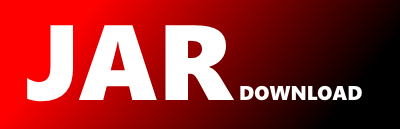
org.graylog.integrations.inputs.paloalto.AutoValue_PaloAltoMessageBase Maven / Gradle / Ivy
package org.graylog.integrations.inputs.paloalto;
import com.google.common.collect.ImmutableList;
import javax.annotation.processing.Generated;
import org.joda.time.DateTime;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_PaloAltoMessageBase extends PaloAltoMessageBase {
private final String source;
private final DateTime timestamp;
private final String payload;
private final String panType;
private final ImmutableList fields;
private AutoValue_PaloAltoMessageBase(
String source,
DateTime timestamp,
String payload,
String panType,
ImmutableList fields) {
this.source = source;
this.timestamp = timestamp;
this.payload = payload;
this.panType = panType;
this.fields = fields;
}
@Override
public String source() {
return source;
}
@Override
public DateTime timestamp() {
return timestamp;
}
@Override
public String payload() {
return payload;
}
@Override
public String panType() {
return panType;
}
@Override
public ImmutableList fields() {
return fields;
}
@Override
public String toString() {
return "PaloAltoMessageBase{"
+ "source=" + source + ", "
+ "timestamp=" + timestamp + ", "
+ "payload=" + payload + ", "
+ "panType=" + panType + ", "
+ "fields=" + fields
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof PaloAltoMessageBase) {
PaloAltoMessageBase that = (PaloAltoMessageBase) o;
return this.source.equals(that.source())
&& this.timestamp.equals(that.timestamp())
&& this.payload.equals(that.payload())
&& this.panType.equals(that.panType())
&& this.fields.equals(that.fields());
}
return false;
}
@Override
public int hashCode() {
int h$ = 1;
h$ *= 1000003;
h$ ^= source.hashCode();
h$ *= 1000003;
h$ ^= timestamp.hashCode();
h$ *= 1000003;
h$ ^= payload.hashCode();
h$ *= 1000003;
h$ ^= panType.hashCode();
h$ *= 1000003;
h$ ^= fields.hashCode();
return h$;
}
static final class Builder extends PaloAltoMessageBase.Builder {
private String source;
private DateTime timestamp;
private String payload;
private String panType;
private ImmutableList fields;
Builder() {
}
@Override
public PaloAltoMessageBase.Builder source(String source) {
if (source == null) {
throw new NullPointerException("Null source");
}
this.source = source;
return this;
}
@Override
public PaloAltoMessageBase.Builder timestamp(DateTime timestamp) {
if (timestamp == null) {
throw new NullPointerException("Null timestamp");
}
this.timestamp = timestamp;
return this;
}
@Override
public PaloAltoMessageBase.Builder payload(String payload) {
if (payload == null) {
throw new NullPointerException("Null payload");
}
this.payload = payload;
return this;
}
@Override
public PaloAltoMessageBase.Builder panType(String panType) {
if (panType == null) {
throw new NullPointerException("Null panType");
}
this.panType = panType;
return this;
}
@Override
public PaloAltoMessageBase.Builder fields(ImmutableList fields) {
if (fields == null) {
throw new NullPointerException("Null fields");
}
this.fields = fields;
return this;
}
@Override
public PaloAltoMessageBase build() {
if (this.source == null
|| this.timestamp == null
|| this.payload == null
|| this.panType == null
|| this.fields == null) {
StringBuilder missing = new StringBuilder();
if (this.source == null) {
missing.append(" source");
}
if (this.timestamp == null) {
missing.append(" timestamp");
}
if (this.payload == null) {
missing.append(" payload");
}
if (this.panType == null) {
missing.append(" panType");
}
if (this.fields == null) {
missing.append(" fields");
}
throw new IllegalStateException("Missing required properties:" + missing);
}
return new AutoValue_PaloAltoMessageBase(
this.source,
this.timestamp,
this.payload,
this.panType,
this.fields);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy