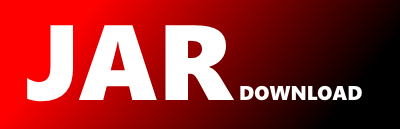
org.granite.client.tide.data.spi.DataManager Maven / Gradle / Ivy
/*
GRANITE DATA SERVICES
Copyright (C) 2012 GRANITE DATA SERVICES S.A.S.
This file is part of Granite Data Services.
Granite Data Services is free software; you can redistribute it and/or modify
it under the terms of the GNU Library General Public License as published by
the Free Software Foundation; either version 2 of the License, or (at your
option) any later version.
Granite Data Services is distributed in the hope that it will be useful, but
WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or
FITNESS FOR A PARTICULAR PURPOSE. See the GNU Library General Public License
for more details.
You should have received a copy of the GNU Library General Public License
along with this library; if not, see .
*/
package org.granite.client.tide.data.spi;
import java.util.List;
import java.util.Map;
import java.util.Set;
import javax.validation.ConstraintViolation;
import javax.validation.TraversableResolver;
import org.granite.client.persistence.LazyableCollection;
import org.granite.client.tide.collections.ManagedPersistentCollection;
import org.granite.client.tide.collections.ManagedPersistentMap;
import org.granite.client.tide.data.Identifiable;
/**
* @author William DRAI
*/
public interface DataManager {
public void setTrackingHandler(TrackingHandler trackingHandler);
public boolean isDirty();
public EntityDescriptor getEntityDescriptor(Object entity);
public Object getProperty(Object object, String propertyName);
public void setProperty(Object object, String propertyName, Object oldValue, Object newValue);
public void setInternalProperty(Object object, String propertyName, Object value);
public Map getPropertyValues(Object object, boolean includeReadOnly, boolean includeTransient);
public Map getPropertyValues(Object object, List excludedProperties, boolean includeReadOnly, boolean includeTransient);
public ManagedPersistentCollection
© 2015 - 2025 Weber Informatics LLC | Privacy Policy