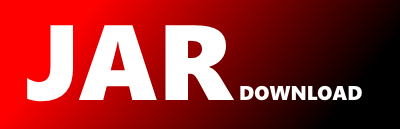
org.graylog.plugins.map.geoip.MaxmindDataAdapter Maven / Gradle / Ivy
/*
* Copyright (C) 2020 Graylog, Inc.
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the Server Side Public License, version 1,
* as published by MongoDB, Inc.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* Server Side Public License for more details.
*
* You should have received a copy of the Server Side Public License
* along with this program. If not, see
* .
*/
package org.graylog.plugins.map.geoip;
import com.codahale.metrics.MetricRegistry;
import com.fasterxml.jackson.annotation.JsonAutoDetect;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import com.google.auto.value.AutoValue;
import com.google.common.annotations.VisibleForTesting;
import com.google.common.collect.ArrayListMultimap;
import com.google.common.collect.ImmutableMap;
import com.google.common.collect.Multimap;
import com.google.common.net.InetAddresses;
import com.google.inject.assistedinject.Assisted;
import com.maxmind.geoip2.exception.AddressNotFoundException;
import com.maxmind.geoip2.model.AsnResponse;
import com.maxmind.geoip2.model.CityResponse;
import com.maxmind.geoip2.model.CountryResponse;
import com.maxmind.geoip2.record.Country;
import com.maxmind.geoip2.record.Location;
import org.graylog.autovalue.WithBeanGetter;
import org.graylog.plugins.map.config.DatabaseType;
import org.graylog2.plugin.lookup.LookupCachePurge;
import org.graylog2.plugin.lookup.LookupDataAdapter;
import org.graylog2.plugin.lookup.LookupDataAdapterConfiguration;
import org.graylog2.plugin.lookup.LookupResult;
import org.graylog2.plugin.utilities.FileInfo;
import org.joda.time.Duration;
import org.slf4j.Logger;
import javax.annotation.Nullable;
import javax.inject.Inject;
import javax.inject.Named;
import javax.validation.constraints.Min;
import javax.validation.constraints.NotEmpty;
import javax.validation.constraints.NotNull;
import java.io.File;
import java.io.IOException;
import java.net.InetAddress;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.util.Collections;
import java.util.HashMap;
import java.util.Map;
import java.util.Optional;
import java.util.concurrent.TimeUnit;
import java.util.concurrent.atomic.AtomicReference;
import static org.graylog.plugins.map.config.GeoIpProcessorConfig.DISABLE_IPINFO_DB_TYPE_CHECK;
import static org.slf4j.LoggerFactory.getLogger;
public class MaxmindDataAdapter extends LookupDataAdapter {
private static final Logger LOG = getLogger(MaxmindDataAdapter.class);
public static final String NAME = "maxmind_geoip";
private final Config config;
private final boolean disableIpInfoDbTypeCheck;
private final AtomicReference databaseAdapter = new AtomicReference<>();
private FileInfo fileInfo = FileInfo.empty();
@Inject
protected MaxmindDataAdapter(@Assisted("id") String id,
@Assisted("name") String name,
@Assisted LookupDataAdapterConfiguration config,
@Named(DISABLE_IPINFO_DB_TYPE_CHECK) boolean disableIpInfoDbTypeCheck,
MetricRegistry metricRegistry) {
super(id, name, config, metricRegistry);
this.config = (Config) config;
this.disableIpInfoDbTypeCheck = disableIpInfoDbTypeCheck;
}
@Override
protected void doStart() throws Exception {
Path path = Paths.get(config.path());
fileInfo = FileInfo.forPath(path);
if (!Files.isReadable(path)) {
LOG.warn("Cannot read database file {}", config.path());
setError(new IllegalStateException("Cannot read database file " + config.path()));
} else {
try {
this.databaseAdapter.set(loadDatabaseAdapter(path.toFile()));
} catch (Exception e) {
LOG.warn("Unable to read data base file {}", config.path());
setError(e);
}
}
}
@Override
protected void doStop() throws Exception {
final IPLocationDatabaseAdapter databaseReader = this.databaseAdapter.get();
if (databaseReader != null) {
databaseReader.close();
}
}
@Override
public Duration refreshInterval() {
if (config.checkIntervalUnit() == null || config.checkInterval() == 0) {
return Duration.ZERO;
}
//noinspection ConstantConditions
return Duration.millis(config.checkIntervalUnit().toMillis(config.checkInterval()));
}
@Override
protected void doRefresh(LookupCachePurge cachePurge) throws Exception {
try {
final FileInfo.Change databaseFileCheck = fileInfo.checkForChange();
if (!databaseFileCheck.isChanged() && !getError().isPresent()) {
return;
}
// file has different attributes, let's reload it
LOG.debug("MaxMind database file has changed, reloading it from {}", config.path());
final IPLocationDatabaseAdapter oldAdapter = this.databaseAdapter.get();
try {
this.databaseAdapter.set(loadDatabaseAdapter(Paths.get(config.path()).toFile()));
cachePurge.purgeAll();
if (oldAdapter != null) {
oldAdapter.close();
}
fileInfo = databaseFileCheck.fileInfo();
clearError();
} catch (IOException e) {
LOG.warn("Unable to load changed database file, leaving old one intact. Error message: {}", e.getMessage());
setError(e);
}
} catch (IllegalArgumentException iae) {
LOG.error("Unable to refresh MaxMind database file: {}", iae.getMessage());
setError(iae);
}
}
private IPLocationDatabaseAdapter loadDatabaseAdapter(File file) throws IOException {
switch (config.dbType()) {
case MAXMIND_ASN:
case MAXMIND_CITY:
case MAXMIND_COUNTRY:
return new MaxMindIPLocationDatabaseAdapter(file);
case IPINFO_ASN:
case IPINFO_STANDARD_LOCATION:
return new IPinfoIPLocationDatabaseAdapter(file, disableIpInfoDbTypeCheck);
default:
throw new IllegalStateException("Unexpected value: " + config.dbType());
}
}
@Override
protected LookupResult doGet(Object key) {
final InetAddress addr;
if (key instanceof InetAddress) {
addr = (InetAddress) key;
} else {
// try to convert it somehow
try {
addr = InetAddresses.forString(key.toString());
} catch (IllegalArgumentException e) {
LOG.warn("Unable to parse IP address, returning empty result.");
return LookupResult.empty();
}
}
final IPLocationDatabaseAdapter reader = this.databaseAdapter.get();
switch (config.dbType()) {
case MAXMIND_CITY:
try {
final CityResponse city = reader.maxMindCity(addr);
if (city == null) {
LOG.debug("No city data for IP address {}, returning empty result.", addr);
return LookupResult.empty();
}
final Location location = city.getLocation();
final Map
© 2015 - 2024 Weber Informatics LLC | Privacy Policy