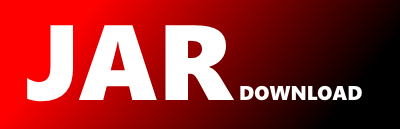
com.oracle.truffle.nfi.backend.libffi.ArrayGen Maven / Gradle / Ivy
// CheckStyle: start generated
package com.oracle.truffle.nfi.backend.libffi;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.CompilerDirectives.TruffleBoundary;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.interop.InteropLibrary;
import com.oracle.truffle.api.interop.InvalidBufferOffsetException;
import com.oracle.truffle.api.interop.UnsupportedMessageException;
import com.oracle.truffle.api.library.DynamicDispatchLibrary;
import com.oracle.truffle.api.library.LibraryExport;
import com.oracle.truffle.api.library.LibraryFactory;
import com.oracle.truffle.api.nodes.DenyReplace;
import com.oracle.truffle.api.nodes.EncapsulatingNodeReference;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.nodes.NodeCost;
import com.oracle.truffle.api.profiles.BranchProfile;
import com.oracle.truffle.nfi.api.SerializableLibrary;
import com.oracle.truffle.nfi.backend.libffi.NativeBuffer.Array;
import java.lang.invoke.VarHandle;
import java.nio.ByteOrder;
import java.util.Objects;
@GeneratedBy(Array.class)
@SuppressWarnings({"javadoc", "unused"})
final class ArrayGen {
private static final LibraryFactory DYNAMIC_DISPATCH_LIBRARY_ = LibraryFactory.resolve(DynamicDispatchLibrary.class);
private static final LibraryFactory INTEROP_LIBRARY_ = LibraryFactory.resolve(InteropLibrary.class);
static {
LibraryExport.register(Array.class, new SerializableLibraryExports(), new InteropLibraryExports());
}
private ArrayGen() {
}
@GeneratedBy(Array.class)
private static final class SerializableLibraryExports extends LibraryExport {
private SerializableLibraryExports() {
super(SerializableLibrary.class, Array.class, false, false, 0);
}
@Override
protected SerializableLibrary createUncached(Object receiver) {
assert receiver instanceof Array;
SerializableLibrary uncached = new Uncached();
return uncached;
}
@Override
protected SerializableLibrary createCached(Object receiver) {
assert receiver instanceof Array;
return new Cached();
}
@GeneratedBy(Array.class)
private static final class Cached extends SerializableLibrary {
/**
* State Info:
* 0: SpecializationActive {@link Array#serialize(Array, Object, InteropLibrary)}
* 1: SpecializationActive {@link Array#serialize(Array, Object, InteropLibrary)}
*
*/
@CompilationFinal private int state_0_;
/**
* Source Info:
* Specialization: {@link Array#serialize(Array, Object, InteropLibrary)}
* Parameter: {@link InteropLibrary} interop
*/
@Child private InteropLibrary serialize0_interop_;
protected Cached() {
}
@Override
public boolean accepts(Object receiver) {
assert !(receiver instanceof Array) || DYNAMIC_DISPATCH_LIBRARY_.getUncached().dispatch(receiver) == null : "Invalid library export. Exported receiver with dynamic dispatch found but not expected.";
return receiver instanceof Array;
}
@Override
public boolean isSerializable(Object receiver) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert getRootNode() != null : "Invalid library usage. Cached library must be adopted by a RootNode before it is executed.";
return (((Array) receiver)).isSerializable();
}
/**
* Debug Info:
* Specialization {@link Array#serialize(Array, Object, InteropLibrary)}
* Activation probability: 0.65000
* With/without class size: 17/4 bytes
* Specialization {@link Array#serialize(Array, Object, InteropLibrary)}
* Activation probability: 0.35000
* With/without class size: 8/0 bytes
*
*/
@Override
public void serialize(Object arg0Value_, Object arg1Value) throws UnsupportedMessageException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert getRootNode() != null : "Invalid library usage. Cached library must be adopted by a RootNode before it is executed.";
Array arg0Value = ((Array) arg0Value_);
int state_0 = this.state_0_;
if (state_0 != 0 /* is SpecializationActive[Array.serialize(Array, Object, InteropLibrary)] || SpecializationActive[Array.serialize(Array, Object, InteropLibrary)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[Array.serialize(Array, Object, InteropLibrary)] */) {
{
InteropLibrary interop__ = this.serialize0_interop_;
if (interop__ != null) {
if ((interop__.accepts(arg1Value))) {
arg0Value.serialize(arg1Value, interop__);
return;
}
}
}
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[Array.serialize(Array, Object, InteropLibrary)] */) {
this.serializeNode__Serialize1Boundary(state_0, arg0Value, arg1Value);
return;
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
executeAndSpecialize(arg0Value, arg1Value);
return;
}
@SuppressWarnings("static-method")
@TruffleBoundary
private void serializeNode__Serialize1Boundary(int state_0, Array arg0Value, Object arg1Value) throws UnsupportedMessageException {
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(this);
try {
{
InteropLibrary interop__ = (INTEROP_LIBRARY_.getUncached(arg1Value));
arg0Value.serialize(arg1Value, interop__);
return;
}
} finally {
encapsulating_.set(prev_);
}
}
private void executeAndSpecialize(Array arg0Value, Object arg1Value) {
int state_0 = this.state_0_;
if (((state_0 & 0b10)) == 0 /* is-not SpecializationActive[Array.serialize(Array, Object, InteropLibrary)] */) {
while (true) {
boolean Serialize0_duplicateFound_ = false;
if ((state_0 & 0b1) != 0 /* is SpecializationActive[Array.serialize(Array, Object, InteropLibrary)] */) {
InteropLibrary interop__ = this.serialize0_interop_;
if (interop__ != null) {
if ((interop__.accepts(arg1Value))) {
Serialize0_duplicateFound_ = true;
}
}
}
if (!Serialize0_duplicateFound_) {
// assert (this.serialize0_interop_.accepts(arg1Value));
if (((state_0 & 0b1)) == 0 /* is-not SpecializationActive[Array.serialize(Array, Object, InteropLibrary)] */) {
InteropLibrary interop__ = this.insert((INTEROP_LIBRARY_.create(arg1Value)));
Objects.requireNonNull(interop__, "Specialization 'serialize(Array, Object, InteropLibrary)' cache 'interop' returned a 'null' default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns 'null'.");
this.serialize0_interop_ = interop__;
state_0 = state_0 | 0b1 /* add SpecializationActive[Array.serialize(Array, Object, InteropLibrary)] */;
this.state_0_ = state_0;
Serialize0_duplicateFound_ = true;
}
}
if (Serialize0_duplicateFound_) {
arg0Value.serialize(arg1Value, this.serialize0_interop_);
return;
}
break;
}
}
{
InteropLibrary interop__ = null;
{
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(this);
try {
interop__ = (INTEROP_LIBRARY_.getUncached(arg1Value));
this.serialize0_interop_ = null;
state_0 = state_0 & 0xfffffffe /* remove SpecializationActive[Array.serialize(Array, Object, InteropLibrary)] */;
state_0 = state_0 | 0b10 /* add SpecializationActive[Array.serialize(Array, Object, InteropLibrary)] */;
this.state_0_ = state_0;
arg0Value.serialize(arg1Value, interop__);
return;
} finally {
encapsulating_.set(prev_);
}
}
}
}
@Override
public NodeCost getCost() {
int state_0 = this.state_0_;
if (state_0 == 0) {
return NodeCost.UNINITIALIZED;
} else {
if ((state_0 & (state_0 - 1)) == 0 /* is-single */) {
return NodeCost.MONOMORPHIC;
}
}
return NodeCost.POLYMORPHIC;
}
}
@GeneratedBy(Array.class)
@DenyReplace
private static final class Uncached extends SerializableLibrary {
protected Uncached() {
}
@Override
@TruffleBoundary
public boolean accepts(Object receiver) {
assert !(receiver instanceof Array) || DYNAMIC_DISPATCH_LIBRARY_.getUncached().dispatch(receiver) == null : "Invalid library export. Exported receiver with dynamic dispatch found but not expected.";
return receiver instanceof Array;
}
@Override
public boolean isAdoptable() {
return false;
}
@Override
public NodeCost getCost() {
return NodeCost.MEGAMORPHIC;
}
@TruffleBoundary
@Override
public boolean isSerializable(Object receiver) {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((Array) receiver) .isSerializable();
}
@TruffleBoundary
@Override
public void serialize(Object arg0Value_, Object arg1Value) {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
Array arg0Value = ((Array) arg0Value_);
arg0Value.serialize(arg1Value, (INTEROP_LIBRARY_.getUncached(arg1Value)));
return;
}
}
}
@GeneratedBy(Array.class)
private static final class InteropLibraryExports extends LibraryExport {
private InteropLibraryExports() {
super(InteropLibrary.class, Array.class, false, false, 0);
}
@Override
protected InteropLibrary createUncached(Object receiver) {
assert receiver instanceof Array;
InteropLibrary uncached = new Uncached();
return uncached;
}
@Override
protected InteropLibrary createCached(Object receiver) {
assert receiver instanceof Array;
return new Cached();
}
@GeneratedBy(Array.class)
private static final class Cached extends InteropLibrary {
/**
* State Info:
* 0: SpecializationActive {@link Array#readBufferByte(Array, long, BranchProfile)}
* 1: SpecializationActive {@link Array#readBufferShort(Array, ByteOrder, long, BranchProfile)}
* 2: SpecializationActive {@link Array#readBufferInt(Array, ByteOrder, long, BranchProfile)}
* 3: SpecializationActive {@link Array#readBufferLong(Array, ByteOrder, long, BranchProfile)}
* 4: SpecializationActive {@link Array#readBufferFloat(Array, ByteOrder, long, BranchProfile)}
* 5: SpecializationActive {@link Array#readBufferDouble(Array, ByteOrder, long, BranchProfile)}
*
*/
@CompilationFinal private int state_0_;
/**
* Source Info:
* Specialization: {@link Array#readBufferByte}
* Parameter: {@link BranchProfile} exception
*/
@CompilationFinal private BranchProfile exception;
protected Cached() {
}
@Override
public boolean accepts(Object receiver) {
assert !(receiver instanceof Array) || DYNAMIC_DISPATCH_LIBRARY_.getUncached().dispatch(receiver) == null : "Invalid library export. Exported receiver with dynamic dispatch found but not expected.";
return receiver instanceof Array;
}
@Override
public boolean hasBufferElements(Object receiver) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
return (((Array) receiver)).hasBufferElements();
}
@Override
public long getBufferSize(Object receiver) throws UnsupportedMessageException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
return (((Array) receiver)).getBufferSize();
}
/**
* Debug Info:
* Specialization {@link Array#readBufferByte(Array, long, BranchProfile)}
* Activation probability: 0.16667
* With/without class size: 6/0 bytes
*
*/
@Override
public byte readBufferByte(Object arg0Value_, long arg1Value) throws UnsupportedMessageException, InvalidBufferOffsetException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
Array arg0Value = ((Array) arg0Value_);
int state_0 = this.state_0_;
if ((state_0 & 0b1) != 0 /* is SpecializationActive[Array.readBufferByte(Array, long, BranchProfile)] */) {
{
BranchProfile exception_ = this.exception;
if (exception_ != null) {
return arg0Value.readBufferByte(arg1Value, exception_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return readBufferByteNode_AndSpecialize(arg0Value, arg1Value);
}
private byte readBufferByteNode_AndSpecialize(Array arg0Value, long arg1Value) throws InvalidBufferOffsetException {
int state_0 = this.state_0_;
BranchProfile exception_;
BranchProfile exception__shared = this.exception;
if (exception__shared != null) {
exception_ = exception__shared;
} else {
exception_ = (BranchProfile.create());
if (exception_ == null) {
throw new IllegalStateException("Specialization 'readBufferByte(Array, long, BranchProfile)' contains a shared cache with name 'exception' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.exception == null) {
VarHandle.storeStoreFence();
this.exception = exception_;
}
state_0 = state_0 | 0b1 /* add SpecializationActive[Array.readBufferByte(Array, long, BranchProfile)] */;
this.state_0_ = state_0;
return arg0Value.readBufferByte(arg1Value, exception_);
}
@Override
public NodeCost getCost() {
int state_0 = this.state_0_;
if ((state_0 & 0b1) == 0) {
return NodeCost.UNINITIALIZED;
} else {
return NodeCost.MONOMORPHIC;
}
}
/**
* Debug Info:
* Specialization {@link Array#readBufferShort(Array, ByteOrder, long, BranchProfile)}
* Activation probability: 0.16667
* With/without class size: 6/0 bytes
*
*/
@Override
public short readBufferShort(Object arg0Value_, ByteOrder arg1Value, long arg2Value) throws UnsupportedMessageException, InvalidBufferOffsetException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
Array arg0Value = ((Array) arg0Value_);
int state_0 = this.state_0_;
if ((state_0 & 0b10) != 0 /* is SpecializationActive[Array.readBufferShort(Array, ByteOrder, long, BranchProfile)] */) {
{
BranchProfile exception_ = this.exception;
if (exception_ != null) {
return arg0Value.readBufferShort(arg1Value, arg2Value, exception_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return readBufferShortNode_AndSpecialize(arg0Value, arg1Value, arg2Value);
}
private short readBufferShortNode_AndSpecialize(Array arg0Value, ByteOrder arg1Value, long arg2Value) throws InvalidBufferOffsetException {
int state_0 = this.state_0_;
BranchProfile exception_;
BranchProfile exception__shared = this.exception;
if (exception__shared != null) {
exception_ = exception__shared;
} else {
exception_ = (BranchProfile.create());
if (exception_ == null) {
throw new IllegalStateException("Specialization 'readBufferShort(Array, ByteOrder, long, BranchProfile)' contains a shared cache with name 'exception' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.exception == null) {
VarHandle.storeStoreFence();
this.exception = exception_;
}
state_0 = state_0 | 0b10 /* add SpecializationActive[Array.readBufferShort(Array, ByteOrder, long, BranchProfile)] */;
this.state_0_ = state_0;
return arg0Value.readBufferShort(arg1Value, arg2Value, exception_);
}
/**
* Debug Info:
* Specialization {@link Array#readBufferInt(Array, ByteOrder, long, BranchProfile)}
* Activation probability: 0.16667
* With/without class size: 6/0 bytes
*
*/
@Override
public int readBufferInt(Object arg0Value_, ByteOrder arg1Value, long arg2Value) throws UnsupportedMessageException, InvalidBufferOffsetException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
Array arg0Value = ((Array) arg0Value_);
int state_0 = this.state_0_;
if ((state_0 & 0b100) != 0 /* is SpecializationActive[Array.readBufferInt(Array, ByteOrder, long, BranchProfile)] */) {
{
BranchProfile exception_ = this.exception;
if (exception_ != null) {
return arg0Value.readBufferInt(arg1Value, arg2Value, exception_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return readBufferIntNode_AndSpecialize(arg0Value, arg1Value, arg2Value);
}
private int readBufferIntNode_AndSpecialize(Array arg0Value, ByteOrder arg1Value, long arg2Value) throws InvalidBufferOffsetException {
int state_0 = this.state_0_;
BranchProfile exception_;
BranchProfile exception__shared = this.exception;
if (exception__shared != null) {
exception_ = exception__shared;
} else {
exception_ = (BranchProfile.create());
if (exception_ == null) {
throw new IllegalStateException("Specialization 'readBufferInt(Array, ByteOrder, long, BranchProfile)' contains a shared cache with name 'exception' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.exception == null) {
VarHandle.storeStoreFence();
this.exception = exception_;
}
state_0 = state_0 | 0b100 /* add SpecializationActive[Array.readBufferInt(Array, ByteOrder, long, BranchProfile)] */;
this.state_0_ = state_0;
return arg0Value.readBufferInt(arg1Value, arg2Value, exception_);
}
/**
* Debug Info:
* Specialization {@link Array#readBufferLong(Array, ByteOrder, long, BranchProfile)}
* Activation probability: 0.16667
* With/without class size: 6/0 bytes
*
*/
@Override
public long readBufferLong(Object arg0Value_, ByteOrder arg1Value, long arg2Value) throws UnsupportedMessageException, InvalidBufferOffsetException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
Array arg0Value = ((Array) arg0Value_);
int state_0 = this.state_0_;
if ((state_0 & 0b1000) != 0 /* is SpecializationActive[Array.readBufferLong(Array, ByteOrder, long, BranchProfile)] */) {
{
BranchProfile exception_ = this.exception;
if (exception_ != null) {
return arg0Value.readBufferLong(arg1Value, arg2Value, exception_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return readBufferLongNode_AndSpecialize(arg0Value, arg1Value, arg2Value);
}
private long readBufferLongNode_AndSpecialize(Array arg0Value, ByteOrder arg1Value, long arg2Value) throws InvalidBufferOffsetException {
int state_0 = this.state_0_;
BranchProfile exception_;
BranchProfile exception__shared = this.exception;
if (exception__shared != null) {
exception_ = exception__shared;
} else {
exception_ = (BranchProfile.create());
if (exception_ == null) {
throw new IllegalStateException("Specialization 'readBufferLong(Array, ByteOrder, long, BranchProfile)' contains a shared cache with name 'exception' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.exception == null) {
VarHandle.storeStoreFence();
this.exception = exception_;
}
state_0 = state_0 | 0b1000 /* add SpecializationActive[Array.readBufferLong(Array, ByteOrder, long, BranchProfile)] */;
this.state_0_ = state_0;
return arg0Value.readBufferLong(arg1Value, arg2Value, exception_);
}
/**
* Debug Info:
* Specialization {@link Array#readBufferFloat(Array, ByteOrder, long, BranchProfile)}
* Activation probability: 0.16667
* With/without class size: 6/0 bytes
*
*/
@Override
public float readBufferFloat(Object arg0Value_, ByteOrder arg1Value, long arg2Value) throws UnsupportedMessageException, InvalidBufferOffsetException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
Array arg0Value = ((Array) arg0Value_);
int state_0 = this.state_0_;
if ((state_0 & 0b10000) != 0 /* is SpecializationActive[Array.readBufferFloat(Array, ByteOrder, long, BranchProfile)] */) {
{
BranchProfile exception_ = this.exception;
if (exception_ != null) {
return arg0Value.readBufferFloat(arg1Value, arg2Value, exception_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return readBufferFloatNode_AndSpecialize(arg0Value, arg1Value, arg2Value);
}
private float readBufferFloatNode_AndSpecialize(Array arg0Value, ByteOrder arg1Value, long arg2Value) throws InvalidBufferOffsetException {
int state_0 = this.state_0_;
BranchProfile exception_;
BranchProfile exception__shared = this.exception;
if (exception__shared != null) {
exception_ = exception__shared;
} else {
exception_ = (BranchProfile.create());
if (exception_ == null) {
throw new IllegalStateException("Specialization 'readBufferFloat(Array, ByteOrder, long, BranchProfile)' contains a shared cache with name 'exception' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.exception == null) {
VarHandle.storeStoreFence();
this.exception = exception_;
}
state_0 = state_0 | 0b10000 /* add SpecializationActive[Array.readBufferFloat(Array, ByteOrder, long, BranchProfile)] */;
this.state_0_ = state_0;
return arg0Value.readBufferFloat(arg1Value, arg2Value, exception_);
}
/**
* Debug Info:
* Specialization {@link Array#readBufferDouble(Array, ByteOrder, long, BranchProfile)}
* Activation probability: 0.16667
* With/without class size: 6/0 bytes
*
*/
@Override
public double readBufferDouble(Object arg0Value_, ByteOrder arg1Value, long arg2Value) throws UnsupportedMessageException, InvalidBufferOffsetException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
Array arg0Value = ((Array) arg0Value_);
int state_0 = this.state_0_;
if ((state_0 & 0b100000) != 0 /* is SpecializationActive[Array.readBufferDouble(Array, ByteOrder, long, BranchProfile)] */) {
{
BranchProfile exception_ = this.exception;
if (exception_ != null) {
return arg0Value.readBufferDouble(arg1Value, arg2Value, exception_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return readBufferDoubleNode_AndSpecialize(arg0Value, arg1Value, arg2Value);
}
private double readBufferDoubleNode_AndSpecialize(Array arg0Value, ByteOrder arg1Value, long arg2Value) throws InvalidBufferOffsetException {
int state_0 = this.state_0_;
BranchProfile exception_;
BranchProfile exception__shared = this.exception;
if (exception__shared != null) {
exception_ = exception__shared;
} else {
exception_ = (BranchProfile.create());
if (exception_ == null) {
throw new IllegalStateException("Specialization 'readBufferDouble(Array, ByteOrder, long, BranchProfile)' contains a shared cache with name 'exception' that returned a default value for the cached initializer. Default values are not supported for shared cached initializers because the default value is reserved for the uninitialized state.");
}
}
if (this.exception == null) {
VarHandle.storeStoreFence();
this.exception = exception_;
}
state_0 = state_0 | 0b100000 /* add SpecializationActive[Array.readBufferDouble(Array, ByteOrder, long, BranchProfile)] */;
this.state_0_ = state_0;
return arg0Value.readBufferDouble(arg1Value, arg2Value, exception_);
}
}
@GeneratedBy(Array.class)
@DenyReplace
private static final class Uncached extends InteropLibrary {
protected Uncached() {
}
@Override
@TruffleBoundary
public boolean accepts(Object receiver) {
assert !(receiver instanceof Array) || DYNAMIC_DISPATCH_LIBRARY_.getUncached().dispatch(receiver) == null : "Invalid library export. Exported receiver with dynamic dispatch found but not expected.";
return receiver instanceof Array;
}
@Override
public boolean isAdoptable() {
return false;
}
@Override
public NodeCost getCost() {
return NodeCost.MEGAMORPHIC;
}
@TruffleBoundary
@Override
public boolean hasBufferElements(Object receiver) {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((Array) receiver) .hasBufferElements();
}
@TruffleBoundary
@Override
public long getBufferSize(Object receiver) throws UnsupportedMessageException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((Array) receiver) .getBufferSize();
}
@TruffleBoundary
@Override
public byte readBufferByte(Object arg0Value_, long arg1Value) throws InvalidBufferOffsetException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
Array arg0Value = ((Array) arg0Value_);
return arg0Value.readBufferByte(arg1Value, (BranchProfile.getUncached()));
}
@TruffleBoundary
@Override
public short readBufferShort(Object arg0Value_, ByteOrder arg1Value, long arg2Value) throws InvalidBufferOffsetException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
Array arg0Value = ((Array) arg0Value_);
return arg0Value.readBufferShort(arg1Value, arg2Value, (BranchProfile.getUncached()));
}
@TruffleBoundary
@Override
public int readBufferInt(Object arg0Value_, ByteOrder arg1Value, long arg2Value) throws InvalidBufferOffsetException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
Array arg0Value = ((Array) arg0Value_);
return arg0Value.readBufferInt(arg1Value, arg2Value, (BranchProfile.getUncached()));
}
@TruffleBoundary
@Override
public long readBufferLong(Object arg0Value_, ByteOrder arg1Value, long arg2Value) throws InvalidBufferOffsetException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
Array arg0Value = ((Array) arg0Value_);
return arg0Value.readBufferLong(arg1Value, arg2Value, (BranchProfile.getUncached()));
}
@TruffleBoundary
@Override
public float readBufferFloat(Object arg0Value_, ByteOrder arg1Value, long arg2Value) throws InvalidBufferOffsetException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
Array arg0Value = ((Array) arg0Value_);
return arg0Value.readBufferFloat(arg1Value, arg2Value, (BranchProfile.getUncached()));
}
@TruffleBoundary
@Override
public double readBufferDouble(Object arg0Value_, ByteOrder arg1Value, long arg2Value) throws InvalidBufferOffsetException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
Array arg0Value = ((Array) arg0Value_);
return arg0Value.readBufferDouble(arg1Value, arg2Value, (BranchProfile.getUncached()));
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy