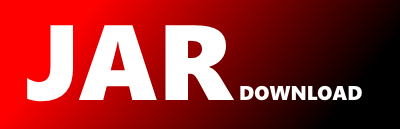
org.grails.async.factory.AbstractPromiseFactory Maven / Gradle / Ivy
/*
* Copyright 2013 SpringSource
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.grails.async.factory;
import grails.async.Promise;
import grails.async.PromiseFactory;
import grails.async.PromiseList;
import grails.async.PromiseMap;
import groovy.lang.Closure;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.List;
import java.util.Map;
import java.util.concurrent.ConcurrentLinkedQueue;
import org.grails.async.decorator.PromiseDecorator;
import org.grails.async.decorator.PromiseDecoratorLookupStrategy;
/**
* Abstract implementation of the {@link grails.async.PromiseFactory} interface, subclasses should extend
* this class to obtain common generic functionality
*
* @author Graeme Rocher
* @since 2.3
*/
public abstract class AbstractPromiseFactory implements PromiseFactory {
protected Collection lookupStrategies = new ConcurrentLinkedQueue();
public void addPromiseDecoratorLookupStrategy(PromiseDecoratorLookupStrategy lookupStrategy) {
lookupStrategies.add(lookupStrategy);
}
public Promise createBoundPromise(T value) {
return new BoundPromise(value);
}
/**
* @see PromiseFactory#createPromise(groovy.lang.Closure, java.util.List)
*/
@SuppressWarnings("unchecked")
public Promise createPromise(Closure c, List decorators) {
c = applyDecorators(c, decorators);
return createPromise(c);
}
@SuppressWarnings({ "rawtypes", "unchecked" })
public Closure applyDecorators(Closure c, List decorators) {
List allDecorators = decorators != null ? new ArrayList(decorators): new ArrayList();
for (PromiseDecoratorLookupStrategy lookupStrategy : lookupStrategies) {
allDecorators.addAll(lookupStrategy.findDecorators());
}
if (!allDecorators.isEmpty()) {
for(PromiseDecorator d : allDecorators) {
c = d.decorate(c);
}
}
return c;
}
/**
* @see PromiseFactory#createPromise(java.util.List)
*/
public Promise> createPromise(List> closures) {
return createPromise(closures,null);
}
/**
* @see PromiseFactory#createPromise(java.util.List, java.util.List)
*/
@SuppressWarnings("unchecked")
public Promise> createPromise(List> closures, List decorators) {
List> newClosures = new ArrayList>(closures.size());
for (Closure closure : closures) {
newClosures.add(applyDecorators(closure, decorators));
}
closures = newClosures;
PromiseList promiseList = new PromiseList();
for (Closure closure : closures) {
promiseList.add(closure);
}
return promiseList;
}
/**
* @see PromiseFactory#createPromise(grails.async.Promise[])
*/
public Promise> createPromise(Promise... promises) {
PromiseList promiseList = new PromiseList();
for(Promise p : promises) {
promiseList.add(p);
}
return promiseList;
}
/**
* @see PromiseFactory#createPromise(java.util.Map)
*/
@SuppressWarnings("unchecked")
public Promise
© 2015 - 2025 Weber Informatics LLC | Privacy Policy