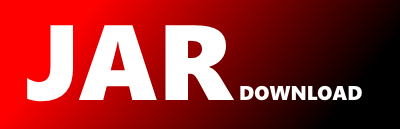
com.oracle.truffle.sl.nodes.local.SLScopedNodeGen Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of truffle-sl Show documentation
Show all versions of truffle-sl Show documentation
Truffle SL is an example language implemented using the Truffle API.
The newest version!
// CheckStyle: start generated
package com.oracle.truffle.sl.nodes.local;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.CompilerDirectives.TruffleBoundary;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.frame.Frame;
import com.oracle.truffle.api.interop.NodeLibrary;
import com.oracle.truffle.api.interop.UnsupportedMessageException;
import com.oracle.truffle.api.library.DynamicDispatchLibrary;
import com.oracle.truffle.api.library.LibraryExport;
import com.oracle.truffle.api.library.LibraryFactory;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.nodes.UnadoptableNode;
import java.lang.invoke.VarHandle;
import java.util.Objects;
@GeneratedBy(SLScopedNode.class)
@SuppressWarnings("javadoc")
public final class SLScopedNodeGen {
private static final LibraryFactory DYNAMIC_DISPATCH_LIBRARY_ = LibraryFactory.resolve(DynamicDispatchLibrary.class);
static {
LibraryExport.register(SLScopedNode.class, new NodeLibraryExports());
}
private SLScopedNodeGen() {
}
@GeneratedBy(SLScopedNode.class)
public static class NodeLibraryExports extends LibraryExport {
private NodeLibraryExports() {
super(NodeLibrary.class, SLScopedNode.class, false, false, 0);
}
@Override
protected NodeLibrary createUncached(Object receiver) {
assert receiver instanceof SLScopedNode;
NodeLibrary uncached = new Uncached(receiver);
return uncached;
}
@Override
protected NodeLibrary createCached(Object receiver) {
assert receiver instanceof SLScopedNode;
return new Cached(receiver);
}
@GeneratedBy(SLScopedNode.class)
public static class Cached extends NodeLibrary {
private final Class extends SLScopedNode> receiverClass_;
/**
* State Info:
* 0: SpecializationActive {@link SLScopedNode#getScope(SLScopedNode, Frame, boolean, SLScopedNode, Node)}
*
*/
@CompilationFinal private int state_0_;
/**
* Source Info:
* Specialization: {@link SLScopedNode#accepts}
* Parameter: {@link SLScopedNode} cachedNode
*/
@CompilationFinal private SLScopedNode node;
/**
* Source Info:
* Specialization: {@link SLScopedNode#getScope(SLScopedNode, Frame, boolean, SLScopedNode, Node)}
* Parameter: {@link Node} blockNode
*/
@CompilationFinal private Node getScopeNode__getScope_blockNode_;
protected Cached(Object receiver) {
SLScopedNode castReceiver = ((SLScopedNode) receiver) ;
SLScopedNode node_ = (castReceiver);
VarHandle.storeStoreFence();
this.node = node_;
this.receiverClass_ = castReceiver.getClass();
}
@Override
public boolean accepts(Object receiver) {
assert receiver.getClass() != this.receiverClass_ || DYNAMIC_DISPATCH_LIBRARY_.getUncached().dispatch(receiver) == null : "Invalid library export. Exported receiver with dynamic dispatch found but not expected.";
return CompilerDirectives.isExact(receiver, this.receiverClass_) && accepts_(receiver);
}
/**
* Debug Info:
* Specialization {@link SLScopedNode#accepts(SLScopedNode, SLScopedNode)}
* Activation probability: 0.50000
* With/without class size: 10/0 bytes
*
*/
private boolean accepts_(Object arg0Value_) {
SLScopedNode arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
return arg0Value.accepts(this.node);
}
@Override
public boolean hasScope(Object receiver, Frame frame) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
return (CompilerDirectives.castExact(receiver, receiverClass_)).hasScope(frame);
}
/**
* Debug Info:
* Specialization {@link SLScopedNode#getScope(SLScopedNode, Frame, boolean, SLScopedNode, Node)}
* Activation probability: 0.50000
* With/without class size: 14/4 bytes
*
*/
@Override
public Object getScope(Object arg0Value_, Frame arg1Value, boolean arg2Value) throws UnsupportedMessageException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
SLScopedNode arg0Value = CompilerDirectives.castExact(arg0Value_, receiverClass_);
int state_0 = this.state_0_;
if (state_0 != 0 /* is SpecializationActive[SLScopedNode.getScope(SLScopedNode, Frame, boolean, SLScopedNode, Node)] */) {
{
Node blockNode__ = this.getScopeNode__getScope_blockNode_;
if (blockNode__ != null) {
return arg0Value.getScope(arg1Value, arg2Value, this.node, blockNode__);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return getScopeNode_AndSpecialize(arg0Value, arg1Value, arg2Value);
}
private Object getScopeNode_AndSpecialize(SLScopedNode arg0Value, Frame arg1Value, boolean arg2Value) {
int state_0 = this.state_0_;
Node blockNode__ = (arg0Value.findBlock());
Objects.requireNonNull(blockNode__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
VarHandle.storeStoreFence();
this.getScopeNode__getScope_blockNode_ = blockNode__;
state_0 = state_0 | 0b1 /* add SpecializationActive[SLScopedNode.getScope(SLScopedNode, Frame, boolean, SLScopedNode, Node)] */;
this.state_0_ = state_0;
return arg0Value.getScope(arg1Value, arg2Value, this.node, blockNode__);
}
@Override
public boolean hasRootInstance(Object receiver, Frame frame) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
return (CompilerDirectives.castExact(receiver, receiverClass_)).hasRootInstance(frame);
}
@Override
public Object getRootInstance(Object receiver, Frame frame) throws UnsupportedMessageException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted(this);
return (CompilerDirectives.castExact(receiver, receiverClass_)).getRootInstance(frame);
}
}
@GeneratedBy(SLScopedNode.class)
public static class Uncached extends NodeLibrary implements UnadoptableNode {
private final Class extends SLScopedNode> receiverClass_;
protected Uncached(Object receiver) {
this.receiverClass_ = ((SLScopedNode) receiver).getClass();
}
@Override
@TruffleBoundary
public boolean accepts(Object receiver) {
assert receiver.getClass() != this.receiverClass_ || DYNAMIC_DISPATCH_LIBRARY_.getUncached().dispatch(receiver) == null : "Invalid library export. Exported receiver with dynamic dispatch found but not expected.";
return CompilerDirectives.isExact(receiver, this.receiverClass_) && accepts_(receiver);
}
@Override
public boolean hasScope(Object receiver, Frame frame) {
// declared: true
CompilerDirectives.transferToInterpreterAndInvalidate();
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((SLScopedNode) receiver) .hasScope(frame);
}
@Override
public Object getScope(Object arg0Value_, Frame arg1Value, boolean arg2Value) throws UnsupportedMessageException {
// declared: true
CompilerDirectives.transferToInterpreterAndInvalidate();
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
SLScopedNode arg0Value = ((SLScopedNode) arg0Value_);
CompilerDirectives.transferToInterpreterAndInvalidate();
return arg0Value.getScope(arg1Value, arg2Value, (arg0Value), (arg0Value.findBlock()));
}
@Override
public boolean hasRootInstance(Object receiver, Frame frame) {
// declared: true
CompilerDirectives.transferToInterpreterAndInvalidate();
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((SLScopedNode) receiver) .hasRootInstance(frame);
}
@Override
public Object getRootInstance(Object receiver, Frame frame) throws UnsupportedMessageException {
// declared: true
CompilerDirectives.transferToInterpreterAndInvalidate();
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((SLScopedNode) receiver) .getRootInstance(frame);
}
@TruffleBoundary
private static boolean accepts_(Object arg0Value_) {
SLScopedNode arg0Value = ((SLScopedNode) arg0Value_);
return arg0Value.accepts((arg0Value));
}
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy