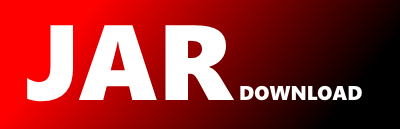
com.oracle.truffle.sl.nodes.expression.SLEqualNodeGen Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of truffle-sl Show documentation
Show all versions of truffle-sl Show documentation
Truffle SL is an example language implemented using the Truffle API.
The newest version!
// CheckStyle: start generated
package com.oracle.truffle.sl.nodes.expression;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.CompilerDirectives.TruffleBoundary;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.NeverDefault;
import com.oracle.truffle.api.dsl.DSLSupport.SpecializationDataNode;
import com.oracle.truffle.api.dsl.InlineSupport.ReferenceField;
import com.oracle.truffle.api.dsl.InlineSupport.UnsafeAccessedField;
import com.oracle.truffle.api.frame.VirtualFrame;
import com.oracle.truffle.api.interop.InteropLibrary;
import com.oracle.truffle.api.library.LibraryFactory;
import com.oracle.truffle.api.nodes.DenyReplace;
import com.oracle.truffle.api.nodes.EncapsulatingNodeReference;
import com.oracle.truffle.api.nodes.ExplodeLoop;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.nodes.UnexpectedResultException;
import com.oracle.truffle.api.strings.TruffleString;
import com.oracle.truffle.api.strings.TruffleString.EqualNode;
import com.oracle.truffle.sl.nodes.SLExpressionNode;
import com.oracle.truffle.sl.nodes.SLTypes;
import com.oracle.truffle.sl.nodes.SLTypesGen;
import com.oracle.truffle.sl.runtime.SLBigInteger;
import com.oracle.truffle.sl.runtime.SLFunction;
import com.oracle.truffle.sl.runtime.SLNull;
import java.lang.invoke.MethodHandles;
import java.lang.invoke.VarHandle;
import java.util.Objects;
/**
* Debug Info:
* Specialization {@link SLEqualNode#doLong}
* Activation probability: 0.19111
* With/without class size: 6/0 bytes
* Specialization {@link SLEqualNode#doBigNumber}
* Activation probability: 0.17111
* With/without class size: 6/0 bytes
* Specialization {@link SLEqualNode#doBoolean}
* Activation probability: 0.15111
* With/without class size: 5/0 bytes
* Specialization {@link SLEqualNode#doString}
* Activation probability: 0.13111
* With/without class size: 5/0 bytes
* Specialization {@link SLEqualNode#doTruffleString}
* Activation probability: 0.11111
* With/without class size: 6/4 bytes
* Specialization {@link SLEqualNode#doNull}
* Activation probability: 0.09111
* With/without class size: 5/0 bytes
* Specialization {@link SLEqualNode#doFunction}
* Activation probability: 0.07111
* With/without class size: 4/0 bytes
* Specialization {@link SLEqualNode#doGeneric}
* Activation probability: 0.05111
* With/without class size: 5/8 bytes
* Specialization {@link SLEqualNode#doGeneric}
* Activation probability: 0.03111
* With/without class size: 4/0 bytes
*
*/
@GeneratedBy(SLEqualNode.class)
@SuppressWarnings({"javadoc", "unused"})
public final class SLEqualNodeGen extends SLEqualNode {
static final ReferenceField GENERIC0_CACHE_UPDATER = ReferenceField.create(MethodHandles.lookup(), "generic0_cache", Generic0Data.class);
private static final LibraryFactory INTEROP_LIBRARY_ = LibraryFactory.resolve(InteropLibrary.class);
@Child private SLExpressionNode leftNode_;
@Child private SLExpressionNode rightNode_;
/**
* State Info:
* 0: SpecializationActive {@link SLEqualNode#doLong}
* 1: SpecializationActive {@link SLEqualNode#doBigNumber}
* 2: SpecializationActive {@link SLEqualNode#doBoolean}
* 3: SpecializationActive {@link SLEqualNode#doString}
* 4: SpecializationActive {@link SLEqualNode#doTruffleString}
* 5: SpecializationActive {@link SLEqualNode#doNull}
* 6: SpecializationActive {@link SLEqualNode#doFunction}
* 7: SpecializationActive {@link SLEqualNode#doGeneric}
* 8: SpecializationActive {@link SLEqualNode#doGeneric}
* 9-10: ImplicitCast[type=SLBigInteger, index=0]
* 11-12: ImplicitCast[type=SLBigInteger, index=1]
*
*/
@CompilationFinal private int state_0_;
/**
* Source Info:
* Specialization: {@link SLEqualNode#doTruffleString}
* Parameter: {@link EqualNode} equalNode
*/
@Child private EqualNode truffleString_equalNode_;
@UnsafeAccessedField @Child private Generic0Data generic0_cache;
private SLEqualNodeGen(SLExpressionNode leftNode, SLExpressionNode rightNode) {
this.leftNode_ = leftNode;
this.rightNode_ = rightNode;
}
@Override
public Object executeGeneric(VirtualFrame frameValue) {
int state_0 = this.state_0_;
if ((state_0 & 0b111111110) == 0 /* only-active SpecializationActive[SLEqualNode.doLong(long, long)] */ && ((state_0 & 0b111111111) != 0 /* is-not SpecializationActive[SLEqualNode.doLong(long, long)] && SpecializationActive[SLEqualNode.doBigNumber(SLBigInteger, SLBigInteger)] && SpecializationActive[SLEqualNode.doBoolean(boolean, boolean)] && SpecializationActive[SLEqualNode.doString(String, String)] && SpecializationActive[SLEqualNode.doTruffleString(TruffleString, TruffleString, EqualNode)] && SpecializationActive[SLEqualNode.doNull(SLNull, SLNull)] && SpecializationActive[SLEqualNode.doFunction(SLFunction, Object)] && SpecializationActive[SLEqualNode.doGeneric(Object, Object, InteropLibrary, InteropLibrary)] && SpecializationActive[SLEqualNode.doGeneric(Object, Object, InteropLibrary, InteropLibrary)] */)) {
return executeGeneric_long_long0(state_0, frameValue);
} else if ((state_0 & 0b111111011) == 0 /* only-active SpecializationActive[SLEqualNode.doBoolean(boolean, boolean)] */ && ((state_0 & 0b111111111) != 0 /* is-not SpecializationActive[SLEqualNode.doLong(long, long)] && SpecializationActive[SLEqualNode.doBigNumber(SLBigInteger, SLBigInteger)] && SpecializationActive[SLEqualNode.doBoolean(boolean, boolean)] && SpecializationActive[SLEqualNode.doString(String, String)] && SpecializationActive[SLEqualNode.doTruffleString(TruffleString, TruffleString, EqualNode)] && SpecializationActive[SLEqualNode.doNull(SLNull, SLNull)] && SpecializationActive[SLEqualNode.doFunction(SLFunction, Object)] && SpecializationActive[SLEqualNode.doGeneric(Object, Object, InteropLibrary, InteropLibrary)] && SpecializationActive[SLEqualNode.doGeneric(Object, Object, InteropLibrary, InteropLibrary)] */)) {
return executeGeneric_boolean_boolean1(state_0, frameValue);
} else {
return executeGeneric_generic2(state_0, frameValue);
}
}
private Object executeGeneric_long_long0(int state_0__, VirtualFrame frameValue) {
int state_0 = state_0__;
long leftNodeValue_;
try {
leftNodeValue_ = this.leftNode_.executeLong(frameValue);
} catch (UnexpectedResultException ex) {
CompilerDirectives.transferToInterpreterAndInvalidate();
Object rightNodeValue = this.rightNode_.executeGeneric(frameValue);
return executeAndSpecialize(ex.getResult(), rightNodeValue);
}
long rightNodeValue_;
try {
rightNodeValue_ = this.rightNode_.executeLong(frameValue);
} catch (UnexpectedResultException ex) {
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(leftNodeValue_, ex.getResult());
}
assert (state_0 & 0b1) != 0 /* is SpecializationActive[SLEqualNode.doLong(long, long)] */;
return doLong(leftNodeValue_, rightNodeValue_);
}
private Object executeGeneric_boolean_boolean1(int state_0__, VirtualFrame frameValue) {
int state_0 = state_0__;
boolean leftNodeValue_;
try {
leftNodeValue_ = this.leftNode_.executeBoolean(frameValue);
} catch (UnexpectedResultException ex) {
CompilerDirectives.transferToInterpreterAndInvalidate();
Object rightNodeValue = this.rightNode_.executeGeneric(frameValue);
return executeAndSpecialize(ex.getResult(), rightNodeValue);
}
boolean rightNodeValue_;
try {
rightNodeValue_ = this.rightNode_.executeBoolean(frameValue);
} catch (UnexpectedResultException ex) {
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(leftNodeValue_, ex.getResult());
}
assert (state_0 & 0b100) != 0 /* is SpecializationActive[SLEqualNode.doBoolean(boolean, boolean)] */;
return doBoolean(leftNodeValue_, rightNodeValue_);
}
@SuppressWarnings("static-method")
@TruffleBoundary
private Object generic1Boundary(int state_0, Object leftNodeValue_, Object rightNodeValue_) {
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(this);
try {
{
InteropLibrary leftInterop__ = (INTEROP_LIBRARY_.getUncached(leftNodeValue_));
InteropLibrary rightInterop__ = (INTEROP_LIBRARY_.getUncached(rightNodeValue_));
return doGeneric(leftNodeValue_, rightNodeValue_, leftInterop__, rightInterop__);
}
} finally {
encapsulating_.set(prev_);
}
}
@ExplodeLoop
private Object executeGeneric_generic2(int state_0__, VirtualFrame frameValue) {
int state_0 = state_0__;
Object leftNodeValue_ = this.leftNode_.executeGeneric(frameValue);
Object rightNodeValue_ = this.rightNode_.executeGeneric(frameValue);
if ((state_0 & 0b111111111) != 0 /* is SpecializationActive[SLEqualNode.doLong(long, long)] || SpecializationActive[SLEqualNode.doBigNumber(SLBigInteger, SLBigInteger)] || SpecializationActive[SLEqualNode.doBoolean(boolean, boolean)] || SpecializationActive[SLEqualNode.doString(String, String)] || SpecializationActive[SLEqualNode.doTruffleString(TruffleString, TruffleString, EqualNode)] || SpecializationActive[SLEqualNode.doNull(SLNull, SLNull)] || SpecializationActive[SLEqualNode.doFunction(SLFunction, Object)] || SpecializationActive[SLEqualNode.doGeneric(Object, Object, InteropLibrary, InteropLibrary)] || SpecializationActive[SLEqualNode.doGeneric(Object, Object, InteropLibrary, InteropLibrary)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[SLEqualNode.doLong(long, long)] */ && leftNodeValue_ instanceof Long) {
long leftNodeValue__ = (long) leftNodeValue_;
if (rightNodeValue_ instanceof Long) {
long rightNodeValue__ = (long) rightNodeValue_;
return doLong(leftNodeValue__, rightNodeValue__);
}
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[SLEqualNode.doBigNumber(SLBigInteger, SLBigInteger)] */ && SLTypesGen.isImplicitSLBigInteger((state_0 & 0b11000000000) >>> 9 /* get-int ImplicitCast[type=SLBigInteger, index=0] */, leftNodeValue_)) {
SLBigInteger leftNodeValue__ = SLTypesGen.asImplicitSLBigInteger((state_0 & 0b11000000000) >>> 9 /* get-int ImplicitCast[type=SLBigInteger, index=0] */, leftNodeValue_);
if (SLTypesGen.isImplicitSLBigInteger((state_0 & 0b1100000000000) >>> 11 /* get-int ImplicitCast[type=SLBigInteger, index=1] */, rightNodeValue_)) {
SLBigInteger rightNodeValue__ = SLTypesGen.asImplicitSLBigInteger((state_0 & 0b1100000000000) >>> 11 /* get-int ImplicitCast[type=SLBigInteger, index=1] */, rightNodeValue_);
return doBigNumber(leftNodeValue__, rightNodeValue__);
}
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[SLEqualNode.doBoolean(boolean, boolean)] */ && leftNodeValue_ instanceof Boolean) {
boolean leftNodeValue__ = (boolean) leftNodeValue_;
if (rightNodeValue_ instanceof Boolean) {
boolean rightNodeValue__ = (boolean) rightNodeValue_;
return doBoolean(leftNodeValue__, rightNodeValue__);
}
}
if ((state_0 & 0b1000) != 0 /* is SpecializationActive[SLEqualNode.doString(String, String)] */ && leftNodeValue_ instanceof String) {
String leftNodeValue__ = (String) leftNodeValue_;
if (rightNodeValue_ instanceof String) {
String rightNodeValue__ = (String) rightNodeValue_;
return doString(leftNodeValue__, rightNodeValue__);
}
}
if ((state_0 & 0b10000) != 0 /* is SpecializationActive[SLEqualNode.doTruffleString(TruffleString, TruffleString, EqualNode)] */ && leftNodeValue_ instanceof TruffleString) {
TruffleString leftNodeValue__ = (TruffleString) leftNodeValue_;
if (rightNodeValue_ instanceof TruffleString) {
TruffleString rightNodeValue__ = (TruffleString) rightNodeValue_;
{
EqualNode equalNode__ = this.truffleString_equalNode_;
if (equalNode__ != null) {
return doTruffleString(leftNodeValue__, rightNodeValue__, equalNode__);
}
}
}
}
if ((state_0 & 0b100000) != 0 /* is SpecializationActive[SLEqualNode.doNull(SLNull, SLNull)] */ && SLTypes.isSLNull(leftNodeValue_)) {
SLNull leftNodeValue__ = SLTypes.asSLNull(leftNodeValue_);
if (SLTypes.isSLNull(rightNodeValue_)) {
SLNull rightNodeValue__ = SLTypes.asSLNull(rightNodeValue_);
return doNull(leftNodeValue__, rightNodeValue__);
}
}
if ((state_0 & 0b111000000) != 0 /* is SpecializationActive[SLEqualNode.doFunction(SLFunction, Object)] || SpecializationActive[SLEqualNode.doGeneric(Object, Object, InteropLibrary, InteropLibrary)] || SpecializationActive[SLEqualNode.doGeneric(Object, Object, InteropLibrary, InteropLibrary)] */) {
if ((state_0 & 0b1000000) != 0 /* is SpecializationActive[SLEqualNode.doFunction(SLFunction, Object)] */ && leftNodeValue_ instanceof SLFunction) {
SLFunction leftNodeValue__ = (SLFunction) leftNodeValue_;
return doFunction(leftNodeValue__, rightNodeValue_);
}
if ((state_0 & 0b110000000) != 0 /* is SpecializationActive[SLEqualNode.doGeneric(Object, Object, InteropLibrary, InteropLibrary)] || SpecializationActive[SLEqualNode.doGeneric(Object, Object, InteropLibrary, InteropLibrary)] */) {
if ((state_0 & 0b10000000) != 0 /* is SpecializationActive[SLEqualNode.doGeneric(Object, Object, InteropLibrary, InteropLibrary)] */) {
Generic0Data s7_ = this.generic0_cache;
while (s7_ != null) {
if ((s7_.leftInterop_.accepts(leftNodeValue_)) && (s7_.rightInterop_.accepts(rightNodeValue_))) {
return doGeneric(leftNodeValue_, rightNodeValue_, s7_.leftInterop_, s7_.rightInterop_);
}
s7_ = s7_.next_;
}
}
if ((state_0 & 0b100000000) != 0 /* is SpecializationActive[SLEqualNode.doGeneric(Object, Object, InteropLibrary, InteropLibrary)] */) {
return this.generic1Boundary(state_0, leftNodeValue_, rightNodeValue_);
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(leftNodeValue_, rightNodeValue_);
}
@Override
public boolean executeBoolean(VirtualFrame frameValue) {
int state_0 = this.state_0_;
if ((state_0 & 0b111111110) == 0 /* only-active SpecializationActive[SLEqualNode.doLong(long, long)] */ && ((state_0 & 0b111111111) != 0 /* is-not SpecializationActive[SLEqualNode.doLong(long, long)] && SpecializationActive[SLEqualNode.doBigNumber(SLBigInteger, SLBigInteger)] && SpecializationActive[SLEqualNode.doBoolean(boolean, boolean)] && SpecializationActive[SLEqualNode.doString(String, String)] && SpecializationActive[SLEqualNode.doTruffleString(TruffleString, TruffleString, EqualNode)] && SpecializationActive[SLEqualNode.doNull(SLNull, SLNull)] && SpecializationActive[SLEqualNode.doFunction(SLFunction, Object)] && SpecializationActive[SLEqualNode.doGeneric(Object, Object, InteropLibrary, InteropLibrary)] && SpecializationActive[SLEqualNode.doGeneric(Object, Object, InteropLibrary, InteropLibrary)] */)) {
return executeBoolean_long_long3(state_0, frameValue);
} else if ((state_0 & 0b111111011) == 0 /* only-active SpecializationActive[SLEqualNode.doBoolean(boolean, boolean)] */ && ((state_0 & 0b111111111) != 0 /* is-not SpecializationActive[SLEqualNode.doLong(long, long)] && SpecializationActive[SLEqualNode.doBigNumber(SLBigInteger, SLBigInteger)] && SpecializationActive[SLEqualNode.doBoolean(boolean, boolean)] && SpecializationActive[SLEqualNode.doString(String, String)] && SpecializationActive[SLEqualNode.doTruffleString(TruffleString, TruffleString, EqualNode)] && SpecializationActive[SLEqualNode.doNull(SLNull, SLNull)] && SpecializationActive[SLEqualNode.doFunction(SLFunction, Object)] && SpecializationActive[SLEqualNode.doGeneric(Object, Object, InteropLibrary, InteropLibrary)] && SpecializationActive[SLEqualNode.doGeneric(Object, Object, InteropLibrary, InteropLibrary)] */)) {
return executeBoolean_boolean_boolean4(state_0, frameValue);
} else {
return executeBoolean_generic5(state_0, frameValue);
}
}
private boolean executeBoolean_long_long3(int state_0__, VirtualFrame frameValue) {
int state_0 = state_0__;
long leftNodeValue_;
try {
leftNodeValue_ = this.leftNode_.executeLong(frameValue);
} catch (UnexpectedResultException ex) {
CompilerDirectives.transferToInterpreterAndInvalidate();
Object rightNodeValue = this.rightNode_.executeGeneric(frameValue);
return executeAndSpecialize(ex.getResult(), rightNodeValue);
}
long rightNodeValue_;
try {
rightNodeValue_ = this.rightNode_.executeLong(frameValue);
} catch (UnexpectedResultException ex) {
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(leftNodeValue_, ex.getResult());
}
assert (state_0 & 0b1) != 0 /* is SpecializationActive[SLEqualNode.doLong(long, long)] */;
return doLong(leftNodeValue_, rightNodeValue_);
}
private boolean executeBoolean_boolean_boolean4(int state_0__, VirtualFrame frameValue) {
int state_0 = state_0__;
boolean leftNodeValue_;
try {
leftNodeValue_ = this.leftNode_.executeBoolean(frameValue);
} catch (UnexpectedResultException ex) {
CompilerDirectives.transferToInterpreterAndInvalidate();
Object rightNodeValue = this.rightNode_.executeGeneric(frameValue);
return executeAndSpecialize(ex.getResult(), rightNodeValue);
}
boolean rightNodeValue_;
try {
rightNodeValue_ = this.rightNode_.executeBoolean(frameValue);
} catch (UnexpectedResultException ex) {
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(leftNodeValue_, ex.getResult());
}
assert (state_0 & 0b100) != 0 /* is SpecializationActive[SLEqualNode.doBoolean(boolean, boolean)] */;
return doBoolean(leftNodeValue_, rightNodeValue_);
}
@SuppressWarnings("static-method")
@TruffleBoundary
private boolean generic1Boundary0(int state_0, Object leftNodeValue_, Object rightNodeValue_) {
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(this);
try {
{
InteropLibrary leftInterop__ = (INTEROP_LIBRARY_.getUncached(leftNodeValue_));
InteropLibrary rightInterop__ = (INTEROP_LIBRARY_.getUncached(rightNodeValue_));
return doGeneric(leftNodeValue_, rightNodeValue_, leftInterop__, rightInterop__);
}
} finally {
encapsulating_.set(prev_);
}
}
@ExplodeLoop
private boolean executeBoolean_generic5(int state_0__, VirtualFrame frameValue) {
int state_0 = state_0__;
Object leftNodeValue_ = this.leftNode_.executeGeneric(frameValue);
Object rightNodeValue_ = this.rightNode_.executeGeneric(frameValue);
if ((state_0 & 0b111111111) != 0 /* is SpecializationActive[SLEqualNode.doLong(long, long)] || SpecializationActive[SLEqualNode.doBigNumber(SLBigInteger, SLBigInteger)] || SpecializationActive[SLEqualNode.doBoolean(boolean, boolean)] || SpecializationActive[SLEqualNode.doString(String, String)] || SpecializationActive[SLEqualNode.doTruffleString(TruffleString, TruffleString, EqualNode)] || SpecializationActive[SLEqualNode.doNull(SLNull, SLNull)] || SpecializationActive[SLEqualNode.doFunction(SLFunction, Object)] || SpecializationActive[SLEqualNode.doGeneric(Object, Object, InteropLibrary, InteropLibrary)] || SpecializationActive[SLEqualNode.doGeneric(Object, Object, InteropLibrary, InteropLibrary)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[SLEqualNode.doLong(long, long)] */ && leftNodeValue_ instanceof Long) {
long leftNodeValue__ = (long) leftNodeValue_;
if (rightNodeValue_ instanceof Long) {
long rightNodeValue__ = (long) rightNodeValue_;
return doLong(leftNodeValue__, rightNodeValue__);
}
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[SLEqualNode.doBigNumber(SLBigInteger, SLBigInteger)] */ && SLTypesGen.isImplicitSLBigInteger((state_0 & 0b11000000000) >>> 9 /* get-int ImplicitCast[type=SLBigInteger, index=0] */, leftNodeValue_)) {
SLBigInteger leftNodeValue__ = SLTypesGen.asImplicitSLBigInteger((state_0 & 0b11000000000) >>> 9 /* get-int ImplicitCast[type=SLBigInteger, index=0] */, leftNodeValue_);
if (SLTypesGen.isImplicitSLBigInteger((state_0 & 0b1100000000000) >>> 11 /* get-int ImplicitCast[type=SLBigInteger, index=1] */, rightNodeValue_)) {
SLBigInteger rightNodeValue__ = SLTypesGen.asImplicitSLBigInteger((state_0 & 0b1100000000000) >>> 11 /* get-int ImplicitCast[type=SLBigInteger, index=1] */, rightNodeValue_);
return doBigNumber(leftNodeValue__, rightNodeValue__);
}
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[SLEqualNode.doBoolean(boolean, boolean)] */ && leftNodeValue_ instanceof Boolean) {
boolean leftNodeValue__ = (boolean) leftNodeValue_;
if (rightNodeValue_ instanceof Boolean) {
boolean rightNodeValue__ = (boolean) rightNodeValue_;
return doBoolean(leftNodeValue__, rightNodeValue__);
}
}
if ((state_0 & 0b1000) != 0 /* is SpecializationActive[SLEqualNode.doString(String, String)] */ && leftNodeValue_ instanceof String) {
String leftNodeValue__ = (String) leftNodeValue_;
if (rightNodeValue_ instanceof String) {
String rightNodeValue__ = (String) rightNodeValue_;
return doString(leftNodeValue__, rightNodeValue__);
}
}
if ((state_0 & 0b10000) != 0 /* is SpecializationActive[SLEqualNode.doTruffleString(TruffleString, TruffleString, EqualNode)] */ && leftNodeValue_ instanceof TruffleString) {
TruffleString leftNodeValue__ = (TruffleString) leftNodeValue_;
if (rightNodeValue_ instanceof TruffleString) {
TruffleString rightNodeValue__ = (TruffleString) rightNodeValue_;
{
EqualNode equalNode__ = this.truffleString_equalNode_;
if (equalNode__ != null) {
return doTruffleString(leftNodeValue__, rightNodeValue__, equalNode__);
}
}
}
}
if ((state_0 & 0b100000) != 0 /* is SpecializationActive[SLEqualNode.doNull(SLNull, SLNull)] */ && SLTypes.isSLNull(leftNodeValue_)) {
SLNull leftNodeValue__ = SLTypes.asSLNull(leftNodeValue_);
if (SLTypes.isSLNull(rightNodeValue_)) {
SLNull rightNodeValue__ = SLTypes.asSLNull(rightNodeValue_);
return doNull(leftNodeValue__, rightNodeValue__);
}
}
if ((state_0 & 0b111000000) != 0 /* is SpecializationActive[SLEqualNode.doFunction(SLFunction, Object)] || SpecializationActive[SLEqualNode.doGeneric(Object, Object, InteropLibrary, InteropLibrary)] || SpecializationActive[SLEqualNode.doGeneric(Object, Object, InteropLibrary, InteropLibrary)] */) {
if ((state_0 & 0b1000000) != 0 /* is SpecializationActive[SLEqualNode.doFunction(SLFunction, Object)] */ && leftNodeValue_ instanceof SLFunction) {
SLFunction leftNodeValue__ = (SLFunction) leftNodeValue_;
return doFunction(leftNodeValue__, rightNodeValue_);
}
if ((state_0 & 0b110000000) != 0 /* is SpecializationActive[SLEqualNode.doGeneric(Object, Object, InteropLibrary, InteropLibrary)] || SpecializationActive[SLEqualNode.doGeneric(Object, Object, InteropLibrary, InteropLibrary)] */) {
if ((state_0 & 0b10000000) != 0 /* is SpecializationActive[SLEqualNode.doGeneric(Object, Object, InteropLibrary, InteropLibrary)] */) {
Generic0Data s7_ = this.generic0_cache;
while (s7_ != null) {
if ((s7_.leftInterop_.accepts(leftNodeValue_)) && (s7_.rightInterop_.accepts(rightNodeValue_))) {
return doGeneric(leftNodeValue_, rightNodeValue_, s7_.leftInterop_, s7_.rightInterop_);
}
s7_ = s7_.next_;
}
}
if ((state_0 & 0b100000000) != 0 /* is SpecializationActive[SLEqualNode.doGeneric(Object, Object, InteropLibrary, InteropLibrary)] */) {
return this.generic1Boundary0(state_0, leftNodeValue_, rightNodeValue_);
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(leftNodeValue_, rightNodeValue_);
}
@Override
public void executeVoid(VirtualFrame frameValue) {
executeBoolean(frameValue);
return;
}
private boolean executeAndSpecialize(Object leftNodeValue, Object rightNodeValue) {
int state_0 = this.state_0_;
if (leftNodeValue instanceof Long) {
long leftNodeValue_ = (long) leftNodeValue;
if (rightNodeValue instanceof Long) {
long rightNodeValue_ = (long) rightNodeValue;
state_0 = state_0 | 0b1 /* add SpecializationActive[SLEqualNode.doLong(long, long)] */;
this.state_0_ = state_0;
return doLong(leftNodeValue_, rightNodeValue_);
}
}
{
int sLBigIntegerCast0;
if ((sLBigIntegerCast0 = SLTypesGen.specializeImplicitSLBigInteger(leftNodeValue)) != 0) {
SLBigInteger leftNodeValue_ = SLTypesGen.asImplicitSLBigInteger(sLBigIntegerCast0, leftNodeValue);
int sLBigIntegerCast1;
if ((sLBigIntegerCast1 = SLTypesGen.specializeImplicitSLBigInteger(rightNodeValue)) != 0) {
SLBigInteger rightNodeValue_ = SLTypesGen.asImplicitSLBigInteger(sLBigIntegerCast1, rightNodeValue);
state_0 = (state_0 | (sLBigIntegerCast0 << 9) /* set-int ImplicitCast[type=SLBigInteger, index=0] */);
state_0 = (state_0 | (sLBigIntegerCast1 << 11) /* set-int ImplicitCast[type=SLBigInteger, index=1] */);
state_0 = state_0 | 0b10 /* add SpecializationActive[SLEqualNode.doBigNumber(SLBigInteger, SLBigInteger)] */;
this.state_0_ = state_0;
return doBigNumber(leftNodeValue_, rightNodeValue_);
}
}
}
if (leftNodeValue instanceof Boolean) {
boolean leftNodeValue_ = (boolean) leftNodeValue;
if (rightNodeValue instanceof Boolean) {
boolean rightNodeValue_ = (boolean) rightNodeValue;
state_0 = state_0 | 0b100 /* add SpecializationActive[SLEqualNode.doBoolean(boolean, boolean)] */;
this.state_0_ = state_0;
return doBoolean(leftNodeValue_, rightNodeValue_);
}
}
if (leftNodeValue instanceof String) {
String leftNodeValue_ = (String) leftNodeValue;
if (rightNodeValue instanceof String) {
String rightNodeValue_ = (String) rightNodeValue;
state_0 = state_0 | 0b1000 /* add SpecializationActive[SLEqualNode.doString(String, String)] */;
this.state_0_ = state_0;
return doString(leftNodeValue_, rightNodeValue_);
}
}
if (leftNodeValue instanceof TruffleString) {
TruffleString leftNodeValue_ = (TruffleString) leftNodeValue;
if (rightNodeValue instanceof TruffleString) {
TruffleString rightNodeValue_ = (TruffleString) rightNodeValue;
EqualNode equalNode__ = this.insert((EqualNode.create()));
Objects.requireNonNull(equalNode__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
VarHandle.storeStoreFence();
this.truffleString_equalNode_ = equalNode__;
state_0 = state_0 | 0b10000 /* add SpecializationActive[SLEqualNode.doTruffleString(TruffleString, TruffleString, EqualNode)] */;
this.state_0_ = state_0;
return doTruffleString(leftNodeValue_, rightNodeValue_, equalNode__);
}
}
if (SLTypes.isSLNull(leftNodeValue)) {
SLNull leftNodeValue_ = SLTypes.asSLNull(leftNodeValue);
if (SLTypes.isSLNull(rightNodeValue)) {
SLNull rightNodeValue_ = SLTypes.asSLNull(rightNodeValue);
state_0 = state_0 | 0b100000 /* add SpecializationActive[SLEqualNode.doNull(SLNull, SLNull)] */;
this.state_0_ = state_0;
return doNull(leftNodeValue_, rightNodeValue_);
}
}
if (leftNodeValue instanceof SLFunction) {
SLFunction leftNodeValue_ = (SLFunction) leftNodeValue;
state_0 = state_0 | 0b1000000 /* add SpecializationActive[SLEqualNode.doFunction(SLFunction, Object)] */;
this.state_0_ = state_0;
return doFunction(leftNodeValue_, rightNodeValue);
}
if (((state_0 & 0b100000000)) == 0 /* is-not SpecializationActive[SLEqualNode.doGeneric(Object, Object, InteropLibrary, InteropLibrary)] */) {
while (true) {
int count7_ = 0;
Generic0Data s7_ = GENERIC0_CACHE_UPDATER.getVolatile(this);
Generic0Data s7_original = s7_;
while (s7_ != null) {
if ((s7_.leftInterop_.accepts(leftNodeValue)) && (s7_.rightInterop_.accepts(rightNodeValue))) {
break;
}
count7_++;
s7_ = s7_.next_;
}
if (s7_ == null) {
// assert (s7_.leftInterop_.accepts(leftNodeValue));
// assert (s7_.rightInterop_.accepts(rightNodeValue));
if (count7_ < (4)) {
s7_ = this.insert(new Generic0Data(s7_original));
InteropLibrary leftInterop__ = s7_.insert((INTEROP_LIBRARY_.create(leftNodeValue)));
Objects.requireNonNull(leftInterop__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
s7_.leftInterop_ = leftInterop__;
InteropLibrary rightInterop__ = s7_.insert((INTEROP_LIBRARY_.create(rightNodeValue)));
Objects.requireNonNull(rightInterop__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
s7_.rightInterop_ = rightInterop__;
if (!GENERIC0_CACHE_UPDATER.compareAndSet(this, s7_original, s7_)) {
continue;
}
state_0 = state_0 | 0b10000000 /* add SpecializationActive[SLEqualNode.doGeneric(Object, Object, InteropLibrary, InteropLibrary)] */;
this.state_0_ = state_0;
}
}
if (s7_ != null) {
return doGeneric(leftNodeValue, rightNodeValue, s7_.leftInterop_, s7_.rightInterop_);
}
break;
}
}
{
InteropLibrary rightInterop__ = null;
InteropLibrary leftInterop__ = null;
{
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(this);
try {
leftInterop__ = (INTEROP_LIBRARY_.getUncached(leftNodeValue));
rightInterop__ = (INTEROP_LIBRARY_.getUncached(rightNodeValue));
this.generic0_cache = null;
state_0 = state_0 & 0xffffff7f /* remove SpecializationActive[SLEqualNode.doGeneric(Object, Object, InteropLibrary, InteropLibrary)] */;
state_0 = state_0 | 0b100000000 /* add SpecializationActive[SLEqualNode.doGeneric(Object, Object, InteropLibrary, InteropLibrary)] */;
this.state_0_ = state_0;
return doGeneric(leftNodeValue, rightNodeValue, leftInterop__, rightInterop__);
} finally {
encapsulating_.set(prev_);
}
}
}
}
@NeverDefault
public static SLEqualNode create(SLExpressionNode leftNode, SLExpressionNode rightNode) {
return new SLEqualNodeGen(leftNode, rightNode);
}
@GeneratedBy(SLEqualNode.class)
@DenyReplace
private static final class Generic0Data extends Node implements SpecializationDataNode {
@Child Generic0Data next_;
/**
* Source Info:
* Specialization: {@link SLEqualNode#doGeneric}
* Parameter: {@link InteropLibrary} leftInterop
*/
@Child InteropLibrary leftInterop_;
/**
* Source Info:
* Specialization: {@link SLEqualNode#doGeneric}
* Parameter: {@link InteropLibrary} rightInterop
*/
@Child InteropLibrary rightInterop_;
Generic0Data(Generic0Data next_) {
this.next_ = next_;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy