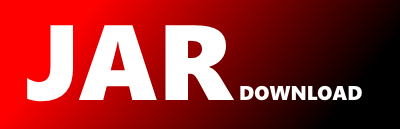
org.graylog2.restclient.models.Extractor Maven / Gradle / Ivy
/**
* This file is part of Graylog.
*
* Graylog is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* Graylog is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with Graylog. If not, see .
*/
package org.graylog2.restclient.models;
import com.google.common.annotations.VisibleForTesting;
import com.google.common.collect.Lists;
import com.google.common.collect.Maps;
import com.google.inject.assistedinject.Assisted;
import com.google.inject.assistedinject.AssistedInject;
import org.graylog2.rest.models.system.inputs.extractors.requests.CreateExtractorRequest;
import org.graylog2.rest.models.system.inputs.extractors.responses.ExtractorSummary;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.util.Collections;
import java.util.List;
import java.util.Map;
import static com.google.common.base.Preconditions.checkNotNull;
public class Extractor {
private static final Logger LOG = LoggerFactory.getLogger(Extractor.class);
public interface Factory {
Extractor fromResponse(ExtractorSummary es);
Extractor forCreate(CursorStrategy cursorStrategy,
@Assisted("title") String title,
@Assisted("sourceField") String sourceField,
@Assisted("targetField") String targetField,
Type type,
User creatorUser,
ConditionType conditionType,
@Assisted("conditionValue") String conditionValue);
}
public enum Type {
SUBSTRING("Substring"),
REGEX("Regular expression"),
REGEX_REPLACE("Replace with regular expression"),
SPLIT_AND_INDEX("Split & Index"),
COPY_INPUT("Copy Input"),
GROK("Grok pattern"),
JSON("JSON");
private final String description;
Type(String description) {
this.description = description;
}
public String toHumanReadable() {
return description;
}
public static Type fromString(String name) {
return valueOf(name.toUpperCase());
}
}
public enum CursorStrategy {
CUT,
COPY;
public static CursorStrategy fromString(String name) {
return valueOf(name.toUpperCase());
}
}
public enum ConditionType {
NONE,
STRING,
REGEX;
public static ConditionType fromString(String name) {
return valueOf(name.toUpperCase());
}
}
private String id;
private final String title;
private final CursorStrategy cursorStrategy;
private final Type extractorType;
private final String sourceField;
private final String targetField;
private final User creatorUser;
private final Map extractorConfig;
private final List converters;
private final ConditionType conditionType;
private final String conditionValue;
private final ExtractorMetrics metrics;
private final long exceptions;
private final long converterExceptions;
private long order;
@AssistedInject
private Extractor(UserService userService, @Assisted ExtractorSummary es) {
this.id = es.id();
this.title = es.title();
this.cursorStrategy = CursorStrategy.fromString(es.cursorStrategy());
this.sourceField = es.sourceField();
this.targetField = es.targetField();
this.extractorType = Type.fromString(es.type());
this.creatorUser = userService.load(es.creatorUserId());
this.extractorConfig = es.extractorConfig();
this.converters = buildConverterList(es.converters());
this.conditionType = ConditionType.fromString(es.conditionType());
this.conditionValue = es.conditionValue();
this.metrics = new ExtractorMetrics(es.metrics().total(), es.metrics().converters());
this.exceptions = es.exceptions();
this.converterExceptions = es.converterExceptions();
this.order = es.order();
}
@VisibleForTesting
@AssistedInject
Extractor(@Assisted CursorStrategy cursorStrategy,
@Assisted("title") String title,
@Assisted("sourceField") String sourceField,
@Assisted("targetField") String targetField,
@Assisted Type type,
@Assisted User creatorUser,
@Assisted ConditionType conditionType,
@Assisted("conditionValue") String conditionValue) {
this.id = null;
this.title = title;
this.cursorStrategy = cursorStrategy;
this.sourceField = sourceField;
this.targetField = targetField;
this.extractorType = type;
this.extractorConfig = Maps.newHashMap();
this.creatorUser = creatorUser;
this.converters = Lists.newArrayList();
this.conditionType = conditionType;
this.conditionValue = conditionValue;
this.metrics = null;
this.exceptions = 0;
this.converterExceptions = 0;
this.order = 0;
}
public CreateExtractorRequest toCreateExtractorRequest() {
final Map> converterList = Maps.newHashMap();
for (Converter converter : converters) {
converterList.put(converter.getType(), converter.getConfig());
}
final CreateExtractorRequest request = CreateExtractorRequest.create(title, cursorStrategy.toString().toLowerCase(), sourceField, targetField,
extractorType.toString().toLowerCase(), extractorConfig, converterList, conditionType.toString().toLowerCase(), conditionValue, order);
return request;
}
public void loadConfigFromForm(Type extractorType, Map form) {
switch (extractorType) {
case REGEX:
loadRegexConfig(form);
break;
case SUBSTRING:
loadSubstringConfig(form);
break;
case SPLIT_AND_INDEX:
loadSplitAndIndexConfig(form);
break;
case REGEX_REPLACE:
loadRegexReplaceConfig(form);
break;
case GROK:
loadGrokConfig(form);
break;
case JSON:
loadJsonConfig(form);
break;
}
}
public void loadConfigFromImport(Type type, Map extractorConfig) {
checkNotNull(type, "Extractor type must not be null.");
checkNotNull(extractorConfig, "Extractor configuration must not be null.");
// we go the really easy way here.
Map looksLikeForm = Maps.newHashMapWithExpectedSize(extractorConfig.size());
for (Map.Entry e : extractorConfig.entrySet()) {
looksLikeForm.put(e.getKey(), new String[]{e.getValue().toString()});
}
loadConfigFromForm(type, looksLikeForm);
}
public void loadConvertersFromForm(Map form) {
if (form == null || form.isEmpty()) {
return;
}
for (String name : extractSelectedConverters(form)) {
Converter.Type converterType = Converter.Type.valueOf(name.toUpperCase());
Map converterConfig = extractConverterConfig(converterType, form);
converters.add(new Converter(converterType, converterConfig));
}
}
@SuppressWarnings("unchecked")
public void loadConvertersFromImport(List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy