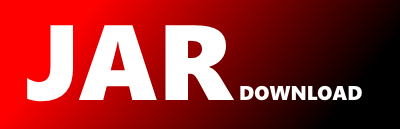
org.graylog2.contentpacks.model.AutoValue_ContentPackV1 Maven / Gradle / Ivy
package org.graylog2.contentpacks.model;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonView;
import com.github.zafarkhaja.semver.Version;
import com.google.common.collect.ImmutableSet;
import java.net.URI;
import javax.annotation.Nullable;
import javax.annotation.processing.Generated;
import org.bson.types.ObjectId;
import org.graylog2.contentpacks.model.entities.Entity;
import org.graylog2.contentpacks.model.parameters.Parameter;
import org.joda.time.DateTime;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_ContentPackV1 extends ContentPackV1 {
private final ModelId id;
private final int revision;
private final ModelVersion version;
@Nullable
private final ObjectId _id;
private final String name;
private final String summary;
private final String description;
private final String vendor;
private final URI url;
private final DateTime createdAt;
private final Version serverVersion;
private final ImmutableSet parameters;
private final ImmutableSet entities;
private AutoValue_ContentPackV1(
ModelId id,
int revision,
ModelVersion version,
@Nullable ObjectId _id,
String name,
String summary,
String description,
String vendor,
URI url,
DateTime createdAt,
Version serverVersion,
ImmutableSet parameters,
ImmutableSet entities) {
this.id = id;
this.revision = revision;
this.version = version;
this._id = _id;
this.name = name;
this.summary = summary;
this.description = description;
this.vendor = vendor;
this.url = url;
this.createdAt = createdAt;
this.serverVersion = serverVersion;
this.parameters = parameters;
this.entities = entities;
}
@JsonProperty("id")
@Override
public ModelId id() {
return id;
}
@JsonProperty("rev")
@Override
public int revision() {
return revision;
}
@JsonProperty("v")
@Override
public ModelVersion version() {
return version;
}
@JsonProperty("_id")
@JsonView(ContentPackView.DBView.class)
@Nullable
@Override
public ObjectId _id() {
return _id;
}
@JsonProperty("name")
@JsonView(ContentPackView.HttpView.class)
@Override
public String name() {
return name;
}
@JsonProperty("summary")
@JsonView(ContentPackView.HttpView.class)
@Override
public String summary() {
return summary;
}
@JsonProperty("description")
@JsonView(ContentPackView.HttpView.class)
@Override
public String description() {
return description;
}
@JsonProperty("vendor")
@JsonView(ContentPackView.HttpView.class)
@Override
public String vendor() {
return vendor;
}
@JsonProperty("url")
@JsonView(ContentPackView.HttpView.class)
@Override
public URI url() {
return url;
}
@JsonProperty("created_at")
@JsonView(ContentPackView.HttpView.class)
@Override
public DateTime createdAt() {
return createdAt;
}
@JsonProperty("server_version")
@JsonView(ContentPackView.HttpView.class)
@Override
public Version serverVersion() {
return serverVersion;
}
@JsonProperty("parameters")
@JsonView(ContentPackView.HttpView.class)
@Override
public ImmutableSet parameters() {
return parameters;
}
@JsonProperty("entities")
@JsonView(ContentPackView.HttpView.class)
@Override
public ImmutableSet entities() {
return entities;
}
@Override
public String toString() {
return "ContentPackV1{"
+ "id=" + id + ", "
+ "revision=" + revision + ", "
+ "version=" + version + ", "
+ "_id=" + _id + ", "
+ "name=" + name + ", "
+ "summary=" + summary + ", "
+ "description=" + description + ", "
+ "vendor=" + vendor + ", "
+ "url=" + url + ", "
+ "createdAt=" + createdAt + ", "
+ "serverVersion=" + serverVersion + ", "
+ "parameters=" + parameters + ", "
+ "entities=" + entities
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof ContentPackV1) {
ContentPackV1 that = (ContentPackV1) o;
return this.id.equals(that.id())
&& this.revision == that.revision()
&& this.version.equals(that.version())
&& (this._id == null ? that._id() == null : this._id.equals(that._id()))
&& this.name.equals(that.name())
&& this.summary.equals(that.summary())
&& this.description.equals(that.description())
&& this.vendor.equals(that.vendor())
&& this.url.equals(that.url())
&& this.createdAt.equals(that.createdAt())
&& this.serverVersion.equals(that.serverVersion())
&& this.parameters.equals(that.parameters())
&& this.entities.equals(that.entities());
}
return false;
}
@Override
public int hashCode() {
int h$ = 1;
h$ *= 1000003;
h$ ^= id.hashCode();
h$ *= 1000003;
h$ ^= revision;
h$ *= 1000003;
h$ ^= version.hashCode();
h$ *= 1000003;
h$ ^= (_id == null) ? 0 : _id.hashCode();
h$ *= 1000003;
h$ ^= name.hashCode();
h$ *= 1000003;
h$ ^= summary.hashCode();
h$ *= 1000003;
h$ ^= description.hashCode();
h$ *= 1000003;
h$ ^= vendor.hashCode();
h$ *= 1000003;
h$ ^= url.hashCode();
h$ *= 1000003;
h$ ^= createdAt.hashCode();
h$ *= 1000003;
h$ ^= serverVersion.hashCode();
h$ *= 1000003;
h$ ^= parameters.hashCode();
h$ *= 1000003;
h$ ^= entities.hashCode();
return h$;
}
static final class Builder extends ContentPackV1.Builder {
private ModelId id;
private int revision;
private ModelVersion version;
private ObjectId _id;
private String name;
private String summary;
private String description;
private String vendor;
private URI url;
private DateTime createdAt;
private Version serverVersion;
private ImmutableSet parameters;
private ImmutableSet entities;
private byte set$0;
Builder() {
}
@Override
public ContentPackV1.Builder id(ModelId id) {
if (id == null) {
throw new NullPointerException("Null id");
}
this.id = id;
return this;
}
@Override
public ModelId id() {
if (this.id == null) {
throw new IllegalStateException("Property \"id\" has not been set");
}
return id;
}
@Override
public ContentPackV1.Builder revision(int revision) {
this.revision = revision;
set$0 |= (byte) 1;
return this;
}
@Override
public ContentPackV1.Builder version(ModelVersion version) {
if (version == null) {
throw new NullPointerException("Null version");
}
this.version = version;
return this;
}
@Override
public ContentPackV1.Builder _id(ObjectId _id) {
this._id = _id;
return this;
}
@Override
public ContentPackV1.Builder name(String name) {
if (name == null) {
throw new NullPointerException("Null name");
}
this.name = name;
return this;
}
@Override
public ContentPackV1.Builder summary(String summary) {
if (summary == null) {
throw new NullPointerException("Null summary");
}
this.summary = summary;
return this;
}
@Override
public ContentPackV1.Builder description(String description) {
if (description == null) {
throw new NullPointerException("Null description");
}
this.description = description;
return this;
}
@Override
public ContentPackV1.Builder vendor(String vendor) {
if (vendor == null) {
throw new NullPointerException("Null vendor");
}
this.vendor = vendor;
return this;
}
@Override
public ContentPackV1.Builder url(URI url) {
if (url == null) {
throw new NullPointerException("Null url");
}
this.url = url;
return this;
}
@Override
public ContentPackV1.Builder createdAt(DateTime createdAt) {
if (createdAt == null) {
throw new NullPointerException("Null createdAt");
}
this.createdAt = createdAt;
return this;
}
@Override
public ContentPackV1.Builder serverVersion(Version serverVersion) {
if (serverVersion == null) {
throw new NullPointerException("Null serverVersion");
}
this.serverVersion = serverVersion;
return this;
}
@Override
public ContentPackV1.Builder parameters(ImmutableSet parameters) {
if (parameters == null) {
throw new NullPointerException("Null parameters");
}
this.parameters = parameters;
return this;
}
@Override
public ContentPackV1.Builder entities(ImmutableSet entities) {
if (entities == null) {
throw new NullPointerException("Null entities");
}
this.entities = entities;
return this;
}
@Override
ContentPackV1 autoBuild() {
if (set$0 != 1
|| this.id == null
|| this.version == null
|| this.name == null
|| this.summary == null
|| this.description == null
|| this.vendor == null
|| this.url == null
|| this.createdAt == null
|| this.serverVersion == null
|| this.parameters == null
|| this.entities == null) {
StringBuilder missing = new StringBuilder();
if (this.id == null) {
missing.append(" id");
}
if ((set$0 & 1) == 0) {
missing.append(" revision");
}
if (this.version == null) {
missing.append(" version");
}
if (this.name == null) {
missing.append(" name");
}
if (this.summary == null) {
missing.append(" summary");
}
if (this.description == null) {
missing.append(" description");
}
if (this.vendor == null) {
missing.append(" vendor");
}
if (this.url == null) {
missing.append(" url");
}
if (this.createdAt == null) {
missing.append(" createdAt");
}
if (this.serverVersion == null) {
missing.append(" serverVersion");
}
if (this.parameters == null) {
missing.append(" parameters");
}
if (this.entities == null) {
missing.append(" entities");
}
throw new IllegalStateException("Missing required properties:" + missing);
}
return new AutoValue_ContentPackV1(
this.id,
this.revision,
this.version,
this._id,
this.name,
this.summary,
this.description,
this.vendor,
this.url,
this.createdAt,
this.serverVersion,
this.parameters,
this.entities);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy