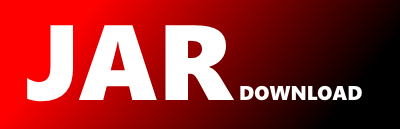
org.graylog.security.authservice.AutoValue_UserDetails Maven / Gradle / Ivy
package org.graylog.security.authservice;
import java.util.Optional;
import java.util.Set;
import javax.annotation.Nullable;
import javax.annotation.processing.Generated;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_UserDetails extends UserDetails {
private final Optional databaseId;
private final String authServiceType;
private final String authServiceId;
private final String base64AuthServiceUid;
private final String username;
private final boolean accountIsEnabled;
private final String email;
private final Optional firstName;
private final Optional lastName;
private final Optional fullName;
private final boolean isExternal;
private final Set defaultRoles;
private final Set groupsFromAuthN;
private AutoValue_UserDetails(
Optional databaseId,
String authServiceType,
String authServiceId,
String base64AuthServiceUid,
String username,
boolean accountIsEnabled,
String email,
Optional firstName,
Optional lastName,
Optional fullName,
boolean isExternal,
Set defaultRoles,
Set groupsFromAuthN) {
this.databaseId = databaseId;
this.authServiceType = authServiceType;
this.authServiceId = authServiceId;
this.base64AuthServiceUid = base64AuthServiceUid;
this.username = username;
this.accountIsEnabled = accountIsEnabled;
this.email = email;
this.firstName = firstName;
this.lastName = lastName;
this.fullName = fullName;
this.isExternal = isExternal;
this.defaultRoles = defaultRoles;
this.groupsFromAuthN = groupsFromAuthN;
}
@Override
public Optional databaseId() {
return databaseId;
}
@Override
public String authServiceType() {
return authServiceType;
}
@Override
public String authServiceId() {
return authServiceId;
}
@Override
public String base64AuthServiceUid() {
return base64AuthServiceUid;
}
@Override
public String username() {
return username;
}
@Override
public boolean accountIsEnabled() {
return accountIsEnabled;
}
@Override
public String email() {
return email;
}
@Override
public Optional firstName() {
return firstName;
}
@Override
public Optional lastName() {
return lastName;
}
@Override
public Optional fullName() {
return fullName;
}
@Override
public boolean isExternal() {
return isExternal;
}
@Override
public Set defaultRoles() {
return defaultRoles;
}
@Override
public Set groupsFromAuthN() {
return groupsFromAuthN;
}
@Override
public String toString() {
return "UserDetails{"
+ "databaseId=" + databaseId + ", "
+ "authServiceType=" + authServiceType + ", "
+ "authServiceId=" + authServiceId + ", "
+ "base64AuthServiceUid=" + base64AuthServiceUid + ", "
+ "username=" + username + ", "
+ "accountIsEnabled=" + accountIsEnabled + ", "
+ "email=" + email + ", "
+ "firstName=" + firstName + ", "
+ "lastName=" + lastName + ", "
+ "fullName=" + fullName + ", "
+ "isExternal=" + isExternal + ", "
+ "defaultRoles=" + defaultRoles + ", "
+ "groupsFromAuthN=" + groupsFromAuthN
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof UserDetails) {
UserDetails that = (UserDetails) o;
return this.databaseId.equals(that.databaseId())
&& this.authServiceType.equals(that.authServiceType())
&& this.authServiceId.equals(that.authServiceId())
&& this.base64AuthServiceUid.equals(that.base64AuthServiceUid())
&& this.username.equals(that.username())
&& this.accountIsEnabled == that.accountIsEnabled()
&& this.email.equals(that.email())
&& this.firstName.equals(that.firstName())
&& this.lastName.equals(that.lastName())
&& this.fullName.equals(that.fullName())
&& this.isExternal == that.isExternal()
&& this.defaultRoles.equals(that.defaultRoles())
&& this.groupsFromAuthN.equals(that.groupsFromAuthN());
}
return false;
}
@Override
public int hashCode() {
int h$ = 1;
h$ *= 1000003;
h$ ^= databaseId.hashCode();
h$ *= 1000003;
h$ ^= authServiceType.hashCode();
h$ *= 1000003;
h$ ^= authServiceId.hashCode();
h$ *= 1000003;
h$ ^= base64AuthServiceUid.hashCode();
h$ *= 1000003;
h$ ^= username.hashCode();
h$ *= 1000003;
h$ ^= accountIsEnabled ? 1231 : 1237;
h$ *= 1000003;
h$ ^= email.hashCode();
h$ *= 1000003;
h$ ^= firstName.hashCode();
h$ *= 1000003;
h$ ^= lastName.hashCode();
h$ *= 1000003;
h$ ^= fullName.hashCode();
h$ *= 1000003;
h$ ^= isExternal ? 1231 : 1237;
h$ *= 1000003;
h$ ^= defaultRoles.hashCode();
h$ *= 1000003;
h$ ^= groupsFromAuthN.hashCode();
return h$;
}
@Override
public UserDetails.Builder toBuilder() {
return new Builder(this);
}
static final class Builder extends UserDetails.Builder {
private Optional databaseId = Optional.empty();
private String authServiceType;
private String authServiceId;
private String base64AuthServiceUid;
private String username;
private boolean accountIsEnabled;
private String email;
private Optional firstName = Optional.empty();
private Optional lastName = Optional.empty();
private Optional fullName = Optional.empty();
private boolean isExternal;
private Set defaultRoles;
private Set groupsFromAuthN;
private byte set$0;
Builder() {
}
private Builder(UserDetails source) {
this.databaseId = source.databaseId();
this.authServiceType = source.authServiceType();
this.authServiceId = source.authServiceId();
this.base64AuthServiceUid = source.base64AuthServiceUid();
this.username = source.username();
this.accountIsEnabled = source.accountIsEnabled();
this.email = source.email();
this.firstName = source.firstName();
this.lastName = source.lastName();
this.fullName = source.fullName();
this.isExternal = source.isExternal();
this.defaultRoles = source.defaultRoles();
this.groupsFromAuthN = source.groupsFromAuthN();
set$0 = (byte) 3;
}
@Override
public UserDetails.Builder databaseId(@Nullable String databaseId) {
this.databaseId = Optional.ofNullable(databaseId);
return this;
}
@Override
public UserDetails.Builder authServiceType(String authServiceType) {
if (authServiceType == null) {
throw new NullPointerException("Null authServiceType");
}
this.authServiceType = authServiceType;
return this;
}
@Override
public UserDetails.Builder authServiceId(String authServiceId) {
if (authServiceId == null) {
throw new NullPointerException("Null authServiceId");
}
this.authServiceId = authServiceId;
return this;
}
@Override
public UserDetails.Builder base64AuthServiceUid(String base64AuthServiceUid) {
if (base64AuthServiceUid == null) {
throw new NullPointerException("Null base64AuthServiceUid");
}
this.base64AuthServiceUid = base64AuthServiceUid;
return this;
}
@Override
public UserDetails.Builder username(String username) {
if (username == null) {
throw new NullPointerException("Null username");
}
this.username = username;
return this;
}
@Override
public UserDetails.Builder accountIsEnabled(boolean accountIsEnabled) {
this.accountIsEnabled = accountIsEnabled;
set$0 |= (byte) 1;
return this;
}
@Override
public UserDetails.Builder email(String email) {
if (email == null) {
throw new NullPointerException("Null email");
}
this.email = email;
return this;
}
@Override
public UserDetails.Builder firstName(@Nullable String firstName) {
this.firstName = Optional.ofNullable(firstName);
return this;
}
@Override
public UserDetails.Builder lastName(@Nullable String lastName) {
this.lastName = Optional.ofNullable(lastName);
return this;
}
@Override
public UserDetails.Builder fullName(@Nullable String fullName) {
this.fullName = Optional.ofNullable(fullName);
return this;
}
@Override
public UserDetails.Builder isExternal(boolean isExternal) {
this.isExternal = isExternal;
set$0 |= (byte) 2;
return this;
}
@Override
public UserDetails.Builder defaultRoles(Set defaultRoles) {
if (defaultRoles == null) {
throw new NullPointerException("Null defaultRoles");
}
this.defaultRoles = defaultRoles;
return this;
}
@Override
public UserDetails.Builder groupsFromAuthN(Set groupsFromAuthN) {
if (groupsFromAuthN == null) {
throw new NullPointerException("Null groupsFromAuthN");
}
this.groupsFromAuthN = groupsFromAuthN;
return this;
}
@Override
UserDetails autoBuild() {
if (set$0 != 3
|| this.authServiceType == null
|| this.authServiceId == null
|| this.base64AuthServiceUid == null
|| this.username == null
|| this.email == null
|| this.defaultRoles == null
|| this.groupsFromAuthN == null) {
StringBuilder missing = new StringBuilder();
if (this.authServiceType == null) {
missing.append(" authServiceType");
}
if (this.authServiceId == null) {
missing.append(" authServiceId");
}
if (this.base64AuthServiceUid == null) {
missing.append(" base64AuthServiceUid");
}
if (this.username == null) {
missing.append(" username");
}
if ((set$0 & 1) == 0) {
missing.append(" accountIsEnabled");
}
if (this.email == null) {
missing.append(" email");
}
if ((set$0 & 2) == 0) {
missing.append(" isExternal");
}
if (this.defaultRoles == null) {
missing.append(" defaultRoles");
}
if (this.groupsFromAuthN == null) {
missing.append(" groupsFromAuthN");
}
throw new IllegalStateException("Missing required properties:" + missing);
}
return new AutoValue_UserDetails(
this.databaseId,
this.authServiceType,
this.authServiceId,
this.base64AuthServiceUid,
this.username,
this.accountIsEnabled,
this.email,
this.firstName,
this.lastName,
this.fullName,
this.isExternal,
this.defaultRoles,
this.groupsFromAuthN);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy