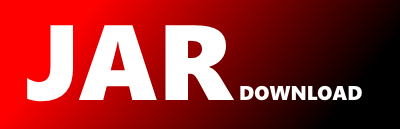
org.graylog.integrations.ipfix.AutoValue_IpfixMessage Maven / Gradle / Ivy
package org.graylog.integrations.ipfix;
import com.google.common.collect.ImmutableList;
import javax.annotation.processing.Generated;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_IpfixMessage extends IpfixMessage {
private final ImmutableList templateRecords;
private final ImmutableList optionsTemplateRecords;
private final ImmutableList flows;
private AutoValue_IpfixMessage(
ImmutableList templateRecords,
ImmutableList optionsTemplateRecords,
ImmutableList flows) {
this.templateRecords = templateRecords;
this.optionsTemplateRecords = optionsTemplateRecords;
this.flows = flows;
}
@Override
public ImmutableList templateRecords() {
return templateRecords;
}
@Override
public ImmutableList optionsTemplateRecords() {
return optionsTemplateRecords;
}
@Override
public ImmutableList flows() {
return flows;
}
@Override
public String toString() {
return "IpfixMessage{"
+ "templateRecords=" + templateRecords + ", "
+ "optionsTemplateRecords=" + optionsTemplateRecords + ", "
+ "flows=" + flows
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof IpfixMessage) {
IpfixMessage that = (IpfixMessage) o;
return this.templateRecords.equals(that.templateRecords())
&& this.optionsTemplateRecords.equals(that.optionsTemplateRecords())
&& this.flows.equals(that.flows());
}
return false;
}
@Override
public int hashCode() {
int h$ = 1;
h$ *= 1000003;
h$ ^= templateRecords.hashCode();
h$ *= 1000003;
h$ ^= optionsTemplateRecords.hashCode();
h$ *= 1000003;
h$ ^= flows.hashCode();
return h$;
}
static final class Builder extends IpfixMessage.Builder {
private ImmutableList.Builder templateRecordsBuilder$;
private ImmutableList templateRecords;
private ImmutableList.Builder optionsTemplateRecordsBuilder$;
private ImmutableList optionsTemplateRecords;
private ImmutableList.Builder flowsBuilder$;
private ImmutableList flows;
Builder() {
}
@Override
public IpfixMessage.Builder templateRecords(ImmutableList templateRecords) {
if (templateRecords == null) {
throw new NullPointerException("Null templateRecords");
}
if (templateRecordsBuilder$ != null) {
throw new IllegalStateException("Cannot set templateRecords after calling templateRecordsBuilder()");
}
this.templateRecords = templateRecords;
return this;
}
@Override
public ImmutableList.Builder templateRecordsBuilder() {
if (templateRecordsBuilder$ == null) {
if (templateRecords == null) {
templateRecordsBuilder$ = ImmutableList.builder();
} else {
templateRecordsBuilder$ = ImmutableList.builder();
templateRecordsBuilder$.addAll(templateRecords);
templateRecords = null;
}
}
return templateRecordsBuilder$;
}
@Override
public IpfixMessage.Builder optionsTemplateRecords(ImmutableList optionsTemplateRecords) {
if (optionsTemplateRecords == null) {
throw new NullPointerException("Null optionsTemplateRecords");
}
if (optionsTemplateRecordsBuilder$ != null) {
throw new IllegalStateException("Cannot set optionsTemplateRecords after calling optionsTemplateRecordsBuilder()");
}
this.optionsTemplateRecords = optionsTemplateRecords;
return this;
}
@Override
public ImmutableList.Builder optionsTemplateRecordsBuilder() {
if (optionsTemplateRecordsBuilder$ == null) {
if (optionsTemplateRecords == null) {
optionsTemplateRecordsBuilder$ = ImmutableList.builder();
} else {
optionsTemplateRecordsBuilder$ = ImmutableList.builder();
optionsTemplateRecordsBuilder$.addAll(optionsTemplateRecords);
optionsTemplateRecords = null;
}
}
return optionsTemplateRecordsBuilder$;
}
@Override
public IpfixMessage.Builder flows(ImmutableList flows) {
if (flows == null) {
throw new NullPointerException("Null flows");
}
if (flowsBuilder$ != null) {
throw new IllegalStateException("Cannot set flows after calling flowsBuilder()");
}
this.flows = flows;
return this;
}
@Override
public ImmutableList.Builder flowsBuilder() {
if (flowsBuilder$ == null) {
if (flows == null) {
flowsBuilder$ = ImmutableList.builder();
} else {
flowsBuilder$ = ImmutableList.builder();
flowsBuilder$.addAll(flows);
flows = null;
}
}
return flowsBuilder$;
}
@Override
public IpfixMessage build() {
if (templateRecordsBuilder$ != null) {
this.templateRecords = templateRecordsBuilder$.build();
} else if (this.templateRecords == null) {
this.templateRecords = ImmutableList.of();
}
if (optionsTemplateRecordsBuilder$ != null) {
this.optionsTemplateRecords = optionsTemplateRecordsBuilder$.build();
} else if (this.optionsTemplateRecords == null) {
this.optionsTemplateRecords = ImmutableList.of();
}
if (flowsBuilder$ != null) {
this.flows = flowsBuilder$.build();
} else if (this.flows == null) {
this.flows = ImmutableList.of();
}
return new AutoValue_IpfixMessage(
this.templateRecords,
this.optionsTemplateRecords,
this.flows);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy