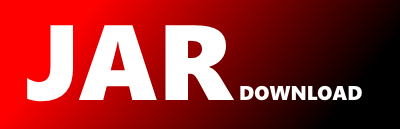
org.graylog.integrations.pagerduty.AutoValue_PagerDutyNotificationConfigEntity Maven / Gradle / Ivy
package org.graylog.integrations.pagerduty;
import com.fasterxml.jackson.annotation.JsonProperty;
import javax.annotation.processing.Generated;
import org.graylog2.contentpacks.model.entities.references.ValueReference;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_PagerDutyNotificationConfigEntity extends PagerDutyNotificationConfigEntity {
private final String type;
private final ValueReference routingKey;
private final ValueReference customIncident;
private final ValueReference keyPrefix;
private final ValueReference clientName;
private final ValueReference clientUrl;
private AutoValue_PagerDutyNotificationConfigEntity(
String type,
ValueReference routingKey,
ValueReference customIncident,
ValueReference keyPrefix,
ValueReference clientName,
ValueReference clientUrl) {
this.type = type;
this.routingKey = routingKey;
this.customIncident = customIncident;
this.keyPrefix = keyPrefix;
this.clientName = clientName;
this.clientUrl = clientUrl;
}
@JsonProperty("type")
@Override
public String type() {
return type;
}
@JsonProperty("routing_key")
@Override
public ValueReference routingKey() {
return routingKey;
}
@JsonProperty("custom_incident")
@Override
public ValueReference customIncident() {
return customIncident;
}
@JsonProperty("key_prefix")
@Override
public ValueReference keyPrefix() {
return keyPrefix;
}
@JsonProperty("client_name")
@Override
public ValueReference clientName() {
return clientName;
}
@JsonProperty("client_url")
@Override
public ValueReference clientUrl() {
return clientUrl;
}
@Override
public String toString() {
return "PagerDutyNotificationConfigEntity{"
+ "type=" + type + ", "
+ "routingKey=" + routingKey + ", "
+ "customIncident=" + customIncident + ", "
+ "keyPrefix=" + keyPrefix + ", "
+ "clientName=" + clientName + ", "
+ "clientUrl=" + clientUrl
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof PagerDutyNotificationConfigEntity) {
PagerDutyNotificationConfigEntity that = (PagerDutyNotificationConfigEntity) o;
return this.type.equals(that.type())
&& this.routingKey.equals(that.routingKey())
&& this.customIncident.equals(that.customIncident())
&& this.keyPrefix.equals(that.keyPrefix())
&& this.clientName.equals(that.clientName())
&& this.clientUrl.equals(that.clientUrl());
}
return false;
}
@Override
public int hashCode() {
int h$ = 1;
h$ *= 1000003;
h$ ^= type.hashCode();
h$ *= 1000003;
h$ ^= routingKey.hashCode();
h$ *= 1000003;
h$ ^= customIncident.hashCode();
h$ *= 1000003;
h$ ^= keyPrefix.hashCode();
h$ *= 1000003;
h$ ^= clientName.hashCode();
h$ *= 1000003;
h$ ^= clientUrl.hashCode();
return h$;
}
@Override
public PagerDutyNotificationConfigEntity.Builder toBuilder() {
return new Builder(this);
}
static final class Builder extends PagerDutyNotificationConfigEntity.Builder {
private String type;
private ValueReference routingKey;
private ValueReference customIncident;
private ValueReference keyPrefix;
private ValueReference clientName;
private ValueReference clientUrl;
Builder() {
}
private Builder(PagerDutyNotificationConfigEntity source) {
this.type = source.type();
this.routingKey = source.routingKey();
this.customIncident = source.customIncident();
this.keyPrefix = source.keyPrefix();
this.clientName = source.clientName();
this.clientUrl = source.clientUrl();
}
@Override
public PagerDutyNotificationConfigEntity.Builder type(String type) {
if (type == null) {
throw new NullPointerException("Null type");
}
this.type = type;
return this;
}
@Override
public PagerDutyNotificationConfigEntity.Builder routingKey(ValueReference routingKey) {
if (routingKey == null) {
throw new NullPointerException("Null routingKey");
}
this.routingKey = routingKey;
return this;
}
@Override
public PagerDutyNotificationConfigEntity.Builder customIncident(ValueReference customIncident) {
if (customIncident == null) {
throw new NullPointerException("Null customIncident");
}
this.customIncident = customIncident;
return this;
}
@Override
public PagerDutyNotificationConfigEntity.Builder keyPrefix(ValueReference keyPrefix) {
if (keyPrefix == null) {
throw new NullPointerException("Null keyPrefix");
}
this.keyPrefix = keyPrefix;
return this;
}
@Override
public PagerDutyNotificationConfigEntity.Builder clientName(ValueReference clientName) {
if (clientName == null) {
throw new NullPointerException("Null clientName");
}
this.clientName = clientName;
return this;
}
@Override
public PagerDutyNotificationConfigEntity.Builder clientUrl(ValueReference clientUrl) {
if (clientUrl == null) {
throw new NullPointerException("Null clientUrl");
}
this.clientUrl = clientUrl;
return this;
}
@Override
public PagerDutyNotificationConfigEntity build() {
if (this.type == null
|| this.routingKey == null
|| this.customIncident == null
|| this.keyPrefix == null
|| this.clientName == null
|| this.clientUrl == null) {
StringBuilder missing = new StringBuilder();
if (this.type == null) {
missing.append(" type");
}
if (this.routingKey == null) {
missing.append(" routingKey");
}
if (this.customIncident == null) {
missing.append(" customIncident");
}
if (this.keyPrefix == null) {
missing.append(" keyPrefix");
}
if (this.clientName == null) {
missing.append(" clientName");
}
if (this.clientUrl == null) {
missing.append(" clientUrl");
}
throw new IllegalStateException("Missing required properties:" + missing);
}
return new AutoValue_PagerDutyNotificationConfigEntity(
this.type,
this.routingKey,
this.customIncident,
this.keyPrefix,
this.clientName,
this.clientUrl);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy