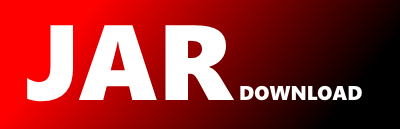
com.oracle.truffle.nfi.backend.libffi.ArrayGen Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of truffle-nfi-libffi Show documentation
Show all versions of truffle-nfi-libffi Show documentation
Implementation of the Truffle NFI using libffi.
The newest version!
// CheckStyle: start generated
package com.oracle.truffle.nfi.backend.libffi;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.CompilerDirectives.TruffleBoundary;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.DSLSupport.SpecializationDataNode;
import com.oracle.truffle.api.dsl.InlineSupport.InlineTarget;
import com.oracle.truffle.api.dsl.InlineSupport.ReferenceField;
import com.oracle.truffle.api.dsl.InlineSupport.StateField;
import com.oracle.truffle.api.dsl.InlineSupport.UnsafeAccessedField;
import com.oracle.truffle.api.interop.InteropLibrary;
import com.oracle.truffle.api.interop.InvalidBufferOffsetException;
import com.oracle.truffle.api.interop.UnsupportedMessageException;
import com.oracle.truffle.api.library.DynamicDispatchLibrary;
import com.oracle.truffle.api.library.LibraryExport;
import com.oracle.truffle.api.library.LibraryFactory;
import com.oracle.truffle.api.nodes.DenyReplace;
import com.oracle.truffle.api.nodes.EncapsulatingNodeReference;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.nodes.UnadoptableNode;
import com.oracle.truffle.api.profiles.InlinedBranchProfile;
import com.oracle.truffle.nfi.api.SerializableLibrary;
import com.oracle.truffle.nfi.backend.libffi.NativeBuffer.Array;
import java.lang.invoke.MethodHandles;
import java.nio.ByteOrder;
import java.util.Objects;
@GeneratedBy(Array.class)
@SuppressWarnings({"javadoc", "unused"})
final class ArrayGen {
private static final LibraryFactory DYNAMIC_DISPATCH_LIBRARY_ = LibraryFactory.resolve(DynamicDispatchLibrary.class);
private static final LibraryFactory INTEROP_LIBRARY_ = LibraryFactory.resolve(InteropLibrary.class);
static {
LibraryExport.register(Array.class, new SerializableLibraryExports(), new InteropLibraryExports());
}
private ArrayGen() {
}
@GeneratedBy(Array.class)
private static final class SerializableLibraryExports extends LibraryExport {
private SerializableLibraryExports() {
super(SerializableLibrary.class, Array.class, false, false, 0);
}
@Override
protected SerializableLibrary createUncached(Object receiver) {
assert receiver instanceof Array;
SerializableLibrary uncached = new Uncached();
return uncached;
}
@Override
protected SerializableLibrary createCached(Object receiver) {
assert receiver instanceof Array;
return new Cached();
}
@GeneratedBy(Array.class)
private static final class Cached extends SerializableLibrary {
static final ReferenceField SERIALIZE0_CACHE_UPDATER = ReferenceField.create(MethodHandles.lookup(), "serialize0_cache", Serialize0Data.class);
/**
* State Info:
* 0: SpecializationActive {@link Array#serialize(Array, Object, InteropLibrary)}
* 1: SpecializationActive {@link Array#serialize(Array, Object, InteropLibrary)}
*
*/
@CompilationFinal private int state_0_;
@UnsafeAccessedField @Child private Serialize0Data serialize0_cache;
protected Cached() {
}
@Override
public boolean accepts(Object receiver) {
assert !(receiver instanceof Array) || DYNAMIC_DISPATCH_LIBRARY_.getUncached().dispatch(receiver) == null : "Invalid library export. Exported receiver with dynamic dispatch found but not expected.";
return receiver instanceof Array;
}
@Override
public boolean isSerializable(Object receiver) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
return (((Array) receiver)).isSerializable();
}
/**
* Debug Info:
* Specialization {@link Array#serialize(Array, Object, InteropLibrary)}
* Activation probability: 0.65000
* With/without class size: 17/4 bytes
* Specialization {@link Array#serialize(Array, Object, InteropLibrary)}
* Activation probability: 0.35000
* With/without class size: 8/0 bytes
*
*/
@Override
public void serialize(Object arg0Value_, Object arg1Value) throws UnsupportedMessageException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
Array arg0Value = ((Array) arg0Value_);
int state_0 = this.state_0_;
if (state_0 != 0 /* is SpecializationActive[Array.serialize(Array, Object, InteropLibrary)] || SpecializationActive[Array.serialize(Array, Object, InteropLibrary)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[Array.serialize(Array, Object, InteropLibrary)] */) {
Serialize0Data s0_ = this.serialize0_cache;
if (s0_ != null) {
if ((s0_.interop_.accepts(arg1Value))) {
arg0Value.serialize(arg1Value, s0_.interop_);
return;
}
}
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[Array.serialize(Array, Object, InteropLibrary)] */) {
this.serializeNode__Serialize1Boundary(state_0, arg0Value, arg1Value);
return;
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
executeAndSpecialize(arg0Value, arg1Value);
return;
}
@SuppressWarnings("static-method")
@TruffleBoundary
private void serializeNode__Serialize1Boundary(int state_0, Array arg0Value, Object arg1Value) throws UnsupportedMessageException {
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(this);
try {
{
InteropLibrary interop__ = (INTEROP_LIBRARY_.getUncached(arg1Value));
arg0Value.serialize(arg1Value, interop__);
return;
}
} finally {
encapsulating_.set(prev_);
}
}
private void executeAndSpecialize(Array arg0Value, Object arg1Value) {
int state_0 = this.state_0_;
if (((state_0 & 0b10)) == 0 /* is-not SpecializationActive[Array.serialize(Array, Object, InteropLibrary)] */) {
while (true) {
int count0_ = 0;
Serialize0Data s0_ = SERIALIZE0_CACHE_UPDATER.getVolatile(this);
Serialize0Data s0_original = s0_;
while (s0_ != null) {
if ((s0_.interop_.accepts(arg1Value))) {
break;
}
count0_++;
s0_ = null;
break;
}
if (s0_ == null && count0_ < 1) {
// assert (s0_.interop_.accepts(arg1Value));
s0_ = this.insert(new Serialize0Data());
InteropLibrary interop__ = s0_.insert((INTEROP_LIBRARY_.create(arg1Value)));
Objects.requireNonNull(interop__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
s0_.interop_ = interop__;
if (!SERIALIZE0_CACHE_UPDATER.compareAndSet(this, s0_original, s0_)) {
continue;
}
state_0 = state_0 | 0b1 /* add SpecializationActive[Array.serialize(Array, Object, InteropLibrary)] */;
this.state_0_ = state_0;
}
if (s0_ != null) {
arg0Value.serialize(arg1Value, s0_.interop_);
return;
}
break;
}
}
{
InteropLibrary interop__ = null;
{
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(this);
try {
interop__ = (INTEROP_LIBRARY_.getUncached(arg1Value));
this.serialize0_cache = null;
state_0 = state_0 & 0xfffffffe /* remove SpecializationActive[Array.serialize(Array, Object, InteropLibrary)] */;
state_0 = state_0 | 0b10 /* add SpecializationActive[Array.serialize(Array, Object, InteropLibrary)] */;
this.state_0_ = state_0;
arg0Value.serialize(arg1Value, interop__);
return;
} finally {
encapsulating_.set(prev_);
}
}
}
}
@GeneratedBy(Array.class)
@DenyReplace
private static final class Serialize0Data extends Node implements SpecializationDataNode {
/**
* Source Info:
* Specialization: {@link Array#serialize(Array, Object, InteropLibrary)}
* Parameter: {@link InteropLibrary} interop
*/
@Child InteropLibrary interop_;
Serialize0Data() {
}
}
}
@GeneratedBy(Array.class)
@DenyReplace
private static final class Uncached extends SerializableLibrary implements UnadoptableNode {
protected Uncached() {
}
@Override
@TruffleBoundary
public boolean accepts(Object receiver) {
assert !(receiver instanceof Array) || DYNAMIC_DISPATCH_LIBRARY_.getUncached().dispatch(receiver) == null : "Invalid library export. Exported receiver with dynamic dispatch found but not expected.";
return receiver instanceof Array;
}
@TruffleBoundary
@Override
public boolean isSerializable(Object receiver) {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((Array) receiver) .isSerializable();
}
@TruffleBoundary
@Override
public void serialize(Object arg0Value_, Object arg1Value) {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
Array arg0Value = ((Array) arg0Value_);
arg0Value.serialize(arg1Value, (INTEROP_LIBRARY_.getUncached(arg1Value)));
return;
}
}
}
@GeneratedBy(Array.class)
private static final class InteropLibraryExports extends LibraryExport {
private InteropLibraryExports() {
super(InteropLibrary.class, Array.class, false, false, 0);
}
@Override
protected InteropLibrary createUncached(Object receiver) {
assert receiver instanceof Array;
InteropLibrary uncached = new Uncached();
return uncached;
}
@Override
protected InteropLibrary createCached(Object receiver) {
assert receiver instanceof Array;
return new Cached();
}
@GeneratedBy(Array.class)
private static final class Cached extends InteropLibrary {
private static final StateField STATE_0_UPDATER = StateField.create(MethodHandles.lookup(), "state_0_");
/**
* Source Info:
* Specialization: {@link Array#readBufferByte}
* Parameter: {@link InlinedBranchProfile} exception
* Inline method: {@link InlinedBranchProfile#inline}
*/
private static final InlinedBranchProfile INLINED_EXCEPTION = InlinedBranchProfile.inline(InlineTarget.create(InlinedBranchProfile.class, STATE_0_UPDATER.subUpdater(0, 1)));
/**
* State Info:
* 0: InlinedCache
* Specialization: {@link Array#readBufferByte}
* Parameter: {@link InlinedBranchProfile} exception
* Inline method: {@link InlinedBranchProfile#inline}
*
*/
@CompilationFinal @UnsafeAccessedField private int state_0_;
protected Cached() {
}
@Override
public boolean accepts(Object receiver) {
assert !(receiver instanceof Array) || DYNAMIC_DISPATCH_LIBRARY_.getUncached().dispatch(receiver) == null : "Invalid library export. Exported receiver with dynamic dispatch found but not expected.";
return receiver instanceof Array;
}
@Override
public boolean hasBufferElements(Object receiver) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
return (((Array) receiver)).hasBufferElements();
}
@Override
public long getBufferSize(Object receiver) throws UnsupportedMessageException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
return (((Array) receiver)).getBufferSize();
}
/**
* Debug Info:
* Specialization {@link Array#readBufferByte(Array, long, Node, InlinedBranchProfile)}
* Activation probability: 0.14286
* With/without class size: 6/0 bytes
*
*/
@Override
public byte readBufferByte(Object arg0Value_, long arg1Value) throws UnsupportedMessageException, InvalidBufferOffsetException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
Array arg0Value = ((Array) arg0Value_);
{
Node node__ = (this);
return arg0Value.readBufferByte(arg1Value, node__, INLINED_EXCEPTION);
}
}
/**
* Debug Info:
* Specialization {@link Array#readBuffer(Array, long, byte[], int, int, Node, InlinedBranchProfile)}
* Activation probability: 0.14286
* With/without class size: 6/0 bytes
*
*/
@Override
public void readBuffer(Object arg0Value_, long arg1Value, byte[] arg2Value, int arg3Value, int arg4Value) throws UnsupportedMessageException, InvalidBufferOffsetException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
Array arg0Value = ((Array) arg0Value_);
{
Node node__ = (this);
arg0Value.readBuffer(arg1Value, arg2Value, arg3Value, arg4Value, node__, INLINED_EXCEPTION);
return;
}
}
/**
* Debug Info:
* Specialization {@link Array#readBufferShort(Array, ByteOrder, long, Node, InlinedBranchProfile)}
* Activation probability: 0.14286
* With/without class size: 6/0 bytes
*
*/
@Override
public short readBufferShort(Object arg0Value_, ByteOrder arg1Value, long arg2Value) throws UnsupportedMessageException, InvalidBufferOffsetException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
Array arg0Value = ((Array) arg0Value_);
{
Node node__ = (this);
return arg0Value.readBufferShort(arg1Value, arg2Value, node__, INLINED_EXCEPTION);
}
}
/**
* Debug Info:
* Specialization {@link Array#readBufferInt(Array, ByteOrder, long, Node, InlinedBranchProfile)}
* Activation probability: 0.14286
* With/without class size: 6/0 bytes
*
*/
@Override
public int readBufferInt(Object arg0Value_, ByteOrder arg1Value, long arg2Value) throws UnsupportedMessageException, InvalidBufferOffsetException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
Array arg0Value = ((Array) arg0Value_);
{
Node node__ = (this);
return arg0Value.readBufferInt(arg1Value, arg2Value, node__, INLINED_EXCEPTION);
}
}
/**
* Debug Info:
* Specialization {@link Array#readBufferLong(Array, ByteOrder, long, Node, InlinedBranchProfile)}
* Activation probability: 0.14286
* With/without class size: 6/0 bytes
*
*/
@Override
public long readBufferLong(Object arg0Value_, ByteOrder arg1Value, long arg2Value) throws UnsupportedMessageException, InvalidBufferOffsetException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
Array arg0Value = ((Array) arg0Value_);
{
Node node__ = (this);
return arg0Value.readBufferLong(arg1Value, arg2Value, node__, INLINED_EXCEPTION);
}
}
/**
* Debug Info:
* Specialization {@link Array#readBufferFloat(Array, ByteOrder, long, Node, InlinedBranchProfile)}
* Activation probability: 0.14286
* With/without class size: 6/0 bytes
*
*/
@Override
public float readBufferFloat(Object arg0Value_, ByteOrder arg1Value, long arg2Value) throws UnsupportedMessageException, InvalidBufferOffsetException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
Array arg0Value = ((Array) arg0Value_);
{
Node node__ = (this);
return arg0Value.readBufferFloat(arg1Value, arg2Value, node__, INLINED_EXCEPTION);
}
}
/**
* Debug Info:
* Specialization {@link Array#readBufferDouble(Array, ByteOrder, long, Node, InlinedBranchProfile)}
* Activation probability: 0.14286
* With/without class size: 6/0 bytes
*
*/
@Override
public double readBufferDouble(Object arg0Value_, ByteOrder arg1Value, long arg2Value) throws UnsupportedMessageException, InvalidBufferOffsetException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
Array arg0Value = ((Array) arg0Value_);
{
Node node__ = (this);
return arg0Value.readBufferDouble(arg1Value, arg2Value, node__, INLINED_EXCEPTION);
}
}
}
@GeneratedBy(Array.class)
@DenyReplace
private static final class Uncached extends InteropLibrary implements UnadoptableNode {
protected Uncached() {
}
@Override
@TruffleBoundary
public boolean accepts(Object receiver) {
assert !(receiver instanceof Array) || DYNAMIC_DISPATCH_LIBRARY_.getUncached().dispatch(receiver) == null : "Invalid library export. Exported receiver with dynamic dispatch found but not expected.";
return receiver instanceof Array;
}
@TruffleBoundary
@Override
public boolean hasBufferElements(Object receiver) {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((Array) receiver) .hasBufferElements();
}
@TruffleBoundary
@Override
public long getBufferSize(Object receiver) throws UnsupportedMessageException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((Array) receiver) .getBufferSize();
}
@TruffleBoundary
@Override
public byte readBufferByte(Object arg0Value_, long arg1Value) throws InvalidBufferOffsetException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
Array arg0Value = ((Array) arg0Value_);
return arg0Value.readBufferByte(arg1Value, (this), (InlinedBranchProfile.getUncached()));
}
@TruffleBoundary
@Override
public void readBuffer(Object arg0Value_, long arg1Value, byte[] arg2Value, int arg3Value, int arg4Value) throws InvalidBufferOffsetException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
Array arg0Value = ((Array) arg0Value_);
arg0Value.readBuffer(arg1Value, arg2Value, arg3Value, arg4Value, (this), (InlinedBranchProfile.getUncached()));
return;
}
@TruffleBoundary
@Override
public short readBufferShort(Object arg0Value_, ByteOrder arg1Value, long arg2Value) throws InvalidBufferOffsetException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
Array arg0Value = ((Array) arg0Value_);
return arg0Value.readBufferShort(arg1Value, arg2Value, (this), (InlinedBranchProfile.getUncached()));
}
@TruffleBoundary
@Override
public int readBufferInt(Object arg0Value_, ByteOrder arg1Value, long arg2Value) throws InvalidBufferOffsetException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
Array arg0Value = ((Array) arg0Value_);
return arg0Value.readBufferInt(arg1Value, arg2Value, (this), (InlinedBranchProfile.getUncached()));
}
@TruffleBoundary
@Override
public long readBufferLong(Object arg0Value_, ByteOrder arg1Value, long arg2Value) throws InvalidBufferOffsetException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
Array arg0Value = ((Array) arg0Value_);
return arg0Value.readBufferLong(arg1Value, arg2Value, (this), (InlinedBranchProfile.getUncached()));
}
@TruffleBoundary
@Override
public float readBufferFloat(Object arg0Value_, ByteOrder arg1Value, long arg2Value) throws InvalidBufferOffsetException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
Array arg0Value = ((Array) arg0Value_);
return arg0Value.readBufferFloat(arg1Value, arg2Value, (this), (InlinedBranchProfile.getUncached()));
}
@TruffleBoundary
@Override
public double readBufferDouble(Object arg0Value_, ByteOrder arg1Value, long arg2Value) throws InvalidBufferOffsetException {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
Array arg0Value = ((Array) arg0Value_);
return arg0Value.readBufferDouble(arg1Value, arg2Value, (this), (InlinedBranchProfile.getUncached()));
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy