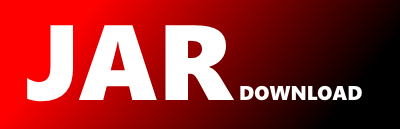
org.graylog2.contentpacks.model.entities.$AutoValue_ViewEntity Maven / Gradle / Ivy
package org.graylog2.contentpacks.model.entities;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.google.common.collect.ImmutableSet;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
import javax.annotation.processing.Generated;
import javax.validation.constraints.NotBlank;
import org.graylog.plugins.views.search.views.PluginMetadataSummary;
import org.graylog2.contentpacks.model.entities.references.ValueReference;
import org.joda.time.DateTime;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
abstract class $AutoValue_ViewEntity extends ViewEntity {
private final ViewEntity.Type type;
private final @NotBlank ValueReference title;
private final ValueReference summary;
private final ValueReference description;
private final SearchEntity search;
private final ImmutableSet properties;
private final Map requires;
private final Map state;
private final Optional owner;
private final DateTime createdAt;
$AutoValue_ViewEntity(
ViewEntity.Type type,
@NotBlank ValueReference title,
ValueReference summary,
ValueReference description,
SearchEntity search,
ImmutableSet properties,
Map requires,
Map state,
Optional owner,
DateTime createdAt) {
if (type == null) {
throw new NullPointerException("Null type");
}
this.type = type;
if (title == null) {
throw new NullPointerException("Null title");
}
this.title = title;
if (summary == null) {
throw new NullPointerException("Null summary");
}
this.summary = summary;
if (description == null) {
throw new NullPointerException("Null description");
}
this.description = description;
if (search == null) {
throw new NullPointerException("Null search");
}
this.search = search;
if (properties == null) {
throw new NullPointerException("Null properties");
}
this.properties = properties;
if (requires == null) {
throw new NullPointerException("Null requires");
}
this.requires = requires;
if (state == null) {
throw new NullPointerException("Null state");
}
this.state = state;
if (owner == null) {
throw new NullPointerException("Null owner");
}
this.owner = owner;
if (createdAt == null) {
throw new NullPointerException("Null createdAt");
}
this.createdAt = createdAt;
}
@JsonProperty("type")
@Override
public ViewEntity.Type type() {
return type;
}
@JsonProperty("title")
@Override
public @NotBlank ValueReference title() {
return title;
}
@JsonProperty("summary")
@Override
public ValueReference summary() {
return summary;
}
@JsonProperty("description")
@Override
public ValueReference description() {
return description;
}
@JsonProperty("search")
@Override
public SearchEntity search() {
return search;
}
@JsonProperty("properties")
@Override
public ImmutableSet properties() {
return properties;
}
@JsonProperty("requires")
@Override
public Map requires() {
return requires;
}
@JsonProperty("state")
@Override
public Map state() {
return state;
}
@JsonProperty("owner")
@Override
public Optional owner() {
return owner;
}
@JsonProperty("created_at")
@Override
public DateTime createdAt() {
return createdAt;
}
@Override
public String toString() {
return "ViewEntity{"
+ "type=" + type + ", "
+ "title=" + title + ", "
+ "summary=" + summary + ", "
+ "description=" + description + ", "
+ "search=" + search + ", "
+ "properties=" + properties + ", "
+ "requires=" + requires + ", "
+ "state=" + state + ", "
+ "owner=" + owner + ", "
+ "createdAt=" + createdAt
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof ViewEntity) {
ViewEntity that = (ViewEntity) o;
return this.type.equals(that.type())
&& this.title.equals(that.title())
&& this.summary.equals(that.summary())
&& this.description.equals(that.description())
&& this.search.equals(that.search())
&& this.properties.equals(that.properties())
&& this.requires.equals(that.requires())
&& this.state.equals(that.state())
&& this.owner.equals(that.owner())
&& this.createdAt.equals(that.createdAt());
}
return false;
}
@Override
public int hashCode() {
int h$ = 1;
h$ *= 1000003;
h$ ^= type.hashCode();
h$ *= 1000003;
h$ ^= title.hashCode();
h$ *= 1000003;
h$ ^= summary.hashCode();
h$ *= 1000003;
h$ ^= description.hashCode();
h$ *= 1000003;
h$ ^= search.hashCode();
h$ *= 1000003;
h$ ^= properties.hashCode();
h$ *= 1000003;
h$ ^= requires.hashCode();
h$ *= 1000003;
h$ ^= state.hashCode();
h$ *= 1000003;
h$ ^= owner.hashCode();
h$ *= 1000003;
h$ ^= createdAt.hashCode();
return h$;
}
@Override
public ViewEntity.Builder toBuilder() {
return new Builder(this);
}
static class Builder extends ViewEntity.Builder {
private ViewEntity.Type type;
private @NotBlank ValueReference title;
private ValueReference summary;
private ValueReference description;
private SearchEntity search;
private ImmutableSet.Builder propertiesBuilder$;
private ImmutableSet properties;
private Map requires;
private Map state;
private Optional owner = Optional.empty();
private DateTime createdAt;
Builder() {
}
private Builder(ViewEntity source) {
this.type = source.type();
this.title = source.title();
this.summary = source.summary();
this.description = source.description();
this.search = source.search();
this.properties = source.properties();
this.requires = source.requires();
this.state = source.state();
this.owner = source.owner();
this.createdAt = source.createdAt();
}
@Override
public ViewEntity.Builder type(ViewEntity.Type type) {
if (type == null) {
throw new NullPointerException("Null type");
}
this.type = type;
return this;
}
@Override
public ViewEntity.Builder title(ValueReference title) {
if (title == null) {
throw new NullPointerException("Null title");
}
this.title = title;
return this;
}
@Override
public ViewEntity.Builder summary(ValueReference summary) {
if (summary == null) {
throw new NullPointerException("Null summary");
}
this.summary = summary;
return this;
}
@Override
public ViewEntity.Builder description(ValueReference description) {
if (description == null) {
throw new NullPointerException("Null description");
}
this.description = description;
return this;
}
@Override
public ViewEntity.Builder search(SearchEntity search) {
if (search == null) {
throw new NullPointerException("Null search");
}
this.search = search;
return this;
}
@Override
ImmutableSet.Builder propertiesBuilder() {
if (propertiesBuilder$ == null) {
if (properties == null) {
propertiesBuilder$ = ImmutableSet.builder();
} else {
propertiesBuilder$ = ImmutableSet.builder();
propertiesBuilder$.addAll(properties);
properties = null;
}
}
return propertiesBuilder$;
}
@Override
public ViewEntity.Builder requires(Map requires) {
if (requires == null) {
throw new NullPointerException("Null requires");
}
this.requires = requires;
return this;
}
@Override
public ViewEntity.Builder state(Map state) {
if (state == null) {
throw new NullPointerException("Null state");
}
this.state = state;
return this;
}
@Override
public ViewEntity.Builder owner(@Nullable String owner) {
this.owner = Optional.ofNullable(owner);
return this;
}
@Override
public ViewEntity.Builder createdAt(DateTime createdAt) {
if (createdAt == null) {
throw new NullPointerException("Null createdAt");
}
this.createdAt = createdAt;
return this;
}
@Override
public ViewEntity build() {
if (propertiesBuilder$ != null) {
this.properties = propertiesBuilder$.build();
} else if (this.properties == null) {
this.properties = ImmutableSet.of();
}
if (this.type == null
|| this.title == null
|| this.summary == null
|| this.description == null
|| this.search == null
|| this.requires == null
|| this.state == null
|| this.createdAt == null) {
StringBuilder missing = new StringBuilder();
if (this.type == null) {
missing.append(" type");
}
if (this.title == null) {
missing.append(" title");
}
if (this.summary == null) {
missing.append(" summary");
}
if (this.description == null) {
missing.append(" description");
}
if (this.search == null) {
missing.append(" search");
}
if (this.requires == null) {
missing.append(" requires");
}
if (this.state == null) {
missing.append(" state");
}
if (this.createdAt == null) {
missing.append(" createdAt");
}
throw new IllegalStateException("Missing required properties:" + missing);
}
return new AutoValue_ViewEntity(
this.type,
this.title,
this.summary,
this.description,
this.search,
this.properties,
this.requires,
this.state,
this.owner,
this.createdAt);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy