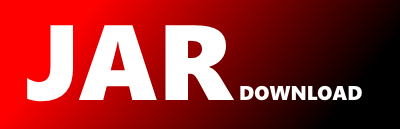
org.graphstream.algorithm.measure.SurpriseMeasure Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gs-algo Show documentation
Show all versions of gs-algo Show documentation
The GraphStream library. With GraphStream you deal with
graphs. Static and Dynamic. You create them from scratch, from a file
or any source. You display and render them. This package contains algorithms and generators.
/*
* Copyright 2006 - 2015
* Stefan Balev
* Julien Baudry
* Antoine Dutot
* Yoann Pigné
* Guilhelm Savin
*
* This file is part of GraphStream .
*
* GraphStream is a library whose purpose is to handle static or dynamic
* graph, create them from scratch, file or any source and display them.
*
* This program is free software distributed under the terms of two licenses, the
* CeCILL-C license that fits European law, and the GNU Lesser General Public
* License. You can use, modify and/ or redistribute the software under the terms
* of the CeCILL-C license as circulated by CEA, CNRS and INRIA at the following
* URL or under the terms of the GNU LGPL as published by
* the Free Software Foundation, either version 3 of the License, or (at your
* option) any later version.
*
* This program is distributed in the hope that it will be useful, but WITHOUT ANY
* WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS FOR A
* PARTICULAR PURPOSE. See the GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program. If not, see .
*
* The fact that you are presently reading this means that you have had
* knowledge of the CeCILL-C and LGPL licenses and that you accept their terms.
*/
package org.graphstream.algorithm.measure;
import java.util.ArrayList;
import java.util.HashMap;
import org.graphstream.algorithm.Algorithm;
import org.graphstream.graph.Edge;
import org.graphstream.graph.Graph;
/**
* Surprise measure.
*
* Description from Wikipedia :
* Surprise (denoted S) is a measure of community structure in complex networks.
* The name Surprise derives from the fact that its maximization finds the most
* surprising partition into communities of the network, that is, the most
* unlikely one. S accurately evaluates, in a global manner, the quality of a
* partition using a cumulative hypergeometric distribution.
*
* @reference Rodrigo Aldecoa, Ignacio Marin,
* "Deciphering Network Community Structure by Surprise", 2011, PLoS
* ONE 6(9)
*
*/
public class SurpriseMeasure implements Algorithm {
/**
* Default attribute key where the result of the algorithm, a double value,
* is stored.
*/
public static final String ATTRIBUTE = "measure.surprise";
//
// Used to group nodes with no meta index under a fake null index.
//
private static final Object NULL = new Object();
/**
* Attribute of nodes containing meta index. Default is "meta.index".
*/
protected String communityAttributeKey;
/**
* Attribute that will contain the result.
*/
protected String surpriseAttributeKey;
/**
* Graph used in the computation.
*/
protected Graph graph;
/**
* Default constructor.
*/
public SurpriseMeasure() {
this("meta.index");
}
/**
* Constructor allowing to set the node attribute key containing index of
* organizations.
*
* @param communityAttributeKey
* key attribute of organizations
*/
public SurpriseMeasure(String communityAttributeKey) {
this(communityAttributeKey, ATTRIBUTE);
}
/**
* Same as {@link #SurpriseMeasure(String)} but allowing to set the graph
* attribute that will contain the result of the computation.
*
* @param communityAttributeKey
* @param surpriseAttributeKey
*/
public SurpriseMeasure(String communityAttributeKey,
String surpriseAttributeKey) {
this.communityAttributeKey = communityAttributeKey;
this.surpriseAttributeKey = surpriseAttributeKey;
}
/*
* (non-Javadoc)
*
* @see
* org.graphstream.algorithm.Algorithm#init(org.graphstream.graph.Graph)
*/
public void init(Graph graph) {
this.graph = graph;
}
/*
* (non-Javadoc)
*
* @see org.graphstream.algorithm.Algorithm#compute()
*/
public void compute() {
HashMap
© 2015 - 2025 Weber Informatics LLC | Privacy Policy