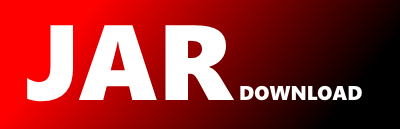
org.graylog2.contentpacks.model.entities.$AutoValue_LookupTableEntity Maven / Gradle / Ivy
package org.graylog2.contentpacks.model.entities;
import com.fasterxml.jackson.annotation.JsonProperty;
import javax.annotation.Nullable;
import javax.annotation.processing.Generated;
import org.graylog2.contentpacks.model.entities.references.ValueReference;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
abstract class $AutoValue_LookupTableEntity extends LookupTableEntity {
@Nullable
private final ValueReference scope;
private final ValueReference name;
private final ValueReference title;
private final ValueReference description;
private final ValueReference cacheName;
private final ValueReference dataAdapterName;
private final ValueReference defaultSingleValue;
private final ValueReference defaultSingleValueType;
private final ValueReference defaultMultiValue;
private final ValueReference defaultMultiValueType;
$AutoValue_LookupTableEntity(
@Nullable ValueReference scope,
ValueReference name,
ValueReference title,
ValueReference description,
ValueReference cacheName,
ValueReference dataAdapterName,
ValueReference defaultSingleValue,
ValueReference defaultSingleValueType,
ValueReference defaultMultiValue,
ValueReference defaultMultiValueType) {
this.scope = scope;
if (name == null) {
throw new NullPointerException("Null name");
}
this.name = name;
if (title == null) {
throw new NullPointerException("Null title");
}
this.title = title;
if (description == null) {
throw new NullPointerException("Null description");
}
this.description = description;
if (cacheName == null) {
throw new NullPointerException("Null cacheName");
}
this.cacheName = cacheName;
if (dataAdapterName == null) {
throw new NullPointerException("Null dataAdapterName");
}
this.dataAdapterName = dataAdapterName;
if (defaultSingleValue == null) {
throw new NullPointerException("Null defaultSingleValue");
}
this.defaultSingleValue = defaultSingleValue;
if (defaultSingleValueType == null) {
throw new NullPointerException("Null defaultSingleValueType");
}
this.defaultSingleValueType = defaultSingleValueType;
if (defaultMultiValue == null) {
throw new NullPointerException("Null defaultMultiValue");
}
this.defaultMultiValue = defaultMultiValue;
if (defaultMultiValueType == null) {
throw new NullPointerException("Null defaultMultiValueType");
}
this.defaultMultiValueType = defaultMultiValueType;
}
@JsonProperty("_scope")
@Nullable
@Override
public ValueReference scope() {
return scope;
}
@JsonProperty("name")
@Override
public ValueReference name() {
return name;
}
@JsonProperty("title")
@Override
public ValueReference title() {
return title;
}
@JsonProperty("description")
@Override
public ValueReference description() {
return description;
}
@JsonProperty("cache_name")
@Override
public ValueReference cacheName() {
return cacheName;
}
@JsonProperty("data_adapter_name")
@Override
public ValueReference dataAdapterName() {
return dataAdapterName;
}
@JsonProperty("default_single_value")
@Override
public ValueReference defaultSingleValue() {
return defaultSingleValue;
}
@JsonProperty("default_single_value_type")
@Override
public ValueReference defaultSingleValueType() {
return defaultSingleValueType;
}
@JsonProperty("default_multi_value")
@Override
public ValueReference defaultMultiValue() {
return defaultMultiValue;
}
@JsonProperty("default_multi_value_type")
@Override
public ValueReference defaultMultiValueType() {
return defaultMultiValueType;
}
@Override
public String toString() {
return "LookupTableEntity{"
+ "scope=" + scope + ", "
+ "name=" + name + ", "
+ "title=" + title + ", "
+ "description=" + description + ", "
+ "cacheName=" + cacheName + ", "
+ "dataAdapterName=" + dataAdapterName + ", "
+ "defaultSingleValue=" + defaultSingleValue + ", "
+ "defaultSingleValueType=" + defaultSingleValueType + ", "
+ "defaultMultiValue=" + defaultMultiValue + ", "
+ "defaultMultiValueType=" + defaultMultiValueType
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof LookupTableEntity) {
LookupTableEntity that = (LookupTableEntity) o;
return (this.scope == null ? that.scope() == null : this.scope.equals(that.scope()))
&& this.name.equals(that.name())
&& this.title.equals(that.title())
&& this.description.equals(that.description())
&& this.cacheName.equals(that.cacheName())
&& this.dataAdapterName.equals(that.dataAdapterName())
&& this.defaultSingleValue.equals(that.defaultSingleValue())
&& this.defaultSingleValueType.equals(that.defaultSingleValueType())
&& this.defaultMultiValue.equals(that.defaultMultiValue())
&& this.defaultMultiValueType.equals(that.defaultMultiValueType());
}
return false;
}
@Override
public int hashCode() {
int h$ = 1;
h$ *= 1000003;
h$ ^= (scope == null) ? 0 : scope.hashCode();
h$ *= 1000003;
h$ ^= name.hashCode();
h$ *= 1000003;
h$ ^= title.hashCode();
h$ *= 1000003;
h$ ^= description.hashCode();
h$ *= 1000003;
h$ ^= cacheName.hashCode();
h$ *= 1000003;
h$ ^= dataAdapterName.hashCode();
h$ *= 1000003;
h$ ^= defaultSingleValue.hashCode();
h$ *= 1000003;
h$ ^= defaultSingleValueType.hashCode();
h$ *= 1000003;
h$ ^= defaultMultiValue.hashCode();
h$ *= 1000003;
h$ ^= defaultMultiValueType.hashCode();
return h$;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy