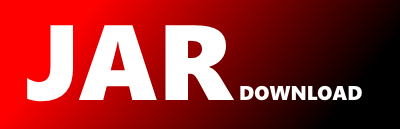
grails.async.PromiseFactory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of grails-async Show documentation
Show all versions of grails-async Show documentation
Grails Web Application Framework
/*
* Copyright 2013 SpringSource
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package grails.async;
import groovy.lang.Closure;
import java.util.List;
import java.util.Map;
import java.util.concurrent.TimeUnit;
import org.grails.async.decorator.PromiseDecorator;
import org.grails.async.decorator.PromiseDecoratorLookupStrategy;
/**
* An interface capable of creating {@link Promise} instances. The {@link Promises} static methods use this
* interface to create promises. The default Promise creation mechanism can be overriden by setting {@link Promises#setPromiseFactory(PromiseFactory)}
*
* @author Graeme Rocher
* @since 2.3
*/
public interface PromiseFactory {
/**
* Adds a PromiseDecoratorLookupStrategy. The strategy implementation must be stateless, do not add a strategy that contains state
*
* @param lookupStrategy The lookup strategy
*/
void addPromiseDecoratorLookupStrategy(PromiseDecoratorLookupStrategy lookupStrategy);
/**
* Applies the registered decorators to the given closure
* @param c The closure
* @param decorators The decorators
* @return The decorated closure
*/
Closure applyDecorators(Closure c, List decorators);
/**
* Creates a promise with a value pre-bound to it
* @param value The value
* @param The type of the value
* @return A Promise
*/
Promise createBoundPromise(T value);
/**
* Creates a promise from the given map where the values of the map are either closures or Promise instances
*
* @param map The map
* @return A promise
*/
Promise
© 2015 - 2025 Weber Informatics LLC | Privacy Policy