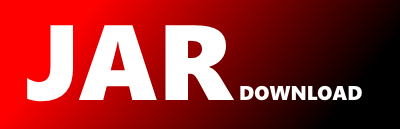
org.graphwalker.dsl.yed.YEdEdgeParser Maven / Gradle / Ivy
The newest version!
// Generated from org/graphwalker/dsl/yed/YEdEdgeParser.g4 by ANTLR 4.13.1
package org.graphwalker.dsl.yed;
import org.antlr.v4.runtime.atn.*;
import org.antlr.v4.runtime.dfa.DFA;
import org.antlr.v4.runtime.*;
import org.antlr.v4.runtime.misc.*;
import org.antlr.v4.runtime.tree.*;
import java.util.List;
import java.util.Iterator;
import java.util.ArrayList;
@SuppressWarnings({"all", "warnings", "unchecked", "unused", "cast", "CheckReturnValue"})
public class YEdEdgeParser extends Parser {
static { RuntimeMetaData.checkVersion("4.13.1", RuntimeMetaData.VERSION); }
protected static final DFA[] _decisionToDFA;
protected static final PredictionContextCache _sharedContextCache =
new PredictionContextCache();
public static final int
DOT=1, SLASH=2, COLON=3, SEMICOLON=4, COMMA=5, ASSIGN=6, BLOCKED=7, SHARED=8,
INIT=9, START=10, REQTAG=11, WEIGHT=12, DEPENDENCY=13, NestedBrackets=14,
Identifier=15, String=16, Value=17, COMMENT=18, LINE_COMMENT=19, WHITESPACE=20,
ANY=21;
public static final int
RULE_parse = 0, RULE_field = 1, RULE_actions = 2, RULE_action = 3, RULE_reqtags = 4,
RULE_reqtagList = 5, RULE_reqtag = 6, RULE_guard = 7, RULE_blocked = 8,
RULE_names = 9, RULE_name = 10, RULE_dependency = 11, RULE_weight = 12;
private static String[] makeRuleNames() {
return new String[] {
"parse", "field", "actions", "action", "reqtags", "reqtagList", "reqtag",
"guard", "blocked", "names", "name", "dependency", "weight"
};
}
public static final String[] ruleNames = makeRuleNames();
private static String[] makeLiteralNames() {
return new String[] {
null, "'.'", "'/'", "':'", "';'", "','", "'='", "'BLOCKED'", "'SHARED'",
"'INIT'", null, "'REQTAG'"
};
}
private static final String[] _LITERAL_NAMES = makeLiteralNames();
private static String[] makeSymbolicNames() {
return new String[] {
null, "DOT", "SLASH", "COLON", "SEMICOLON", "COMMA", "ASSIGN", "BLOCKED",
"SHARED", "INIT", "START", "REQTAG", "WEIGHT", "DEPENDENCY", "NestedBrackets",
"Identifier", "String", "Value", "COMMENT", "LINE_COMMENT", "WHITESPACE",
"ANY"
};
}
private static final String[] _SYMBOLIC_NAMES = makeSymbolicNames();
public static final Vocabulary VOCABULARY = new VocabularyImpl(_LITERAL_NAMES, _SYMBOLIC_NAMES);
/**
* @deprecated Use {@link #VOCABULARY} instead.
*/
@Deprecated
public static final String[] tokenNames;
static {
tokenNames = new String[_SYMBOLIC_NAMES.length];
for (int i = 0; i < tokenNames.length; i++) {
tokenNames[i] = VOCABULARY.getLiteralName(i);
if (tokenNames[i] == null) {
tokenNames[i] = VOCABULARY.getSymbolicName(i);
}
if (tokenNames[i] == null) {
tokenNames[i] = "";
}
}
}
@Override
@Deprecated
public String[] getTokenNames() {
return tokenNames;
}
@Override
public Vocabulary getVocabulary() {
return VOCABULARY;
}
@Override
public String getGrammarFileName() { return "YEdEdgeParser.g4"; }
@Override
public String[] getRuleNames() { return ruleNames; }
@Override
public String getSerializedATN() { return _serializedATN; }
@Override
public ATN getATN() { return _ATN; }
public YEdEdgeParser(TokenStream input) {
super(input);
_interp = new ParserATNSimulator(this,_ATN,_decisionToDFA,_sharedContextCache);
}
@SuppressWarnings("CheckReturnValue")
public static class ParseContext extends ParserRuleContext {
public java.util.Set fields = new java.util.HashSet();;
public TerminalNode EOF() { return getToken(YEdEdgeParser.EOF, 0); }
public List field() {
return getRuleContexts(FieldContext.class);
}
public FieldContext field(int i) {
return getRuleContext(FieldContext.class,i);
}
public ParseContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_parse; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof YEdEdgeParserListener ) ((YEdEdgeParserListener)listener).enterParse(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof YEdEdgeParserListener ) ((YEdEdgeParserListener)listener).exitParse(this);
}
}
public final ParseContext parse() throws RecognitionException {
ParseContext _localctx = new ParseContext(_ctx, getState());
enterRule(_localctx, 0, RULE_parse);
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(29);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,0,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(26);
field();
}
}
}
setState(31);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,0,_ctx);
}
setState(32);
match(EOF);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class FieldContext extends ParserRuleContext {
public NamesContext names() {
return getRuleContext(NamesContext.class,0);
}
public GuardContext guard() {
return getRuleContext(GuardContext.class,0);
}
public ActionsContext actions() {
return getRuleContext(ActionsContext.class,0);
}
public BlockedContext blocked() {
return getRuleContext(BlockedContext.class,0);
}
public ReqtagsContext reqtags() {
return getRuleContext(ReqtagsContext.class,0);
}
public WeightContext weight() {
return getRuleContext(WeightContext.class,0);
}
public DependencyContext dependency() {
return getRuleContext(DependencyContext.class,0);
}
public TerminalNode WHITESPACE() { return getToken(YEdEdgeParser.WHITESPACE, 0); }
public FieldContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_field; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof YEdEdgeParserListener ) ((YEdEdgeParserListener)listener).enterField(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof YEdEdgeParserListener ) ((YEdEdgeParserListener)listener).exitField(this);
}
}
public final FieldContext field() throws RecognitionException {
FieldContext _localctx = new FieldContext(_ctx, getState());
enterRule(_localctx, 2, RULE_field);
try {
setState(63);
_errHandler.sync(this);
switch ( getInterpreter().adaptivePredict(_input,1,_ctx) ) {
case 1:
enterOuterAlt(_localctx, 1);
{
setState(34);
if (!(!((ParseContext)getInvokingContext(0)).fields.contains("names"))) throw new FailedPredicateException(this, "!$parse::fields.contains(\"names\")");
setState(35);
names();
((ParseContext)getInvokingContext(0)).fields.add("names");
}
break;
case 2:
enterOuterAlt(_localctx, 2);
{
setState(38);
if (!(!((ParseContext)getInvokingContext(0)).fields.contains("guard"))) throw new FailedPredicateException(this, "!$parse::fields.contains(\"guard\")");
setState(39);
guard();
((ParseContext)getInvokingContext(0)).fields.add("guard");
}
break;
case 3:
enterOuterAlt(_localctx, 3);
{
setState(42);
if (!(!((ParseContext)getInvokingContext(0)).fields.contains("actions"))) throw new FailedPredicateException(this, "!$parse::fields.contains(\"actions\")");
setState(43);
actions();
((ParseContext)getInvokingContext(0)).fields.add("actions");
}
break;
case 4:
enterOuterAlt(_localctx, 4);
{
setState(46);
if (!(!((ParseContext)getInvokingContext(0)).fields.contains("blocked"))) throw new FailedPredicateException(this, "!$parse::fields.contains(\"blocked\")");
setState(47);
blocked();
((ParseContext)getInvokingContext(0)).fields.add("blocked");
}
break;
case 5:
enterOuterAlt(_localctx, 5);
{
setState(50);
if (!(!((ParseContext)getInvokingContext(0)).fields.contains("reqtags"))) throw new FailedPredicateException(this, "!$parse::fields.contains(\"reqtags\")");
setState(51);
reqtags();
((ParseContext)getInvokingContext(0)).fields.add("reqtags");
}
break;
case 6:
enterOuterAlt(_localctx, 6);
{
setState(54);
if (!(!((ParseContext)getInvokingContext(0)).fields.contains("weight"))) throw new FailedPredicateException(this, "!$parse::fields.contains(\"weight\")");
setState(55);
weight();
((ParseContext)getInvokingContext(0)).fields.add("weight");
}
break;
case 7:
enterOuterAlt(_localctx, 7);
{
setState(58);
if (!(!((ParseContext)getInvokingContext(0)).fields.contains("dependency"))) throw new FailedPredicateException(this, "!$parse::fields.contains(\"dependency\")");
setState(59);
dependency();
((ParseContext)getInvokingContext(0)).fields.add("dependency");
}
break;
case 8:
enterOuterAlt(_localctx, 8);
{
setState(62);
match(WHITESPACE);
}
break;
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ActionsContext extends ParserRuleContext {
public TerminalNode SLASH() { return getToken(YEdEdgeParser.SLASH, 0); }
public List action() {
return getRuleContexts(ActionContext.class);
}
public ActionContext action(int i) {
return getRuleContext(ActionContext.class,i);
}
public ActionsContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_actions; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof YEdEdgeParserListener ) ((YEdEdgeParserListener)listener).enterActions(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof YEdEdgeParserListener ) ((YEdEdgeParserListener)listener).exitActions(this);
}
}
public final ActionsContext actions() throws RecognitionException {
ActionsContext _localctx = new ActionsContext(_ctx, getState());
enterRule(_localctx, 4, RULE_actions);
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(65);
match(SLASH);
setState(67);
_errHandler.sync(this);
_alt = 1;
do {
switch (_alt) {
case 1:
{
{
setState(66);
action();
}
}
break;
default:
throw new NoViableAltException(this);
}
setState(69);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,2,_ctx);
} while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER );
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ActionContext extends ParserRuleContext {
public TerminalNode SEMICOLON() { return getToken(YEdEdgeParser.SEMICOLON, 0); }
public ActionContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_action; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof YEdEdgeParserListener ) ((YEdEdgeParserListener)listener).enterAction(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof YEdEdgeParserListener ) ((YEdEdgeParserListener)listener).exitAction(this);
}
}
public final ActionContext action() throws RecognitionException {
ActionContext _localctx = new ActionContext(_ctx, getState());
enterRule(_localctx, 6, RULE_action);
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(72);
_errHandler.sync(this);
_alt = 1;
do {
switch (_alt) {
case 1:
{
{
setState(71);
matchWildcard();
}
}
break;
default:
throw new NoViableAltException(this);
}
setState(74);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,3,_ctx);
} while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER );
setState(76);
match(SEMICOLON);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ReqtagsContext extends ParserRuleContext {
public TerminalNode REQTAG() { return getToken(YEdEdgeParser.REQTAG, 0); }
public ReqtagListContext reqtagList() {
return getRuleContext(ReqtagListContext.class,0);
}
public TerminalNode COLON() { return getToken(YEdEdgeParser.COLON, 0); }
public TerminalNode ASSIGN() { return getToken(YEdEdgeParser.ASSIGN, 0); }
public List WHITESPACE() { return getTokens(YEdEdgeParser.WHITESPACE); }
public TerminalNode WHITESPACE(int i) {
return getToken(YEdEdgeParser.WHITESPACE, i);
}
public ReqtagsContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_reqtags; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof YEdEdgeParserListener ) ((YEdEdgeParserListener)listener).enterReqtags(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof YEdEdgeParserListener ) ((YEdEdgeParserListener)listener).exitReqtags(this);
}
}
public final ReqtagsContext reqtags() throws RecognitionException {
ReqtagsContext _localctx = new ReqtagsContext(_ctx, getState());
enterRule(_localctx, 8, RULE_reqtags);
int _la;
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(78);
match(REQTAG);
setState(82);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==WHITESPACE) {
{
{
setState(79);
match(WHITESPACE);
}
}
setState(84);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(85);
_la = _input.LA(1);
if ( !(_la==COLON || _la==ASSIGN) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
setState(89);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,5,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(86);
match(WHITESPACE);
}
}
}
setState(91);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,5,_ctx);
}
setState(92);
reqtagList();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ReqtagListContext extends ParserRuleContext {
public List reqtag() {
return getRuleContexts(ReqtagContext.class);
}
public ReqtagContext reqtag(int i) {
return getRuleContext(ReqtagContext.class,i);
}
public List COMMA() { return getTokens(YEdEdgeParser.COMMA); }
public TerminalNode COMMA(int i) {
return getToken(YEdEdgeParser.COMMA, i);
}
public List WHITESPACE() { return getTokens(YEdEdgeParser.WHITESPACE); }
public TerminalNode WHITESPACE(int i) {
return getToken(YEdEdgeParser.WHITESPACE, i);
}
public ReqtagListContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_reqtagList; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof YEdEdgeParserListener ) ((YEdEdgeParserListener)listener).enterReqtagList(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof YEdEdgeParserListener ) ((YEdEdgeParserListener)listener).exitReqtagList(this);
}
}
public final ReqtagListContext reqtagList() throws RecognitionException {
ReqtagListContext _localctx = new ReqtagListContext(_ctx, getState());
enterRule(_localctx, 10, RULE_reqtagList);
int _la;
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(110);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,8,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(94);
reqtag();
setState(98);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==WHITESPACE) {
{
{
setState(95);
match(WHITESPACE);
}
}
setState(100);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(101);
match(COMMA);
setState(105);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,7,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(102);
match(WHITESPACE);
}
}
}
setState(107);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,7,_ctx);
}
}
}
}
setState(112);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,8,_ctx);
}
setState(113);
reqtag();
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class ReqtagContext extends ParserRuleContext {
public List COMMA() { return getTokens(YEdEdgeParser.COMMA); }
public TerminalNode COMMA(int i) {
return getToken(YEdEdgeParser.COMMA, i);
}
public ReqtagContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_reqtag; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof YEdEdgeParserListener ) ((YEdEdgeParserListener)listener).enterReqtag(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof YEdEdgeParserListener ) ((YEdEdgeParserListener)listener).exitReqtag(this);
}
}
public final ReqtagContext reqtag() throws RecognitionException {
ReqtagContext _localctx = new ReqtagContext(_ctx, getState());
enterRule(_localctx, 12, RULE_reqtag);
int _la;
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(116);
_errHandler.sync(this);
_alt = 1;
do {
switch (_alt) {
case 1:
{
{
setState(115);
_la = _input.LA(1);
if ( _la <= 0 || (_la==COMMA) ) {
_errHandler.recoverInline(this);
}
else {
if ( _input.LA(1)==Token.EOF ) matchedEOF = true;
_errHandler.reportMatch(this);
consume();
}
}
}
break;
default:
throw new NoViableAltException(this);
}
setState(118);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,9,_ctx);
} while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER );
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class GuardContext extends ParserRuleContext {
public TerminalNode NestedBrackets() { return getToken(YEdEdgeParser.NestedBrackets, 0); }
public GuardContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_guard; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof YEdEdgeParserListener ) ((YEdEdgeParserListener)listener).enterGuard(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof YEdEdgeParserListener ) ((YEdEdgeParserListener)listener).exitGuard(this);
}
}
public final GuardContext guard() throws RecognitionException {
GuardContext _localctx = new GuardContext(_ctx, getState());
enterRule(_localctx, 14, RULE_guard);
try {
enterOuterAlt(_localctx, 1);
{
setState(120);
match(NestedBrackets);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class BlockedContext extends ParserRuleContext {
public TerminalNode BLOCKED() { return getToken(YEdEdgeParser.BLOCKED, 0); }
public BlockedContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_blocked; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof YEdEdgeParserListener ) ((YEdEdgeParserListener)listener).enterBlocked(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof YEdEdgeParserListener ) ((YEdEdgeParserListener)listener).exitBlocked(this);
}
}
public final BlockedContext blocked() throws RecognitionException {
BlockedContext _localctx = new BlockedContext(_ctx, getState());
enterRule(_localctx, 16, RULE_blocked);
try {
enterOuterAlt(_localctx, 1);
{
setState(122);
match(BLOCKED);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class NamesContext extends ParserRuleContext {
public List name() {
return getRuleContexts(NameContext.class);
}
public NameContext name(int i) {
return getRuleContext(NameContext.class,i);
}
public List SEMICOLON() { return getTokens(YEdEdgeParser.SEMICOLON); }
public TerminalNode SEMICOLON(int i) {
return getToken(YEdEdgeParser.SEMICOLON, i);
}
public NamesContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_names; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof YEdEdgeParserListener ) ((YEdEdgeParserListener)listener).enterNames(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof YEdEdgeParserListener ) ((YEdEdgeParserListener)listener).exitNames(this);
}
}
public final NamesContext names() throws RecognitionException {
NamesContext _localctx = new NamesContext(_ctx, getState());
enterRule(_localctx, 18, RULE_names);
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(124);
name();
setState(129);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,10,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(125);
match(SEMICOLON);
setState(126);
name();
}
}
}
setState(131);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,10,_ctx);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class NameContext extends ParserRuleContext {
public List Identifier() { return getTokens(YEdEdgeParser.Identifier); }
public TerminalNode Identifier(int i) {
return getToken(YEdEdgeParser.Identifier, i);
}
public List DOT() { return getTokens(YEdEdgeParser.DOT); }
public TerminalNode DOT(int i) {
return getToken(YEdEdgeParser.DOT, i);
}
public NameContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_name; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof YEdEdgeParserListener ) ((YEdEdgeParserListener)listener).enterName(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof YEdEdgeParserListener ) ((YEdEdgeParserListener)listener).exitName(this);
}
}
public final NameContext name() throws RecognitionException {
NameContext _localctx = new NameContext(_ctx, getState());
enterRule(_localctx, 20, RULE_name);
try {
int _alt;
enterOuterAlt(_localctx, 1);
{
setState(132);
match(Identifier);
setState(137);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,11,_ctx);
while ( _alt!=2 && _alt!=org.antlr.v4.runtime.atn.ATN.INVALID_ALT_NUMBER ) {
if ( _alt==1 ) {
{
{
setState(133);
match(DOT);
setState(134);
match(Identifier);
}
}
}
setState(139);
_errHandler.sync(this);
_alt = getInterpreter().adaptivePredict(_input,11,_ctx);
}
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class DependencyContext extends ParserRuleContext {
public TerminalNode DEPENDENCY() { return getToken(YEdEdgeParser.DEPENDENCY, 0); }
public TerminalNode ASSIGN() { return getToken(YEdEdgeParser.ASSIGN, 0); }
public TerminalNode Value() { return getToken(YEdEdgeParser.Value, 0); }
public List WHITESPACE() { return getTokens(YEdEdgeParser.WHITESPACE); }
public TerminalNode WHITESPACE(int i) {
return getToken(YEdEdgeParser.WHITESPACE, i);
}
public DependencyContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_dependency; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof YEdEdgeParserListener ) ((YEdEdgeParserListener)listener).enterDependency(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof YEdEdgeParserListener ) ((YEdEdgeParserListener)listener).exitDependency(this);
}
}
public final DependencyContext dependency() throws RecognitionException {
DependencyContext _localctx = new DependencyContext(_ctx, getState());
enterRule(_localctx, 22, RULE_dependency);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(140);
match(DEPENDENCY);
setState(144);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==WHITESPACE) {
{
{
setState(141);
match(WHITESPACE);
}
}
setState(146);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(147);
match(ASSIGN);
setState(151);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==WHITESPACE) {
{
{
setState(148);
match(WHITESPACE);
}
}
setState(153);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(154);
match(Value);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
@SuppressWarnings("CheckReturnValue")
public static class WeightContext extends ParserRuleContext {
public TerminalNode WEIGHT() { return getToken(YEdEdgeParser.WEIGHT, 0); }
public TerminalNode ASSIGN() { return getToken(YEdEdgeParser.ASSIGN, 0); }
public TerminalNode Value() { return getToken(YEdEdgeParser.Value, 0); }
public List WHITESPACE() { return getTokens(YEdEdgeParser.WHITESPACE); }
public TerminalNode WHITESPACE(int i) {
return getToken(YEdEdgeParser.WHITESPACE, i);
}
public WeightContext(ParserRuleContext parent, int invokingState) {
super(parent, invokingState);
}
@Override public int getRuleIndex() { return RULE_weight; }
@Override
public void enterRule(ParseTreeListener listener) {
if ( listener instanceof YEdEdgeParserListener ) ((YEdEdgeParserListener)listener).enterWeight(this);
}
@Override
public void exitRule(ParseTreeListener listener) {
if ( listener instanceof YEdEdgeParserListener ) ((YEdEdgeParserListener)listener).exitWeight(this);
}
}
public final WeightContext weight() throws RecognitionException {
WeightContext _localctx = new WeightContext(_ctx, getState());
enterRule(_localctx, 24, RULE_weight);
int _la;
try {
enterOuterAlt(_localctx, 1);
{
setState(156);
match(WEIGHT);
setState(160);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==WHITESPACE) {
{
{
setState(157);
match(WHITESPACE);
}
}
setState(162);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(163);
match(ASSIGN);
setState(167);
_errHandler.sync(this);
_la = _input.LA(1);
while (_la==WHITESPACE) {
{
{
setState(164);
match(WHITESPACE);
}
}
setState(169);
_errHandler.sync(this);
_la = _input.LA(1);
}
setState(170);
match(Value);
}
}
catch (RecognitionException re) {
_localctx.exception = re;
_errHandler.reportError(this, re);
_errHandler.recover(this, re);
}
finally {
exitRule();
}
return _localctx;
}
public boolean sempred(RuleContext _localctx, int ruleIndex, int predIndex) {
switch (ruleIndex) {
case 1:
return field_sempred((FieldContext)_localctx, predIndex);
}
return true;
}
private boolean field_sempred(FieldContext _localctx, int predIndex) {
switch (predIndex) {
case 0:
return !((ParseContext)getInvokingContext(0)).fields.contains("names");
case 1:
return !((ParseContext)getInvokingContext(0)).fields.contains("guard");
case 2:
return !((ParseContext)getInvokingContext(0)).fields.contains("actions");
case 3:
return !((ParseContext)getInvokingContext(0)).fields.contains("blocked");
case 4:
return !((ParseContext)getInvokingContext(0)).fields.contains("reqtags");
case 5:
return !((ParseContext)getInvokingContext(0)).fields.contains("weight");
case 6:
return !((ParseContext)getInvokingContext(0)).fields.contains("dependency");
}
return true;
}
public static final String _serializedATN =
"\u0004\u0001\u0015\u00ad\u0002\u0000\u0007\u0000\u0002\u0001\u0007\u0001"+
"\u0002\u0002\u0007\u0002\u0002\u0003\u0007\u0003\u0002\u0004\u0007\u0004"+
"\u0002\u0005\u0007\u0005\u0002\u0006\u0007\u0006\u0002\u0007\u0007\u0007"+
"\u0002\b\u0007\b\u0002\t\u0007\t\u0002\n\u0007\n\u0002\u000b\u0007\u000b"+
"\u0002\f\u0007\f\u0001\u0000\u0005\u0000\u001c\b\u0000\n\u0000\f\u0000"+
"\u001f\t\u0000\u0001\u0000\u0001\u0000\u0001\u0001\u0001\u0001\u0001\u0001"+
"\u0001\u0001\u0001\u0001\u0001\u0001\u0001\u0001\u0001\u0001\u0001\u0001"+
"\u0001\u0001\u0001\u0001\u0001\u0001\u0001\u0001\u0001\u0001\u0001\u0001"+
"\u0001\u0001\u0001\u0001\u0001\u0001\u0001\u0001\u0001\u0001\u0001\u0001"+
"\u0001\u0001\u0001\u0001\u0001\u0001\u0001\u0001\u0001\u0001\u0001\u0001"+
"\u0001\u0001\u0001\u0001\u0003\u0001@\b\u0001\u0001\u0002\u0001\u0002"+
"\u0004\u0002D\b\u0002\u000b\u0002\f\u0002E\u0001\u0003\u0004\u0003I\b"+
"\u0003\u000b\u0003\f\u0003J\u0001\u0003\u0001\u0003\u0001\u0004\u0001"+
"\u0004\u0005\u0004Q\b\u0004\n\u0004\f\u0004T\t\u0004\u0001\u0004\u0001"+
"\u0004\u0005\u0004X\b\u0004\n\u0004\f\u0004[\t\u0004\u0001\u0004\u0001"+
"\u0004\u0001\u0005\u0001\u0005\u0005\u0005a\b\u0005\n\u0005\f\u0005d\t"+
"\u0005\u0001\u0005\u0001\u0005\u0005\u0005h\b\u0005\n\u0005\f\u0005k\t"+
"\u0005\u0005\u0005m\b\u0005\n\u0005\f\u0005p\t\u0005\u0001\u0005\u0001"+
"\u0005\u0001\u0006\u0004\u0006u\b\u0006\u000b\u0006\f\u0006v\u0001\u0007"+
"\u0001\u0007\u0001\b\u0001\b\u0001\t\u0001\t\u0001\t\u0005\t\u0080\b\t"+
"\n\t\f\t\u0083\t\t\u0001\n\u0001\n\u0001\n\u0005\n\u0088\b\n\n\n\f\n\u008b"+
"\t\n\u0001\u000b\u0001\u000b\u0005\u000b\u008f\b\u000b\n\u000b\f\u000b"+
"\u0092\t\u000b\u0001\u000b\u0001\u000b\u0005\u000b\u0096\b\u000b\n\u000b"+
"\f\u000b\u0099\t\u000b\u0001\u000b\u0001\u000b\u0001\f\u0001\f\u0005\f"+
"\u009f\b\f\n\f\f\f\u00a2\t\f\u0001\f\u0001\f\u0005\f\u00a6\b\f\n\f\f\f"+
"\u00a9\t\f\u0001\f\u0001\f\u0001\f\u0000\u0000\r\u0000\u0002\u0004\u0006"+
"\b\n\f\u000e\u0010\u0012\u0014\u0016\u0018\u0000\u0002\u0002\u0000\u0003"+
"\u0003\u0006\u0006\u0001\u0000\u0005\u0005\u00b5\u0000\u001d\u0001\u0000"+
"\u0000\u0000\u0002?\u0001\u0000\u0000\u0000\u0004A\u0001\u0000\u0000\u0000"+
"\u0006H\u0001\u0000\u0000\u0000\bN\u0001\u0000\u0000\u0000\nn\u0001\u0000"+
"\u0000\u0000\ft\u0001\u0000\u0000\u0000\u000ex\u0001\u0000\u0000\u0000"+
"\u0010z\u0001\u0000\u0000\u0000\u0012|\u0001\u0000\u0000\u0000\u0014\u0084"+
"\u0001\u0000\u0000\u0000\u0016\u008c\u0001\u0000\u0000\u0000\u0018\u009c"+
"\u0001\u0000\u0000\u0000\u001a\u001c\u0003\u0002\u0001\u0000\u001b\u001a"+
"\u0001\u0000\u0000\u0000\u001c\u001f\u0001\u0000\u0000\u0000\u001d\u001b"+
"\u0001\u0000\u0000\u0000\u001d\u001e\u0001\u0000\u0000\u0000\u001e \u0001"+
"\u0000\u0000\u0000\u001f\u001d\u0001\u0000\u0000\u0000 !\u0005\u0000\u0000"+
"\u0001!\u0001\u0001\u0000\u0000\u0000\"#\u0004\u0001\u0000\u0001#$\u0003"+
"\u0012\t\u0000$%\u0006\u0001\uffff\uffff\u0000%@\u0001\u0000\u0000\u0000"+
"&\'\u0004\u0001\u0001\u0001\'(\u0003\u000e\u0007\u0000()\u0006\u0001\uffff"+
"\uffff\u0000)@\u0001\u0000\u0000\u0000*+\u0004\u0001\u0002\u0001+,\u0003"+
"\u0004\u0002\u0000,-\u0006\u0001\uffff\uffff\u0000-@\u0001\u0000\u0000"+
"\u0000./\u0004\u0001\u0003\u0001/0\u0003\u0010\b\u000001\u0006\u0001\uffff"+
"\uffff\u00001@\u0001\u0000\u0000\u000023\u0004\u0001\u0004\u000134\u0003"+
"\b\u0004\u000045\u0006\u0001\uffff\uffff\u00005@\u0001\u0000\u0000\u0000"+
"67\u0004\u0001\u0005\u000178\u0003\u0018\f\u000089\u0006\u0001\uffff\uffff"+
"\u00009@\u0001\u0000\u0000\u0000:;\u0004\u0001\u0006\u0001;<\u0003\u0016"+
"\u000b\u0000<=\u0006\u0001\uffff\uffff\u0000=@\u0001\u0000\u0000\u0000"+
">@\u0005\u0014\u0000\u0000?\"\u0001\u0000\u0000\u0000?&\u0001\u0000\u0000"+
"\u0000?*\u0001\u0000\u0000\u0000?.\u0001\u0000\u0000\u0000?2\u0001\u0000"+
"\u0000\u0000?6\u0001\u0000\u0000\u0000?:\u0001\u0000\u0000\u0000?>\u0001"+
"\u0000\u0000\u0000@\u0003\u0001\u0000\u0000\u0000AC\u0005\u0002\u0000"+
"\u0000BD\u0003\u0006\u0003\u0000CB\u0001\u0000\u0000\u0000DE\u0001\u0000"+
"\u0000\u0000EC\u0001\u0000\u0000\u0000EF\u0001\u0000\u0000\u0000F\u0005"+
"\u0001\u0000\u0000\u0000GI\t\u0000\u0000\u0000HG\u0001\u0000\u0000\u0000"+
"IJ\u0001\u0000\u0000\u0000JH\u0001\u0000\u0000\u0000JK\u0001\u0000\u0000"+
"\u0000KL\u0001\u0000\u0000\u0000LM\u0005\u0004\u0000\u0000M\u0007\u0001"+
"\u0000\u0000\u0000NR\u0005\u000b\u0000\u0000OQ\u0005\u0014\u0000\u0000"+
"PO\u0001\u0000\u0000\u0000QT\u0001\u0000\u0000\u0000RP\u0001\u0000\u0000"+
"\u0000RS\u0001\u0000\u0000\u0000SU\u0001\u0000\u0000\u0000TR\u0001\u0000"+
"\u0000\u0000UY\u0007\u0000\u0000\u0000VX\u0005\u0014\u0000\u0000WV\u0001"+
"\u0000\u0000\u0000X[\u0001\u0000\u0000\u0000YW\u0001\u0000\u0000\u0000"+
"YZ\u0001\u0000\u0000\u0000Z\\\u0001\u0000\u0000\u0000[Y\u0001\u0000\u0000"+
"\u0000\\]\u0003\n\u0005\u0000]\t\u0001\u0000\u0000\u0000^b\u0003\f\u0006"+
"\u0000_a\u0005\u0014\u0000\u0000`_\u0001\u0000\u0000\u0000ad\u0001\u0000"+
"\u0000\u0000b`\u0001\u0000\u0000\u0000bc\u0001\u0000\u0000\u0000ce\u0001"+
"\u0000\u0000\u0000db\u0001\u0000\u0000\u0000ei\u0005\u0005\u0000\u0000"+
"fh\u0005\u0014\u0000\u0000gf\u0001\u0000\u0000\u0000hk\u0001\u0000\u0000"+
"\u0000ig\u0001\u0000\u0000\u0000ij\u0001\u0000\u0000\u0000jm\u0001\u0000"+
"\u0000\u0000ki\u0001\u0000\u0000\u0000l^\u0001\u0000\u0000\u0000mp\u0001"+
"\u0000\u0000\u0000nl\u0001\u0000\u0000\u0000no\u0001\u0000\u0000\u0000"+
"oq\u0001\u0000\u0000\u0000pn\u0001\u0000\u0000\u0000qr\u0003\f\u0006\u0000"+
"r\u000b\u0001\u0000\u0000\u0000su\b\u0001\u0000\u0000ts\u0001\u0000\u0000"+
"\u0000uv\u0001\u0000\u0000\u0000vt\u0001\u0000\u0000\u0000vw\u0001\u0000"+
"\u0000\u0000w\r\u0001\u0000\u0000\u0000xy\u0005\u000e\u0000\u0000y\u000f"+
"\u0001\u0000\u0000\u0000z{\u0005\u0007\u0000\u0000{\u0011\u0001\u0000"+
"\u0000\u0000|\u0081\u0003\u0014\n\u0000}~\u0005\u0004\u0000\u0000~\u0080"+
"\u0003\u0014\n\u0000\u007f}\u0001\u0000\u0000\u0000\u0080\u0083\u0001"+
"\u0000\u0000\u0000\u0081\u007f\u0001\u0000\u0000\u0000\u0081\u0082\u0001"+
"\u0000\u0000\u0000\u0082\u0013\u0001\u0000\u0000\u0000\u0083\u0081\u0001"+
"\u0000\u0000\u0000\u0084\u0089\u0005\u000f\u0000\u0000\u0085\u0086\u0005"+
"\u0001\u0000\u0000\u0086\u0088\u0005\u000f\u0000\u0000\u0087\u0085\u0001"+
"\u0000\u0000\u0000\u0088\u008b\u0001\u0000\u0000\u0000\u0089\u0087\u0001"+
"\u0000\u0000\u0000\u0089\u008a\u0001\u0000\u0000\u0000\u008a\u0015\u0001"+
"\u0000\u0000\u0000\u008b\u0089\u0001\u0000\u0000\u0000\u008c\u0090\u0005"+
"\r\u0000\u0000\u008d\u008f\u0005\u0014\u0000\u0000\u008e\u008d\u0001\u0000"+
"\u0000\u0000\u008f\u0092\u0001\u0000\u0000\u0000\u0090\u008e\u0001\u0000"+
"\u0000\u0000\u0090\u0091\u0001\u0000\u0000\u0000\u0091\u0093\u0001\u0000"+
"\u0000\u0000\u0092\u0090\u0001\u0000\u0000\u0000\u0093\u0097\u0005\u0006"+
"\u0000\u0000\u0094\u0096\u0005\u0014\u0000\u0000\u0095\u0094\u0001\u0000"+
"\u0000\u0000\u0096\u0099\u0001\u0000\u0000\u0000\u0097\u0095\u0001\u0000"+
"\u0000\u0000\u0097\u0098\u0001\u0000\u0000\u0000\u0098\u009a\u0001\u0000"+
"\u0000\u0000\u0099\u0097\u0001\u0000\u0000\u0000\u009a\u009b\u0005\u0011"+
"\u0000\u0000\u009b\u0017\u0001\u0000\u0000\u0000\u009c\u00a0\u0005\f\u0000"+
"\u0000\u009d\u009f\u0005\u0014\u0000\u0000\u009e\u009d\u0001\u0000\u0000"+
"\u0000\u009f\u00a2\u0001\u0000\u0000\u0000\u00a0\u009e\u0001\u0000\u0000"+
"\u0000\u00a0\u00a1\u0001\u0000\u0000\u0000\u00a1\u00a3\u0001\u0000\u0000"+
"\u0000\u00a2\u00a0\u0001\u0000\u0000\u0000\u00a3\u00a7\u0005\u0006\u0000"+
"\u0000\u00a4\u00a6\u0005\u0014\u0000\u0000\u00a5\u00a4\u0001\u0000\u0000"+
"\u0000\u00a6\u00a9\u0001\u0000\u0000\u0000\u00a7\u00a5\u0001\u0000\u0000"+
"\u0000\u00a7\u00a8\u0001\u0000\u0000\u0000\u00a8\u00aa\u0001\u0000\u0000"+
"\u0000\u00a9\u00a7\u0001\u0000\u0000\u0000\u00aa\u00ab\u0005\u0011\u0000"+
"\u0000\u00ab\u0019\u0001\u0000\u0000\u0000\u0010\u001d?EJRYbinv\u0081"+
"\u0089\u0090\u0097\u00a0\u00a7";
public static final ATN _ATN =
new ATNDeserializer().deserialize(_serializedATN.toCharArray());
static {
_decisionToDFA = new DFA[_ATN.getNumberOfDecisions()];
for (int i = 0; i < _ATN.getNumberOfDecisions(); i++) {
_decisionToDFA[i] = new DFA(_ATN.getDecisionState(i), i);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy