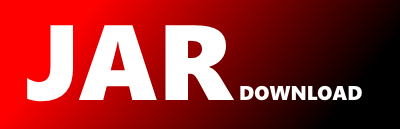
org.graylog2.contentpacks.model.entities.AutoValue_EntityExcerpt Maven / Gradle / Ivy
package org.graylog2.contentpacks.model.entities;
import com.fasterxml.jackson.annotation.JsonProperty;
import javax.annotation.Nullable;
import javax.annotation.processing.Generated;
import org.graylog2.contentpacks.model.ModelId;
import org.graylog2.contentpacks.model.ModelType;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_EntityExcerpt extends EntityExcerpt {
private final ModelId id;
private final ModelType type;
@Nullable
private final String title;
private AutoValue_EntityExcerpt(
ModelId id,
ModelType type,
@Nullable String title) {
this.id = id;
this.type = type;
this.title = title;
}
@JsonProperty("id")
@Override
public ModelId id() {
return id;
}
@JsonProperty("type")
@Override
public ModelType type() {
return type;
}
@JsonProperty("title")
@Nullable
@Override
public String title() {
return title;
}
@Override
public String toString() {
return "EntityExcerpt{"
+ "id=" + id + ", "
+ "type=" + type + ", "
+ "title=" + title
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof EntityExcerpt) {
EntityExcerpt that = (EntityExcerpt) o;
return this.id.equals(that.id())
&& this.type.equals(that.type())
&& (this.title == null ? that.title() == null : this.title.equals(that.title()));
}
return false;
}
@Override
public int hashCode() {
int h$ = 1;
h$ *= 1000003;
h$ ^= id.hashCode();
h$ *= 1000003;
h$ ^= type.hashCode();
h$ *= 1000003;
h$ ^= (title == null) ? 0 : title.hashCode();
return h$;
}
static final class Builder implements EntityExcerpt.Builder {
private ModelId id;
private ModelType type;
private String title;
Builder() {
}
@Override
public EntityExcerpt.Builder id(ModelId id) {
if (id == null) {
throw new NullPointerException("Null id");
}
this.id = id;
return this;
}
@Override
public ModelId id() {
if (this.id == null) {
throw new IllegalStateException("Property \"id\" has not been set");
}
return id;
}
@Override
public EntityExcerpt.Builder type(ModelType type) {
if (type == null) {
throw new NullPointerException("Null type");
}
this.type = type;
return this;
}
@Override
public EntityExcerpt.Builder title(String title) {
this.title = title;
return this;
}
@Override
public EntityExcerpt build() {
if (this.id == null
|| this.type == null) {
StringBuilder missing = new StringBuilder();
if (this.id == null) {
missing.append(" id");
}
if (this.type == null) {
missing.append(" type");
}
throw new IllegalStateException("Missing required properties:" + missing);
}
return new AutoValue_EntityExcerpt(
this.id,
this.type,
this.title);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy