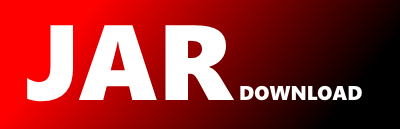
org.graylog.plugins.beats.MapUtils Maven / Gradle / Ivy
/*
* Copyright (C) 2020 Graylog, Inc.
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the Server Side Public License, version 1,
* as published by MongoDB, Inc.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* Server Side Public License for more details.
*
* You should have received a copy of the Server Side Public License
* along with this program. If not, see
* .
*/
package org.graylog.plugins.beats;
import java.util.HashMap;
import java.util.Map;
public final class MapUtils {
public static Map flatten(Map originalMap, String parentKey, String separator) {
final Map result = new HashMap<>();
for (Map.Entry entry : originalMap.entrySet()) {
final String key = parentKey.isEmpty() ? entry.getKey() : parentKey + separator + entry.getKey();
final Object value = entry.getValue();
if (value instanceof Map) {
@SuppressWarnings("unchecked")
final Map valueMap = (Map) value;
result.putAll(flatten(valueMap, key, separator));
} else {
result.put(key, value);
}
}
return result;
}
public static void renameKey(Map map, String originalKey, String newKey) {
if (map.containsKey(originalKey)) {
final Object value = map.remove(originalKey);
map.put(newKey, value);
}
}
public static Map replaceKeyCharacter(Map map, char oldChar, char newChar) {
final Map result = new HashMap<>(map.size());
for (Map.Entry entry : map.entrySet()) {
final String key = entry.getKey().replace(oldChar, newChar);
final Object value = entry.getValue();
result.put(key, value);
}
return result;
}
private MapUtils() {
throw new AssertionError("No instances allowed");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy