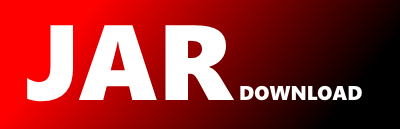
org.graylog.plugins.map.geoip.$AutoValue_IPinfoStandardLocation Maven / Gradle / Ivy
package org.graylog.plugins.map.geoip;
import com.fasterxml.jackson.annotation.JsonProperty;
import javax.annotation.Nullable;
import javax.annotation.processing.Generated;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
abstract class $AutoValue_IPinfoStandardLocation extends IPinfoStandardLocation {
@Nullable
private final String city;
@Nullable
private final String country;
@Nullable
private final String timezone;
@Nullable
private final String region;
@Nullable
private final Long geoNameId;
private final double latitude;
private final double longitude;
$AutoValue_IPinfoStandardLocation(
@Nullable String city,
@Nullable String country,
@Nullable String timezone,
@Nullable String region,
@Nullable Long geoNameId,
double latitude,
double longitude) {
this.city = city;
this.country = country;
this.timezone = timezone;
this.region = region;
this.geoNameId = geoNameId;
this.latitude = latitude;
this.longitude = longitude;
}
@JsonProperty("city")
@Nullable
@Override
public String city() {
return city;
}
@JsonProperty("country")
@Nullable
@Override
public String country() {
return country;
}
@JsonProperty("timezone")
@Nullable
@Override
public String timezone() {
return timezone;
}
@JsonProperty("region")
@Nullable
@Override
public String region() {
return region;
}
@JsonProperty("geoname_id")
@Nullable
@Override
public Long geoNameId() {
return geoNameId;
}
@JsonProperty("lat")
@Override
public double latitude() {
return latitude;
}
@JsonProperty("lng")
@Override
public double longitude() {
return longitude;
}
@Override
public String toString() {
return "IPinfoStandardLocation{"
+ "city=" + city + ", "
+ "country=" + country + ", "
+ "timezone=" + timezone + ", "
+ "region=" + region + ", "
+ "geoNameId=" + geoNameId + ", "
+ "latitude=" + latitude + ", "
+ "longitude=" + longitude
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof IPinfoStandardLocation) {
IPinfoStandardLocation that = (IPinfoStandardLocation) o;
return (this.city == null ? that.city() == null : this.city.equals(that.city()))
&& (this.country == null ? that.country() == null : this.country.equals(that.country()))
&& (this.timezone == null ? that.timezone() == null : this.timezone.equals(that.timezone()))
&& (this.region == null ? that.region() == null : this.region.equals(that.region()))
&& (this.geoNameId == null ? that.geoNameId() == null : this.geoNameId.equals(that.geoNameId()))
&& Double.doubleToLongBits(this.latitude) == Double.doubleToLongBits(that.latitude())
&& Double.doubleToLongBits(this.longitude) == Double.doubleToLongBits(that.longitude());
}
return false;
}
@Override
public int hashCode() {
int h$ = 1;
h$ *= 1000003;
h$ ^= (city == null) ? 0 : city.hashCode();
h$ *= 1000003;
h$ ^= (country == null) ? 0 : country.hashCode();
h$ *= 1000003;
h$ ^= (timezone == null) ? 0 : timezone.hashCode();
h$ *= 1000003;
h$ ^= (region == null) ? 0 : region.hashCode();
h$ *= 1000003;
h$ ^= (geoNameId == null) ? 0 : geoNameId.hashCode();
h$ *= 1000003;
h$ ^= (int) ((Double.doubleToLongBits(latitude) >>> 32) ^ Double.doubleToLongBits(latitude));
h$ *= 1000003;
h$ ^= (int) ((Double.doubleToLongBits(longitude) >>> 32) ^ Double.doubleToLongBits(longitude));
return h$;
}
static class Builder extends IPinfoStandardLocation.Builder {
private String city;
private String country;
private String timezone;
private String region;
private Long geoNameId;
private double latitude;
private double longitude;
private byte set$0;
Builder() {
}
@Override
public IPinfoStandardLocation.Builder city(String city) {
this.city = city;
return this;
}
@Override
public IPinfoStandardLocation.Builder country(String country) {
this.country = country;
return this;
}
@Override
public IPinfoStandardLocation.Builder timezone(String timezone) {
this.timezone = timezone;
return this;
}
@Override
public IPinfoStandardLocation.Builder region(String region) {
this.region = region;
return this;
}
@Override
public IPinfoStandardLocation.Builder geoNameId(Long geoNameId) {
this.geoNameId = geoNameId;
return this;
}
@Override
public IPinfoStandardLocation.Builder latitude(double latitude) {
this.latitude = latitude;
set$0 |= (byte) 1;
return this;
}
@Override
public IPinfoStandardLocation.Builder longitude(double longitude) {
this.longitude = longitude;
set$0 |= (byte) 2;
return this;
}
@Override
public IPinfoStandardLocation build() {
if (set$0 != 3) {
StringBuilder missing = new StringBuilder();
if ((set$0 & 1) == 0) {
missing.append(" latitude");
}
if ((set$0 & 2) == 0) {
missing.append(" longitude");
}
throw new IllegalStateException("Missing required properties:" + missing);
}
return new AutoValue_IPinfoStandardLocation(
this.city,
this.country,
this.timezone,
this.region,
this.geoNameId,
this.latitude,
this.longitude);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy