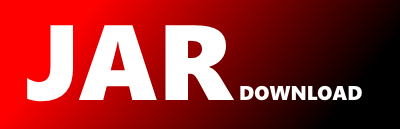
org.graylog2.plugin.journal.JournalMessages Maven / Gradle / Ivy
/*
* Copyright (C) 2020 Graylog, Inc.
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the Server Side Public License, version 1,
* as published by MongoDB, Inc.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* Server Side Public License for more details.
*
* You should have received a copy of the Server Side Public License
* along with this program. If not, see
* .
*/
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: resources/org/graylog2/plugin/journal/raw_message.proto
// to regenerate:
// install protobuf compiler (this was generated with 2.5.0)
// cd graylog2-server/src/main && protoc --java_out=java resources/org/graylog2/plugin/journal/raw_message.proto
package org.graylog2.plugin.journal;
public final class JournalMessages {
private JournalMessages() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
}
public interface JournalMessageOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// optional uint32 version = 1;
/**
* optional uint32 version = 1;
*
*
* the version of the message format (used for simplifying code when deserializing messages)
*
*/
boolean hasVersion();
/**
* optional uint32 version = 1;
*
*
* the version of the message format (used for simplifying code when deserializing messages)
*
*/
int getVersion();
// optional fixed64 uuid_time = 2;
/**
* optional fixed64 uuid_time = 2;
*
*
* uuid, time is upper 64 bits, clockseq is lower 64 bits of the 128 bit uuid value
*
*/
boolean hasUuidTime();
/**
* optional fixed64 uuid_time = 2;
*
*
* uuid, time is upper 64 bits, clockseq is lower 64 bits of the 128 bit uuid value
*
*/
long getUuidTime();
// optional fixed64 uuid_clockseq = 3;
/**
* optional fixed64 uuid_clockseq = 3;
*/
boolean hasUuidClockseq();
/**
* optional fixed64 uuid_clockseq = 3;
*/
long getUuidClockseq();
// optional fixed64 timestamp = 4;
/**
* optional fixed64 timestamp = 4;
*
*
* milliseconds since Java epoch (1970/01/01 00:00:00.000)
*
*/
boolean hasTimestamp();
/**
* optional fixed64 timestamp = 4;
*
*
* milliseconds since Java epoch (1970/01/01 00:00:00.000)
*
*/
long getTimestamp();
// optional .org.graylog2.plugin.journal.CodecInfo codec = 5;
/**
* optional .org.graylog2.plugin.journal.CodecInfo codec = 5;
*
*
* which format the payload is supposed to have
*
*/
boolean hasCodec();
/**
* optional .org.graylog2.plugin.journal.CodecInfo codec = 5;
*
*
* which format the payload is supposed to have
*
*/
org.graylog2.plugin.journal.JournalMessages.CodecInfo getCodec();
/**
* optional .org.graylog2.plugin.journal.CodecInfo codec = 5;
*
*
* which format the payload is supposed to have
*
*/
org.graylog2.plugin.journal.JournalMessages.CodecInfoOrBuilder getCodecOrBuilder();
// repeated .org.graylog2.plugin.journal.SourceNode source_nodes = 6;
/**
* repeated .org.graylog2.plugin.journal.SourceNode source_nodes = 6;
*
*
* the list of graylog2 nodes which have handled the message (radios, servers) in receive order
*
*/
java.util.List
getSourceNodesList();
/**
* repeated .org.graylog2.plugin.journal.SourceNode source_nodes = 6;
*
*
* the list of graylog2 nodes which have handled the message (radios, servers) in receive order
*
*/
org.graylog2.plugin.journal.JournalMessages.SourceNode getSourceNodes(int index);
/**
* repeated .org.graylog2.plugin.journal.SourceNode source_nodes = 6;
*
*
* the list of graylog2 nodes which have handled the message (radios, servers) in receive order
*
*/
int getSourceNodesCount();
/**
* repeated .org.graylog2.plugin.journal.SourceNode source_nodes = 6;
*
*
* the list of graylog2 nodes which have handled the message (radios, servers) in receive order
*
*/
java.util.List extends org.graylog2.plugin.journal.JournalMessages.SourceNodeOrBuilder>
getSourceNodesOrBuilderList();
/**
* repeated .org.graylog2.plugin.journal.SourceNode source_nodes = 6;
*
*
* the list of graylog2 nodes which have handled the message (radios, servers) in receive order
*
*/
org.graylog2.plugin.journal.JournalMessages.SourceNodeOrBuilder getSourceNodesOrBuilder(
int index);
// optional .org.graylog2.plugin.journal.RemoteAddress remote = 7;
/**
* optional .org.graylog2.plugin.journal.RemoteAddress remote = 7;
*/
boolean hasRemote();
/**
* optional .org.graylog2.plugin.journal.RemoteAddress remote = 7;
*/
org.graylog2.plugin.journal.JournalMessages.RemoteAddress getRemote();
/**
* optional .org.graylog2.plugin.journal.RemoteAddress remote = 7;
*/
org.graylog2.plugin.journal.JournalMessages.RemoteAddressOrBuilder getRemoteOrBuilder();
// optional bytes payload = 8;
/**
* optional bytes payload = 8;
*/
boolean hasPayload();
/**
* optional bytes payload = 8;
*/
com.google.protobuf.ByteString getPayload();
// optional uint32 sequence_nr = 9;
/**
* optional uint32 sequence_nr = 9;
*/
boolean hasSequenceNr();
/**
* optional uint32 sequence_nr = 9;
*/
int getSequenceNr();
}
/**
* Protobuf type {@code org.graylog2.plugin.journal.JournalMessage}
*/
public static final class JournalMessage extends
com.google.protobuf.GeneratedMessage
implements JournalMessageOrBuilder {
// Use JournalMessage.newBuilder() to construct.
private JournalMessage(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private JournalMessage(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final JournalMessage defaultInstance;
public static JournalMessage getDefaultInstance() {
return defaultInstance;
}
public JournalMessage getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private JournalMessage(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
bitField0_ |= 0x00000001;
version_ = input.readUInt32();
break;
}
case 17: {
bitField0_ |= 0x00000002;
uuidTime_ = input.readFixed64();
break;
}
case 25: {
bitField0_ |= 0x00000004;
uuidClockseq_ = input.readFixed64();
break;
}
case 33: {
bitField0_ |= 0x00000008;
timestamp_ = input.readFixed64();
break;
}
case 42: {
org.graylog2.plugin.journal.JournalMessages.CodecInfo.Builder subBuilder = null;
if (((bitField0_ & 0x00000010) == 0x00000010)) {
subBuilder = codec_.toBuilder();
}
codec_ = input.readMessage(org.graylog2.plugin.journal.JournalMessages.CodecInfo.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(codec_);
codec_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000010;
break;
}
case 50: {
if (!((mutable_bitField0_ & 0x00000020) == 0x00000020)) {
sourceNodes_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000020;
}
sourceNodes_.add(input.readMessage(org.graylog2.plugin.journal.JournalMessages.SourceNode.PARSER, extensionRegistry));
break;
}
case 58: {
org.graylog2.plugin.journal.JournalMessages.RemoteAddress.Builder subBuilder = null;
if (((bitField0_ & 0x00000020) == 0x00000020)) {
subBuilder = remote_.toBuilder();
}
remote_ = input.readMessage(org.graylog2.plugin.journal.JournalMessages.RemoteAddress.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(remote_);
remote_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000020;
break;
}
case 66: {
bitField0_ |= 0x00000040;
payload_ = input.readBytes();
break;
}
case 72: {
bitField0_ |= 0x00000080;
sequenceNr_ = input.readUInt32();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000020) == 0x00000020)) {
sourceNodes_ = java.util.Collections.unmodifiableList(sourceNodes_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.graylog2.plugin.journal.JournalMessages.internal_static_org_graylog2_plugin_journal_JournalMessage_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.graylog2.plugin.journal.JournalMessages.internal_static_org_graylog2_plugin_journal_JournalMessage_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.graylog2.plugin.journal.JournalMessages.JournalMessage.class, org.graylog2.plugin.journal.JournalMessages.JournalMessage.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public JournalMessage parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new JournalMessage(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// optional uint32 version = 1;
public static final int VERSION_FIELD_NUMBER = 1;
private int version_;
/**
* optional uint32 version = 1;
*
*
* the version of the message format (used for simplifying code when deserializing messages)
*
*/
public boolean hasVersion() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional uint32 version = 1;
*
*
* the version of the message format (used for simplifying code when deserializing messages)
*
*/
public int getVersion() {
return version_;
}
// optional fixed64 uuid_time = 2;
public static final int UUID_TIME_FIELD_NUMBER = 2;
private long uuidTime_;
/**
* optional fixed64 uuid_time = 2;
*
*
* uuid, time is upper 64 bits, clockseq is lower 64 bits of the 128 bit uuid value
*
*/
public boolean hasUuidTime() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional fixed64 uuid_time = 2;
*
*
* uuid, time is upper 64 bits, clockseq is lower 64 bits of the 128 bit uuid value
*
*/
public long getUuidTime() {
return uuidTime_;
}
// optional fixed64 uuid_clockseq = 3;
public static final int UUID_CLOCKSEQ_FIELD_NUMBER = 3;
private long uuidClockseq_;
/**
* optional fixed64 uuid_clockseq = 3;
*/
public boolean hasUuidClockseq() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional fixed64 uuid_clockseq = 3;
*/
public long getUuidClockseq() {
return uuidClockseq_;
}
// optional fixed64 timestamp = 4;
public static final int TIMESTAMP_FIELD_NUMBER = 4;
private long timestamp_;
/**
* optional fixed64 timestamp = 4;
*
*
* milliseconds since Java epoch (1970/01/01 00:00:00.000)
*
*/
public boolean hasTimestamp() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional fixed64 timestamp = 4;
*
*
* milliseconds since Java epoch (1970/01/01 00:00:00.000)
*
*/
public long getTimestamp() {
return timestamp_;
}
// optional .org.graylog2.plugin.journal.CodecInfo codec = 5;
public static final int CODEC_FIELD_NUMBER = 5;
private org.graylog2.plugin.journal.JournalMessages.CodecInfo codec_;
/**
* optional .org.graylog2.plugin.journal.CodecInfo codec = 5;
*
*
* which format the payload is supposed to have
*
*/
public boolean hasCodec() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional .org.graylog2.plugin.journal.CodecInfo codec = 5;
*
*
* which format the payload is supposed to have
*
*/
public org.graylog2.plugin.journal.JournalMessages.CodecInfo getCodec() {
return codec_;
}
/**
* optional .org.graylog2.plugin.journal.CodecInfo codec = 5;
*
*
* which format the payload is supposed to have
*
*/
public org.graylog2.plugin.journal.JournalMessages.CodecInfoOrBuilder getCodecOrBuilder() {
return codec_;
}
// repeated .org.graylog2.plugin.journal.SourceNode source_nodes = 6;
public static final int SOURCE_NODES_FIELD_NUMBER = 6;
private java.util.List sourceNodes_;
/**
* repeated .org.graylog2.plugin.journal.SourceNode source_nodes = 6;
*
*
* the list of graylog2 nodes which have handled the message (radios, servers) in receive order
*
*/
public java.util.List getSourceNodesList() {
return sourceNodes_;
}
/**
* repeated .org.graylog2.plugin.journal.SourceNode source_nodes = 6;
*
*
* the list of graylog2 nodes which have handled the message (radios, servers) in receive order
*
*/
public java.util.List extends org.graylog2.plugin.journal.JournalMessages.SourceNodeOrBuilder>
getSourceNodesOrBuilderList() {
return sourceNodes_;
}
/**
* repeated .org.graylog2.plugin.journal.SourceNode source_nodes = 6;
*
*
* the list of graylog2 nodes which have handled the message (radios, servers) in receive order
*
*/
public int getSourceNodesCount() {
return sourceNodes_.size();
}
/**
* repeated .org.graylog2.plugin.journal.SourceNode source_nodes = 6;
*
*
* the list of graylog2 nodes which have handled the message (radios, servers) in receive order
*
*/
public org.graylog2.plugin.journal.JournalMessages.SourceNode getSourceNodes(int index) {
return sourceNodes_.get(index);
}
/**
* repeated .org.graylog2.plugin.journal.SourceNode source_nodes = 6;
*
*
* the list of graylog2 nodes which have handled the message (radios, servers) in receive order
*
*/
public org.graylog2.plugin.journal.JournalMessages.SourceNodeOrBuilder getSourceNodesOrBuilder(
int index) {
return sourceNodes_.get(index);
}
// optional .org.graylog2.plugin.journal.RemoteAddress remote = 7;
public static final int REMOTE_FIELD_NUMBER = 7;
private org.graylog2.plugin.journal.JournalMessages.RemoteAddress remote_;
/**
* optional .org.graylog2.plugin.journal.RemoteAddress remote = 7;
*/
public boolean hasRemote() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
* optional .org.graylog2.plugin.journal.RemoteAddress remote = 7;
*/
public org.graylog2.plugin.journal.JournalMessages.RemoteAddress getRemote() {
return remote_;
}
/**
* optional .org.graylog2.plugin.journal.RemoteAddress remote = 7;
*/
public org.graylog2.plugin.journal.JournalMessages.RemoteAddressOrBuilder getRemoteOrBuilder() {
return remote_;
}
// optional bytes payload = 8;
public static final int PAYLOAD_FIELD_NUMBER = 8;
private com.google.protobuf.ByteString payload_;
/**
* optional bytes payload = 8;
*/
public boolean hasPayload() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
* optional bytes payload = 8;
*/
public com.google.protobuf.ByteString getPayload() {
return payload_;
}
// optional uint32 sequence_nr = 9;
public static final int SEQUENCE_NR_FIELD_NUMBER = 9;
private int sequenceNr_;
/**
* optional uint32 sequence_nr = 9;
*/
public boolean hasSequenceNr() {
return ((bitField0_ & 0x00000080) == 0x00000080);
}
/**
* optional uint32 sequence_nr = 9;
*/
public int getSequenceNr() {
return sequenceNr_;
}
private void initFields() {
version_ = 0;
uuidTime_ = 0L;
uuidClockseq_ = 0L;
timestamp_ = 0L;
codec_ = org.graylog2.plugin.journal.JournalMessages.CodecInfo.getDefaultInstance();
sourceNodes_ = java.util.Collections.emptyList();
remote_ = org.graylog2.plugin.journal.JournalMessages.RemoteAddress.getDefaultInstance();
payload_ = com.google.protobuf.ByteString.EMPTY;
sequenceNr_ = 0;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeUInt32(1, version_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeFixed64(2, uuidTime_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeFixed64(3, uuidClockseq_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeFixed64(4, timestamp_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
output.writeMessage(5, codec_);
}
for (int i = 0; i < sourceNodes_.size(); i++) {
output.writeMessage(6, sourceNodes_.get(i));
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
output.writeMessage(7, remote_);
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
output.writeBytes(8, payload_);
}
if (((bitField0_ & 0x00000080) == 0x00000080)) {
output.writeUInt32(9, sequenceNr_);
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(1, version_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeFixed64Size(2, uuidTime_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeFixed64Size(3, uuidClockseq_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeFixed64Size(4, timestamp_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, codec_);
}
for (int i = 0; i < sourceNodes_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(6, sourceNodes_.get(i));
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(7, remote_);
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(8, payload_);
}
if (((bitField0_ & 0x00000080) == 0x00000080)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(9, sequenceNr_);
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static org.graylog2.plugin.journal.JournalMessages.JournalMessage parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.graylog2.plugin.journal.JournalMessages.JournalMessage parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.graylog2.plugin.journal.JournalMessages.JournalMessage parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.graylog2.plugin.journal.JournalMessages.JournalMessage parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.graylog2.plugin.journal.JournalMessages.JournalMessage parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.graylog2.plugin.journal.JournalMessages.JournalMessage parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.graylog2.plugin.journal.JournalMessages.JournalMessage parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.graylog2.plugin.journal.JournalMessages.JournalMessage parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.graylog2.plugin.journal.JournalMessages.JournalMessage parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.graylog2.plugin.journal.JournalMessages.JournalMessage parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(org.graylog2.plugin.journal.JournalMessages.JournalMessage prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code org.graylog2.plugin.journal.JournalMessage}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements org.graylog2.plugin.journal.JournalMessages.JournalMessageOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.graylog2.plugin.journal.JournalMessages.internal_static_org_graylog2_plugin_journal_JournalMessage_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.graylog2.plugin.journal.JournalMessages.internal_static_org_graylog2_plugin_journal_JournalMessage_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.graylog2.plugin.journal.JournalMessages.JournalMessage.class, org.graylog2.plugin.journal.JournalMessages.JournalMessage.Builder.class);
}
// Construct using org.graylog2.plugin.journal.JournalMessages.JournalMessage.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getCodecFieldBuilder();
getSourceNodesFieldBuilder();
getRemoteFieldBuilder();
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
version_ = 0;
bitField0_ = (bitField0_ & ~0x00000001);
uuidTime_ = 0L;
bitField0_ = (bitField0_ & ~0x00000002);
uuidClockseq_ = 0L;
bitField0_ = (bitField0_ & ~0x00000004);
timestamp_ = 0L;
bitField0_ = (bitField0_ & ~0x00000008);
if (codecBuilder_ == null) {
codec_ = org.graylog2.plugin.journal.JournalMessages.CodecInfo.getDefaultInstance();
} else {
codecBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000010);
if (sourceNodesBuilder_ == null) {
sourceNodes_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000020);
} else {
sourceNodesBuilder_.clear();
}
if (remoteBuilder_ == null) {
remote_ = org.graylog2.plugin.journal.JournalMessages.RemoteAddress.getDefaultInstance();
} else {
remoteBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000040);
payload_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000080);
sequenceNr_ = 0;
bitField0_ = (bitField0_ & ~0x00000100);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.graylog2.plugin.journal.JournalMessages.internal_static_org_graylog2_plugin_journal_JournalMessage_descriptor;
}
public org.graylog2.plugin.journal.JournalMessages.JournalMessage getDefaultInstanceForType() {
return org.graylog2.plugin.journal.JournalMessages.JournalMessage.getDefaultInstance();
}
public org.graylog2.plugin.journal.JournalMessages.JournalMessage build() {
org.graylog2.plugin.journal.JournalMessages.JournalMessage result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.graylog2.plugin.journal.JournalMessages.JournalMessage buildPartial() {
org.graylog2.plugin.journal.JournalMessages.JournalMessage result = new org.graylog2.plugin.journal.JournalMessages.JournalMessage(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.version_ = version_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.uuidTime_ = uuidTime_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.uuidClockseq_ = uuidClockseq_;
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000008;
}
result.timestamp_ = timestamp_;
if (((from_bitField0_ & 0x00000010) == 0x00000010)) {
to_bitField0_ |= 0x00000010;
}
if (codecBuilder_ == null) {
result.codec_ = codec_;
} else {
result.codec_ = codecBuilder_.build();
}
if (sourceNodesBuilder_ == null) {
if (((bitField0_ & 0x00000020) == 0x00000020)) {
sourceNodes_ = java.util.Collections.unmodifiableList(sourceNodes_);
bitField0_ = (bitField0_ & ~0x00000020);
}
result.sourceNodes_ = sourceNodes_;
} else {
result.sourceNodes_ = sourceNodesBuilder_.build();
}
if (((from_bitField0_ & 0x00000040) == 0x00000040)) {
to_bitField0_ |= 0x00000020;
}
if (remoteBuilder_ == null) {
result.remote_ = remote_;
} else {
result.remote_ = remoteBuilder_.build();
}
if (((from_bitField0_ & 0x00000080) == 0x00000080)) {
to_bitField0_ |= 0x00000040;
}
result.payload_ = payload_;
if (((from_bitField0_ & 0x00000100) == 0x00000100)) {
to_bitField0_ |= 0x00000080;
}
result.sequenceNr_ = sequenceNr_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.graylog2.plugin.journal.JournalMessages.JournalMessage) {
return mergeFrom((org.graylog2.plugin.journal.JournalMessages.JournalMessage)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.graylog2.plugin.journal.JournalMessages.JournalMessage other) {
if (other == org.graylog2.plugin.journal.JournalMessages.JournalMessage.getDefaultInstance()) return this;
if (other.hasVersion()) {
setVersion(other.getVersion());
}
if (other.hasUuidTime()) {
setUuidTime(other.getUuidTime());
}
if (other.hasUuidClockseq()) {
setUuidClockseq(other.getUuidClockseq());
}
if (other.hasTimestamp()) {
setTimestamp(other.getTimestamp());
}
if (other.hasCodec()) {
mergeCodec(other.getCodec());
}
if (sourceNodesBuilder_ == null) {
if (!other.sourceNodes_.isEmpty()) {
if (sourceNodes_.isEmpty()) {
sourceNodes_ = other.sourceNodes_;
bitField0_ = (bitField0_ & ~0x00000020);
} else {
ensureSourceNodesIsMutable();
sourceNodes_.addAll(other.sourceNodes_);
}
onChanged();
}
} else {
if (!other.sourceNodes_.isEmpty()) {
if (sourceNodesBuilder_.isEmpty()) {
sourceNodesBuilder_.dispose();
sourceNodesBuilder_ = null;
sourceNodes_ = other.sourceNodes_;
bitField0_ = (bitField0_ & ~0x00000020);
sourceNodesBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getSourceNodesFieldBuilder() : null;
} else {
sourceNodesBuilder_.addAllMessages(other.sourceNodes_);
}
}
}
if (other.hasRemote()) {
mergeRemote(other.getRemote());
}
if (other.hasPayload()) {
setPayload(other.getPayload());
}
if (other.hasSequenceNr()) {
setSequenceNr(other.getSequenceNr());
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.graylog2.plugin.journal.JournalMessages.JournalMessage parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.graylog2.plugin.journal.JournalMessages.JournalMessage) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// optional uint32 version = 1;
private int version_ ;
/**
* optional uint32 version = 1;
*
*
* the version of the message format (used for simplifying code when deserializing messages)
*
*/
public boolean hasVersion() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional uint32 version = 1;
*
*
* the version of the message format (used for simplifying code when deserializing messages)
*
*/
public int getVersion() {
return version_;
}
/**
* optional uint32 version = 1;
*
*
* the version of the message format (used for simplifying code when deserializing messages)
*
*/
public Builder setVersion(int value) {
bitField0_ |= 0x00000001;
version_ = value;
onChanged();
return this;
}
/**
* optional uint32 version = 1;
*
*
* the version of the message format (used for simplifying code when deserializing messages)
*
*/
public Builder clearVersion() {
bitField0_ = (bitField0_ & ~0x00000001);
version_ = 0;
onChanged();
return this;
}
// optional fixed64 uuid_time = 2;
private long uuidTime_ ;
/**
* optional fixed64 uuid_time = 2;
*
*
* uuid, time is upper 64 bits, clockseq is lower 64 bits of the 128 bit uuid value
*
*/
public boolean hasUuidTime() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional fixed64 uuid_time = 2;
*
*
* uuid, time is upper 64 bits, clockseq is lower 64 bits of the 128 bit uuid value
*
*/
public long getUuidTime() {
return uuidTime_;
}
/**
* optional fixed64 uuid_time = 2;
*
*
* uuid, time is upper 64 bits, clockseq is lower 64 bits of the 128 bit uuid value
*
*/
public Builder setUuidTime(long value) {
bitField0_ |= 0x00000002;
uuidTime_ = value;
onChanged();
return this;
}
/**
* optional fixed64 uuid_time = 2;
*
*
* uuid, time is upper 64 bits, clockseq is lower 64 bits of the 128 bit uuid value
*
*/
public Builder clearUuidTime() {
bitField0_ = (bitField0_ & ~0x00000002);
uuidTime_ = 0L;
onChanged();
return this;
}
// optional fixed64 uuid_clockseq = 3;
private long uuidClockseq_ ;
/**
* optional fixed64 uuid_clockseq = 3;
*/
public boolean hasUuidClockseq() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional fixed64 uuid_clockseq = 3;
*/
public long getUuidClockseq() {
return uuidClockseq_;
}
/**
* optional fixed64 uuid_clockseq = 3;
*/
public Builder setUuidClockseq(long value) {
bitField0_ |= 0x00000004;
uuidClockseq_ = value;
onChanged();
return this;
}
/**
* optional fixed64 uuid_clockseq = 3;
*/
public Builder clearUuidClockseq() {
bitField0_ = (bitField0_ & ~0x00000004);
uuidClockseq_ = 0L;
onChanged();
return this;
}
// optional fixed64 timestamp = 4;
private long timestamp_ ;
/**
* optional fixed64 timestamp = 4;
*
*
* milliseconds since Java epoch (1970/01/01 00:00:00.000)
*
*/
public boolean hasTimestamp() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional fixed64 timestamp = 4;
*
*
* milliseconds since Java epoch (1970/01/01 00:00:00.000)
*
*/
public long getTimestamp() {
return timestamp_;
}
/**
* optional fixed64 timestamp = 4;
*
*
* milliseconds since Java epoch (1970/01/01 00:00:00.000)
*
*/
public Builder setTimestamp(long value) {
bitField0_ |= 0x00000008;
timestamp_ = value;
onChanged();
return this;
}
/**
* optional fixed64 timestamp = 4;
*
*
* milliseconds since Java epoch (1970/01/01 00:00:00.000)
*
*/
public Builder clearTimestamp() {
bitField0_ = (bitField0_ & ~0x00000008);
timestamp_ = 0L;
onChanged();
return this;
}
// optional .org.graylog2.plugin.journal.CodecInfo codec = 5;
private org.graylog2.plugin.journal.JournalMessages.CodecInfo codec_ = org.graylog2.plugin.journal.JournalMessages.CodecInfo.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
org.graylog2.plugin.journal.JournalMessages.CodecInfo, org.graylog2.plugin.journal.JournalMessages.CodecInfo.Builder, org.graylog2.plugin.journal.JournalMessages.CodecInfoOrBuilder> codecBuilder_;
/**
* optional .org.graylog2.plugin.journal.CodecInfo codec = 5;
*
*
* which format the payload is supposed to have
*
*/
public boolean hasCodec() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
* optional .org.graylog2.plugin.journal.CodecInfo codec = 5;
*
*
* which format the payload is supposed to have
*
*/
public org.graylog2.plugin.journal.JournalMessages.CodecInfo getCodec() {
if (codecBuilder_ == null) {
return codec_;
} else {
return codecBuilder_.getMessage();
}
}
/**
* optional .org.graylog2.plugin.journal.CodecInfo codec = 5;
*
*
* which format the payload is supposed to have
*
*/
public Builder setCodec(org.graylog2.plugin.journal.JournalMessages.CodecInfo value) {
if (codecBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
codec_ = value;
onChanged();
} else {
codecBuilder_.setMessage(value);
}
bitField0_ |= 0x00000010;
return this;
}
/**
* optional .org.graylog2.plugin.journal.CodecInfo codec = 5;
*
*
* which format the payload is supposed to have
*
*/
public Builder setCodec(
org.graylog2.plugin.journal.JournalMessages.CodecInfo.Builder builderForValue) {
if (codecBuilder_ == null) {
codec_ = builderForValue.build();
onChanged();
} else {
codecBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000010;
return this;
}
/**
* optional .org.graylog2.plugin.journal.CodecInfo codec = 5;
*
*
* which format the payload is supposed to have
*
*/
public Builder mergeCodec(org.graylog2.plugin.journal.JournalMessages.CodecInfo value) {
if (codecBuilder_ == null) {
if (((bitField0_ & 0x00000010) == 0x00000010) &&
codec_ != org.graylog2.plugin.journal.JournalMessages.CodecInfo.getDefaultInstance()) {
codec_ =
org.graylog2.plugin.journal.JournalMessages.CodecInfo.newBuilder(codec_).mergeFrom(value).buildPartial();
} else {
codec_ = value;
}
onChanged();
} else {
codecBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000010;
return this;
}
/**
* optional .org.graylog2.plugin.journal.CodecInfo codec = 5;
*
*
* which format the payload is supposed to have
*
*/
public Builder clearCodec() {
if (codecBuilder_ == null) {
codec_ = org.graylog2.plugin.journal.JournalMessages.CodecInfo.getDefaultInstance();
onChanged();
} else {
codecBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000010);
return this;
}
/**
* optional .org.graylog2.plugin.journal.CodecInfo codec = 5;
*
*
* which format the payload is supposed to have
*
*/
public org.graylog2.plugin.journal.JournalMessages.CodecInfo.Builder getCodecBuilder() {
bitField0_ |= 0x00000010;
onChanged();
return getCodecFieldBuilder().getBuilder();
}
/**
* optional .org.graylog2.plugin.journal.CodecInfo codec = 5;
*
*
* which format the payload is supposed to have
*
*/
public org.graylog2.plugin.journal.JournalMessages.CodecInfoOrBuilder getCodecOrBuilder() {
if (codecBuilder_ != null) {
return codecBuilder_.getMessageOrBuilder();
} else {
return codec_;
}
}
/**
* optional .org.graylog2.plugin.journal.CodecInfo codec = 5;
*
*
* which format the payload is supposed to have
*
*/
private com.google.protobuf.SingleFieldBuilder<
org.graylog2.plugin.journal.JournalMessages.CodecInfo, org.graylog2.plugin.journal.JournalMessages.CodecInfo.Builder, org.graylog2.plugin.journal.JournalMessages.CodecInfoOrBuilder>
getCodecFieldBuilder() {
if (codecBuilder_ == null) {
codecBuilder_ = new com.google.protobuf.SingleFieldBuilder<
org.graylog2.plugin.journal.JournalMessages.CodecInfo, org.graylog2.plugin.journal.JournalMessages.CodecInfo.Builder, org.graylog2.plugin.journal.JournalMessages.CodecInfoOrBuilder>(
codec_,
getParentForChildren(),
isClean());
codec_ = null;
}
return codecBuilder_;
}
// repeated .org.graylog2.plugin.journal.SourceNode source_nodes = 6;
private java.util.List sourceNodes_ =
java.util.Collections.emptyList();
private void ensureSourceNodesIsMutable() {
if (!((bitField0_ & 0x00000020) == 0x00000020)) {
sourceNodes_ = new java.util.ArrayList(sourceNodes_);
bitField0_ |= 0x00000020;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
org.graylog2.plugin.journal.JournalMessages.SourceNode, org.graylog2.plugin.journal.JournalMessages.SourceNode.Builder, org.graylog2.plugin.journal.JournalMessages.SourceNodeOrBuilder> sourceNodesBuilder_;
/**
* repeated .org.graylog2.plugin.journal.SourceNode source_nodes = 6;
*
*
* the list of graylog2 nodes which have handled the message (radios, servers) in receive order
*
*/
public java.util.List getSourceNodesList() {
if (sourceNodesBuilder_ == null) {
return java.util.Collections.unmodifiableList(sourceNodes_);
} else {
return sourceNodesBuilder_.getMessageList();
}
}
/**
* repeated .org.graylog2.plugin.journal.SourceNode source_nodes = 6;
*
*
* the list of graylog2 nodes which have handled the message (radios, servers) in receive order
*
*/
public int getSourceNodesCount() {
if (sourceNodesBuilder_ == null) {
return sourceNodes_.size();
} else {
return sourceNodesBuilder_.getCount();
}
}
/**
* repeated .org.graylog2.plugin.journal.SourceNode source_nodes = 6;
*
*
* the list of graylog2 nodes which have handled the message (radios, servers) in receive order
*
*/
public org.graylog2.plugin.journal.JournalMessages.SourceNode getSourceNodes(int index) {
if (sourceNodesBuilder_ == null) {
return sourceNodes_.get(index);
} else {
return sourceNodesBuilder_.getMessage(index);
}
}
/**
* repeated .org.graylog2.plugin.journal.SourceNode source_nodes = 6;
*
*
* the list of graylog2 nodes which have handled the message (radios, servers) in receive order
*
*/
public Builder setSourceNodes(
int index, org.graylog2.plugin.journal.JournalMessages.SourceNode value) {
if (sourceNodesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureSourceNodesIsMutable();
sourceNodes_.set(index, value);
onChanged();
} else {
sourceNodesBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .org.graylog2.plugin.journal.SourceNode source_nodes = 6;
*
*
* the list of graylog2 nodes which have handled the message (radios, servers) in receive order
*
*/
public Builder setSourceNodes(
int index, org.graylog2.plugin.journal.JournalMessages.SourceNode.Builder builderForValue) {
if (sourceNodesBuilder_ == null) {
ensureSourceNodesIsMutable();
sourceNodes_.set(index, builderForValue.build());
onChanged();
} else {
sourceNodesBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .org.graylog2.plugin.journal.SourceNode source_nodes = 6;
*
*
* the list of graylog2 nodes which have handled the message (radios, servers) in receive order
*
*/
public Builder addSourceNodes(org.graylog2.plugin.journal.JournalMessages.SourceNode value) {
if (sourceNodesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureSourceNodesIsMutable();
sourceNodes_.add(value);
onChanged();
} else {
sourceNodesBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .org.graylog2.plugin.journal.SourceNode source_nodes = 6;
*
*
* the list of graylog2 nodes which have handled the message (radios, servers) in receive order
*
*/
public Builder addSourceNodes(
int index, org.graylog2.plugin.journal.JournalMessages.SourceNode value) {
if (sourceNodesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureSourceNodesIsMutable();
sourceNodes_.add(index, value);
onChanged();
} else {
sourceNodesBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .org.graylog2.plugin.journal.SourceNode source_nodes = 6;
*
*
* the list of graylog2 nodes which have handled the message (radios, servers) in receive order
*
*/
public Builder addSourceNodes(
org.graylog2.plugin.journal.JournalMessages.SourceNode.Builder builderForValue) {
if (sourceNodesBuilder_ == null) {
ensureSourceNodesIsMutable();
sourceNodes_.add(builderForValue.build());
onChanged();
} else {
sourceNodesBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .org.graylog2.plugin.journal.SourceNode source_nodes = 6;
*
*
* the list of graylog2 nodes which have handled the message (radios, servers) in receive order
*
*/
public Builder addSourceNodes(
int index, org.graylog2.plugin.journal.JournalMessages.SourceNode.Builder builderForValue) {
if (sourceNodesBuilder_ == null) {
ensureSourceNodesIsMutable();
sourceNodes_.add(index, builderForValue.build());
onChanged();
} else {
sourceNodesBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .org.graylog2.plugin.journal.SourceNode source_nodes = 6;
*
*
* the list of graylog2 nodes which have handled the message (radios, servers) in receive order
*
*/
public Builder addAllSourceNodes(
java.lang.Iterable extends org.graylog2.plugin.journal.JournalMessages.SourceNode> values) {
if (sourceNodesBuilder_ == null) {
ensureSourceNodesIsMutable();
super.addAll(values, sourceNodes_);
onChanged();
} else {
sourceNodesBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .org.graylog2.plugin.journal.SourceNode source_nodes = 6;
*
*
* the list of graylog2 nodes which have handled the message (radios, servers) in receive order
*
*/
public Builder clearSourceNodes() {
if (sourceNodesBuilder_ == null) {
sourceNodes_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000020);
onChanged();
} else {
sourceNodesBuilder_.clear();
}
return this;
}
/**
* repeated .org.graylog2.plugin.journal.SourceNode source_nodes = 6;
*
*
* the list of graylog2 nodes which have handled the message (radios, servers) in receive order
*
*/
public Builder removeSourceNodes(int index) {
if (sourceNodesBuilder_ == null) {
ensureSourceNodesIsMutable();
sourceNodes_.remove(index);
onChanged();
} else {
sourceNodesBuilder_.remove(index);
}
return this;
}
/**
* repeated .org.graylog2.plugin.journal.SourceNode source_nodes = 6;
*
*
* the list of graylog2 nodes which have handled the message (radios, servers) in receive order
*
*/
public org.graylog2.plugin.journal.JournalMessages.SourceNode.Builder getSourceNodesBuilder(
int index) {
return getSourceNodesFieldBuilder().getBuilder(index);
}
/**
* repeated .org.graylog2.plugin.journal.SourceNode source_nodes = 6;
*
*
* the list of graylog2 nodes which have handled the message (radios, servers) in receive order
*
*/
public org.graylog2.plugin.journal.JournalMessages.SourceNodeOrBuilder getSourceNodesOrBuilder(
int index) {
if (sourceNodesBuilder_ == null) {
return sourceNodes_.get(index); } else {
return sourceNodesBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .org.graylog2.plugin.journal.SourceNode source_nodes = 6;
*
*
* the list of graylog2 nodes which have handled the message (radios, servers) in receive order
*
*/
public java.util.List extends org.graylog2.plugin.journal.JournalMessages.SourceNodeOrBuilder>
getSourceNodesOrBuilderList() {
if (sourceNodesBuilder_ != null) {
return sourceNodesBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(sourceNodes_);
}
}
/**
* repeated .org.graylog2.plugin.journal.SourceNode source_nodes = 6;
*
*
* the list of graylog2 nodes which have handled the message (radios, servers) in receive order
*
*/
public org.graylog2.plugin.journal.JournalMessages.SourceNode.Builder addSourceNodesBuilder() {
return getSourceNodesFieldBuilder().addBuilder(
org.graylog2.plugin.journal.JournalMessages.SourceNode.getDefaultInstance());
}
/**
* repeated .org.graylog2.plugin.journal.SourceNode source_nodes = 6;
*
*
* the list of graylog2 nodes which have handled the message (radios, servers) in receive order
*
*/
public org.graylog2.plugin.journal.JournalMessages.SourceNode.Builder addSourceNodesBuilder(
int index) {
return getSourceNodesFieldBuilder().addBuilder(
index, org.graylog2.plugin.journal.JournalMessages.SourceNode.getDefaultInstance());
}
/**
* repeated .org.graylog2.plugin.journal.SourceNode source_nodes = 6;
*
*
* the list of graylog2 nodes which have handled the message (radios, servers) in receive order
*
*/
public java.util.List
getSourceNodesBuilderList() {
return getSourceNodesFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
org.graylog2.plugin.journal.JournalMessages.SourceNode, org.graylog2.plugin.journal.JournalMessages.SourceNode.Builder, org.graylog2.plugin.journal.JournalMessages.SourceNodeOrBuilder>
getSourceNodesFieldBuilder() {
if (sourceNodesBuilder_ == null) {
sourceNodesBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
org.graylog2.plugin.journal.JournalMessages.SourceNode, org.graylog2.plugin.journal.JournalMessages.SourceNode.Builder, org.graylog2.plugin.journal.JournalMessages.SourceNodeOrBuilder>(
sourceNodes_,
((bitField0_ & 0x00000020) == 0x00000020),
getParentForChildren(),
isClean());
sourceNodes_ = null;
}
return sourceNodesBuilder_;
}
// optional .org.graylog2.plugin.journal.RemoteAddress remote = 7;
private org.graylog2.plugin.journal.JournalMessages.RemoteAddress remote_ = org.graylog2.plugin.journal.JournalMessages.RemoteAddress.getDefaultInstance();
private com.google.protobuf.SingleFieldBuilder<
org.graylog2.plugin.journal.JournalMessages.RemoteAddress, org.graylog2.plugin.journal.JournalMessages.RemoteAddress.Builder, org.graylog2.plugin.journal.JournalMessages.RemoteAddressOrBuilder> remoteBuilder_;
/**
* optional .org.graylog2.plugin.journal.RemoteAddress remote = 7;
*/
public boolean hasRemote() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
* optional .org.graylog2.plugin.journal.RemoteAddress remote = 7;
*/
public org.graylog2.plugin.journal.JournalMessages.RemoteAddress getRemote() {
if (remoteBuilder_ == null) {
return remote_;
} else {
return remoteBuilder_.getMessage();
}
}
/**
* optional .org.graylog2.plugin.journal.RemoteAddress remote = 7;
*/
public Builder setRemote(org.graylog2.plugin.journal.JournalMessages.RemoteAddress value) {
if (remoteBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
remote_ = value;
onChanged();
} else {
remoteBuilder_.setMessage(value);
}
bitField0_ |= 0x00000040;
return this;
}
/**
* optional .org.graylog2.plugin.journal.RemoteAddress remote = 7;
*/
public Builder setRemote(
org.graylog2.plugin.journal.JournalMessages.RemoteAddress.Builder builderForValue) {
if (remoteBuilder_ == null) {
remote_ = builderForValue.build();
onChanged();
} else {
remoteBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000040;
return this;
}
/**
* optional .org.graylog2.plugin.journal.RemoteAddress remote = 7;
*/
public Builder mergeRemote(org.graylog2.plugin.journal.JournalMessages.RemoteAddress value) {
if (remoteBuilder_ == null) {
if (((bitField0_ & 0x00000040) == 0x00000040) &&
remote_ != org.graylog2.plugin.journal.JournalMessages.RemoteAddress.getDefaultInstance()) {
remote_ =
org.graylog2.plugin.journal.JournalMessages.RemoteAddress.newBuilder(remote_).mergeFrom(value).buildPartial();
} else {
remote_ = value;
}
onChanged();
} else {
remoteBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000040;
return this;
}
/**
* optional .org.graylog2.plugin.journal.RemoteAddress remote = 7;
*/
public Builder clearRemote() {
if (remoteBuilder_ == null) {
remote_ = org.graylog2.plugin.journal.JournalMessages.RemoteAddress.getDefaultInstance();
onChanged();
} else {
remoteBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000040);
return this;
}
/**
* optional .org.graylog2.plugin.journal.RemoteAddress remote = 7;
*/
public org.graylog2.plugin.journal.JournalMessages.RemoteAddress.Builder getRemoteBuilder() {
bitField0_ |= 0x00000040;
onChanged();
return getRemoteFieldBuilder().getBuilder();
}
/**
* optional .org.graylog2.plugin.journal.RemoteAddress remote = 7;
*/
public org.graylog2.plugin.journal.JournalMessages.RemoteAddressOrBuilder getRemoteOrBuilder() {
if (remoteBuilder_ != null) {
return remoteBuilder_.getMessageOrBuilder();
} else {
return remote_;
}
}
/**
* optional .org.graylog2.plugin.journal.RemoteAddress remote = 7;
*/
private com.google.protobuf.SingleFieldBuilder<
org.graylog2.plugin.journal.JournalMessages.RemoteAddress, org.graylog2.plugin.journal.JournalMessages.RemoteAddress.Builder, org.graylog2.plugin.journal.JournalMessages.RemoteAddressOrBuilder>
getRemoteFieldBuilder() {
if (remoteBuilder_ == null) {
remoteBuilder_ = new com.google.protobuf.SingleFieldBuilder<
org.graylog2.plugin.journal.JournalMessages.RemoteAddress, org.graylog2.plugin.journal.JournalMessages.RemoteAddress.Builder, org.graylog2.plugin.journal.JournalMessages.RemoteAddressOrBuilder>(
remote_,
getParentForChildren(),
isClean());
remote_ = null;
}
return remoteBuilder_;
}
// optional bytes payload = 8;
private com.google.protobuf.ByteString payload_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes payload = 8;
*/
public boolean hasPayload() {
return ((bitField0_ & 0x00000080) == 0x00000080);
}
/**
* optional bytes payload = 8;
*/
public com.google.protobuf.ByteString getPayload() {
return payload_;
}
/**
* optional bytes payload = 8;
*/
public Builder setPayload(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000080;
payload_ = value;
onChanged();
return this;
}
/**
* optional bytes payload = 8;
*/
public Builder clearPayload() {
bitField0_ = (bitField0_ & ~0x00000080);
payload_ = getDefaultInstance().getPayload();
onChanged();
return this;
}
// optional uint32 sequence_nr = 9;
private int sequenceNr_ ;
/**
* optional uint32 sequence_nr = 9;
*/
public boolean hasSequenceNr() {
return ((bitField0_ & 0x00000100) == 0x00000100);
}
/**
* optional uint32 sequence_nr = 9;
*/
public int getSequenceNr() {
return sequenceNr_;
}
/**
* optional uint32 sequence_nr = 9;
*/
public Builder setSequenceNr(int value) {
bitField0_ |= 0x00000100;
sequenceNr_ = value;
onChanged();
return this;
}
/**
* optional uint32 sequence_nr = 9;
*/
public Builder clearSequenceNr() {
bitField0_ = (bitField0_ & ~0x00000100);
sequenceNr_ = 0;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:org.graylog2.plugin.journal.JournalMessage)
}
static {
defaultInstance = new JournalMessage(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:org.graylog2.plugin.journal.JournalMessage)
}
public interface RemoteAddressOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// optional bytes address = 1;
/**
* optional bytes address = 1;
*
*
* the original remote (IP) address of the message sender, unresolved,
*
*/
boolean hasAddress();
/**
* optional bytes address = 1;
*
*
* the original remote (IP) address of the message sender, unresolved,
*
*/
com.google.protobuf.ByteString getAddress();
// optional uint32 port = 2;
/**
* optional uint32 port = 2;
*
*
* the port of the sender, if available/applicable
*
*/
boolean hasPort();
/**
* optional uint32 port = 2;
*
*
* the port of the sender, if available/applicable
*
*/
int getPort();
// optional string resolved = 3;
/**
* optional string resolved = 3;
*
*
* a processing node can optionally resolve the address early
*
*/
boolean hasResolved();
/**
* optional string resolved = 3;
*
*
* a processing node can optionally resolve the address early
*
*/
java.lang.String getResolved();
/**
* optional string resolved = 3;
*
*
* a processing node can optionally resolve the address early
*
*/
com.google.protobuf.ByteString
getResolvedBytes();
}
/**
* Protobuf type {@code org.graylog2.plugin.journal.RemoteAddress}
*/
public static final class RemoteAddress extends
com.google.protobuf.GeneratedMessage
implements RemoteAddressOrBuilder {
// Use RemoteAddress.newBuilder() to construct.
private RemoteAddress(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private RemoteAddress(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final RemoteAddress defaultInstance;
public static RemoteAddress getDefaultInstance() {
return defaultInstance;
}
public RemoteAddress getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private RemoteAddress(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
bitField0_ |= 0x00000001;
address_ = input.readBytes();
break;
}
case 16: {
bitField0_ |= 0x00000002;
port_ = input.readUInt32();
break;
}
case 26: {
bitField0_ |= 0x00000004;
resolved_ = input.readBytes();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.graylog2.plugin.journal.JournalMessages.internal_static_org_graylog2_plugin_journal_RemoteAddress_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.graylog2.plugin.journal.JournalMessages.internal_static_org_graylog2_plugin_journal_RemoteAddress_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.graylog2.plugin.journal.JournalMessages.RemoteAddress.class, org.graylog2.plugin.journal.JournalMessages.RemoteAddress.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public RemoteAddress parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new RemoteAddress(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// optional bytes address = 1;
public static final int ADDRESS_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString address_;
/**
* optional bytes address = 1;
*
*
* the original remote (IP) address of the message sender, unresolved,
*
*/
public boolean hasAddress() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional bytes address = 1;
*
*
* the original remote (IP) address of the message sender, unresolved,
*
*/
public com.google.protobuf.ByteString getAddress() {
return address_;
}
// optional uint32 port = 2;
public static final int PORT_FIELD_NUMBER = 2;
private int port_;
/**
* optional uint32 port = 2;
*
*
* the port of the sender, if available/applicable
*
*/
public boolean hasPort() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional uint32 port = 2;
*
*
* the port of the sender, if available/applicable
*
*/
public int getPort() {
return port_;
}
// optional string resolved = 3;
public static final int RESOLVED_FIELD_NUMBER = 3;
private java.lang.Object resolved_;
/**
* optional string resolved = 3;
*
*
* a processing node can optionally resolve the address early
*
*/
public boolean hasResolved() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional string resolved = 3;
*
*
* a processing node can optionally resolve the address early
*
*/
public java.lang.String getResolved() {
java.lang.Object ref = resolved_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
resolved_ = s;
}
return s;
}
}
/**
* optional string resolved = 3;
*
*
* a processing node can optionally resolve the address early
*
*/
public com.google.protobuf.ByteString
getResolvedBytes() {
java.lang.Object ref = resolved_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
resolved_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private void initFields() {
address_ = com.google.protobuf.ByteString.EMPTY;
port_ = 0;
resolved_ = "";
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(1, address_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeUInt32(2, port_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeBytes(3, getResolvedBytes());
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, address_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(2, port_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(3, getResolvedBytes());
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static org.graylog2.plugin.journal.JournalMessages.RemoteAddress parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.graylog2.plugin.journal.JournalMessages.RemoteAddress parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.graylog2.plugin.journal.JournalMessages.RemoteAddress parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.graylog2.plugin.journal.JournalMessages.RemoteAddress parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.graylog2.plugin.journal.JournalMessages.RemoteAddress parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.graylog2.plugin.journal.JournalMessages.RemoteAddress parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.graylog2.plugin.journal.JournalMessages.RemoteAddress parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.graylog2.plugin.journal.JournalMessages.RemoteAddress parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.graylog2.plugin.journal.JournalMessages.RemoteAddress parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.graylog2.plugin.journal.JournalMessages.RemoteAddress parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(org.graylog2.plugin.journal.JournalMessages.RemoteAddress prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code org.graylog2.plugin.journal.RemoteAddress}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements org.graylog2.plugin.journal.JournalMessages.RemoteAddressOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.graylog2.plugin.journal.JournalMessages.internal_static_org_graylog2_plugin_journal_RemoteAddress_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.graylog2.plugin.journal.JournalMessages.internal_static_org_graylog2_plugin_journal_RemoteAddress_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.graylog2.plugin.journal.JournalMessages.RemoteAddress.class, org.graylog2.plugin.journal.JournalMessages.RemoteAddress.Builder.class);
}
// Construct using org.graylog2.plugin.journal.JournalMessages.RemoteAddress.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
address_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
port_ = 0;
bitField0_ = (bitField0_ & ~0x00000002);
resolved_ = "";
bitField0_ = (bitField0_ & ~0x00000004);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.graylog2.plugin.journal.JournalMessages.internal_static_org_graylog2_plugin_journal_RemoteAddress_descriptor;
}
public org.graylog2.plugin.journal.JournalMessages.RemoteAddress getDefaultInstanceForType() {
return org.graylog2.plugin.journal.JournalMessages.RemoteAddress.getDefaultInstance();
}
public org.graylog2.plugin.journal.JournalMessages.RemoteAddress build() {
org.graylog2.plugin.journal.JournalMessages.RemoteAddress result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.graylog2.plugin.journal.JournalMessages.RemoteAddress buildPartial() {
org.graylog2.plugin.journal.JournalMessages.RemoteAddress result = new org.graylog2.plugin.journal.JournalMessages.RemoteAddress(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.address_ = address_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.port_ = port_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.resolved_ = resolved_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.graylog2.plugin.journal.JournalMessages.RemoteAddress) {
return mergeFrom((org.graylog2.plugin.journal.JournalMessages.RemoteAddress)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.graylog2.plugin.journal.JournalMessages.RemoteAddress other) {
if (other == org.graylog2.plugin.journal.JournalMessages.RemoteAddress.getDefaultInstance()) return this;
if (other.hasAddress()) {
setAddress(other.getAddress());
}
if (other.hasPort()) {
setPort(other.getPort());
}
if (other.hasResolved()) {
bitField0_ |= 0x00000004;
resolved_ = other.resolved_;
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.graylog2.plugin.journal.JournalMessages.RemoteAddress parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.graylog2.plugin.journal.JournalMessages.RemoteAddress) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// optional bytes address = 1;
private com.google.protobuf.ByteString address_ = com.google.protobuf.ByteString.EMPTY;
/**
* optional bytes address = 1;
*
*
* the original remote (IP) address of the message sender, unresolved,
*
*/
public boolean hasAddress() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional bytes address = 1;
*
*
* the original remote (IP) address of the message sender, unresolved,
*
*/
public com.google.protobuf.ByteString getAddress() {
return address_;
}
/**
* optional bytes address = 1;
*
*
* the original remote (IP) address of the message sender, unresolved,
*
*/
public Builder setAddress(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
address_ = value;
onChanged();
return this;
}
/**
* optional bytes address = 1;
*
*
* the original remote (IP) address of the message sender, unresolved,
*
*/
public Builder clearAddress() {
bitField0_ = (bitField0_ & ~0x00000001);
address_ = getDefaultInstance().getAddress();
onChanged();
return this;
}
// optional uint32 port = 2;
private int port_ ;
/**
* optional uint32 port = 2;
*
*
* the port of the sender, if available/applicable
*
*/
public boolean hasPort() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional uint32 port = 2;
*
*
* the port of the sender, if available/applicable
*
*/
public int getPort() {
return port_;
}
/**
* optional uint32 port = 2;
*
*
* the port of the sender, if available/applicable
*
*/
public Builder setPort(int value) {
bitField0_ |= 0x00000002;
port_ = value;
onChanged();
return this;
}
/**
* optional uint32 port = 2;
*
*
* the port of the sender, if available/applicable
*
*/
public Builder clearPort() {
bitField0_ = (bitField0_ & ~0x00000002);
port_ = 0;
onChanged();
return this;
}
// optional string resolved = 3;
private java.lang.Object resolved_ = "";
/**
* optional string resolved = 3;
*
*
* a processing node can optionally resolve the address early
*
*/
public boolean hasResolved() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional string resolved = 3;
*
*
* a processing node can optionally resolve the address early
*
*/
public java.lang.String getResolved() {
java.lang.Object ref = resolved_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
resolved_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string resolved = 3;
*
*
* a processing node can optionally resolve the address early
*
*/
public com.google.protobuf.ByteString
getResolvedBytes() {
java.lang.Object ref = resolved_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
resolved_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string resolved = 3;
*
*
* a processing node can optionally resolve the address early
*
*/
public Builder setResolved(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
resolved_ = value;
onChanged();
return this;
}
/**
* optional string resolved = 3;
*
*
* a processing node can optionally resolve the address early
*
*/
public Builder clearResolved() {
bitField0_ = (bitField0_ & ~0x00000004);
resolved_ = getDefaultInstance().getResolved();
onChanged();
return this;
}
/**
* optional string resolved = 3;
*
*
* a processing node can optionally resolve the address early
*
*/
public Builder setResolvedBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
resolved_ = value;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:org.graylog2.plugin.journal.RemoteAddress)
}
static {
defaultInstance = new RemoteAddress(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:org.graylog2.plugin.journal.RemoteAddress)
}
public interface CodecInfoOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// optional string name = 1;
/**
* optional string name = 1;
*/
boolean hasName();
/**
* optional string name = 1;
*/
java.lang.String getName();
/**
* optional string name = 1;
*/
com.google.protobuf.ByteString
getNameBytes();
// optional string config = 2;
/**
* optional string config = 2;
*
*
* JSON description of configuration settings necessary to create the codec with
* for optimal performance make sure the serialization is stable, i.e. same config == same serialization bytes
*
*/
boolean hasConfig();
/**
* optional string config = 2;
*
*
* JSON description of configuration settings necessary to create the codec with
* for optimal performance make sure the serialization is stable, i.e. same config == same serialization bytes
*
*/
java.lang.String getConfig();
/**
* optional string config = 2;
*
*
* JSON description of configuration settings necessary to create the codec with
* for optimal performance make sure the serialization is stable, i.e. same config == same serialization bytes
*
*/
com.google.protobuf.ByteString
getConfigBytes();
}
/**
* Protobuf type {@code org.graylog2.plugin.journal.CodecInfo}
*/
public static final class CodecInfo extends
com.google.protobuf.GeneratedMessage
implements CodecInfoOrBuilder {
// Use CodecInfo.newBuilder() to construct.
private CodecInfo(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private CodecInfo(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final CodecInfo defaultInstance;
public static CodecInfo getDefaultInstance() {
return defaultInstance;
}
public CodecInfo getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private CodecInfo(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
bitField0_ |= 0x00000001;
name_ = input.readBytes();
break;
}
case 18: {
bitField0_ |= 0x00000002;
config_ = input.readBytes();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.graylog2.plugin.journal.JournalMessages.internal_static_org_graylog2_plugin_journal_CodecInfo_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.graylog2.plugin.journal.JournalMessages.internal_static_org_graylog2_plugin_journal_CodecInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.graylog2.plugin.journal.JournalMessages.CodecInfo.class, org.graylog2.plugin.journal.JournalMessages.CodecInfo.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public CodecInfo parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new CodecInfo(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
private int bitField0_;
// optional string name = 1;
public static final int NAME_FIELD_NUMBER = 1;
private java.lang.Object name_;
/**
* optional string name = 1;
*/
public boolean hasName() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional string name = 1;
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
name_ = s;
}
return s;
}
}
/**
* optional string name = 1;
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional string config = 2;
public static final int CONFIG_FIELD_NUMBER = 2;
private java.lang.Object config_;
/**
* optional string config = 2;
*
*
* JSON description of configuration settings necessary to create the codec with
* for optimal performance make sure the serialization is stable, i.e. same config == same serialization bytes
*
*/
public boolean hasConfig() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional string config = 2;
*
*
* JSON description of configuration settings necessary to create the codec with
* for optimal performance make sure the serialization is stable, i.e. same config == same serialization bytes
*
*/
public java.lang.String getConfig() {
java.lang.Object ref = config_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
config_ = s;
}
return s;
}
}
/**
* optional string config = 2;
*
*
* JSON description of configuration settings necessary to create the codec with
* for optimal performance make sure the serialization is stable, i.e. same config == same serialization bytes
*
*/
public com.google.protobuf.ByteString
getConfigBytes() {
java.lang.Object ref = config_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
config_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private void initFields() {
name_ = "";
config_ = "";
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(1, getNameBytes());
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeBytes(2, getConfigBytes());
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, getNameBytes());
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, getConfigBytes());
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static org.graylog2.plugin.journal.JournalMessages.CodecInfo parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.graylog2.plugin.journal.JournalMessages.CodecInfo parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.graylog2.plugin.journal.JournalMessages.CodecInfo parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.graylog2.plugin.journal.JournalMessages.CodecInfo parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.graylog2.plugin.journal.JournalMessages.CodecInfo parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.graylog2.plugin.journal.JournalMessages.CodecInfo parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.graylog2.plugin.journal.JournalMessages.CodecInfo parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.graylog2.plugin.journal.JournalMessages.CodecInfo parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.graylog2.plugin.journal.JournalMessages.CodecInfo parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.graylog2.plugin.journal.JournalMessages.CodecInfo parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(org.graylog2.plugin.journal.JournalMessages.CodecInfo prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code org.graylog2.plugin.journal.CodecInfo}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements org.graylog2.plugin.journal.JournalMessages.CodecInfoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.graylog2.plugin.journal.JournalMessages.internal_static_org_graylog2_plugin_journal_CodecInfo_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.graylog2.plugin.journal.JournalMessages.internal_static_org_graylog2_plugin_journal_CodecInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.graylog2.plugin.journal.JournalMessages.CodecInfo.class, org.graylog2.plugin.journal.JournalMessages.CodecInfo.Builder.class);
}
// Construct using org.graylog2.plugin.journal.JournalMessages.CodecInfo.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
name_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
config_ = "";
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.graylog2.plugin.journal.JournalMessages.internal_static_org_graylog2_plugin_journal_CodecInfo_descriptor;
}
public org.graylog2.plugin.journal.JournalMessages.CodecInfo getDefaultInstanceForType() {
return org.graylog2.plugin.journal.JournalMessages.CodecInfo.getDefaultInstance();
}
public org.graylog2.plugin.journal.JournalMessages.CodecInfo build() {
org.graylog2.plugin.journal.JournalMessages.CodecInfo result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.graylog2.plugin.journal.JournalMessages.CodecInfo buildPartial() {
org.graylog2.plugin.journal.JournalMessages.CodecInfo result = new org.graylog2.plugin.journal.JournalMessages.CodecInfo(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.name_ = name_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.config_ = config_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.graylog2.plugin.journal.JournalMessages.CodecInfo) {
return mergeFrom((org.graylog2.plugin.journal.JournalMessages.CodecInfo)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.graylog2.plugin.journal.JournalMessages.CodecInfo other) {
if (other == org.graylog2.plugin.journal.JournalMessages.CodecInfo.getDefaultInstance()) return this;
if (other.hasName()) {
bitField0_ |= 0x00000001;
name_ = other.name_;
onChanged();
}
if (other.hasConfig()) {
bitField0_ |= 0x00000002;
config_ = other.config_;
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.graylog2.plugin.journal.JournalMessages.CodecInfo parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.graylog2.plugin.journal.JournalMessages.CodecInfo) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// optional string name = 1;
private java.lang.Object name_ = "";
/**
* optional string name = 1;
*/
public boolean hasName() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional string name = 1;
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
name_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string name = 1;
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string name = 1;
*/
public Builder setName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
name_ = value;
onChanged();
return this;
}
/**
* optional string name = 1;
*/
public Builder clearName() {
bitField0_ = (bitField0_ & ~0x00000001);
name_ = getDefaultInstance().getName();
onChanged();
return this;
}
/**
* optional string name = 1;
*/
public Builder setNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
name_ = value;
onChanged();
return this;
}
// optional string config = 2;
private java.lang.Object config_ = "";
/**
* optional string config = 2;
*
*
* JSON description of configuration settings necessary to create the codec with
* for optimal performance make sure the serialization is stable, i.e. same config == same serialization bytes
*
*/
public boolean hasConfig() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional string config = 2;
*
*
* JSON description of configuration settings necessary to create the codec with
* for optimal performance make sure the serialization is stable, i.e. same config == same serialization bytes
*
*/
public java.lang.String getConfig() {
java.lang.Object ref = config_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
config_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string config = 2;
*
*
* JSON description of configuration settings necessary to create the codec with
* for optimal performance make sure the serialization is stable, i.e. same config == same serialization bytes
*
*/
public com.google.protobuf.ByteString
getConfigBytes() {
java.lang.Object ref = config_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
config_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string config = 2;
*
*
* JSON description of configuration settings necessary to create the codec with
* for optimal performance make sure the serialization is stable, i.e. same config == same serialization bytes
*
*/
public Builder setConfig(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
config_ = value;
onChanged();
return this;
}
/**
* optional string config = 2;
*
*
* JSON description of configuration settings necessary to create the codec with
* for optimal performance make sure the serialization is stable, i.e. same config == same serialization bytes
*
*/
public Builder clearConfig() {
bitField0_ = (bitField0_ & ~0x00000002);
config_ = getDefaultInstance().getConfig();
onChanged();
return this;
}
/**
* optional string config = 2;
*
*
* JSON description of configuration settings necessary to create the codec with
* for optimal performance make sure the serialization is stable, i.e. same config == same serialization bytes
*
*/
public Builder setConfigBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
config_ = value;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:org.graylog2.plugin.journal.CodecInfo)
}
static {
defaultInstance = new CodecInfo(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:org.graylog2.plugin.journal.CodecInfo)
}
public interface SourceNodeOrBuilder
extends com.google.protobuf.MessageOrBuilder {
// optional string id = 1;
/**
* optional string id = 1;
*/
boolean hasId();
/**
* optional string id = 1;
*/
java.lang.String getId();
/**
* optional string id = 1;
*/
com.google.protobuf.ByteString
getIdBytes();
// optional .org.graylog2.plugin.journal.SourceNode.Type type = 2 [default = SERVER];
/**
* optional .org.graylog2.plugin.journal.SourceNode.Type type = 2 [default = SERVER];
*/
boolean hasType();
/**
* optional .org.graylog2.plugin.journal.SourceNode.Type type = 2 [default = SERVER];
*/
org.graylog2.plugin.journal.JournalMessages.SourceNode.Type getType();
// optional string input_id = 3;
/**
* optional string input_id = 3;
*/
boolean hasInputId();
/**
* optional string input_id = 3;
*/
java.lang.String getInputId();
/**
* optional string input_id = 3;
*/
com.google.protobuf.ByteString
getInputIdBytes();
}
/**
* Protobuf type {@code org.graylog2.plugin.journal.SourceNode}
*/
public static final class SourceNode extends
com.google.protobuf.GeneratedMessage
implements SourceNodeOrBuilder {
// Use SourceNode.newBuilder() to construct.
private SourceNode(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
this.unknownFields = builder.getUnknownFields();
}
private SourceNode(boolean noInit) { this.unknownFields = com.google.protobuf.UnknownFieldSet.getDefaultInstance(); }
private static final SourceNode defaultInstance;
public static SourceNode getDefaultInstance() {
return defaultInstance;
}
public SourceNode getDefaultInstanceForType() {
return defaultInstance;
}
private final com.google.protobuf.UnknownFieldSet unknownFields;
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private SourceNode(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
initFields();
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownField(input, unknownFields,
extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
bitField0_ |= 0x00000001;
id_ = input.readBytes();
break;
}
case 16: {
int rawValue = input.readEnum();
org.graylog2.plugin.journal.JournalMessages.SourceNode.Type value = org.graylog2.plugin.journal.JournalMessages.SourceNode.Type.valueOf(rawValue);
if (value == null) {
unknownFields.mergeVarintField(2, rawValue);
} else {
bitField0_ |= 0x00000002;
type_ = value;
}
break;
}
case 26: {
bitField0_ |= 0x00000004;
inputId_ = input.readBytes();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e.getMessage()).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.graylog2.plugin.journal.JournalMessages.internal_static_org_graylog2_plugin_journal_SourceNode_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.graylog2.plugin.journal.JournalMessages.internal_static_org_graylog2_plugin_journal_SourceNode_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.graylog2.plugin.journal.JournalMessages.SourceNode.class, org.graylog2.plugin.journal.JournalMessages.SourceNode.Builder.class);
}
public static com.google.protobuf.Parser PARSER =
new com.google.protobuf.AbstractParser() {
public SourceNode parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new SourceNode(input, extensionRegistry);
}
};
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
/**
* Protobuf enum {@code org.graylog2.plugin.journal.SourceNode.Type}
*/
public enum Type
implements com.google.protobuf.ProtocolMessageEnum {
/**
* SERVER = 0;
*/
SERVER(0, 0),
/**
* RADIO = 1;
*/
RADIO(1, 1),
;
/**
* SERVER = 0;
*/
public static final int SERVER_VALUE = 0;
/**
* RADIO = 1;
*/
public static final int RADIO_VALUE = 1;
public final int getNumber() { return value; }
public static Type valueOf(int value) {
switch (value) {
case 0: return SERVER;
case 1: return RADIO;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static com.google.protobuf.Internal.EnumLiteMap
internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public Type findValueByNumber(int number) {
return Type.valueOf(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(index);
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return org.graylog2.plugin.journal.JournalMessages.SourceNode.getDescriptor().getEnumTypes().get(0);
}
private static final Type[] VALUES = values();
public static Type valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int index;
private final int value;
private Type(int index, int value) {
this.index = index;
this.value = value;
}
// @@protoc_insertion_point(enum_scope:org.graylog2.plugin.journal.SourceNode.Type)
}
private int bitField0_;
// optional string id = 1;
public static final int ID_FIELD_NUMBER = 1;
private java.lang.Object id_;
/**
* optional string id = 1;
*/
public boolean hasId() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional string id = 1;
*/
public java.lang.String getId() {
java.lang.Object ref = id_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
id_ = s;
}
return s;
}
}
/**
* optional string id = 1;
*/
public com.google.protobuf.ByteString
getIdBytes() {
java.lang.Object ref = id_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
id_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
// optional .org.graylog2.plugin.journal.SourceNode.Type type = 2 [default = SERVER];
public static final int TYPE_FIELD_NUMBER = 2;
private org.graylog2.plugin.journal.JournalMessages.SourceNode.Type type_;
/**
* optional .org.graylog2.plugin.journal.SourceNode.Type type = 2 [default = SERVER];
*/
public boolean hasType() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional .org.graylog2.plugin.journal.SourceNode.Type type = 2 [default = SERVER];
*/
public org.graylog2.plugin.journal.JournalMessages.SourceNode.Type getType() {
return type_;
}
// optional string input_id = 3;
public static final int INPUT_ID_FIELD_NUMBER = 3;
private java.lang.Object inputId_;
/**
* optional string input_id = 3;
*/
public boolean hasInputId() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional string input_id = 3;
*/
public java.lang.String getInputId() {
java.lang.Object ref = inputId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
inputId_ = s;
}
return s;
}
}
/**
* optional string input_id = 3;
*/
public com.google.protobuf.ByteString
getInputIdBytes() {
java.lang.Object ref = inputId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
inputId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private void initFields() {
id_ = "";
type_ = org.graylog2.plugin.journal.JournalMessages.SourceNode.Type.SERVER;
inputId_ = "";
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized != -1) return isInitialized == 1;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(1, getIdBytes());
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeEnum(2, type_.getNumber());
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeBytes(3, getInputIdBytes());
}
getUnknownFields().writeTo(output);
}
private int memoizedSerializedSize = -1;
public int getSerializedSize() {
int size = memoizedSerializedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, getIdBytes());
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(2, type_.getNumber());
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(3, getInputIdBytes());
}
size += getUnknownFields().getSerializedSize();
memoizedSerializedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
protected java.lang.Object writeReplace()
throws java.io.ObjectStreamException {
return super.writeReplace();
}
public static org.graylog2.plugin.journal.JournalMessages.SourceNode parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.graylog2.plugin.journal.JournalMessages.SourceNode parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.graylog2.plugin.journal.JournalMessages.SourceNode parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.graylog2.plugin.journal.JournalMessages.SourceNode parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.graylog2.plugin.journal.JournalMessages.SourceNode parseFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.graylog2.plugin.journal.JournalMessages.SourceNode parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static org.graylog2.plugin.journal.JournalMessages.SourceNode parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input);
}
public static org.graylog2.plugin.journal.JournalMessages.SourceNode parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseDelimitedFrom(input, extensionRegistry);
}
public static org.graylog2.plugin.journal.JournalMessages.SourceNode parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return PARSER.parseFrom(input);
}
public static org.graylog2.plugin.journal.JournalMessages.SourceNode parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return PARSER.parseFrom(input, extensionRegistry);
}
public static Builder newBuilder() { return Builder.create(); }
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder(org.graylog2.plugin.journal.JournalMessages.SourceNode prototype) {
return newBuilder().mergeFrom(prototype);
}
public Builder toBuilder() { return newBuilder(this); }
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code org.graylog2.plugin.journal.SourceNode}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder
implements org.graylog2.plugin.journal.JournalMessages.SourceNodeOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.graylog2.plugin.journal.JournalMessages.internal_static_org_graylog2_plugin_journal_SourceNode_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.graylog2.plugin.journal.JournalMessages.internal_static_org_graylog2_plugin_journal_SourceNode_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.graylog2.plugin.journal.JournalMessages.SourceNode.class, org.graylog2.plugin.journal.JournalMessages.SourceNode.Builder.class);
}
// Construct using org.graylog2.plugin.journal.JournalMessages.SourceNode.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
}
}
private static Builder create() {
return new Builder();
}
public Builder clear() {
super.clear();
id_ = "";
bitField0_ = (bitField0_ & ~0x00000001);
type_ = org.graylog2.plugin.journal.JournalMessages.SourceNode.Type.SERVER;
bitField0_ = (bitField0_ & ~0x00000002);
inputId_ = "";
bitField0_ = (bitField0_ & ~0x00000004);
return this;
}
public Builder clone() {
return create().mergeFrom(buildPartial());
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.graylog2.plugin.journal.JournalMessages.internal_static_org_graylog2_plugin_journal_SourceNode_descriptor;
}
public org.graylog2.plugin.journal.JournalMessages.SourceNode getDefaultInstanceForType() {
return org.graylog2.plugin.journal.JournalMessages.SourceNode.getDefaultInstance();
}
public org.graylog2.plugin.journal.JournalMessages.SourceNode build() {
org.graylog2.plugin.journal.JournalMessages.SourceNode result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.graylog2.plugin.journal.JournalMessages.SourceNode buildPartial() {
org.graylog2.plugin.journal.JournalMessages.SourceNode result = new org.graylog2.plugin.journal.JournalMessages.SourceNode(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.id_ = id_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.type_ = type_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.inputId_ = inputId_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.graylog2.plugin.journal.JournalMessages.SourceNode) {
return mergeFrom((org.graylog2.plugin.journal.JournalMessages.SourceNode)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.graylog2.plugin.journal.JournalMessages.SourceNode other) {
if (other == org.graylog2.plugin.journal.JournalMessages.SourceNode.getDefaultInstance()) return this;
if (other.hasId()) {
bitField0_ |= 0x00000001;
id_ = other.id_;
onChanged();
}
if (other.hasType()) {
setType(other.getType());
}
if (other.hasInputId()) {
bitField0_ |= 0x00000004;
inputId_ = other.inputId_;
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.graylog2.plugin.journal.JournalMessages.SourceNode parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.graylog2.plugin.journal.JournalMessages.SourceNode) e.getUnfinishedMessage();
throw e;
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
// optional string id = 1;
private java.lang.Object id_ = "";
/**
* optional string id = 1;
*/
public boolean hasId() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* optional string id = 1;
*/
public java.lang.String getId() {
java.lang.Object ref = id_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
id_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string id = 1;
*/
public com.google.protobuf.ByteString
getIdBytes() {
java.lang.Object ref = id_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
id_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string id = 1;
*/
public Builder setId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
id_ = value;
onChanged();
return this;
}
/**
* optional string id = 1;
*/
public Builder clearId() {
bitField0_ = (bitField0_ & ~0x00000001);
id_ = getDefaultInstance().getId();
onChanged();
return this;
}
/**
* optional string id = 1;
*/
public Builder setIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
id_ = value;
onChanged();
return this;
}
// optional .org.graylog2.plugin.journal.SourceNode.Type type = 2 [default = SERVER];
private org.graylog2.plugin.journal.JournalMessages.SourceNode.Type type_ = org.graylog2.plugin.journal.JournalMessages.SourceNode.Type.SERVER;
/**
* optional .org.graylog2.plugin.journal.SourceNode.Type type = 2 [default = SERVER];
*/
public boolean hasType() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* optional .org.graylog2.plugin.journal.SourceNode.Type type = 2 [default = SERVER];
*/
public org.graylog2.plugin.journal.JournalMessages.SourceNode.Type getType() {
return type_;
}
/**
* optional .org.graylog2.plugin.journal.SourceNode.Type type = 2 [default = SERVER];
*/
public Builder setType(org.graylog2.plugin.journal.JournalMessages.SourceNode.Type value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
type_ = value;
onChanged();
return this;
}
/**
* optional .org.graylog2.plugin.journal.SourceNode.Type type = 2 [default = SERVER];
*/
public Builder clearType() {
bitField0_ = (bitField0_ & ~0x00000002);
type_ = org.graylog2.plugin.journal.JournalMessages.SourceNode.Type.SERVER;
onChanged();
return this;
}
// optional string input_id = 3;
private java.lang.Object inputId_ = "";
/**
* optional string input_id = 3;
*/
public boolean hasInputId() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional string input_id = 3;
*/
public java.lang.String getInputId() {
java.lang.Object ref = inputId_;
if (!(ref instanceof java.lang.String)) {
java.lang.String s = ((com.google.protobuf.ByteString) ref)
.toStringUtf8();
inputId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* optional string input_id = 3;
*/
public com.google.protobuf.ByteString
getInputIdBytes() {
java.lang.Object ref = inputId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
inputId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* optional string input_id = 3;
*/
public Builder setInputId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
inputId_ = value;
onChanged();
return this;
}
/**
* optional string input_id = 3;
*/
public Builder clearInputId() {
bitField0_ = (bitField0_ & ~0x00000004);
inputId_ = getDefaultInstance().getInputId();
onChanged();
return this;
}
/**
* optional string input_id = 3;
*/
public Builder setInputIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
inputId_ = value;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:org.graylog2.plugin.journal.SourceNode)
}
static {
defaultInstance = new SourceNode(true);
defaultInstance.initFields();
}
// @@protoc_insertion_point(class_scope:org.graylog2.plugin.journal.SourceNode)
}
private static com.google.protobuf.Descriptors.Descriptor
internal_static_org_graylog2_plugin_journal_JournalMessage_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_org_graylog2_plugin_journal_JournalMessage_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_org_graylog2_plugin_journal_RemoteAddress_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_org_graylog2_plugin_journal_RemoteAddress_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_org_graylog2_plugin_journal_CodecInfo_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_org_graylog2_plugin_journal_CodecInfo_fieldAccessorTable;
private static com.google.protobuf.Descriptors.Descriptor
internal_static_org_graylog2_plugin_journal_SourceNode_descriptor;
private static
com.google.protobuf.GeneratedMessage.FieldAccessorTable
internal_static_org_graylog2_plugin_journal_SourceNode_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n7resources/org/graylog2/plugin/journal/" +
"raw_message.proto\022\033org.graylog2.plugin.j" +
"ournal\"\266\002\n\016JournalMessage\022\017\n\007version\030\001 \001" +
"(\r\022\021\n\tuuid_time\030\002 \001(\006\022\025\n\ruuid_clockseq\030\003" +
" \001(\006\022\021\n\ttimestamp\030\004 \001(\006\0225\n\005codec\030\005 \001(\0132&" +
".org.graylog2.plugin.journal.CodecInfo\022=" +
"\n\014source_nodes\030\006 \003(\0132\'.org.graylog2.plug" +
"in.journal.SourceNode\022:\n\006remote\030\007 \001(\0132*." +
"org.graylog2.plugin.journal.RemoteAddres" +
"s\022\017\n\007payload\030\010 \001(\014\022\023\n\013sequence_nr\030\t \001(\r\"",
"@\n\rRemoteAddress\022\017\n\007address\030\001 \001(\014\022\014\n\004por" +
"t\030\002 \001(\r\022\020\n\010resolved\030\003 \001(\t\")\n\tCodecInfo\022\014" +
"\n\004name\030\001 \001(\t\022\016\n\006config\030\002 \001(\t\"\215\001\n\nSourceN" +
"ode\022\n\n\002id\030\001 \001(\t\022B\n\004type\030\002 \001(\0162,.org.gray" +
"log2.plugin.journal.SourceNode.Type:\006SER" +
"VER\022\020\n\010input_id\030\003 \001(\t\"\035\n\004Type\022\n\n\006SERVER\020" +
"\000\022\t\n\005RADIO\020\001B.\n\033org.graylog2.plugin.jour" +
"nalB\017JournalMessages"
};
com.google.protobuf.Descriptors.FileDescriptor.InternalDescriptorAssigner assigner =
new com.google.protobuf.Descriptors.FileDescriptor.InternalDescriptorAssigner() {
public com.google.protobuf.ExtensionRegistry assignDescriptors(
com.google.protobuf.Descriptors.FileDescriptor root) {
descriptor = root;
internal_static_org_graylog2_plugin_journal_JournalMessage_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_org_graylog2_plugin_journal_JournalMessage_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_org_graylog2_plugin_journal_JournalMessage_descriptor,
new java.lang.String[] { "Version", "UuidTime", "UuidClockseq", "Timestamp", "Codec", "SourceNodes", "Remote", "Payload", "SequenceNr", });
internal_static_org_graylog2_plugin_journal_RemoteAddress_descriptor =
getDescriptor().getMessageTypes().get(1);
internal_static_org_graylog2_plugin_journal_RemoteAddress_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_org_graylog2_plugin_journal_RemoteAddress_descriptor,
new java.lang.String[] { "Address", "Port", "Resolved", });
internal_static_org_graylog2_plugin_journal_CodecInfo_descriptor =
getDescriptor().getMessageTypes().get(2);
internal_static_org_graylog2_plugin_journal_CodecInfo_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_org_graylog2_plugin_journal_CodecInfo_descriptor,
new java.lang.String[] { "Name", "Config", });
internal_static_org_graylog2_plugin_journal_SourceNode_descriptor =
getDescriptor().getMessageTypes().get(3);
internal_static_org_graylog2_plugin_journal_SourceNode_fieldAccessorTable = new
com.google.protobuf.GeneratedMessage.FieldAccessorTable(
internal_static_org_graylog2_plugin_journal_SourceNode_descriptor,
new java.lang.String[] { "Id", "Type", "InputId", });
return null;
}
};
com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
}, assigner);
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy