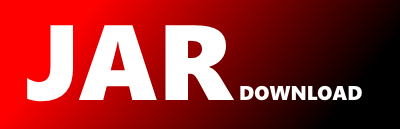
org.neo4j.function.Predicates Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ongdb-common Show documentation
Show all versions of ongdb-common Show documentation
Non-domain infrastructure code that is common to many Neo4j components.
/*
* Copyright (c) 2018-2020 "Graph Foundation,"
* Graph Foundation, Inc. [https://graphfoundation.org]
*
* This file is part of ONgDB.
*
* ONgDB is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see .
*/
/*
* Copyright (c) 2002-2020 "Neo4j,"
* Neo4j Sweden AB [http://neo4j.com]
*
* This file is part of Neo4j.
*
* Neo4j is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see .
*/
package org.neo4j.function;
import java.time.Clock;
import java.util.Arrays;
import java.util.HashSet;
import java.util.Objects;
import java.util.Set;
import java.util.concurrent.TimeUnit;
import java.util.concurrent.TimeoutException;
import java.util.concurrent.locks.LockSupport;
import java.util.function.BooleanSupplier;
import java.util.function.IntPredicate;
import java.util.function.Predicate;
import java.util.function.Supplier;
import javax.annotation.Nonnull;
import static org.neo4j.function.ThrowingPredicate.throwingPredicate;
import static org.neo4j.function.ThrowingSupplier.throwingSupplier;
/**
* Constructors for basic {@link Predicate} types
*/
public class Predicates
{
public static final IntPredicate ALWAYS_TRUE_INT = v -> true;
private static final int DEFAULT_POLL_INTERVAL = 20;
private Predicates()
{
}
public static Predicate alwaysTrue()
{
return x -> true;
}
public static Predicate alwaysFalse()
{
return x -> false;
}
public static Predicate notNull()
{
return Objects::nonNull;
}
@SafeVarargs
public static Predicate all( final Predicate... predicates )
{
return all( Arrays.asList( predicates ) );
}
public static Predicate all( final Iterable> predicates )
{
return item ->
{
for ( Predicate predicate : predicates )
{
if ( !predicate.test( item ) )
{
return false;
}
}
return true;
};
}
@SafeVarargs
public static Predicate any( final Predicate... predicates )
{
return any( Arrays.asList( predicates ) );
}
public static Predicate any( final Iterable> predicates )
{
return item ->
{
for ( Predicate predicate : predicates )
{
if ( predicate.test( item ) )
{
return true;
}
}
return false;
};
}
public static Predicate instanceOf( @Nonnull final Class> clazz )
{
return clazz::isInstance;
}
public static Predicate instanceOfAny( final Class>... classes )
{
return item ->
{
if ( item != null )
{
for ( Class> clazz : classes )
{
if ( clazz.isInstance( item ) )
{
return true;
}
}
}
return false;
};
}
public static Predicate noDuplicates()
{
return new Predicate()
{
private final Set visitedItems = new HashSet<>();
@Override
public boolean test( T item )
{
return visitedItems.add( item );
}
};
}
public static TYPE await( Supplier supplier, Predicate predicate, long timeout,
TimeUnit timeoutUnit, long pollInterval, TimeUnit pollUnit ) throws TimeoutException
{
return awaitEx( supplier::get, predicate::test, timeout, timeoutUnit, pollInterval, pollUnit );
}
public static TYPE await( Supplier supplier, Predicate predicate, long timeout,
TimeUnit timeoutUnit ) throws TimeoutException
{
return awaitEx( throwingSupplier( supplier ), throwingPredicate( predicate ), timeout, timeoutUnit );
}
public static TYPE awaitEx( ThrowingSupplier supplier,
ThrowingPredicate predicate, long timeout, TimeUnit timeoutUnit, long pollInterval,
TimeUnit pollUnit ) throws TimeoutException, EXCEPTION
{
Suppliers.ThrowingCapturingSupplier composed = Suppliers.compose( supplier, predicate );
awaitEx( composed, timeout, timeoutUnit, pollInterval, pollUnit );
return composed.lastInput();
}
public static TYPE awaitEx( ThrowingSupplier supplier,
ThrowingPredicate predicate, long timeout, TimeUnit timeoutUnit )
throws TimeoutException, EXCEPTION
{
Suppliers.ThrowingCapturingSupplier composed = Suppliers.compose( supplier, predicate );
awaitEx( composed, timeout, timeoutUnit );
return composed.lastInput();
}
public static void await( BooleanSupplier condition, long timeout, TimeUnit unit ) throws TimeoutException
{
awaitEx( condition::getAsBoolean, timeout, unit );
}
public static void awaitEx( ThrowingSupplier condition,
long timeout, TimeUnit unit ) throws TimeoutException, EXCEPTION
{
awaitEx( condition, timeout, unit, DEFAULT_POLL_INTERVAL, TimeUnit.MILLISECONDS );
}
public static void await( BooleanSupplier condition, long timeout, TimeUnit timeoutUnit, long pollInterval,
TimeUnit pollUnit ) throws TimeoutException
{
awaitEx( condition::getAsBoolean, timeout, timeoutUnit, pollInterval, pollUnit );
}
public static void awaitEx( ThrowingSupplier condition,
long timeout, TimeUnit unit, long pollInterval, TimeUnit pollUnit ) throws TimeoutException, EXCEPTION
{
if ( !tryAwaitEx( condition, timeout, unit, pollInterval, pollUnit ) )
{
throw new TimeoutException(
"Waited for " + timeout + " " + unit + ", but " + condition + " was not accepted." );
}
}
public static boolean tryAwaitEx( ThrowingSupplier condition,
long timeout, TimeUnit timeoutUnit, long pollInterval, TimeUnit pollUnit ) throws EXCEPTION
{
return tryAwaitEx( condition, timeout, timeoutUnit, pollInterval, pollUnit, Clock.systemUTC() );
}
public static boolean tryAwaitEx( ThrowingSupplier condition,
long timeout, TimeUnit timeoutUnit, long pollInterval, TimeUnit pollUnit, Clock clock ) throws EXCEPTION
{
long deadlineMillis = clock.millis() + timeoutUnit.toMillis( timeout );
long pollIntervalNanos = pollUnit.toNanos( pollInterval );
do
{
if ( condition.get() )
{
return true;
}
LockSupport.parkNanos( pollIntervalNanos );
}
while ( clock.millis() < deadlineMillis );
return false;
}
public static void awaitForever( BooleanSupplier condition, long checkInterval, TimeUnit unit )
{
long sleep = unit.toNanos( checkInterval );
do
{
if ( condition.getAsBoolean() )
{
return;
}
LockSupport.parkNanos( sleep );
}
while ( true );
}
@SafeVarargs
public static Predicate in( final T... allowed )
{
return in( Arrays.asList( allowed ) );
}
public static Predicate not( Predicate predicate )
{
return t -> !predicate.test( t );
}
public static Predicate in( final Iterable allowed )
{
return item ->
{
for ( T allow : allowed )
{
if ( allow.equals( item ) )
{
return true;
}
}
return false;
};
}
public static IntPredicate any( int[] values )
{
return v ->
{
for ( int value : values )
{
if ( v == value )
{
return true;
}
}
return false;
};
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy