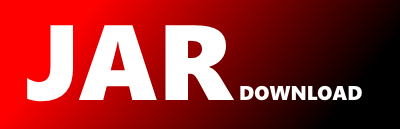
org.graylog.integrations.pagerduty.AutoValue_PagerDutyNotificationConfig Maven / Gradle / Ivy
package org.graylog.integrations.pagerduty;
import com.fasterxml.jackson.annotation.JsonProperty;
import javax.annotation.processing.Generated;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_PagerDutyNotificationConfig extends PagerDutyNotificationConfig {
private final String type;
private final String routingKey;
private final boolean customIncident;
private final String keyPrefix;
private final String clientName;
private final String clientUrl;
private AutoValue_PagerDutyNotificationConfig(
String type,
String routingKey,
boolean customIncident,
String keyPrefix,
String clientName,
String clientUrl) {
this.type = type;
this.routingKey = routingKey;
this.customIncident = customIncident;
this.keyPrefix = keyPrefix;
this.clientName = clientName;
this.clientUrl = clientUrl;
}
@JsonProperty("type")
@Override
public String type() {
return type;
}
@JsonProperty("routing_key")
@Override
public String routingKey() {
return routingKey;
}
@JsonProperty("custom_incident")
@Override
public boolean customIncident() {
return customIncident;
}
@JsonProperty("key_prefix")
@Override
public String keyPrefix() {
return keyPrefix;
}
@JsonProperty("client_name")
@Override
public String clientName() {
return clientName;
}
@JsonProperty("client_url")
@Override
public String clientUrl() {
return clientUrl;
}
@Override
public String toString() {
return "PagerDutyNotificationConfig{"
+ "type=" + type + ", "
+ "routingKey=" + routingKey + ", "
+ "customIncident=" + customIncident + ", "
+ "keyPrefix=" + keyPrefix + ", "
+ "clientName=" + clientName + ", "
+ "clientUrl=" + clientUrl
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof PagerDutyNotificationConfig) {
PagerDutyNotificationConfig that = (PagerDutyNotificationConfig) o;
return this.type.equals(that.type())
&& this.routingKey.equals(that.routingKey())
&& this.customIncident == that.customIncident()
&& this.keyPrefix.equals(that.keyPrefix())
&& this.clientName.equals(that.clientName())
&& this.clientUrl.equals(that.clientUrl());
}
return false;
}
@Override
public int hashCode() {
int h$ = 1;
h$ *= 1000003;
h$ ^= type.hashCode();
h$ *= 1000003;
h$ ^= routingKey.hashCode();
h$ *= 1000003;
h$ ^= customIncident ? 1231 : 1237;
h$ *= 1000003;
h$ ^= keyPrefix.hashCode();
h$ *= 1000003;
h$ ^= clientName.hashCode();
h$ *= 1000003;
h$ ^= clientUrl.hashCode();
return h$;
}
static final class Builder extends PagerDutyNotificationConfig.Builder {
private String type;
private String routingKey;
private boolean customIncident;
private String keyPrefix;
private String clientName;
private String clientUrl;
private byte set$0;
Builder() {
}
@Override
public PagerDutyNotificationConfig.Builder type(String type) {
if (type == null) {
throw new NullPointerException("Null type");
}
this.type = type;
return this;
}
@Override
public PagerDutyNotificationConfig.Builder routingKey(String routingKey) {
if (routingKey == null) {
throw new NullPointerException("Null routingKey");
}
this.routingKey = routingKey;
return this;
}
@Override
public PagerDutyNotificationConfig.Builder customIncident(boolean customIncident) {
this.customIncident = customIncident;
set$0 |= (byte) 1;
return this;
}
@Override
public PagerDutyNotificationConfig.Builder keyPrefix(String keyPrefix) {
if (keyPrefix == null) {
throw new NullPointerException("Null keyPrefix");
}
this.keyPrefix = keyPrefix;
return this;
}
@Override
public PagerDutyNotificationConfig.Builder clientName(String clientName) {
if (clientName == null) {
throw new NullPointerException("Null clientName");
}
this.clientName = clientName;
return this;
}
@Override
public PagerDutyNotificationConfig.Builder clientUrl(String clientUrl) {
if (clientUrl == null) {
throw new NullPointerException("Null clientUrl");
}
this.clientUrl = clientUrl;
return this;
}
@Override
public PagerDutyNotificationConfig build() {
if (set$0 != 1
|| this.type == null
|| this.routingKey == null
|| this.keyPrefix == null
|| this.clientName == null
|| this.clientUrl == null) {
StringBuilder missing = new StringBuilder();
if (this.type == null) {
missing.append(" type");
}
if (this.routingKey == null) {
missing.append(" routingKey");
}
if ((set$0 & 1) == 0) {
missing.append(" customIncident");
}
if (this.keyPrefix == null) {
missing.append(" keyPrefix");
}
if (this.clientName == null) {
missing.append(" clientName");
}
if (this.clientUrl == null) {
missing.append(" clientUrl");
}
throw new IllegalStateException("Missing required properties:" + missing);
}
return new AutoValue_PagerDutyNotificationConfig(
this.type,
this.routingKey,
this.customIncident,
this.keyPrefix,
this.clientName,
this.clientUrl);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy