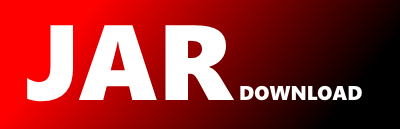
org.graylog2.contentpacks.model.entities.AutoValue_PivotEntity Maven / Gradle / Ivy
package org.graylog2.contentpacks.model.entities;
import com.fasterxml.jackson.annotation.JsonProperty;
import java.util.List;
import java.util.Optional;
import java.util.Set;
import javax.annotation.Nullable;
import javax.annotation.processing.Generated;
import org.graylog.plugins.views.search.Filter;
import org.graylog.plugins.views.search.engine.BackendQuery;
import org.graylog.plugins.views.search.searchfilters.model.UsedSearchFilter;
import org.graylog.plugins.views.search.searchtypes.pivot.BucketSpec;
import org.graylog.plugins.views.search.searchtypes.pivot.SeriesSpec;
import org.graylog.plugins.views.search.searchtypes.pivot.SortSpec;
import org.graylog.plugins.views.search.timeranges.DerivedTimeRange;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_PivotEntity extends PivotEntity {
private final Optional timerange;
private final Optional query;
private final Set streams;
private final String type;
@Nullable
private final String id;
private final Optional name;
private final List rowGroups;
private final List columnGroups;
private final List series;
private final List sort;
private final boolean rollup;
@Nullable
private final Filter filter;
private final List filters;
private final Optional rowLimit;
private final Optional columnLimit;
private AutoValue_PivotEntity(
Optional timerange,
Optional query,
Set streams,
String type,
@Nullable String id,
Optional name,
List rowGroups,
List columnGroups,
List series,
List sort,
boolean rollup,
@Nullable Filter filter,
List filters,
Optional rowLimit,
Optional columnLimit) {
this.timerange = timerange;
this.query = query;
this.streams = streams;
this.type = type;
this.id = id;
this.name = name;
this.rowGroups = rowGroups;
this.columnGroups = columnGroups;
this.series = series;
this.sort = sort;
this.rollup = rollup;
this.filter = filter;
this.filters = filters;
this.rowLimit = rowLimit;
this.columnLimit = columnLimit;
}
@JsonProperty
@Override
public Optional timerange() {
return timerange;
}
@JsonProperty
@Override
public Optional query() {
return query;
}
@JsonProperty
@Override
public Set streams() {
return streams;
}
@Override
public String type() {
return type;
}
@JsonProperty
@Nullable
@Override
public String id() {
return id;
}
@JsonProperty
@Override
public Optional name() {
return name;
}
@JsonProperty("row_groups")
@Override
public List rowGroups() {
return rowGroups;
}
@JsonProperty("column_groups")
@Override
public List columnGroups() {
return columnGroups;
}
@JsonProperty
@Override
public List series() {
return series;
}
@JsonProperty
@Override
public List sort() {
return sort;
}
@JsonProperty
@Override
public boolean rollup() {
return rollup;
}
@Nullable
@Override
public Filter filter() {
return filter;
}
@JsonProperty("filters")
@Override
public List filters() {
return filters;
}
@JsonProperty("row_limit")
@Override
public Optional rowLimit() {
return rowLimit;
}
@JsonProperty("column_limit")
@Override
public Optional columnLimit() {
return columnLimit;
}
@Override
public String toString() {
return "PivotEntity{"
+ "timerange=" + timerange + ", "
+ "query=" + query + ", "
+ "streams=" + streams + ", "
+ "type=" + type + ", "
+ "id=" + id + ", "
+ "name=" + name + ", "
+ "rowGroups=" + rowGroups + ", "
+ "columnGroups=" + columnGroups + ", "
+ "series=" + series + ", "
+ "sort=" + sort + ", "
+ "rollup=" + rollup + ", "
+ "filter=" + filter + ", "
+ "filters=" + filters + ", "
+ "rowLimit=" + rowLimit + ", "
+ "columnLimit=" + columnLimit
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof PivotEntity) {
PivotEntity that = (PivotEntity) o;
return this.timerange.equals(that.timerange())
&& this.query.equals(that.query())
&& this.streams.equals(that.streams())
&& this.type.equals(that.type())
&& (this.id == null ? that.id() == null : this.id.equals(that.id()))
&& this.name.equals(that.name())
&& this.rowGroups.equals(that.rowGroups())
&& this.columnGroups.equals(that.columnGroups())
&& this.series.equals(that.series())
&& this.sort.equals(that.sort())
&& this.rollup == that.rollup()
&& (this.filter == null ? that.filter() == null : this.filter.equals(that.filter()))
&& this.filters.equals(that.filters())
&& this.rowLimit.equals(that.rowLimit())
&& this.columnLimit.equals(that.columnLimit());
}
return false;
}
@Override
public int hashCode() {
int h$ = 1;
h$ *= 1000003;
h$ ^= timerange.hashCode();
h$ *= 1000003;
h$ ^= query.hashCode();
h$ *= 1000003;
h$ ^= streams.hashCode();
h$ *= 1000003;
h$ ^= type.hashCode();
h$ *= 1000003;
h$ ^= (id == null) ? 0 : id.hashCode();
h$ *= 1000003;
h$ ^= name.hashCode();
h$ *= 1000003;
h$ ^= rowGroups.hashCode();
h$ *= 1000003;
h$ ^= columnGroups.hashCode();
h$ *= 1000003;
h$ ^= series.hashCode();
h$ *= 1000003;
h$ ^= sort.hashCode();
h$ *= 1000003;
h$ ^= rollup ? 1231 : 1237;
h$ *= 1000003;
h$ ^= (filter == null) ? 0 : filter.hashCode();
h$ *= 1000003;
h$ ^= filters.hashCode();
h$ *= 1000003;
h$ ^= rowLimit.hashCode();
h$ *= 1000003;
h$ ^= columnLimit.hashCode();
return h$;
}
@Override
public PivotEntity.Builder toBuilder() {
return new Builder(this);
}
static final class Builder extends PivotEntity.Builder {
private Optional timerange = Optional.empty();
private Optional query = Optional.empty();
private Set streams;
private String type;
private String id;
private Optional name = Optional.empty();
private List rowGroups;
private List columnGroups;
private List series;
private List sort;
private boolean rollup;
private Filter filter;
private List filters;
private Optional rowLimit = Optional.empty();
private Optional columnLimit = Optional.empty();
private byte set$0;
Builder() {
}
private Builder(PivotEntity source) {
this.timerange = source.timerange();
this.query = source.query();
this.streams = source.streams();
this.type = source.type();
this.id = source.id();
this.name = source.name();
this.rowGroups = source.rowGroups();
this.columnGroups = source.columnGroups();
this.series = source.series();
this.sort = source.sort();
this.rollup = source.rollup();
this.filter = source.filter();
this.filters = source.filters();
this.rowLimit = source.rowLimit();
this.columnLimit = source.columnLimit();
set$0 = (byte) 1;
}
@Override
public PivotEntity.Builder timerange(@Nullable DerivedTimeRange timerange) {
this.timerange = Optional.ofNullable(timerange);
return this;
}
@Override
public PivotEntity.Builder query(@Nullable BackendQuery query) {
this.query = Optional.ofNullable(query);
return this;
}
@Override
public PivotEntity.Builder streams(Set streams) {
if (streams == null) {
throw new NullPointerException("Null streams");
}
this.streams = streams;
return this;
}
@Override
public PivotEntity.Builder type(String type) {
if (type == null) {
throw new NullPointerException("Null type");
}
this.type = type;
return this;
}
@Override
public PivotEntity.Builder id(@Nullable String id) {
this.id = id;
return this;
}
@Override
public PivotEntity.Builder name(@Nullable String name) {
this.name = Optional.ofNullable(name);
return this;
}
@Override
public PivotEntity.Builder rowGroups(List rowGroups) {
if (rowGroups == null) {
throw new NullPointerException("Null rowGroups");
}
this.rowGroups = rowGroups;
return this;
}
@Override
public PivotEntity.Builder columnGroups(List columnGroups) {
if (columnGroups == null) {
throw new NullPointerException("Null columnGroups");
}
this.columnGroups = columnGroups;
return this;
}
@Override
public PivotEntity.Builder series(List series) {
if (series == null) {
throw new NullPointerException("Null series");
}
this.series = series;
return this;
}
@Override
public PivotEntity.Builder sort(List sort) {
if (sort == null) {
throw new NullPointerException("Null sort");
}
this.sort = sort;
return this;
}
@Override
public PivotEntity.Builder rollup(boolean rollup) {
this.rollup = rollup;
set$0 |= (byte) 1;
return this;
}
@Override
public PivotEntity.Builder filter(@Nullable Filter filter) {
this.filter = filter;
return this;
}
@Override
public PivotEntity.Builder filters(List filters) {
if (filters == null) {
throw new NullPointerException("Null filters");
}
this.filters = filters;
return this;
}
@Override
public PivotEntity.Builder rowLimit(@Nullable Integer rowLimit) {
this.rowLimit = Optional.ofNullable(rowLimit);
return this;
}
@Override
public PivotEntity.Builder columnLimit(@Nullable Integer columnLimit) {
this.columnLimit = Optional.ofNullable(columnLimit);
return this;
}
@Override
public PivotEntity build() {
if (set$0 != 1
|| this.streams == null
|| this.type == null
|| this.rowGroups == null
|| this.columnGroups == null
|| this.series == null
|| this.sort == null
|| this.filters == null) {
StringBuilder missing = new StringBuilder();
if (this.streams == null) {
missing.append(" streams");
}
if (this.type == null) {
missing.append(" type");
}
if (this.rowGroups == null) {
missing.append(" rowGroups");
}
if (this.columnGroups == null) {
missing.append(" columnGroups");
}
if (this.series == null) {
missing.append(" series");
}
if (this.sort == null) {
missing.append(" sort");
}
if ((set$0 & 1) == 0) {
missing.append(" rollup");
}
if (this.filters == null) {
missing.append(" filters");
}
throw new IllegalStateException("Missing required properties:" + missing);
}
return new AutoValue_PivotEntity(
this.timerange,
this.query,
this.streams,
this.type,
this.id,
this.name,
this.rowGroups,
this.columnGroups,
this.series,
this.sort,
this.rollup,
this.filter,
this.filters,
this.rowLimit,
this.columnLimit);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy