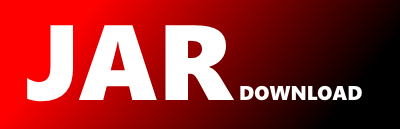
org.graylog.events.configuration.AutoValue_EventsConfiguration Maven / Gradle / Ivy
package org.graylog.events.configuration;
import com.fasterxml.jackson.annotation.JsonProperty;
import javax.annotation.processing.Generated;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_EventsConfiguration extends EventsConfiguration {
private final long eventsSearchTimeout;
private final long eventNotificationsRetry;
private final long eventNotificationsBacklog;
private final long eventCatchupWindow;
private final boolean notificationsKeepAliveProbe;
private AutoValue_EventsConfiguration(
long eventsSearchTimeout,
long eventNotificationsRetry,
long eventNotificationsBacklog,
long eventCatchupWindow,
boolean notificationsKeepAliveProbe) {
this.eventsSearchTimeout = eventsSearchTimeout;
this.eventNotificationsRetry = eventNotificationsRetry;
this.eventNotificationsBacklog = eventNotificationsBacklog;
this.eventCatchupWindow = eventCatchupWindow;
this.notificationsKeepAliveProbe = notificationsKeepAliveProbe;
}
@JsonProperty("events_search_timeout")
@Override
public long eventsSearchTimeout() {
return eventsSearchTimeout;
}
@JsonProperty("events_notification_retry_period")
@Override
public long eventNotificationsRetry() {
return eventNotificationsRetry;
}
@JsonProperty("events_notification_default_backlog")
@Override
public long eventNotificationsBacklog() {
return eventNotificationsBacklog;
}
@JsonProperty("events_catchup_window")
@Override
public long eventCatchupWindow() {
return eventCatchupWindow;
}
@JsonProperty("events_notification_tcp_keepalive")
@Override
public boolean notificationsKeepAliveProbe() {
return notificationsKeepAliveProbe;
}
@Override
public String toString() {
return "EventsConfiguration{"
+ "eventsSearchTimeout=" + eventsSearchTimeout + ", "
+ "eventNotificationsRetry=" + eventNotificationsRetry + ", "
+ "eventNotificationsBacklog=" + eventNotificationsBacklog + ", "
+ "eventCatchupWindow=" + eventCatchupWindow + ", "
+ "notificationsKeepAliveProbe=" + notificationsKeepAliveProbe
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof EventsConfiguration) {
EventsConfiguration that = (EventsConfiguration) o;
return this.eventsSearchTimeout == that.eventsSearchTimeout()
&& this.eventNotificationsRetry == that.eventNotificationsRetry()
&& this.eventNotificationsBacklog == that.eventNotificationsBacklog()
&& this.eventCatchupWindow == that.eventCatchupWindow()
&& this.notificationsKeepAliveProbe == that.notificationsKeepAliveProbe();
}
return false;
}
@Override
public int hashCode() {
int h$ = 1;
h$ *= 1000003;
h$ ^= (int) ((eventsSearchTimeout >>> 32) ^ eventsSearchTimeout);
h$ *= 1000003;
h$ ^= (int) ((eventNotificationsRetry >>> 32) ^ eventNotificationsRetry);
h$ *= 1000003;
h$ ^= (int) ((eventNotificationsBacklog >>> 32) ^ eventNotificationsBacklog);
h$ *= 1000003;
h$ ^= (int) ((eventCatchupWindow >>> 32) ^ eventCatchupWindow);
h$ *= 1000003;
h$ ^= notificationsKeepAliveProbe ? 1231 : 1237;
return h$;
}
@Override
public EventsConfiguration.Builder toBuilder() {
return new Builder(this);
}
static final class Builder extends EventsConfiguration.Builder {
private long eventsSearchTimeout;
private long eventNotificationsRetry;
private long eventNotificationsBacklog;
private long eventCatchupWindow;
private boolean notificationsKeepAliveProbe;
private byte set$0;
Builder() {
}
private Builder(EventsConfiguration source) {
this.eventsSearchTimeout = source.eventsSearchTimeout();
this.eventNotificationsRetry = source.eventNotificationsRetry();
this.eventNotificationsBacklog = source.eventNotificationsBacklog();
this.eventCatchupWindow = source.eventCatchupWindow();
this.notificationsKeepAliveProbe = source.notificationsKeepAliveProbe();
set$0 = (byte) 0x1f;
}
@Override
public EventsConfiguration.Builder eventsSearchTimeout(long eventsSearchTimeout) {
this.eventsSearchTimeout = eventsSearchTimeout;
set$0 |= (byte) 1;
return this;
}
@Override
public EventsConfiguration.Builder eventNotificationsRetry(long eventNotificationsRetry) {
this.eventNotificationsRetry = eventNotificationsRetry;
set$0 |= (byte) 2;
return this;
}
@Override
public EventsConfiguration.Builder eventNotificationsBacklog(long eventNotificationsBacklog) {
this.eventNotificationsBacklog = eventNotificationsBacklog;
set$0 |= (byte) 4;
return this;
}
@Override
public EventsConfiguration.Builder eventCatchupWindow(long eventCatchupWindow) {
this.eventCatchupWindow = eventCatchupWindow;
set$0 |= (byte) 8;
return this;
}
@Override
public EventsConfiguration.Builder notificationsKeepAliveProbe(boolean notificationsKeepAliveProbe) {
this.notificationsKeepAliveProbe = notificationsKeepAliveProbe;
set$0 |= (byte) 0x10;
return this;
}
@Override
public EventsConfiguration build() {
if (set$0 != 0x1f) {
StringBuilder missing = new StringBuilder();
if ((set$0 & 1) == 0) {
missing.append(" eventsSearchTimeout");
}
if ((set$0 & 2) == 0) {
missing.append(" eventNotificationsRetry");
}
if ((set$0 & 4) == 0) {
missing.append(" eventNotificationsBacklog");
}
if ((set$0 & 8) == 0) {
missing.append(" eventCatchupWindow");
}
if ((set$0 & 0x10) == 0) {
missing.append(" notificationsKeepAliveProbe");
}
throw new IllegalStateException("Missing required properties:" + missing);
}
return new AutoValue_EventsConfiguration(
this.eventsSearchTimeout,
this.eventNotificationsRetry,
this.eventNotificationsBacklog,
this.eventCatchupWindow,
this.notificationsKeepAliveProbe);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy