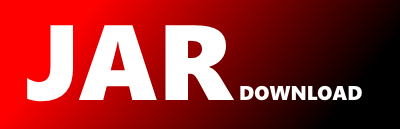
org.graphstream.algorithm.Centroid Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gs-algo Show documentation
Show all versions of gs-algo Show documentation
The GraphStream library. With GraphStream you deal with
graphs. Static and Dynamic. You create them from scratch, from a file
or any source. You display and render them. This package contains algorithms and generators.
/*
* Copyright 2006 - 2015
* Stefan Balev
* Julien Baudry
* Antoine Dutot
* Yoann Pigné
* Guilhelm Savin
*
* This file is part of GraphStream .
*
* GraphStream is a library whose purpose is to handle static or dynamic
* graph, create them from scratch, file or any source and display them.
*
* This program is free software distributed under the terms of two licenses, the
* CeCILL-C license that fits European law, and the GNU Lesser General Public
* License. You can use, modify and/ or redistribute the software under the terms
* of the CeCILL-C license as circulated by CEA, CNRS and INRIA at the following
* URL or under the terms of the GNU LGPL as published by
* the Free Software Foundation, either version 3 of the License, or (at your
* option) any later version.
*
* This program is distributed in the hope that it will be useful, but WITHOUT ANY
* WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS FOR A
* PARTICULAR PURPOSE. See the GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program. If not, see .
*
* The fact that you are presently reading this means that you have had
* knowledge of the CeCILL-C and LGPL licenses and that you accept their terms.
*/
package org.graphstream.algorithm;
import java.util.HashSet;
import org.graphstream.graph.Graph;
import org.graphstream.graph.Node;
/**
* Compute the centroid of a connected graph.
*
*
* In a graph G, if d(u,v) is the shortest length between two nodes u and v (ie
* the number of edges of the shortest path) let m(u) be the sum of d(u,v) for
* all nodes v of G. Centroid of a graph G is a subgraph induced by vertices u
* with minimum m(u).
*
*
* Requirements
*
*
* This algorithm needs that APSP algorithm has been computed before its own
* computation.
*
*
* Example
*
*
* import java.io.StringReader;
* import java.io.IOException;
*
* import org.graphstream.algorithm.APSP;
* import org.graphstream.algorithm.Centroid;
* import org.graphstream.graph.Graph;
* import org.graphstream.graph.Node;
* import org.graphstream.graph.implementations.DefaultGraph;
* import org.graphstream.stream.file.FileSourceDGS;
*
* // +--- E
* // A --- B --- C -- D -|--- F
* // +--- G
*
* public class CentroidTest {
* static String my_graph = "DGS004\n" + "my 0 0\n" + "an A \n" + "an B \n"
* + "an C \n" + "an D \n" + "an E \n" + "an F \n" + "an G \n"
* + "ae AB A B \n" + "ae BC B C \n" + "ae CD C D \n" + "ae DE D E \n"
* + "ae DF D F \n" + "ae DG D G \n";
*
* public static void main(String[] args) throws IOException {
* Graph graph = new DefaultGraph("Centroid Test");
* StringReader reader = new StringReader(my_graph);
*
* FileSourceDGS source = new FileSourceDGS();
* source.addSink(graph);
* source.readAll(reader);
*
* APSP apsp = new APSP();
* apsp.init(graph);
* apsp.compute();
*
* Centroid centroid = new Centroid();
* centroid.init(graph);
* centroid.compute();
*
* for (Node n : graph.getEachNode()) {
* Boolean in = n.getAttribute("centroid");
*
* System.out.printf("%s is%s in the centroid.\n", n.getId(), in ? ""
* : " not");
* }
*
* // Output will be :
* //
* // A is not in the centroid
* // B is not in the centroid
* // C is not in the centroid
* // D is in the centroid
* // E is not in the centroid
* // F is not in the centroid
* // G is not in the centroid
* }
* }
*
*
* @complexity O(n2)
* @see org.graphstream.algorithm.APSP.APSPInfo
* @reference F. Harary, Graph Theory. Westview Press, Oct. 1969. [Online].
* Available: http://www.amazon.com/exec/obidos/
* redirect?tag=citeulike07-20\&path=ASIN/ 0201410338
*/
public class Centroid implements Algorithm {
/**
* The graph on which centroid is computed.
*/
protected Graph graph;
/**
* Attribute in which APSPInfo are stored.
*/
protected String apspInfoAttribute;
/**
* Attribute to store centroid information.
*/
protected String centroidAttribute;
/**
* Value of the attribute if node is in the centroid.
*/
protected Object isInCentroid;
/**
* Value of the attribute if node is not in the centroid.
*/
protected Object isNotInCentroid;
/**
* Build a new centroid algorithm with default parameters.
*/
public Centroid() {
this("centroid");
}
/**
* Build a new centroid algorithm, specifying the attribute name of the
* computation result
*
* @param centroidAttribute
* attribute name of the computation result.
*/
public Centroid(String centroidAttribute) {
this(centroidAttribute, Boolean.TRUE, Boolean.FALSE);
}
/**
* Build a new centroid as in {@link #Centroid(String)} but specifying
* values of centroid membership.
*
* @param centroidAttribute
* attribute name of the computation result.
* @param isInCentroid
* the value of elements centroid attribute when this element is
* in the centroid.
* @param isNotInCentroid
* the value of elements centroid attribute when this element is
* not in the centroid.
*/
public Centroid(String centroidAttribute, Object isInCentroid,
Object isNotInCentroid) {
this(centroidAttribute, Boolean.TRUE, Boolean.FALSE,
APSP.APSPInfo.ATTRIBUTE_NAME);
}
/**
* Build a new centroid algorithm as in
* {@link #Centroid(String, Object, Object)} but specifying the name of the
* attribute where the APSP informations are stored.
*
* @param centroidAttribute
* attribute name of the computation result.
* @param isInCentroid
* the value of elements centroid attribute when this element is
* in the centroid.
* @param isNotInCentroid
* the value of elements centroid attribute when this element is
* not in the centroid.
* @param apspInfoAttribute
* the name of the attribute where the APSP informations are
* stored
*/
public Centroid(String centroidAttribute, Object isInCentroid,
Object isNotInCentroid, String apspInfoAttribute) {
this.centroidAttribute = centroidAttribute;
this.isInCentroid = isInCentroid;
this.isNotInCentroid = isNotInCentroid;
this.apspInfoAttribute = apspInfoAttribute;
}
/*
* (non-Javadoc)
*
* @see
* org.graphstream.algorithm.Algorithm#init(org.graphstream.graph.Graph)
*/
public void init(Graph graph) {
this.graph = graph;
}
/*
* (non-Javadoc)
*
* @see org.graphstream.algorithm.Algorithm#compute()
*/
public void compute() {
float min = Float.MAX_VALUE;
HashSet centroid = new HashSet();
for (Node node : graph.getEachNode()) {
float m = 0;
APSP.APSPInfo info = node.getAttribute(apspInfoAttribute);
if (info == null)
System.err
.printf("APSPInfo missing. Did you compute APSP before ?\n");
for (Node other : graph.getEachNode()) {
if (node != other) {
double d = info.getLengthTo(other.getId());
if (d < 0)
System.err
.printf("Found a negative length value in centroid algorithm. "
+ "Is graph connected ?\n");
else
m += d;
}
}
if (m < min) {
centroid.clear();
centroid.add(node);
min = m;
} else if (m == min) {
centroid.add(node);
}
}
for (Node node : graph.getEachNode())
node.setAttribute(centroidAttribute,
centroid.contains(node) ? isInCentroid : isNotInCentroid);
centroid.clear();
}
/**
* Get the APSP info attribute name.
*
* @return the name of the attribute where the APSP informations are stored.
*/
public String getAPSPInfoAttribute() {
return apspInfoAttribute;
}
/**
* Set the APSP info attribute name.
*
* @param attribute
* the name of the attribute where the APSP informations are
* stored.
*/
public void setAPSPInfoAttribute(String attribute) {
apspInfoAttribute = attribute;
}
/**
* Get the value of the centroid attribute when element is in the centroid.
* Default value is Boolean.TRUE.
*
* @return the value of elements centroid attribute when this element is in
* the centroid.
*/
public Object getIsInCentroidValue() {
return isInCentroid;
}
/**
* Set the value of the centroid attribute when element is in the centroid.
* On computation, this value is used to set the centroid attribute.
*
* @param value
* the value of elements centroid attribute when this element is
* in the centroid.
*/
public void setIsInCentroidValue(Object value) {
isInCentroid = value;
}
/**
* Get the value of the centroid attribute when element is not in the
* centroid. Default value is Boolean.FALSE.
*
* @return the value of elements centroid attribute when this element is not
* in the centroid.
*/
public Object getIsNotInCentroidValue() {
return isNotInCentroid;
}
/**
* Set the value of the centroid attribute when element is not in the
* centroid. On computation, this value is used to set the centroid
* attribute.
*
* @param value
* the value of elements centroid attribute when this element is
* not in the centroid.
*/
public void setIsNotInCentroidValue(Object value) {
isNotInCentroid = value;
}
/**
* Get the name of the attribute where computation result is stored. Value
* of this attribute can be {@link #getIsInCentroidValue()} if the element
* is in the centroid, {@link #getIsNotInCentroidValue()} else.
*
* @return the centroid attribute name.
*/
public String getCentroidAttribute() {
return centroidAttribute;
}
/**
* Set the name of the attribute where computation result is stored.
*
* @param centroidAttribute
* the name of the element attribute where computation result is
* stored.
*/
public void setCentroidAttribute(String centroidAttribute) {
this.centroidAttribute = centroidAttribute;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy