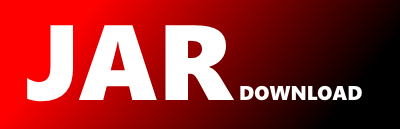
org.graylog2.commands.journal.JournalDecode Maven / Gradle / Ivy
/*
* Copyright (C) 2020 Graylog, Inc.
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the Server Side Public License, version 1,
* as published by MongoDB, Inc.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* Server Side Public License for more details.
*
* You should have received a copy of the Server Side Public License
* along with this program. If not, see
* .
*/
package org.graylog2.commands.journal;
import com.github.rvesse.airline.annotations.Arguments;
import com.github.rvesse.airline.annotations.Command;
import com.github.rvesse.airline.annotations.restrictions.Required;
import com.google.common.base.Splitter;
import com.google.common.collect.ImmutableList;
import com.google.common.collect.Range;
import com.google.inject.Key;
import com.google.inject.Module;
import com.google.inject.TypeLiteral;
import org.graylog2.featureflag.FeatureFlags;
import org.graylog2.inputs.codecs.CodecsModule;
import org.graylog2.plugin.Message;
import org.graylog2.plugin.ResolvableInetSocketAddress;
import org.graylog2.plugin.inject.Graylog2Module;
import org.graylog2.plugin.inputs.codecs.Codec;
import org.graylog2.plugin.journal.RawMessage;
import org.graylog2.shared.bindings.ObjectMapperModule;
import org.graylog2.shared.journal.Journal;
import org.slf4j.helpers.MessageFormatter;
import java.util.List;
import java.util.Map;
@Command(name = "decode", description = "Decodes messages from the journal")
public class JournalDecode extends AbstractJournalCommand {
@Arguments(description = "Range of message offsets to decode, e.g. single number 1234567, upper bound ..123456, lower bound 123456..., both 123456..123458")
@Required
private String rangeArg;
public JournalDecode() {
super("decode-journal");
}
@Override
protected List getCommandBindings(FeatureFlags featureFlags) {
return ImmutableList.builder()
.addAll(super.getCommandBindings(featureFlags))
.add(new CodecsModule())
.add(new ObjectMapperModule(getClass().getClassLoader()))
.add(new Graylog2Module() {
@Override
protected void configure() {
inputsMapBinder();
}
})
.build();
}
@Override
protected void runCommand() {
Range range;
try {
final List offsets = Splitter.on("..").limit(2).splitToList(rangeArg);
if (offsets.size() == 1) {
range = Range.singleton(Long.valueOf(offsets.get(0)));
} else if (offsets.size() == 2) {
final String first = offsets.get(0);
final String second = offsets.get(1);
if (first.isEmpty()) {
range = Range.atMost(Long.valueOf(second));
} else if (second.isEmpty()) {
range = Range.atLeast(Long.valueOf(first));
} else {
range = Range.closed(Long.valueOf(first), Long.valueOf(second));
}
} else {
throw new RuntimeException();
}
} catch (Exception e) {
System.err.println("Malformed offset range: " + rangeArg);
return;
}
final Map> codecFactory =
injector.getInstance(Key.get(new TypeLiteral
© 2015 - 2024 Weber Informatics LLC | Privacy Policy