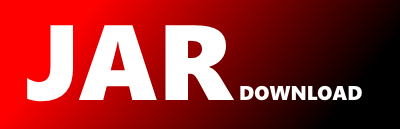
com.oracle.truffle.sl.builtins.SLAddToHostClassPathBuiltinFactory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of truffle-sl Show documentation
Show all versions of truffle-sl Show documentation
Truffle SL is an example language implemented using the Truffle API.
The newest version!
// CheckStyle: start generated
package com.oracle.truffle.sl.builtins;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.NeverDefault;
import com.oracle.truffle.api.dsl.NodeFactory;
import com.oracle.truffle.api.dsl.UnsupportedSpecializationException;
import com.oracle.truffle.api.frame.VirtualFrame;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.strings.TruffleString;
import com.oracle.truffle.api.strings.TruffleString.ToJavaStringNode;
import com.oracle.truffle.sl.nodes.SLExpressionNode;
import java.lang.invoke.VarHandle;
import java.util.List;
import java.util.Objects;
@GeneratedBy(SLAddToHostClassPathBuiltin.class)
@SuppressWarnings("javadoc")
public final class SLAddToHostClassPathBuiltinFactory implements NodeFactory {
private static final SLAddToHostClassPathBuiltinFactory INSTANCE = new SLAddToHostClassPathBuiltinFactory();
private SLAddToHostClassPathBuiltinFactory() {
}
@Override
public Class getNodeClass() {
return SLAddToHostClassPathBuiltin.class;
}
@Override
public List> getExecutionSignature() {
return List.of(SLExpressionNode.class);
}
@Override
public List>> getNodeSignatures() {
return List.of(List.of(SLExpressionNode[].class));
}
@Override
public SLAddToHostClassPathBuiltin createNode(Object... arguments) {
if (arguments.length == 1 && (arguments[0] == null || arguments[0] instanceof SLExpressionNode[])) {
return create((SLExpressionNode[]) arguments[0]);
} else {
throw new IllegalArgumentException("Invalid create signature.");
}
}
public static NodeFactory getInstance() {
return INSTANCE;
}
@NeverDefault
public static SLAddToHostClassPathBuiltin create(SLExpressionNode[] arguments) {
return new SLAddToHostClassPathBuiltinNodeGen(arguments);
}
/**
* Debug Info:
* Specialization {@link SLAddToHostClassPathBuiltin#doDefault}
* Activation probability: 1.00000
* With/without class size: 24/4 bytes
*
*/
@GeneratedBy(SLAddToHostClassPathBuiltin.class)
@SuppressWarnings("javadoc")
public static final class SLAddToHostClassPathBuiltinNodeGen extends SLAddToHostClassPathBuiltin {
@Child private SLExpressionNode arguments0_;
/**
* State Info:
* 0: SpecializationActive {@link SLAddToHostClassPathBuiltin#doDefault}
*
*/
@CompilationFinal private int state_0_;
/**
* Source Info:
* Specialization: {@link SLAddToHostClassPathBuiltin#doDefault}
* Parameter: {@link ToJavaStringNode} toJavaStringNode
*/
@Child private ToJavaStringNode toJavaStringNode_;
private SLAddToHostClassPathBuiltinNodeGen(SLExpressionNode[] arguments) {
this.arguments0_ = arguments != null && 0 < arguments.length ? arguments[0] : null;
}
@Override
protected Object execute(VirtualFrame frameValue) {
int state_0 = this.state_0_;
Object arguments0Value_ = this.arguments0_.executeGeneric(frameValue);
if (state_0 != 0 /* is SpecializationActive[SLAddToHostClassPathBuiltin.doDefault(TruffleString, ToJavaStringNode)] */ && arguments0Value_ instanceof TruffleString) {
TruffleString arguments0Value__ = (TruffleString) arguments0Value_;
{
ToJavaStringNode toJavaStringNode__ = this.toJavaStringNode_;
if (toJavaStringNode__ != null) {
return doDefault(arguments0Value__, toJavaStringNode__);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arguments0Value_);
}
private Object executeAndSpecialize(Object arguments0Value) {
int state_0 = this.state_0_;
if (arguments0Value instanceof TruffleString) {
TruffleString arguments0Value_ = (TruffleString) arguments0Value;
ToJavaStringNode toJavaStringNode__ = this.insert((ToJavaStringNode.create()));
Objects.requireNonNull(toJavaStringNode__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
VarHandle.storeStoreFence();
this.toJavaStringNode_ = toJavaStringNode__;
state_0 = state_0 | 0b1 /* add SpecializationActive[SLAddToHostClassPathBuiltin.doDefault(TruffleString, ToJavaStringNode)] */;
this.state_0_ = state_0;
return doDefault(arguments0Value_, toJavaStringNode__);
}
throw new UnsupportedSpecializationException(this, new Node[] {this.arguments0_}, arguments0Value);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy