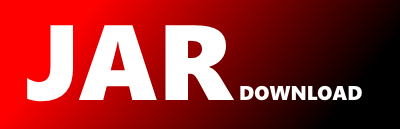
org.graylog.plugins.map.config.AutoValue_GeoIpResolverConfig Maven / Gradle / Ivy
package org.graylog.plugins.map.config;
import com.fasterxml.jackson.annotation.JsonProperty;
import java.util.concurrent.TimeUnit;
import javax.annotation.Nullable;
import javax.annotation.processing.Generated;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_GeoIpResolverConfig extends GeoIpResolverConfig {
private final boolean enabled;
private final boolean enforceGraylogSchema;
private final DatabaseVendorType databaseVendorType;
private final String cityDbPath;
private final String asnDbPath;
private final boolean useS3;
@Nullable
private final TimeUnit refreshIntervalUnit;
private final Long refreshInterval;
private AutoValue_GeoIpResolverConfig(
boolean enabled,
boolean enforceGraylogSchema,
DatabaseVendorType databaseVendorType,
String cityDbPath,
String asnDbPath,
boolean useS3,
@Nullable TimeUnit refreshIntervalUnit,
Long refreshInterval) {
this.enabled = enabled;
this.enforceGraylogSchema = enforceGraylogSchema;
this.databaseVendorType = databaseVendorType;
this.cityDbPath = cityDbPath;
this.asnDbPath = asnDbPath;
this.useS3 = useS3;
this.refreshIntervalUnit = refreshIntervalUnit;
this.refreshInterval = refreshInterval;
}
@JsonProperty("enabled")
@Override
public boolean enabled() {
return enabled;
}
@JsonProperty("enforce_graylog_schema")
@Override
public boolean enforceGraylogSchema() {
return enforceGraylogSchema;
}
@JsonProperty("db_vendor_type")
@Override
public DatabaseVendorType databaseVendorType() {
return databaseVendorType;
}
@JsonProperty("city_db_path")
@Override
public String cityDbPath() {
return cityDbPath;
}
@JsonProperty("asn_db_path")
@Override
public String asnDbPath() {
return asnDbPath;
}
@JsonProperty("use_s3")
@Override
public boolean useS3() {
return useS3;
}
@JsonProperty("refresh_interval_unit")
@Nullable
@Override
public TimeUnit refreshIntervalUnit() {
return refreshIntervalUnit;
}
@JsonProperty("refresh_interval")
@Override
public Long refreshInterval() {
return refreshInterval;
}
@Override
public String toString() {
return "GeoIpResolverConfig{"
+ "enabled=" + enabled + ", "
+ "enforceGraylogSchema=" + enforceGraylogSchema + ", "
+ "databaseVendorType=" + databaseVendorType + ", "
+ "cityDbPath=" + cityDbPath + ", "
+ "asnDbPath=" + asnDbPath + ", "
+ "useS3=" + useS3 + ", "
+ "refreshIntervalUnit=" + refreshIntervalUnit + ", "
+ "refreshInterval=" + refreshInterval
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof GeoIpResolverConfig) {
GeoIpResolverConfig that = (GeoIpResolverConfig) o;
return this.enabled == that.enabled()
&& this.enforceGraylogSchema == that.enforceGraylogSchema()
&& this.databaseVendorType.equals(that.databaseVendorType())
&& this.cityDbPath.equals(that.cityDbPath())
&& this.asnDbPath.equals(that.asnDbPath())
&& this.useS3 == that.useS3()
&& (this.refreshIntervalUnit == null ? that.refreshIntervalUnit() == null : this.refreshIntervalUnit.equals(that.refreshIntervalUnit()))
&& this.refreshInterval.equals(that.refreshInterval());
}
return false;
}
@Override
public int hashCode() {
int h$ = 1;
h$ *= 1000003;
h$ ^= enabled ? 1231 : 1237;
h$ *= 1000003;
h$ ^= enforceGraylogSchema ? 1231 : 1237;
h$ *= 1000003;
h$ ^= databaseVendorType.hashCode();
h$ *= 1000003;
h$ ^= cityDbPath.hashCode();
h$ *= 1000003;
h$ ^= asnDbPath.hashCode();
h$ *= 1000003;
h$ ^= useS3 ? 1231 : 1237;
h$ *= 1000003;
h$ ^= (refreshIntervalUnit == null) ? 0 : refreshIntervalUnit.hashCode();
h$ *= 1000003;
h$ ^= refreshInterval.hashCode();
return h$;
}
@Override
public GeoIpResolverConfig.Builder toBuilder() {
return new Builder(this);
}
static final class Builder extends GeoIpResolverConfig.Builder {
private boolean enabled;
private boolean enforceGraylogSchema;
private DatabaseVendorType databaseVendorType;
private String cityDbPath;
private String asnDbPath;
private boolean useS3;
private TimeUnit refreshIntervalUnit;
private Long refreshInterval;
private byte set$0;
Builder() {
}
private Builder(GeoIpResolverConfig source) {
this.enabled = source.enabled();
this.enforceGraylogSchema = source.enforceGraylogSchema();
this.databaseVendorType = source.databaseVendorType();
this.cityDbPath = source.cityDbPath();
this.asnDbPath = source.asnDbPath();
this.useS3 = source.useS3();
this.refreshIntervalUnit = source.refreshIntervalUnit();
this.refreshInterval = source.refreshInterval();
set$0 = (byte) 7;
}
@Override
public GeoIpResolverConfig.Builder enabled(boolean enabled) {
this.enabled = enabled;
set$0 |= (byte) 1;
return this;
}
@Override
public GeoIpResolverConfig.Builder enforceGraylogSchema(boolean enforceGraylogSchema) {
this.enforceGraylogSchema = enforceGraylogSchema;
set$0 |= (byte) 2;
return this;
}
@Override
public GeoIpResolverConfig.Builder databaseVendorType(DatabaseVendorType databaseVendorType) {
if (databaseVendorType == null) {
throw new NullPointerException("Null databaseVendorType");
}
this.databaseVendorType = databaseVendorType;
return this;
}
@Override
public GeoIpResolverConfig.Builder cityDbPath(String cityDbPath) {
if (cityDbPath == null) {
throw new NullPointerException("Null cityDbPath");
}
this.cityDbPath = cityDbPath;
return this;
}
@Override
public GeoIpResolverConfig.Builder asnDbPath(String asnDbPath) {
if (asnDbPath == null) {
throw new NullPointerException("Null asnDbPath");
}
this.asnDbPath = asnDbPath;
return this;
}
@Override
public GeoIpResolverConfig.Builder useS3(boolean useS3) {
this.useS3 = useS3;
set$0 |= (byte) 4;
return this;
}
@Override
public GeoIpResolverConfig.Builder refreshIntervalUnit(TimeUnit refreshIntervalUnit) {
this.refreshIntervalUnit = refreshIntervalUnit;
return this;
}
@Override
public GeoIpResolverConfig.Builder refreshInterval(Long refreshInterval) {
if (refreshInterval == null) {
throw new NullPointerException("Null refreshInterval");
}
this.refreshInterval = refreshInterval;
return this;
}
@Override
public GeoIpResolverConfig build() {
if (set$0 != 7
|| this.databaseVendorType == null
|| this.cityDbPath == null
|| this.asnDbPath == null
|| this.refreshInterval == null) {
StringBuilder missing = new StringBuilder();
if ((set$0 & 1) == 0) {
missing.append(" enabled");
}
if ((set$0 & 2) == 0) {
missing.append(" enforceGraylogSchema");
}
if (this.databaseVendorType == null) {
missing.append(" databaseVendorType");
}
if (this.cityDbPath == null) {
missing.append(" cityDbPath");
}
if (this.asnDbPath == null) {
missing.append(" asnDbPath");
}
if ((set$0 & 4) == 0) {
missing.append(" useS3");
}
if (this.refreshInterval == null) {
missing.append(" refreshInterval");
}
throw new IllegalStateException("Missing required properties:" + missing);
}
return new AutoValue_GeoIpResolverConfig(
this.enabled,
this.enforceGraylogSchema,
this.databaseVendorType,
this.cityDbPath,
this.asnDbPath,
this.useS3,
this.refreshIntervalUnit,
this.refreshInterval);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy