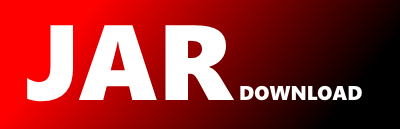
org.graylog.plugins.threatintel.AutoValue_ThreatIntelPluginConfiguration Maven / Gradle / Ivy
package org.graylog.plugins.threatintel;
import com.fasterxml.jackson.annotation.JsonProperty;
import javax.annotation.Nullable;
import javax.annotation.processing.Generated;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_ThreatIntelPluginConfiguration extends ThreatIntelPluginConfiguration {
private final boolean otxEnabled;
@Nullable
private final String otxApiKey;
private final boolean torEnabled;
private final boolean spamhausEnabled;
private final boolean abusechRansomEnabled;
private AutoValue_ThreatIntelPluginConfiguration(
boolean otxEnabled,
@Nullable String otxApiKey,
boolean torEnabled,
boolean spamhausEnabled,
boolean abusechRansomEnabled) {
this.otxEnabled = otxEnabled;
this.otxApiKey = otxApiKey;
this.torEnabled = torEnabled;
this.spamhausEnabled = spamhausEnabled;
this.abusechRansomEnabled = abusechRansomEnabled;
}
@JsonProperty("otx_enabled")
@Deprecated
@Override
public boolean otxEnabled() {
return otxEnabled;
}
@JsonProperty("otx_api_key")
@Deprecated
@Nullable
@Override
public String otxApiKey() {
return otxApiKey;
}
@JsonProperty("tor_enabled")
@Override
public boolean torEnabled() {
return torEnabled;
}
@JsonProperty("spamhaus_enabled")
@Override
public boolean spamhausEnabled() {
return spamhausEnabled;
}
@JsonProperty("abusech_ransom_enabled")
@Override
public boolean abusechRansomEnabled() {
return abusechRansomEnabled;
}
@Override
public String toString() {
return "ThreatIntelPluginConfiguration{"
+ "otxEnabled=" + otxEnabled + ", "
+ "otxApiKey=" + otxApiKey + ", "
+ "torEnabled=" + torEnabled + ", "
+ "spamhausEnabled=" + spamhausEnabled + ", "
+ "abusechRansomEnabled=" + abusechRansomEnabled
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof ThreatIntelPluginConfiguration) {
ThreatIntelPluginConfiguration that = (ThreatIntelPluginConfiguration) o;
return this.otxEnabled == that.otxEnabled()
&& (this.otxApiKey == null ? that.otxApiKey() == null : this.otxApiKey.equals(that.otxApiKey()))
&& this.torEnabled == that.torEnabled()
&& this.spamhausEnabled == that.spamhausEnabled()
&& this.abusechRansomEnabled == that.abusechRansomEnabled();
}
return false;
}
@Override
public int hashCode() {
int h$ = 1;
h$ *= 1000003;
h$ ^= otxEnabled ? 1231 : 1237;
h$ *= 1000003;
h$ ^= (otxApiKey == null) ? 0 : otxApiKey.hashCode();
h$ *= 1000003;
h$ ^= torEnabled ? 1231 : 1237;
h$ *= 1000003;
h$ ^= spamhausEnabled ? 1231 : 1237;
h$ *= 1000003;
h$ ^= abusechRansomEnabled ? 1231 : 1237;
return h$;
}
static final class Builder extends ThreatIntelPluginConfiguration.Builder {
private boolean otxEnabled;
private String otxApiKey;
private boolean torEnabled;
private boolean spamhausEnabled;
private boolean abusechRansomEnabled;
private byte set$0;
Builder() {
}
@Override
public ThreatIntelPluginConfiguration.Builder otxEnabled(boolean otxEnabled) {
this.otxEnabled = otxEnabled;
set$0 |= (byte) 1;
return this;
}
@Override
ThreatIntelPluginConfiguration.Builder otxApiKey(String otxApiKey) {
this.otxApiKey = otxApiKey;
return this;
}
@Override
public ThreatIntelPluginConfiguration.Builder torEnabled(boolean torEnabled) {
this.torEnabled = torEnabled;
set$0 |= (byte) 2;
return this;
}
@Override
public ThreatIntelPluginConfiguration.Builder spamhausEnabled(boolean spamhausEnabled) {
this.spamhausEnabled = spamhausEnabled;
set$0 |= (byte) 4;
return this;
}
@Override
public ThreatIntelPluginConfiguration.Builder abusechRansomEnabled(boolean abusechRansomEnabled) {
this.abusechRansomEnabled = abusechRansomEnabled;
set$0 |= (byte) 8;
return this;
}
@Override
public ThreatIntelPluginConfiguration build() {
if (set$0 != 0xf) {
StringBuilder missing = new StringBuilder();
if ((set$0 & 1) == 0) {
missing.append(" otxEnabled");
}
if ((set$0 & 2) == 0) {
missing.append(" torEnabled");
}
if ((set$0 & 4) == 0) {
missing.append(" spamhausEnabled");
}
if ((set$0 & 8) == 0) {
missing.append(" abusechRansomEnabled");
}
throw new IllegalStateException("Missing required properties:" + missing);
}
return new AutoValue_ThreatIntelPluginConfiguration(
this.otxEnabled,
this.otxApiKey,
this.torEnabled,
this.spamhausEnabled,
this.abusechRansomEnabled);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy