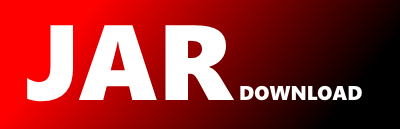
org.graylog.security.$AutoValue_GrantDTO Maven / Gradle / Ivy
package org.graylog.security;
import com.fasterxml.jackson.annotation.JsonProperty;
import java.time.ZonedDateTime;
import java.util.Optional;
import javax.annotation.Nullable;
import javax.annotation.processing.Generated;
import javax.validation.constraints.NotNull;
import org.graylog.grn.GRN;
import org.mongojack.Id;
import org.mongojack.ObjectId;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
abstract class $AutoValue_GrantDTO extends GrantDTO {
@Nullable
private final String id;
private final GRN grantee;
private final @NotNull Capability capability;
private final @NotNull GRN target;
private final String createdBy;
private final ZonedDateTime createdAt;
private final String updatedBy;
private final ZonedDateTime updatedAt;
private final Optional expiresAt;
$AutoValue_GrantDTO(
@Nullable String id,
GRN grantee,
@NotNull Capability capability,
@NotNull GRN target,
String createdBy,
ZonedDateTime createdAt,
String updatedBy,
ZonedDateTime updatedAt,
Optional expiresAt) {
this.id = id;
if (grantee == null) {
throw new NullPointerException("Null grantee");
}
this.grantee = grantee;
if (capability == null) {
throw new NullPointerException("Null capability");
}
this.capability = capability;
if (target == null) {
throw new NullPointerException("Null target");
}
this.target = target;
if (createdBy == null) {
throw new NullPointerException("Null createdBy");
}
this.createdBy = createdBy;
if (createdAt == null) {
throw new NullPointerException("Null createdAt");
}
this.createdAt = createdAt;
if (updatedBy == null) {
throw new NullPointerException("Null updatedBy");
}
this.updatedBy = updatedBy;
if (updatedAt == null) {
throw new NullPointerException("Null updatedAt");
}
this.updatedAt = updatedAt;
if (expiresAt == null) {
throw new NullPointerException("Null expiresAt");
}
this.expiresAt = expiresAt;
}
@JsonProperty("id")
@Nullable
@Id
@ObjectId
@Override
public String id() {
return id;
}
@JsonProperty("grantee")
@Override
public GRN grantee() {
return grantee;
}
@JsonProperty("capability")
@Override
public @NotNull Capability capability() {
return capability;
}
@JsonProperty("target")
@Override
public @NotNull GRN target() {
return target;
}
@JsonProperty("created_by")
@Override
public String createdBy() {
return createdBy;
}
@JsonProperty("created_at")
@Override
public ZonedDateTime createdAt() {
return createdAt;
}
@JsonProperty("updated_by")
@Override
public String updatedBy() {
return updatedBy;
}
@JsonProperty("updated_at")
@Override
public ZonedDateTime updatedAt() {
return updatedAt;
}
@JsonProperty("expires_at")
@Override
public Optional expiresAt() {
return expiresAt;
}
@Override
public String toString() {
return "GrantDTO{"
+ "id=" + id + ", "
+ "grantee=" + grantee + ", "
+ "capability=" + capability + ", "
+ "target=" + target + ", "
+ "createdBy=" + createdBy + ", "
+ "createdAt=" + createdAt + ", "
+ "updatedBy=" + updatedBy + ", "
+ "updatedAt=" + updatedAt + ", "
+ "expiresAt=" + expiresAt
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof GrantDTO) {
GrantDTO that = (GrantDTO) o;
return (this.id == null ? that.id() == null : this.id.equals(that.id()))
&& this.grantee.equals(that.grantee())
&& this.capability.equals(that.capability())
&& this.target.equals(that.target())
&& this.createdBy.equals(that.createdBy())
&& this.createdAt.equals(that.createdAt())
&& this.updatedBy.equals(that.updatedBy())
&& this.updatedAt.equals(that.updatedAt())
&& this.expiresAt.equals(that.expiresAt());
}
return false;
}
@Override
public int hashCode() {
int h$ = 1;
h$ *= 1000003;
h$ ^= (id == null) ? 0 : id.hashCode();
h$ *= 1000003;
h$ ^= grantee.hashCode();
h$ *= 1000003;
h$ ^= capability.hashCode();
h$ *= 1000003;
h$ ^= target.hashCode();
h$ *= 1000003;
h$ ^= createdBy.hashCode();
h$ *= 1000003;
h$ ^= createdAt.hashCode();
h$ *= 1000003;
h$ ^= updatedBy.hashCode();
h$ *= 1000003;
h$ ^= updatedAt.hashCode();
h$ *= 1000003;
h$ ^= expiresAt.hashCode();
return h$;
}
@Override
public GrantDTO.Builder toBuilder() {
return new Builder(this);
}
static class Builder extends GrantDTO.Builder {
private String id;
private GRN grantee;
private @NotNull Capability capability;
private @NotNull GRN target;
private String createdBy;
private ZonedDateTime createdAt;
private String updatedBy;
private ZonedDateTime updatedAt;
private Optional expiresAt = Optional.empty();
Builder() {
}
private Builder(GrantDTO source) {
this.id = source.id();
this.grantee = source.grantee();
this.capability = source.capability();
this.target = source.target();
this.createdBy = source.createdBy();
this.createdAt = source.createdAt();
this.updatedBy = source.updatedBy();
this.updatedAt = source.updatedAt();
this.expiresAt = source.expiresAt();
}
@Override
public GrantDTO.Builder id(@Nullable String id) {
this.id = id;
return this;
}
@Override
public GrantDTO.Builder grantee(GRN grantee) {
if (grantee == null) {
throw new NullPointerException("Null grantee");
}
this.grantee = grantee;
return this;
}
@Override
public GrantDTO.Builder capability(Capability capability) {
if (capability == null) {
throw new NullPointerException("Null capability");
}
this.capability = capability;
return this;
}
@Override
public GrantDTO.Builder target(GRN target) {
if (target == null) {
throw new NullPointerException("Null target");
}
this.target = target;
return this;
}
@Override
public GrantDTO.Builder createdBy(String createdBy) {
if (createdBy == null) {
throw new NullPointerException("Null createdBy");
}
this.createdBy = createdBy;
return this;
}
@Override
public GrantDTO.Builder createdAt(ZonedDateTime createdAt) {
if (createdAt == null) {
throw new NullPointerException("Null createdAt");
}
this.createdAt = createdAt;
return this;
}
@Override
public GrantDTO.Builder updatedBy(String updatedBy) {
if (updatedBy == null) {
throw new NullPointerException("Null updatedBy");
}
this.updatedBy = updatedBy;
return this;
}
@Override
public GrantDTO.Builder updatedAt(ZonedDateTime updatedAt) {
if (updatedAt == null) {
throw new NullPointerException("Null updatedAt");
}
this.updatedAt = updatedAt;
return this;
}
@Override
public GrantDTO.Builder expiresAt(@Nullable ZonedDateTime expiresAt) {
this.expiresAt = Optional.ofNullable(expiresAt);
return this;
}
@Override
public GrantDTO build() {
if (this.grantee == null
|| this.capability == null
|| this.target == null
|| this.createdBy == null
|| this.createdAt == null
|| this.updatedBy == null
|| this.updatedAt == null) {
StringBuilder missing = new StringBuilder();
if (this.grantee == null) {
missing.append(" grantee");
}
if (this.capability == null) {
missing.append(" capability");
}
if (this.target == null) {
missing.append(" target");
}
if (this.createdBy == null) {
missing.append(" createdBy");
}
if (this.createdAt == null) {
missing.append(" createdAt");
}
if (this.updatedBy == null) {
missing.append(" updatedBy");
}
if (this.updatedAt == null) {
missing.append(" updatedAt");
}
throw new IllegalStateException("Missing required properties:" + missing);
}
return new AutoValue_GrantDTO(
this.id,
this.grantee,
this.capability,
this.target,
this.createdBy,
this.createdAt,
this.updatedBy,
this.updatedAt,
this.expiresAt);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy