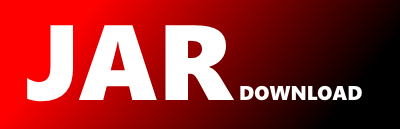
org.graylog.security.authservice.AutoValue_AuthServiceCredentials Maven / Gradle / Ivy
package org.graylog.security.authservice;
import javax.annotation.processing.Generated;
import org.graylog2.security.encryption.EncryptedValue;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_AuthServiceCredentials extends AuthServiceCredentials {
private final String username;
private final EncryptedValue password;
private final boolean isAuthenticated;
private AutoValue_AuthServiceCredentials(
String username,
EncryptedValue password,
boolean isAuthenticated) {
this.username = username;
this.password = password;
this.isAuthenticated = isAuthenticated;
}
@Override
public String username() {
return username;
}
@Override
public EncryptedValue password() {
return password;
}
@Override
public boolean isAuthenticated() {
return isAuthenticated;
}
@Override
public String toString() {
return "AuthServiceCredentials{"
+ "username=" + username + ", "
+ "password=" + password + ", "
+ "isAuthenticated=" + isAuthenticated
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof AuthServiceCredentials) {
AuthServiceCredentials that = (AuthServiceCredentials) o;
return this.username.equals(that.username())
&& this.password.equals(that.password())
&& this.isAuthenticated == that.isAuthenticated();
}
return false;
}
@Override
public int hashCode() {
int h$ = 1;
h$ *= 1000003;
h$ ^= username.hashCode();
h$ *= 1000003;
h$ ^= password.hashCode();
h$ *= 1000003;
h$ ^= isAuthenticated ? 1231 : 1237;
return h$;
}
static final class Builder extends AuthServiceCredentials.Builder {
private String username;
private EncryptedValue password;
private boolean isAuthenticated;
private byte set$0;
Builder() {
}
@Override
public AuthServiceCredentials.Builder username(String username) {
if (username == null) {
throw new NullPointerException("Null username");
}
this.username = username;
return this;
}
@Override
public AuthServiceCredentials.Builder password(EncryptedValue password) {
if (password == null) {
throw new NullPointerException("Null password");
}
this.password = password;
return this;
}
@Override
public AuthServiceCredentials.Builder isAuthenticated(boolean isAuthenticated) {
this.isAuthenticated = isAuthenticated;
set$0 |= (byte) 1;
return this;
}
@Override
public AuthServiceCredentials build() {
if (set$0 != 1
|| this.username == null
|| this.password == null) {
StringBuilder missing = new StringBuilder();
if (this.username == null) {
missing.append(" username");
}
if (this.password == null) {
missing.append(" password");
}
if ((set$0 & 1) == 0) {
missing.append(" isAuthenticated");
}
throw new IllegalStateException("Missing required properties:" + missing);
}
return new AutoValue_AuthServiceCredentials(
this.username,
this.password,
this.isAuthenticated);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy