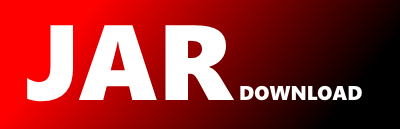
org.opencypher.railroad.AsciiArtRenderer Maven / Gradle / Ivy
The newest version!
/*
* Copyright (c) 2015-2018 "Neo Technology,"
* Network Engine for Objects in Lund AB [http://neotechnology.com]
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
* Attribution Notice under the terms of the Apache License 2.0
*
* This work was created by the collective efforts of the openCypher community.
* Without limiting the terms of Section 6, any Derivative Work that is not
* approved by the public consensus process of the openCypher Implementers Group
* should not be described as “Cypher” (and Cypher® is a registered trademark of
* Neo4j Inc.) or as "openCypher". Extensions by implementers or prototypes or
* proposals for change that have been documented or implemented should only be
* described as "implementation extensions to Cypher" or as "proposed changes to
* Cypher that are not yet approved by the openCypher community".
*/
package org.opencypher.railroad;
import java.util.Collection;
import java.util.List;
public class AsciiArtRenderer extends PositionedText.Renderer
{
@Override
public Size sizeOfBullet()
{
return new Size( 1, 1, 1 );
}
/**
* Example:
* {@code o}
*/
@Override
public void renderBullet( PositionedText target, double x, double y )
{
target.add( x, y, "o" );
}
@Override
public Size sizeOfNothing()
{
return new Size( 1, 1, 1 );
}
/**
* Example:
* {@code >}
*/
@Override
public void renderNothing( PositionedText target, double x, double y, boolean forward )
{
target.add( x, y, ">" );
}
@Override
public Size sizeOfText( String text )
{
return new Size( text.length() + 2, 1, 1 );
}
/**
* Example:
* {@code (FOO)}
*/
@Override
public void renderText( PositionedText target, double x, double y, String text )
{
target.add( x, y, "(" + text + ")" );
}
@Override
public Size sizeOfAnyCase( String text )
{
return new Size( text.length() + 2, 1, 1 );
}
/**
* Example:
* {@code /FOO/}
*/
@Override
public void renderAnyCase( PositionedText target, double x, double y, String text )
{
target.add( x, y, "/" + text + "/" );
}
@Override
public Size sizeOfReference( String name )
{
return new Size( name.length() + 2, 1, 1 );
}
/**
* Example:
* {@code |foo|}
*/
@Override
public void renderReference( PositionedText canvas, double x, double y, String target, String name )
{
canvas.add( x, y, "|" + name + "|" );
}
@Override
public Size sizeOfCharset( String text )
{
return new Size( text.length(), 1, 1 );
}
/**
* Examples:
*
* - {@code [:ID_Start:]}
*
- {@code [^a-z]}
*
*/
@Override
public void renderCharset( PositionedText target, double x, double y, String text, String set )
{
target.add( x, y, text );
}
@Override
public Size sizeOfLine( Collection sequence )
{
throw new UnsupportedOperationException( "not implemented" );
}
/**
* Example:
*
* o->|alpha|->(,)->|beta|->o
*
*/
@Override
public void renderLine( PositionedText target, double x, double y,
Size size, List sequence, boolean forward )
{
throw new UnsupportedOperationException( "not implemented" );
}
@Override
public Size sizeOfBranch( Collection branches )
{
throw new UnsupportedOperationException( "not implemented" );
}
/**
* Examples:
*
* o-+-->|one|--+->o
* | |
* +-->|two|--+
* | |
* +->|three|-+
*
*/
@Override
public void renderBranch( PositionedText target, double x, double y,
Size size, Collection branches, boolean forward )
{
throw new UnsupportedOperationException( "not implemented" );
}
@Override
public Size sizeOfLoop( Diagram.Figure forward, Diagram.Figure backward, String description )
{
throw new UnsupportedOperationException( "not implemented" );
}
/**
* Example:
*
* +--|two|<-+
* | |
* o-+->|one|--+->o
*
*/
@Override
public void renderLoop( PositionedText target, double x, double y, Size size, Diagram.Figure forward,
Diagram.Figure backward, String description, boolean forwardDirection )
{
throw new UnsupportedOperationException( "not implemented" );
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy