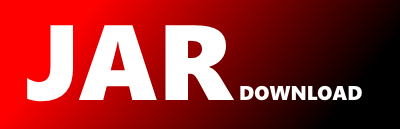
org.grails.web.mapping.UrlCreatorCache Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of grails-web-url-mappings Show documentation
Show all versions of grails-web-url-mappings Show documentation
Grails Web Application Framework
The newest version!
/*
* Copyright 2004-2005 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.grails.web.mapping;
import java.util.Arrays;
import java.util.Collection;
import java.util.Map;
import java.util.concurrent.ConcurrentHashMap;
import com.github.benmanes.caffeine.cache.Cache;
import com.github.benmanes.caffeine.cache.Caffeine;
import com.github.benmanes.caffeine.cache.Weigher;
import grails.web.mapping.UrlCreator;
import grails.web.mapping.UrlMapping;
import org.codehaus.groovy.runtime.DefaultGroovyMethods;
/**
* Implements caching layer for UrlCreator
*
* The "weight" of the cache is the estimated number of characters all cache entries will consume in memory.
* The estimate is not accurate. It's just used as a hard limit for limiting the cache size.
*
* You can tune the maximum weight of the cache by setting "grails.urlcreator.cache.maxsize" in Config.groovy.
* The default value is 160000 .
*
* @author Lari Hotari
* @since 1.3.5
*/
@SuppressWarnings({ "unchecked", "rawtypes" })
public class UrlCreatorCache {
private final Cache cacheMap;
private enum CachingUrlCreatorWeigher implements Weigher {
INSTANCE;
@Override
public int weigh(ReverseMappingKey key, CachingUrlCreator value) {
return value.weight() + 1;
}
}
public UrlCreatorCache(int maxSize) {
cacheMap = Caffeine.newBuilder()
.maximumWeight(maxSize).weigher(CachingUrlCreatorWeigher.INSTANCE).build();
}
public void clear() {
cacheMap.invalidateAll();
}
public ReverseMappingKey createKey(String controller, String action, String namespace, String pluginName, String httpMethod, Map params) {
return new ReverseMappingKey(controller, action, namespace, pluginName,httpMethod, params);
}
public UrlCreator lookup(ReverseMappingKey key) {
return cacheMap.getIfPresent(key);
}
public UrlCreator putAndDecorate(ReverseMappingKey key, UrlCreator delegate) {
CachingUrlCreator cachingUrlCreator = new CachingUrlCreator(delegate, key.weight() * 2);
CachingUrlCreator prevCachingUrlCreator = cacheMap.asMap()
.putIfAbsent(key, cachingUrlCreator);
if (prevCachingUrlCreator != null) {
return prevCachingUrlCreator;
}
return cachingUrlCreator;
}
private class CachingUrlCreator implements UrlCreator {
private UrlCreator delegate;
private ConcurrentHashMap cache = new ConcurrentHashMap();
private final int weight;
public CachingUrlCreator(UrlCreator delegate, int weight) {
this.delegate = delegate;
this.weight = weight;
}
public int weight() {
return weight;
}
public String createRelativeURL(String controller, String action, Map parameterValues,
String encoding, String fragment) {
return createRelativeURL(controller, action, null, null, parameterValues, encoding, fragment);
}
@Override
public String createRelativeURL(String controller, String action,
String pluginName, Map parameterValues, String encoding) {
return createRelativeURL(controller, action, null, pluginName, parameterValues, encoding);
}
public String createRelativeURL(String controller, String action, String namespace, String pluginName, Map parameterValues,
String encoding, String fragment) {
UrlCreatorKey key = new UrlCreatorKey(controller, action, namespace, pluginName, null,parameterValues, encoding, fragment, 0);
String url = cache.get(key);
if (url == null) {
url = delegate.createRelativeURL(controller, action, namespace, pluginName, parameterValues, encoding, fragment);
cache.put(key, url);
}
return url;
}
public String createRelativeURL(String controller, String action, Map parameterValues, String encoding) {
return createRelativeURL(controller, action, null, null, parameterValues, encoding);
}
public String createRelativeURL(String controller, String action, String namespace, String pluginName, Map parameterValues, String encoding) {
UrlCreatorKey key = new UrlCreatorKey(controller, action, namespace, pluginName, null,parameterValues, encoding, null, 0);
String url = cache.get(key);
if (url == null) {
url = delegate.createRelativeURL(controller, action, namespace, pluginName, parameterValues, encoding);
cache.put(key, url);
}
return url;
}
public String createURL(String controller, String action, Map parameterValues, String encoding, String fragment) {
return createURL(controller, action, null, null, parameterValues, encoding, fragment);
}
public String createURL(String controller, String action, String pluginName, Map parameterValues, String encoding) {
return createURL(controller, action, null, pluginName, parameterValues, encoding);
}
public String createURL(String controller, String action, String namespace, String pluginName, Map parameterValues, String encoding, String fragment) {
UrlCreatorKey key = new UrlCreatorKey(controller, action, namespace, pluginName,null, parameterValues, encoding, fragment, 1);
String url = cache.get(key);
if (url == null) {
url = delegate.createURL(controller, action, namespace, pluginName, parameterValues, encoding, fragment);
cache.put(key, url);
}
return url;
}
public String createURL(String controller, String action, Map parameterValues, String encoding) {
return createURL(controller, action, null, null, parameterValues, encoding);
}
public String createURL(String controller, String action, String namespace, String pluginName, Map parameterValues, String encoding) {
UrlCreatorKey key = new UrlCreatorKey(controller, action, namespace, pluginName,null, parameterValues, encoding, null, 1);
String url = cache.get(key);
if (url == null) {
url = delegate.createURL(controller, action, namespace, pluginName, parameterValues, encoding);
cache.put(key, url);
}
return url;
}
// don't cache these methods at all
public String createURL(Map parameterValues, String encoding, String fragment) {
return delegate.createURL(parameterValues, encoding, fragment);
}
public String createURL(Map parameterValues, String encoding) {
return delegate.createURL(parameterValues, encoding);
}
}
public static class ReverseMappingKey {
protected final String controller;
protected final String action;
protected final String namespace;
protected final String pluginName;
protected final String httpMethod;
protected final String[] paramKeys;
protected final String[] paramValues;
public ReverseMappingKey(String controller, String action, String namespace, String pluginName, String httpMethod, Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy