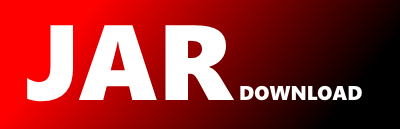
com.oracle.truffle.sl.nodes.util.SLUnboxNodeGen Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of truffle-sl Show documentation
Show all versions of truffle-sl Show documentation
Truffle SL is an example language implemented using the Truffle API.
The newest version!
// CheckStyle: start generated
package com.oracle.truffle.sl.nodes.util;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.CompilerDirectives.TruffleBoundary;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.NeverDefault;
import com.oracle.truffle.api.dsl.DSLSupport.SpecializationDataNode;
import com.oracle.truffle.api.dsl.InlineSupport.ReferenceField;
import com.oracle.truffle.api.dsl.InlineSupport.UnsafeAccessedField;
import com.oracle.truffle.api.frame.VirtualFrame;
import com.oracle.truffle.api.interop.InteropLibrary;
import com.oracle.truffle.api.library.LibraryFactory;
import com.oracle.truffle.api.nodes.DenyReplace;
import com.oracle.truffle.api.nodes.EncapsulatingNodeReference;
import com.oracle.truffle.api.nodes.ExplodeLoop;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.nodes.UnexpectedResultException;
import com.oracle.truffle.api.strings.TruffleString;
import com.oracle.truffle.api.strings.TruffleString.FromJavaStringNode;
import com.oracle.truffle.sl.nodes.SLExpressionNode;
import com.oracle.truffle.sl.nodes.SLTypes;
import com.oracle.truffle.sl.nodes.SLTypesGen;
import com.oracle.truffle.sl.runtime.SLBigInteger;
import com.oracle.truffle.sl.runtime.SLFunction;
import com.oracle.truffle.sl.runtime.SLNull;
import java.lang.invoke.MethodHandles;
import java.lang.invoke.VarHandle;
import java.util.Objects;
/**
* Debug Info:
* Specialization {@link SLUnboxNode#fromString}
* Activation probability: 0.19111
* With/without class size: 7/4 bytes
* Specialization {@link SLUnboxNode#fromTruffleString}
* Activation probability: 0.17111
* With/without class size: 6/0 bytes
* Specialization {@link SLUnboxNode#fromBoolean}
* Activation probability: 0.15111
* With/without class size: 5/0 bytes
* Specialization {@link SLUnboxNode#fromLong}
* Activation probability: 0.13111
* With/without class size: 5/0 bytes
* Specialization {@link SLUnboxNode#fromBigNumber}
* Activation probability: 0.11111
* With/without class size: 5/0 bytes
* Specialization {@link SLUnboxNode#fromFunction(SLFunction)}
* Activation probability: 0.09111
* With/without class size: 5/0 bytes
* Specialization {@link SLUnboxNode#fromFunction(SLNull)}
* Activation probability: 0.07111
* With/without class size: 4/0 bytes
* Specialization {@link SLUnboxNode#fromForeign}
* Activation probability: 0.05111
* With/without class size: 5/4 bytes
* Specialization {@link SLUnboxNode#fromForeign}
* Activation probability: 0.03111
* With/without class size: 4/0 bytes
*
*/
@GeneratedBy(SLUnboxNode.class)
@SuppressWarnings({"javadoc", "unused"})
public final class SLUnboxNodeGen extends SLUnboxNode {
static final ReferenceField FROM_FOREIGN0_CACHE_UPDATER = ReferenceField.create(MethodHandles.lookup(), "fromForeign0_cache", FromForeign0Data.class);
private static final LibraryFactory INTEROP_LIBRARY_ = LibraryFactory.resolve(InteropLibrary.class);
@Child private SLExpressionNode child0_;
/**
* State Info:
* 0: SpecializationActive {@link SLUnboxNode#fromString}
* 1: SpecializationActive {@link SLUnboxNode#fromTruffleString}
* 2: SpecializationActive {@link SLUnboxNode#fromBoolean}
* 3: SpecializationActive {@link SLUnboxNode#fromLong}
* 4: SpecializationActive {@link SLUnboxNode#fromBigNumber}
* 5: SpecializationActive {@link SLUnboxNode#fromFunction(SLFunction)}
* 6: SpecializationActive {@link SLUnboxNode#fromFunction(SLNull)}
* 7: SpecializationActive {@link SLUnboxNode#fromForeign}
* 8: SpecializationActive {@link SLUnboxNode#fromForeign}
* 9-10: ImplicitCast[type=SLBigInteger, index=0]
*
*/
@CompilationFinal private int state_0_;
/**
* Source Info:
* Specialization: {@link SLUnboxNode#fromString}
* Parameter: {@link FromJavaStringNode} fromJavaStringNode
*/
@Child private FromJavaStringNode fromString_fromJavaStringNode_;
@UnsafeAccessedField @Child private FromForeign0Data fromForeign0_cache;
private SLUnboxNodeGen(SLExpressionNode child0) {
this.child0_ = child0;
}
@Override
public Object executeGeneric(VirtualFrame frameValue) {
int state_0 = this.state_0_;
if ((state_0 & 0b111111011) == 0 /* only-active SpecializationActive[SLUnboxNode.fromBoolean(boolean)] */ && ((state_0 & 0b111111111) != 0 /* is-not SpecializationActive[SLUnboxNode.fromString(String, FromJavaStringNode)] && SpecializationActive[SLUnboxNode.fromTruffleString(TruffleString)] && SpecializationActive[SLUnboxNode.fromBoolean(boolean)] && SpecializationActive[SLUnboxNode.fromLong(long)] && SpecializationActive[SLUnboxNode.fromBigNumber(SLBigInteger)] && SpecializationActive[SLUnboxNode.fromFunction(SLFunction)] && SpecializationActive[SLUnboxNode.fromFunction(SLNull)] && SpecializationActive[SLUnboxNode.fromForeign(Object, InteropLibrary)] && SpecializationActive[SLUnboxNode.fromForeign(Object, InteropLibrary)] */)) {
return executeGeneric_boolean0(state_0, frameValue);
} else if ((state_0 & 0b111110111) == 0 /* only-active SpecializationActive[SLUnboxNode.fromLong(long)] */ && ((state_0 & 0b111111111) != 0 /* is-not SpecializationActive[SLUnboxNode.fromString(String, FromJavaStringNode)] && SpecializationActive[SLUnboxNode.fromTruffleString(TruffleString)] && SpecializationActive[SLUnboxNode.fromBoolean(boolean)] && SpecializationActive[SLUnboxNode.fromLong(long)] && SpecializationActive[SLUnboxNode.fromBigNumber(SLBigInteger)] && SpecializationActive[SLUnboxNode.fromFunction(SLFunction)] && SpecializationActive[SLUnboxNode.fromFunction(SLNull)] && SpecializationActive[SLUnboxNode.fromForeign(Object, InteropLibrary)] && SpecializationActive[SLUnboxNode.fromForeign(Object, InteropLibrary)] */)) {
return executeGeneric_long1(state_0, frameValue);
} else {
return executeGeneric_generic2(state_0, frameValue);
}
}
private Object executeGeneric_boolean0(int state_0__, VirtualFrame frameValue) {
int state_0 = state_0__;
boolean child0Value_;
try {
child0Value_ = this.child0_.executeBoolean(frameValue);
} catch (UnexpectedResultException ex) {
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(ex.getResult());
}
assert (state_0 & 0b100) != 0 /* is SpecializationActive[SLUnboxNode.fromBoolean(boolean)] */;
return SLUnboxNode.fromBoolean(child0Value_);
}
private Object executeGeneric_long1(int state_0__, VirtualFrame frameValue) {
int state_0 = state_0__;
long child0Value_;
try {
child0Value_ = this.child0_.executeLong(frameValue);
} catch (UnexpectedResultException ex) {
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(ex.getResult());
}
assert (state_0 & 0b1000) != 0 /* is SpecializationActive[SLUnboxNode.fromLong(long)] */;
return SLUnboxNode.fromLong(child0Value_);
}
@SuppressWarnings("static-method")
@TruffleBoundary
private Object fromForeign1Boundary(int state_0, Object child0Value_) {
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(this);
try {
{
InteropLibrary interop__ = (INTEROP_LIBRARY_.getUncached(child0Value_));
return SLUnboxNode.fromForeign(child0Value_, interop__);
}
} finally {
encapsulating_.set(prev_);
}
}
@ExplodeLoop
private Object executeGeneric_generic2(int state_0__, VirtualFrame frameValue) {
int state_0 = state_0__;
Object child0Value_ = this.child0_.executeGeneric(frameValue);
if ((state_0 & 0b111111111) != 0 /* is SpecializationActive[SLUnboxNode.fromString(String, FromJavaStringNode)] || SpecializationActive[SLUnboxNode.fromTruffleString(TruffleString)] || SpecializationActive[SLUnboxNode.fromBoolean(boolean)] || SpecializationActive[SLUnboxNode.fromLong(long)] || SpecializationActive[SLUnboxNode.fromBigNumber(SLBigInteger)] || SpecializationActive[SLUnboxNode.fromFunction(SLFunction)] || SpecializationActive[SLUnboxNode.fromFunction(SLNull)] || SpecializationActive[SLUnboxNode.fromForeign(Object, InteropLibrary)] || SpecializationActive[SLUnboxNode.fromForeign(Object, InteropLibrary)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[SLUnboxNode.fromString(String, FromJavaStringNode)] */ && child0Value_ instanceof String) {
String child0Value__ = (String) child0Value_;
{
FromJavaStringNode fromJavaStringNode__ = this.fromString_fromJavaStringNode_;
if (fromJavaStringNode__ != null) {
return SLUnboxNode.fromString(child0Value__, fromJavaStringNode__);
}
}
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[SLUnboxNode.fromTruffleString(TruffleString)] */ && child0Value_ instanceof TruffleString) {
TruffleString child0Value__ = (TruffleString) child0Value_;
return SLUnboxNode.fromTruffleString(child0Value__);
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[SLUnboxNode.fromBoolean(boolean)] */ && child0Value_ instanceof Boolean) {
boolean child0Value__ = (boolean) child0Value_;
return SLUnboxNode.fromBoolean(child0Value__);
}
if ((state_0 & 0b1000) != 0 /* is SpecializationActive[SLUnboxNode.fromLong(long)] */ && child0Value_ instanceof Long) {
long child0Value__ = (long) child0Value_;
return SLUnboxNode.fromLong(child0Value__);
}
if ((state_0 & 0b10000) != 0 /* is SpecializationActive[SLUnboxNode.fromBigNumber(SLBigInteger)] */ && SLTypesGen.isImplicitSLBigInteger((state_0 & 0b11000000000) >>> 9 /* get-int ImplicitCast[type=SLBigInteger, index=0] */, child0Value_)) {
SLBigInteger child0Value__ = SLTypesGen.asImplicitSLBigInteger((state_0 & 0b11000000000) >>> 9 /* get-int ImplicitCast[type=SLBigInteger, index=0] */, child0Value_);
return SLUnboxNode.fromBigNumber(child0Value__);
}
if ((state_0 & 0b100000) != 0 /* is SpecializationActive[SLUnboxNode.fromFunction(SLFunction)] */ && child0Value_ instanceof SLFunction) {
SLFunction child0Value__ = (SLFunction) child0Value_;
return SLUnboxNode.fromFunction(child0Value__);
}
if ((state_0 & 0b1000000) != 0 /* is SpecializationActive[SLUnboxNode.fromFunction(SLNull)] */ && SLTypes.isSLNull(child0Value_)) {
SLNull child0Value__ = SLTypes.asSLNull(child0Value_);
return SLUnboxNode.fromFunction(child0Value__);
}
if ((state_0 & 0b110000000) != 0 /* is SpecializationActive[SLUnboxNode.fromForeign(Object, InteropLibrary)] || SpecializationActive[SLUnboxNode.fromForeign(Object, InteropLibrary)] */) {
if ((state_0 & 0b10000000) != 0 /* is SpecializationActive[SLUnboxNode.fromForeign(Object, InteropLibrary)] */) {
FromForeign0Data s7_ = this.fromForeign0_cache;
while (s7_ != null) {
if ((s7_.interop_.accepts(child0Value_))) {
return SLUnboxNode.fromForeign(child0Value_, s7_.interop_);
}
s7_ = s7_.next_;
}
}
if ((state_0 & 0b100000000) != 0 /* is SpecializationActive[SLUnboxNode.fromForeign(Object, InteropLibrary)] */) {
return this.fromForeign1Boundary(state_0, child0Value_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(child0Value_);
}
@Override
public boolean executeBoolean(VirtualFrame frameValue) throws UnexpectedResultException {
int state_0 = this.state_0_;
if ((state_0 & 0b110000000) != 0 /* is SpecializationActive[SLUnboxNode.fromForeign(Object, InteropLibrary)] || SpecializationActive[SLUnboxNode.fromForeign(Object, InteropLibrary)] */) {
return SLTypesGen.expectBoolean(executeGeneric(frameValue));
}
boolean child0Value_;
try {
child0Value_ = this.child0_.executeBoolean(frameValue);
} catch (UnexpectedResultException ex) {
CompilerDirectives.transferToInterpreterAndInvalidate();
return SLTypesGen.expectBoolean(executeAndSpecialize(ex.getResult()));
}
if ((state_0 & 0b100) != 0 /* is SpecializationActive[SLUnboxNode.fromBoolean(boolean)] */) {
return SLUnboxNode.fromBoolean(child0Value_);
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return SLTypesGen.expectBoolean(executeAndSpecialize(child0Value_));
}
@Override
public long executeLong(VirtualFrame frameValue) throws UnexpectedResultException {
int state_0 = this.state_0_;
if ((state_0 & 0b110000000) != 0 /* is SpecializationActive[SLUnboxNode.fromForeign(Object, InteropLibrary)] || SpecializationActive[SLUnboxNode.fromForeign(Object, InteropLibrary)] */) {
return SLTypesGen.expectLong(executeGeneric(frameValue));
}
long child0Value_;
try {
child0Value_ = this.child0_.executeLong(frameValue);
} catch (UnexpectedResultException ex) {
CompilerDirectives.transferToInterpreterAndInvalidate();
return SLTypesGen.expectLong(executeAndSpecialize(ex.getResult()));
}
if ((state_0 & 0b1000) != 0 /* is SpecializationActive[SLUnboxNode.fromLong(long)] */) {
return SLUnboxNode.fromLong(child0Value_);
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return SLTypesGen.expectLong(executeAndSpecialize(child0Value_));
}
@Override
public void executeVoid(VirtualFrame frameValue) {
int state_0 = this.state_0_;
try {
if ((state_0 & 0b111110111) == 0 /* only-active SpecializationActive[SLUnboxNode.fromLong(long)] */ && ((state_0 & 0b111111111) != 0 /* is-not SpecializationActive[SLUnboxNode.fromString(String, FromJavaStringNode)] && SpecializationActive[SLUnboxNode.fromTruffleString(TruffleString)] && SpecializationActive[SLUnboxNode.fromBoolean(boolean)] && SpecializationActive[SLUnboxNode.fromLong(long)] && SpecializationActive[SLUnboxNode.fromBigNumber(SLBigInteger)] && SpecializationActive[SLUnboxNode.fromFunction(SLFunction)] && SpecializationActive[SLUnboxNode.fromFunction(SLNull)] && SpecializationActive[SLUnboxNode.fromForeign(Object, InteropLibrary)] && SpecializationActive[SLUnboxNode.fromForeign(Object, InteropLibrary)] */)) {
executeLong(frameValue);
return;
} else if ((state_0 & 0b111111011) == 0 /* only-active SpecializationActive[SLUnboxNode.fromBoolean(boolean)] */ && ((state_0 & 0b111111111) != 0 /* is-not SpecializationActive[SLUnboxNode.fromString(String, FromJavaStringNode)] && SpecializationActive[SLUnboxNode.fromTruffleString(TruffleString)] && SpecializationActive[SLUnboxNode.fromBoolean(boolean)] && SpecializationActive[SLUnboxNode.fromLong(long)] && SpecializationActive[SLUnboxNode.fromBigNumber(SLBigInteger)] && SpecializationActive[SLUnboxNode.fromFunction(SLFunction)] && SpecializationActive[SLUnboxNode.fromFunction(SLNull)] && SpecializationActive[SLUnboxNode.fromForeign(Object, InteropLibrary)] && SpecializationActive[SLUnboxNode.fromForeign(Object, InteropLibrary)] */)) {
executeBoolean(frameValue);
return;
}
executeGeneric(frameValue);
return;
} catch (UnexpectedResultException ex) {
CompilerDirectives.transferToInterpreterAndInvalidate();
return;
}
}
private Object executeAndSpecialize(Object child0Value) {
int state_0 = this.state_0_;
if (child0Value instanceof String) {
String child0Value_ = (String) child0Value;
FromJavaStringNode fromJavaStringNode__ = this.insert((FromJavaStringNode.create()));
Objects.requireNonNull(fromJavaStringNode__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
VarHandle.storeStoreFence();
this.fromString_fromJavaStringNode_ = fromJavaStringNode__;
state_0 = state_0 | 0b1 /* add SpecializationActive[SLUnboxNode.fromString(String, FromJavaStringNode)] */;
this.state_0_ = state_0;
return SLUnboxNode.fromString(child0Value_, fromJavaStringNode__);
}
if (child0Value instanceof TruffleString) {
TruffleString child0Value_ = (TruffleString) child0Value;
state_0 = state_0 | 0b10 /* add SpecializationActive[SLUnboxNode.fromTruffleString(TruffleString)] */;
this.state_0_ = state_0;
return SLUnboxNode.fromTruffleString(child0Value_);
}
if (child0Value instanceof Boolean) {
boolean child0Value_ = (boolean) child0Value;
state_0 = state_0 | 0b100 /* add SpecializationActive[SLUnboxNode.fromBoolean(boolean)] */;
this.state_0_ = state_0;
return SLUnboxNode.fromBoolean(child0Value_);
}
if (child0Value instanceof Long) {
long child0Value_ = (long) child0Value;
state_0 = state_0 | 0b1000 /* add SpecializationActive[SLUnboxNode.fromLong(long)] */;
this.state_0_ = state_0;
return SLUnboxNode.fromLong(child0Value_);
}
{
int sLBigIntegerCast0;
if ((sLBigIntegerCast0 = SLTypesGen.specializeImplicitSLBigInteger(child0Value)) != 0) {
SLBigInteger child0Value_ = SLTypesGen.asImplicitSLBigInteger(sLBigIntegerCast0, child0Value);
state_0 = (state_0 | (sLBigIntegerCast0 << 9) /* set-int ImplicitCast[type=SLBigInteger, index=0] */);
state_0 = state_0 | 0b10000 /* add SpecializationActive[SLUnboxNode.fromBigNumber(SLBigInteger)] */;
this.state_0_ = state_0;
return SLUnboxNode.fromBigNumber(child0Value_);
}
}
if (child0Value instanceof SLFunction) {
SLFunction child0Value_ = (SLFunction) child0Value;
state_0 = state_0 | 0b100000 /* add SpecializationActive[SLUnboxNode.fromFunction(SLFunction)] */;
this.state_0_ = state_0;
return SLUnboxNode.fromFunction(child0Value_);
}
if (SLTypes.isSLNull(child0Value)) {
SLNull child0Value_ = SLTypes.asSLNull(child0Value);
state_0 = state_0 | 0b1000000 /* add SpecializationActive[SLUnboxNode.fromFunction(SLNull)] */;
this.state_0_ = state_0;
return SLUnboxNode.fromFunction(child0Value_);
}
if (((state_0 & 0b100000000)) == 0 /* is-not SpecializationActive[SLUnboxNode.fromForeign(Object, InteropLibrary)] */) {
while (true) {
int count7_ = 0;
FromForeign0Data s7_ = FROM_FOREIGN0_CACHE_UPDATER.getVolatile(this);
FromForeign0Data s7_original = s7_;
while (s7_ != null) {
if ((s7_.interop_.accepts(child0Value))) {
break;
}
count7_++;
s7_ = s7_.next_;
}
if (s7_ == null) {
// assert (s7_.interop_.accepts(child0Value));
if (count7_ < (SLUnboxNode.LIMIT)) {
s7_ = this.insert(new FromForeign0Data(s7_original));
InteropLibrary interop__ = s7_.insert((INTEROP_LIBRARY_.create(child0Value)));
Objects.requireNonNull(interop__, "A specialization cache returned a default value. The cache initializer must never return a default value for this cache. Use @Cached(neverDefault=false) to allow default values for this cached value or make sure the cache initializer never returns the default value.");
s7_.interop_ = interop__;
if (!FROM_FOREIGN0_CACHE_UPDATER.compareAndSet(this, s7_original, s7_)) {
continue;
}
state_0 = state_0 | 0b10000000 /* add SpecializationActive[SLUnboxNode.fromForeign(Object, InteropLibrary)] */;
this.state_0_ = state_0;
}
}
if (s7_ != null) {
return SLUnboxNode.fromForeign(child0Value, s7_.interop_);
}
break;
}
}
{
InteropLibrary interop__ = null;
{
EncapsulatingNodeReference encapsulating_ = EncapsulatingNodeReference.getCurrent();
Node prev_ = encapsulating_.set(this);
try {
interop__ = (INTEROP_LIBRARY_.getUncached(child0Value));
this.fromForeign0_cache = null;
state_0 = state_0 & 0xffffff7f /* remove SpecializationActive[SLUnboxNode.fromForeign(Object, InteropLibrary)] */;
state_0 = state_0 | 0b100000000 /* add SpecializationActive[SLUnboxNode.fromForeign(Object, InteropLibrary)] */;
this.state_0_ = state_0;
return SLUnboxNode.fromForeign(child0Value, interop__);
} finally {
encapsulating_.set(prev_);
}
}
}
}
@NeverDefault
public static SLUnboxNode create(SLExpressionNode child0) {
return new SLUnboxNodeGen(child0);
}
@GeneratedBy(SLUnboxNode.class)
@DenyReplace
private static final class FromForeign0Data extends Node implements SpecializationDataNode {
@Child FromForeign0Data next_;
/**
* Source Info:
* Specialization: {@link SLUnboxNode#fromForeign}
* Parameter: {@link InteropLibrary} interop
*/
@Child InteropLibrary interop_;
FromForeign0Data(FromForeign0Data next_) {
this.next_ = next_;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy