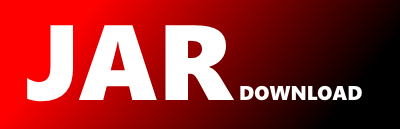
com.oracle.truffle.sl.nodes.expression.SLMulNodeGen Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of truffle-sl Show documentation
Show all versions of truffle-sl Show documentation
Truffle SL is an example language implemented using the Truffle API.
The newest version!
// CheckStyle: start generated
package com.oracle.truffle.sl.nodes.expression;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.CompilerDirectives.TruffleBoundary;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.NeverDefault;
import com.oracle.truffle.api.dsl.DSLSupport.SpecializationDataNode;
import com.oracle.truffle.api.dsl.InlineSupport.ReferenceField;
import com.oracle.truffle.api.dsl.InlineSupport.UnsafeAccessedField;
import com.oracle.truffle.api.frame.VirtualFrame;
import com.oracle.truffle.api.interop.InteropLibrary;
import com.oracle.truffle.api.library.LibraryFactory;
import com.oracle.truffle.api.nodes.DenyReplace;
import com.oracle.truffle.api.nodes.EncapsulatingNodeReference;
import com.oracle.truffle.api.nodes.ExplodeLoop;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.nodes.UnexpectedResultException;
import com.oracle.truffle.sl.nodes.SLExpressionNode;
import com.oracle.truffle.sl.nodes.SLTypesGen;
import com.oracle.truffle.sl.runtime.SLBigInteger;
import java.lang.invoke.MethodHandles;
import java.util.Objects;
/**
* Debug Info:
* Specialization {@link SLMulNode#doLong}
* Activation probability: 0.32000
* With/without class size: 7/0 bytes
* Specialization {@link SLMulNode#doSLBigInteger}
* Activation probability: 0.26000
* With/without class size: 7/0 bytes
* Specialization {@link SLMulNode#doInteropBigInteger}
* Activation probability: 0.20000
* With/without class size: 9/8 bytes
* Specialization {@link SLMulNode#doInteropBigInteger}
* Activation probability: 0.14000
* With/without class size: 5/0 bytes
* Specialization {@link SLMulNode#typeError}
* Activation probability: 0.08000
* With/without class size: 4/0 bytes
*
*/
@GeneratedBy(SLMulNode.class)
@SuppressWarnings({"javadoc", "unused"})
public final class SLMulNodeGen extends SLMulNode {
static final ReferenceField INTEROP_BIG_INTEGER0_CACHE_UPDATER = ReferenceField.create(MethodHandles.lookup(), "interopBigInteger0_cache", InteropBigInteger0Data.class);
private static final LibraryFactory INTEROP_LIBRARY_ = LibraryFactory.resolve(InteropLibrary.class);
@Child private SLExpressionNode leftNode_;
@Child private SLExpressionNode rightNode_;
/**
* State Info:
* 0: SpecializationActive {@link SLMulNode#doLong}
* 1: SpecializationExcluded {@link SLMulNode#doLong}
* 2: SpecializationActive {@link SLMulNode#doSLBigInteger}
* 3: SpecializationActive {@link SLMulNode#doInteropBigInteger}
* 4: SpecializationActive {@link SLMulNode#doInteropBigInteger}
* 5: SpecializationActive {@link SLMulNode#typeError}
* 6-7: ImplicitCast[type=SLBigInteger, index=0]
* 8-9: ImplicitCast[type=SLBigInteger, index=1]
*
*/
@CompilationFinal private int state_0_;
@UnsafeAccessedField @Child private InteropBigInteger0Data interopBigInteger0_cache;
private SLMulNodeGen(SLExpressionNode leftNode, SLExpressionNode rightNode) {
this.leftNode_ = leftNode;
this.rightNode_ = rightNode;
}
@SuppressWarnings("static-method")
private boolean fallbackGuard_(int state_0, Object leftNodeValue, Object rightNodeValue) {
if (!((state_0 & 0b10000) != 0 /* is SpecializationActive[SLMulNode.doInteropBigInteger(Object, Object, InteropLibrary, InteropLibrary)] */) && ((INTEROP_LIBRARY_.getUncached()).fitsInBigInteger(leftNodeValue)) && ((INTEROP_LIBRARY_.getUncached()).fitsInBigInteger(rightNodeValue))) {
return false;
}
return true;
}
@Override
public Object executeGeneric(VirtualFrame frameValue) {
int state_0 = this.state_0_;
if ((state_0 & 0b111100) == 0 /* only-active SpecializationActive[SLMulNode.doLong(long, long)] */ && ((state_0 & 0b111101) != 0 /* is-not SpecializationActive[SLMulNode.doLong(long, long)] && SpecializationActive[SLMulNode.doSLBigInteger(SLBigInteger, SLBigInteger)] && SpecializationActive[SLMulNode.doInteropBigInteger(Object, Object, InteropLibrary, InteropLibrary)] && SpecializationActive[SLMulNode.doInteropBigInteger(Object, Object, InteropLibrary, InteropLibrary)] && SpecializationActive[SLMulNode.typeError(Object, Object)] */)) {
return executeGeneric_long_long0(state_0, frameValue);
} else {
return executeGeneric_generic1(state_0, frameValue);
}
}
private Object executeGeneric_long_long0(int state_0__, VirtualFrame frameValue) {
int state_0 = state_0__;
long leftNodeValue_;
try {
leftNodeValue_ = this.leftNode_.executeLong(frameValue);
} catch (UnexpectedResultException ex) {
CompilerDirectives.transferToInterpreterAndInvalidate();
Object rightNodeValue = this.rightNode_.executeGeneric(frameValue);
return executeAndSpecialize(ex.getResult(), rightNodeValue);
}
long rightNodeValue_;
try {
rightNodeValue_ = this.rightNode_.executeLong(frameValue);
} catch (UnexpectedResultException ex) {
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(leftNodeValue_, ex.getResult());
}
assert (state_0 & 0b1) != 0 /* is SpecializationActive[SLMulNode.doLong(long, long)] */;
try {
return doLong(leftNodeValue_, rightNodeValue_);
} catch (ArithmeticException ex) {
CompilerDirectives.transferToInterpreterAndInvalidate();
state_0 = this.state_0_;
state_0 = state_0 & 0xfffffffe /* remove SpecializationActive[SLMulNode.doLong(long, long)] */;
state_0 = state_0 | 0b10 /* add SpecializationExcluded */;
this.state_0_ = state_0;
return executeAndSpecialize(leftNodeValue_, rightNodeValue_);
}
}
@SuppressWarnings("static-method")
@TruffleBoundary
private Object interopBigInteger1Boundary(int state_0, Object leftNodeValue_, Object rightNodeValue_) {
{
InteropLibrary leftLibrary__ = (INTEROP_LIBRARY_.getUncached());
InteropLibrary rightLibrary__ = (INTEROP_LIBRARY_.getUncached());
return doInteropBigInteger(leftNodeValue_, rightNodeValue_, leftLibrary__, rightLibrary__);
}
}
@ExplodeLoop
private Object executeGeneric_generic1(int state_0__, VirtualFrame frameValue) {
int state_0 = state_0__;
Object leftNodeValue_ = this.leftNode_.executeGeneric(frameValue);
Object rightNodeValue_ = this.rightNode_.executeGeneric(frameValue);
if ((state_0 & 0b111101) != 0 /* is SpecializationActive[SLMulNode.doLong(long, long)] || SpecializationActive[SLMulNode.doSLBigInteger(SLBigInteger, SLBigInteger)] || SpecializationActive[SLMulNode.doInteropBigInteger(Object, Object, InteropLibrary, InteropLibrary)] || SpecializationActive[SLMulNode.doInteropBigInteger(Object, Object, InteropLibrary, InteropLibrary)] || SpecializationActive[SLMulNode.typeError(Object, Object)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[SLMulNode.doLong(long, long)] */ && leftNodeValue_ instanceof Long) {
long leftNodeValue__ = (long) leftNodeValue_;
if (rightNodeValue_ instanceof Long) {
long rightNodeValue__ = (long) rightNodeValue_;
try {
return doLong(leftNodeValue__, rightNodeValue__);
} catch (ArithmeticException ex) {
CompilerDirectives.transferToInterpreterAndInvalidate();
state_0 = this.state_0_;
state_0 = state_0 & 0xfffffffe /* remove SpecializationActive[SLMulNode.doLong(long, long)] */;
state_0 = state_0 | 0b10 /* add SpecializationExcluded */;
this.state_0_ = state_0;
return executeAndSpecialize(leftNodeValue__, rightNodeValue__);
}
}
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[SLMulNode.doSLBigInteger(SLBigInteger, SLBigInteger)] */ && SLTypesGen.isImplicitSLBigInteger((state_0 & 0b11000000) >>> 6 /* get-int ImplicitCast[type=SLBigInteger, index=0] */, leftNodeValue_)) {
SLBigInteger leftNodeValue__ = SLTypesGen.asImplicitSLBigInteger((state_0 & 0b11000000) >>> 6 /* get-int ImplicitCast[type=SLBigInteger, index=0] */, leftNodeValue_);
if (SLTypesGen.isImplicitSLBigInteger((state_0 & 0b1100000000) >>> 8 /* get-int ImplicitCast[type=SLBigInteger, index=1] */, rightNodeValue_)) {
SLBigInteger rightNodeValue__ = SLTypesGen.asImplicitSLBigInteger((state_0 & 0b1100000000) >>> 8 /* get-int ImplicitCast[type=SLBigInteger, index=1] */, rightNodeValue_);
return doSLBigInteger(leftNodeValue__, rightNodeValue__);
}
}
if ((state_0 & 0b111000) != 0 /* is SpecializationActive[SLMulNode.doInteropBigInteger(Object, Object, InteropLibrary, InteropLibrary)] || SpecializationActive[SLMulNode.doInteropBigInteger(Object, Object, InteropLibrary, InteropLibrary)] || SpecializationActive[SLMulNode.typeError(Object, Object)] */) {
if ((state_0 & 0b1000) != 0 /* is SpecializationActive[SLMulNode.doInteropBigInteger(Object, Object, InteropLibrary, InteropLibrary)] */) {
InteropBigInteger0Data s2_ = this.interopBigInteger0_cache;
while (s2_ != null) {
if ((s2_.leftLibrary_.accepts(leftNodeValue_)) && (s2_.rightLibrary_.accepts(rightNodeValue_)) && (s2_.leftLibrary_.fitsInBigInteger(leftNodeValue_)) && (s2_.rightLibrary_.fitsInBigInteger(rightNodeValue_))) {
return doInteropBigInteger(leftNodeValue_, rightNodeValue_, s2_.leftLibrary_, s2_.rightLibrary_);
}
s2_ = s2_.next_;
}
}
if ((state_0 & 0b10000) != 0 /* is SpecializationActive[SLMulNode.doInteropBigInteger(Object, Object, InteropLibrary, InteropLibrary)] */) {
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(this);
try {
{
InteropLibrary leftLibrary__ = (INTEROP_LIBRARY_.getUncached());
if ((leftLibrary__.fitsInBigInteger(leftNodeValue_))) {
InteropLibrary rightLibrary__ = (INTEROP_LIBRARY_.getUncached());
if ((rightLibrary__.fitsInBigInteger(rightNodeValue_))) {
return this.interopBigInteger1Boundary(state_0, leftNodeValue_, rightNodeValue_);
}
}
}
} finally {
encapsulating_.set(prev_);
}
}
if ((state_0 & 0b100000) != 0 /* is SpecializationActive[SLMulNode.typeError(Object, Object)] */) {
if (fallbackGuard_(state_0, leftNodeValue_, rightNodeValue_)) {
return typeError(leftNodeValue_, rightNodeValue_);
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(leftNodeValue_, rightNodeValue_);
}
@Override
public long executeLong(VirtualFrame frameValue) throws UnexpectedResultException {
int state_0 = this.state_0_;
if ((state_0 & 0b100000) != 0 /* is SpecializationActive[SLMulNode.typeError(Object, Object)] */) {
return SLTypesGen.expectLong(executeGeneric(frameValue));
}
long leftNodeValue_;
try {
leftNodeValue_ = this.leftNode_.executeLong(frameValue);
} catch (UnexpectedResultException ex) {
CompilerDirectives.transferToInterpreterAndInvalidate();
Object rightNodeValue = this.rightNode_.executeGeneric(frameValue);
return SLTypesGen.expectLong(executeAndSpecialize(ex.getResult(), rightNodeValue));
}
long rightNodeValue_;
try {
rightNodeValue_ = this.rightNode_.executeLong(frameValue);
} catch (UnexpectedResultException ex) {
CompilerDirectives.transferToInterpreterAndInvalidate();
return SLTypesGen.expectLong(executeAndSpecialize(leftNodeValue_, ex.getResult()));
}
if ((state_0 & 0b1) != 0 /* is SpecializationActive[SLMulNode.doLong(long, long)] */) {
try {
return doLong(leftNodeValue_, rightNodeValue_);
} catch (ArithmeticException ex) {
CompilerDirectives.transferToInterpreterAndInvalidate();
state_0 = this.state_0_;
state_0 = state_0 & 0xfffffffe /* remove SpecializationActive[SLMulNode.doLong(long, long)] */;
state_0 = state_0 | 0b10 /* add SpecializationExcluded */;
this.state_0_ = state_0;
return SLTypesGen.expectLong(executeAndSpecialize(leftNodeValue_, rightNodeValue_));
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return SLTypesGen.expectLong(executeAndSpecialize(leftNodeValue_, rightNodeValue_));
}
@Override
public void executeVoid(VirtualFrame frameValue) {
int state_0 = this.state_0_;
try {
if ((state_0 & 0b111100) == 0 /* only-active SpecializationActive[SLMulNode.doLong(long, long)] */ && ((state_0 & 0b111101) != 0 /* is-not SpecializationActive[SLMulNode.doLong(long, long)] && SpecializationActive[SLMulNode.doSLBigInteger(SLBigInteger, SLBigInteger)] && SpecializationActive[SLMulNode.doInteropBigInteger(Object, Object, InteropLibrary, InteropLibrary)] && SpecializationActive[SLMulNode.doInteropBigInteger(Object, Object, InteropLibrary, InteropLibrary)] && SpecializationActive[SLMulNode.typeError(Object, Object)] */)) {
executeLong(frameValue);
return;
}
executeGeneric(frameValue);
return;
} catch (UnexpectedResultException ex) {
CompilerDirectives.transferToInterpreterAndInvalidate();
return;
}
}
@SuppressWarnings("unused")
private Object executeAndSpecialize(Object leftNodeValue, Object rightNodeValue) {
int state_0 = this.state_0_;
if (((state_0 & 0b10)) == 0 /* is-not SpecializationExcluded */ && leftNodeValue instanceof Long) {
long leftNodeValue_ = (long) leftNodeValue;
if (rightNodeValue instanceof Long) {
long rightNodeValue_ = (long) rightNodeValue;
state_0 = state_0 | 0b1 /* add SpecializationActive[SLMulNode.doLong(long, long)] */;
this.state_0_ = state_0;
try {
return doLong(leftNodeValue_, rightNodeValue_);
} catch (ArithmeticException ex) {
CompilerDirectives.transferToInterpreterAndInvalidate();
state_0 = this.state_0_;
state_0 = state_0 & 0xfffffffe /* remove SpecializationActive[SLMulNode.doLong(long, long)] */;
state_0 = state_0 | 0b10 /* add SpecializationExcluded */;
this.state_0_ = state_0;
return executeAndSpecialize(leftNodeValue_, rightNodeValue_);
}
}
}
if (((state_0 & 0b11000)) == 0 /* is-not SpecializationActive[SLMulNode.doInteropBigInteger(Object, Object, InteropLibrary, InteropLibrary)] && SpecializationActive[SLMulNode.doInteropBigInteger(Object, Object, InteropLibrary, InteropLibrary)] */) {
int sLBigIntegerCast0;
if ((sLBigIntegerCast0 = SLTypesGen.specializeImplicitSLBigInteger(leftNodeValue)) != 0) {
SLBigInteger leftNodeValue_ = SLTypesGen.asImplicitSLBigInteger(sLBigIntegerCast0, leftNodeValue);
int sLBigIntegerCast1;
if ((sLBigIntegerCast1 = SLTypesGen.specializeImplicitSLBigInteger(rightNodeValue)) != 0) {
SLBigInteger rightNodeValue_ = SLTypesGen.asImplicitSLBigInteger(sLBigIntegerCast1, rightNodeValue);
state_0 = (state_0 | (sLBigIntegerCast0 << 6) /* set-int ImplicitCast[type=SLBigInteger, index=0] */);
state_0 = (state_0 | (sLBigIntegerCast1 << 8) /* set-int ImplicitCast[type=SLBigInteger, index=1] */);
state_0 = state_0 | 0b100 /* add SpecializationActive[SLMulNode.doSLBigInteger(SLBigInteger, SLBigInteger)] */;
this.state_0_ = state_0;
return doSLBigInteger(leftNodeValue_, rightNodeValue_);
}
}
}
if (((state_0 & 0b10000)) == 0 /* is-not SpecializationActive[SLMulNode.doInteropBigInteger(Object, Object, InteropLibrary, InteropLibrary)] */) {
while (true) {
int count2_ = 0;
InteropBigInteger0Data s2_ = INTEROP_BIG_INTEGER0_CACHE_UPDATER.getVolatile(this);
InteropBigInteger0Data s2_original = s2_;
while (s2_ != null) {
if ((s2_.leftLibrary_.accepts(leftNodeValue)) && (s2_.rightLibrary_.accepts(rightNodeValue)) && (s2_.leftLibrary_.fitsInBigInteger(leftNodeValue)) && (s2_.rightLibrary_.fitsInBigInteger(rightNodeValue))) {
break;
}
count2_++;
s2_ = s2_.next_;
}
if (s2_ == null) {
{
InteropLibrary leftLibrary__ = this.insert((INTEROP_LIBRARY_.create(leftNodeValue)));
// assert (s2_.leftLibrary_.accepts(leftNodeValue));
// assert (s2_.rightLibrary_.accepts(rightNodeValue));
if ((leftLibrary__.fitsInBigInteger(leftNodeValue))) {
InteropLibrary rightLibrary__ = this.insert((INTEROP_LIBRARY_.create(rightNodeValue)));
if ((rightLibrary__.fitsInBigInteger(rightNodeValue)) && count2_ < (3)) {
s2_ = this.insert(new InteropBigInteger0Data(s2_original));
Objects.requireNonNull(s2_.insert(leftLibrary__), "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
s2_.leftLibrary_ = leftLibrary__;
Objects.requireNonNull(s2_.insert(rightLibrary__), "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
s2_.rightLibrary_ = rightLibrary__;
if (!INTEROP_BIG_INTEGER0_CACHE_UPDATER.compareAndSet(this, s2_original, s2_)) {
continue;
}
state_0 = state_0 & 0xfffffffb /* remove SpecializationActive[SLMulNode.doSLBigInteger(SLBigInteger, SLBigInteger)] */;
state_0 = state_0 | 0b1000 /* add SpecializationActive[SLMulNode.doInteropBigInteger(Object, Object, InteropLibrary, InteropLibrary)] */;
this.state_0_ = state_0;
}
}
}
}
if (s2_ != null) {
return doInteropBigInteger(leftNodeValue, rightNodeValue, s2_.leftLibrary_, s2_.rightLibrary_);
}
break;
}
}
{
InteropLibrary rightLibrary__ = null;
InteropLibrary leftLibrary__ = null;
{
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(this);
try {
{
leftLibrary__ = (INTEROP_LIBRARY_.getUncached());
if ((leftLibrary__.fitsInBigInteger(leftNodeValue))) {
rightLibrary__ = (INTEROP_LIBRARY_.getUncached());
if ((rightLibrary__.fitsInBigInteger(rightNodeValue))) {
this.interopBigInteger0_cache = null;
state_0 = state_0 & 0xfffffff3 /* remove SpecializationActive[SLMulNode.doSLBigInteger(SLBigInteger, SLBigInteger)], SpecializationActive[SLMulNode.doInteropBigInteger(Object, Object, InteropLibrary, InteropLibrary)] */;
state_0 = state_0 | 0b10000 /* add SpecializationActive[SLMulNode.doInteropBigInteger(Object, Object, InteropLibrary, InteropLibrary)] */;
this.state_0_ = state_0;
return doInteropBigInteger(leftNodeValue, rightNodeValue, leftLibrary__, rightLibrary__);
}
}
}
} finally {
encapsulating_.set(prev_);
}
}
}
state_0 = state_0 | 0b100000 /* add SpecializationActive[SLMulNode.typeError(Object, Object)] */;
this.state_0_ = state_0;
return typeError(leftNodeValue, rightNodeValue);
}
@NeverDefault
public static SLMulNode create(SLExpressionNode leftNode, SLExpressionNode rightNode) {
return new SLMulNodeGen(leftNode, rightNode);
}
@GeneratedBy(SLMulNode.class)
@DenyReplace
private static final class InteropBigInteger0Data extends Node implements SpecializationDataNode {
@Child InteropBigInteger0Data next_;
/**
* Source Info:
* Specialization: {@link SLMulNode#doInteropBigInteger}
* Parameter: {@link InteropLibrary} leftLibrary
*/
@Child InteropLibrary leftLibrary_;
/**
* Source Info:
* Specialization: {@link SLMulNode#doInteropBigInteger}
* Parameter: {@link InteropLibrary} rightLibrary
*/
@Child InteropLibrary rightLibrary_;
InteropBigInteger0Data(InteropBigInteger0Data next_) {
this.next_ = next_;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy