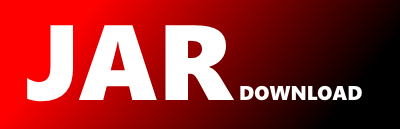
org.graylog.grn.AutoValue_GRN Maven / Gradle / Ivy
package org.graylog.grn;
import javax.annotation.processing.Generated;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_GRN extends GRN {
private final String cluster;
private final String tenant;
private final String scope;
private final String type;
private final String entity;
private final GRNType grnType;
private AutoValue_GRN(
String cluster,
String tenant,
String scope,
String type,
String entity,
GRNType grnType) {
this.cluster = cluster;
this.tenant = tenant;
this.scope = scope;
this.type = type;
this.entity = entity;
this.grnType = grnType;
}
@Override
public String cluster() {
return cluster;
}
@Override
public String tenant() {
return tenant;
}
@Override
public String scope() {
return scope;
}
@Override
public String type() {
return type;
}
@Override
public String entity() {
return entity;
}
@Override
public GRNType grnType() {
return grnType;
}
@Override
public GRN.Builder toBuilder() {
return new Builder(this);
}
static final class Builder extends GRN.Builder {
private String cluster;
private String tenant;
private String scope;
private String type;
private String entity;
private GRNType grnType;
Builder() {
}
private Builder(GRN source) {
this.cluster = source.cluster();
this.tenant = source.tenant();
this.scope = source.scope();
this.type = source.type();
this.entity = source.entity();
this.grnType = source.grnType();
}
@Override
public GRN.Builder cluster(String cluster) {
if (cluster == null) {
throw new NullPointerException("Null cluster");
}
this.cluster = cluster;
return this;
}
@Override
public GRN.Builder tenant(String tenant) {
if (tenant == null) {
throw new NullPointerException("Null tenant");
}
this.tenant = tenant;
return this;
}
@Override
public GRN.Builder scope(String scope) {
if (scope == null) {
throw new NullPointerException("Null scope");
}
this.scope = scope;
return this;
}
@Override
public GRN.Builder type(String type) {
if (type == null) {
throw new NullPointerException("Null type");
}
this.type = type;
return this;
}
@Override
public GRN.Builder entity(String entity) {
if (entity == null) {
throw new NullPointerException("Null entity");
}
this.entity = entity;
return this;
}
@Override
public GRN.Builder grnType(GRNType grnType) {
if (grnType == null) {
throw new NullPointerException("Null grnType");
}
this.grnType = grnType;
return this;
}
@Override
public GRN build() {
if (this.cluster == null
|| this.tenant == null
|| this.scope == null
|| this.type == null
|| this.entity == null
|| this.grnType == null) {
StringBuilder missing = new StringBuilder();
if (this.cluster == null) {
missing.append(" cluster");
}
if (this.tenant == null) {
missing.append(" tenant");
}
if (this.scope == null) {
missing.append(" scope");
}
if (this.type == null) {
missing.append(" type");
}
if (this.entity == null) {
missing.append(" entity");
}
if (this.grnType == null) {
missing.append(" grnType");
}
throw new IllegalStateException("Missing required properties:" + missing);
}
return new AutoValue_GRN(
this.cluster,
this.tenant,
this.scope,
this.type,
this.entity,
this.grnType);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy