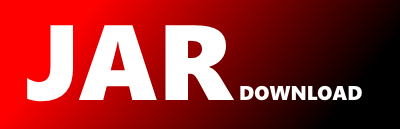
com.oracle.truffle.sl.runtime.SLFunctionGen Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of truffle-sl Show documentation
Show all versions of truffle-sl Show documentation
Truffle SL is an example language implemented using the Truffle API.
The newest version!
// CheckStyle: start generated
package com.oracle.truffle.sl.runtime;
import com.oracle.truffle.api.Assumption;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.RootCallTarget;
import com.oracle.truffle.api.TruffleLanguage;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.CompilerDirectives.TruffleBoundary;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.DSLSupport.SpecializationDataNode;
import com.oracle.truffle.api.dsl.InlineSupport.ReferenceField;
import com.oracle.truffle.api.dsl.InlineSupport.UnsafeAccessedField;
import com.oracle.truffle.api.interop.ArityException;
import com.oracle.truffle.api.interop.InteropLibrary;
import com.oracle.truffle.api.interop.UnsupportedMessageException;
import com.oracle.truffle.api.interop.UnsupportedTypeException;
import com.oracle.truffle.api.library.DynamicDispatchLibrary;
import com.oracle.truffle.api.library.LibraryExport;
import com.oracle.truffle.api.library.LibraryFactory;
import com.oracle.truffle.api.nodes.DenyReplace;
import com.oracle.truffle.api.nodes.DirectCallNode;
import com.oracle.truffle.api.nodes.ExplodeLoop;
import com.oracle.truffle.api.nodes.IndirectCallNode;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.nodes.UnadoptableNode;
import com.oracle.truffle.api.source.SourceSection;
import com.oracle.truffle.api.utilities.TriState;
import com.oracle.truffle.sl.runtime.SLFunction.Execute;
import com.oracle.truffle.sl.runtime.SLFunction.IsIdenticalOrUndefined;
import java.lang.invoke.MethodHandles;
import java.lang.invoke.VarHandle;
@GeneratedBy(SLFunction.class)
@SuppressWarnings({"javadoc", "unused"})
final class SLFunctionGen {
private static final LibraryFactory DYNAMIC_DISPATCH_LIBRARY_ = LibraryFactory.resolve(DynamicDispatchLibrary.class);
static {
LibraryExport.register(SLFunction.class, new InteropLibraryExports());
}
private SLFunctionGen() {
}
@GeneratedBy(SLFunction.class)
private static final class InteropLibraryExports extends LibraryExport {
private InteropLibraryExports() {
super(InteropLibrary.class, SLFunction.class, false, false, 0);
}
@Override
protected InteropLibrary createUncached(Object receiver) {
assert receiver instanceof SLFunction;
InteropLibrary uncached = new Uncached();
return uncached;
}
@Override
protected InteropLibrary createCached(Object receiver) {
assert receiver instanceof SLFunction;
return new Cached();
}
@GeneratedBy(SLFunction.class)
private static final class Cached extends InteropLibrary {
static final ReferenceField EXECUTE_DIRECT_CACHE_UPDATER = ReferenceField.create(MethodHandles.lookup(), "execute_direct_cache", ExecuteDirectData.class);
/**
* State Info:
* 0: SpecializationActive {@link IsIdenticalOrUndefined#doSLFunction}
* 1: SpecializationActive {@link IsIdenticalOrUndefined#doOther}
* 2: SpecializationActive {@link Execute#doDirect}
* 3: SpecializationActive {@link Execute#doIndirect}
*
*/
@CompilationFinal private int state_0_;
@UnsafeAccessedField @Child private ExecuteDirectData execute_direct_cache;
/**
* Source Info:
* Specialization: {@link Execute#doIndirect}
* Parameter: {@link IndirectCallNode} callNode
*/
@Child private IndirectCallNode execute_indirect_callNode_;
protected Cached() {
}
@Override
public boolean accepts(Object receiver) {
assert !(receiver instanceof SLFunction) || DYNAMIC_DISPATCH_LIBRARY_.getUncached().dispatch(receiver) == null : "Invalid library export. Exported receiver with dynamic dispatch found but not expected.";
return receiver instanceof SLFunction;
}
@SuppressWarnings("static-method")
private boolean isIdenticalOrUndefinedFallbackGuard_(int state_0, SLFunction arg0Value, Object arg1Value) {
if (!((state_0 & 0b1) != 0 /* is SpecializationActive[SLFunction.IsIdenticalOrUndefined.doSLFunction(SLFunction, SLFunction)] */) && arg1Value instanceof SLFunction) {
return false;
}
return true;
}
/**
* Debug Info:
* Specialization {@link IsIdenticalOrUndefined#doSLFunction}
* Activation probability: 0.32500
* With/without class size: 7/0 bytes
* Specialization {@link IsIdenticalOrUndefined#doOther}
* Activation probability: 0.17500
* With/without class size: 6/0 bytes
*
*/
@Override
protected TriState isIdenticalOrUndefined(Object arg0Value_, Object arg1Value) {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
SLFunction arg0Value = ((SLFunction) arg0Value_);
int state_0 = this.state_0_;
if ((state_0 & 0b11) != 0 /* is SpecializationActive[SLFunction.IsIdenticalOrUndefined.doSLFunction(SLFunction, SLFunction)] || SpecializationActive[SLFunction.IsIdenticalOrUndefined.doOther(SLFunction, Object)] */) {
if ((state_0 & 0b1) != 0 /* is SpecializationActive[SLFunction.IsIdenticalOrUndefined.doSLFunction(SLFunction, SLFunction)] */ && arg1Value instanceof SLFunction) {
SLFunction arg1Value_ = (SLFunction) arg1Value;
return IsIdenticalOrUndefined.doSLFunction(arg0Value, arg1Value_);
}
if ((state_0 & 0b10) != 0 /* is SpecializationActive[SLFunction.IsIdenticalOrUndefined.doOther(SLFunction, Object)] */) {
if (isIdenticalOrUndefinedFallbackGuard_(state_0, arg0Value, arg1Value)) {
return IsIdenticalOrUndefined.doOther(arg0Value, arg1Value);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return isIdenticalOrUndefinedAndSpecialize(arg0Value, arg1Value);
}
private TriState isIdenticalOrUndefinedAndSpecialize(SLFunction arg0Value, Object arg1Value) {
int state_0 = this.state_0_;
if (arg1Value instanceof SLFunction) {
SLFunction arg1Value_ = (SLFunction) arg1Value;
state_0 = state_0 | 0b1 /* add SpecializationActive[SLFunction.IsIdenticalOrUndefined.doSLFunction(SLFunction, SLFunction)] */;
this.state_0_ = state_0;
return IsIdenticalOrUndefined.doSLFunction(arg0Value, arg1Value_);
}
state_0 = state_0 | 0b10 /* add SpecializationActive[SLFunction.IsIdenticalOrUndefined.doOther(SLFunction, Object)] */;
this.state_0_ = state_0;
return IsIdenticalOrUndefined.doOther(arg0Value, arg1Value);
}
/**
* Debug Info:
* Specialization {@link Execute#doDirect}
* Activation probability: 0.32500
* With/without class size: 14/12 bytes
* Specialization {@link Execute#doIndirect}
* Activation probability: 0.17500
* With/without class size: 7/4 bytes
*
*/
@ExplodeLoop
@Override
public Object execute(Object arg0Value_, Object... arg1Value) throws UnsupportedTypeException, ArityException, UnsupportedMessageException {
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
SLFunction arg0Value = ((SLFunction) arg0Value_);
int state_0 = this.state_0_;
if ((state_0 & 0b1100) != 0 /* is SpecializationActive[SLFunction.Execute.doDirect(SLFunction, Object[], Assumption, RootCallTarget, DirectCallNode)] || SpecializationActive[SLFunction.Execute.doIndirect(SLFunction, Object[], IndirectCallNode)] */) {
if ((state_0 & 0b100) != 0 /* is SpecializationActive[SLFunction.Execute.doDirect(SLFunction, Object[], Assumption, RootCallTarget, DirectCallNode)] */) {
ExecuteDirectData s0_ = this.execute_direct_cache;
while (s0_ != null) {
if (!Assumption.isValidAssumption((s0_.callTargetStable_))) {
CompilerDirectives.transferToInterpreterAndInvalidate();
removeDirect_(s0_);
return executeAndSpecialize(arg0Value, arg1Value);
}
if ((arg0Value.getCallTarget() == s0_.cachedTarget_)) {
return Execute.doDirect(arg0Value, arg1Value, s0_.callTargetStable_, s0_.cachedTarget_, s0_.callNode_);
}
s0_ = s0_.next_;
}
}
if ((state_0 & 0b1000) != 0 /* is SpecializationActive[SLFunction.Execute.doIndirect(SLFunction, Object[], IndirectCallNode)] */) {
{
IndirectCallNode callNode__ = this.execute_indirect_callNode_;
if (callNode__ != null) {
return Execute.doIndirect(arg0Value, arg1Value, callNode__);
}
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(arg0Value, arg1Value);
}
private Object executeAndSpecialize(SLFunction arg0Value, Object[] arg1Value) {
int state_0 = this.state_0_;
int oldState_0 = (state_0 & 0b1100);
int oldCacheCount = execute_countCaches();
try {
if (((state_0 & 0b1000)) == 0 /* is-not SpecializationActive[SLFunction.Execute.doIndirect(SLFunction, Object[], IndirectCallNode)] */) {
while (true) {
int count0_ = 0;
ExecuteDirectData s0_ = EXECUTE_DIRECT_CACHE_UPDATER.getVolatile(this);
ExecuteDirectData s0_original = s0_;
while (s0_ != null) {
if ((arg0Value.getCallTarget() == s0_.cachedTarget_) && Assumption.isValidAssumption((s0_.callTargetStable_))) {
break;
}
count0_++;
s0_ = s0_.next_;
}
if (s0_ == null) {
{
RootCallTarget cachedTarget__ = (arg0Value.getCallTarget());
if ((arg0Value.getCallTarget() == cachedTarget__)) {
Assumption callTargetStable__ = (arg0Value.getCallTargetStable());
Assumption assumption0 = (callTargetStable__);
if (Assumption.isValidAssumption(assumption0)) {
if (count0_ < (SLFunction.INLINE_CACHE_SIZE)) {
s0_ = this.insert(new ExecuteDirectData(s0_original));
s0_.callTargetStable_ = callTargetStable__;
s0_.cachedTarget_ = cachedTarget__;
s0_.callNode_ = s0_.insert((DirectCallNode.create(cachedTarget__)));
if (!EXECUTE_DIRECT_CACHE_UPDATER.compareAndSet(this, s0_original, s0_)) {
continue;
}
state_0 = state_0 | 0b100 /* add SpecializationActive[SLFunction.Execute.doDirect(SLFunction, Object[], Assumption, RootCallTarget, DirectCallNode)] */;
this.state_0_ = state_0;
}
}
}
}
}
if (s0_ != null) {
return Execute.doDirect(arg0Value, arg1Value, s0_.callTargetStable_, s0_.cachedTarget_, s0_.callNode_);
}
break;
}
}
VarHandle.storeStoreFence();
this.execute_indirect_callNode_ = this.insert((IndirectCallNode.create()));
this.execute_direct_cache = null;
state_0 = state_0 & 0xfffffffb /* remove SpecializationActive[SLFunction.Execute.doDirect(SLFunction, Object[], Assumption, RootCallTarget, DirectCallNode)] */;
state_0 = state_0 | 0b1000 /* add SpecializationActive[SLFunction.Execute.doIndirect(SLFunction, Object[], IndirectCallNode)] */;
this.state_0_ = state_0;
return Execute.doIndirect(arg0Value, arg1Value, this.execute_indirect_callNode_);
} finally {
if (oldState_0 != 0) {
execute_checkForPolymorphicSpecialize(oldState_0, oldCacheCount);
}
}
}
private void execute_checkForPolymorphicSpecialize(int oldState_0, int oldCacheCount) {
int state_0 = this.state_0_;
int newState_0 = (state_0 & 0b1100);
if (((oldState_0 ^ newState_0) != 0) || oldCacheCount < execute_countCaches()) {
this.reportPolymorphicSpecialize();
}
}
private int execute_countCaches() {
int cacheCount = 0;
ExecuteDirectData s0_ = this.execute_direct_cache;
while (s0_ != null) {
cacheCount++;
s0_ = s0_.next_;
}
return cacheCount;
}
void removeDirect_(ExecuteDirectData s0_) {
while (true) {
ExecuteDirectData cur = this.execute_direct_cache;
ExecuteDirectData original = cur;
ExecuteDirectData update = null;
while (cur != null) {
if (cur == s0_) {
if (cur == original) {
update = cur.next_;
} else {
update = original.remove(this, s0_);
}
break;
}
cur = cur.next_;
}
if (cur != null && !EXECUTE_DIRECT_CACHE_UPDATER.compareAndSet(this, original, update)) {
continue;
}
break;
}
}
@Override
public boolean hasLanguage(Object receiver) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
return (((SLFunction) receiver)).hasLanguage();
}
@Override
public Class extends TruffleLanguage>> getLanguage(Object receiver) throws UnsupportedMessageException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
return (((SLFunction) receiver)).getLanguage();
}
@TruffleBoundary
@Override
public SourceSection getSourceLocation(Object receiver) throws UnsupportedMessageException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
return (((SLFunction) receiver)).getSourceLocation();
}
@Override
public boolean hasSourceLocation(Object receiver) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
return (((SLFunction) receiver)).hasSourceLocation();
}
@Override
public boolean isExecutable(Object receiver) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
return (((SLFunction) receiver)).isExecutable();
}
@Override
public boolean hasMetaObject(Object receiver) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
return (((SLFunction) receiver)).hasMetaObject();
}
@Override
public Object getMetaObject(Object receiver) throws UnsupportedMessageException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
return (((SLFunction) receiver)).getMetaObject();
}
@TruffleBoundary
@Override
public int identityHashCode(Object receiver) throws UnsupportedMessageException {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
return SLFunction.identityHashCode((((SLFunction) receiver)));
}
@Override
public Object toDisplayString(Object receiver, boolean allowSideEffects) {
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
assert assertAdopted();
return (((SLFunction) receiver)).toDisplayString(allowSideEffects);
}
@GeneratedBy(SLFunction.class)
@DenyReplace
private static final class ExecuteDirectData extends Node implements SpecializationDataNode {
@Child ExecuteDirectData next_;
/**
* Source Info:
* Specialization: {@link Execute#doDirect}
* Parameter: {@link Assumption} callTargetStable
*/
@CompilationFinal Assumption callTargetStable_;
/**
* Source Info:
* Specialization: {@link Execute#doDirect}
* Parameter: {@link RootCallTarget} cachedTarget
*/
@CompilationFinal RootCallTarget cachedTarget_;
/**
* Source Info:
* Specialization: {@link Execute#doDirect}
* Parameter: {@link DirectCallNode} callNode
*/
@Child DirectCallNode callNode_;
ExecuteDirectData(ExecuteDirectData next_) {
this.next_ = next_;
}
ExecuteDirectData remove(Node parent, ExecuteDirectData search) {
ExecuteDirectData newNext = this.next_;
if (newNext != null) {
if (search == newNext) {
newNext = newNext.next_;
} else {
newNext = newNext.remove(this, search);
}
}
ExecuteDirectData copy = parent.insert(new ExecuteDirectData(newNext));
copy.callTargetStable_ = this.callTargetStable_;
copy.cachedTarget_ = this.cachedTarget_;
copy.callNode_ = copy.insert(this.callNode_);
return copy;
}
}
}
@GeneratedBy(SLFunction.class)
@DenyReplace
private static final class Uncached extends InteropLibrary implements UnadoptableNode {
protected Uncached() {
}
@Override
@TruffleBoundary
public boolean accepts(Object receiver) {
assert !(receiver instanceof SLFunction) || DYNAMIC_DISPATCH_LIBRARY_.getUncached().dispatch(receiver) == null : "Invalid library export. Exported receiver with dynamic dispatch found but not expected.";
return receiver instanceof SLFunction;
}
@TruffleBoundary
@Override
public TriState isIdenticalOrUndefined(Object arg0Value_, Object arg1Value) {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
SLFunction arg0Value = ((SLFunction) arg0Value_);
if (arg1Value instanceof SLFunction) {
SLFunction arg1Value_ = (SLFunction) arg1Value;
return IsIdenticalOrUndefined.doSLFunction(arg0Value, arg1Value_);
}
return IsIdenticalOrUndefined.doOther(arg0Value, arg1Value);
}
@TruffleBoundary
@Override
public Object execute(Object arg0Value_, Object... arg1Value) {
// declared: true
assert this.accepts(arg0Value_) : "Invalid library usage. Library does not accept given receiver.";
SLFunction arg0Value = ((SLFunction) arg0Value_);
return Execute.doIndirect(arg0Value, arg1Value, (IndirectCallNode.getUncached()));
}
@TruffleBoundary
@Override
public boolean hasLanguage(Object receiver) {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((SLFunction) receiver) .hasLanguage();
}
@TruffleBoundary
@Override
public Class extends TruffleLanguage>> getLanguage(Object receiver) throws UnsupportedMessageException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((SLFunction) receiver) .getLanguage();
}
@TruffleBoundary
@Override
public SourceSection getSourceLocation(Object receiver) throws UnsupportedMessageException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((SLFunction) receiver) .getSourceLocation();
}
@TruffleBoundary
@Override
public boolean hasSourceLocation(Object receiver) {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((SLFunction) receiver) .hasSourceLocation();
}
@TruffleBoundary
@Override
public boolean isExecutable(Object receiver) {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((SLFunction) receiver) .isExecutable();
}
@TruffleBoundary
@Override
public boolean hasMetaObject(Object receiver) {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((SLFunction) receiver) .hasMetaObject();
}
@TruffleBoundary
@Override
public Object getMetaObject(Object receiver) throws UnsupportedMessageException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((SLFunction) receiver) .getMetaObject();
}
@TruffleBoundary
@Override
public int identityHashCode(Object receiver) throws UnsupportedMessageException {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return SLFunction.identityHashCode(((SLFunction) receiver) );
}
@TruffleBoundary
@Override
public Object toDisplayString(Object receiver, boolean allowSideEffects) {
// declared: true
assert this.accepts(receiver) : "Invalid library usage. Library does not accept given receiver.";
return ((SLFunction) receiver) .toDisplayString(allowSideEffects);
}
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy