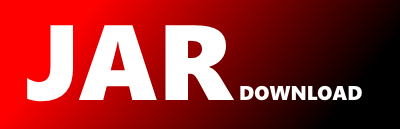
org.graylog.security.authservice.test.AutoValue_AuthServiceBackendTestRequest Maven / Gradle / Ivy
package org.graylog.security.authservice.test;
import com.fasterxml.jackson.annotation.JsonProperty;
import java.util.Optional;
import javax.annotation.Nullable;
import javax.annotation.processing.Generated;
import org.graylog.security.authservice.AuthServiceBackendDTO;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_AuthServiceBackendTestRequest extends AuthServiceBackendTestRequest {
private final Optional backendId;
private final AuthServiceBackendDTO backendConfiguration;
private final Optional userLogin;
private AutoValue_AuthServiceBackendTestRequest(
Optional backendId,
AuthServiceBackendDTO backendConfiguration,
Optional userLogin) {
this.backendId = backendId;
this.backendConfiguration = backendConfiguration;
this.userLogin = userLogin;
}
@JsonProperty("backend_id")
@Override
public Optional backendId() {
return backendId;
}
@JsonProperty("backend_configuration")
@Override
public AuthServiceBackendDTO backendConfiguration() {
return backendConfiguration;
}
@JsonProperty("user_login")
@Override
public Optional userLogin() {
return userLogin;
}
@Override
public String toString() {
return "AuthServiceBackendTestRequest{"
+ "backendId=" + backendId + ", "
+ "backendConfiguration=" + backendConfiguration + ", "
+ "userLogin=" + userLogin
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof AuthServiceBackendTestRequest) {
AuthServiceBackendTestRequest that = (AuthServiceBackendTestRequest) o;
return this.backendId.equals(that.backendId())
&& this.backendConfiguration.equals(that.backendConfiguration())
&& this.userLogin.equals(that.userLogin());
}
return false;
}
@Override
public int hashCode() {
int h$ = 1;
h$ *= 1000003;
h$ ^= backendId.hashCode();
h$ *= 1000003;
h$ ^= backendConfiguration.hashCode();
h$ *= 1000003;
h$ ^= userLogin.hashCode();
return h$;
}
static final class Builder extends AuthServiceBackendTestRequest.Builder {
private Optional backendId = Optional.empty();
private AuthServiceBackendDTO backendConfiguration;
private Optional userLogin = Optional.empty();
Builder() {
}
@Override
public AuthServiceBackendTestRequest.Builder backendId(@Nullable String backendId) {
this.backendId = Optional.ofNullable(backendId);
return this;
}
@Override
public AuthServiceBackendTestRequest.Builder backendConfiguration(AuthServiceBackendDTO backendConfiguration) {
if (backendConfiguration == null) {
throw new NullPointerException("Null backendConfiguration");
}
this.backendConfiguration = backendConfiguration;
return this;
}
@Override
public AuthServiceBackendTestRequest.Builder userLogin(@Nullable AuthServiceBackendTestRequest.UserLogin userLogin) {
this.userLogin = Optional.ofNullable(userLogin);
return this;
}
@Override
public AuthServiceBackendTestRequest build() {
if (this.backendConfiguration == null) {
String missing = " backendConfiguration";
throw new IllegalStateException("Missing required properties:" + missing);
}
return new AutoValue_AuthServiceBackendTestRequest(
this.backendId,
this.backendConfiguration,
this.userLogin);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy