-int EncodedEnum[cache=TStringOpsNodes.RawLastIndexOfCodePointNode.cached(..., CompactionLevel cachedCompaction)] */) - 2))) {
return RawLastIndexOfCodePointNode.cached(arg0Value, arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, compaction__, decodeCompactionLevel(((state_0 & 0b1110) >>> 1 /* get-int EncodedEnum[cache=TStringOpsNodes.RawLastIndexOfCodePointNode.cached(..., CompactionLevel cachedCompaction)] */) - 2));
}
}
}
if ((state_0 & 0b10000) != 0 /* is SpecializationActive[TStringOpsNodes.RawLastIndexOfCodePointNode.cached(Node, AbstractTruffleString, Object, int, int, int, CompactionLevel, CompactionLevel)] */) {
if ((state_0 & 0b11100000) >>> 5 /* get-int EncodedEnum[cache=TStringOpsNodes.RawLastIndexOfCodePointNode.cached(..., CompactionLevel cachedCompaction)] */ != 0) {
CompactionLevel compaction__ = (CompactionLevel.fromStride(arg1Value.stride()));
if ((compaction__ == decodeCompactionLevel(((state_0 & 0b11100000) >>> 5 /* get-int EncodedEnum[cache=TStringOpsNodes.RawLastIndexOfCodePointNode.cached(..., CompactionLevel cachedCompaction)] */) - 2))) {
return RawLastIndexOfCodePointNode.cached(arg0Value, arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, compaction__, decodeCompactionLevel(((state_0 & 0b11100000) >>> 5 /* get-int EncodedEnum[cache=TStringOpsNodes.RawLastIndexOfCodePointNode.cached(..., CompactionLevel cachedCompaction)] */) - 2));
}
}
}
if ((state_0 & 0b100000000) != 0 /* is SpecializationActive[TStringOpsNodes.RawLastIndexOfCodePointNode.cached(Node, AbstractTruffleString, Object, int, int, int, CompactionLevel, CompactionLevel)] */) {
if ((state_0 & 0b111000000000) >>> 9 /* get-int EncodedEnum[cache=TStringOpsNodes.RawLastIndexOfCodePointNode.cached(..., CompactionLevel cachedCompaction)] */ != 0) {
CompactionLevel compaction__ = (CompactionLevel.fromStride(arg1Value.stride()));
if ((compaction__ == decodeCompactionLevel(((state_0 & 0b111000000000) >>> 9 /* get-int EncodedEnum[cache=TStringOpsNodes.RawLastIndexOfCodePointNode.cached(..., CompactionLevel cachedCompaction)] */) - 2))) {
return RawLastIndexOfCodePointNode.cached(arg0Value, arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, compaction__, decodeCompactionLevel(((state_0 & 0b111000000000) >>> 9 /* get-int EncodedEnum[cache=TStringOpsNodes.RawLastIndexOfCodePointNode.cached(..., CompactionLevel cachedCompaction)] */) - 2));
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value, arg1Value, arg2Value, arg3Value, arg4Value, arg5Value);
}
private int executeAndSpecialize(Node arg0Value, AbstractTruffleString arg1Value, Object arg2Value, int arg3Value, int arg4Value, int arg5Value) {
int state_0 = this.state_0_.get(arg0Value);
{
CompactionLevel compaction__ = null;
while (true) {
boolean Cached0_duplicateFound_ = false;
if ((state_0 & 0b1) != 0 /* is SpecializationActive[TStringOpsNodes.RawLastIndexOfCodePointNode.cached(Node, AbstractTruffleString, Object, int, int, int, CompactionLevel, CompactionLevel)] */ && (state_0 & 0b1110) >>> 1 /* get-int EncodedEnum[cache=TStringOpsNodes.RawLastIndexOfCodePointNode.cached(..., CompactionLevel cachedCompaction)] */ != 0) {
compaction__ = (CompactionLevel.fromStride(arg1Value.stride()));
if ((compaction__ == decodeCompactionLevel(((state_0 & 0b1110) >>> 1 /* get-int EncodedEnum[cache=TStringOpsNodes.RawLastIndexOfCodePointNode.cached(..., CompactionLevel cachedCompaction)] */) - 2))) {
Cached0_duplicateFound_ = true;
}
}
if (!Cached0_duplicateFound_) {
// assert ((CompactionLevel.fromStride(arg1Value.stride())) == decodeCompactionLevel(((state_0 & 0b1110) >>> 1 /* get-int EncodedEnum[cache=TStringOpsNodes.RawLastIndexOfCodePointNode.cached(..., CompactionLevel cachedCompaction)] */) - 2));
if (((state_0 & 0b1)) == 0 /* is-not SpecializationActive[TStringOpsNodes.RawLastIndexOfCodePointNode.cached(Node, AbstractTruffleString, Object, int, int, int, CompactionLevel, CompactionLevel)] */) {
compaction__ = (CompactionLevel.fromStride(arg1Value.stride()));
state_0 = (state_0 | ((encodeCompactionLevel((compaction__)) + 2) << 1) /* set-int EncodedEnum[cache=TStringOpsNodes.RawLastIndexOfCodePointNode.cached(..., CompactionLevel cachedCompaction)] */);
state_0 = state_0 | 0b1 /* add SpecializationActive[TStringOpsNodes.RawLastIndexOfCodePointNode.cached(Node, AbstractTruffleString, Object, int, int, int, CompactionLevel, CompactionLevel)] */;
this.state_0_.set(arg0Value, state_0);
Cached0_duplicateFound_ = true;
}
}
if (Cached0_duplicateFound_) {
return RawLastIndexOfCodePointNode.cached(arg0Value, arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, compaction__, decodeCompactionLevel(((state_0 & 0b1110) >>> 1 /* get-int EncodedEnum[cache=TStringOpsNodes.RawLastIndexOfCodePointNode.cached(..., CompactionLevel cachedCompaction)] */) - 2));
}
break;
}
}
{
CompactionLevel compaction__ = null;
while (true) {
boolean Cached1_duplicateFound_ = false;
if ((state_0 & 0b10000) != 0 /* is SpecializationActive[TStringOpsNodes.RawLastIndexOfCodePointNode.cached(Node, AbstractTruffleString, Object, int, int, int, CompactionLevel, CompactionLevel)] */ && (state_0 & 0b11100000) >>> 5 /* get-int EncodedEnum[cache=TStringOpsNodes.RawLastIndexOfCodePointNode.cached(..., CompactionLevel cachedCompaction)] */ != 0) {
compaction__ = (CompactionLevel.fromStride(arg1Value.stride()));
if ((compaction__ == decodeCompactionLevel(((state_0 & 0b11100000) >>> 5 /* get-int EncodedEnum[cache=TStringOpsNodes.RawLastIndexOfCodePointNode.cached(..., CompactionLevel cachedCompaction)] */) - 2))) {
Cached1_duplicateFound_ = true;
}
}
if (!Cached1_duplicateFound_) {
// assert ((CompactionLevel.fromStride(arg1Value.stride())) == decodeCompactionLevel(((state_0 & 0b11100000) >>> 5 /* get-int EncodedEnum[cache=TStringOpsNodes.RawLastIndexOfCodePointNode.cached(..., CompactionLevel cachedCompaction)] */) - 2));
if (((state_0 & 0b10000)) == 0 /* is-not SpecializationActive[TStringOpsNodes.RawLastIndexOfCodePointNode.cached(Node, AbstractTruffleString, Object, int, int, int, CompactionLevel, CompactionLevel)] */) {
compaction__ = (CompactionLevel.fromStride(arg1Value.stride()));
state_0 = (state_0 | ((encodeCompactionLevel((compaction__)) + 2) << 5) /* set-int EncodedEnum[cache=TStringOpsNodes.RawLastIndexOfCodePointNode.cached(..., CompactionLevel cachedCompaction)] */);
state_0 = state_0 | 0b10000 /* add SpecializationActive[TStringOpsNodes.RawLastIndexOfCodePointNode.cached(Node, AbstractTruffleString, Object, int, int, int, CompactionLevel, CompactionLevel)] */;
this.state_0_.set(arg0Value, state_0);
Cached1_duplicateFound_ = true;
}
}
if (Cached1_duplicateFound_) {
return RawLastIndexOfCodePointNode.cached(arg0Value, arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, compaction__, decodeCompactionLevel(((state_0 & 0b11100000) >>> 5 /* get-int EncodedEnum[cache=TStringOpsNodes.RawLastIndexOfCodePointNode.cached(..., CompactionLevel cachedCompaction)] */) - 2));
}
break;
}
}
{
CompactionLevel compaction__ = null;
while (true) {
boolean Cached2_duplicateFound_ = false;
if ((state_0 & 0b100000000) != 0 /* is SpecializationActive[TStringOpsNodes.RawLastIndexOfCodePointNode.cached(Node, AbstractTruffleString, Object, int, int, int, CompactionLevel, CompactionLevel)] */ && (state_0 & 0b111000000000) >>> 9 /* get-int EncodedEnum[cache=TStringOpsNodes.RawLastIndexOfCodePointNode.cached(..., CompactionLevel cachedCompaction)] */ != 0) {
compaction__ = (CompactionLevel.fromStride(arg1Value.stride()));
if ((compaction__ == decodeCompactionLevel(((state_0 & 0b111000000000) >>> 9 /* get-int EncodedEnum[cache=TStringOpsNodes.RawLastIndexOfCodePointNode.cached(..., CompactionLevel cachedCompaction)] */) - 2))) {
Cached2_duplicateFound_ = true;
}
}
if (!Cached2_duplicateFound_) {
// assert ((CompactionLevel.fromStride(arg1Value.stride())) == decodeCompactionLevel(((state_0 & 0b111000000000) >>> 9 /* get-int EncodedEnum[cache=TStringOpsNodes.RawLastIndexOfCodePointNode.cached(..., CompactionLevel cachedCompaction)] */) - 2));
if (((state_0 & 0b100000000)) == 0 /* is-not SpecializationActive[TStringOpsNodes.RawLastIndexOfCodePointNode.cached(Node, AbstractTruffleString, Object, int, int, int, CompactionLevel, CompactionLevel)] */) {
compaction__ = (CompactionLevel.fromStride(arg1Value.stride()));
state_0 = (state_0 | ((encodeCompactionLevel((compaction__)) + 2) << 9) /* set-int EncodedEnum[cache=TStringOpsNodes.RawLastIndexOfCodePointNode.cached(..., CompactionLevel cachedCompaction)] */);
state_0 = state_0 | 0b100000000 /* add SpecializationActive[TStringOpsNodes.RawLastIndexOfCodePointNode.cached(Node, AbstractTruffleString, Object, int, int, int, CompactionLevel, CompactionLevel)] */;
this.state_0_.set(arg0Value, state_0);
Cached2_duplicateFound_ = true;
}
}
if (Cached2_duplicateFound_) {
return RawLastIndexOfCodePointNode.cached(arg0Value, arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, compaction__, decodeCompactionLevel(((state_0 & 0b111000000000) >>> 9 /* get-int EncodedEnum[cache=TStringOpsNodes.RawLastIndexOfCodePointNode.cached(..., CompactionLevel cachedCompaction)] */) - 2));
}
break;
}
}
throw new UnsupportedSpecializationException(this, new Node[] {null, null, null, null, null, null}, arg0Value, arg1Value, arg2Value, arg3Value, arg4Value, arg5Value);
}
@Override
public boolean isAdoptable() {
return false;
}
}
@GeneratedBy(RawLastIndexOfCodePointNode.class)
@DenyReplace
private static final class Uncached extends RawLastIndexOfCodePointNode {
@TruffleBoundary
@Override
int execute(Node arg0Value, AbstractTruffleString arg1Value, Object arg2Value, int arg3Value, int arg4Value, int arg5Value) {
// assert ((CompactionLevel.fromStride(arg1Value.stride())) == ((CompactionLevel.fromStride(arg1Value.stride()))));
return RawLastIndexOfCodePointNode.cached(arg0Value, arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, (CompactionLevel.fromStride(arg1Value.stride())), ((CompactionLevel.fromStride(arg1Value.stride()))));
}
@Override
public NodeCost getCost() {
return NodeCost.MEGAMORPHIC;
}
@Override
public boolean isAdoptable() {
return false;
}
}
}
/**
* Debug Info:
* Specialization {@link RawIndexOfStringNode#doCached}
* Activation probability: 1.00000
* With/without class size: 36/9 bytes
*
*/
@GeneratedBy(RawIndexOfStringNode.class)
@SuppressWarnings("javadoc")
static final class RawIndexOfStringNodeGen {
private static final StateField CACHED__RAW_INDEX_OF_STRING_NODE_CACHED_STATE_0_UPDATER = StateField.create(CachedData.lookup_(), "cached_state_0_");
private static final Uncached UNCACHED = new Uncached();
@NeverDefault
public static RawIndexOfStringNode getUncached() {
return RawIndexOfStringNodeGen.UNCACHED;
}
/**
* Required Fields:
* - {@link Inlined#state_0_}
*
- {@link Inlined#cached_cache}
*
*/
@NeverDefault
public static RawIndexOfStringNode inline(@RequiredField(bits = 1, value = StateField.class)@RequiredField(type = Node.class, value = ReferenceField.class) InlineTarget target) {
return new RawIndexOfStringNodeGen.Inlined(target);
}
@GeneratedBy(RawIndexOfStringNode.class)
@DenyReplace
private static final class Inlined extends RawIndexOfStringNode {
/**
* State Info:
* 0: SpecializationActive {@link RawIndexOfStringNode#doCached}
*
*/
private final StateField state_0_;
private final ReferenceField cached_cache;
/**
* Source Info:
* Specialization: {@link RawIndexOfStringNode#doCached}
* Parameter: {@link InlinedConditionProfile} oneLength
* Inline method: {@link InlinedConditionProfile#inline}
*/
private final InlinedConditionProfile oneLength_;
@SuppressWarnings("unchecked")
private Inlined(InlineTarget target) {
assert target.getTargetClass().isAssignableFrom(RawIndexOfStringNode.class);
this.state_0_ = target.getState(0, 1);
this.cached_cache = target.getReference(1, CachedData.class);
this.oneLength_ = InlinedConditionProfile.inline(InlineTarget.create(InlinedConditionProfile.class, CACHED__RAW_INDEX_OF_STRING_NODE_CACHED_STATE_0_UPDATER.subUpdater(6, 2)));
}
@ExplodeLoop
@Override
int execute(Node arg0Value, AbstractTruffleString arg1Value, Object arg2Value, AbstractTruffleString arg3Value, Object arg4Value, int arg5Value, int arg6Value, byte[] arg7Value) {
int state_0 = this.state_0_.get(arg0Value);
if (state_0 != 0 /* is SpecializationActive[TStringOpsNodes.RawIndexOfStringNode.doCached(Node, AbstractTruffleString, Object, AbstractTruffleString, Object, int, int, byte[], CompactionLevel, CompactionLevel, CompactionLevel, CompactionLevel, InlinedConditionProfile)] */) {
CachedData s0_ = this.cached_cache.get(arg0Value);
while (s0_ != null) {
{
CompactionLevel compactionA__ = (CompactionLevel.fromStride(arg1Value.stride()));
if ((compactionA__ == decodeCompactionLevel(((s0_.cached_state_0_ & 0b111) >>> 0 /* get-int EncodedEnum[cache=TStringOpsNodes.RawIndexOfStringNode.doCached(..., CompactionLevel cachedCompactionA, ...)] */) - 2))) {
CompactionLevel compactionB__ = (CompactionLevel.fromStride(arg3Value.stride()));
if ((compactionB__ == decodeCompactionLevel(((s0_.cached_state_0_ & 0b111000) >>> 3 /* get-int EncodedEnum[cache=TStringOpsNodes.RawIndexOfStringNode.doCached(..., CompactionLevel cachedCompactionB, ...)] */) - 2))) {
return RawIndexOfStringNode.doCached(s0_, arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, arg6Value, arg7Value, compactionA__, decodeCompactionLevel(((s0_.cached_state_0_ & 0b111) >>> 0 /* get-int EncodedEnum[cache=TStringOpsNodes.RawIndexOfStringNode.doCached(..., CompactionLevel cachedCompactionA, ...)] */) - 2), compactionB__, decodeCompactionLevel(((s0_.cached_state_0_ & 0b111000) >>> 3 /* get-int EncodedEnum[cache=TStringOpsNodes.RawIndexOfStringNode.doCached(..., CompactionLevel cachedCompactionB, ...)] */) - 2), this.oneLength_);
}
}
}
s0_ = s0_.next_;
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value, arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, arg6Value, arg7Value);
}
private int executeAndSpecialize(Node arg0Value, AbstractTruffleString arg1Value, Object arg2Value, AbstractTruffleString arg3Value, Object arg4Value, int arg5Value, int arg6Value, byte[] arg7Value) {
int state_0 = this.state_0_.get(arg0Value);
{
CompactionLevel compactionB__ = null;
CompactionLevel compactionA__ = null;
while (true) {
int count0_ = 0;
CachedData s0_ = this.cached_cache.getVolatile(arg0Value);
CachedData s0_original = s0_;
while (s0_ != null) {
{
compactionA__ = (CompactionLevel.fromStride(arg1Value.stride()));
if ((compactionA__ == decodeCompactionLevel(((s0_.cached_state_0_ & 0b111) >>> 0 /* get-int EncodedEnum[cache=TStringOpsNodes.RawIndexOfStringNode.doCached(..., CompactionLevel cachedCompactionA, ...)] */) - 2))) {
compactionB__ = (CompactionLevel.fromStride(arg3Value.stride()));
if ((compactionB__ == decodeCompactionLevel(((s0_.cached_state_0_ & 0b111000) >>> 3 /* get-int EncodedEnum[cache=TStringOpsNodes.RawIndexOfStringNode.doCached(..., CompactionLevel cachedCompactionB, ...)] */) - 2))) {
break;
}
}
}
count0_++;
s0_ = s0_.next_;
}
if (s0_ == null) {
// assert ((CompactionLevel.fromStride(arg1Value.stride())) == decodeCompactionLevel(((s0_.cached_state_0_ & 0b111) >>> 0 /* get-int EncodedEnum[cache=TStringOpsNodes.RawIndexOfStringNode.doCached(..., CompactionLevel cachedCompactionA, ...)] */) - 2));
// assert ((CompactionLevel.fromStride(arg3Value.stride())) == decodeCompactionLevel(((s0_.cached_state_0_ & 0b111000) >>> 3 /* get-int EncodedEnum[cache=TStringOpsNodes.RawIndexOfStringNode.doCached(..., CompactionLevel cachedCompactionB, ...)] */) - 2));
if (count0_ < (9)) {
s0_ = arg0Value.insert(new CachedData(s0_original));
compactionA__ = (CompactionLevel.fromStride(arg1Value.stride()));
s0_.cached_state_0_ = (s0_.cached_state_0_ | ((encodeCompactionLevel((compactionA__)) + 2) << 0) /* set-int EncodedEnum[cache=TStringOpsNodes.RawIndexOfStringNode.doCached(..., CompactionLevel cachedCompactionA, ...)] */);
compactionB__ = (CompactionLevel.fromStride(arg3Value.stride()));
s0_.cached_state_0_ = (s0_.cached_state_0_ | ((encodeCompactionLevel((compactionB__)) + 2) << 3) /* set-int EncodedEnum[cache=TStringOpsNodes.RawIndexOfStringNode.doCached(..., CompactionLevel cachedCompactionB, ...)] */);
if (!this.cached_cache.compareAndSet(arg0Value, s0_original, s0_)) {
continue;
}
state_0 = state_0 | 0b1 /* add SpecializationActive[TStringOpsNodes.RawIndexOfStringNode.doCached(Node, AbstractTruffleString, Object, AbstractTruffleString, Object, int, int, byte[], CompactionLevel, CompactionLevel, CompactionLevel, CompactionLevel, InlinedConditionProfile)] */;
this.state_0_.set(arg0Value, state_0);
}
}
if (s0_ != null) {
return RawIndexOfStringNode.doCached(s0_, arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, arg6Value, arg7Value, compactionA__, decodeCompactionLevel(((s0_.cached_state_0_ & 0b111) >>> 0 /* get-int EncodedEnum[cache=TStringOpsNodes.RawIndexOfStringNode.doCached(..., CompactionLevel cachedCompactionA, ...)] */) - 2), compactionB__, decodeCompactionLevel(((s0_.cached_state_0_ & 0b111000) >>> 3 /* get-int EncodedEnum[cache=TStringOpsNodes.RawIndexOfStringNode.doCached(..., CompactionLevel cachedCompactionB, ...)] */) - 2), this.oneLength_);
}
break;
}
}
throw new UnsupportedSpecializationException(this, new Node[] {null, null, null, null, null, null, null, null}, arg0Value, arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, arg6Value, arg7Value);
}
@Override
public boolean isAdoptable() {
return false;
}
}
@GeneratedBy(RawIndexOfStringNode.class)
@DenyReplace
private static final class CachedData extends Node implements SpecializationDataNode {
@Child CachedData next_;
/**
* State Info:
* 0-2: EncodedEnum[cache=TStringOpsNodes.RawIndexOfStringNode.doCached(..., CompactionLevel cachedCompactionA, ...)]
* 3-5: EncodedEnum[cache=TStringOpsNodes.RawIndexOfStringNode.doCached(..., CompactionLevel cachedCompactionB, ...)]
* 6-7: InlinedCache
* Specialization: {@link RawIndexOfStringNode#doCached}
* Parameter: {@link InlinedConditionProfile} oneLength
* Inline method: {@link InlinedConditionProfile#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int cached_state_0_;
CachedData(CachedData next_) {
this.next_ = next_;
}
@Override
public NodeCost getCost() {
return NodeCost.NONE;
}
private static Lookup lookup_() {
return MethodHandles.lookup();
}
}
@GeneratedBy(RawIndexOfStringNode.class)
@DenyReplace
private static final class Uncached extends RawIndexOfStringNode {
@TruffleBoundary
@Override
int execute(Node arg0Value, AbstractTruffleString arg1Value, Object arg2Value, AbstractTruffleString arg3Value, Object arg4Value, int arg5Value, int arg6Value, byte[] arg7Value) {
// assert ((CompactionLevel.fromStride(arg1Value.stride())) == ((CompactionLevel.fromStride(arg1Value.stride()))));
// assert ((CompactionLevel.fromStride(arg3Value.stride())) == ((CompactionLevel.fromStride(arg3Value.stride()))));
return RawIndexOfStringNode.doCached(arg0Value, arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, arg6Value, arg7Value, (CompactionLevel.fromStride(arg1Value.stride())), ((CompactionLevel.fromStride(arg1Value.stride()))), (CompactionLevel.fromStride(arg3Value.stride())), ((CompactionLevel.fromStride(arg3Value.stride()))), (InlinedConditionProfile.getUncached()));
}
@Override
public NodeCost getCost() {
return NodeCost.MEGAMORPHIC;
}
@Override
public boolean isAdoptable() {
return false;
}
}
}
/**
* Debug Info:
* Specialization {@link RawLastIndexOfStringNode#cachedLen1}
* Activation probability: 1.00000
* With/without class size: 36/9 bytes
*
*/
@GeneratedBy(RawLastIndexOfStringNode.class)
@SuppressWarnings("javadoc")
static final class RawLastIndexOfStringNodeGen {
private static final StateField CACHED_LEN1__RAW_LAST_INDEX_OF_STRING_NODE_CACHED_LEN1_STATE_0_UPDATER = StateField.create(CachedLen1Data.lookup_(), "cachedLen1_state_0_");
private static final Uncached UNCACHED = new Uncached();
@NeverDefault
public static RawLastIndexOfStringNode getUncached() {
return RawLastIndexOfStringNodeGen.UNCACHED;
}
/**
* Required Fields:
* - {@link Inlined#state_0_}
*
- {@link Inlined#cachedLen1_cache}
*
*/
@NeverDefault
public static RawLastIndexOfStringNode inline(@RequiredField(bits = 1, value = StateField.class)@RequiredField(type = Node.class, value = ReferenceField.class) InlineTarget target) {
return new RawLastIndexOfStringNodeGen.Inlined(target);
}
@GeneratedBy(RawLastIndexOfStringNode.class)
@DenyReplace
private static final class Inlined extends RawLastIndexOfStringNode {
/**
* State Info:
* 0: SpecializationActive {@link RawLastIndexOfStringNode#cachedLen1}
*
*/
private final StateField state_0_;
private final ReferenceField cachedLen1_cache;
/**
* Source Info:
* Specialization: {@link RawLastIndexOfStringNode#cachedLen1}
* Parameter: {@link InlinedConditionProfile} oneLength
* Inline method: {@link InlinedConditionProfile#inline}
*/
private final InlinedConditionProfile oneLength_;
@SuppressWarnings("unchecked")
private Inlined(InlineTarget target) {
assert target.getTargetClass().isAssignableFrom(RawLastIndexOfStringNode.class);
this.state_0_ = target.getState(0, 1);
this.cachedLen1_cache = target.getReference(1, CachedLen1Data.class);
this.oneLength_ = InlinedConditionProfile.inline(InlineTarget.create(InlinedConditionProfile.class, CACHED_LEN1__RAW_LAST_INDEX_OF_STRING_NODE_CACHED_LEN1_STATE_0_UPDATER.subUpdater(6, 2)));
}
@ExplodeLoop
@Override
int execute(Node arg0Value, AbstractTruffleString arg1Value, Object arg2Value, AbstractTruffleString arg3Value, Object arg4Value, int arg5Value, int arg6Value, byte[] arg7Value) {
int state_0 = this.state_0_.get(arg0Value);
if (state_0 != 0 /* is SpecializationActive[TStringOpsNodes.RawLastIndexOfStringNode.cachedLen1(Node, AbstractTruffleString, Object, AbstractTruffleString, Object, int, int, byte[], CompactionLevel, CompactionLevel, CompactionLevel, CompactionLevel, InlinedConditionProfile)] */) {
CachedLen1Data s0_ = this.cachedLen1_cache.get(arg0Value);
while (s0_ != null) {
{
CompactionLevel compactionA__ = (CompactionLevel.fromStride(arg1Value.stride()));
if ((compactionA__ == decodeCompactionLevel(((s0_.cachedLen1_state_0_ & 0b111) >>> 0 /* get-int EncodedEnum[cache=TStringOpsNodes.RawLastIndexOfStringNode.cachedLen1(..., CompactionLevel cachedCompactionA, ...)] */) - 2))) {
CompactionLevel compactionB__ = (CompactionLevel.fromStride(arg3Value.stride()));
if ((compactionB__ == decodeCompactionLevel(((s0_.cachedLen1_state_0_ & 0b111000) >>> 3 /* get-int EncodedEnum[cache=TStringOpsNodes.RawLastIndexOfStringNode.cachedLen1(..., CompactionLevel cachedCompactionB, ...)] */) - 2))) {
return RawLastIndexOfStringNode.cachedLen1(s0_, arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, arg6Value, arg7Value, compactionA__, decodeCompactionLevel(((s0_.cachedLen1_state_0_ & 0b111) >>> 0 /* get-int EncodedEnum[cache=TStringOpsNodes.RawLastIndexOfStringNode.cachedLen1(..., CompactionLevel cachedCompactionA, ...)] */) - 2), compactionB__, decodeCompactionLevel(((s0_.cachedLen1_state_0_ & 0b111000) >>> 3 /* get-int EncodedEnum[cache=TStringOpsNodes.RawLastIndexOfStringNode.cachedLen1(..., CompactionLevel cachedCompactionB, ...)] */) - 2), this.oneLength_);
}
}
}
s0_ = s0_.next_;
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value, arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, arg6Value, arg7Value);
}
private int executeAndSpecialize(Node arg0Value, AbstractTruffleString arg1Value, Object arg2Value, AbstractTruffleString arg3Value, Object arg4Value, int arg5Value, int arg6Value, byte[] arg7Value) {
int state_0 = this.state_0_.get(arg0Value);
{
CompactionLevel compactionB__ = null;
CompactionLevel compactionA__ = null;
while (true) {
int count0_ = 0;
CachedLen1Data s0_ = this.cachedLen1_cache.getVolatile(arg0Value);
CachedLen1Data s0_original = s0_;
while (s0_ != null) {
{
compactionA__ = (CompactionLevel.fromStride(arg1Value.stride()));
if ((compactionA__ == decodeCompactionLevel(((s0_.cachedLen1_state_0_ & 0b111) >>> 0 /* get-int EncodedEnum[cache=TStringOpsNodes.RawLastIndexOfStringNode.cachedLen1(..., CompactionLevel cachedCompactionA, ...)] */) - 2))) {
compactionB__ = (CompactionLevel.fromStride(arg3Value.stride()));
if ((compactionB__ == decodeCompactionLevel(((s0_.cachedLen1_state_0_ & 0b111000) >>> 3 /* get-int EncodedEnum[cache=TStringOpsNodes.RawLastIndexOfStringNode.cachedLen1(..., CompactionLevel cachedCompactionB, ...)] */) - 2))) {
break;
}
}
}
count0_++;
s0_ = s0_.next_;
}
if (s0_ == null) {
// assert ((CompactionLevel.fromStride(arg1Value.stride())) == decodeCompactionLevel(((s0_.cachedLen1_state_0_ & 0b111) >>> 0 /* get-int EncodedEnum[cache=TStringOpsNodes.RawLastIndexOfStringNode.cachedLen1(..., CompactionLevel cachedCompactionA, ...)] */) - 2));
// assert ((CompactionLevel.fromStride(arg3Value.stride())) == decodeCompactionLevel(((s0_.cachedLen1_state_0_ & 0b111000) >>> 3 /* get-int EncodedEnum[cache=TStringOpsNodes.RawLastIndexOfStringNode.cachedLen1(..., CompactionLevel cachedCompactionB, ...)] */) - 2));
if (count0_ < (9)) {
s0_ = arg0Value.insert(new CachedLen1Data(s0_original));
compactionA__ = (CompactionLevel.fromStride(arg1Value.stride()));
s0_.cachedLen1_state_0_ = (s0_.cachedLen1_state_0_ | ((encodeCompactionLevel((compactionA__)) + 2) << 0) /* set-int EncodedEnum[cache=TStringOpsNodes.RawLastIndexOfStringNode.cachedLen1(..., CompactionLevel cachedCompactionA, ...)] */);
compactionB__ = (CompactionLevel.fromStride(arg3Value.stride()));
s0_.cachedLen1_state_0_ = (s0_.cachedLen1_state_0_ | ((encodeCompactionLevel((compactionB__)) + 2) << 3) /* set-int EncodedEnum[cache=TStringOpsNodes.RawLastIndexOfStringNode.cachedLen1(..., CompactionLevel cachedCompactionB, ...)] */);
if (!this.cachedLen1_cache.compareAndSet(arg0Value, s0_original, s0_)) {
continue;
}
state_0 = state_0 | 0b1 /* add SpecializationActive[TStringOpsNodes.RawLastIndexOfStringNode.cachedLen1(Node, AbstractTruffleString, Object, AbstractTruffleString, Object, int, int, byte[], CompactionLevel, CompactionLevel, CompactionLevel, CompactionLevel, InlinedConditionProfile)] */;
this.state_0_.set(arg0Value, state_0);
}
}
if (s0_ != null) {
return RawLastIndexOfStringNode.cachedLen1(s0_, arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, arg6Value, arg7Value, compactionA__, decodeCompactionLevel(((s0_.cachedLen1_state_0_ & 0b111) >>> 0 /* get-int EncodedEnum[cache=TStringOpsNodes.RawLastIndexOfStringNode.cachedLen1(..., CompactionLevel cachedCompactionA, ...)] */) - 2), compactionB__, decodeCompactionLevel(((s0_.cachedLen1_state_0_ & 0b111000) >>> 3 /* get-int EncodedEnum[cache=TStringOpsNodes.RawLastIndexOfStringNode.cachedLen1(..., CompactionLevel cachedCompactionB, ...)] */) - 2), this.oneLength_);
}
break;
}
}
throw new UnsupportedSpecializationException(this, new Node[] {null, null, null, null, null, null, null, null}, arg0Value, arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, arg6Value, arg7Value);
}
@Override
public boolean isAdoptable() {
return false;
}
}
@GeneratedBy(RawLastIndexOfStringNode.class)
@DenyReplace
private static final class CachedLen1Data extends Node implements SpecializationDataNode {
@Child CachedLen1Data next_;
/**
* State Info:
* 0-2: EncodedEnum[cache=TStringOpsNodes.RawLastIndexOfStringNode.cachedLen1(..., CompactionLevel cachedCompactionA, ...)]
* 3-5: EncodedEnum[cache=TStringOpsNodes.RawLastIndexOfStringNode.cachedLen1(..., CompactionLevel cachedCompactionB, ...)]
* 6-7: InlinedCache
* Specialization: {@link RawLastIndexOfStringNode#cachedLen1}
* Parameter: {@link InlinedConditionProfile} oneLength
* Inline method: {@link InlinedConditionProfile#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int cachedLen1_state_0_;
CachedLen1Data(CachedLen1Data next_) {
this.next_ = next_;
}
@Override
public NodeCost getCost() {
return NodeCost.NONE;
}
private static Lookup lookup_() {
return MethodHandles.lookup();
}
}
@GeneratedBy(RawLastIndexOfStringNode.class)
@DenyReplace
private static final class Uncached extends RawLastIndexOfStringNode {
@TruffleBoundary
@Override
int execute(Node arg0Value, AbstractTruffleString arg1Value, Object arg2Value, AbstractTruffleString arg3Value, Object arg4Value, int arg5Value, int arg6Value, byte[] arg7Value) {
// assert ((CompactionLevel.fromStride(arg1Value.stride())) == ((CompactionLevel.fromStride(arg1Value.stride()))));
// assert ((CompactionLevel.fromStride(arg3Value.stride())) == ((CompactionLevel.fromStride(arg3Value.stride()))));
return RawLastIndexOfStringNode.cachedLen1(arg0Value, arg1Value, arg2Value, arg3Value, arg4Value, arg5Value, arg6Value, arg7Value, (CompactionLevel.fromStride(arg1Value.stride())), ((CompactionLevel.fromStride(arg1Value.stride()))), (CompactionLevel.fromStride(arg3Value.stride())), ((CompactionLevel.fromStride(arg3Value.stride()))), (InlinedConditionProfile.getUncached()));
}
@Override
public NodeCost getCost() {
return NodeCost.MEGAMORPHIC;
}
@Override
public boolean isAdoptable() {
return false;
}
}
}
/**
* Debug Info:
* Specialization {@link CalculateHashCodeNode#cached}
* Activation probability: 0.48333
* With/without class size: 11/4 bytes
* Specialization {@link CalculateHashCodeNode#cached}
* Activation probability: 0.33333
* With/without class size: 9/4 bytes
* Specialization {@link CalculateHashCodeNode#cached}
* Activation probability: 0.18333
* With/without class size: 6/4 bytes
*
*/
@GeneratedBy(CalculateHashCodeNode.class)
@SuppressWarnings("javadoc")
static final class CalculateHashCodeNodeGen {
private static final Uncached UNCACHED = new Uncached();
@NeverDefault
public static CalculateHashCodeNode getUncached() {
return CalculateHashCodeNodeGen.UNCACHED;
}
/**
* Required Fields:
* - {@link Inlined#state_0_}
*
*/
@NeverDefault
public static CalculateHashCodeNode inline(@RequiredField(bits = 12, value = StateField.class) InlineTarget target) {
return new CalculateHashCodeNodeGen.Inlined(target);
}
@GeneratedBy(CalculateHashCodeNode.class)
@DenyReplace
private static final class Inlined extends CalculateHashCodeNode {
/**
* State Info:
* 0: SpecializationActive {@link CalculateHashCodeNode#cached}
* 1-3: EncodedEnum[cache=TStringOpsNodes.CalculateHashCodeNode.cached(..., CompactionLevel cachedCompaction)]
* 4: SpecializationActive {@link CalculateHashCodeNode#cached}
* 5-7: EncodedEnum[cache=TStringOpsNodes.CalculateHashCodeNode.cached(..., CompactionLevel cachedCompaction)]
* 8: SpecializationActive {@link CalculateHashCodeNode#cached}
* 9-11: EncodedEnum[cache=TStringOpsNodes.CalculateHashCodeNode.cached(..., CompactionLevel cachedCompaction)]
*
*/
private final StateField state_0_;
private Inlined(InlineTarget target) {
assert target.getTargetClass().isAssignableFrom(CalculateHashCodeNode.class);
this.state_0_ = target.getState(0, 12);
}
@Override
int execute(Node arg0Value, AbstractTruffleString arg1Value, Object arg2Value) {
int state_0 = this.state_0_.get(arg0Value);
if ((state_0 & 0b100010001) != 0 /* is SpecializationActive[TStringOpsNodes.CalculateHashCodeNode.cached(Node, AbstractTruffleString, Object, CompactionLevel, CompactionLevel)] || SpecializationActive[TStringOpsNodes.CalculateHashCodeNode.cached(Node, AbstractTruffleString, Object, CompactionLevel, CompactionLevel)] || SpecializationActive[TStringOpsNodes.CalculateHashCodeNode.cached(Node, AbstractTruffleString, Object, CompactionLevel, CompactionLevel)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[TStringOpsNodes.CalculateHashCodeNode.cached(Node, AbstractTruffleString, Object, CompactionLevel, CompactionLevel)] */) {
if ((state_0 & 0b1110) >>> 1 /* get-int EncodedEnum[cache=TStringOpsNodes.CalculateHashCodeNode.cached(..., CompactionLevel cachedCompaction)] */ != 0) {
CompactionLevel compaction__ = (CompactionLevel.fromStride(arg1Value.stride()));
if ((compaction__ == decodeCompactionLevel(((state_0 & 0b1110) >>> 1 /* get-int EncodedEnum[cache=TStringOpsNodes.CalculateHashCodeNode.cached(..., CompactionLevel cachedCompaction)] */) - 2))) {
return CalculateHashCodeNode.cached(arg0Value, arg1Value, arg2Value, compaction__, decodeCompactionLevel(((state_0 & 0b1110) >>> 1 /* get-int EncodedEnum[cache=TStringOpsNodes.CalculateHashCodeNode.cached(..., CompactionLevel cachedCompaction)] */) - 2));
}
}
}
if ((state_0 & 0b10000) != 0 /* is SpecializationActive[TStringOpsNodes.CalculateHashCodeNode.cached(Node, AbstractTruffleString, Object, CompactionLevel, CompactionLevel)] */) {
if ((state_0 & 0b11100000) >>> 5 /* get-int EncodedEnum[cache=TStringOpsNodes.CalculateHashCodeNode.cached(..., CompactionLevel cachedCompaction)] */ != 0) {
CompactionLevel compaction__ = (CompactionLevel.fromStride(arg1Value.stride()));
if ((compaction__ == decodeCompactionLevel(((state_0 & 0b11100000) >>> 5 /* get-int EncodedEnum[cache=TStringOpsNodes.CalculateHashCodeNode.cached(..., CompactionLevel cachedCompaction)] */) - 2))) {
return CalculateHashCodeNode.cached(arg0Value, arg1Value, arg2Value, compaction__, decodeCompactionLevel(((state_0 & 0b11100000) >>> 5 /* get-int EncodedEnum[cache=TStringOpsNodes.CalculateHashCodeNode.cached(..., CompactionLevel cachedCompaction)] */) - 2));
}
}
}
if ((state_0 & 0b100000000) != 0 /* is SpecializationActive[TStringOpsNodes.CalculateHashCodeNode.cached(Node, AbstractTruffleString, Object, CompactionLevel, CompactionLevel)] */) {
if ((state_0 & 0b111000000000) >>> 9 /* get-int EncodedEnum[cache=TStringOpsNodes.CalculateHashCodeNode.cached(..., CompactionLevel cachedCompaction)] */ != 0) {
CompactionLevel compaction__ = (CompactionLevel.fromStride(arg1Value.stride()));
if ((compaction__ == decodeCompactionLevel(((state_0 & 0b111000000000) >>> 9 /* get-int EncodedEnum[cache=TStringOpsNodes.CalculateHashCodeNode.cached(..., CompactionLevel cachedCompaction)] */) - 2))) {
return CalculateHashCodeNode.cached(arg0Value, arg1Value, arg2Value, compaction__, decodeCompactionLevel(((state_0 & 0b111000000000) >>> 9 /* get-int EncodedEnum[cache=TStringOpsNodes.CalculateHashCodeNode.cached(..., CompactionLevel cachedCompaction)] */) - 2));
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value, arg1Value, arg2Value);
}
private int executeAndSpecialize(Node arg0Value, AbstractTruffleString arg1Value, Object arg2Value) {
int state_0 = this.state_0_.get(arg0Value);
{
CompactionLevel compaction__ = null;
while (true) {
boolean Cached0_duplicateFound_ = false;
if ((state_0 & 0b1) != 0 /* is SpecializationActive[TStringOpsNodes.CalculateHashCodeNode.cached(Node, AbstractTruffleString, Object, CompactionLevel, CompactionLevel)] */ && (state_0 & 0b1110) >>> 1 /* get-int EncodedEnum[cache=TStringOpsNodes.CalculateHashCodeNode.cached(..., CompactionLevel cachedCompaction)] */ != 0) {
compaction__ = (CompactionLevel.fromStride(arg1Value.stride()));
if ((compaction__ == decodeCompactionLevel(((state_0 & 0b1110) >>> 1 /* get-int EncodedEnum[cache=TStringOpsNodes.CalculateHashCodeNode.cached(..., CompactionLevel cachedCompaction)] */) - 2))) {
Cached0_duplicateFound_ = true;
}
}
if (!Cached0_duplicateFound_) {
// assert ((CompactionLevel.fromStride(arg1Value.stride())) == decodeCompactionLevel(((state_0 & 0b1110) >>> 1 /* get-int EncodedEnum[cache=TStringOpsNodes.CalculateHashCodeNode.cached(..., CompactionLevel cachedCompaction)] */) - 2));
if (((state_0 & 0b1)) == 0 /* is-not SpecializationActive[TStringOpsNodes.CalculateHashCodeNode.cached(Node, AbstractTruffleString, Object, CompactionLevel, CompactionLevel)] */) {
compaction__ = (CompactionLevel.fromStride(arg1Value.stride()));
state_0 = (state_0 | ((encodeCompactionLevel((compaction__)) + 2) << 1) /* set-int EncodedEnum[cache=TStringOpsNodes.CalculateHashCodeNode.cached(..., CompactionLevel cachedCompaction)] */);
state_0 = state_0 | 0b1 /* add SpecializationActive[TStringOpsNodes.CalculateHashCodeNode.cached(Node, AbstractTruffleString, Object, CompactionLevel, CompactionLevel)] */;
this.state_0_.set(arg0Value, state_0);
Cached0_duplicateFound_ = true;
}
}
if (Cached0_duplicateFound_) {
return CalculateHashCodeNode.cached(arg0Value, arg1Value, arg2Value, compaction__, decodeCompactionLevel(((state_0 & 0b1110) >>> 1 /* get-int EncodedEnum[cache=TStringOpsNodes.CalculateHashCodeNode.cached(..., CompactionLevel cachedCompaction)] */) - 2));
}
break;
}
}
{
CompactionLevel compaction__ = null;
while (true) {
boolean Cached1_duplicateFound_ = false;
if ((state_0 & 0b10000) != 0 /* is SpecializationActive[TStringOpsNodes.CalculateHashCodeNode.cached(Node, AbstractTruffleString, Object, CompactionLevel, CompactionLevel)] */ && (state_0 & 0b11100000) >>> 5 /* get-int EncodedEnum[cache=TStringOpsNodes.CalculateHashCodeNode.cached(..., CompactionLevel cachedCompaction)] */ != 0) {
compaction__ = (CompactionLevel.fromStride(arg1Value.stride()));
if ((compaction__ == decodeCompactionLevel(((state_0 & 0b11100000) >>> 5 /* get-int EncodedEnum[cache=TStringOpsNodes.CalculateHashCodeNode.cached(..., CompactionLevel cachedCompaction)] */) - 2))) {
Cached1_duplicateFound_ = true;
}
}
if (!Cached1_duplicateFound_) {
// assert ((CompactionLevel.fromStride(arg1Value.stride())) == decodeCompactionLevel(((state_0 & 0b11100000) >>> 5 /* get-int EncodedEnum[cache=TStringOpsNodes.CalculateHashCodeNode.cached(..., CompactionLevel cachedCompaction)] */) - 2));
if (((state_0 & 0b10000)) == 0 /* is-not SpecializationActive[TStringOpsNodes.CalculateHashCodeNode.cached(Node, AbstractTruffleString, Object, CompactionLevel, CompactionLevel)] */) {
compaction__ = (CompactionLevel.fromStride(arg1Value.stride()));
state_0 = (state_0 | ((encodeCompactionLevel((compaction__)) + 2) << 5) /* set-int EncodedEnum[cache=TStringOpsNodes.CalculateHashCodeNode.cached(..., CompactionLevel cachedCompaction)] */);
state_0 = state_0 | 0b10000 /* add SpecializationActive[TStringOpsNodes.CalculateHashCodeNode.cached(Node, AbstractTruffleString, Object, CompactionLevel, CompactionLevel)] */;
this.state_0_.set(arg0Value, state_0);
Cached1_duplicateFound_ = true;
}
}
if (Cached1_duplicateFound_) {
return CalculateHashCodeNode.cached(arg0Value, arg1Value, arg2Value, compaction__, decodeCompactionLevel(((state_0 & 0b11100000) >>> 5 /* get-int EncodedEnum[cache=TStringOpsNodes.CalculateHashCodeNode.cached(..., CompactionLevel cachedCompaction)] */) - 2));
}
break;
}
}
{
CompactionLevel compaction__ = null;
while (true) {
boolean Cached2_duplicateFound_ = false;
if ((state_0 & 0b100000000) != 0 /* is SpecializationActive[TStringOpsNodes.CalculateHashCodeNode.cached(Node, AbstractTruffleString, Object, CompactionLevel, CompactionLevel)] */ && (state_0 & 0b111000000000) >>> 9 /* get-int EncodedEnum[cache=TStringOpsNodes.CalculateHashCodeNode.cached(..., CompactionLevel cachedCompaction)] */ != 0) {
compaction__ = (CompactionLevel.fromStride(arg1Value.stride()));
if ((compaction__ == decodeCompactionLevel(((state_0 & 0b111000000000) >>> 9 /* get-int EncodedEnum[cache=TStringOpsNodes.CalculateHashCodeNode.cached(..., CompactionLevel cachedCompaction)] */) - 2))) {
Cached2_duplicateFound_ = true;
}
}
if (!Cached2_duplicateFound_) {
// assert ((CompactionLevel.fromStride(arg1Value.stride())) == decodeCompactionLevel(((state_0 & 0b111000000000) >>> 9 /* get-int EncodedEnum[cache=TStringOpsNodes.CalculateHashCodeNode.cached(..., CompactionLevel cachedCompaction)] */) - 2));
if (((state_0 & 0b100000000)) == 0 /* is-not SpecializationActive[TStringOpsNodes.CalculateHashCodeNode.cached(Node, AbstractTruffleString, Object, CompactionLevel, CompactionLevel)] */) {
compaction__ = (CompactionLevel.fromStride(arg1Value.stride()));
state_0 = (state_0 | ((encodeCompactionLevel((compaction__)) + 2) << 9) /* set-int EncodedEnum[cache=TStringOpsNodes.CalculateHashCodeNode.cached(..., CompactionLevel cachedCompaction)] */);
state_0 = state_0 | 0b100000000 /* add SpecializationActive[TStringOpsNodes.CalculateHashCodeNode.cached(Node, AbstractTruffleString, Object, CompactionLevel, CompactionLevel)] */;
this.state_0_.set(arg0Value, state_0);
Cached2_duplicateFound_ = true;
}
}
if (Cached2_duplicateFound_) {
return CalculateHashCodeNode.cached(arg0Value, arg1Value, arg2Value, compaction__, decodeCompactionLevel(((state_0 & 0b111000000000) >>> 9 /* get-int EncodedEnum[cache=TStringOpsNodes.CalculateHashCodeNode.cached(..., CompactionLevel cachedCompaction)] */) - 2));
}
break;
}
}
throw new UnsupportedSpecializationException(this, new Node[] {null, null, null}, arg0Value, arg1Value, arg2Value);
}
@Override
public boolean isAdoptable() {
return false;
}
}
@GeneratedBy(CalculateHashCodeNode.class)
@DenyReplace
private static final class Uncached extends CalculateHashCodeNode {
@TruffleBoundary
@Override
int execute(Node arg0Value, AbstractTruffleString arg1Value, Object arg2Value) {
// assert ((CompactionLevel.fromStride(arg1Value.stride())) == ((CompactionLevel.fromStride(arg1Value.stride()))));
return CalculateHashCodeNode.cached(arg0Value, arg1Value, arg2Value, (CompactionLevel.fromStride(arg1Value.stride())), ((CompactionLevel.fromStride(arg1Value.stride()))));
}
@Override
public NodeCost getCost() {
return NodeCost.MEGAMORPHIC;
}
@Override
public boolean isAdoptable() {
return false;
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy