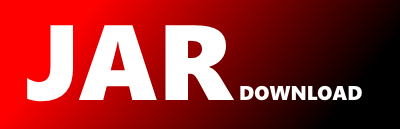
org.graylog.integrations.aws.cloudwatch.$AutoValue_KinesisLogEntry Maven / Gradle / Ivy
package org.graylog.integrations.aws.cloudwatch;
import com.fasterxml.jackson.annotation.JsonProperty;
import javax.annotation.processing.Generated;
import org.joda.time.DateTime;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
abstract class $AutoValue_KinesisLogEntry extends KinesisLogEntry {
private final String kinesisStream;
private final String logGroup;
private final String logStream;
private final DateTime timestamp;
private final String message;
$AutoValue_KinesisLogEntry(
String kinesisStream,
String logGroup,
String logStream,
DateTime timestamp,
String message) {
if (kinesisStream == null) {
throw new NullPointerException("Null kinesisStream");
}
this.kinesisStream = kinesisStream;
if (logGroup == null) {
throw new NullPointerException("Null logGroup");
}
this.logGroup = logGroup;
if (logStream == null) {
throw new NullPointerException("Null logStream");
}
this.logStream = logStream;
if (timestamp == null) {
throw new NullPointerException("Null timestamp");
}
this.timestamp = timestamp;
if (message == null) {
throw new NullPointerException("Null message");
}
this.message = message;
}
@JsonProperty("kinesis_stream")
@Override
public String kinesisStream() {
return kinesisStream;
}
@JsonProperty("log_group")
@Override
public String logGroup() {
return logGroup;
}
@JsonProperty("log_stream")
@Override
public String logStream() {
return logStream;
}
@JsonProperty("timestamp")
@Override
public DateTime timestamp() {
return timestamp;
}
@JsonProperty("message")
@Override
public String message() {
return message;
}
@Override
public String toString() {
return "KinesisLogEntry{"
+ "kinesisStream=" + kinesisStream + ", "
+ "logGroup=" + logGroup + ", "
+ "logStream=" + logStream + ", "
+ "timestamp=" + timestamp + ", "
+ "message=" + message
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof KinesisLogEntry) {
KinesisLogEntry that = (KinesisLogEntry) o;
return this.kinesisStream.equals(that.kinesisStream())
&& this.logGroup.equals(that.logGroup())
&& this.logStream.equals(that.logStream())
&& this.timestamp.equals(that.timestamp())
&& this.message.equals(that.message());
}
return false;
}
@Override
public int hashCode() {
int h$ = 1;
h$ *= 1000003;
h$ ^= kinesisStream.hashCode();
h$ *= 1000003;
h$ ^= logGroup.hashCode();
h$ *= 1000003;
h$ ^= logStream.hashCode();
h$ *= 1000003;
h$ ^= timestamp.hashCode();
h$ *= 1000003;
h$ ^= message.hashCode();
return h$;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy