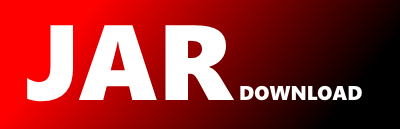
org.graylog.events.search.AutoValue_EventsSearchResult Maven / Gradle / Ivy
package org.graylog.events.search;
import com.fasterxml.jackson.annotation.JsonProperty;
import java.util.List;
import java.util.Set;
import javax.annotation.processing.Generated;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_EventsSearchResult extends EventsSearchResult {
private final List events;
private final Set usedIndices;
private final EventsSearchParameters parameters;
private final long totalEvents;
private final long duration;
private final EventsSearchResult.Context context;
private AutoValue_EventsSearchResult(
List events,
Set usedIndices,
EventsSearchParameters parameters,
long totalEvents,
long duration,
EventsSearchResult.Context context) {
this.events = events;
this.usedIndices = usedIndices;
this.parameters = parameters;
this.totalEvents = totalEvents;
this.duration = duration;
this.context = context;
}
@JsonProperty("events")
@Override
public List events() {
return events;
}
@JsonProperty("used_indices")
@Override
public Set usedIndices() {
return usedIndices;
}
@JsonProperty("parameters")
@Override
public EventsSearchParameters parameters() {
return parameters;
}
@JsonProperty("total_events")
@Override
public long totalEvents() {
return totalEvents;
}
@JsonProperty("duration")
@Override
public long duration() {
return duration;
}
@JsonProperty("context")
@Override
public EventsSearchResult.Context context() {
return context;
}
@Override
public String toString() {
return "EventsSearchResult{"
+ "events=" + events + ", "
+ "usedIndices=" + usedIndices + ", "
+ "parameters=" + parameters + ", "
+ "totalEvents=" + totalEvents + ", "
+ "duration=" + duration + ", "
+ "context=" + context
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof EventsSearchResult) {
EventsSearchResult that = (EventsSearchResult) o;
return this.events.equals(that.events())
&& this.usedIndices.equals(that.usedIndices())
&& this.parameters.equals(that.parameters())
&& this.totalEvents == that.totalEvents()
&& this.duration == that.duration()
&& this.context.equals(that.context());
}
return false;
}
@Override
public int hashCode() {
int h$ = 1;
h$ *= 1000003;
h$ ^= events.hashCode();
h$ *= 1000003;
h$ ^= usedIndices.hashCode();
h$ *= 1000003;
h$ ^= parameters.hashCode();
h$ *= 1000003;
h$ ^= (int) ((totalEvents >>> 32) ^ totalEvents);
h$ *= 1000003;
h$ ^= (int) ((duration >>> 32) ^ duration);
h$ *= 1000003;
h$ ^= context.hashCode();
return h$;
}
static final class Builder extends EventsSearchResult.Builder {
private List events;
private Set usedIndices;
private EventsSearchParameters parameters;
private long totalEvents;
private long duration;
private EventsSearchResult.Context context;
private byte set$0;
Builder() {
}
@Override
public EventsSearchResult.Builder events(List events) {
if (events == null) {
throw new NullPointerException("Null events");
}
this.events = events;
return this;
}
@Override
public EventsSearchResult.Builder usedIndices(Set usedIndices) {
if (usedIndices == null) {
throw new NullPointerException("Null usedIndices");
}
this.usedIndices = usedIndices;
return this;
}
@Override
public EventsSearchResult.Builder parameters(EventsSearchParameters parameters) {
if (parameters == null) {
throw new NullPointerException("Null parameters");
}
this.parameters = parameters;
return this;
}
@Override
public EventsSearchResult.Builder totalEvents(long totalEvents) {
this.totalEvents = totalEvents;
set$0 |= (byte) 1;
return this;
}
@Override
public EventsSearchResult.Builder duration(long duration) {
this.duration = duration;
set$0 |= (byte) 2;
return this;
}
@Override
public EventsSearchResult.Builder context(EventsSearchResult.Context context) {
if (context == null) {
throw new NullPointerException("Null context");
}
this.context = context;
return this;
}
@Override
public EventsSearchResult build() {
if (set$0 != 3
|| this.events == null
|| this.usedIndices == null
|| this.parameters == null
|| this.context == null) {
StringBuilder missing = new StringBuilder();
if (this.events == null) {
missing.append(" events");
}
if (this.usedIndices == null) {
missing.append(" usedIndices");
}
if (this.parameters == null) {
missing.append(" parameters");
}
if ((set$0 & 1) == 0) {
missing.append(" totalEvents");
}
if ((set$0 & 2) == 0) {
missing.append(" duration");
}
if (this.context == null) {
missing.append(" context");
}
throw new IllegalStateException("Missing required properties:" + missing);
}
return new AutoValue_EventsSearchResult(
this.events,
this.usedIndices,
this.parameters,
this.totalEvents,
this.duration,
this.context);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy