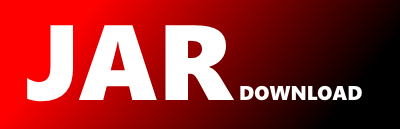
org.grails.async.factory.SynchronousPromiseFactory.groovy Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of grails-async Show documentation
Show all versions of grails-async Show documentation
Grails Web Application Framework
/*
* Copyright 2013 SpringSource
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.grails.async.factory
import grails.async.Promise
import grails.async.PromiseList
import groovy.transform.CompileStatic
import java.util.concurrent.TimeUnit
/**
* A {@link grails.async.PromiseFactory} implementation that constructors promises that execute synchronously.
* Useful for testing environments.
*
* @author Graeme Rocher
* @since 2.3
*/
@CompileStatic
class SynchronousPromiseFactory extends AbstractPromiseFactory {
@Override
def Promise createPromise(Closure... closures) {
if (closures.length == 1) {
return new SynchronousPromise(closures[0])
}
def promiseList = new PromiseList()
for(p in closures) {
promiseList << p
}
return promiseList
}
@Override
def List waitAll(List> promises) {
return promises.collect() { Promise p -> p.get() }
}
@Override
def List waitAll(List> promises, long timeout, TimeUnit units) {
return promises.collect() { Promise p -> p.get() }
}
def Promise> onComplete(List> promises, Closure> callable) {
try {
List values = promises.collect { Promise p -> p.get() }
final result = callable.call(values)
return new BoundPromise(result)
} catch (Throwable e) {
return new BoundPromise(e)
}
}
def Promise> onError(List> promises, Closure> callable) {
try {
final values = promises.collect() { Promise p -> p.get() }
return new BoundPromise>(values)
} catch (Throwable e) {
callable.call(e)
return new BoundPromise(e)
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy